#include "ServerSocket.h"
#include <iostream>
using namespace std;
#include <sys/socket.h>
#include <sys/types.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <sys/epoll.h>
#include <assert.h>
#include <string.h>
const int maxEvents = 100;
ServerSocket::ServerSocket(char *ip, short port)
{
listenFd = socket(AF_INET, SOCK_STREAM, 0);
assert(-1 != listenFd);
struct sockaddr_in ser;
memset(&ser, 0, sizeof(ser));
ser.sin_family = AF_INET;
ser.sin_port = htons(port);
ser.sin_addr.s_addr = inet_addr(ip);
int res = bind(listenFd, (struct sockaddr*)&ser, sizeof(ser));
assert(-1 != res);
listen(listenFd, 5);
epollFd = epoll_create(5);
assert(-1 != epollFd);
struct epoll_event event;
event.events = EPOLLIN;
event.data.fd = listenFd;
epoll_ctl(epollFd, EPOLL_CTL_ADD, listenFd, &event);
}
ServerSocket::~ServerSocket()
{
close(listenFd);
close(epollFd);
}
void ServerSocket::Start()
{
while(1)
{
struct epoll_event orderEvents[maxEvents];
int n = epoll_wait(epollFd, orderEvents, maxEvents, -1);
int i = 0;
for(; i < n; ++i)
{
int fd = orderEvents[i].data.fd;
if(fd == listenFd)
{
AcceptClientLink();
}
else
{
if(orderEvents[i].events & EPOLLRDHUP)
{
close(fd);
epoll_ctl(epollFd, EPOLL_CTL_DEL, fd, NULL);
}
else if(orderEvents[i].events & EPOLLIN)
{
RecvData(fd);
}
else
{
cout<<"event is error"<<endl;
}
}
}
}
}
void ServerSocket::AcceptClientLink()
{
struct sockaddr_in cli;
socklen_t len = (socklen_t)sizeof(cli);
int c = accept(listenFd, (struct sockaddr*)&cli, &len);
if(-1 == c)
{
cout<<"one client link error"<<endl;
return;
}
struct epoll_event event;
event.events = EPOLLIN | EPOLLRDHUP;
event.data.fd = c;
epoll_ctl(epollFd, EPOLL_CTL_ADD, c, &event);
}
void ServerSocket::RecvData(int fd)
{
Data data; // type command
memset(&data, 0, sizeof(data));
int n = recv(fd, &data, sizeof(data), 0);
if(n <= 0)
{
return;
}
switch(data.type)
{
case 1:
CarryOutCmd(fd, data.command);
break;
case 2:
RecvFile(fd, data.command);
break;
case 3:
SendFile(fd, data.command);
break;
default:
send(fd, "type error", 10, 0);
break;
}
}
void ServerSocket::CmdCd(char *path)
{
chdir(path);
}
// 服务器执行系统命令,将执行结果发送给客户端
void ServerSocket::CarryOutCmd(int fd, char *cmd)
{
char *argv[128] = {0};
char *p = strtok(cmd, " ");
int count = 0;
while(p != NULL)
{
argv[count++] = p;
p = strtok(NULL, " ");
}
if(strncmp(argv[0], "cd", 2) == 0)
{
CmdCd(argv[1]);
char path[128] = {0};
getcwd(path, 127);
send(fd, path, strlen(path), 0);
return;
}
int fds[2] = {-1,-1};
pipe(fds);
pid_t pid = fork();
assert(-1 != pid);
if(pid == 0)
{
close(1);
close(2);
dup(fds[1]);
dup(fds[1]);
char path[128] = "/bin/";
strcat(path, argv[0]);
execv(path, argv);
}
else
{
close(fds[1]);
int flag = 0;
while(1)
{
char buff[128] = {0};
int n = read(fds[0], buff, 127);
if(n <= 0)
{
break;
}
flag = 1;
send(fd, buff, n, 0);
}
if(!flag)
send(fd, "success", strlen("success"), 0);
close(fds[0]);
}
return;
}

童小纯
- 粉丝: 3w+
- 资源: 289
最新资源
- 基于Java spring boot冷链溯源管理系统文档+源码+全部资料+高分项目.zip
- 基于HyperledgerFabric实现的牛奶溯源项目文档+源码+全部资料+高分项目.zip
- 基于frp-0.58.1魔改二开,随机化socks5账户密码及端口、钉钉上线下线通知、配置文件oss加密读取、域前置防止溯源、源码替换编译混淆等文档+源码+全部资料+高分项目.zip
- 基于nem链的区块链汽车零配件溯源项目文档+源码+全部资料+高分项目.zip
- 基于SpringBoot框架的、基于国密算法与群签名的可溯源区块链模拟系统文档+源码+全部资料+高分项目.zip
- 基于lumen开发的微信小程序农场生态管理API设计,文档+源码+全部资料+高分项目.zip
- 基于vue2.0+vuex+vue-router+element-ui开发的农产品溯源系统后台文档+源码+全部资料+高分项目.zip
- 基于超级账本的简易牛奶溯源系统的区块链子系统实现文档+源码+全部资料+高分项目.zip
- 基于开发者平台的供应链溯源案例(网易云课程)文档+源码+全部资料+高分项目.zip
- 基于农产品溯源 基于fisco-bcos实现 分管理和商城两端文档+源码+全部资料+高分项目.zip
- 基于可视水印检测识别的数字媒体溯源应用系统,文档+源码+全部资料+高分项目.zip
- 基于区块链的供应链金融溯源系统文档+源码+全部资料+高分项目.zip
- 基于区块链(fabric)农产品溯源平台文档+源码+全部资料+高分项目.zip
- 基于区块链Hyperledger Fabric V2.5的农产品溯源商品通用溯源应用模板,部署简单,附压测工具、区块链浏览器,文档+源码+全部资料+高分项目.z
- 基于区块链的商品溯源系统文档+源码+全部资料+高分项目.zip
- 基于区块链的食品溯源系统文档+源码+全部资料+高分项目.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


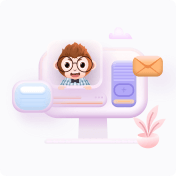