# Bootstrap Tree View
---

[](https://www.npmjs.com/package/bootstrap-treeview)
[](https://travis-ci.org/jonmiles/bootstrap-treeview)
A simple and elegant solution to displaying hierarchical tree structures (i.e. a Tree View) while leveraging the best that Twitter Bootstrap has to offer.
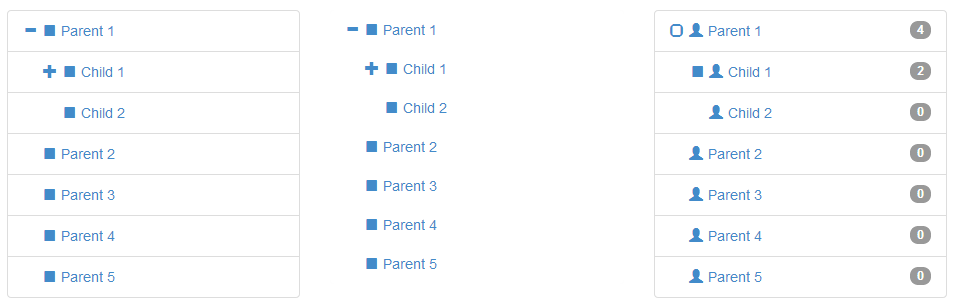
## Dependencies
Where provided these are the actual versions bootstrap-treeview has been tested against.
- [Bootstrap v3.3.4 (>= 3.0.0)](http://getbootstrap.com/)
- [jQuery v2.1.3 (>= 1.9.0)](http://jquery.com/)
## Getting Started
### Install
You can install using bower (recommended):
```javascript
$ bower install bootstrap-treeview
```
or using npm:
```javascript
$ npm install bootstrap-treeview
```
or [download](https://github.com/jonmiles/bootstrap-treeview/releases/tag/v1.2.0) manually.
### Usage
Add the following resources for the bootstrap-treeview to function correctly.
```html
<!-- Required Stylesheets -->
<link href="bootstrap.css" rel="stylesheet">
<!-- Required Javascript -->
<script src="jquery.js"></script>
<script src="bootstrap-treeview.js"></script>
```
The component will bind to any existing DOM element.
```html
<div id="tree"></div>
```
Basic usage may look something like this.
```javascript
function getTree() {
// Some logic to retrieve, or generate tree structure
return data;
}
$('#tree').treeview({data: getTree()});
```
## Data Structure
In order to define the hierarchical structure needed for the tree it's necessary to provide a nested array of JavaScript objects.
Example
```javascript
var tree = [
{
text: "Parent 1",
nodes: [
{
text: "Child 1",
nodes: [
{
text: "Grandchild 1"
},
{
text: "Grandchild 2"
}
]
},
{
text: "Child 2"
}
]
},
{
text: "Parent 2"
},
{
text: "Parent 3"
},
{
text: "Parent 4"
},
{
text: "Parent 5"
}
];
```
At the lowest level a tree node is a represented as a simple JavaScript object. This one required property `text` will build you a tree.
```javascript
{
text: "Node 1"
}
```
If you want to do more, here's the full node specification
```javascript
{
text: "Node 1",
icon: "glyphicon glyphicon-stop",
selectedIcon: "glyphicon glyphicon-stop",
color: "#000000",
backColor: "#FFFFFF",
href: "#node-1",
selectable: true,
state: {
checked: true,
disabled: true,
expanded: true,
selected: true
},
tags: ['available'],
nodes: [
{},
...
]
}
```
### Node Properties
The following properties are defined to allow node level overrides, such as node specific icons, colours and tags.
#### text
`String` `Mandatory`
The text value displayed for a given tree node, typically to the right of the nodes icon.
#### icon
`String` `Optional`
The icon displayed on a given node, typically to the left of the text.
For simplicity we directly leverage [Bootstraps Glyphicons support](http://getbootstrap.com/components/#glyphicons) and as such you should provide both the base class and individual icon class separated by a space.
By providing the base class you retain full control over the icons used. If you want to use your own then just add your class to this icon field.
#### selectedIcon
`String` `Optional`
The icon displayed on a given node when selected, typically to the left of the text.
#### color
`String` `Optional`
The foreground color used on a given node, overrides global color option.
#### backColor
`String` `Optional`
The background color used on a given node, overrides global color option.
#### href
`String` `Optional`
Used in conjunction with global enableLinks option to specify anchor tag URL on a given node.
#### selectable
`Boolean` `Default: true`
Whether or not a node is selectable in the tree. False indicates the node should act as an expansion heading and will not fire selection events.
#### state
`Object` `Optional`
Describes a node's initial state.
#### state.checked
`Boolean` `Default: false`
Whether or not a node is checked, represented by a checkbox style glyphicon.
#### state.disabled
`Boolean` `Default: false`
Whether or not a node is disabled (not selectable, expandable or checkable).
#### state.expanded
`Boolean` `Default: false`
Whether or not a node is expanded i.e. open. Takes precedence over global option levels.
#### state.selected
`Boolean` `Default: false`
Whether or not a node is selected.
#### tags
`Array of Strings` `Optional`
Used in conjunction with global showTags option to add additional information to the right of each node; using [Bootstrap Badges](http://getbootstrap.com/components/#badges)
### Extendible
You can extend the node object by adding any number of additional key value pairs that you require for your application. Remember this is the object which will be passed around during selection events.
## Options
Options allow you to customise the treeview's default appearance and behaviour. They are passed to the plugin on initialization, as an object.
```javascript
// Example: initializing the treeview
// expanded to 5 levels
// with a background color of green
$('#tree').treeview({
data: data, // data is not optional
levels: 5,
backColor: 'green'
});
```
You can pass a new options object to the treeview at any time but this will have the effect of re-initializing the treeview.
### List of Options
The following is a list of all available options.
#### data
Array of Objects. No default, expects data
This is the core data to be displayed by the tree view.
#### backColor
String, [any legal color value](http://www.w3schools.com/cssref/css_colors_legal.asp). Default: inherits from Bootstrap.css.
Sets the default background color used by all nodes, except when overridden on a per node basis in data.
#### borderColor
String, [any legal color value](http://www.w3schools.com/cssref/css_colors_legal.asp). Default: inherits from Bootstrap.css.
Sets the border color for the component; set showBorder to false if you don't want a visible border.
#### checkedIcon
String, class names(s). Default: "glyphicon glyphicon-check" as defined by [Bootstrap Glyphicons](http://getbootstrap.com/components/#glyphicons)
Sets the icon to be as a checked checkbox, used in conjunction with showCheckbox.
#### collapseIcon
String, class name(s). Default: "glyphicon glyphicon-minus" as defined by [Bootstrap Glyphicons](http://getbootstrap.com/components/#glyphicons)
Sets the icon to be used on a collapsible tree node.
#### color
String, [any legal color value](http://www.w3schools.com/cssref/css_colors_legal.asp). Default: inherits from Bootstrap.css.
Sets the default foreground color used by all nodes, except when overridden on a per node basis in data.
#### emptyIcon
String, class name(s). Default: "glyphicon" as defined by [Bootstrap Glyphicons](http://getbootstrap.com/components/#glyphicons)
Sets the icon to be used on a tree node with no child nodes.
#### enableLinks
Boolean. Default: false
Whether or not to present node text as a hyperlink. The href value of which must be provided in the data structure on a per node basis.
#### expandIcon
String, class name(s). Default: "glyphicon glyphicon-plus" as defined by [Bootstrap Glyphicons](http://getbootstrap.com/components/#glyphicons)
Sets the icon to be used on an expandable tree node.
#### highlightSearchResults
Boolean. Default: true
Whether or not to highlight search results.
#### highlightSelected
Boolean. Default: true
Whether or not to highlight the selected node.
#### levels
Int
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Spring Boot和Vue.js是两个流行的开发框架,结合使用可以实现一个功能强大的商城系统。下面是关于Spring Boot和Vue.js结合开发商城的简述: 后端开发(Spring Boot): 使用Spring Boot构建后端服务,提供商城系统所需的接口和逻辑处理。 设计数据库模型,创建相应的实体类和数据访问层(DAO)。 实现业务逻辑,包括商品管理、用户管理、订单管理等。 集成第三方支付接口和物流接口,以支持在线支付和订单配送功能。 前端开发(Vue.js): 使用Vue.js构建前端界面,实现用户交互和页面展示。 利用Vue Router进行路由管理,实现不同页面之间的跳转和导航。 使用Vuex进行状态管理,共享数据和状态,确保数据的一致性。 结合Element UI或其他UI库,设计并实现美观、响应式的用户界面。 利用Axios或其他HTTP库与后端接口进行数据交互。 前后端通信: 通过RESTful API进行前后端数据交互,使用JSON格式进行数据传输。 前端发送请求到后端接口,获取数据并进行展示或修改。 后端接收前端请求,进行数据处理和业务逻辑操作,并返
资源推荐
资源详情
资源评论
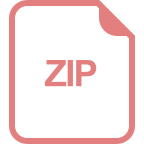
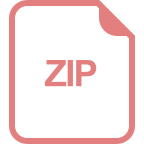
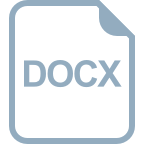
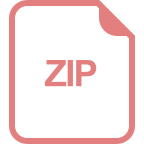
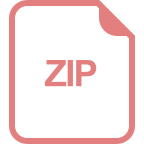
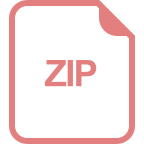
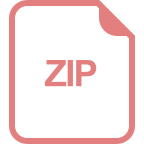
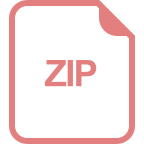
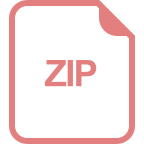
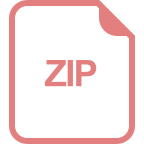
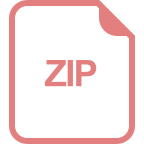
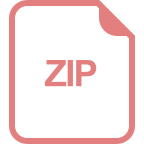
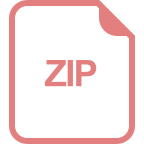
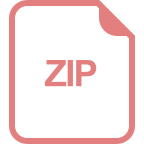
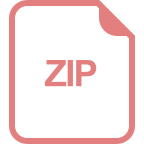
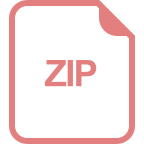
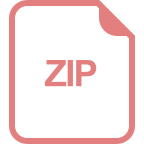
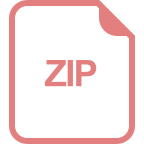
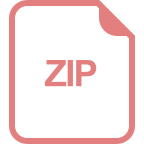
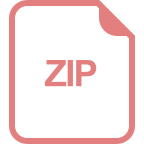
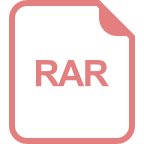
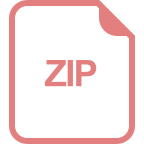
收起资源包目录

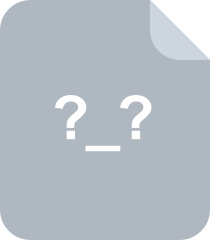
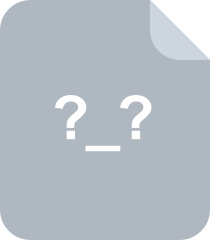
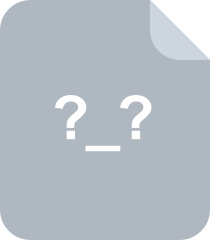
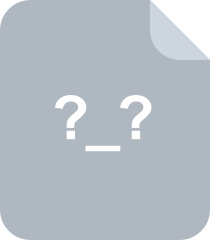
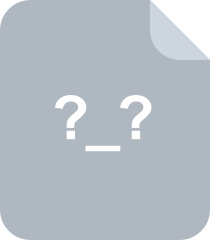
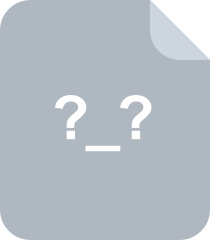
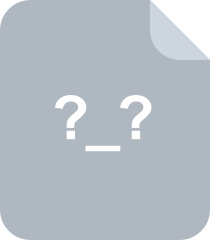
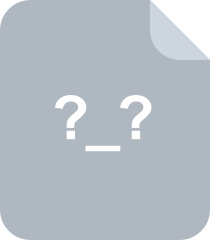
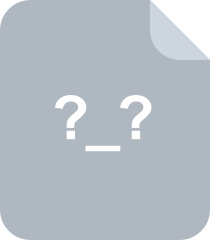
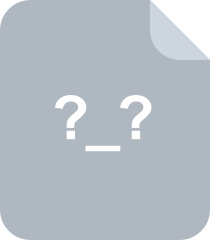
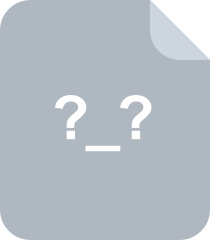
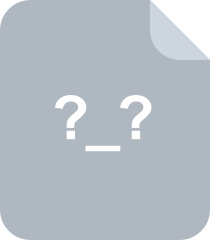
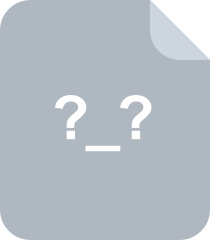
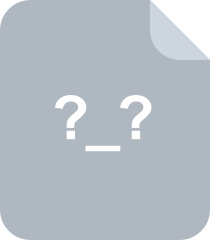
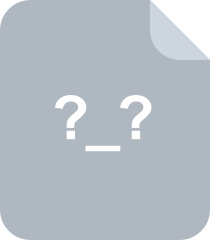
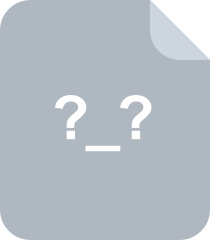
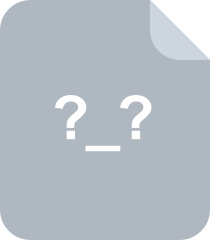
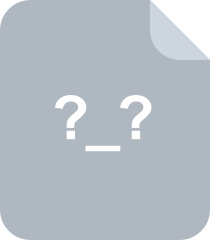
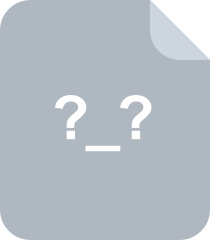
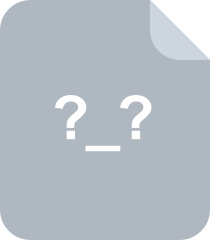
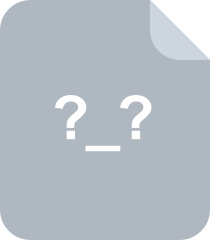
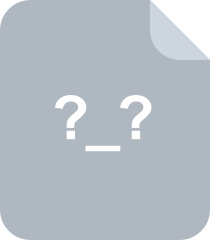
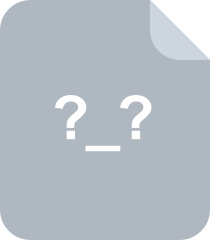
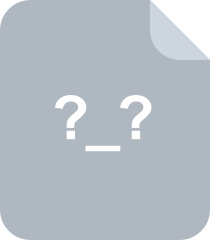
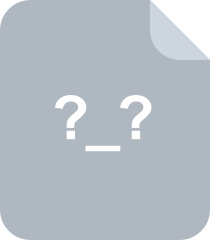
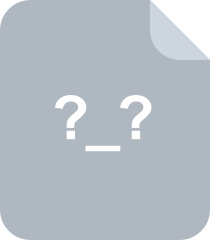
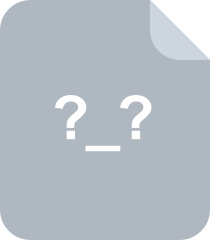
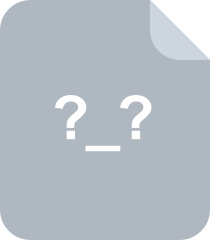
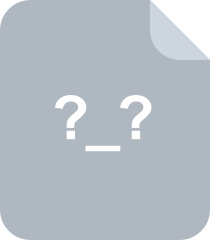
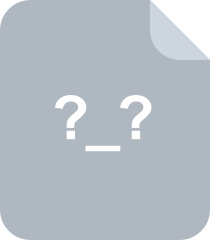
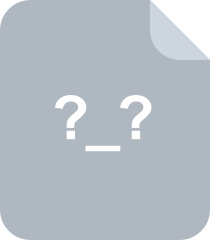
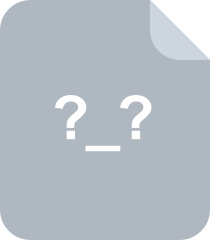
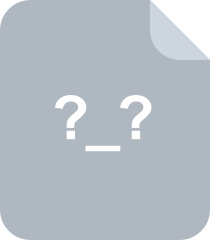
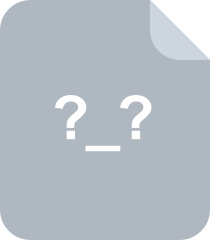
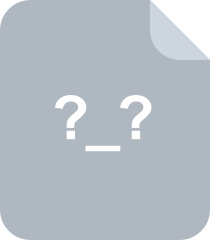
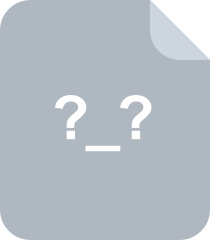
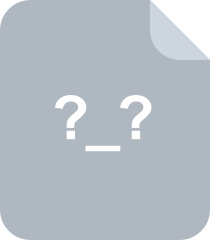
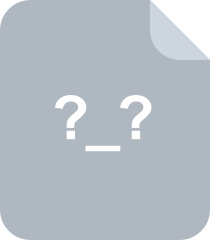
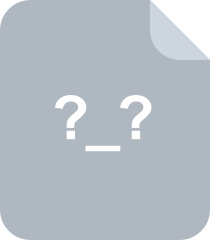
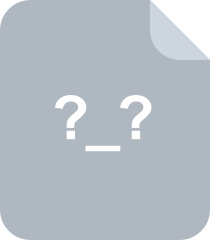
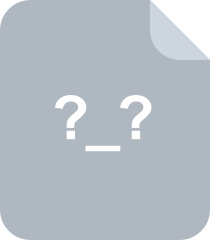
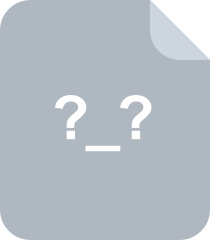
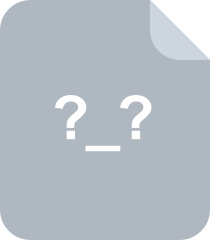
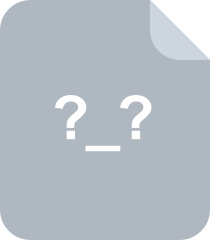
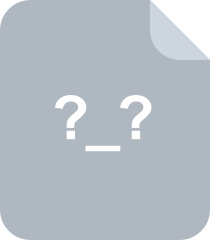
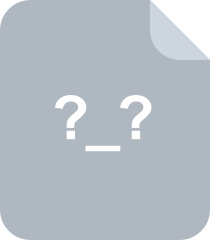
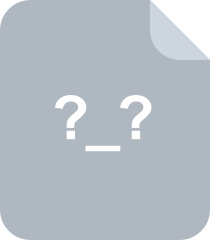
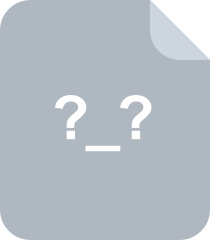
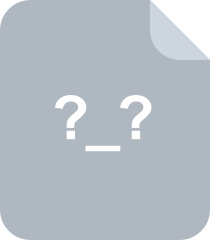
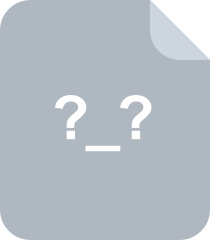
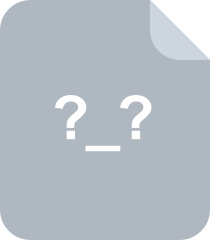
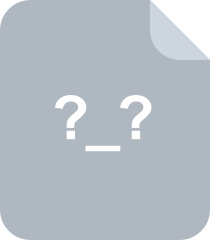
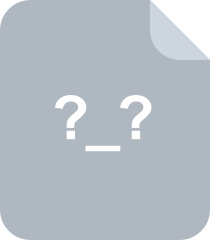
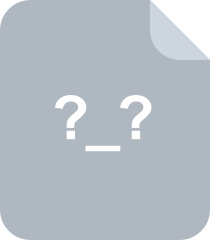
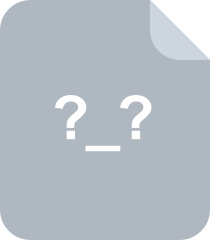
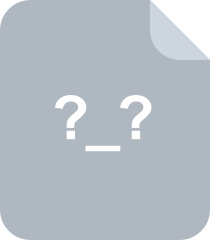
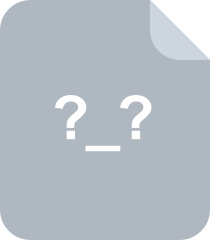
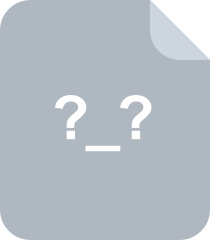
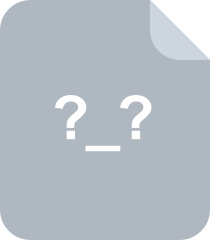
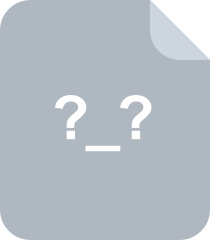
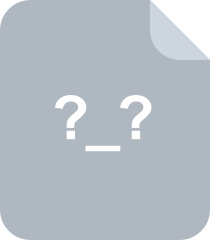
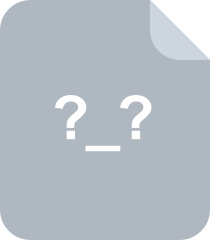
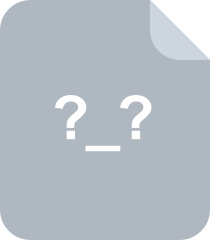
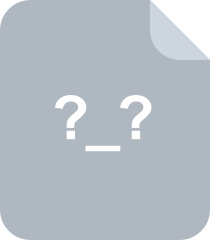
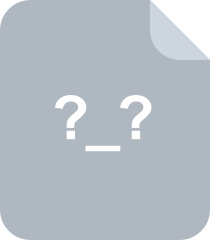
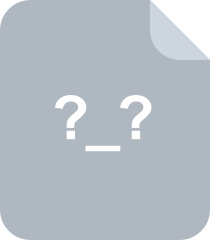
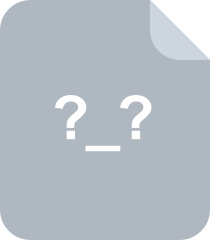
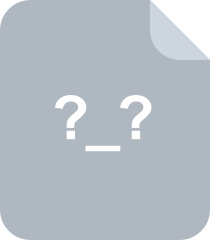
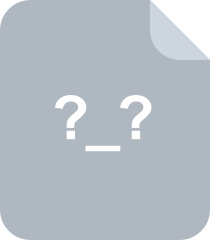
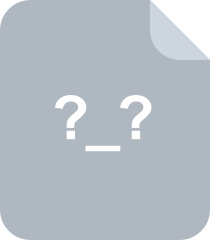
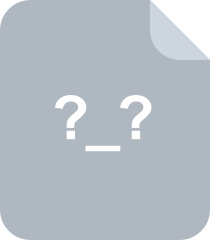
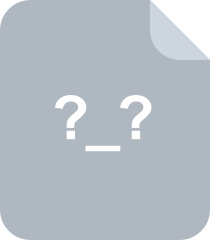
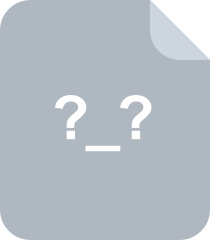
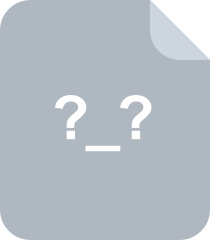
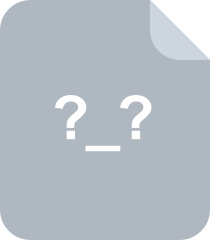
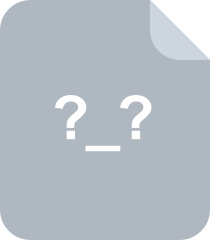
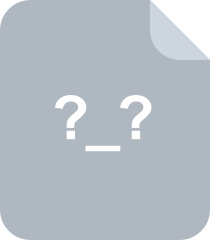
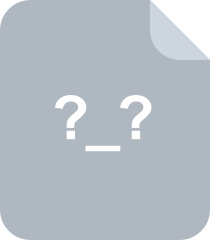
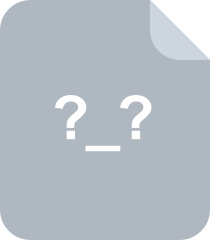
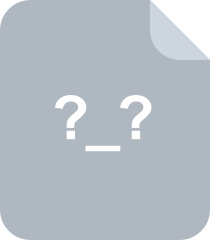
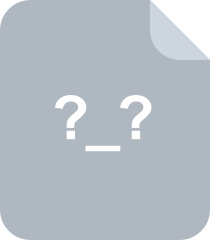
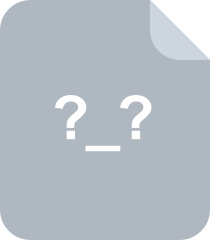
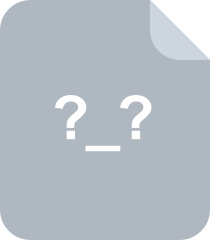
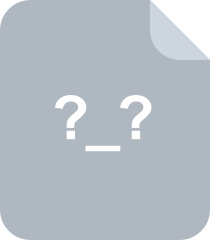
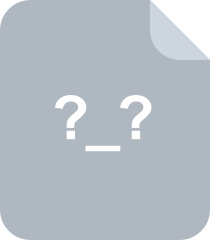
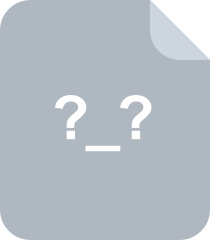
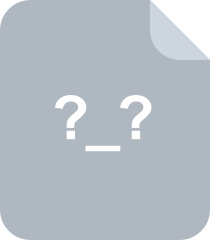
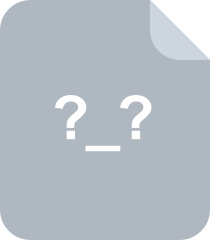
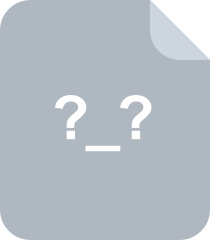
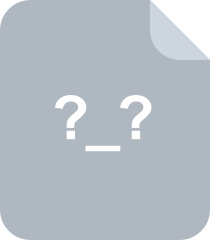
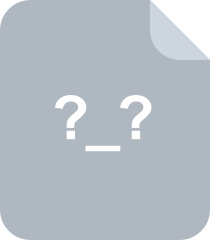
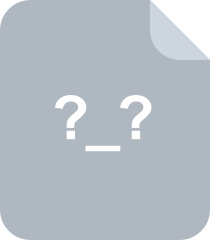
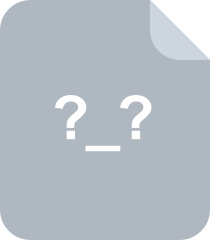
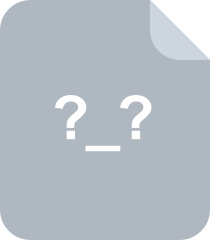
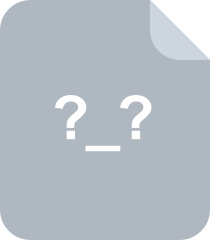
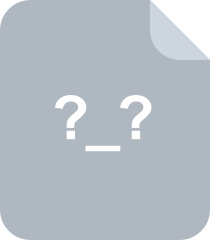
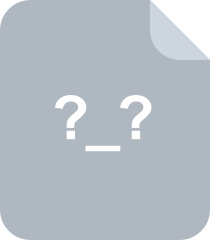
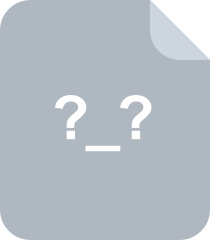
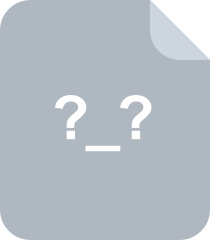
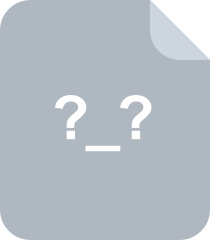
共 5937 条
- 1
- 2
- 3
- 4
- 5
- 6
- 60
资源评论
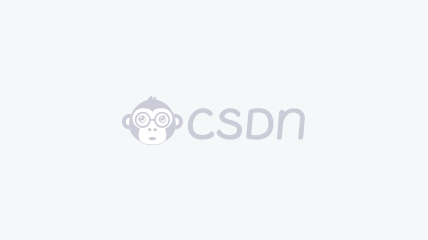

童小纯
- 粉丝: 3w+
- 资源: 289
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

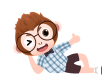
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


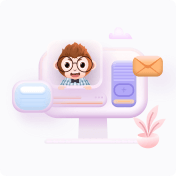
安全验证
文档复制为VIP权益,开通VIP直接复制
