#include "hbattery.h"
hBattery::hBattery(QWidget *parent) : QWidget(parent)
{
m_CurrentValue = 20;
m_Margin = 1;
m_BatteryMAXValue = 100;
m_BatteryMinValue = 0;
m_BatteryWidth = 50;
m_BatteryHeight = 25;
m_IsRecharge = false;
m_BorderPen = QPen(Qt::gray, 2);
m_TextPen = QPen(Qt::white);
m_LightingPen = QPen(Qt::yellow, 2);
m_TextFont = QFont("Arial", 11);
p_RechargeTimer = new QTimer;
connect(p_RechargeTimer, &QTimer::timeout, this, &hBattery::fRechargeRun);
}
/** *******************************************************************
* @Function : paintEvent
* @Parameter : QPaintEvent
* @Return : 无
* @Author : hx
* @Description : 重写绘制事件
* @Date : 2024-10-25 14:24:44
******************************************************************* **/
void hBattery::paintEvent(QPaintEvent * event)
{
Q_UNUSED(event);
//绘制准备工作,启用反锯齿
QPainter painter(this);
painter.setRenderHints(QPainter::Antialiasing | QPainter::TextAntialiasing);
//1.绘制边框和头部
fDrawBorder(&painter);
//2.绘制填充
fDrawBackground(&painter);
//3.内部百分比
fDrawText(&painter);
//4.是否充电
if(m_IsRecharge)
fDrawLightning(&painter);
}
/** *******************************************************************
* @Function : fDrawBorder
* @Parameter : QPainter 画笔
* @Return : 无
* @Author : hx
* @Description : 绘制电池边宽
* @Date : 2024-10-25 14:24:44
******************************************************************* **/
void hBattery::fDrawBorder(QPainter *painter)
{
//保存状态
painter->save();
//设置笔的颜色和粗细
painter->setPen(m_BorderPen);
//没有画刷填充
painter->setBrush(Qt::NoBrush);
//绘制给定的圆角矩形 矩形 xRadius yRadius
//painter->drawRoundedRect(batteryRect, borderRadius, borderRadius);
//电池边框居中
m_BatteryRect = QRectF((width() - m_BatteryWidth)/2, (height() - m_BatteryHeight)/2, m_BatteryWidth, m_BatteryHeight);
painter->drawRoundedRect(m_BatteryRect, 3, 3);
//电池头部:画一条直线
painter->setPen(QPen(Qt::gray, 5));
QLineF line(m_BatteryRect.topRight().x() + 3, m_BatteryRect.topRight().y() + 8, m_BatteryRect.topRight().x() + 3, m_BatteryRect.bottomRight().y() - 8);
painter->drawLine(line);
//恢复状态
painter->restore();
}
/** *******************************************************************
* @Function : fDrawBackground
* @Parameter : QPainter 画笔
* @Return : 无
* @Author : hx
* @Description : 绘制电池电量背景
* @Date : 2024-10-25 14:24:44
******************************************************************* **/
void hBattery::fDrawBackground(QPainter *painter)
{
int tempBattery;
if(!m_IsRecharge)
tempBattery = m_CurrentValue;
else
tempBattery = m_RechargeValue;
//保存状态
painter->save();
//确定画刷颜色
if(tempBattery <= 10)
//红
painter->setBrush(QColor(204, 38, 38));
else if (tempBattery <= 30)
//黄
painter->setBrush(QColor(198, 163, 0));
else
//绿
painter->setBrush(QColor(50, 205, 51));
//当前电量转化为宽
double width = tempBattery * (m_BatteryRect.width() - (m_Margin * 2)) / 100;
//确定左上角位置
QPointF topLeft(m_BatteryRect.topLeft().x() + m_Margin, m_BatteryRect.topLeft().y() + m_Margin);
//确定变化的右下角, 最小给个10, 显示内部的填充
QPointF bottomRight(m_BatteryRect.topLeft().x() + width + m_Margin, m_BatteryRect.bottomRight().y() - m_Margin);
QRectF rect(topLeft, bottomRight);
//没有线宽
painter->setPen(Qt::NoPen);
painter->drawRoundedRect(rect, 5, 5);
//恢复状态
painter->restore();
}
/** *******************************************************************
* @Function : fDrawText
* @Parameter : QPainter 画笔描述
* @Return : 无
* @Author : hx
* @Description : 绘制文字
* @Date : 2024-10-25 14:24:44
******************************************************************* **/
void hBattery::fDrawText(QPainter *painter)
{
//保存状态
painter->save();
painter->setPen(m_TextPen);
painter->setFont(m_TextFont);
QString value;
if(!m_IsRecharge)
value = QString::number(m_CurrentValue) + "%";
else
value = QString::number(m_RechargeValue) + "%";
//文本居中的好方法
if(m_IsRecharge)
painter->drawText(m_BatteryRect, Qt::AlignLeft | Qt::AlignVCenter, value);
else
painter->drawText(m_BatteryRect, Qt::AlignHCenter | Qt::AlignVCenter, value);
//恢复状态
painter->restore();
}
/** *******************************************************************
* @Function : fDrawLightning
* @Parameter : QPainter 画笔
* @Return : 无
* @Author : hx
* @Description : 绘制充电符号
* @Date : 2024-10-25 14:24:44
******************************************************************* **/
void hBattery::fDrawLightning(QPainter *painter)
{
// 保存状态
painter->save();
// 设置闪电的颜色5
painter->setPen(m_LightingPen); // 更细的线条
// 计算文本的中心位置
QPointF textCenter = m_BatteryRect.center();
// 计算闪电的起始点(在文本右侧)
QPointF startPoint(textCenter.x() + 15, textCenter.y() - 8); // 向右偏移
// 绘制闪电,只拐两下
QPolygon lightning;
lightning << QPoint(startPoint.x(), startPoint.y()) //起点
<< QPoint(startPoint.x() - 5, startPoint.y() + 8) // 向左下
<< QPoint(startPoint.x() + 5, startPoint.y() + 8) // 向右下
<< QPoint(startPoint.x() - 5, startPoint.y() + 16) // 向左下
<< QPoint(startPoint.x(), startPoint.y() + 8); // 终点
painter->drawPolygon(lightning);
// 恢复状态
painter->restore();
}
/** *******************************************************************
* @Function : fSetBatteryValue
* @Parameter : batteryValue 电量
* @Return : 无
* @Author : hx
* @Description : 设置当前电池电量
* @Date : 2024-10-25 16:58:17
******************************************************************* **/
void hBattery::fSetBatteryValue(int batteryValue)
{
m_CurrentValue = batteryValue;
this->update();
}
/** *******************************************************************
* @Function : fSetBatteryStatus
* @Parameter : isRecharge 是否充电
* @Return : 无
* @Author : hx态
* @Description : 设置电池充放电状态
* @Date : 2024-10-25 16:59:25
******************************************************************* **/
void hBattery::fSetBatteryStatus(bool isRecharge)
{
m_IsRecharge = isRecharge;
m_RechargeValue = m_CurrentValue;
if(m_IsRecharge)
p_RechargeTimer->start(100);
else
{
p_RechargeTimer->stop();
this->update();
}
}
/** *******************************************************************
* @Function : fSetColorstyle
* @Parameter : borderPen 边框样式
* textPen 文字样式
* lightingPen 充电符号样式
* @Return : 无
* @Author : hx
* @Description : 设置电池样式
* @Date : 2024-10-25 17:00:26
******************************************************************* **/
void hBattery::fSetColorstyle(const QPen &borderPen, const QPen &textPen, const QPen &lightingPen)
{
m_BorderPen = borderPen;
m_TextPen = textPen;
m_LightingPen = lightingPen;
this->update();
}
/** ******************

三分糖丶去冰
- 粉丝: 54
- 资源: 3
最新资源
- Redis核心数据结构解析:字符串与列表的实现及应用场景
- PyTorch模型部署与服务化:模型导出优化、容器化、服务化架构及安全措施
- 基于储能的直驱风电机组并网仿真模型 直驱风电机组,先整流后逆变,不控整流器?pwm控制逆变器,出口电压380v,蓄电池储能经dcdc变器接入直流母线,可控制充放电,直流母线接有直流负载,可做加减负载突
- Go实战全家桶之三十三: go pprof定位问题,自己埋的坑
- Go实战全家桶之三十三: go pprof定位问题,自己埋的坑
- MATLAB代码:用于平抑可再生能源功率波动的储能电站建模及评价 关键词:储能电站 功率波动 并网 平抑可再生能源 参考文档:《用于平抑可再生能源功率波动的储能电站建模及评价》仅参考 光伏发电容量可
- STM32驱动lcd1602显示adc采集电压显示程序源码 主控芯片采用stm32f103,包括程序源码和protues仿真protues版本8.8. 需要做AD转的不要错过 程序源码注释详细,非
- 三相UPS不间断电源 从工频交流电开始,完成三相桥式整流电路、升压斩波电路及三相桥式PWM逆变电路的交-直-交变整个流程 类似于一个UPS对输入电源的变过程
- sTM32 ADC采集滤波算法,卡尔曼 中位值 同步对比输出源程序,芯片采用STM32f103c8t6.算法采用卡尔曼滤波算法中位值滤波算法, 波形输出正常采集的卡尔曼 中位值三个波形输出,程序注释详
- MMC并网逆变器(滑模控制) 1.MMC工作在整流侧,子模块个数N=22, 直流侧电压Udc=11kV,交流侧电压6.6kV 2.控制器采用双闭环控制,外环控制有功功率,采用PI调节器,电流内环采用无
- 2023-04-06-项目笔记 - 第三百七十二阶段 - 4.4.2.370全局变量的作用域-370 -2025.01.08
- 西门子界面官方精美触摸屏+WINCC程序模板 西门子官方触摸屏程序模板,炫酷的扁平式动画效果,脚本动画,自动生成二维码,可仿真,堪比智能手机,有精简,精致,wincc,无线面板等包含了所有西门子人机界
- 永磁同步电机的脉振高频注入仿真,可实现零速带满载启动,转速估算精度与角度估算精度非常高
- 双 向 绑 定~~~~~~~~~~~~~~~~~~~~~~~~
- bugreport-2025-01-08-220002.zip
- 高通量计算(Pandat代算或自己操作) 高通量计算筛选材料 实例6:在 Ni-xCr-yAl (x=10-100,y=10-100)成分空间中,合金的液相线、固相线、相含量的变化
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


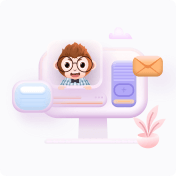