/*
软件作者:https://xuhss.com/oxox/pro
*/
/**
* 使用说明请看 https://github.com/e1399579/autojs/blob/master/README.md
* @author ridersam <e1399579@gmail.com>
*/
auto(); // 自动打开无障碍服务
//初始化配置
if (!files.isFile("config.js")) {
log("config.js配置文件丢失,请检查");
exit();
}
var config = require("config.js");
var options = Object.assign({}, config);; // 用户配置
// 所有操作都是竖屏
const WIDTH = Math.min(device.width, device.height);
const HEIGHT = Math.max(device.width, device.height);
//检查是否有ROOT权限
const IS_ROOT = files.exists("/sbin/su") || files.exists("/system/xbin/su") || files.exists("/system/bin/su");
//设置脚本坐标点击所适合的屏幕宽高
setScreenMetrics(WIDTH, HEIGHT);
var source = "antForest";
var stateStorage = storages.create(source);
var times = 0; //脚本执行计数
var sleepTime = 300; //默认循环时间
var isCircu = true; //是否循环
var Robot = require("Robot.js");
var robot = new Robot(options.max_retry_times);
var package = "com.eg.android.AlipayGphone"; // 支付宝包名
var state = {};
var capture = null;
var bounds = [0, 0, WIDTH, 1100];
if (device.isScreenOn()) {
toastLog("请关闭手机屏幕,手机才会执行脚本");
while (device.isScreenOn()) {
sleep(1000);
}
saveState();
log("锁屏后开始计时");
var d1 = new Date();
var d2 = new Date();
d2 = d2.setSeconds(d2.getSeconds() + 10);
while (true) {
sleep(1000);
d1 = new Date();
if (d1 > d2) {
log("计时结束");
break;
}
}
device.wakeUp();
}
//循环脚本
while (true) {
auto(); // 自动打开无障碍服
log("---------------------------");
log("进入脚本");
checkTask(); //检测任务
var runTime = new Date();
log("运行时间:" + runTime.toDateString() + " " + runTime.getHours() + ":" + runTime.getMinutes() + ":" + runTime.getSeconds());
times += 1;
log("运行次数:" + times);
start();
}
/**
* 开始运行
* @param options
*/
function start() {
checkModule();
//获取手机当前状态
//saveState();
while (!device.isScreenOn()) {
device.wakeUp();
sleep(1000); // 等待屏幕亮起
}
toastLog("即将收取能量,按音量上键停止");
// 子线程监听音量上键
threads.start(function() {
events.observeKey();
events.onceKeyDown("volume_up", function(event) {
toastLog("停止脚本");
engines.stopAll();
exit();
});
});
// 监听退出事件
events.on("exit", function() {
running = stateStorage.get("running", false);
if (running) {
storages.remove(source);
toastLog("完全退出");
}
});
// 先打开APP,节省等待时间
threads.start(function() {
openApp();
});
//解锁
if (files.exists("Secure.js")) {
var Secure = require("Secure.js");
var secure = new Secure(robot, options.max_retry_times);
if (!secure.openLock(options.password, options.pattern_size)) {
log("解锁失败!");
exit();
}
// 拉起到前台界面
openApp();
} else {
log("解锁脚本不存在:Secure.js");
}
//启动APP
enterApp();
//收取能量
work();
//回到原来的状态
resumeState();
//外部循环检测
checkCirculationScript();
//退出脚本
storages.remove(source);
//exit();
}
//检测外部是否有循环脚本
function checkCirculationScript() {
if (isCircu) {
//判断是否处于夜晚(夜晚不收能量)
var today = new Date();
var hour = today.getHours();
var starHour = 0;
var endHour = 7;
var isEnter = false;
while (true) {
if (hour >= 0 && hour < 7) {
sleep(1000);
today = new Date();
hour = today.getHours();
if (!isEnter) {
log("进入夜间");
isEnter = true; //跳入夜间
}
continue;
} else {
var addTime = new Date();
var nowTime = new Date();
addTime = addTime.setSeconds(addTime.getSeconds() + sleepTime); //获取下次收取能量时间
log("进入白天");
while (true) {
//判断是否刚刚跳出夜间
if (isEnter) {
hour = nowTime.getHours();
if (hour >= 7 && hour <= 23) {
return; //跳出夜间
}
}
if (nowTime > addTime) {
return; //到达收取能量的时候了
} else {
sleep(1000);
}
nowTime = new Date();
}
}
}
}
}
/**
* 检查任务
*/
function checkTask() {
// 连续运行处理
var running = stateStorage.get("running", false);
if (!running) { //没有其他线程运行
log("队列:无其他任务");
stateStorage.put("running", true);
} else {
log("队列:当前有任务");
//exit();
}
}
/**
* 检查必要模块
*/
function checkModule() {
if (!files.exists("Robot.js")) {
throw new Error("缺少Robot.js文件,请核对第一条");
}
if (!files.exists("Secure.js") && context.getSystemService(context.KEYGUARD_SERVICE).inKeyguardRestrictedInputMode()) {
throw new Error("缺少Secure.js文件,请核对第一条");
}
} //检查必要模块
/**
* 蚂蚁森林的各个操作
* @param robot
* @param options
* @constructor
*/
function saveState() {
state.isScreenOn = device.isScreenOn();
state.currentPackage = currentPackage(); // 当前运行的程序
state.isRunning = IS_ROOT ? parseInt(shell("ps | grep 'AlipayGphone' | wc -l", true).result) : 0; // 支付宝是否运行
state.version = context.getPackageManager().getPackageInfo(this.package, 0).versionName;
log(state);
};
function resumeState() {
if (state.currentPackage !== package) {
back(); // 回到之前运行的程序
sleep(1500);
}
if (!state.isRunning) {
closeApp();
}
if (!state.isScreenOn) {
KeyCode("KEYCODE_POWER");
}
};
function openApp() {
launch(package);
};
function closeApp() {
robot.kill(package);
};
function enterApp() {
var times = 0;
do {
if (this.doLaunch()) {
return;
} else {
times++;
this.back();
sleep(1500);
this.openApp();
}
} while (times < this.options.max_retry_times);
toastLog("运行失败");
exit();
};
function doLaunch() {
// 可能出现的红包弹框,点击取消
var timeout = options.timeout;
threads.start(function() {
var cancelBtn;
if (cancelBtn = id("com.alipay.android.phone.wallet.sharetoken:id/btn1").findOne(timeout)) {
cancelBtn.click();
}
});
var home = id("com.alipay.android.phone.openplatform:id/tab_description").text("首页").findOne(timeout);
if (!home) {
toastLog("进入支付宝首页失败");
return false;
}
home.parent().click();
var success = false;
var btn = id("com.alipay.android.phone.openplatform:id/app_text").text("蚂蚁森林").findOne(timeout);
if (!btn) {
toastLog("查找蚂蚁�

[虚幻私塾】
- 粉丝: 337
- 资源: 1558
最新资源
- HTML5实现好看的毛绒玩具网上商城网站源码.zip
- HTML5实现好看的美容化妆品购物网站源码.zip
- HTML5实现好看的美容美颜化妆品公司网站源码.zip
- HTML5实现好看的美容美甲学校网站网站源码.zip
- HTML5实现好看的美食餐厅网红店网站源码.zip
- 知名大厂的逆向ADC电路, SAR ADC ,sigma-delta ADC 采用的是标准单元库器件,可以直接导入到cadence环境下打开 有对应文档说明,适合有较好的模拟IC功底的小伙伴 两个
- 基于matlab的继电保护原理仿真源码(高分项目).zip
- MATLAB 实现基于SAO(雪消融优化算法)进行时间序列预测模型的项目详细实例(含完整的程序,GUI设计和代码详解)
- 重庆邮电大学概率论真题及答案(13套题目,13套答案)
- Matlab实现POA-BP鹈鹕算法优化BP神经网络多变量回归预测(含完整的程序,GUI设计和代码详解)
- 基于Matlab实现继电保护原理源码+数据+运行说明(高分项目)
- 随机美图视频HTML源码.zip
- 基于改进鹈鹕算法优化支持向量机的数据分类预测(IPOA-SVM) 改进鹈鹕算法IPOA改进点为加入混沌映射、反向差分进化和萤火虫扰动,加快鹈鹕算法的收敛速度,避免鹈鹕算法陷入局部最优 改进鹈鹕算法IP
- 使用AUTO CAD2024手工绘制CAD图签名
- Matlab实现mRMR-CNN-LSTM-Mutilhead-Attention最大相关最小冗余特征选择卷积长短期记忆神经网络融合多头注意力机制多特征分类预测(含完整的程序,GUI设计和代码详解)
- 海康物流行业读码选型培训
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


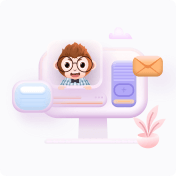