package com.xznu.edu.leave.utils;
import java.sql.Timestamp;
import java.text.DateFormat;
import java.text.ParseException;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
/**
* 日期格式化类
*/
public class DateUtils extends java.util.Date {
private static final long serialVersionUID = 1L;
private static final DateFormat datetimeFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
private static final DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
private static final String strFormat1 = "yyyy-MM-dd HH:mm";
/**
M 年中的月份 Month July; Jul; 07
w 年中的周数 Number 27
W 月份中的周数 Number 2
D 年中的天数 Number 189
d 月份中的天数 Number 10
F 月份中的星期 Number 2
E 星期中的天数 Text Tuesday; Tue
a Am/pm 标记 Text PM
H 一天中的小时数(0-23) Number 0
k 一天中的小时数(1-24) Number 24
K am/pm 中的小时数(0-11) Number 0
h am/pm 中的小时数(1-12) Number 12
m 小时中的分钟数 Number 30
s 分钟中的秒数 Number 55
S 毫秒数 Number 978
z 时区 General time zone Pacific Standard Time; PST; GMT-08:00
Z 时区 RFC 822 time zone -0800
*/
/**
* 构造函数
*/
public DateUtils() {
super(getSystemDate().getTime().getTime());
}
/**
* 当前时间
*
* @return 时间Timestamp
*/
public java.sql.Timestamp parseTime() {
return new java.sql.Timestamp(this.getTime());
}
/**
* 将Date类型转换为字符串 yyyy-MM-dd HH:mm:ss
*
* @param Date
* @return String
*/
public static String format(Date date) {
return format(date, null);
}
/**
* 将Date类型转换为字符串
*
* @param Date
* @param pattern 字符串格式
* @return String
*/
public static String format(Date date, String pattern) {
if (date == null) {
return "";
} else if (pattern == null || pattern.equals("") || pattern.equals("null")) {
return datetimeFormat.format(date);
} else {
return new SimpleDateFormat(pattern).format(date);
}
}
/**
* 将Date类型转换为字符串 yyyy-MM-dd
*
* @param Date
* @return String
*/
public static String formatDate(Date date) {
if (date == null) {
return "";
}
return dateFormat.format(date);
}
/**
* 将字符串转换为Date类型
*
* @param sDate yyyy-MM-dd HH:mm:ss
* @return
*/
public static Date convert(String sDate) {
try {
if (sDate != null) {
if (sDate.length() == 10) {
return dateFormat.parse(sDate);
} else if (sDate.length() == 19) {
return datetimeFormat.parse(sDate);
}
}
} catch (ParseException pe) {
}
return convert(sDate, null);
}
/**
* 将字符串转换为Date类型
*
* @param sDate
* @param pattern 格式
* @return
*/
public static Date convert(String sDate, String pattern) {
Date date = null;
try {
if (sDate == null || sDate.equals("") || sDate.equals("null")) {
return null;
} else if (pattern == null || pattern.equals("") || pattern.equals("null")) {
return datetimeFormat.parse(sDate);
} else {
return new SimpleDateFormat(pattern).parse(sDate);
}
} catch (ParseException pe) {
}
return date;
}
/**
* String转换为Date
*
* @param sDate 日期"yyyy-MM-dd"
* @return 日期Date
*/
public static Date convertDate(String dateStr) {
return convert(dateStr, "yyyy-MM-dd");
}
/**
* String转换为Timestamp
*
* @param sDate 日期 "yyyy-MM-dd" / "yyyy-MM-dd HH:mm:ss"
* @return 日期Timestamp
*/
public static Timestamp convertTimestamp(String sDate) {
if (sDate.length() == 10) {
sDate = sDate + " 00:00:00";
}
if (sDate.length() == 16) {
sDate = sDate + ":00";
}
return Timestamp.valueOf(sDate);
}
/**
* Date转换为Timestamp
*/
public static Timestamp convert(Date date) {
return new Timestamp(date.getTime());
}
/**
* 取当前日期(yyyy-mm-dd)
*
* @return 时间Timestamp
*/
public static String getTodayDate() {
return formatDate(new Date());
}
/**
* 取当前日期(yyyy-mm-dd hh:mm:ss)
*
* @return 时间Timestamp
*/
public static String getTodayDateTime() {
return format(new Date());
}
/**
* 取得n分钟后的时间
*
* @param date 日期
* @param afterMins
* @return 时间Timestamp
*/
public static Date getAfterMinute(Date date, long afterMins) {
if (date == null)
date = new Date();
long longTime = date.getTime() + afterMins * 60 * 1000;
return new Date(longTime);
}
// public static void main(String[] arg) {
// System.err.println(format((new Date())));
// System.err.println(format(getAfterMinute(new Date(), 3)));
// }
/**
* 取得指定日期几天后的日期
*
* @param date 日期
* @param afterDays 天数
* @return 日期
*/
public static Date getAfterDay(Date date, int afterDays) {
if (date == null)
date = new Date();
GregorianCalendar cal = new GregorianCalendar();
cal.setTime(date);
cal.add(java.util.Calendar.DATE, afterDays);
return cal.getTime();
}
/**
* 取得指定日期几天后的日期
*
* @param sDate 日期 yyyy-MM-dd
* @param afterDays 天数
* @return 日期
*/
public static String getAfterDay(String sDate, int afterDays) {
Date date = convertDate(sDate);
date = getAfterDay(date, afterDays);
return formatDate(date);
}
/**
* 取得指定日期几天前的日期
*
* @param date 日期
* @param beforeDays 天数(大于0)
* @return 日期
*/
public static Date getBeforeDay(Date date, int beforeDays) {
if (date == null)
date = new Date();
GregorianCalendar cal = new GregorianCalendar();
cal.setTime(date);
cal.add(java.util.Calendar.DATE, 0 - Math.abs(beforeDays));
return cal.getTime();
}
/**
* 取得指定日期几天前的日期
*
* @param sDate 日期 yyyy-MM-dd
* @param beforeDays 天数(大于0)
* @return 日期
*/
public static String getBeforeDay(String sDate, int beforeDays) {
Date date = convertDate(sDate);
date = getBeforeDay(date, beforeDays);
return formatDate(date);
}
/**
* 获得几个月后的日期
*
* @param date 日期
* @param afterMonth 月数
* @return 日期Date
*/
public static Date getAfterMonth(Date date, int afterMonth) {
if (date == null)
date = new Date();
GregorianCalendar cal = new GregorianCalendar();
cal.setTime(date);
cal.add(java.util.Calendar.MONTH, afterMonth);
return cal.getTime();
}
/**
* 获得几个月后的日期
*
* @param sDate 日期
* @param afterMont
没有合适的资源?快使用搜索试试~ 我知道了~
Java图书借阅管理系统源码(前台+后台)
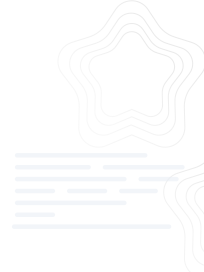
共1594个文件
png:327个
js:321个
jpg:197个

1 下载量 16 浏览量
2023-02-14
09:53:25
上传
评论 2
收藏 77.74MB ZIP 举报
温馨提示
1.管理员只能有一个。 注意:会员查看借阅记录只能看到自己的。管理员可以看到所有 会员权限: 对个人信息的查看 密码修改。 图书管理:对书籍的添加 修改上架 删除(仅能查看自己的) 借阅管理:出借确认,归还确认,历史记录(仅能查看自己的) 管理员: 对用户的禁用启用 分类管理 2.用户上架的书籍需要通过管理员审核才能在前台显示。 对所有借阅记录的查看 3.点击图书名称能够显示书籍详情(在后台凡是有书名的地方都可以点击看详情)。 4.评论的时候乱码 5.在管理员 对图书管理的时候 加上一列 书籍所有人的名字就好 6.对书籍的统计(统计最近哪些类型的书借了多少)
资源推荐
资源详情
资源评论
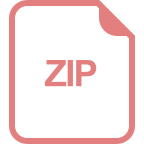
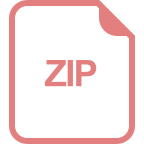
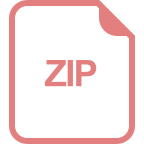
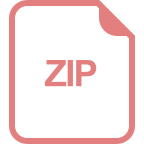
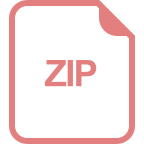
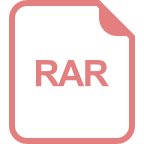
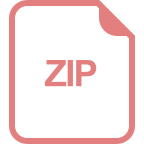
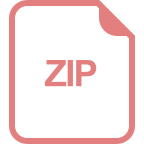
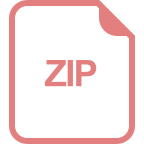
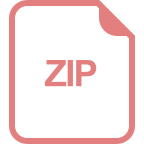
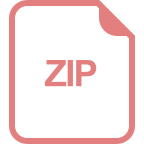
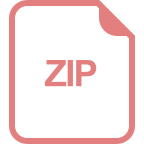
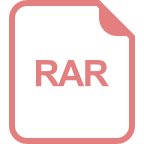
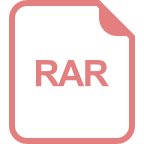
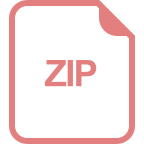
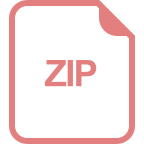
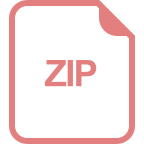
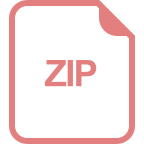
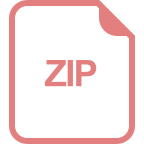
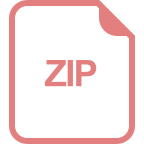
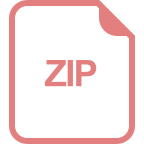
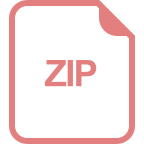
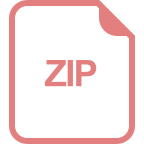
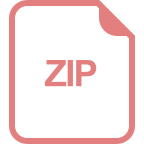
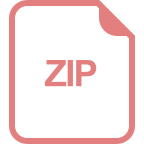
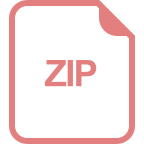
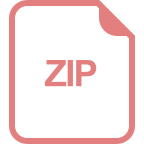
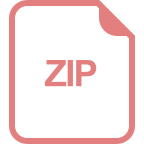
收起资源包目录

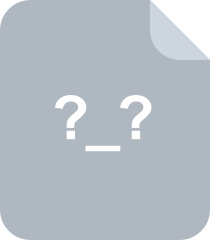
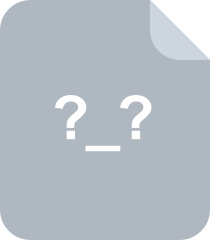
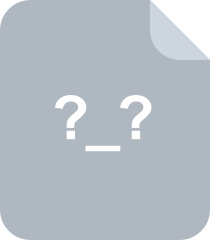
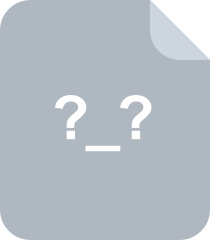
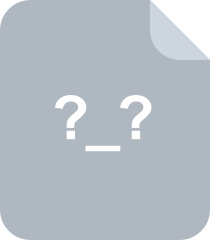
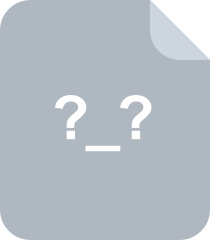
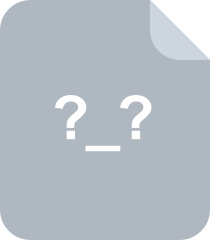
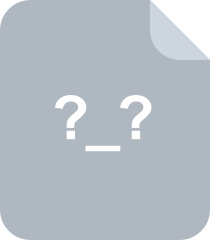
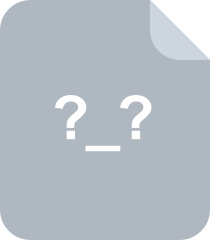
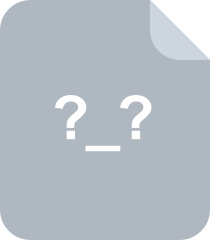
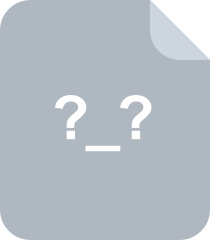
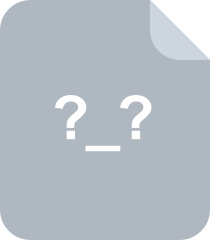
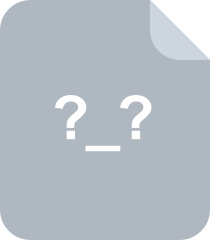
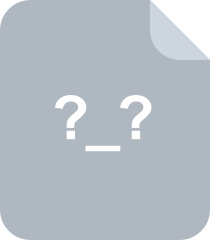
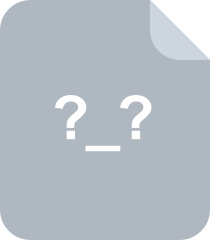
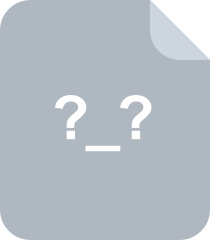
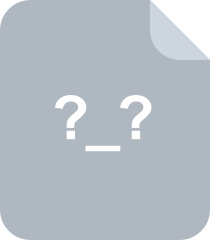
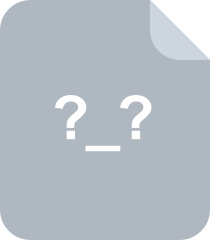
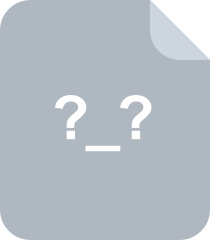
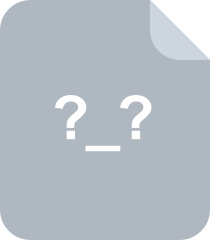
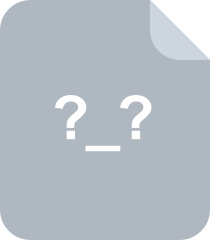
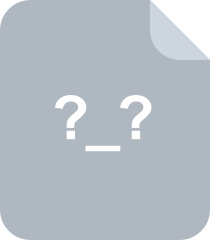
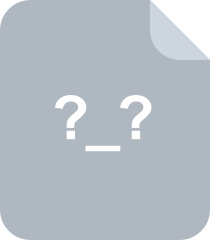
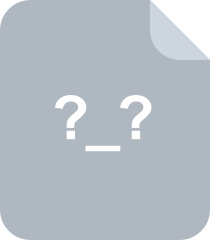
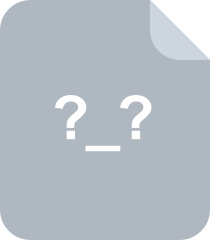
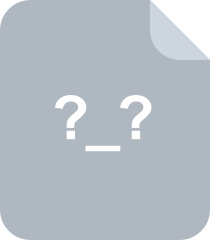
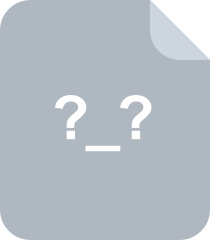
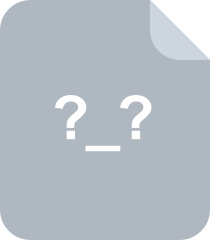
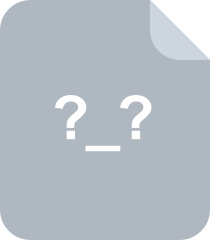
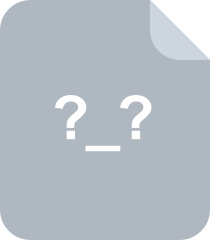
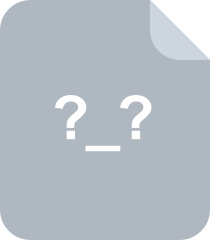
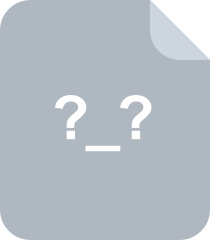
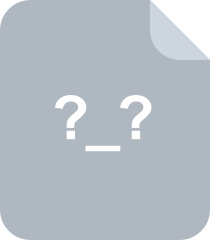
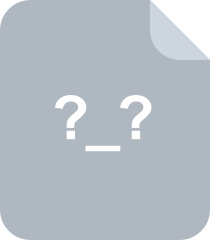
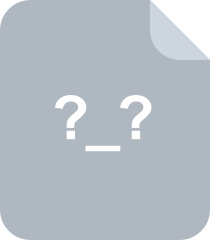
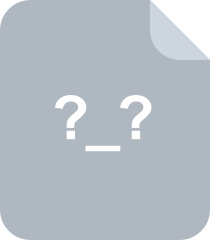
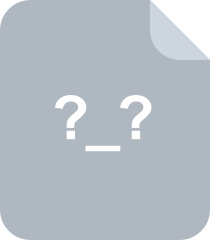
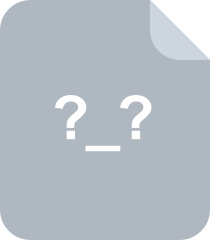
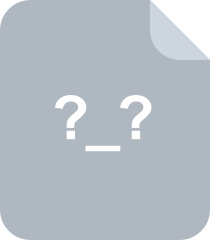
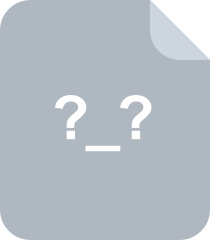
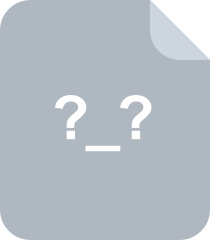
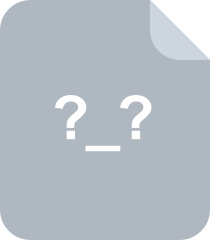
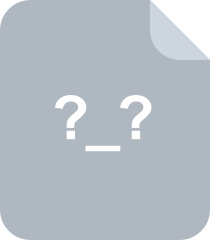
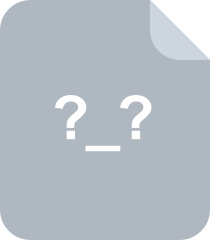
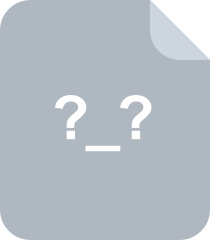
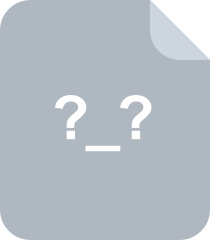
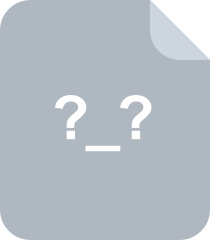
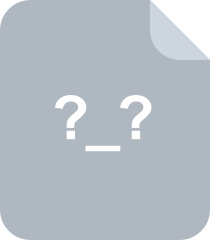
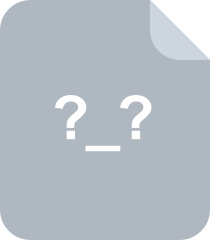
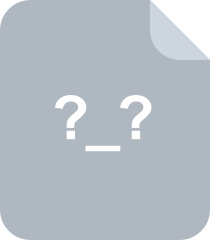
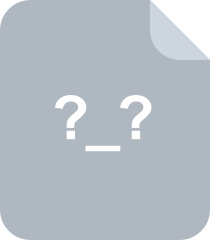
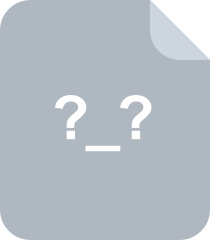
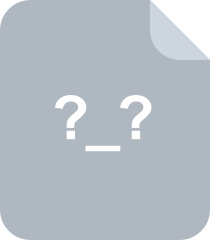
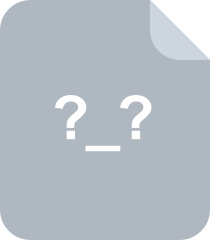
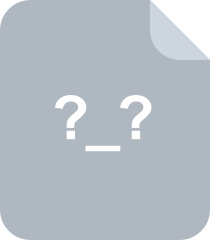
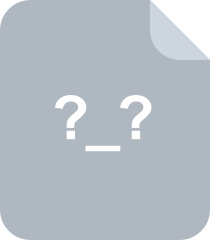
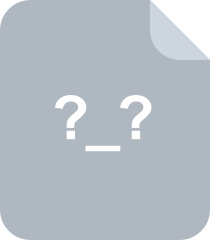
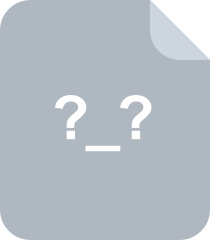
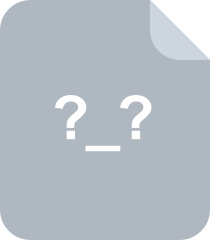
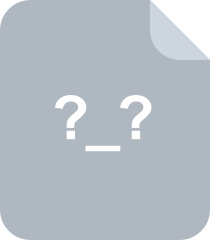
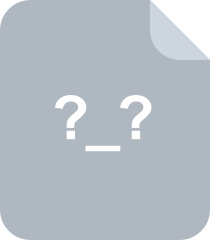
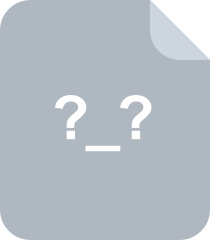
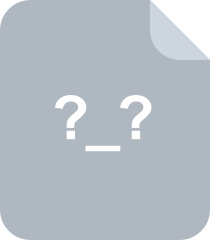
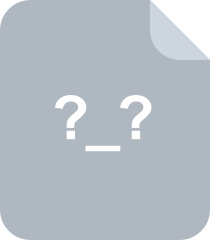
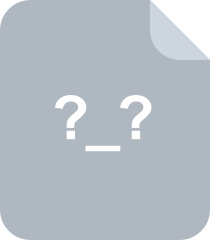
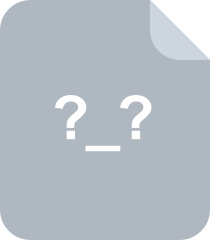
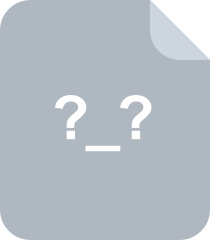
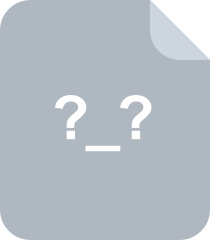
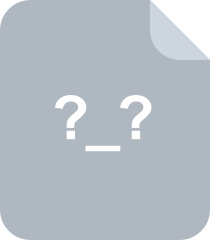
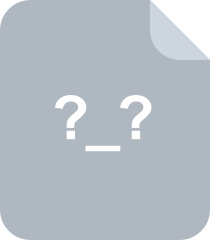
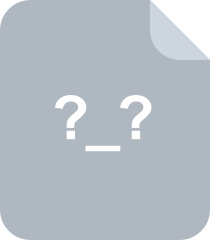
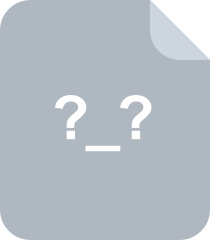
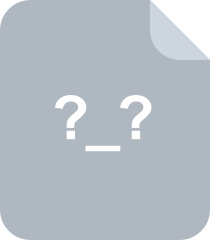
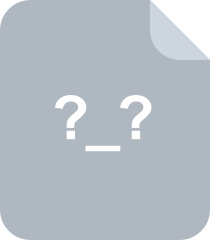
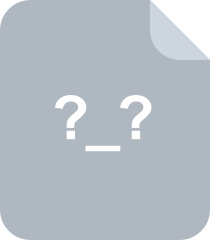
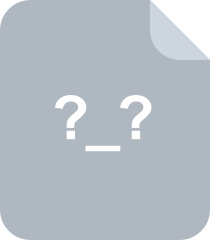
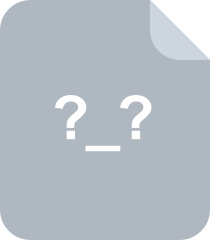
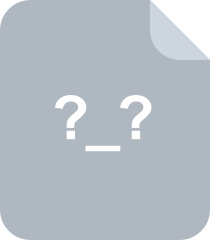
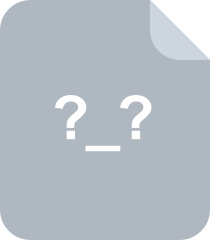
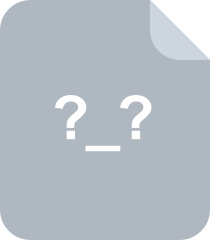
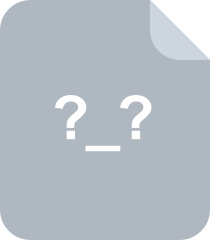
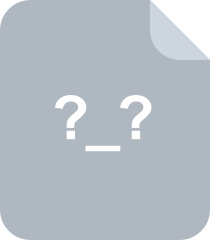
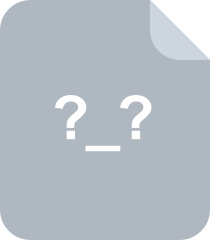
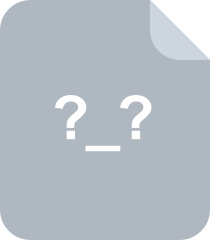
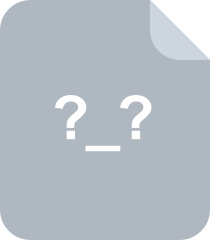
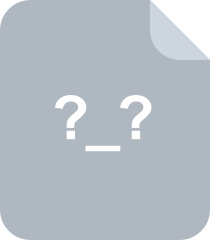
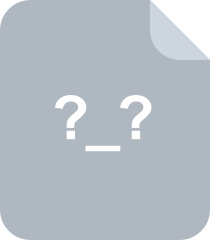
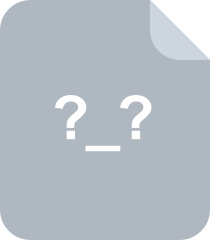
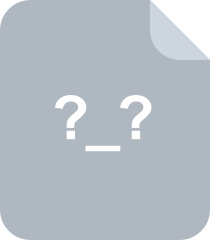
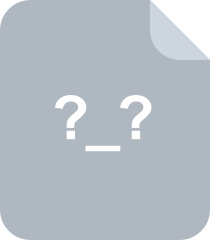
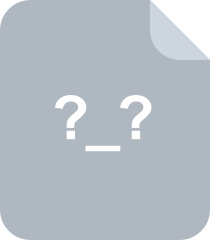
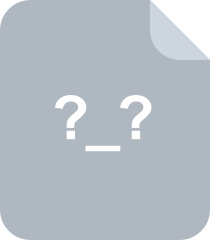
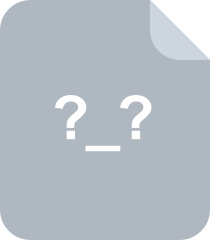
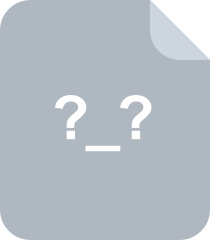
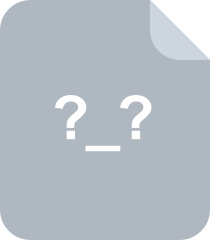
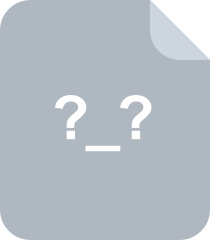
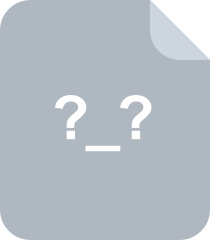
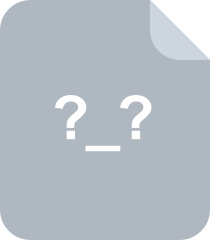
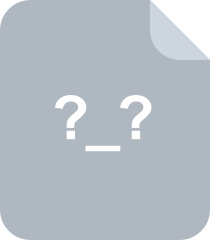
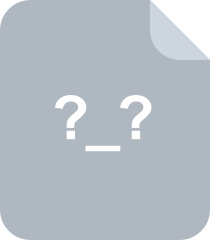
共 1594 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
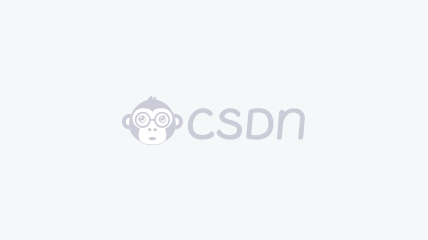

大山源码
- 粉丝: 42
- 资源: 107
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

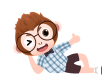
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


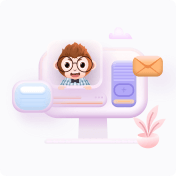
安全验证
文档复制为VIP权益,开通VIP直接复制
