<?php
/**
* 旺铺点餐模块小程序接口定义
*
* @author 易-福-网
* @author 易-福-网 */
ini_set("memory_limit", "500M");
defined('IN_IA') or exit('Access Denied');
class Zh_cjdiancModuleWxapp extends WeModuleWxapp
{
public function doPageSystem()
{
global $_W, $_GPC;
$res = pdo_get('cjdc_system', array('uniacid' => $_W['uniacid']));
if ($res['gs_img']) {
if (strpos($res['gs_img'], ',')) {
$res['gs_img'] = explode(',', $res['gs_img']);
} else {
$res['gs_img'] = array(
0 => $res['gs_img']
);
}
}
$res['attachurl'] = $_W['attachurl'];
echo json_encode($res);
}
//url(七牛)
public function doPageUrl()
{
global $_GPC, $_W;
echo $_W['attachurl'];
}
//获取openid
public function doPageOpenid()
{
global $_W, $_GPC;
$res = pdo_get('cjdc_system', array('uniacid' => $_W['uniacid']));
$code = $_GPC['code'];
$appid = $res['appid'];
$secret = $res['appsecret'];
// echo $appid;die;
$url = "https://api.weixin.qq.com/sns/jscode2session?appid=" . $appid . "&secret=" . $secret . "&js_code=" . $code . "&grant_type=authorization_code";
function httpRequest($url, $data = null)
{
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE);
if (!empty($data)) {
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_POSTFIELDS, $data);
}
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
//执行
$output = curl_exec($curl);
curl_close($curl);
return $output;
}
$res = httpRequest($url);
print_r($res);
}
//登录用户信息
public function doPageLogin()
{
global $_GPC, $_W;
$openid = $_GPC['openid'];
$res = pdo_get('cjdc_user', array('openid' => $openid, 'uniacid' => $_W['uniacid']));
if ($res) {
$user_id = $res['id'];
if ($_GPC['img']) {
$data['img'] = $_GPC['img'];
}
if ($_GPC['name']) {
$data['name'] = $_GPC['name'];
}
if ($_GPC['openid']) {
$data['openid'] = $_GPC['openid'];
}
$res = pdo_update('cjdc_user', $data, array('id' => $user_id));
$user = pdo_get('cjdc_user', array('openid' => $openid, 'uniacid' => $_W['uniacid']));
echo json_encode($user);
} else {
$data['openid'] = $_GPC['openid'];
$data['img'] = $_GPC['img'];
$data['name'] = $_GPC['name'];
$data['uniacid'] = $_W['uniacid'];
$data['join_time'] = time();
$res2 = pdo_insert('cjdc_user', $data);
$user = pdo_get('cjdc_user', array('openid' => $openid, 'uniacid' => $_W['uniacid']));
echo json_encode($user);
}
}
//通过用户id请求用户信息
public function doPageUserInfo()
{
global $_W, $_GPC;
$res = pdo_get('cjdc_user', array('id' => $_GPC['user_id']));
echo json_encode($res);
}
//分类列表
public function doPageDishesType()
{
global $_W, $_GPC;
$type = pdo_getall('cjdc_type', array('uniacid' => $_W['uniacid'], 'store_id' => $_GPC['store_id'], 'is_open' => 1), array(), '', 'order_by ASC');
// $list=pdo_getall('cjdc_goods',array('uniacid'=>$_W['uniacid'],'is_show'=>1,'type='=>$_GPC['type'],'store_id'=>$_GPC['id']),array(),'','sorting ASC');
$data = array();
for ($i = 0; $i < count($type); $i++) {
$list = pdo_getall('cjdc_goods', array('uniacid' => $_W['uniacid'], 'is_show' => 1, 'type !=' => $_GPC['type'], 'type_id' => $type[$i]['id'], 'store_id' => $_GPC['store_id']), array(), '', 'num ASC');
if ($list) {
$data[] = array(
'id' => $type[$i]['id'],
'type_name' => $type[$i]['type_name'],
'good' => array()
);
}
}
echo json_encode($data);
}
//分类下菜品
public function doPageDishes()
{
global $_W, $_GPC;
$res = pdo_getall('cjdc_goods', array('type_id' => $_GPC['type_id'], 'is_show' => 1, 'type !=' => $_GPC['type']), array(), '', array('num asc'));
echo json_encode($res);
}
//菜品列表
public function doPageDishesList()
{
global $_W, $_GPC;
// $type = pdo_getall('cjdc_type', array('uniacid' => $_W['uniacid'], 'store_id' => $_GPC['store_id'], 'is_open' => 1), array(), '', 'order_by ASC');
$sql = " select * from" . tablename('cjdc_type') . " where uniacid={$_W['uniacid']} and store_id={$_GPC['store_id']} and is_open=1 and id in(select type_id from" . tablename('cjdc_goods') . " where uniacid={$_W['uniacid']} and is_show=1 and type !={$_GPC['type']} and store_id={$_GPC['store_id']}) order by order_by asc";
$type = pdo_fetchall($sql);
$list = pdo_getall('cjdc_goods', array('uniacid' => $_W['uniacid'], 'is_show' => 1, 'type !=' => $_GPC['type'], 'store_id' => $_GPC['store_id']), array(), '', 'num ASC');
$data2 = array();
for ($i = 0; $i < count($type); $i++) {
$data = array();
for ($k = 0; $k < count($list); $k++) {
if ($type[$i]['id'] == $list[$k]['type_id']) {
$data[] = $list[$k];
}
}
$data2[] = array('id' => $type[$i]['id'], 'type_name' => $type[$i]['type_name'], 'good' => $data);
}
echo json_encode($data2);
}
//商品详情
public function doPageGoodInfo()
{
global $_W, $_GPC;
$good = pdo_get('cjdc_goods', array('id' => $_GPC['good_id'], 'uniacid' => $_W['uniacid']));
$spec = pdo_getall('cjdc_spec', array('good_id' => $_GPC['good_id'], 'uniacid' => $_W['uniacid']), array(), '', 'num asc');
$specval = pdo_getall('cjdc_spec_val', array('uniacid' => $_W['uniacid']), array(), '', 'num asc');
$data2 = array();
for ($i = 0; $i < count($spec); $i++) {
$data = array();
for ($k = 0; $k < count($specval); $k++) {
if ($spec[$i]['id'] == $specval[$k]['spec_id']) {
$data[] = array(
'spec_val_id' => $specval[$k]['id'],
'spec_val_name' => $specval[$k]['name'],
'spec_val_num' => $specval[$k]['num']
);
}
}
$data2[] = array(
'spec_id' => $spec[$i]['id'],
'spec_name' => $spec[$i]['name'],
'spec_num' => $spec[$i]['num'],
'spec_val' => $data
);
}
$good['spec'] = $data2;
echo json_encode($good);
}
//规格组合
public function doPageGgZh()
{
global $_W, $_GPC;
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
经导师精心指导并认可、获 98 分的毕业设计项目!【项目资源】:微信小程序。【项目说明】:聚焦计算机相关专业毕设及实战操练,可作课程设计与期末大作业,含全部源码,能直用于毕设,经严格调试,运行有保障!【项目服务】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。 经导师精心指导并认可、获 98 分的毕业设计项目!【项目资源】:微信小程序。【项目说明】:聚焦计算机相关专业毕设及实战操练,可作课程设计与期末大作业,含全部源码,能直用于毕设,经严格调试,运行有保障!【项目服务】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。 经导师精心指导并认可、获 98 分的毕业设计项目!【项目资源】:微信小程序。【项目说明】:聚焦计算机相关专业毕设及实战操练,可作课程设计与期末大作业,含全部源码,能直用于毕设,经严格调试,运行有保障!【项目服务】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。 经导师精心指导并认可、获 98 分的毕业设计项目!【项目资源】:微信小程序。【项目说明】:聚焦计算机相关专业毕设及实战操练,可作课程设计与期末大作业,含全部源码,能直用于毕设,经严格调试,运行有保障!【项目服务】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。
资源推荐
资源详情
资源评论
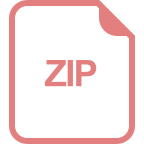
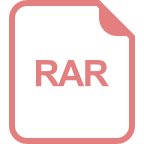
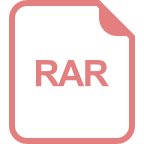
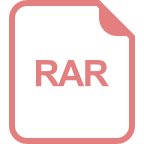
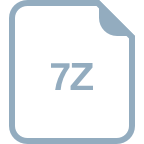
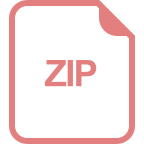
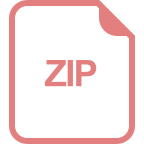
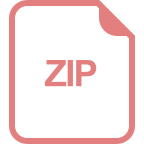
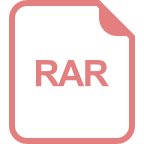
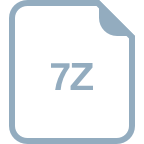
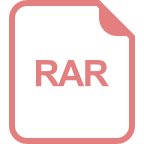
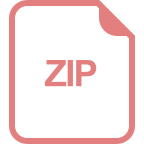
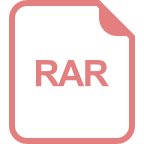
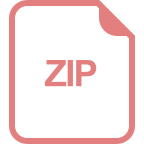
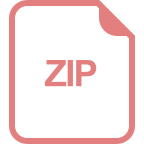
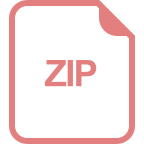
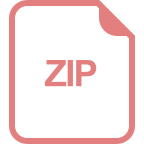
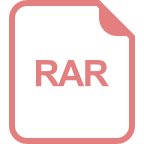
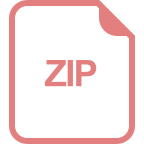
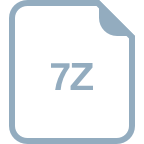
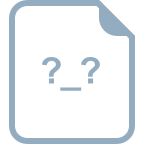
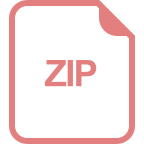
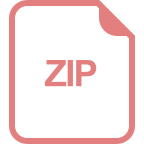
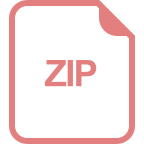
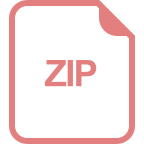
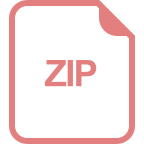
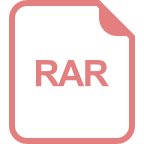
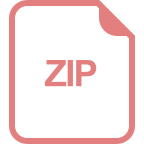
收起资源包目录

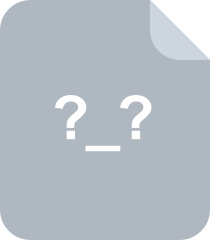
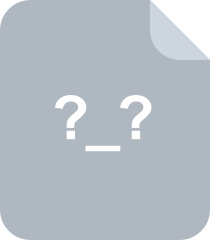
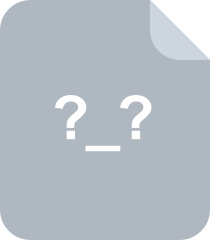
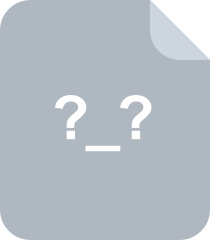
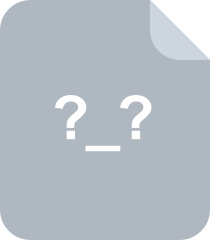
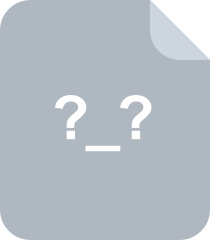
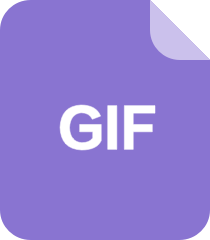
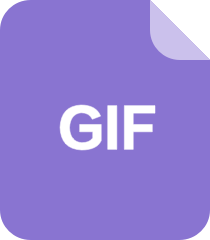
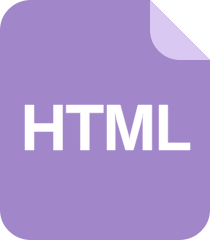
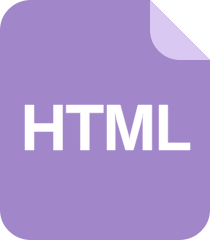
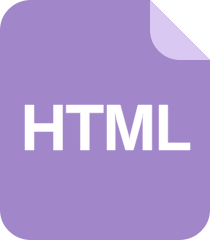
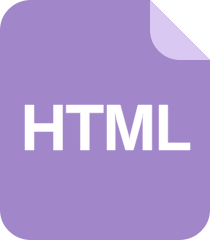
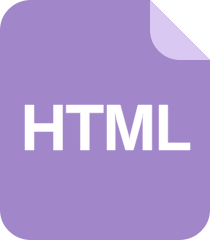
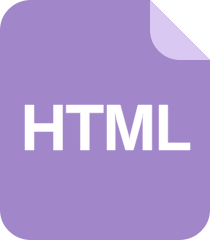
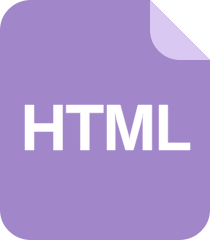
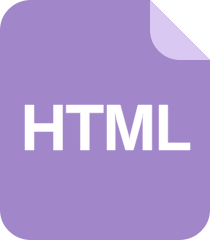
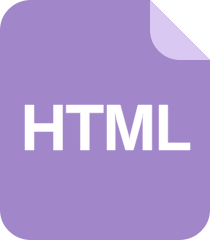
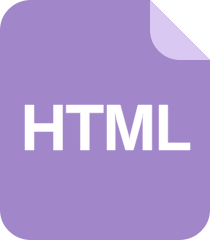
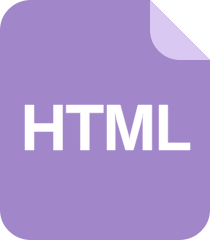
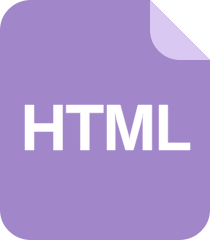
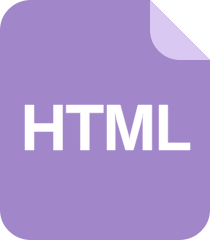
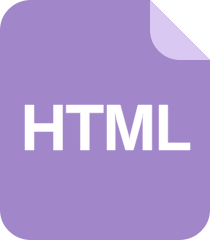
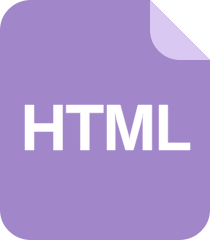
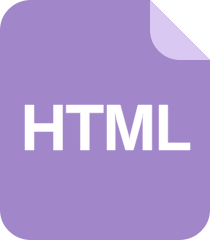
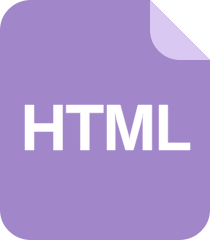
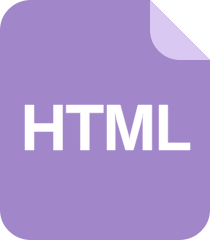
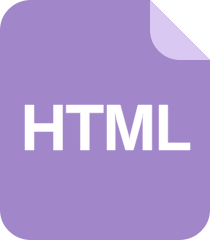
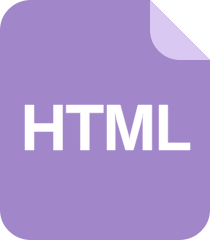
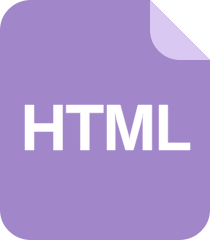
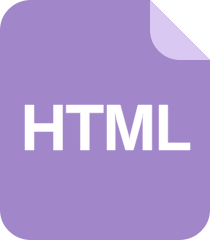
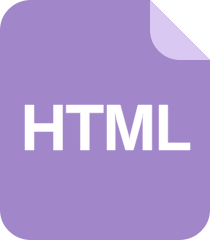
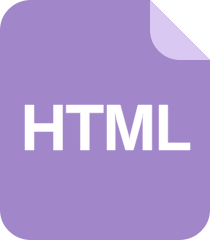
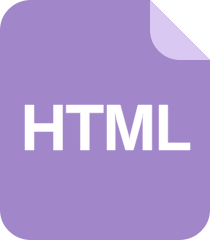
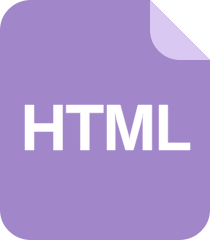
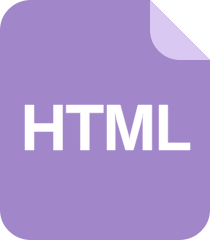
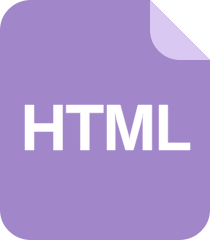
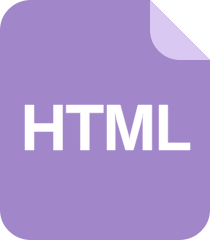
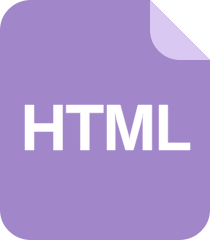
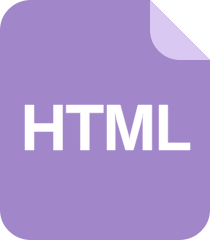
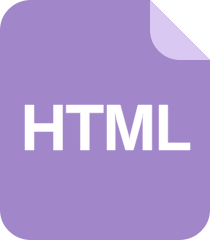
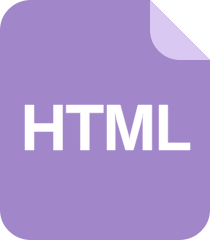
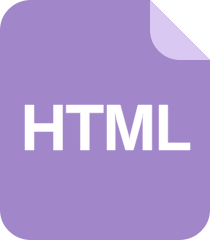
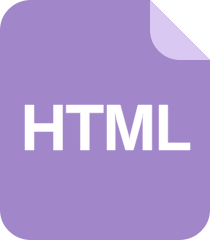
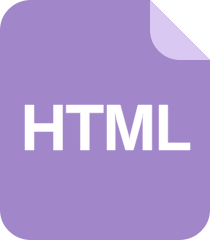
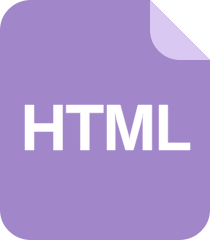
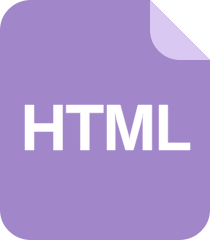
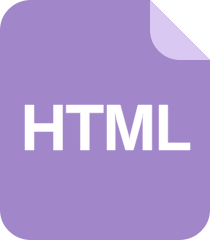
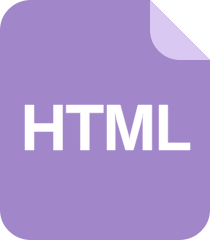
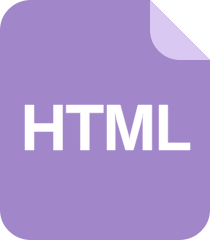
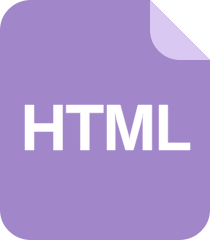
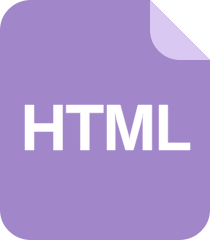
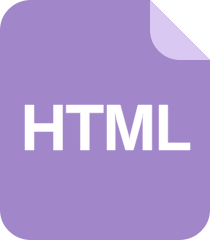
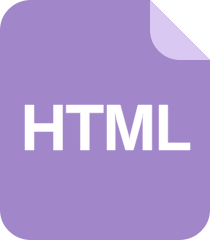
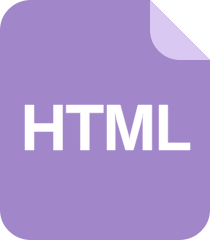
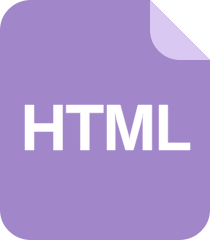
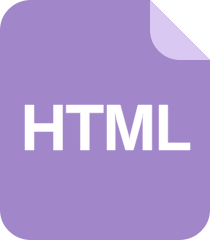
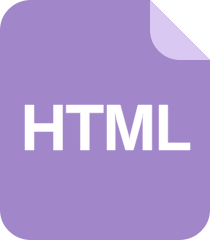
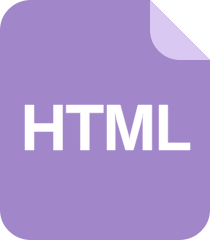
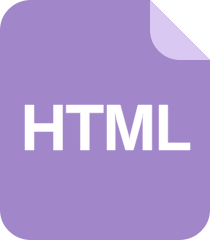
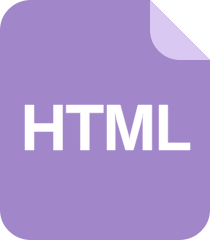
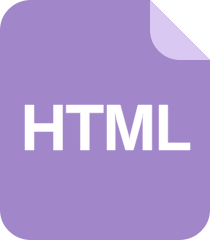
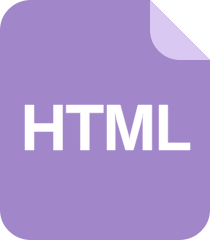
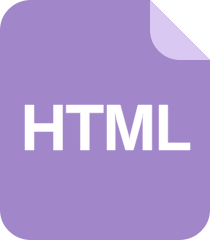
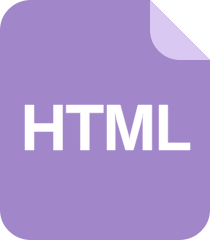
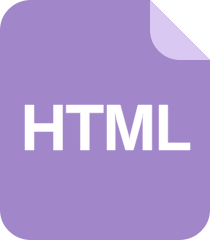
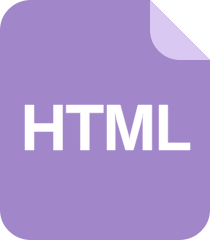
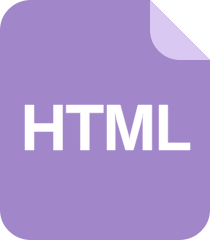
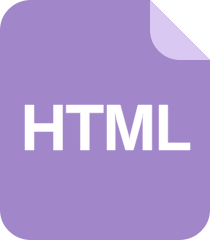
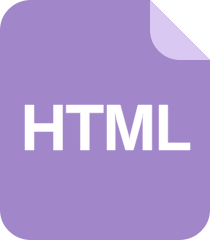
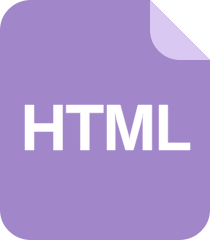
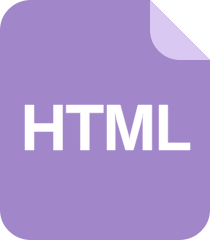
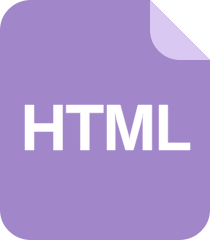
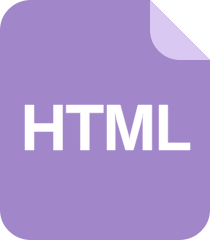
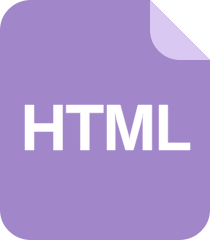
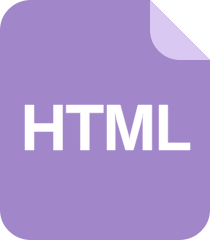
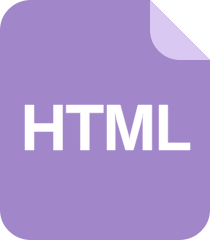
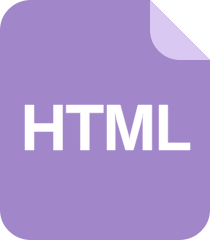
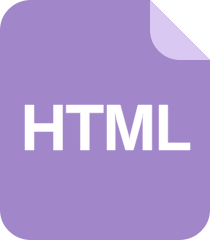
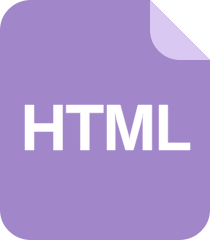
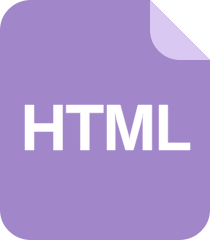
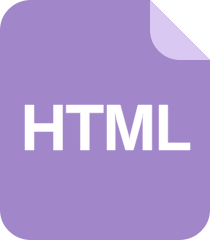
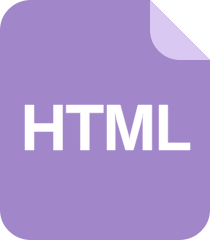
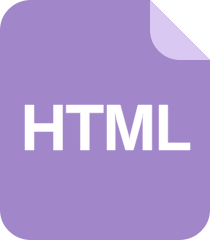
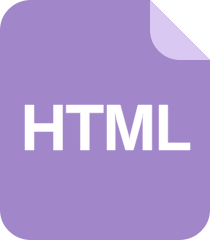
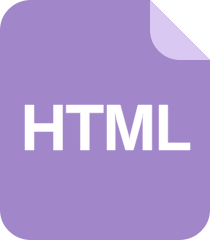
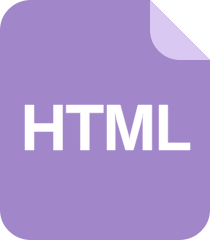
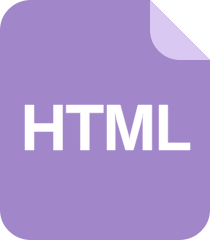
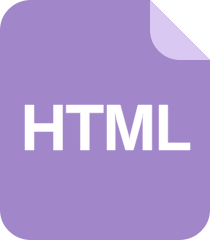
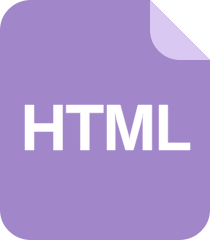
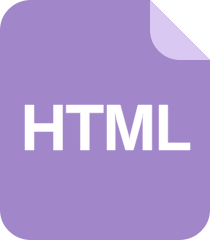
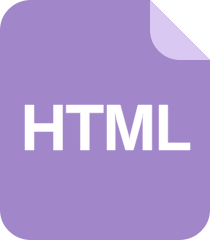
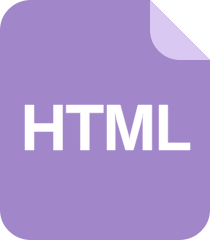
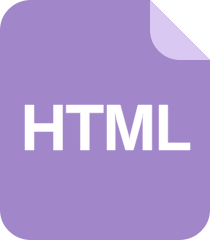
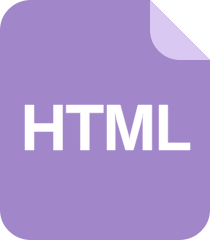
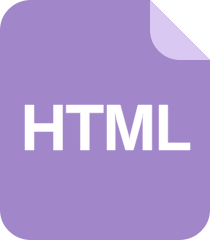
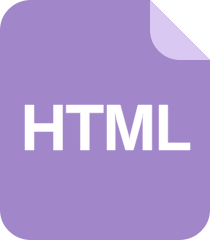
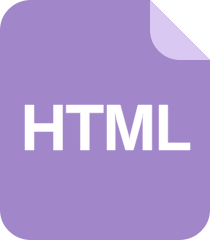
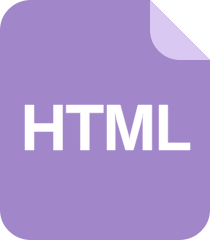
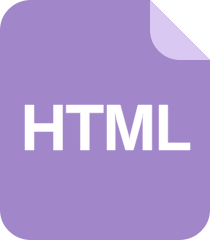
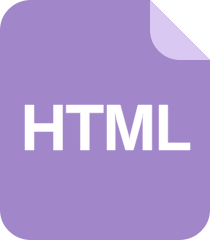
共 1716 条
- 1
- 2
- 3
- 4
- 5
- 6
- 18
资源评论
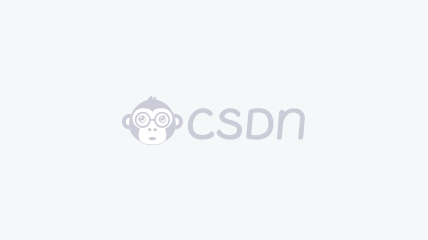


AICurator
- 粉丝: 8683
- 资源: 469
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

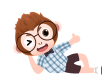
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


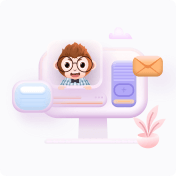
安全验证
文档复制为VIP权益,开通VIP直接复制
