#include "speechManager.h"
//构造函数,
speechManager::speechManager() {
//初始化属性
this->initSpeech();
//创建选手
this->createSpeaker();
//加载往届记录
this->loadRecord();
}
//菜单
void speechManager::show_Menu() {
cout << "*****************************************" << endl;
cout << "*********** 欢迎参加演讲比赛 ************" << endl;
cout << "*********** 1.开始演讲比赛 *************" << endl;
cout << "*********** 2.查看往届记录 *************" << endl;
cout << "*********** 3.清空比赛记录 *************" << endl;
cout << "*********** 0.退出比赛程序 *************" << endl;
cout << "*****************************************" << endl << endl;
}
//退出系统
void speechManager::exitSystem() {
cout << "欢迎下次使用" << endl;
system("pause");
exit(0);
}
//初始化属性
void speechManager::initSpeech() {
//保证容器为空
this->v1.clear();
this->v2.clear();
this->victor.clear();
this->m_speaker.clear();
//初始化比赛轮数
this->m_Index = 1;
//初始化记录容器(在查看往届记录过程中,临时记录)
this->m_Record.clear();
}
//创建选手
void speechManager::createSpeaker() {
string nameSeed = "ABCDEFGHIJKL";
speaker sp;//创建具体选手
for (int i = 0; i < 12; i++) {
string name = "选手";//若定义在循环外,则nameSeed[i]会累加
name += nameSeed[i];
//选手姓名成绩赋值
sp.m_name = name;
for (int i = 0; i < 2; i++)
sp.m_score[i] = 0;
//12名选手编号 放入容器
this->v1.push_back(i + 10001);
//编号及对应选手姓名成绩 放入容器
this->m_speaker.insert(make_pair(i + 10001, sp));
}
}
//显示选手编号v1,v2
void speechManager::show_number(vector<int>&v) {
vector<int>::iterator it = v.begin();
for (; it != v.end(); it++)
cout << *it << " ";
cout << endl;
}
//显示选手信息
void speechManager::show_Speaker() {
map<int, speaker>::iterator it = this->m_speaker.begin();
for (; it != this->m_speaker.end(); it++)
cout << "选手编号:" << it->first << " "
<< "姓名:" << it->second.m_name << " "
<< "成绩:" << it->second.m_score[0] << endl;
}
//开始比赛
void speechManager::startSpeech()
{
//第一轮比赛
//1、抽签
speechDraw();
//2、比赛
speechContest();
//3、显示晋级结果
showScore();
//第二轮比赛
this->m_Index++;
//1、抽签
speechDraw();
//2、比赛
speechContest();
//3、显示最终结果
showScore();
//4、保存分数
saveRecord();
//下面这三行,内容和构造函数一样,但不能把构造函数写这里
//重置初始化数据:更新本届比赛到历史记录,创建新选手
//初始化属性
this->initSpeech();
//创建选手(为下一届创建新选手)
this->createSpeaker();
//加载往届记录(本届比赛记录,实时更新)
this->loadRecord();
cout << "本届比赛完毕!" << endl;
system("pause");
system("cls");
}
//抽签
void speechManager::speechDraw() {
cout << "第<< " << this->m_Index << " >> 轮选手比赛抽签" << endl;
cout << "----------------------------------------" << endl;
cout << "抽签后演讲顺序如下:" << endl;
if (this->m_Index == 1) {
random_shuffle(this->v1.begin(),this->v1.end());
show_number(v1);
}
else {
random_shuffle(this->v2.begin(), this->v2.end());
show_number(v2);
}
cout << "----------------------------------------" << endl;
system("pause");
cout << endl;
}
//比赛
void speechManager::speechContest() {
cout << "---------------第" << this->m_Index << "轮比赛正式开始:-------------" << endl;
multimap<double, int, greater<int>> groupScore;//临时容器,记录每组6人成绩;key成绩,value编号,按成绩降序排列
int num = 0;//记录人员数 每6人一组
vector<int> v;//比赛人员容器
if (this->m_Index == 1)
v = v1;
if (this->m_Index == 2)
v = v2;
//遍历所有参赛选手
for (vector<int>::iterator it = v.begin(); it != v.end(); it++) {
num++;
//10名评委打分
deque<double>d;
for (int i = 0; i < 10; i++) {
double score = (rand() % 401 + 600) / 10.f;//600~1000分,除以10.f得到60~100的浮点分数
d.push_back(score);
}
//排序,去掉一个最低分,去掉一个最高分
sort(d.begin(), d.end(), greater<double>());
d.pop_back();
d.pop_front();
//取平均分
double sum = accumulate(d.begin(),d.end(),0.0f);
double average = sum / d.size();
//成绩和对应选手存入speaker
this->m_speaker[*it].m_score[this->m_Index - 1] = average;
//6人一组用临时容器存储
groupScore.insert(make_pair(average,*it));
if (num % 6 == 0) {
cout << "第" << num / 6 << "小组比赛名次:" << endl;
multimap<double, int, greater<int>>::iterator it = groupScore.begin();
for (; it != groupScore.end(); it++) {
cout << "编号:" << it->second << " " << "姓名:" << this->m_speaker[it->second].m_name << " "
//<< "成绩:" << it->first << endl;//不对
<< "成绩:" << this->m_speaker[it->second].m_score[this->m_Index - 1]<<endl;
}
int count = 0;
//取前三名
it = groupScore.begin();
for (; it != groupScore.end() && count < 3; it++, count++) {
if (this->m_Index == 1)
v2.push_back((*it).second);//第一轮每组前三名晋级
else {
victor.push_back((*it).second);//第二轮,胜出前三名
}
}
groupScore.clear();
cout << endl;
}
}
cout << "---------------第" << this->m_Index << "轮比赛结束:-------------" << endl;
system("pause");
}
//显示比赛分数
void speechManager::showScore() {
cout << "\n---------第" << this->m_Index << "轮晋级选手信息如下:-----------" << endl;
vector<int>v;
if (this->m_Index == 1)
v = v2;
else
v = victor;
vector<int>::iterator it = v.begin();
for (; it != v.end(); it++) {
cout << "编号:" << *it << " 姓名:" << this->m_speaker[*it].m_name << " 得分:" << this->m_speaker[*it].m_score[this->m_Index - 1] << endl;
}
cout << endl;
system("pause");
system("cls");
this->show_Menu();//进入第二轮时,显示菜单信息
}
//保存记录
void speechManager::saveRecord() {
ofstream ofs;//#include<fstream>
//.csv模式 可以excel和记事本打开,,,注意:这里是按位或,不是逻辑或,刚开始写成逻辑或,文件下都创建不了ios::out | ios::app
ofs.open("speech.csv",ios::out | ios::app);//输出方式打开文件,写模式或追加写模式
vector<int>::iterator it = this->victor.begin();
for (; it != this->victor.end(); it++) {
//Excel以逗号分格,逗号另一个作用在读取往届记录时,以逗号分割字符串
ofs << *it << "," << this->m_speaker[*it].m_name<<","<< m_speaker[*it].m_score[1] << ",";
}
ofs << endl;
ofs.close();
cout << "记录已保存 " << endl;
//更改文件不为空标志
this->fileIsEmpty = false;
}
//读取记录
void speechManager::loadRecord() {
ifstream ifs("speech.csv",ios::in);//输入流对象 读取文件
//文件不存在
if (!ifs.is_open()) {
this->fileIsEmpty = true;
cout << "文件不存在!" << endl;
ifs.close();
return;
}
//文件存在,但为空
char ch;
ifs >> ch;
if (ifs.eof()) {//文件结尾 eof ,end of file,"文件结束"
//cout << "文件为空 !" << endl;
this->fileIsEmpty = true;
ifs.close();
return;
}
//文件存在,且不为空
this->fileIsEmpty = false;
ifs.putback(ch);//若文件不为空,将刚才读走的字符再放回来
string data;
int index = 0;//记录第几届
while (ifs >> data) {
vector<string> v;
int pos = -1;
int start = 0;
//cout << data << endl;//记录输出测试
while (true) {
pos = data.find(",",start);//从下标0开始查找","
//若没找到,退出while;;;字符串全部分割完毕,退出while
if (pos == -1)
break;
//若找到,进行分割
string temp = data.substr(start,pos - start);
v.push_back(temp);
start = pos + 1;
}
this->m_Record.insert(make_pair(index, v));//index届数,v存储每一届3名获胜者信息
index++;//届数加1
}
ifs.close();
////测试
//map<int, vector<string>>::iterator it = this->m_Record.begin();
//for (; it != this->m_Record.end(); it++) {
// cout << "第" << it->first + 1 << "届最终获胜前三名: " << endl
// << it->second[0] << " " << it->second[1] << " " << it->second[2] << endl
// << it->second[3] << " " << it->second[4] << " " << it->second[5] << endl
// << it-
没有合适的资源?快使用搜索试试~ 我知道了~
C++ 基于STL的演讲比赛管理系统 工程源码+详细注释
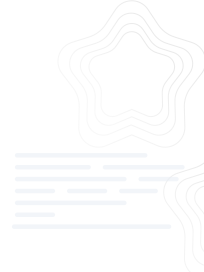
共53个文件
tlog:12个
ipch:5个
pdb:4个

需积分: 9 0 下载量 33 浏览量
2022-11-02
15:16:15
上传
评论
收藏 51.46MB ZIP 举报
温馨提示
C++ 演讲比赛管理系统 STL泛型编程 工程源码+详细注释; 1 比赛规则 ·学校举行一场演讲比赛,共有12个人参加。比赛共两轮,第一轮为淘汰赛,第二轮为决赛。 ·比赛方式:分组比赛,每组6个人;选手每次要随机分组,进行比赛; ·每名选手都有对应的编号,如 10001 ~ 10012; ·第一轮分为两个小组,每组6个人。 整体按照选手编号进行抽签后顺序演讲。 ·当小组演讲完后,淘汰组内排名最后的三个选手,前三名晋级,进入下一轮的比赛。 ·第二轮为决赛,前三名胜出; ·每轮比赛过后需要显示晋级选手的信息; 2 程序功能 ·开始演讲比赛:完成整届比赛的流程,每个比赛阶段需要给用户一个提示,用户按任意键后继续下一个阶段; ·查看往届记录:查看之前比赛前三名结果,每次比赛都会记录到文件中,文件用.csv后缀名保存(.csv可用Excel和记事本打开); ·清空比赛记录:将文件中数据清空; ·退出比赛程序:可以退出当前程序;
资源详情
资源评论
资源推荐
收起资源包目录




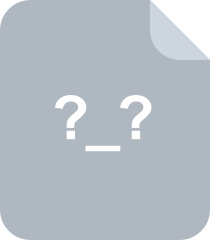

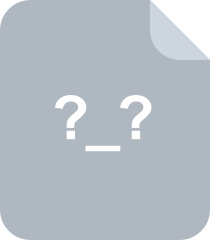
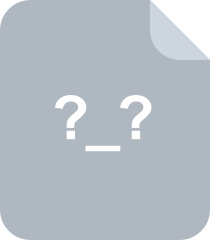
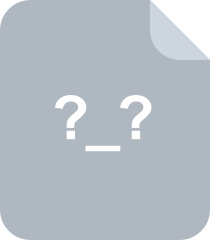
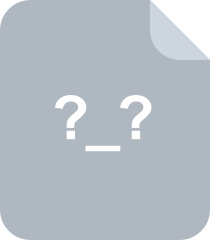

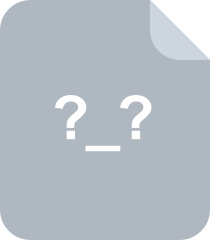
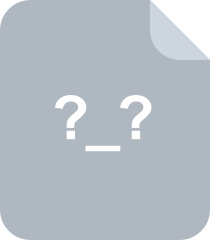



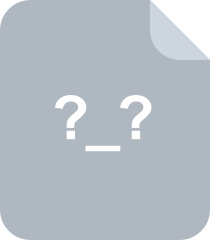


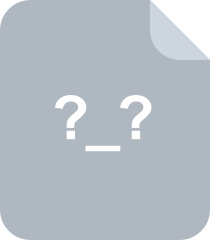

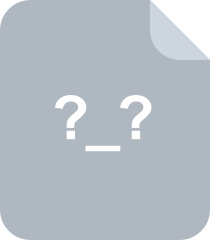

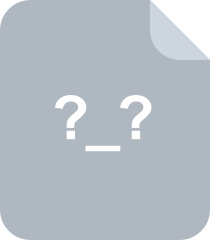

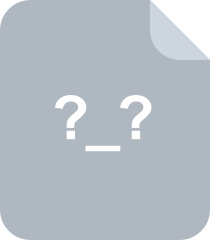

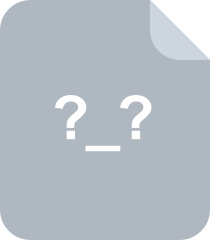
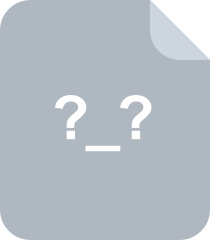
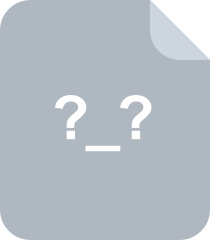
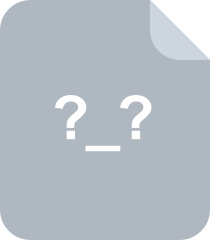
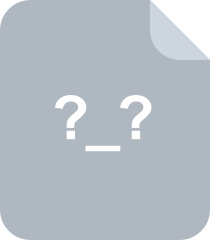


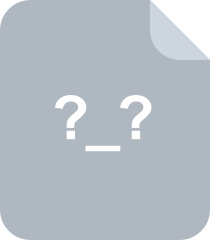
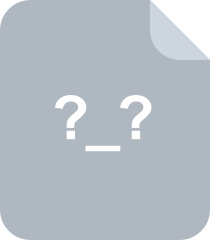
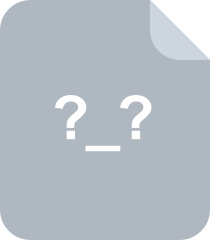
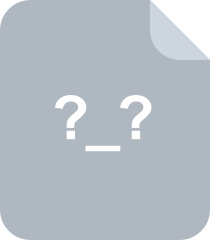
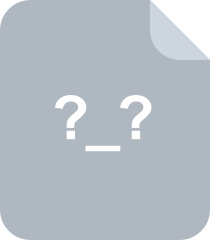

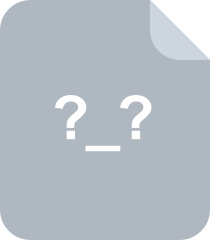
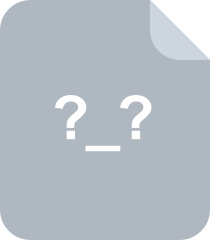
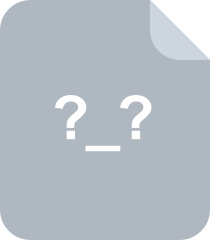
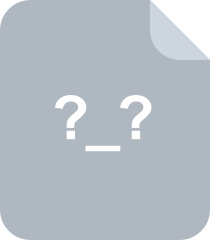
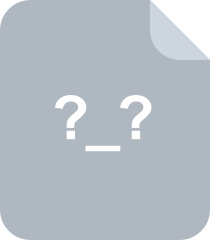
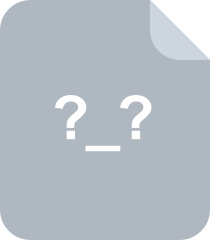
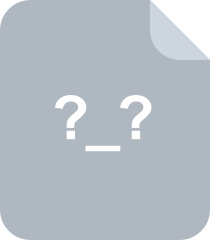
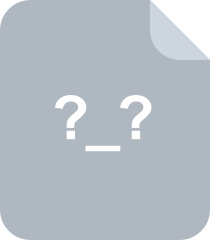
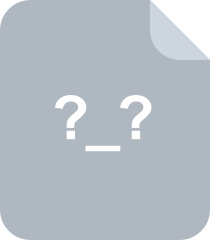

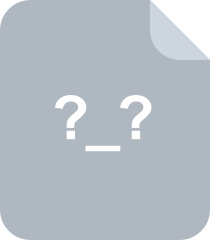
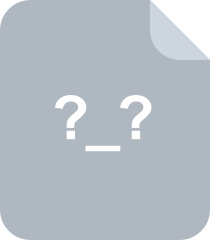
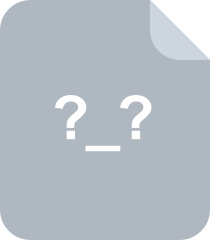
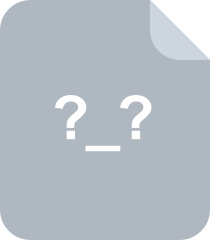
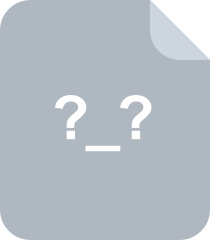
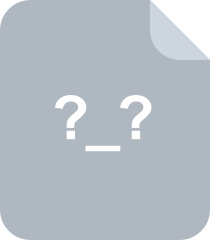
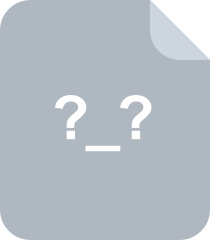

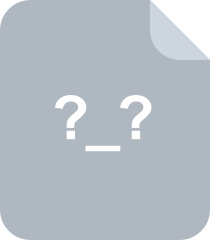
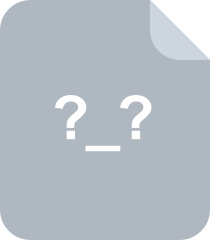
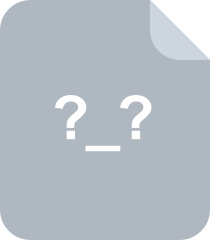
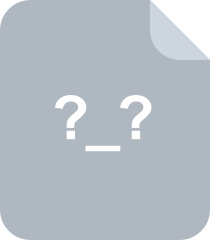
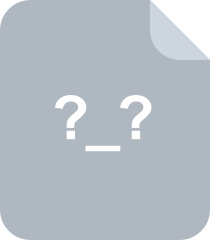
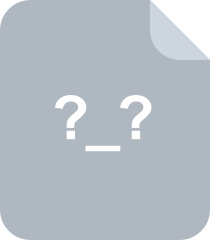
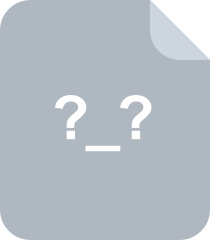
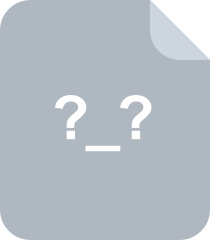
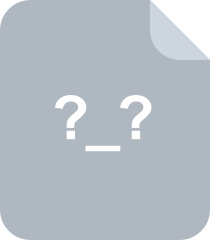
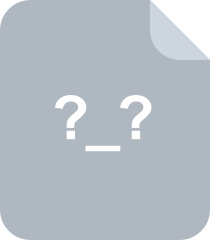


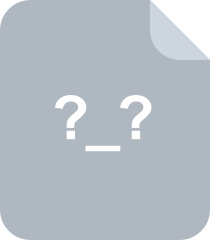
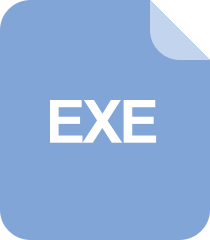

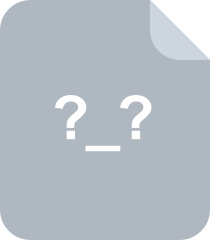
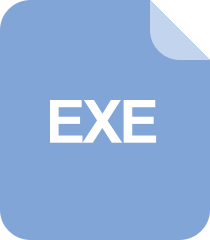
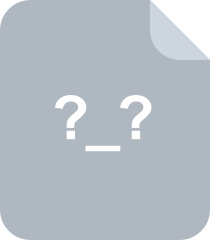
共 53 条
- 1
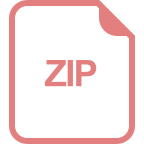
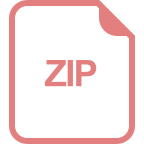
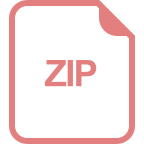
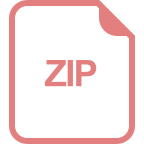
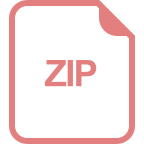
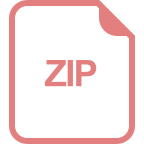
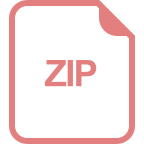
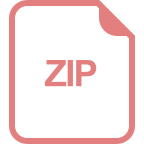
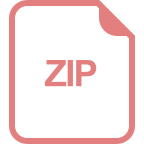
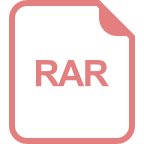
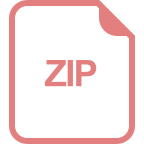
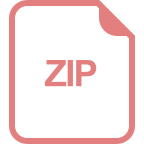
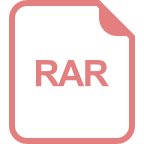
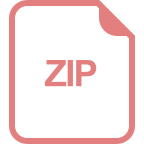
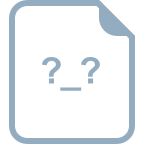
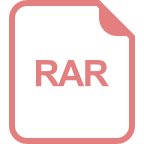
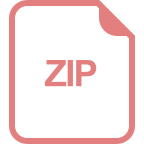
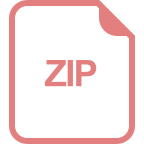
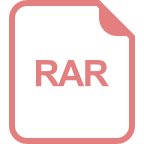
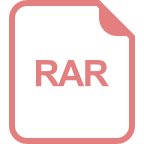
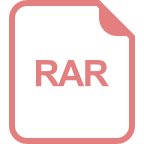
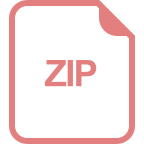
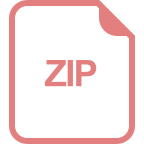
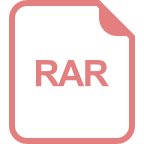
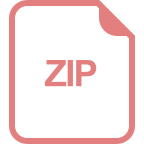
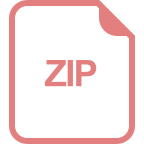

R-G-B
- 粉丝: 1775
- 资源: 114
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

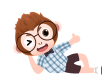
最新资源
- asm-西电微机原理实验
- Arduino-arduino
- C语言-leetcode题解之70-climbing-stairs.c
- C语言-leetcode题解之68-text-justification.c
- C语言-leetcode题解之66-plus-one.c
- C语言-leetcode题解之64-minimum-path-sum.c
- C语言-leetcode题解之63-unique-paths-ii.c
- C语言-leetcode题解之62-unique-paths.c
- C语言-leetcode题解之61-rotate-list.c
- C语言-leetcode题解之59-spiral-matrix-ii.c
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


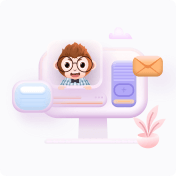
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0