package stream;
import entity.Author;
import entity.Book;
import java.util.*;
import java.util.function.Supplier;
import java.util.stream.Collectors;
public class StreamDem01 {
public static void main(String[] args) {
//打印所有姓名长度大于1的作家
//test01();
//打印所有作家的名字
//test02();
//去除重复作家名称
//test03();
//对流中的元素按照年龄进行降序排列,并且要求不能有重复元素
//test04();
//对流中的元素按照年龄进行降序排列,并且要求不能有重复元素,然后打印其中年龄最大的两个作家的姓名
//test05();
//打印除了年龄最大的作家外的其他作家,要求不能有重复元素,并且按照年龄降序排列
//test06();
//打印所有书籍的名字,要求对重复元素进行去重操作
//test07();
//打印现有数据的所有分类,要求对分类进行去重,不能出现格式:哲学,爱情
//test08();
//打印这些作家的所出书籍的数目,注意删除重复元素
//test09();
//分别获取这些作家所出书籍的最高分和最低分并打印
//test10();
//获取一个存放所有作者名字的List集合
//test11();
//判断是否有年龄在29以上的作家
//test12();
//获取任意一个大于18的作家,如果存在就输出他的名字
//test13();
//获取一个年龄最小的作家,并输出他的姓名
//test14();
//使用reduce求所有作者年龄的和
//test15();
test16();
}
public static void test01(){
List<Author> authors = getAuthors();
//打印所有姓名长度大于1的作家
authors.stream()
.filter(name->name.getName().length()>1)
.forEach(name->System.out.println(name));
}
public static void test02(){
List<Author> authors = getAuthors();
//打印所有作家的名字
authors.stream()
.distinct()
.map(author -> author.getName())
.forEach(name-> System.out.println(name));
}
public static void test03(){
List<Author> authors = getAuthors();
//去除重复作家名称
authors.stream()
.distinct()
.map(Author::getName)
.forEach(System.out::println);
}
public static void test04(){
List<Author> authors = getAuthors();
//对流中的元素按照年龄进行降序排列,并且要求不能有重复元素
// authors.stream()
// .distinct()
// .sorted(new Comparator<Author>() {
// @Override
// public int compare(Author o1, Author o2) {
// return o2.getAge()-o1.getAge();
// }
// })
// .map(author -> author.getAge())
// .forEach(System.out::println);
authors.stream()
.distinct()
.sorted()
.map(author -> author.getAge())
.forEach(System.out::println);
}
public static void test05(){
List<Author> authors = getAuthors();
//对流中的元素按照年龄进行降序排列,并且要求不能有重复元素,然后打印其中年龄最大的两个作家的姓名
authors.stream()
.sorted()
.distinct()
.limit(2)
.map(author -> author.getName())
.forEach(System.out::println);
}
public static void test06(){
List<Author> authors = getAuthors();
//打印除了年龄最大的作家外的其他作家,要求不能有重复元素,并且按照年龄降序排列
authors.stream()
.sorted((o1, o2) -> o2.getAge()-o1.getAge())
.distinct()
.skip(1)
.map(author -> author.getAge())
.forEach(System.out::println);
}
public static void test07(){
List<Author> authors = getAuthors();
//打印所有书籍的名字,要求对重复元素进行去重操作
authors.stream()
.flatMap(author -> author.getBooks().stream())
.distinct()
.forEach(System.out::println);
}
public static void test08(){
List<Author> authors = getAuthors();
//打印现有数据的所有分类,要求对分类进行去重,不能出现格式:哲学,爱情
authors.stream()
.flatMap(author -> author.getBooks().stream())
//.distinct()
.flatMap(book -> Arrays.stream(book.getCategory().split(",")))
.distinct()
.forEach(System.out::println);
}
public static void test09(){
List<Author> authors = getAuthors();
//打印这些作家的所出书籍的数目,注意删除重复元素
long count = authors.stream()
.flatMap(author -> author.getBooks().stream())
.distinct()
.count();
System.out.println(count);
}
public static void test10(){
List<Author> authors = getAuthors();
// //分别获取这些作家所出书籍的最高分和最低分并打印
// Optional<Integer> min = authors.stream()
// .flatMap(book -> book.getBooks().stream())
// .map(book -> book.getScore())
// .min(Comparator.comparingInt(o -> o));
// System.out.println(min.get());
Optional<Integer> max = authors.stream()
.flatMap(book -> book.getBooks().stream())
.map(book -> book.getScore())
.max((o1, o2) -> o1 - o2);
System.out.println(max.get());
}
public static void test11(){
List<Author> authors = getAuthors();
// //获取一个存放所有作者名字的List集合
// List<String> collect = authors.stream()
// .map(author -> author.getName())
// .distinct()
// .collect(Collectors.toList());
// System.out.println(collect.toString());
//获取一个所有书名的Set集合
// Set<String> bookNames = authors.stream()
// .flatMap(author -> author.getBooks().stream())
// .map(book -> book.getName()).distinct()
// .collect(Collectors.toSet());
// System.out.println(bookNames);
//获取一个map集合,map的key为作家名称,value为List<Book>
Map<String, List<Book>> collect = authors.stream()
.distinct()
.collect(Collectors.toMap(author -> author.getName(), author -> author.getBooks()));
List<Author> collect1 = authors.stream()
.distinct()
.collect(Collectors.toList());
for (Author col:collect1
) {
List<Book> books = collect.get(col.getName());
System.out.println(books);
}
}
public static void test12(){
List<Author> authors = getAuthors();
//判断是否有年龄在29以上的作家
boolean match = authors.stream()
.anyMatch(author -> author.getAge() > 29);
System.out.println(match);
}
public static void test13(){
List<Author> authors = getAuthors();
//获取任意一个大于18的作家,如果存在就输出他的名字
Optional<Author> optionalAuthor = authors.stream()
.filter(author -> author.getAge()>13)
.findAny();
optionalAuthor.ifPresent(author -> System.out.println(au
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
在这本《函数式编程教程代码示例》中,您将沉浸于函数式编程的世界,通过一系列精心挑选的代码片段来探索。这些示例展示了函数式编程范式的优雅和威力,是掌握这一变革性软件开发方法的指南。通过深入理解和实践这些示例,您将学会如何利用函数式编程的原则和技巧来构建高效、模块化的程序。这本教程不仅仅是一个代码示例集合,更是一段启发性的旅程,将带领您走向函数式编程的新境界。无论您是新手还是有经验的开发者,都能从中获益,并将函数式编程的优势应用到自己的项目中。让我们一起探索函数式编程的美妙世界吧!
资源推荐
资源详情
资源评论
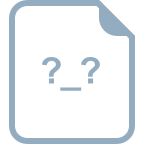
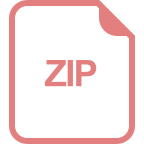
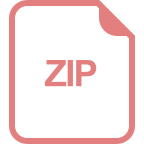
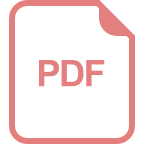
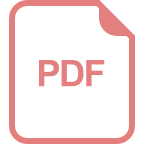
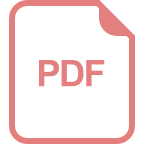
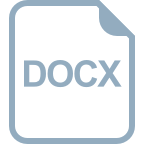
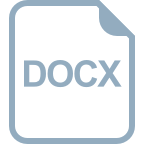
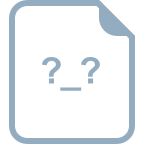
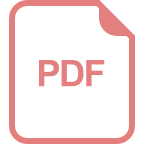
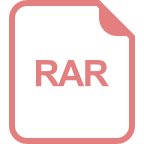
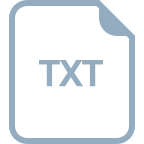
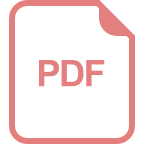
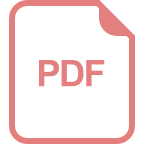
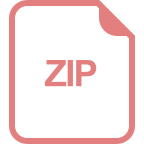
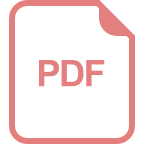
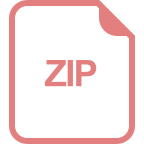
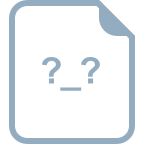
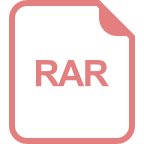
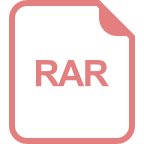
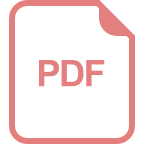
收起资源包目录






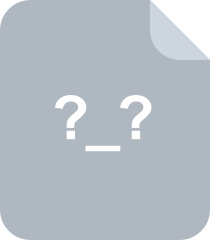
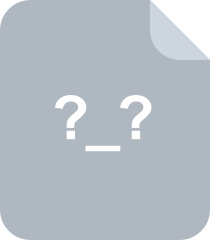
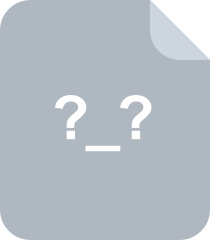

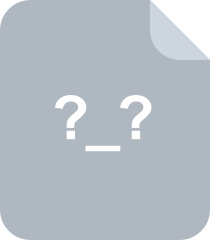


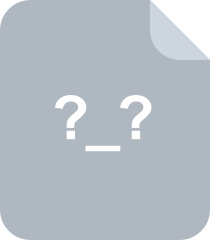
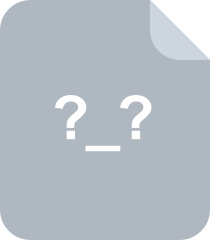
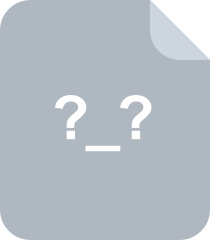
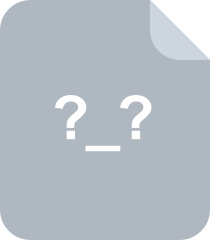
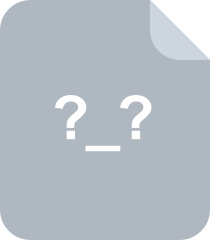
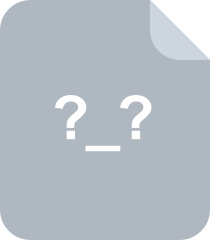
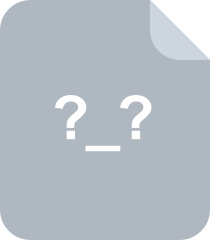
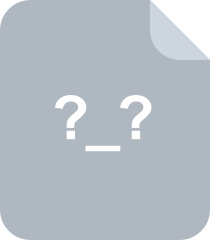
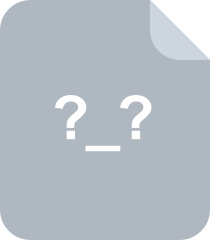
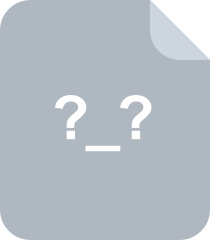
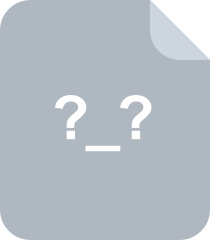
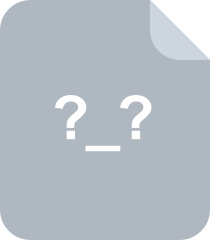
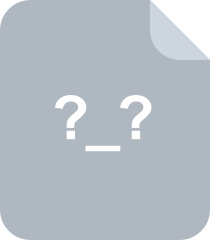
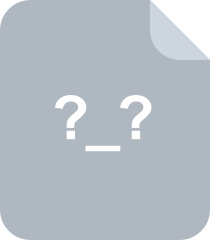
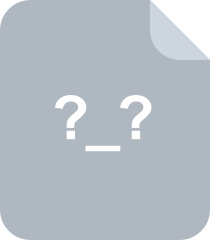

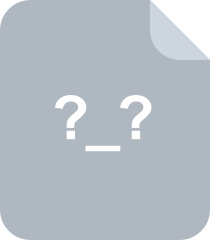
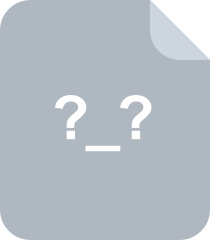
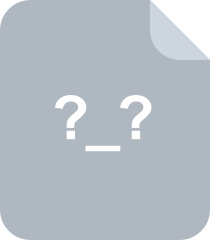
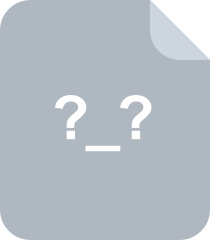
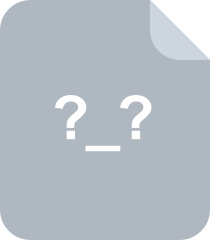
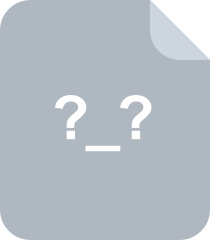

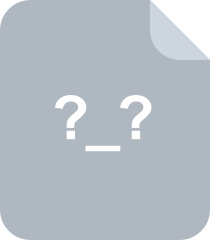
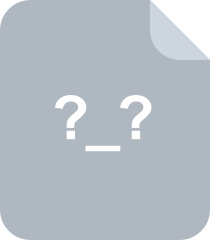

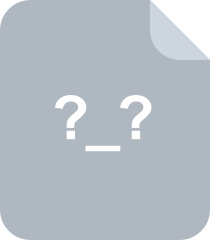
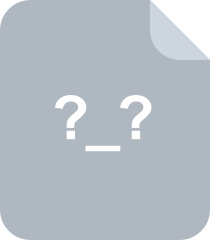
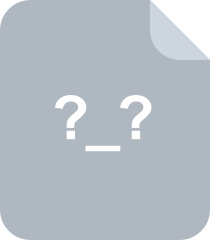
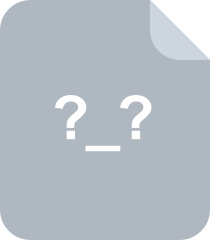
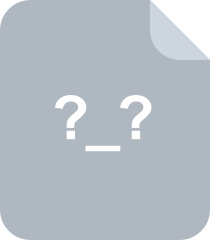
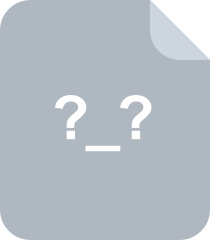
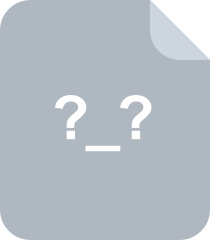



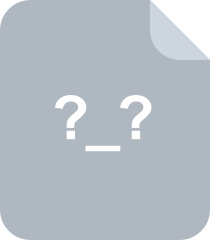
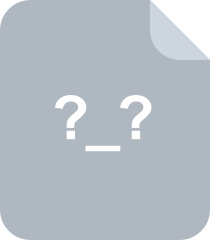
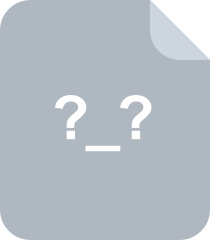

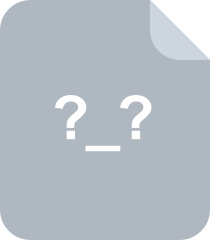

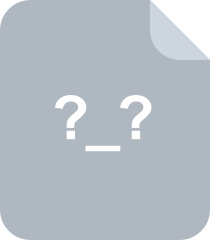
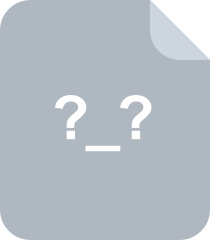
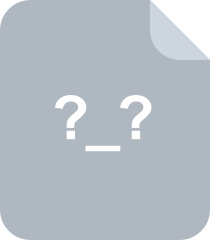
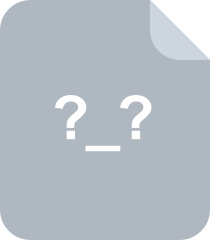
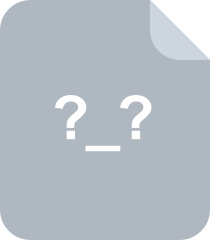
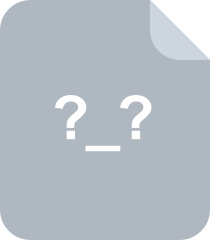
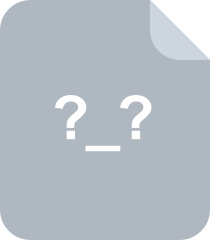
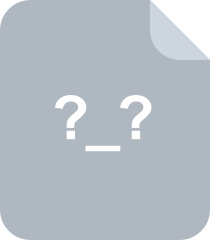
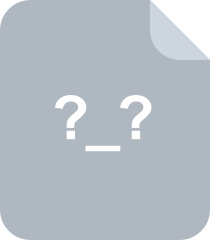
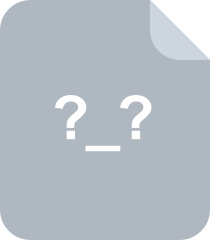
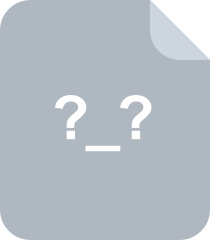
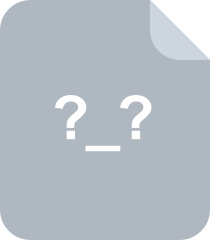
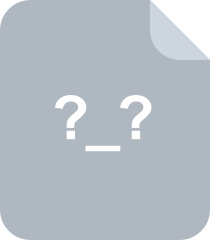

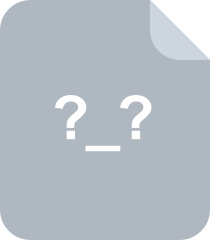
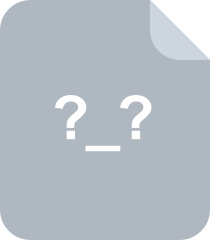
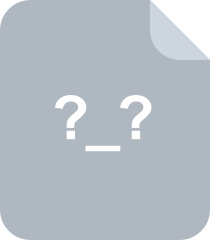
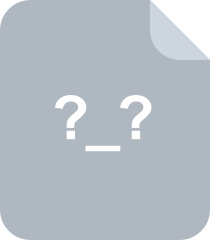
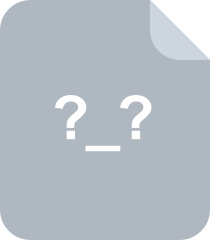
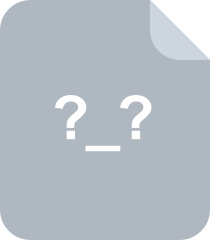

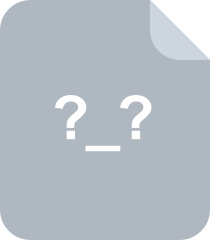
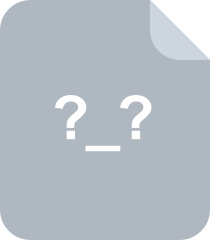
共 59 条
- 1
资源评论
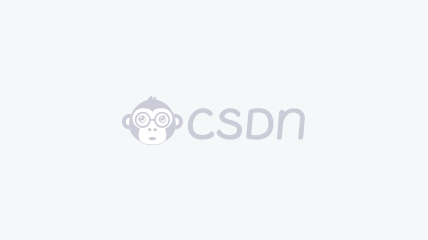

MidnightWhisper
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

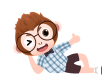
最新资源
- 09MnNiDR钢制低温压力容器焊接工艺的确定.pdf
- 09MnNiDR钢制球罐用W707DRQ焊条焊接性能 - .pdf
- 09MnNiDR低温压力容器钢板的试验及焊接检验.pdf
- 9Ni钢低温储罐焊接工艺研究.pdf
- 9Cr与CrMoV异种焊接接头疲劳裂纹扩展门槛值研究.pdf
- 10CrMo910耐热钢的焊接工艺.pdf
- 10CrMo910炉管焊接.pdf
- 10Ni3MoVD锻件焊接裂纹敏感性试验研究.pdf
- 10t电动单梁桥式起重机主梁焊接变形的控制 - .pdf
- 10CrNi3MoV钢双面双弧焊接头组织与性能研究 - .pdf
- 10焊接工字形钢轴压构件截面设计的直接算法.pdf
- 10吨级叉车驱动桥体焊接工艺设计 - .pdf
- 10T级后桥焊接工艺分析.pdf
- 10万m 3原油储罐底板现场焊接及变形控制.pdf
- 10万m3大型原油储罐底板焊接质量控制分析.pdf
- 10万m3原油储罐典型焊接质量缺陷分析与处理.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


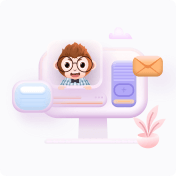
安全验证
文档复制为VIP权益,开通VIP直接复制
