/*
* This file is part of Pimple.
*
* Copyright (c) 2014 Fabien Potencier
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is furnished
* to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
#ifdef HAVE_CONFIG_H
#include "config.h"
#endif
#include "php.h"
#include "php_ini.h"
#include "ext/standard/info.h"
#include "php_pimple.h"
#include "pimple_compat.h"
#include "zend_interfaces.h"
#include "zend.h"
#include "Zend/zend_closures.h"
#include "ext/spl/spl_exceptions.h"
#include "Zend/zend_exceptions.h"
#include "main/php_output.h"
#include "SAPI.h"
static zend_class_entry *pimple_ce_PsrContainerInterface;
static zend_class_entry *pimple_ce_PsrContainerExceptionInterface;
static zend_class_entry *pimple_ce_PsrNotFoundExceptionInterface;
static zend_class_entry *pimple_ce_ExpectedInvokableException;
static zend_class_entry *pimple_ce_FrozenServiceException;
static zend_class_entry *pimple_ce_InvalidServiceIdentifierException;
static zend_class_entry *pimple_ce_UnknownIdentifierException;
static zend_class_entry *pimple_ce;
static zend_object_handlers pimple_object_handlers;
static zend_class_entry *pimple_closure_ce;
static zend_class_entry *pimple_serviceprovider_ce;
static zend_object_handlers pimple_closure_object_handlers;
static zend_internal_function pimple_closure_invoker_function;
#define FETCH_DIM_HANDLERS_VARS pimple_object *pimple_obj = NULL; \
ulong index; \
pimple_obj = (pimple_object *)zend_object_store_get_object(object TSRMLS_CC); \
#define PIMPLE_OBJECT_HANDLE_INHERITANCE_OBJECT_HANDLERS do { \
if (ce != pimple_ce) { \
zend_hash_find(&ce->function_table, ZEND_STRS("offsetget"), (void **)&function); \
if (function->common.scope != ce) { /* if the function is not defined in this actual class */ \
pimple_object_handlers.read_dimension = pimple_object_read_dimension; /* then overwrite the handler to use custom one */ \
} \
zend_hash_find(&ce->function_table, ZEND_STRS("offsetset"), (void **)&function); \
if (function->common.scope != ce) { \
pimple_object_handlers.write_dimension = pimple_object_write_dimension; \
} \
zend_hash_find(&ce->function_table, ZEND_STRS("offsetexists"), (void **)&function); \
if (function->common.scope != ce) { \
pimple_object_handlers.has_dimension = pimple_object_has_dimension; \
} \
zend_hash_find(&ce->function_table, ZEND_STRS("offsetunset"), (void **)&function); \
if (function->common.scope != ce) { \
pimple_object_handlers.unset_dimension = pimple_object_unset_dimension; \
} \
} else { \
pimple_object_handlers.read_dimension = pimple_object_read_dimension; \
pimple_object_handlers.write_dimension = pimple_object_write_dimension; \
pimple_object_handlers.has_dimension = pimple_object_has_dimension; \
pimple_object_handlers.unset_dimension = pimple_object_unset_dimension; \
}\
} while(0);
#define PIMPLE_CALL_CB do { \
zend_fcall_info_argn(&fci TSRMLS_CC, 1, &object); \
fci.size = sizeof(fci); \
fci.object_ptr = retval->fcc.object_ptr; \
fci.function_name = retval->value; \
fci.no_separation = 1; \
fci.retval_ptr_ptr = &retval_ptr_ptr; \
\
zend_call_function(&fci, &retval->fcc TSRMLS_CC); \
efree(fci.params); \
if (EG(exception)) { \
return EG(uninitialized_zval_ptr); \
} \
} while(0);
/* Psr\Container\ContainerInterface */
ZEND_BEGIN_ARG_INFO_EX(arginfo_pimple_PsrContainerInterface_get, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_pimple_PsrContainerInterface_has, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_PsrContainerInterface_functions[] = {
PHP_ABSTRACT_ME(ContainerInterface, get, arginfo_pimple_PsrContainerInterface_get)
PHP_ABSTRACT_ME(ContainerInterface, has, arginfo_pimple_PsrContainerInterface_has)
PHP_FE_END
};
/* Psr\Container\ContainerExceptionInterface */
static const zend_function_entry pimple_ce_PsrContainerExceptionInterface_functions[] = {
PHP_FE_END
};
/* Psr\Container\NotFoundExceptionInterface */
static const zend_function_entry pimple_ce_PsrNotFoundExceptionInterface_functions[] = {
PHP_FE_END
};
/* Pimple\Exception\FrozenServiceException */
ZEND_BEGIN_ARG_INFO_EX(arginfo_FrozenServiceException___construct, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_FrozenServiceException_functions[] = {
PHP_ME(FrozenServiceException, __construct, arginfo_FrozenServiceException___construct, ZEND_ACC_PUBLIC)
PHP_FE_END
};
/* Pimple\Exception\InvalidServiceIdentifierException */
ZEND_BEGIN_ARG_INFO_EX(arginfo_InvalidServiceIdentifierException___construct, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_InvalidServiceIdentifierException_functions[] = {
PHP_ME(InvalidServiceIdentifierException, __construct, arginfo_InvalidServiceIdentifierException___construct, ZEND_ACC_PUBLIC)
PHP_FE_END
};
/* Pimple\Exception\UnknownIdentifierException */
ZEND_BEGIN_ARG_INFO_EX(arginfo_UnknownIdentifierException___construct, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_UnknownIdentifierException_functions[] = {
PHP_ME(UnknownIdentifierException, __construct, arginfo_UnknownIdentifierException___construct, ZEND_ACC_PUBLIC)
PHP_FE_END
};
/* Pimple\Container */
ZEND_BEGIN_ARG_INFO_EX(arginfo___construct, 0, 0, 0)
ZEND_ARG_ARRAY_INFO(0, value, 0)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_offsetset, 0, 0, 2)
ZEND_ARG_INFO(0, offset)
ZEND_ARG_INFO(0, value)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_offsetget, 0, 0, 1)
ZEND_ARG_INFO(0, offset)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_offsetexists, 0, 0, 1)
ZEND_ARG_INFO(0, offset)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_offsetunset, 0, 0, 1)
ZEND_ARG_INFO(0, offset)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_factory, 0, 0, 1)
ZEND_ARG_INFO(0, callable)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_protect, 0, 0, 1)
ZEND_ARG_INFO(0, callable)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_raw, 0, 0, 1)
ZEND_ARG_INFO(0, id)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_extend, 0, 0, 2)
ZEND_ARG_INFO(0, id)
ZEND_ARG_INFO(0, callable)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_keys, 0, 0, 0)
ZEND_END_ARG_INFO()
ZEND_BEGIN_ARG_INFO_EX(arginfo_register, 0, 0, 1)
ZEND_ARG_OBJ_INFO(0, provider, Pimple\\ServiceProviderInterface, 0)
ZEND_ARG_ARRAY_INFO(0, values, 1)
ZEND_END_ARG_INFO()
static const zend_function_entry pimple_ce_functions[] = {
PHP_ME(Pimple, __construct, arginfo___construct, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, factory, arginfo_factory, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, protect, arginfo_protect, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, raw, arginfo_raw, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, extend, arginfo_extend, ZEND_ACC_PUBLIC)
PHP_ME(Pimple, keys, arginfo_keys, ZEND_ACC_PUBLIC)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
梦想贩卖机v2-1.0.67 简单介绍资源价值,让用户产生想要获取的冲动。 资源社群: 付费或者获取资源后方可进群。未来星知识杜郡精准付费运营的时代。每个资酒都可以创速独立的项目交流群,专业的服务和共同的目标人正样,会王加容易让用户产生付费购买的冲动。因为每个项目资源就是一个社群圈子。为知识付费他犹像再加上人脉圈子已经可以完全攻破用户的心理防线。轻松付费成者完成任务 内页展示: 支持挂载小程序跳转、H5跳转、内部跳转、付费进群 支持卡密资源自动发卡、实时展示库存剩余! 支持多种形式得流量主广告,赚取广告展示和点击收益! 支持挂载社群,完成任务或者付费方可进群! 支持百度网盘等附件链接,完成任务或者付费购买方可获得附件查看权限! 所有资源商品支持通过任务获取和付费购买。
资源推荐
资源详情
资源评论
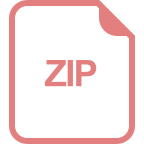
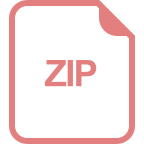
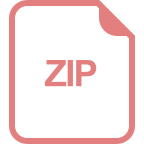
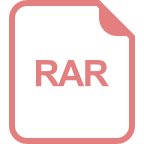
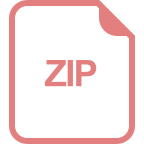
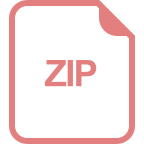
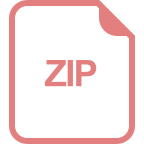
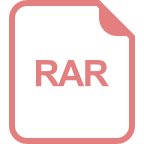
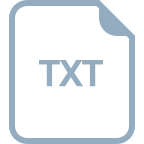
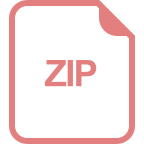
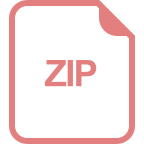
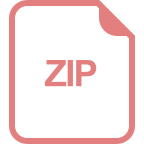
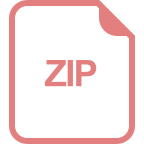
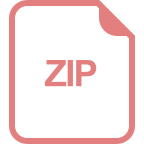
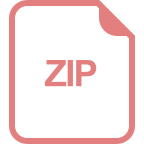
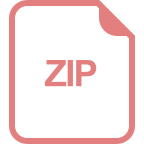
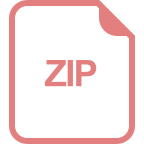
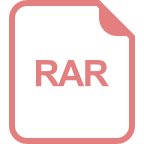
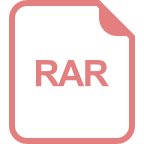
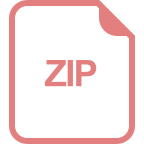
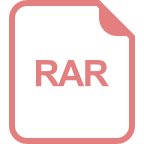
收起资源包目录

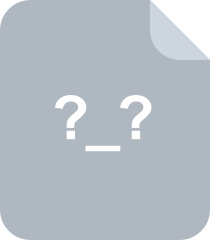
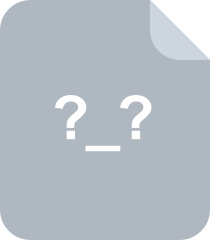
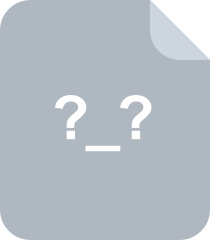
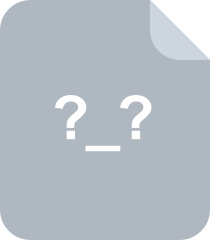
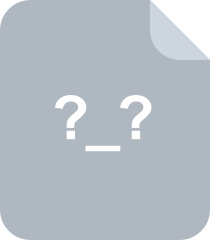
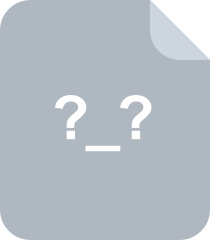
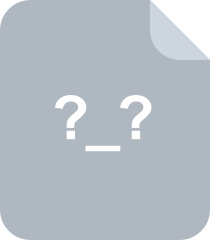
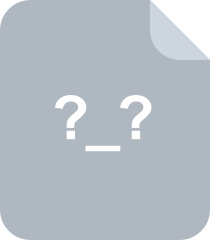
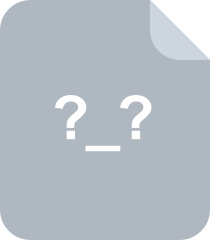
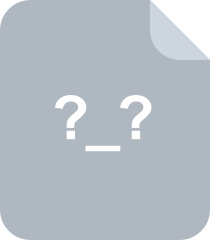
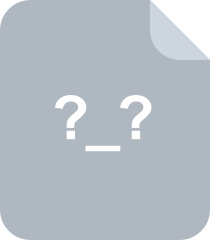
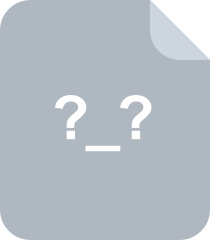
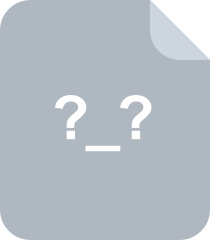
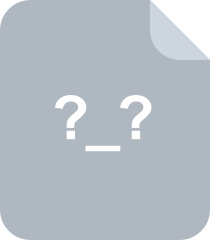
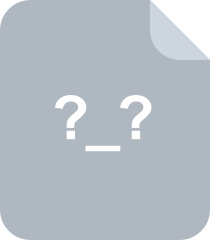
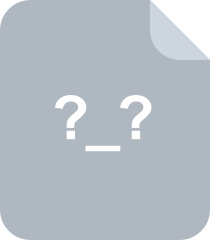
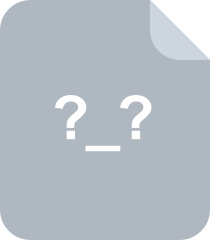
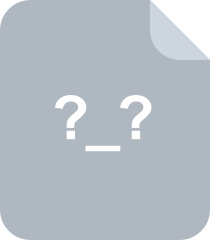
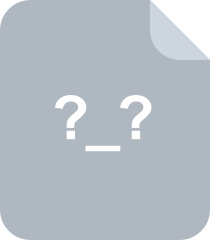
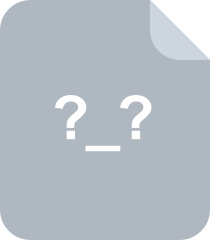
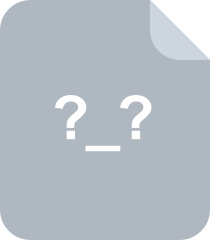
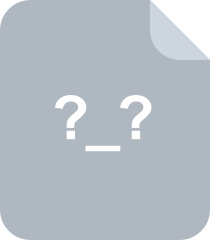
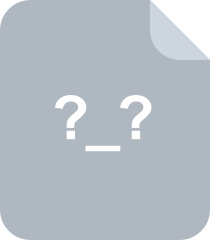
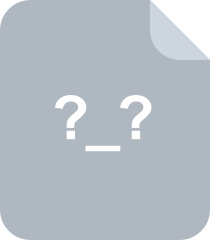
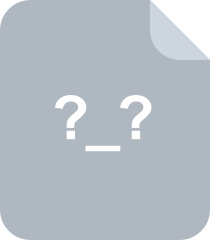
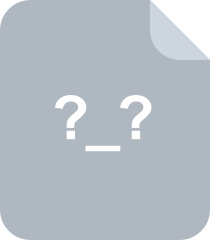
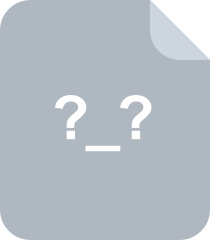
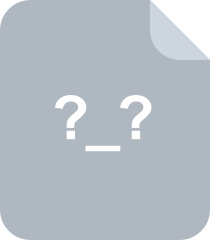
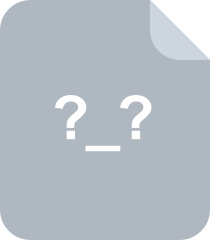
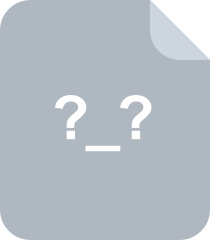
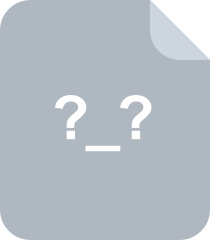
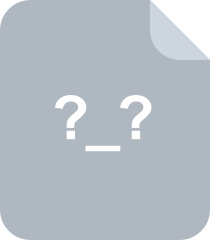
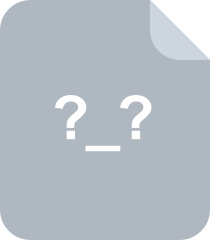
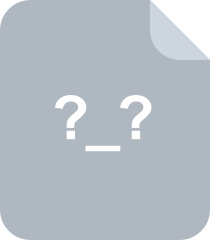
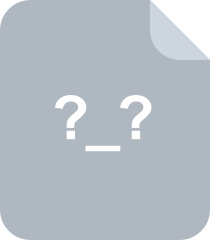
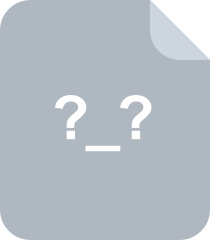
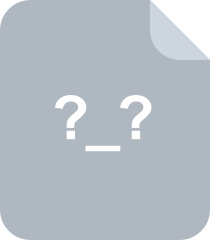
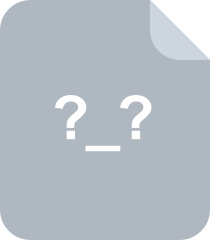
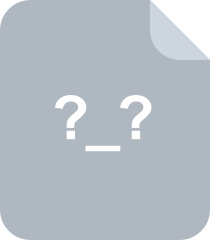
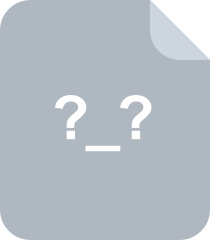
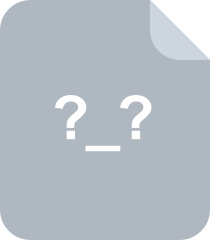
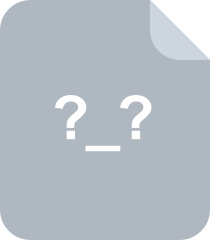
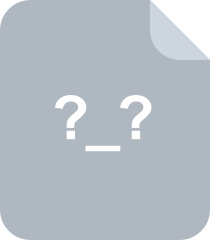
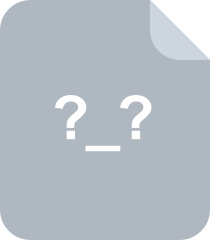
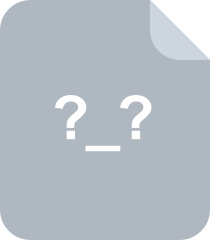
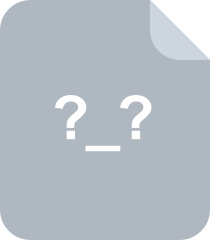
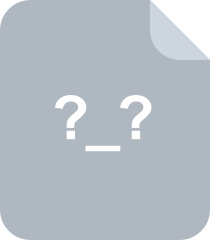
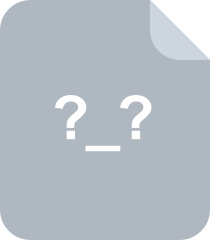
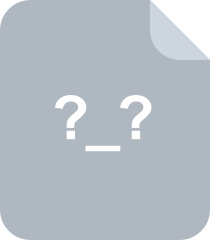
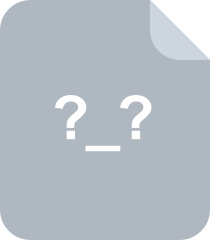
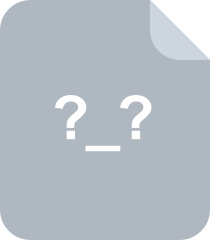
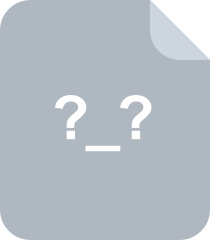
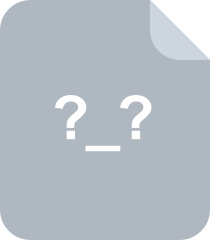
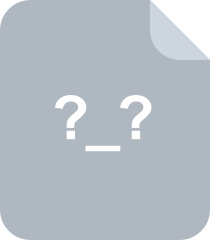
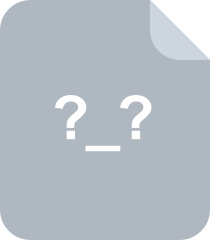
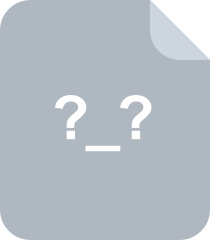
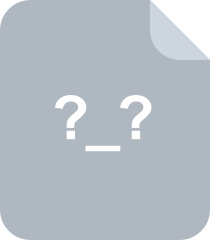
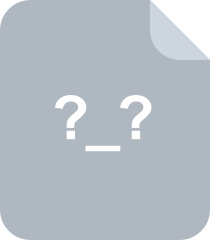
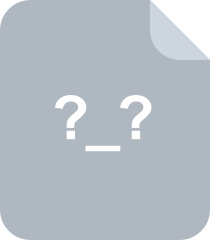
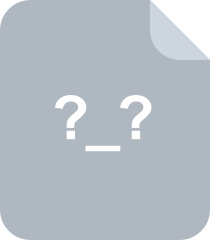
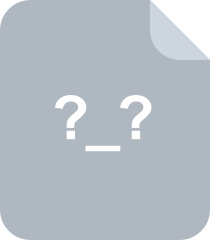
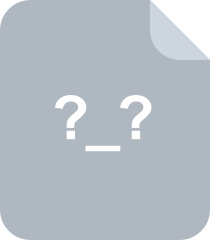
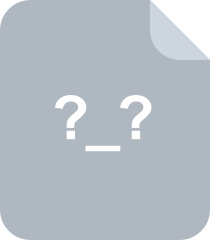
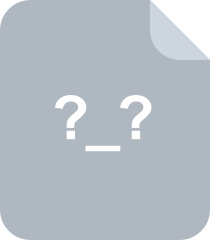
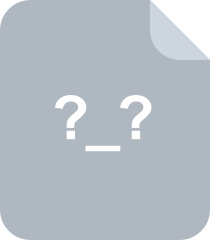
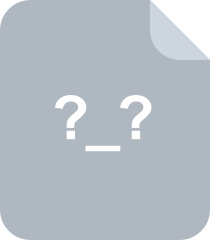
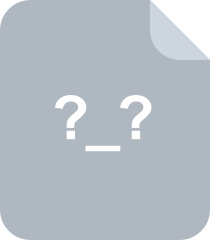
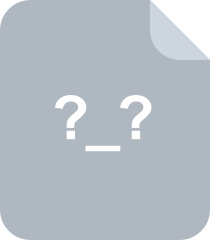
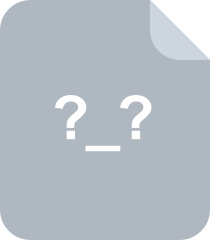
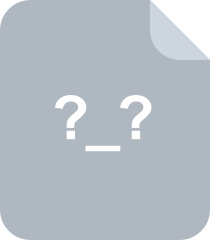
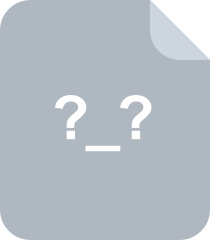
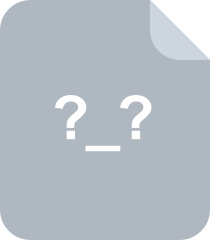
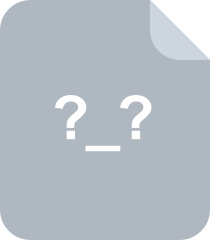
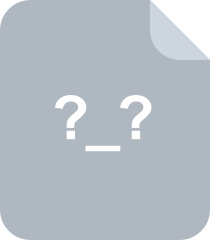
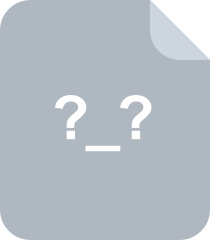
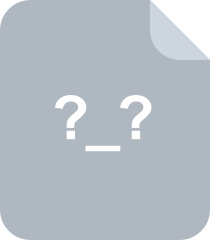
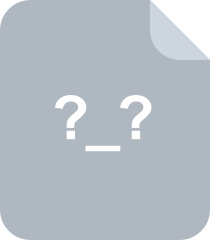
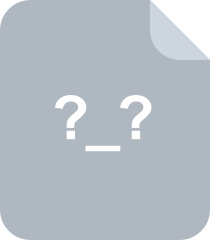
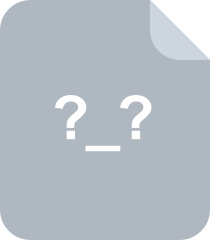
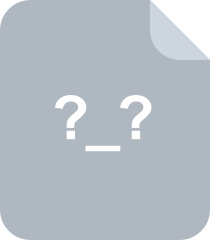
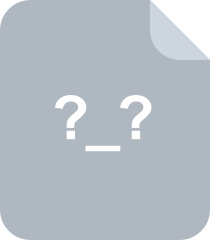
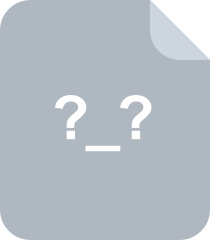
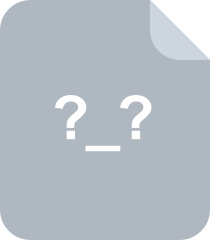
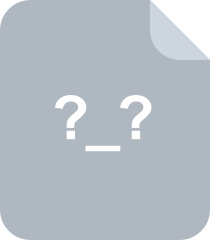
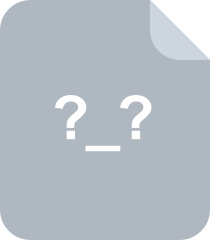
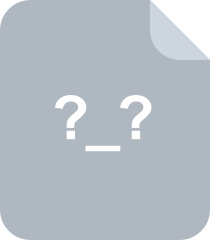
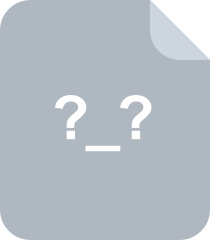
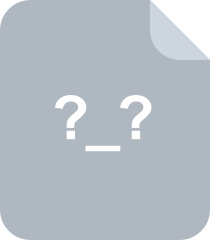
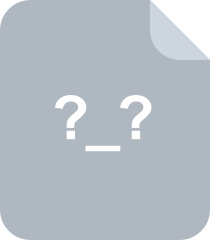
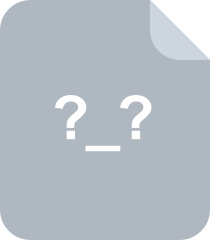
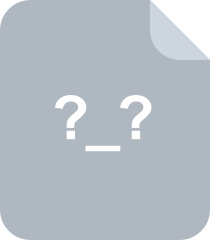
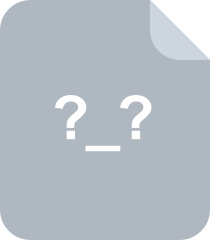
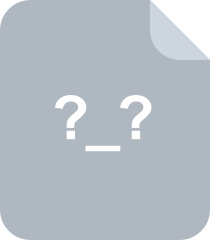
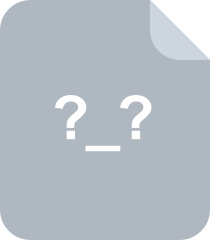
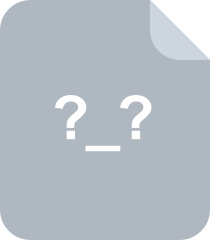
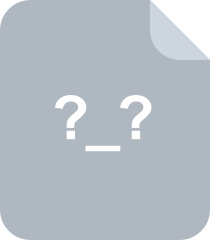
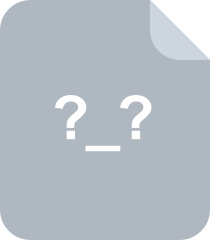
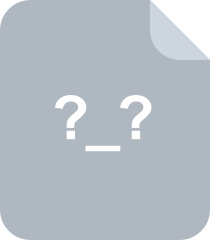
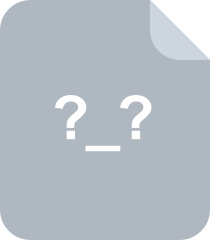
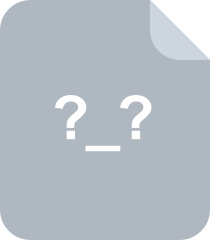
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
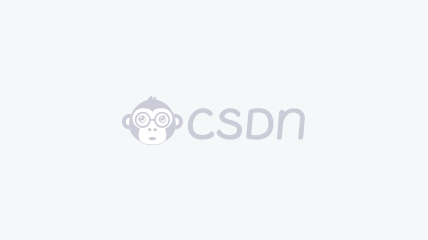
- YONE_hk2022-01-14为什么上传了前端打开空白??
- 李涛9702022-01-24用户下载后在一定时间内未进行评价,系统默认好评。
- dikz19982022-02-08用户下载后在一定时间内未进行评价,系统默认好评。

阿峰博客
- 粉丝: 11
- 资源: 13
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

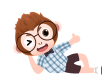
最新资源
- 城镇老旧小区改造(加装电梯)考评内容和评价标准表.docx
- 城镇老旧小区改造及既有住宅加装电梯赋分权重.docx
- 底板隐蔽前监理检查记录.docx
- 出差审批单(表格模板).docx
- 第三方技术服务机构消防验收项目情况工作月汇报表.docx
- 电梯质量安全风险管控清单(安装(含修理).docx
- 飞机舱位代码表.docx
- 顶板隐蔽前监理检查记录表.docx
- 高危妊娠产前评分标准表.docx
- 高温中暑病例报告卡表格.docx
- 个体工商户营业执照颁发及归档记录表.doc
- 更换输液流程表.docx
- 公务接待审批单(表格模板).docx
- 古今地名对照表.docx
- 固定资产验收单、移交清单、处置清单.docx
- 骨关节损伤鉴定标准条款表.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


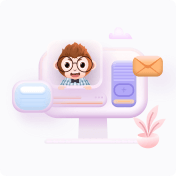
安全验证
文档复制为VIP权益,开通VIP直接复制
