package com.example.controller;
import cn.hutool.core.collection.CollUtil;
import cn.hutool.core.collection.CollectionUtil;
import cn.hutool.core.io.IoUtil;
import cn.hutool.core.util.ObjectUtil;
import cn.hutool.core.util.StrUtil;
import cn.hutool.crypto.SecureUtil;
import cn.hutool.json.JSONObject;
import cn.hutool.poi.excel.ExcelUtil;
import cn.hutool.poi.excel.ExcelWriter;
import com.example.common.Result;
import com.example.common.ResultCode;
import com.example.entity.GoumaiInfo;
import com.example.dao.GoumaiInfoDao;
import com.example.service.GoumaiInfoService;
import com.example.exception.CustomException;
import com.example.common.ResultCode;
import com.example.vo.EchartsData;
import com.example.vo.GoumaiInfoVo;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import com.example.service.*;
import org.springframework.web.bind.annotation.*;
import org.springframework.beans.factory.annotation.Value;
import cn.hutool.core.util.StrUtil;
import org.springframework.web.multipart.MultipartFile;
import javax.annotation.Resource;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.*;
import java.util.stream.Collectors;
@RestController
@RequestMapping(value = "/goumaiInfo")
public class GoumaiInfoController {
@Resource
private GoumaiInfoService goumaiInfoService;
@Resource
private GoumaiInfoDao goumaiInfoDao;
@PostMapping
public Result<GoumaiInfo> add(@RequestBody GoumaiInfoVo goumaiInfo) {
//mixmajixami
goumaiInfoService.add(goumaiInfo);
return Result.success(goumaiInfo);
}
//youtixing1
//youtixing2
@GetMapping("/getByDiqu")
public Result<List<Map<String,String>>> qidu() {
return Result.success(goumaiInfoService.findByDiqu());
}
@DeleteMapping("/{id}")
public Result delete(@PathVariable Long id) {
goumaiInfoService.delete(id);
return Result.success();
}
@GetMapping("/getByzhuceyonghuZhanghao/{zhanghao}")
public Result<List<GoumaiInfo>> getByzhuceyonghuZhanghao(@PathVariable String zhanghao) {
List<GoumaiInfo> goumaiInfo = goumaiInfoService.getByzhuceyonghuZhanghao(zhanghao);
return Result.success(goumaiInfo);
}
@PutMapping
public Result update(@RequestBody GoumaiInfoVo goumaiInfo) {
goumaiInfoService.update(goumaiInfo);
return Result.success();
}
//@PutMapping("/update2")
// public Result update2(@RequestBody GoumaiInfoVo goumaiInfo) {
// goumaiInfoService.update2(goumaiInfo);
// return Result.success();
// }
@GetMapping("/{id}")
public Result<GoumaiInfo> detail(@PathVariable Long id) {
GoumaiInfo goumaiInfo = goumaiInfoService.findById(id);
return Result.success(goumaiInfo);
}
@GetMapping("/changeStatus/{id}")
public Result<GoumaiInfo> changeStatus(@PathVariable Long id) {
goumaiInfoService.changeStatus(id);
return Result.success();
}
@GetMapping("/changeIszf/{id}")
public Result<GoumaiInfo> changeIszf(@PathVariable Long id) {
goumaiInfoService.changeIszf(id);
return Result.success();
}
@GetMapping
public Result<List<GoumaiInfoVo>> all() {
return Result.success(goumaiInfoService.findAll());
}
@GetMapping("/page/{name}")
public Result<PageInfo<GoumaiInfoVo>> page(@PathVariable String name,
@RequestParam(defaultValue = "1") Integer pageNum,
@RequestParam(defaultValue = "5") Integer pageSize,
HttpServletRequest request) {
return Result.success(goumaiInfoService.findPage(name, pageNum, pageSize, request));
}
@GetMapping("/pageqt/{name}")
public Result<PageInfo<GoumaiInfoVo>> pageqt(@PathVariable String name,
@RequestParam(defaultValue = "1") Integer pageNum,
@RequestParam(defaultValue = "8") Integer pageSize,
HttpServletRequest request) {
return Result.success(goumaiInfoService.findPageqt(name, pageNum, pageSize, request));
}
// @PostMapping("/register")
// public Result<GoumaiInfo> register(@RequestBody GoumaiInfo goumaiInfo) {
// if (StrUtil.isBlank(goumaiInfo.getName()) || StrUtil.isBlank(goumaiInfo.getPassword())) {
// throw new CustomException(ResultCode.PARAM_ERROR);
// }
// return Result.success(goumaiInfoService.add(goumaiInfo));
// }
/**
* 批量通过excel添加信息
* @param file excel文件
* @throws IOException
*/
@PostMapping("/upload")
public Result upload(MultipartFile file) throws IOException {
List<GoumaiInfo> infoList = ExcelUtil.getReader(file.getInputStream()).readAll(GoumaiInfo.class);
if (!CollectionUtil.isEmpty(infoList)) {
// 处理一下空数据
List<GoumaiInfo> resultList = infoList.stream().filter(x -> ObjectUtil.isNotEmpty(x.getZhajibianhao())).collect(Collectors.toList());
for (GoumaiInfo info : resultList) {
goumaiInfoService.add(info);
}
}
return Result.success();
}
//yoxutonxgjitu
@GetMapping("/getExcelModel")
public void getExcelModel(HttpServletResponse response) throws IOException {
// 1. 生成excel
Map<String, Object> row = new LinkedHashMap<>();
row.put("zhajibianhao", "A炸鸡编号");
row.put("zhajimingcheng", "A炸鸡名称");
row.put("zhajileibie", "A炸鸡类别");
row.put("jiage", "A价格");
row.put("kucun", "A库存");
row.put("xiaoliang", "A销量");
row.put("zhekou", "A折扣");
row.put("goumaishuliang", "A购买数量");
row.put("goumaijine", "A购买金额");
row.put("dizhi", "A地址");
row.put("zhanghao", "A账号");
row.put("xingming", "A姓名");
row.put("shouji", "A手机");
row.put("waimaiyuanxingming", "A外卖员姓名");
row.put("waimaiyuandianhua", "A外卖员电话");
row.put("status", "是");
row.put("level", "goumai");
List<Map<String, Object>> list = CollUtil.newArrayList(row);
// 2. 写excel
ExcelWriter writer = ExcelUtil.getWriter(true);
writer.write(list, true);
response.setContentType("application/vnd.openxmlformats-officedocument.spreadsheetml.sheet;charset=utf-8");
response.setHeader("Content-Disposition","attachment;filename=goumaiInfoModel.xlsx");
ServletOutputStream out = response.getOutputStream();
writer.flush(out, true);
writer.close();
IoUtil.close(System.out);
}
@GetMapping("/getExcel")
public void getExcel(HttpServletResponse response) throws IOException {
// 1. 生成excel
Map<String, Object> row = new LinkedHashMap<>();
row.put("zhajibianhao", "A炸鸡编号");
row.put("zhajimingcheng", "A炸鸡名称");
row.put("zhajileibie", "A炸鸡类别");
row.put("jiage", "A价格");
row.put("kucun", "A库存");
row.put("xiaoliang", "A销量");
row.put("zhekou", "A折扣");
row.put("goumaishuliang", "A购买数量");
row.put("goumaijine", "A购买金额");
row.put("dizhi", "A地址");
row.put("zhanghao", "A账号");
row.put("xingming", "A姓名");
row.put("shouji", "A手机");
row.put("waimaiyuanxingming", "A外卖员姓名");
row.put("waimaiyuandianhua", "A外卖员电话");
row.put("status", "是");
row.put("level", "权限");
List<Map<String, Object>> list = CollUtil.newArrayList(row);
List<Map<String, Object>> daochuexcellist = goumaiInfoDao.daochuexcel();
Map<String, Double> typeMap = new HashMap<>()
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
微信小程序源码-炸鸡外卖小程序的微信小程序毕业设计(源码+说明+数据库+录像).zip 【项目技术】 微信小程序开发工具+mysql 【实现功能】 前台: (1)用户注册登录:用户需要按照相关的法律法规进行注册,注册后才可以使用本系统。 (2)首页模块:网站的前台页面主要对所有菜单进行显示,除此之外还能显示最新的菜品信息、活动公告等。 (3)菜品查询模块:用户通过此模块可以对自己感兴趣的菜品进行精准或模糊查询,查询之后可以进入菜品详情页面,可以进行下单或收藏等操作。 (4)个人后台:用户进入个人后台可以维护个人信息及查看菜品订单信息。 (5)公告活动:用户可以在前台进行公告活动的浏览,可以更好的了解炸鸡店的活动优惠。 (6)在线留言:用户在使用本系统的过程中如果发现订单或者菜品有问题可以通过在线留言与商家进行交流。 后台: (1)管理员信息维护模块:管理员对此系统非常关键,所以管理员的信息也是需要进行定期维护的。 (2)用户信息管理模块:所有在本网站进行注册的用户信息可以通过本模块进行查询和维护。 (3)菜品类别及菜品信息模块:管理员可以通过本模块添加新的菜品类别或菜品信息,也可以对已有的菜品和类别信息。
资源推荐
资源详情
资源评论















收起资源包目录

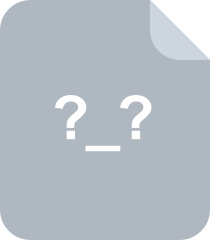
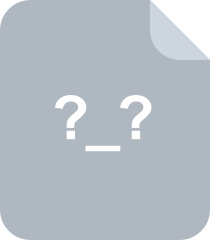
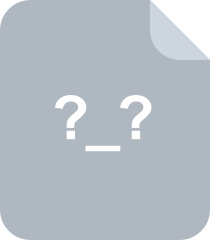
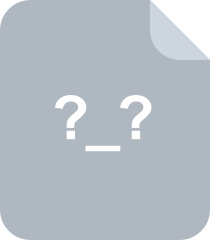
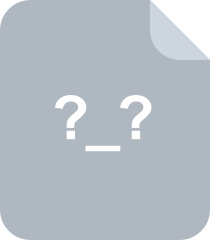
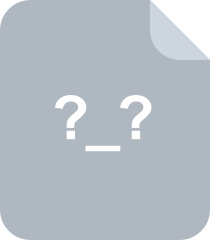
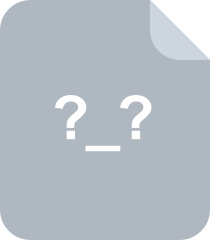
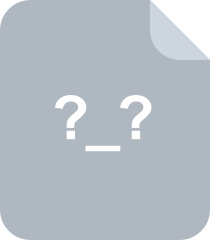
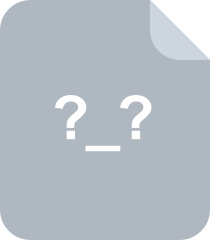
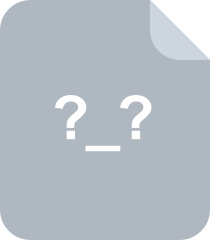
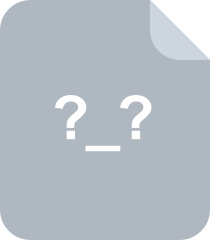
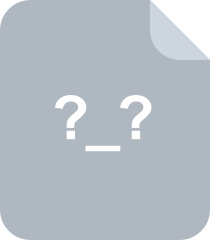
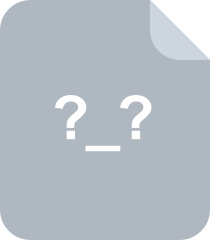
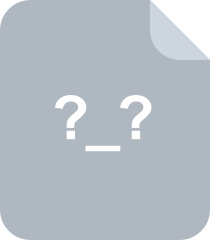
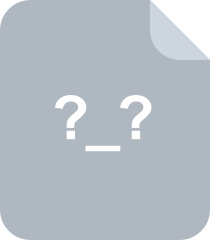
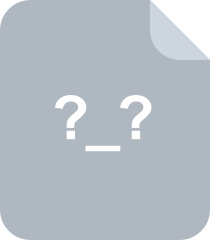
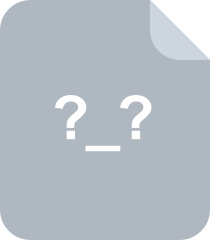
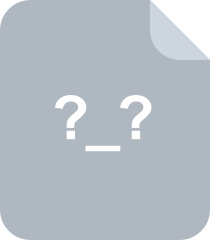
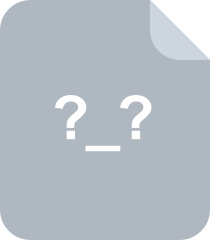
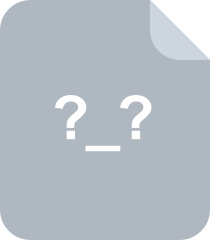
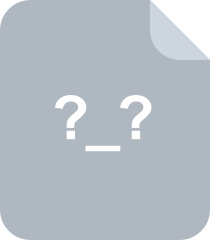
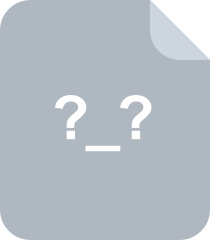
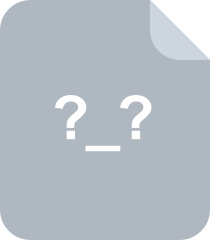
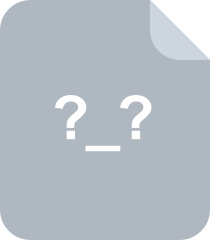
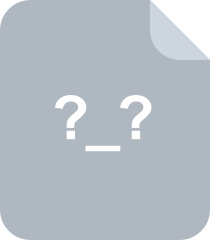
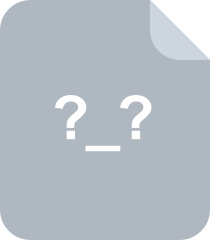
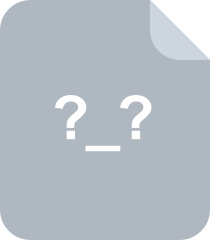
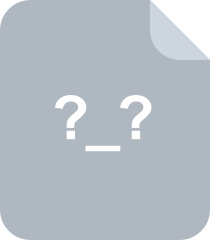
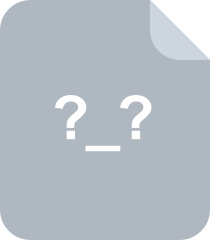
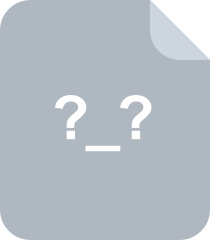
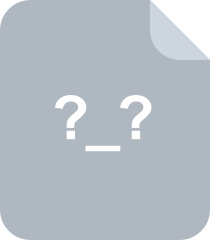
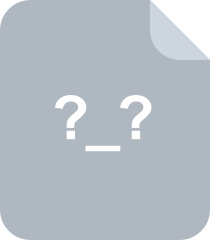
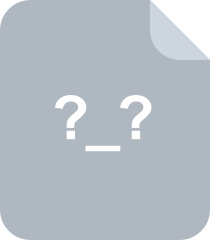
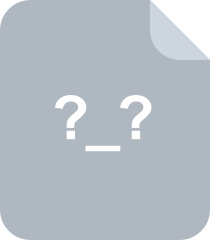
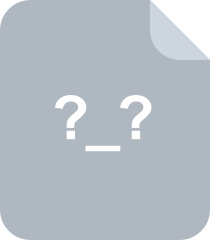
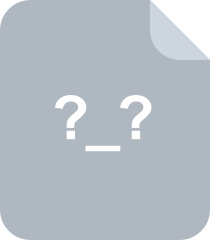
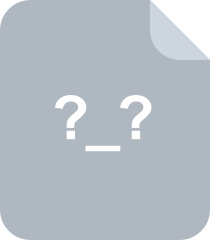
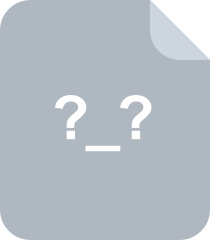
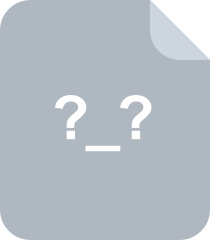
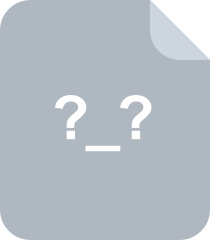
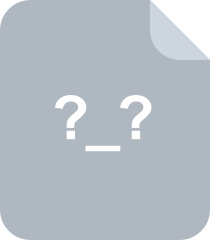
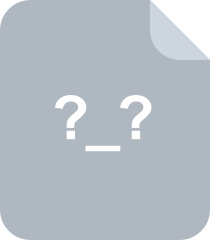
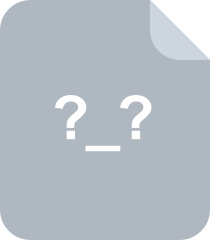
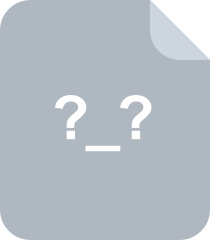
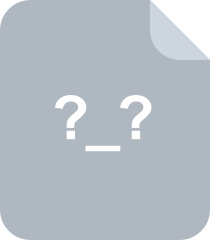
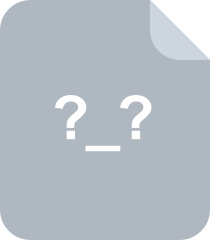
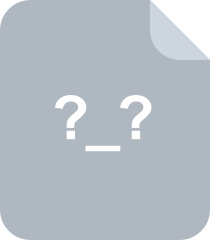
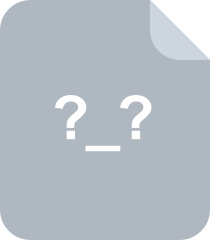
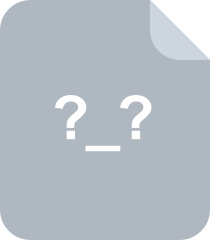
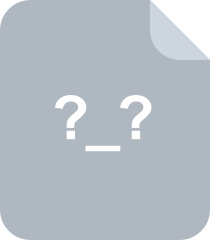
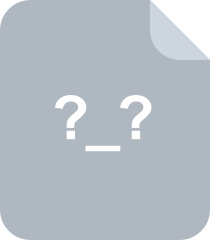
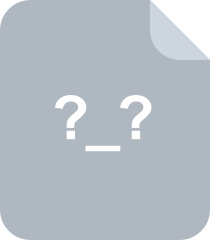
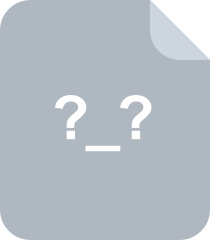
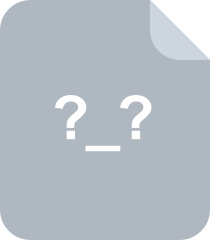
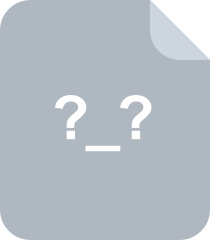
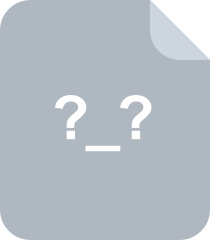
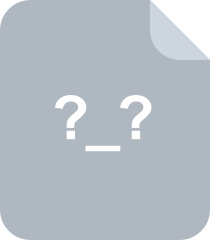
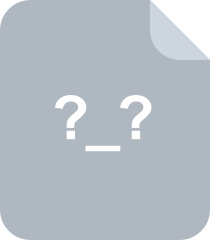
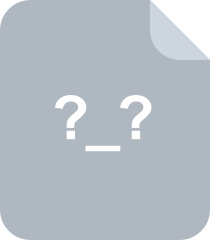
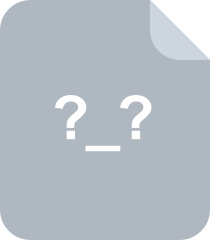
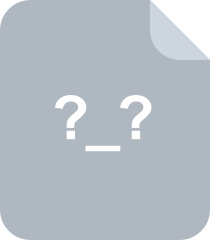
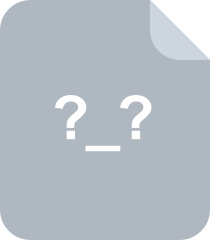
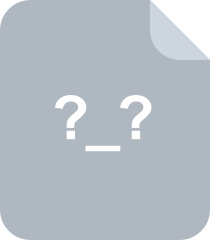
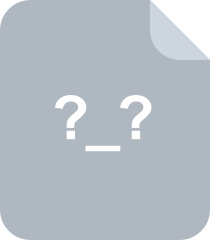
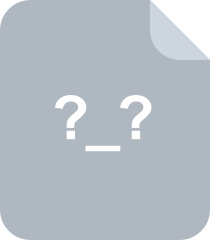
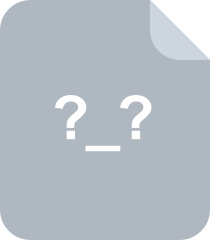
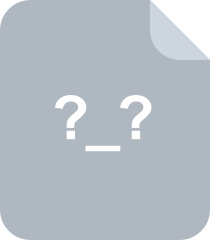
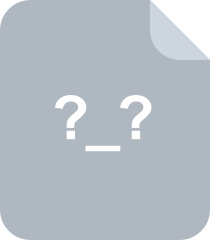
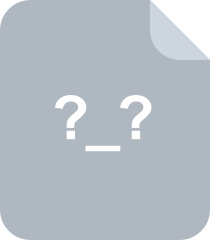
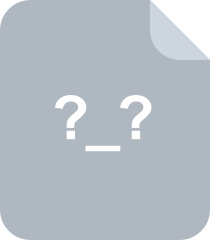
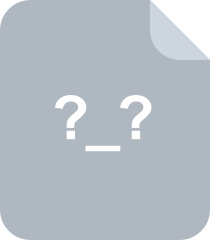
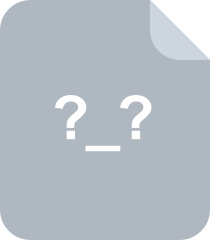
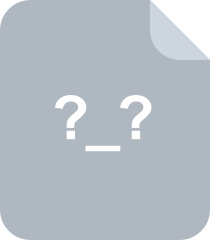
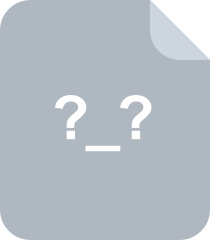
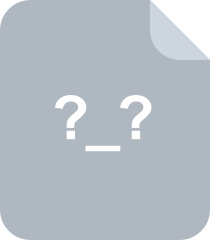
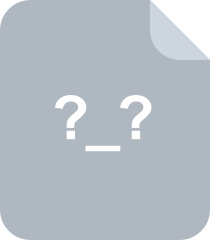
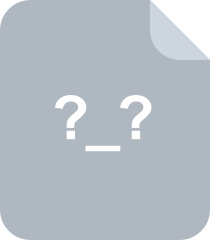
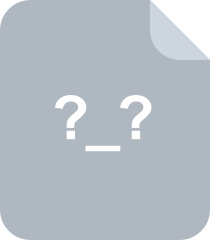
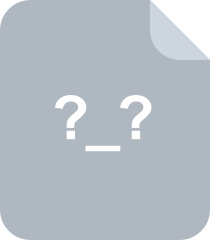
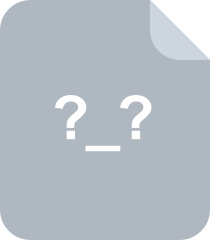
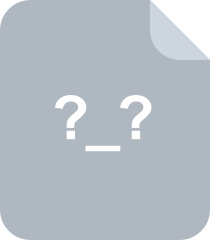
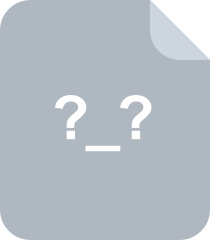
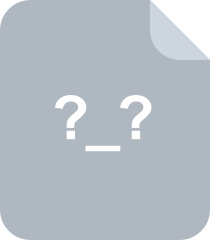
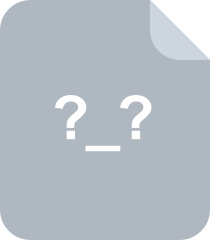
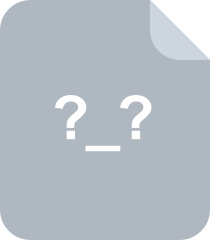
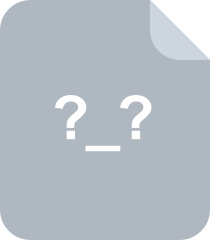
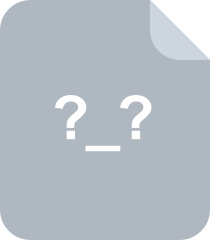
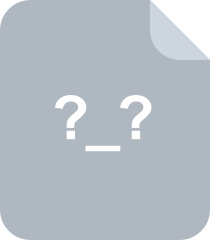
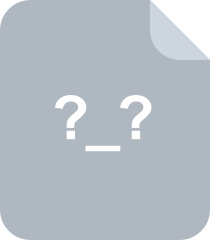
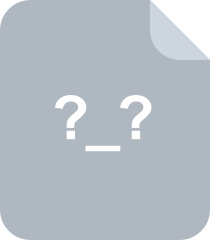
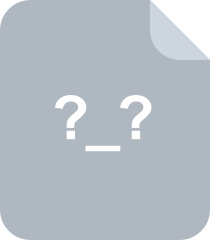
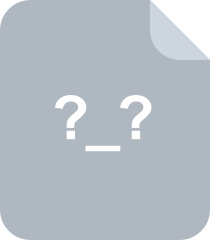
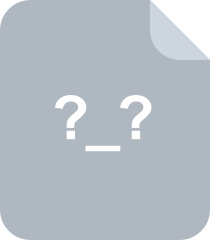
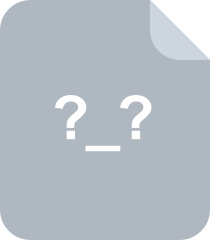
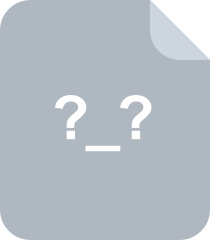
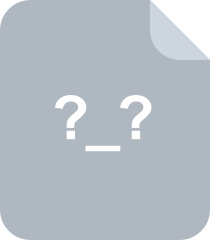
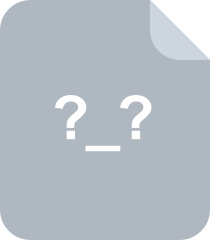
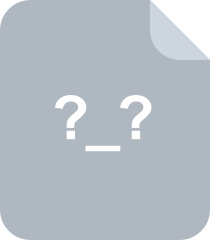
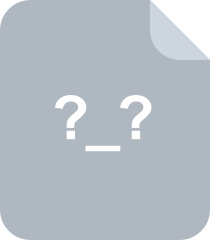
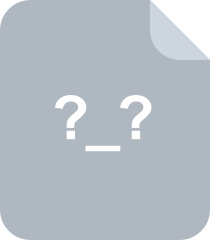
共 745 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
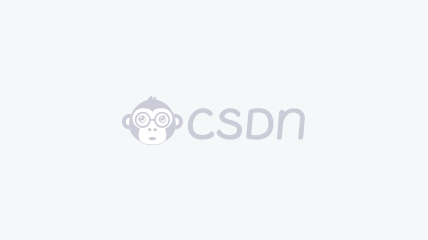
- weixin_590748332023-08-25资源很赞,希望多一些这类资源。

岛上程序猿
- 粉丝: 6041
- 资源: 4248
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

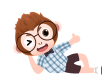
最新资源
- 学生管理系统(PDF).pdf
- 部署手册-Linux环境下Nginx、Tomcat、JDK11和MySQL的配置与项目部署指南
- 1_4_永合创信LOGO(2024)无白底色.psd
- 中分子量聚异丁烯市场分析:2025-2031期间年复合增长率(CAGR)为9.2%
- 点餐平台网站(基于springboot,mysql,java).zip
- 易语言OCR本地文字识别:支持图片识别及无网离线操作,精准高效无库轻便应用,基于飞浆OCR技术的易语言本地文字识别系统:无网离线识别,适应多种系统环境与图片格式,易语言基于飞浆的本地ocr文字识别
- 公开整理-数字普惠金融指数-省级2011-2023年.dta
- SQL数据库图书管理系统课程设计.doc
- 基于C++(MFC)的细胞识别程序【100010154】
- 自己编写和收集的文华财经量化交易源代码 仅供学习
- 《STM32单片机+HC-SR04超声波测距传感器+OLED屏幕+蜂鸣器报警+超声波测距数据发送到串口调试助手》源代码
- 基于腾讯地图的北斗_GPS_GSM三定位车辆监控系统.pdf
- (完整word版)银行信息科技外包风险管理办法.pdf
- MVVM及数据代理本文介绍了MVVM架构及其在Vue中的应用
- 波段王抄底_0.tne超选股指标
- 中文3DMAX测量标注插件DIMaster v2.0下载
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


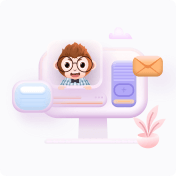
安全验证
文档复制为VIP权益,开通VIP直接复制
