package com.zyy.book.controller;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.zyy.book.component.UserInterceptor;
import com.zyy.book.entity.*;
import com.zyy.book.exception.MyException;
import com.zyy.book.output.MyMsg;
import com.zyy.book.service.*;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.DigestUtils;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
import java.math.BigDecimal;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.time.LocalDateTime;
import java.time.ZoneOffset;
import java.util.Calendar;
import java.util.Date;
/**
* <p>
* 前端控制器
* </p>
*
* @author zyy
* @since 2021-02-23
*/
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private IUserService userService;
@Autowired
private IOrderService orderService;
@Autowired
private IRechargeService rechargeService;
@Autowired
private IBookService bookService;
@Autowired
private IBookChapterService bookChapterService;
@RequestMapping(method = RequestMethod.GET, path = "/logout")
public void logout(HttpServletRequest request, HttpServletResponse response) throws IOException {
HttpSession session = request.getSession();
session.removeAttribute(UserInterceptor.USER_SESSION_KEY);
response.sendRedirect("/");
}
@RequestMapping(method = RequestMethod.GET, path = "/login")
public ModelAndView login() {
return new ModelAndView("home/user/login");
}
@RequestMapping(method = RequestMethod.POST, path = "/login")
public MyMsg loginPost(@RequestParam String username,
@RequestParam String password,
HttpServletRequest request) throws MyException {
QueryWrapper<User> userQueryWrapper = new QueryWrapper<>();
userQueryWrapper.eq("username", username);
User user = userService.getOne(userQueryWrapper);
if (user == null) {
throw new MyException("用户或密码不正确");
}
if (!user.getPassword().equals(DigestUtils.md5DigestAsHex(password.getBytes()))) {
throw new MyException("用户或密码不正确");
}
HttpSession session = request.getSession();
session.setAttribute(UserInterceptor.USER_SESSION_KEY, user);
/*更新时间*/
user.setUpdatedAt(LocalDateTime.now());
userService.saveOrUpdate(user);
return new MyMsg(MyMsg.SUCCESS, "登陆成功");
}
@RequestMapping(method = RequestMethod.GET, path = "/reg")
public ModelAndView reg() {
return new ModelAndView("home/user/reg");
}
@RequestMapping(method = RequestMethod.POST, path = "/reg")
public MyMsg regPost(@RequestBody User user) throws MyException {
if (!StringUtils.hasText(user.getUsername()) || !StringUtils.hasText(user.getPassword())) {
throw new MyException("用户名或密码不能为空");
}
QueryWrapper<User> userQueryWrapper = new QueryWrapper<>();
userQueryWrapper.eq("username", user.getUsername());
int count = userService.count(userQueryWrapper);
if (count > 0) {
throw new MyException("用户名已存在");
}
if (user.getPhone().length() != 11) {
throw new MyException("手机号码长度为11位");
}
user.setPassword(DigestUtils.md5DigestAsHex(user.getPassword().getBytes()));
user.setBalance(new BigDecimal(0));
boolean save = userService.save(user);
if (!save) {
throw new MyException("注册失败");
}
return new MyMsg(MyMsg.SUCCESS, "", user);
}
@RequestMapping(method = RequestMethod.GET, path = "/index")
public ModelAndView index() {
return new ModelAndView("home/user/index");
}
@RequestMapping(method = RequestMethod.POST, path = "/vip")
public MyMsg vip(HttpServletRequest request) throws MyException {
HttpSession session = request.getSession();
User user1 = (User) session.getAttribute(UserInterceptor.USER_SESSION_KEY);
User user = userService.getById(user1.getId());
if (user.getVipExpirationAt() != null && user.getVipExpirationAt().isAfter(LocalDateTime.now())) {
throw new MyException("会员未过期");
}
if (user.getBalance().compareTo(new BigDecimal(100)) < 0) {
throw new MyException("开通VIP余额不足 ¥" + User.VIP_PRICE);
}
user.setBalance(user.getBalance().subtract(new BigDecimal(User.VIP_PRICE)));
Calendar calendar = Calendar.getInstance();
calendar.setTime(new Date());
calendar.add(Calendar.YEAR, 1);
LocalDateTime localDateTime = LocalDateTime.ofInstant(calendar.toInstant(), ZoneOffset.systemDefault());
user.setVipExpirationAt(localDateTime);
userService.saveOrUpdate(user);
return new MyMsg(MyMsg.SUCCESS, "开通成功", user);
}
@RequestMapping(method = RequestMethod.GET, path = "/info")
public ModelAndView info(HttpServletRequest request) {
HttpSession session = request.getSession();
User user1 = (User) session.getAttribute(UserInterceptor.USER_SESSION_KEY);
User user = userService.getById(user1.getId());
return new ModelAndView("home/user/info", "user", user);
}
@RequestMapping(method = RequestMethod.POST, path = "/info")
public MyMsg infoPost(@RequestParam String gender,
@RequestParam String birthday,
@RequestParam String phone,
HttpServletRequest request) throws MyException, ParseException {
if (phone == null || phone.length() != 11) {
throw new MyException("手机号必须为11位");
}
HttpSession session = request.getSession();
User user1 = (User) session.getAttribute(UserInterceptor.USER_SESSION_KEY);
User user = userService.getById(user1.getId());
user.setGender(gender);
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd");
user.setBirthday(simpleDateFormat.parse(birthday));
user.setPhone(phone);
userService.saveOrUpdate(user);
return new MyMsg(MyMsg.SUCCESS, "操作成功");
}
@RequestMapping(method = RequestMethod.GET, path = "/recharge")
public ModelAndView recharge(HttpServletRequest request) {
HttpSession session = request.getSession();
User user1 = (User) session.getAttribute(UserInterceptor.USER_SESSION_KEY);
User user = userService.getById(user1.getId());
ModelAndView view = new ModelAndView();
view.setViewName("home/user/recharge");
view.addObject("user", user);
view.addObject("vipPrice", User.VIP_PRICE);
return view;
}
@RequestMapping(method = RequestMethod.POST, path = "/recharge")
public MyMsg recharge(@RequestParam BigDecimal balance, HttpServletRequest request) throws MyException {
if (balance.compareTo(BigDecimal.ZERO) < 0) {
throw new MyException("金额必须大于0");
}
HttpSession session = request.getSession();
User user1 = (User) session.getAttribute(UserInterceptor.USER_SESSION_KEY);
User user = userService.getById(user1.getId());
if (user.getBalance() == null) {
user.setBalance(new BigDecimal(0));
}
BigDecimal newBalance = user.getBalance().add(balance);
user.setBalance(newBalance);
userService.saveOrUpdate(user);
/*log*/
Recharge recharge = new Recharge()
没有合适的资源?快使用搜索试试~ 我知道了~
Springboot项目-线上阅读系统项目实战(源码+说明+演示视频).zip
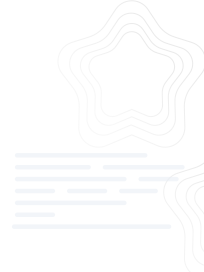
共486个文件
js:125个
png:83个
java:67个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 96 浏览量
2023-07-05
18:30:07
上传
评论
收藏 38.49MB ZIP 举报
温馨提示
Springboot项目-线上阅读系统项目实战(源码+说明+演示视频).zip 【项目技术】 java+mysql+b/s+springboot 【实现功能】 包括管理模块、留言交流区,新闻浏览模块、书籍查询等
资源推荐
资源详情
资源评论
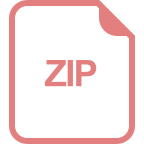
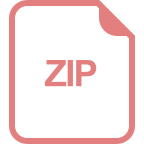
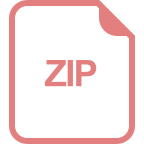
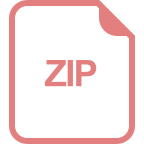
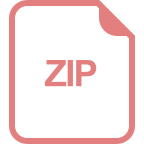
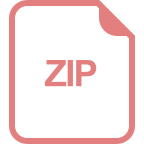
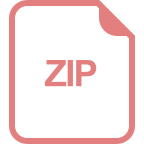
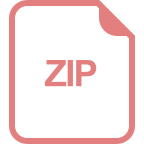
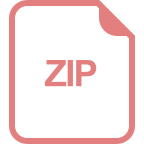
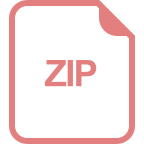
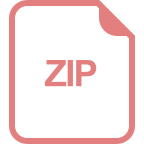
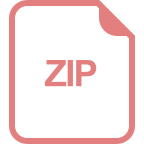
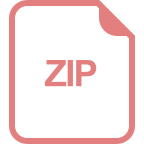
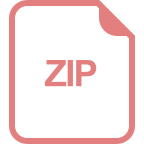
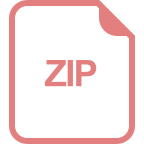
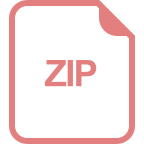
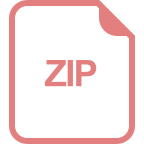
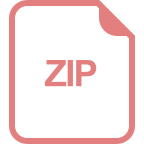
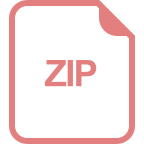
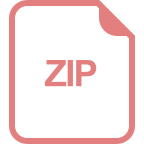
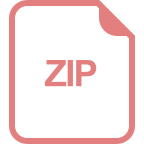
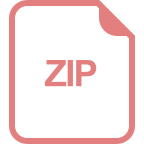
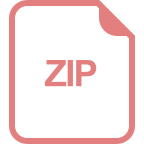
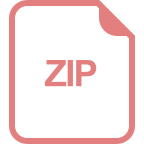
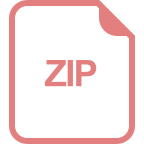
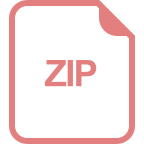
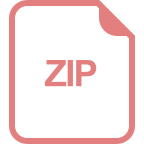
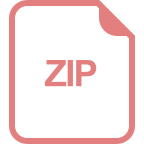
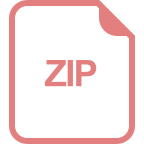
收起资源包目录

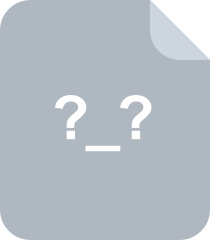
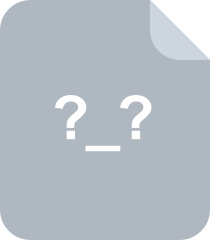
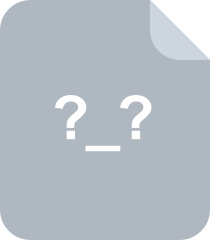
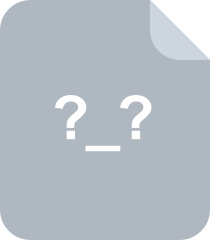
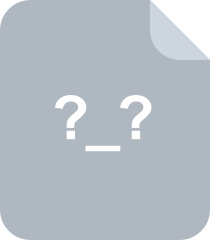
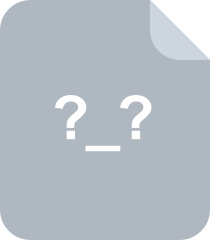
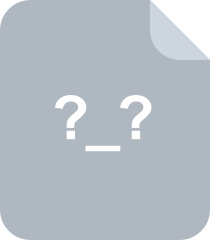
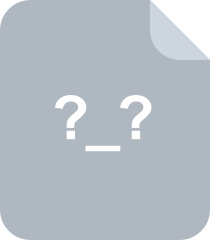
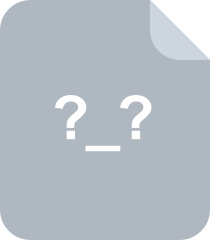
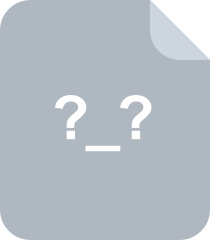
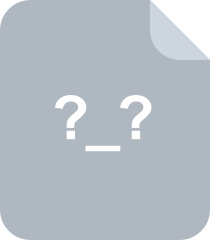
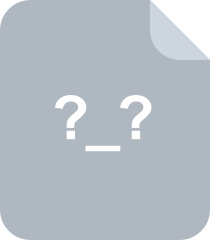
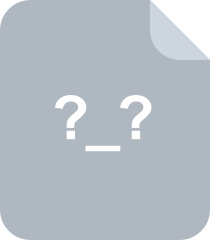
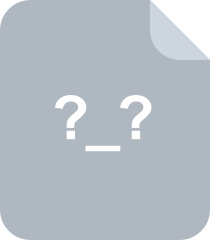
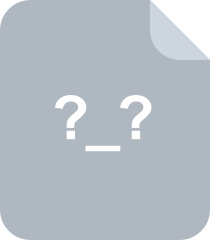
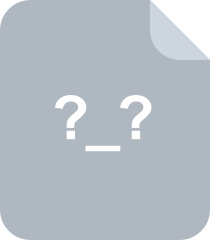
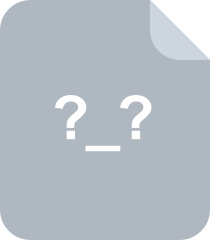
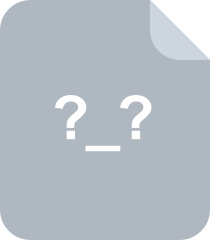
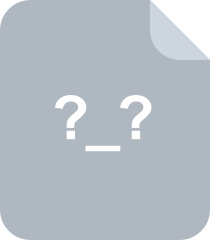
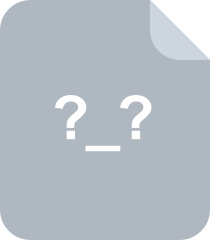
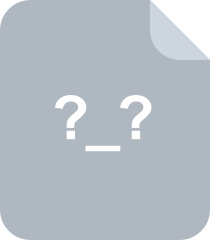
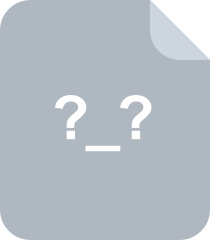
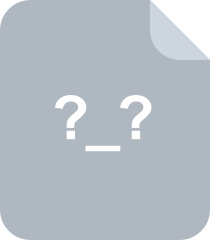
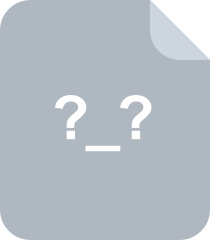
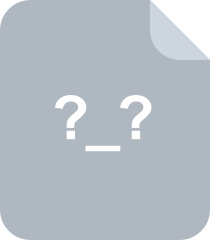
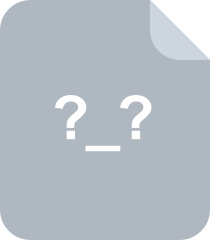
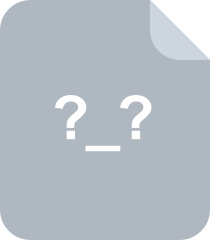
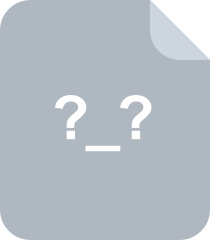
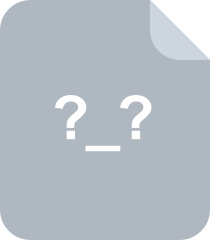
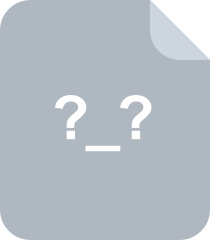
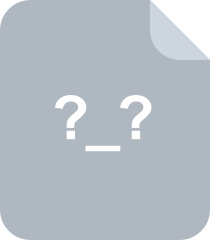
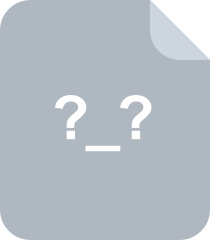
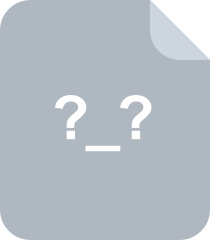
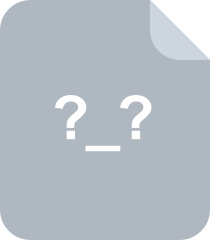
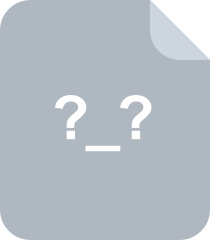
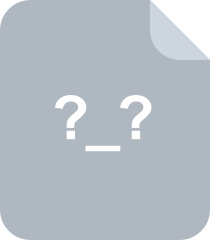
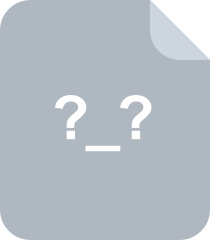
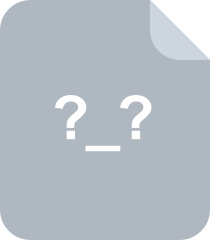
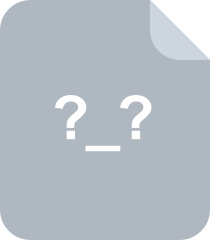
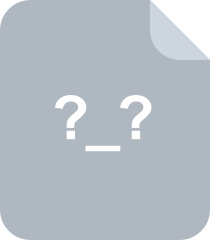
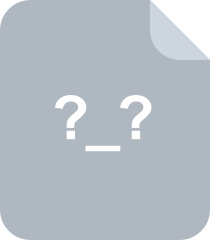
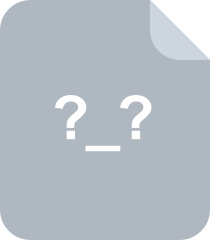
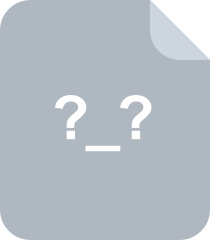
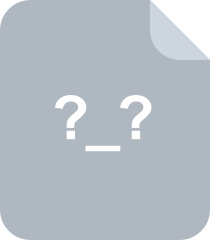
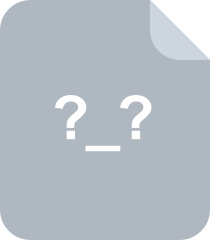
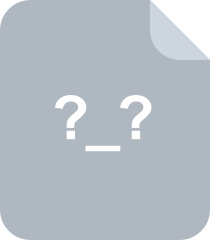
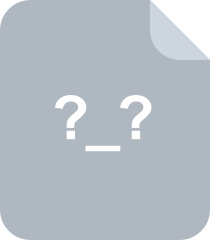
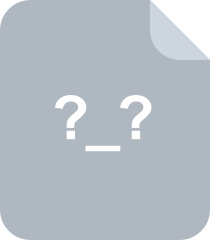
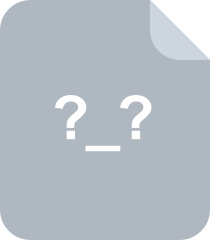
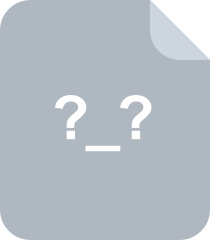
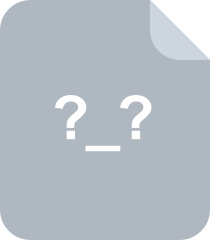
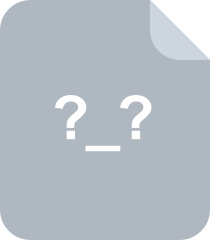
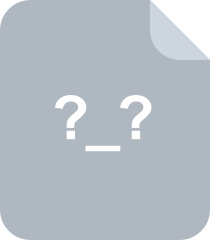
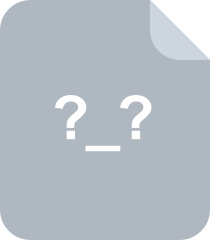
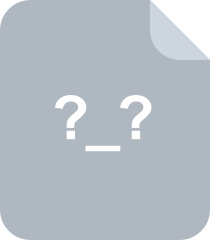
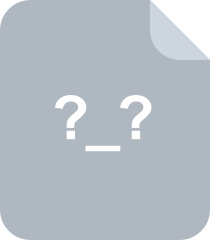
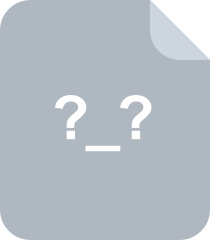
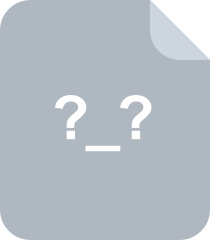
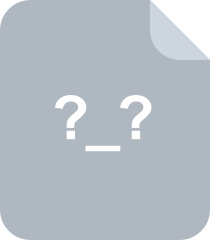
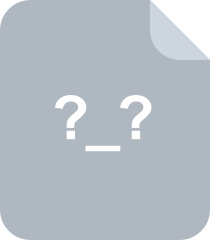
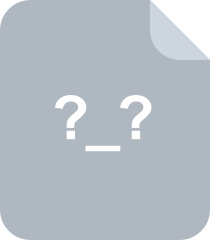
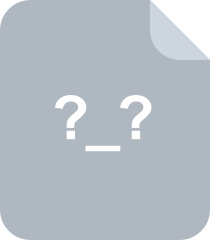
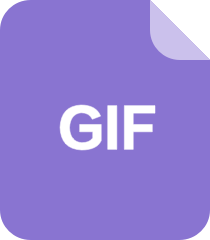
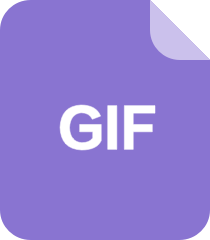
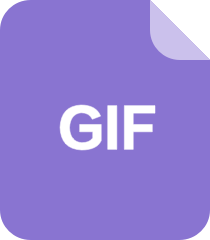
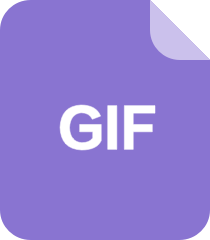
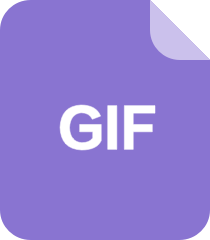
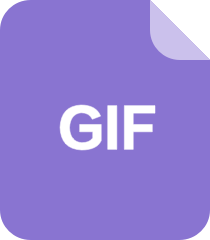
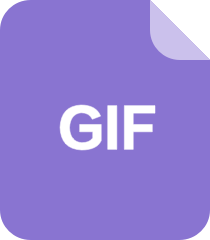
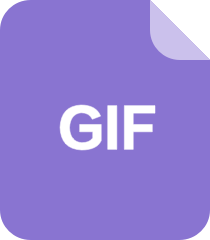
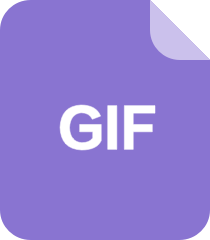
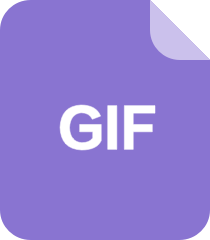
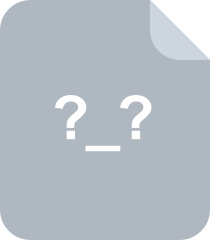
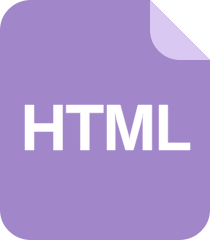
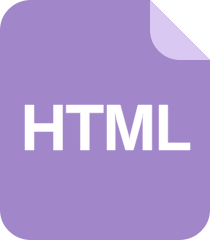
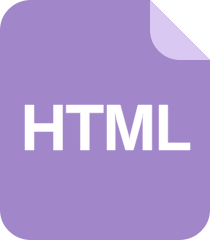
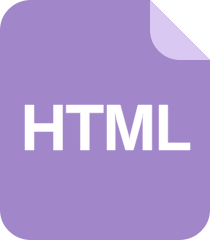
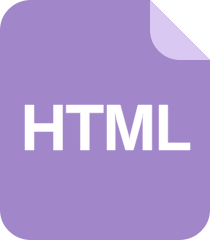
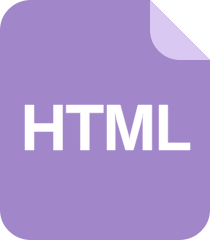
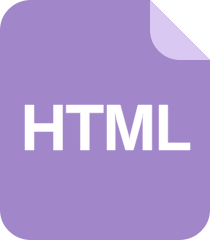
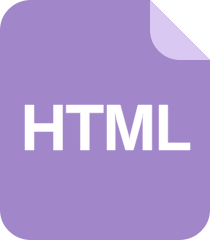
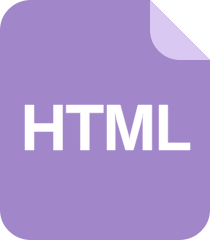
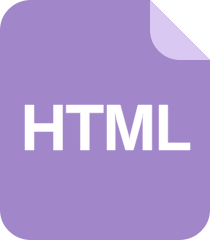
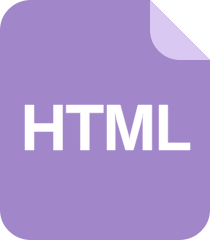
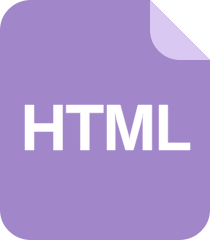
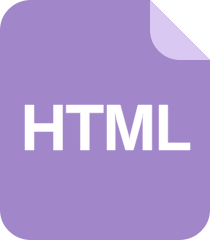
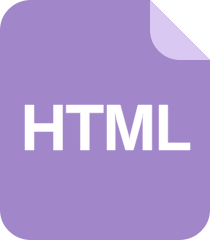
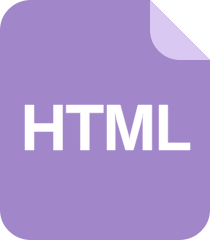
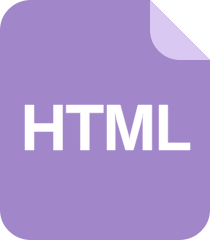
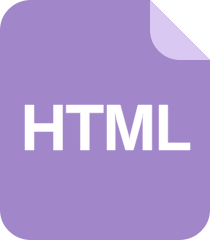
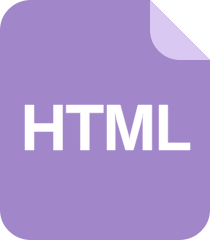
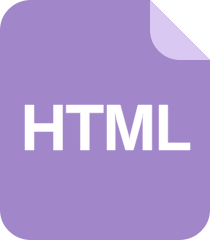
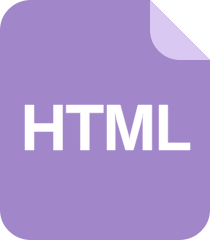
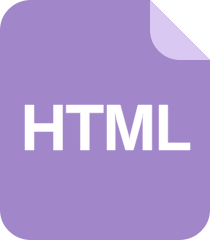
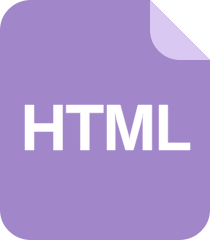
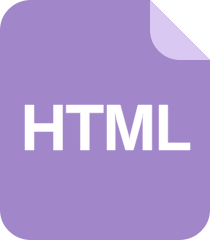
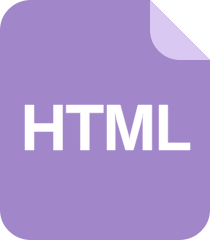
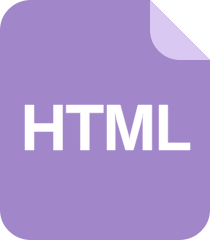
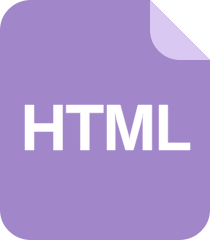
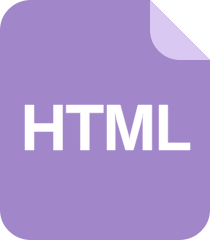
共 486 条
- 1
- 2
- 3
- 4
- 5
资源评论
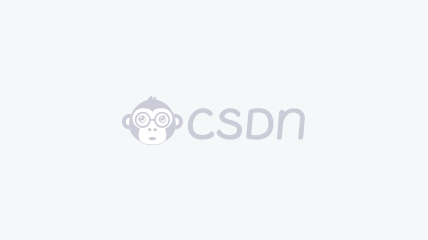

岛上程序猿
- 粉丝: 5732
- 资源: 4245
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

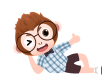
最新资源
- 职工上、下班交通费补贴规定.docx
- 房地产公司圣诞活动策划方案.docx
- 全球旅游与经济指标数据集,旅游影响因素数据集,旅游与收入数据(六千六百多条数据)
- 公司下午茶费用预算.xlsx
- 下午茶.docx
- 毕设和企业适用springboot计算机视觉平台类及在线平台源码+论文+视频.zip
- 2014年度体检项目.xls
- 年度员工体检项目.xls
- 年度体检.xlsx
- 毕设和企业适用springboot跨境电商平台类及虚拟现实体验平台源码+论文+视频.zip
- 毕设和企业适用springboot平台对接类及全球电商管理平台源码+论文+视频.zip
- 数据库-sqlite客户端-sqlite-访问sqlite数据库
- 住宅小区汽车超速检测及报警系统设计(单片机源码+图+报告)
- 毕设和企业适用springboot区块链技术类及客户关系管理平台源码+论文+视频.zip
- 毕设和企业适用springboot区块链技术类及音频处理平台源码+论文+视频.zip
- 毕设和企业适用springboot区块链交易平台类及交通信息平台源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


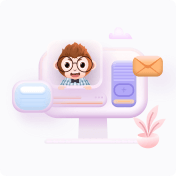
安全验证
文档复制为VIP权益,开通VIP直接复制
