package com.util;
import java.io.BufferedInputStream;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.GregorianCalendar;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Random;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/*import dao.CommDAO;*/
import jxl.Workbook;
import jxl.write.Label;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
import jxl.write.WriteException;
import jxl.write.biff.RowsExceededException;
public class Info {
//public static String popheight = "alliframe.height=document.body.clientHeight+";
public static String popheight = "alliframe.style.height=document.body.scrollHeight+";
public static HashMap getUser(HttpServletRequest request)
{
HashMap map = (HashMap)(request.getSession().getAttribute("admin")==null?request.getSession().getAttribute("user"):request.getSession().getAttribute("admin"));
return map;
}
public static int getBetweenDayNumber(String dateA, String dateB) {
long dayNumber = 0;
//1小时=60分钟=3600秒=3600000
long mins = 60L * 1000L;
//long day= 24L * 60L * 60L * 1000L;计算天数之差
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm");
try {
java.util.Date d1 = df.parse(dateA);
java.util.Date d2 = df.parse(dateB);
dayNumber = (d2.getTime() - d1.getTime()) / mins;
} catch (Exception e) {
e.printStackTrace();
}
return (int) dayNumber;
}
public static void main(String[] g )
{
System.out.print(Info.getBetweenDayNumber("2012-11-11 11:19", "2012-11-11 11:11"));
}
public static String getselect(String name,String tablename,String zdname)
{
String select = "<select name=\""+name+"\" id=\""+name+"\" >";
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" order by id desc")){
select+="<option value=\""+permap.get(zdname)+"\">"+permap.get(zdname)+"</option>";
}
select+="</select>";*/
return select;
}
public static String getselect(String name,String tablename,String zdname,String where)
{
String select = "<select name=\""+name+"\" id=\""+name+"\" >";
select+="<option value=\"\">不限</option>";
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" where "+where+" order by id desc")){
String optionstr = "";
if(zdname.split(";").length==1){
optionstr=permap.get(zdname.split("~")[0]).toString();
}else{
for(String str:zdname.split(";"))
{
String zdstr = str.split("~")[0];
String zdnamestr = str.split("~")[1].equals("无名")?"":(str.split("~")[1]+":");
optionstr+=zdnamestr+permap.get(zdstr)+" - ";
}
}
if(optionstr.indexOf(" - ")>-1)optionstr=optionstr.substring(0,optionstr.length()-3);
select+="<option value=\""+optionstr+"\">"+optionstr+"</option>";
}
select+="</select>";*/
return select;
}
public static String getradio(String name,String tablename,String zdname,String where)
{
String radio="";
int dxii = 0;
/*for(HashMap permap:new CommDAO().select("select * from "+tablename+" where "+where+" order by id desc")){
String check="";
if(dxii==0)check="checked=checked";
String optionstr = "";
for(String str:zdname.split(";"))
{
String zdstr = str.split("~")[0];
String zdnamestr = str.split("~")[1].equals("无名")?"":(str.split("~")[1]+":");
optionstr+=zdnamestr+permap.get(zdstr)+" - ";
}
if(optionstr.length()>0)optionstr=optionstr.substring(0,optionstr.length()-3);
radio+="<label><input type='radio' name='"+name+"' "+check+" value=\""+optionstr+"\">"+optionstr+"</label>\n";
dxii++;
}*/
return radio;
}
public static void writeExcel(String fileName,String prosstr,java.util.List<List> plist,HttpServletRequest request, HttpServletResponse response){
WritableWorkbook wwb = null;
String cols = "";
for(String str:prosstr.split("@"))
{
cols+=str.split("-")[0]+",";
}
cols = cols.substring(0,cols.length()-1);
String where = request.getAttribute("where")==null?"":request.getAttribute("where").toString();
/* List<List> mlist = new CommDAO().selectforlist("select "+cols+" from "+fileName+" "+where+" order by id desc");*/
fileName = request.getRealPath("/")+"/upfile/"+Info.generalFileName("a.xls");
String[] pros = prosstr.split("@");
try {
//首先要使用Workbook类的工厂方法创建一个可写入的工作薄(Workbook)对象
wwb = Workbook.createWorkbook(new File(fileName));
} catch (IOException e) {
e.printStackTrace();
}
if(wwb!=null){
//创建一个可写入的工作表
//Workbook的createSheet方法有两个参数,第一个是工作表的名称,第二个是工作表在工作薄中的位置
WritableSheet ws = wwb.createSheet("sheet1", 0);
ws.setColumnView(0,20);
ws.setColumnView(1,20);
ws.setColumnView(2,20);
ws.setColumnView(3,20);
ws.setColumnView(4,20);
ws.setColumnView(5,20);
try {
for(int i=0;i<pros.length;i++)
{
Label label1 = new Label(i, 0,"");
label1.setString(pros[i]);
ws.addCell(label1);
}
} catch (RowsExceededException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (WriteException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
//下面开始添加单元格
/*int i=1;*/
/* for(List t:mlist){
try {
Iterator it = t.iterator();
int jj=0;
while(it.hasNext())
{
Label label1 = new Label(jj, i,"");
String a = it.next().toString();
label1.setString(a);
ws.addCell(label1);
jj++;
}
i++;
} catch (RowsExceededException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
} catch (WriteException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}
}
try {
//从内存中写入文件中
wwb.write();
//关闭资源,释放内存
wwb.close();
} catch (IOException e) {
e.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
}
}
try {
response.sendRedirect("/pianotrainwebstie/upload?filename="+fileName.substring(fileName.lastIndexOf("/")+1));
} catch (IOException e) {
e.printStackTrace();*/
}
}
public static String getcheckbox(String name,String tablename,String zdname,String where)
{
String checkbox="";
/* f
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
Java毕业设计-基于ssm框架的学生网上请假系统(源码+说明+演示视频) 【项目技术】 java+mysql+ssm+b/s 【实现功能】 本系统主要包含了学生请假管理、班级信息管理、基础信息、用户权限管理、用户信息管理、留言等多个功能模块。
资源推荐
资源详情
资源评论
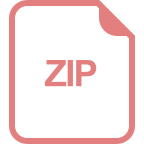
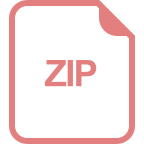
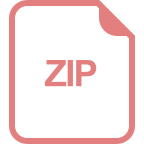
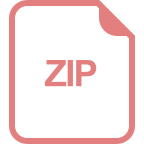
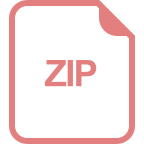
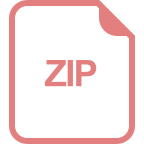
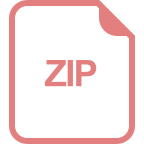
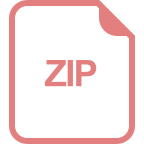
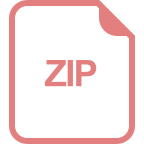
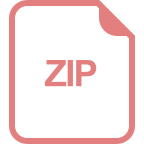
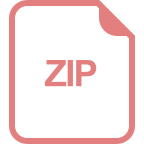
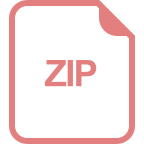
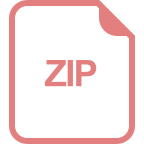
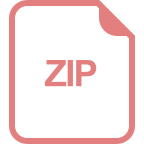
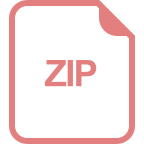
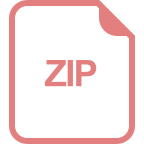
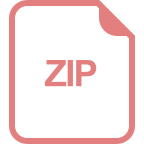
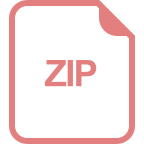
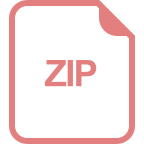
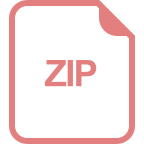
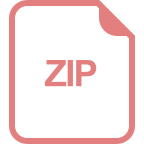
收起资源包目录

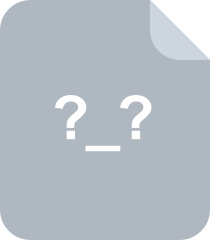
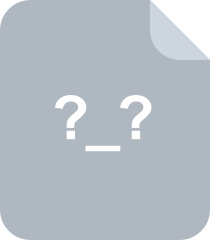
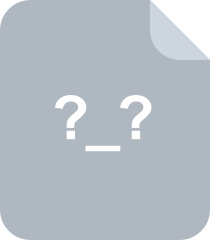
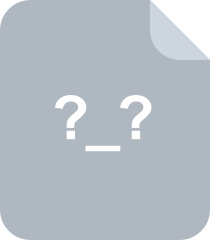
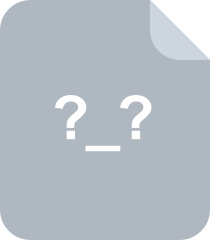
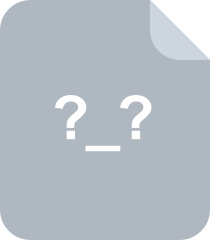
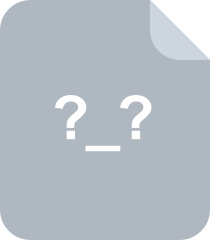
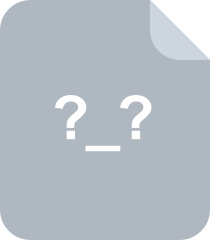
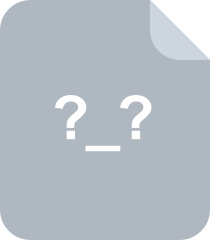
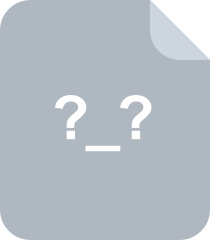
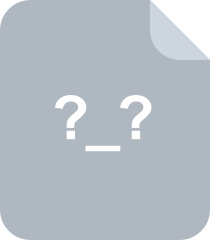
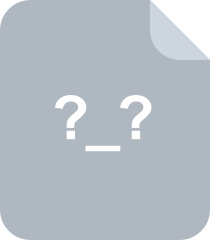
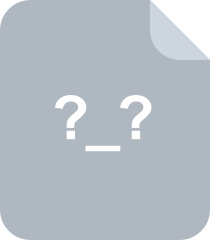
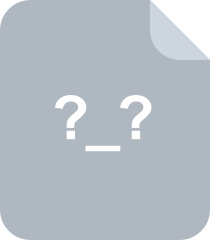
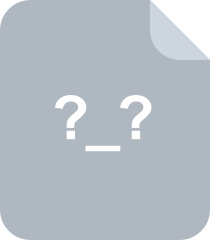
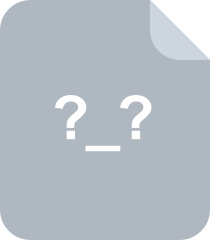
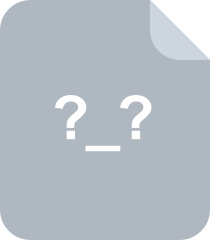
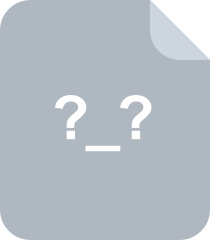
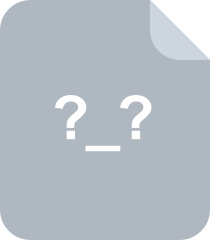
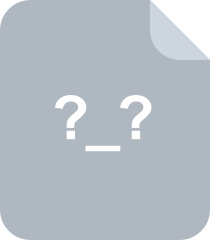
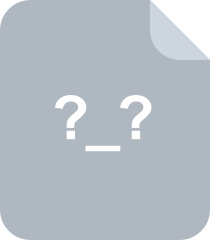
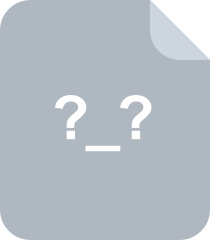
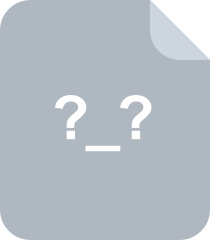
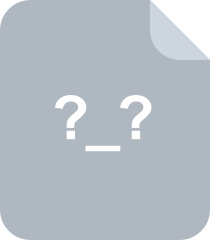
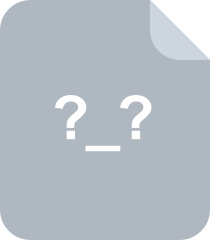
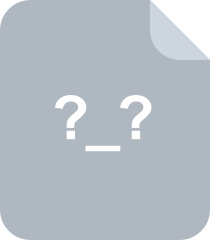
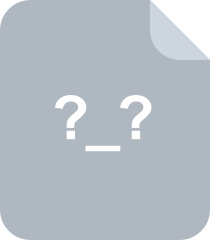
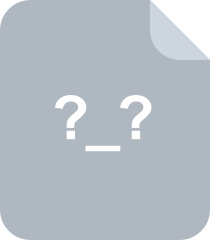
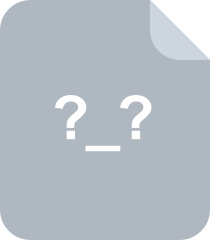
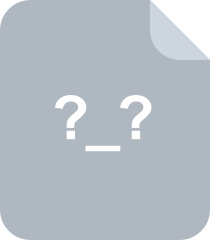
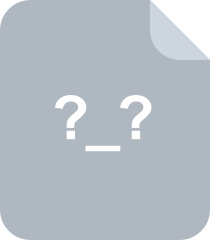
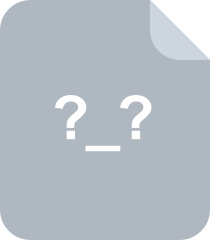
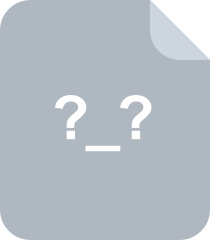
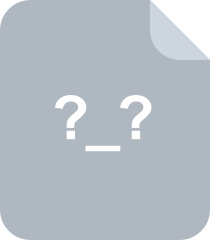
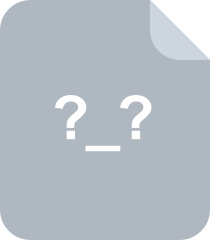
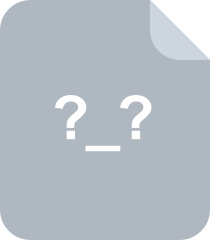
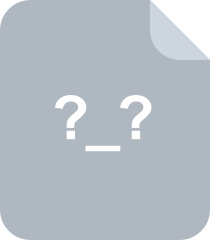
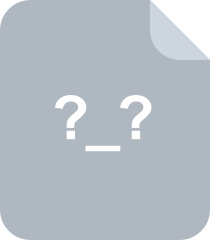
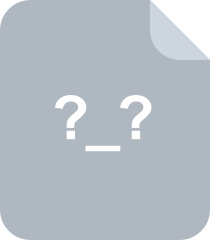
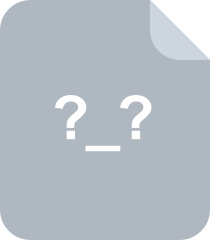
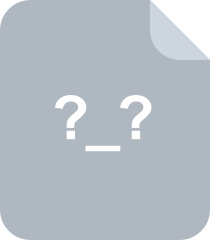
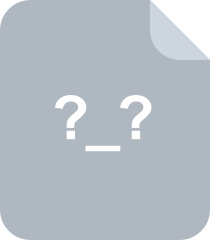
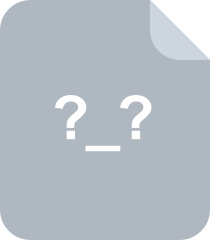
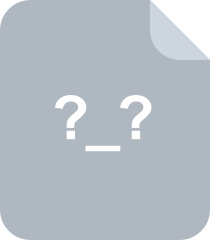
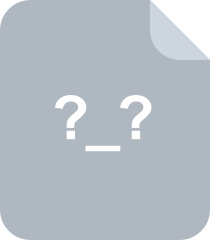
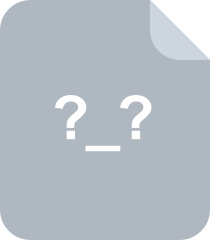
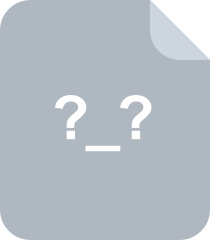
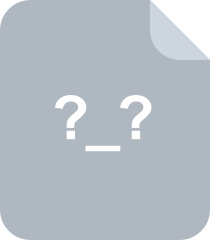
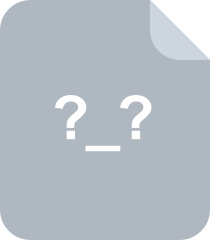
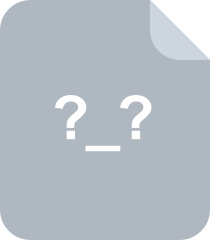
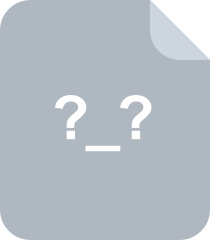
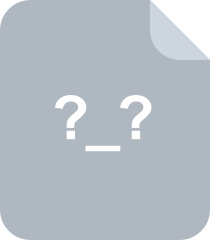
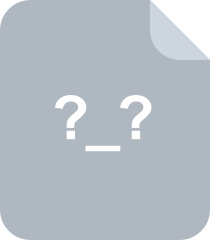
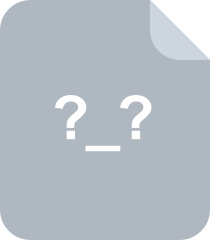
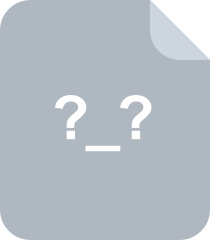
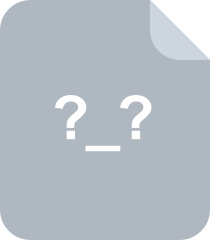
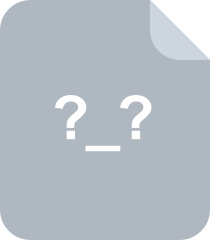
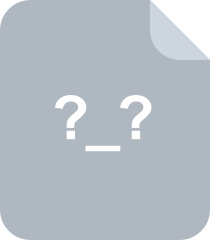
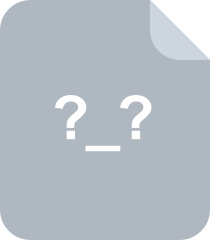
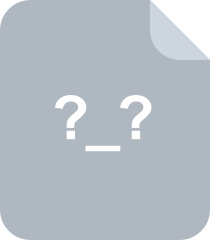
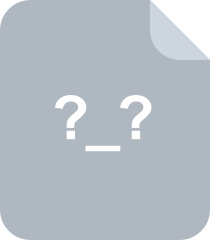
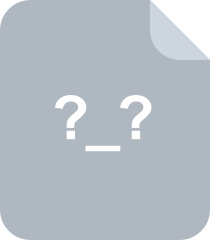
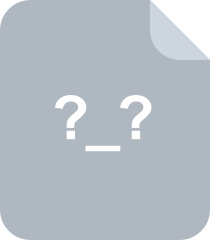
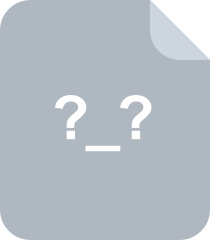
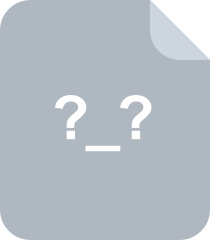
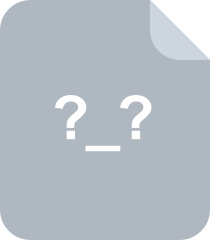
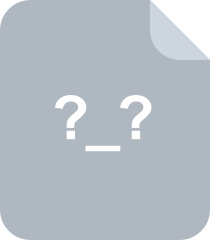
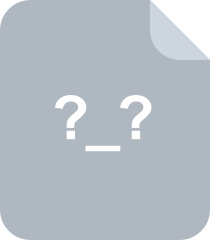
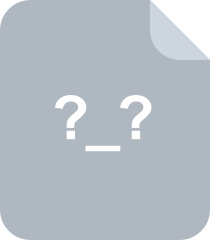
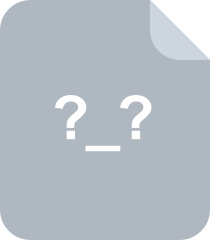
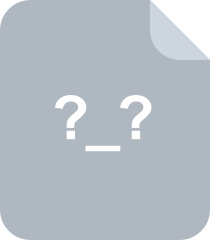
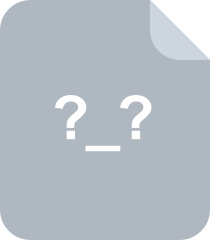
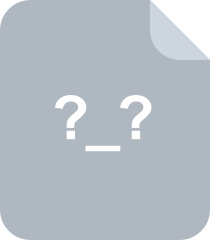
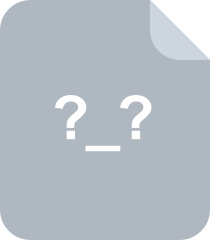
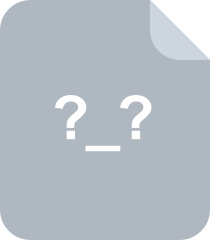
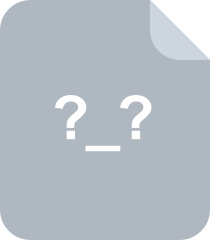
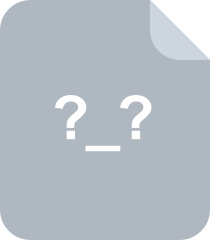
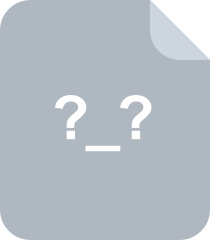
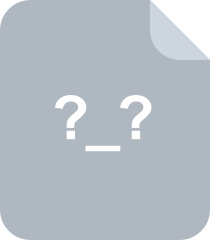
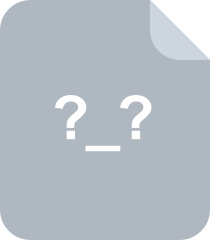
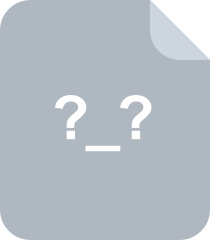
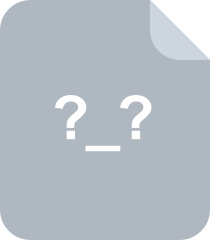
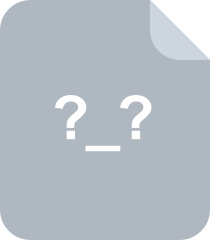
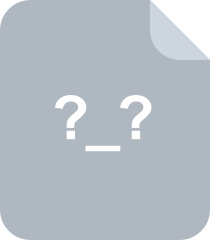
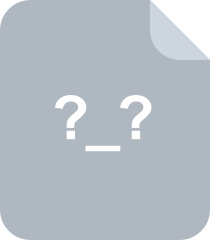
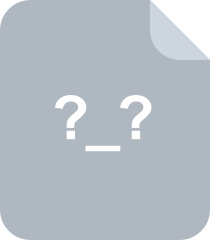
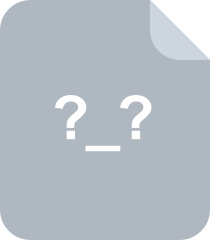
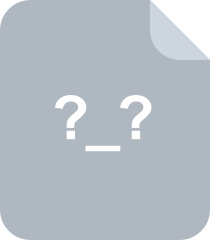
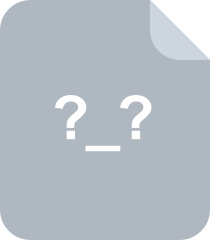
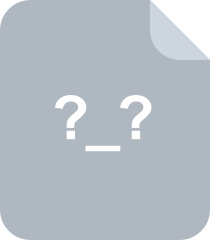
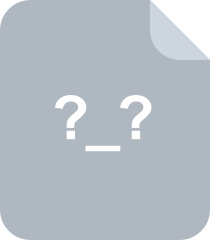
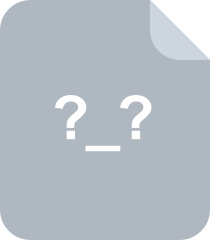
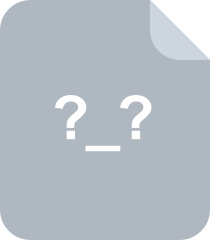
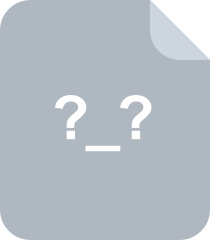
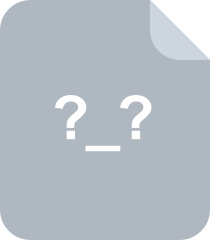
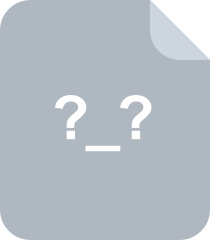
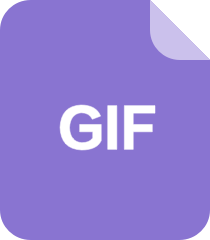
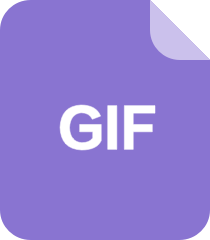
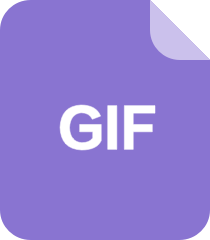
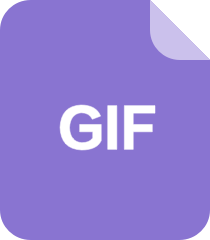
共 419 条
- 1
- 2
- 3
- 4
- 5
资源评论
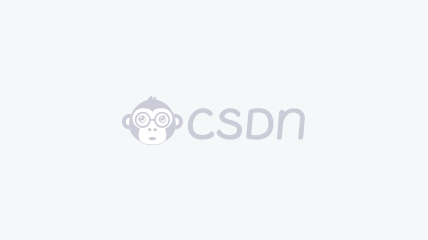

岛上程序猿
- 粉丝: 5301
- 资源: 4179
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

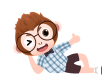
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


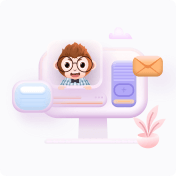
安全验证
文档复制为VIP权益,开通VIP直接复制
