<p align="center">
<a href="https://github.com/geekidea/spring-boot-plus">
<img alt="spring-boot-plus logo" src="https://springboot.plus/img/logo.png">
</a>
</p>
<p align="center">
Everyone can develop projects independently, quickly and efficiently!
</p>
<p align="center">
<a href="https://github.com/geekidea/spring-boot-plus/">
<img alt="spring-boot-plus version" src="https://img.shields.io/badge/spring--boot--plus-1.4.0-blue">
</a>
<a href="https://github.com/spring-projects/spring-boot">
<img alt="spring boot version" src="https://img.shields.io/badge/spring%20boot-2.2.0.RELEASE-brightgreen">
</a>
<a href="https://www.apache.org/licenses/LICENSE-2.0">
<img alt="code style" src="https://img.shields.io/badge/license-Apache%202-4EB1BA.svg?style=flat-square">
</a>
</p>
## What is spring-boot-plus?
### A **easy-to-use**, **high-speed**, **high-efficient**, **feature-rich**, **open source** spring boot scaffolding.
> spring-boot-plus is a background rapid development framework that integrates spring boot common development components.
> Front-end and back-end separation, focusing on back-end services
## Purpose
> Everyone can develop projects independently, quickly and efficiently!
## Repository
#### [GITHUB](https://github.com/geekidea/spring-boot-plus) | [GITEE](https://gitee.com/geekidea/spring-boot-plus)
## Website
#### [springboot.plus](http://springboot.plus)
## Features
- Integrated spring boot common development component set, common configuration, AOP log, etc
- Integrated mybatis-plus fast dao operation
- Quickly generate background code:entity/param/vo/controller/service/mapper/xml
- Integrated swagger2, automatic generation of api documents
- Integrated JWT,Shiro/Spring security permission control
- Integrated Redis、spring cache、ehcache,etc
- Integrated Rabbit/Rocket/Kafka MQ
- Integration HikariCP connection pool, A solid, high-performance, JDBC connection pool at last.
- Integrated Spring Boot Admin, real-time detection of project operation
- Integrate maven-assembly-plugin for different environment package deployment, including startup and restart commands, and extract configuration files to external config directory
## Architecture

### Project Environment
Middleware | Version | Remark
-|-|-
JDK | 1.8+ | JDK1.8 and above |
MySQL | 5.7+ | 5.7 and above |
Redis | 3.2+ | |
### Technology stack
Component| Version | Remark
-|-|-
Spring Boot | 2.2.0.RELEASE | Latest release stable version |
Spring Framework | 5.2.0.RELEASE | Latest release stable version |
Mybatis | 3.5.2 | DAO Framework |
Mybatis Plus | 3.2.0 | mybatis Enhanced framework |
Alibaba Druid | 1.1.20 | Data source |
Fastjson | 1.2.62 | JSON processing toolset |
swagger2 | 2.6.1 | Api document generation tool |
commons-lang3 | 3.9 | Apache language toolkit |
commons-io | 2.6 | Apache IO Toolkit |
commons-codec | 1.13 | Apache Toolkit such as encryption and decryption |
commons-collections4 | 4.4 | Apache collections toolkit |
reflections | 0.9.11 | Reflection Toolkit |
hibernate-validator | 6.0.17.Final | Validator toolkit |
Shiro | 1.4.1 | Permission control |
JWT | 3.8.3 | JSON WEB TOKEN |
hutool-all | 5.0.3 | Common toolset |
lombok | 1.18.10 | Automatically plugs |
mapstruct | 1.3.1.Final | Object property replication tool |
## CHANGELOG
#### [CHANGELOG.md](https://github.com/geekidea/spring-boot-plus/blob/master/CHANGELOG.md)
## Java Docs
#### [Java Api Docs](http://geekidea.io/spring-boot-plus-apidocs/)
## Getting started
### Clone spring-boot-plus
```bash
git clone -b 1.5 https://github.com/geekidea/spring-boot-plus.git
cd spring-boot-plus
```
### Maven Build
> Local environment is used by default, The configuration file:application-local.yml
```bash
mvn clean package -Pdev
```
## 5 Minutes Finish CRUD
### 1. Create Table
```sql
-- ----------------------------
-- Table structure for foo_bar
-- ----------------------------
DROP TABLE IF EXISTS `foo_bar`;
CREATE TABLE `foo_bar`
(
`id` bigint(20) NOT NULL COMMENT 'ID',
`name` varchar(20) NOT NULL COMMENT 'Name',
`foo` varchar(20) DEFAULT NULL COMMENT 'Foo',
`bar` varchar(20) NOT NULL COMMENT 'Bar',
`remark` varchar(200) DEFAULT NULL COMMENT 'Remark',
`state` int(11) NOT NULL DEFAULT '1' COMMENT 'State,0:Disable,1:Enable',
`version` int(11) NOT NULL DEFAULT '0' COMMENT 'Version',
`create_time` timestamp NULL DEFAULT CURRENT_TIMESTAMP COMMENT 'Create Time',
`update_time` timestamp NULL DEFAULT NULL COMMENT 'Update Time',
PRIMARY KEY (`id`)
) ENGINE = InnoDB
DEFAULT CHARSET = utf8mb4
COLLATE = utf8mb4_general_ci COMMENT ='FooBar';
-- ----------------------------
-- Records of foo_bar
-- ----------------------------
INSERT INTO foo_bar (id, name, foo, bar, remark, state, version, create_time, update_time)
VALUES (1, 'FooBar', 'foo', 'bar', 'remark...', 1, 0, '2019-11-01 14:05:14', null);
INSERT INTO foo_bar (id, name, foo, bar, remark, state, version, create_time, update_time)
VALUES (2, 'HelloWorld', 'hello', 'world', null, 1, 0, '2019-11-01 14:05:14', null);
```
### 2. Generator CRUD CODE
> Modify database info
> Modify module name / author / table name / primary key id
```text
/src/test/java/io/geekidea/springbootplus/test/SpringBootPlusGenerator.java
```
```java
/**
* spring-boot-plus Code Generator
*
* @author geekidea
* @date 2019-10-22
**/
public class SpringBootPlusGenerator {
public static void main(String[] args) {
CodeGenerator codeGenerator = new CodeGenerator();
// Common configuration
// Database configuration
codeGenerator
.setUserName("root")
.setPassword("root")
.setDriverName("com.mysql.jdbc.Driver")
.setDriverUrl("jdbc:mysql://localhost:3306/spring_boot_plus?useUnicode=true&characterEncoding=UTF-8&useSSL=false");
// Configuration package information
codeGenerator
.setProjectPackagePath("io/geekidea/springbootplus")
.setParentPackage("io.geekidea.springbootplus");
// Configuration of component author, etc.
codeGenerator
.setModuleName("foobar")
.setAuthor("geekidea")
.setPkIdColumnName("id");
// Generation strategy
codeGenerator
.setGeneratorStrategy(CodeGenerator.GeneratorStrategy.ALL)
.setPageListOrder(true)
.setParamValidation(true);
// Customize which files are generated automatically
codeGenerator
.setGeneratorEntity(true)
.setGeneratorPageParam(true)
.setGeneratorQueryVo(true);
// Generate business related codes
codeGenerator
.setGeneratorController(true)
.setGeneratorService(true)
.setGeneratorServiceImpl(true)
.setGeneratorMapper(true)
.setGeneratorMapperXml(true);
// Generated RequiresPermissions Annotation
codeGenerator.setRequiresPermissions(false);
// Overwrite existing file or not
codeGenerator.setFileOverride(true);
// Initialize common variables
codeGenerator.init();
// Table array to be generated
String[] tables = {
"foo_bar"
};
// Cycle generation
for (String table : tables) {
// Set the name of the table to be generated
codeGenerator.setTableName(table);
// Generate code
codeGenerator.generator();
}
}
}
```
> Generated code structure
```text
/src/main/java/io/geekidea/springbootplus/foobar
```
```text
└── foobar
├── controller
│
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
(基于android的毕业设计)学生选课系统App(源码+演示视频+部署说明) 【项目技术】 android+java 【实现功能】 1.用户登陆和注册 2.学生功能: 学生成功登录系统后,可以通过课程管理中的我要选课进入选课界面,学生可以浏览到所有教师开出的课程信息,从中选择自己喜欢的课程到自己的课表中,学生选完课程后,对应的开课教师和管理员也可以查看到学生的选课信息。应用还支持课程退订、选课信息查看和课程成绩查询等 3.教师功能: 教师登陆系统后,可以编辑课程信息机课表,发至管理员通过后即可供学生浏览选择,并支持对查看已申请课程的学生信息及考试成绩。 4.管理员后台: 后台管理针对管理系统管理,主要实现对课程的管理、学生的管理和老师的管理。管理员可以查看并通过教师提交的课程申请,还能对课程进行修改。
资源推荐
资源详情
资源评论
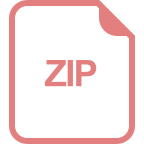
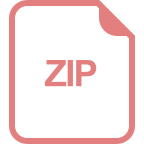
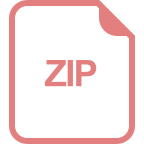
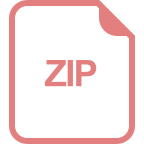
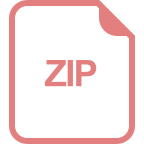
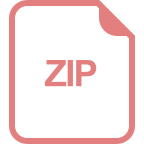
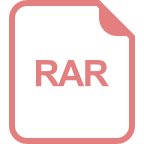
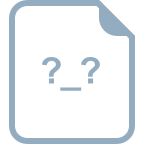
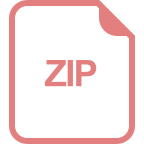
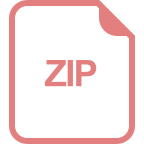
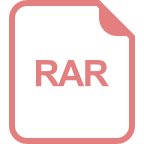
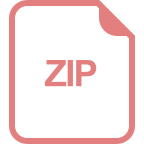
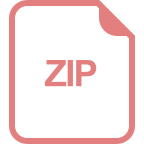
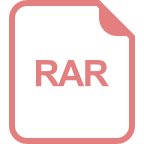
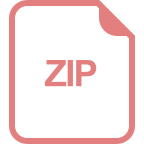
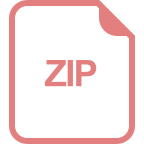
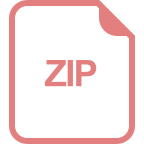
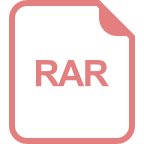
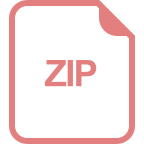
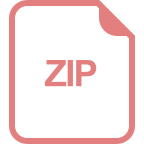
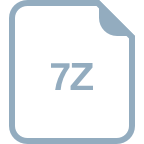
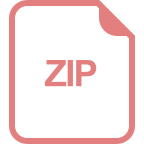
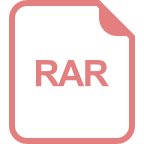
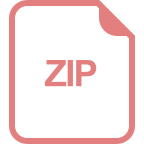
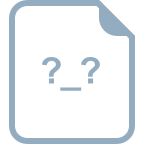
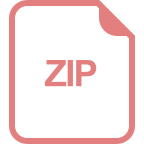
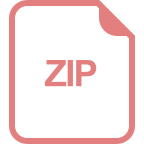
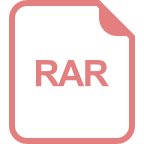
收起资源包目录

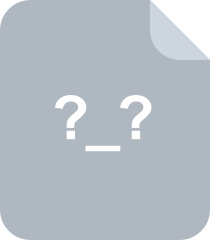
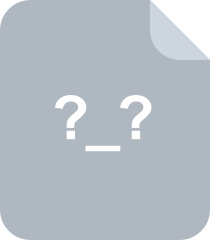
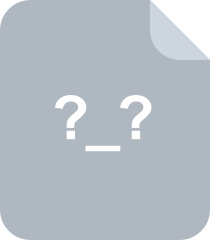
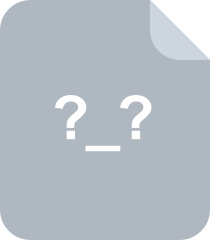
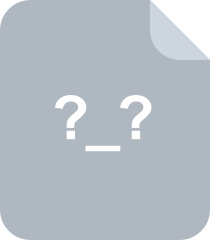
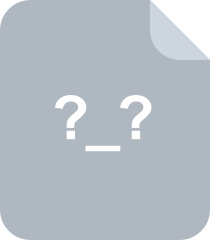
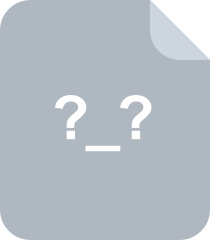
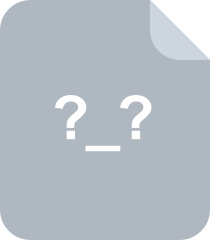
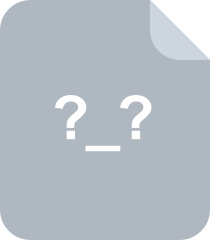
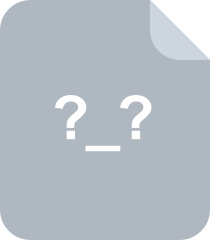
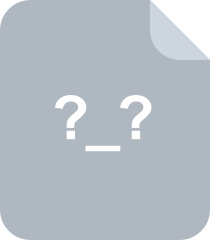
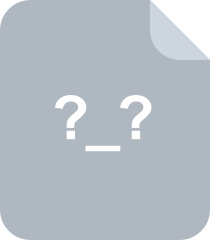
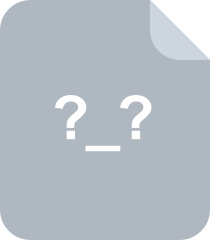
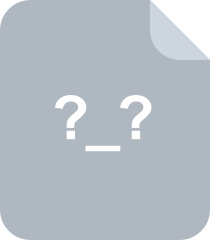
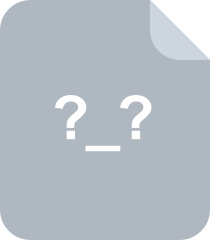
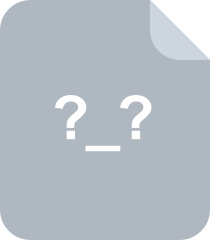
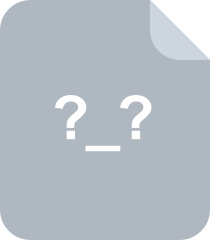
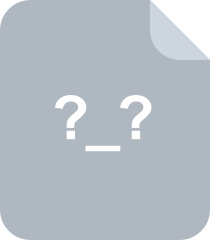
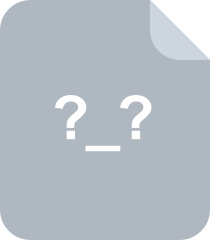
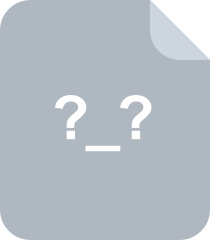
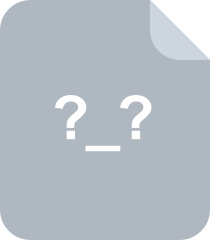
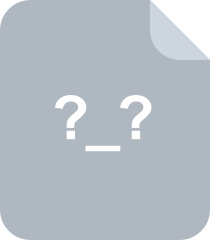
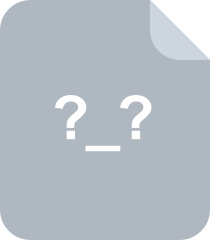
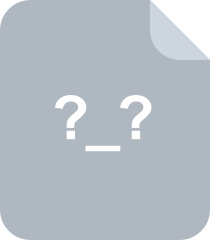
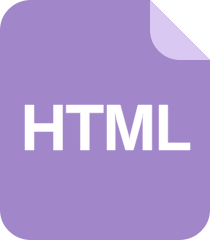
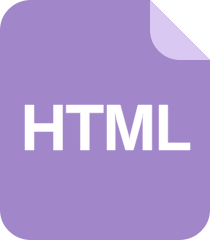
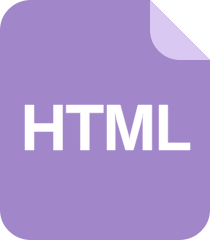
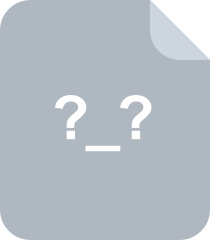
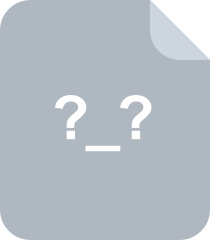
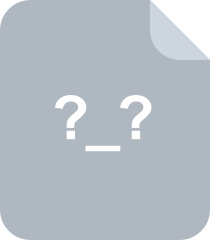
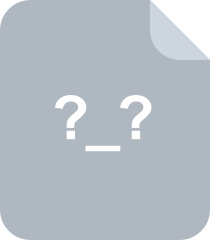
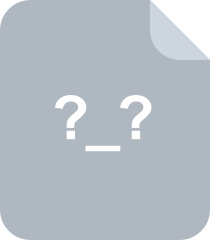
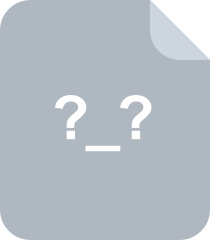
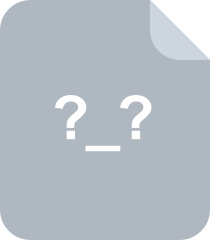
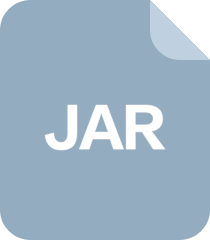
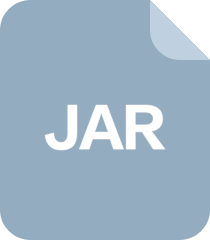
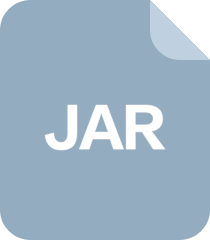
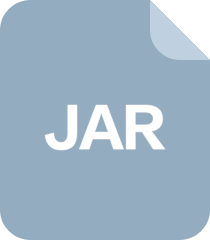
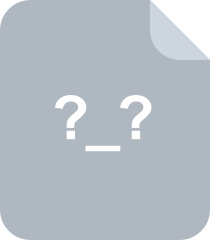
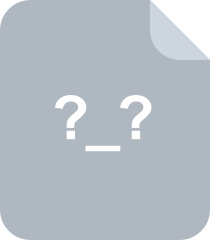
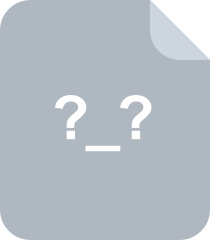
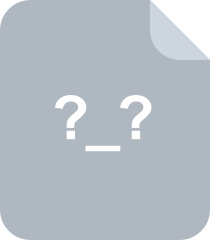
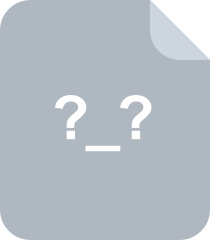
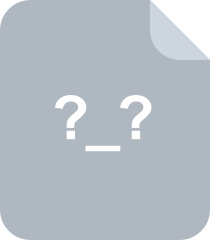
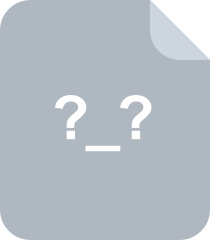
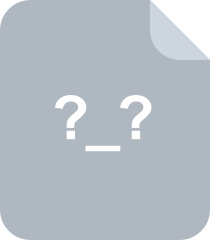
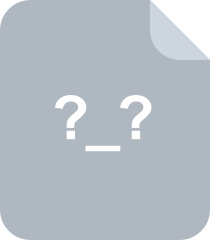
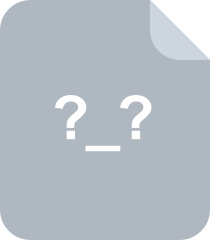
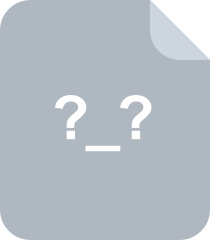
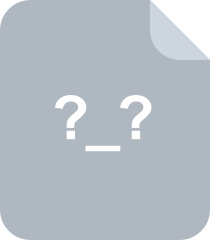
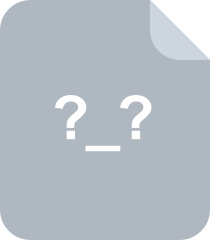
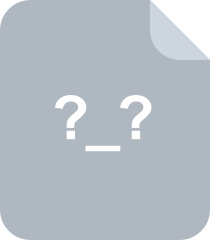
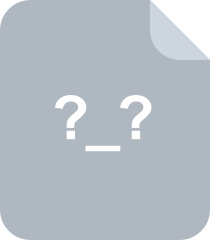
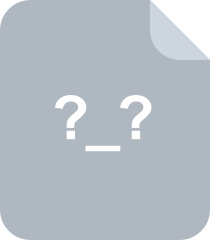
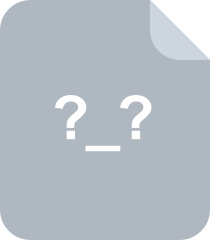
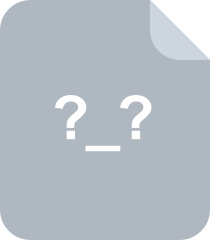
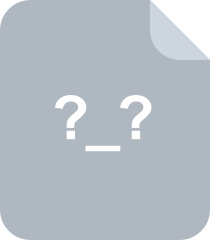
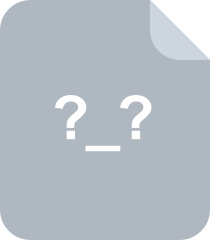
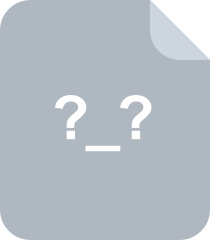
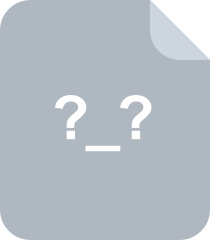
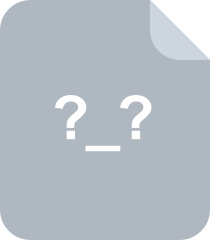
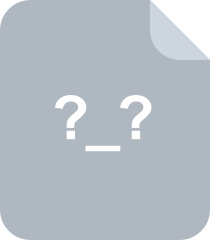
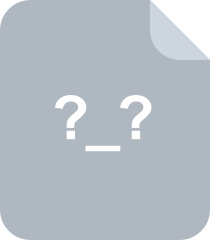
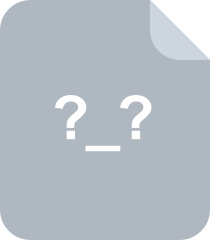
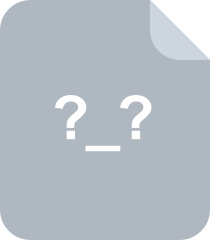
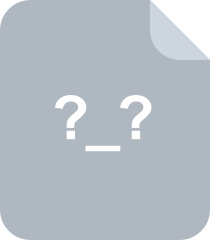
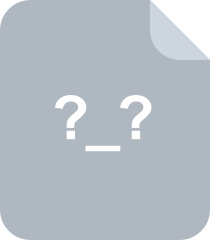
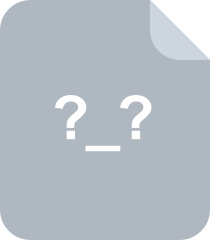
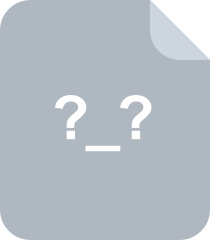
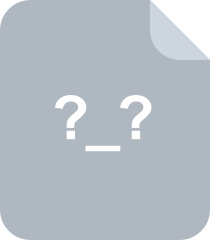
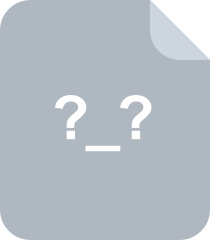
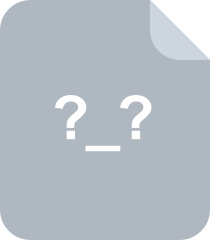
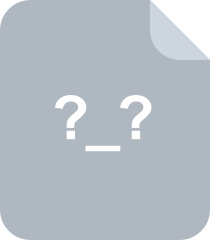
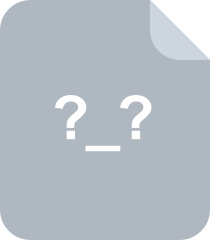
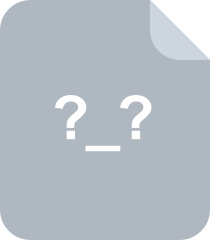
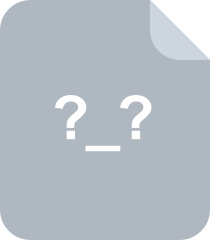
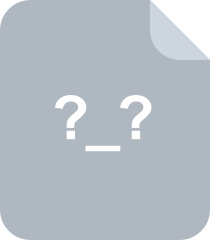
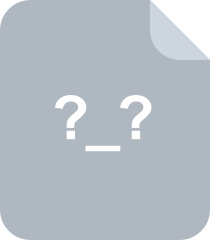
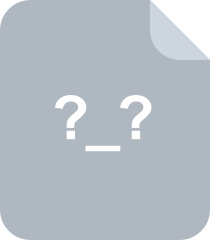
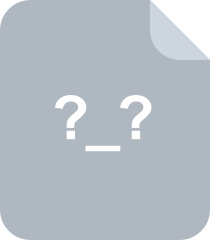
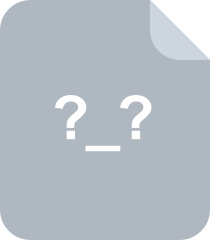
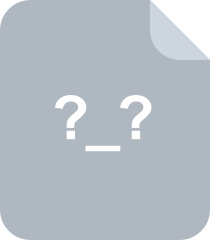
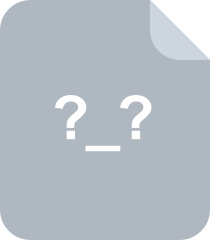
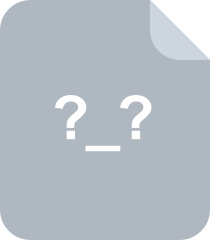
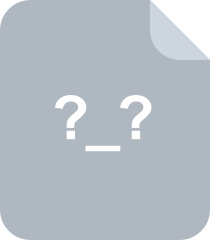
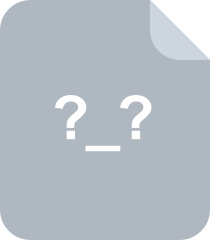
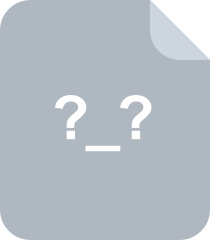
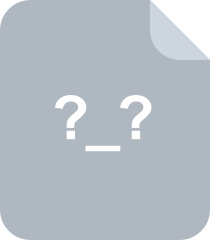
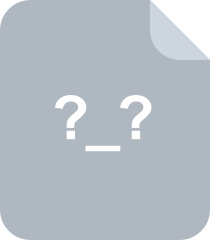
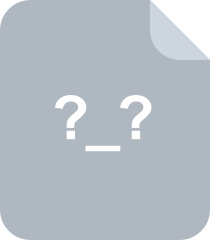
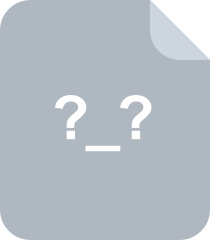
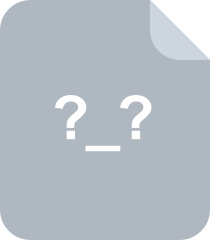
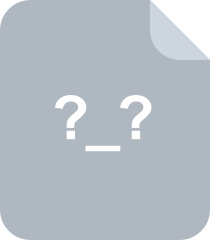
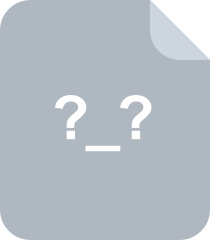
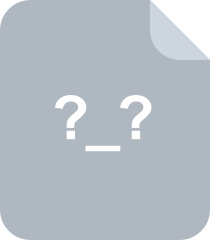
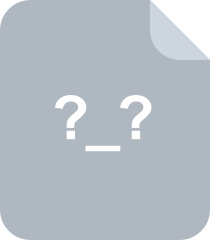
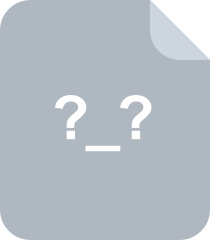
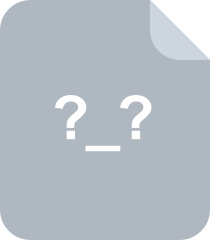
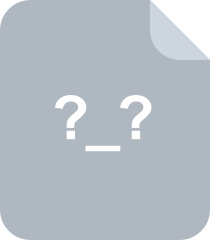
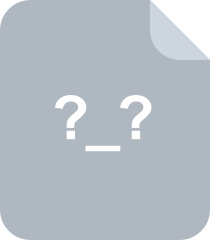
共 1599 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
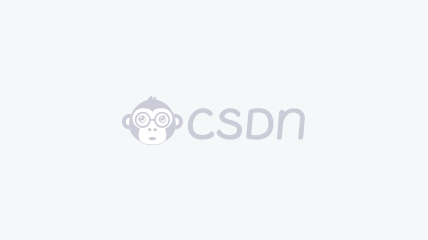
- KlayChi2023-05-31资源质量不错,和资源描述一致,内容详细,对我很有用。

岛上程序猿
- 粉丝: 5204
- 资源: 4157
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

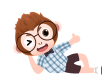
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


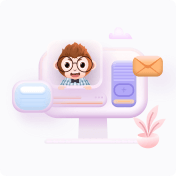
安全验证
文档复制为VIP权益,开通VIP直接复制
