<p align="center">
<img src="https://cloud.githubusercontent.com/assets/835857/14581711/ba623018-0436-11e6-8fce-d2ccd4d379c9.gif">
</p>
# JavaScript Cookie [](https://travis-ci.com/js-cookie/js-cookie) [](https://www.browserstack.com/automate/public-build/b3VDaHAxVDg0NDdCRmtUOWg0SlQzK2NsRVhWTjlDQS9qdGJoak1GMzJiVT0tLVhwZHNvdGRoY284YVRrRnI3eU1JTnc9PQ==--5e88ffb3ca116001d7ef2cfb97a4128ac31174c2) [](https://standardjs.com) [](https://codeclimate.com/github/js-cookie/js-cookie) [](https://www.npmjs.com/package/js-cookie) [](https://www.npmjs.com/package/js-cookie) [](https://www.jsdelivr.com/package/npm/js-cookie)
A simple, lightweight JavaScript API for handling cookies
- Works in [all](https://www.browserstack.com/automate/public-build/b3VDaHAxVDg0NDdCRmtUOWg0SlQzK2NsRVhWTjlDQS9qdGJoak1GMzJiVT0tLVhwZHNvdGRoY284YVRrRnI3eU1JTnc9PQ==--5e88ffb3ca116001d7ef2cfb97a4128ac31174c2) browsers
- Accepts [any](#encoding) character
- [Heavily](test) tested
- No dependency
- Supports ES modules
- Supports AMD/CommonJS
- [RFC 6265](https://tools.ietf.org/html/rfc6265) compliant
- Useful [Wiki](https://github.com/js-cookie/js-cookie/wiki)
- Enable [custom encoding/decoding](#converters)
- **< 800 bytes** gzipped!
**ðð If you're viewing this at https://github.com/js-cookie/js-cookie, you're reading the documentation for the master branch.
[View documentation for the latest release.](https://github.com/js-cookie/js-cookie/tree/latest#readme) ðð**
## Installation
### NPM
JavaScript Cookie supports [npm](https://www.npmjs.com/package/js-cookie) under the name `js-cookie`.
```
$ npm i js-cookie
```
The npm package has a `module` field pointing to an ES module variant of the library, mainly to provide support for ES module aware bundlers, whereas its `browser` field points to an UMD module for full backward compatibility.
### Direct download
Starting with version 3 [releases](https://github.com/js-cookie/js-cookie/releases) are distributed with two variants of this library, an ES module as well as an UMD module.
Note the different extensions: `.mjs` denotes the ES module, whereas `.js` is the UMD one.
Example for how to load the ES module in a browser:
```html
<script type="module" src="/path/to/js.cookie.mjs"></script>
<script type="module">
import Cookies from '/path/to/js.cookie.mjs'
Cookies.set('foo', 'bar')
</script>
```
_Not all browsers support ES modules natively yet_. For this reason the npm package/release provides both the ES and UMD module variant and you may want to include the ES module along with the UMD fallback to account for this:
```html
<script type="module" src="/path/to/js.cookie.mjs"></script>
<script nomodule defer src="/path/to/js.cookie.js"></script>
```
Here we're loading the nomodule script in a deferred fashion, because ES modules are deferred by default. This may not be strictly necessary depending on how you're using the library.
### CDN
Alternatively, include it via [jsDelivr CDN](https://www.jsdelivr.com/package/npm/js-cookie).
## ES Module
Example for how to import the ES module from another module:
```javascript
import Cookies from 'js-cookie'
Cookies.set('foo', 'bar')
```
## Basic Usage
Create a cookie, valid across the entire site:
```javascript
Cookies.set('name', 'value')
```
Create a cookie that expires 7 days from now, valid across the entire site:
```javascript
Cookies.set('name', 'value', { expires: 7 })
```
Create an expiring cookie, valid to the path of the current page:
```javascript
Cookies.set('name', 'value', { expires: 7, path: '' })
```
Read cookie:
```javascript
Cookies.get('name') // => 'value'
Cookies.get('nothing') // => undefined
```
Read all visible cookies:
```javascript
Cookies.get() // => { name: 'value' }
```
_Note: It is not possible to read a particular cookie by passing one of the cookie attributes (which may or may not
have been used when writing the cookie in question):_
```javascript
Cookies.get('foo', { domain: 'sub.example.com' }) // `domain` won't have any effect...!
```
The cookie with the name `foo` will only be available on `.get()` if it's visible from where the
code is called; the domain and/or path attribute will not have an effect when reading.
Delete cookie:
```javascript
Cookies.remove('name')
```
Delete a cookie valid to the path of the current page:
```javascript
Cookies.set('name', 'value', { path: '' })
Cookies.remove('name') // fail!
Cookies.remove('name', { path: '' }) // removed!
```
_IMPORTANT! When deleting a cookie and you're not relying on the [default attributes](#cookie-attributes), you must pass the exact same path and domain attributes that were used to set the cookie:_
```javascript
Cookies.remove('name', { path: '', domain: '.yourdomain.com' })
```
_Note: Removing a nonexistent cookie neither raises any exception nor returns any value._
## Namespace conflicts
If there is any danger of a conflict with the namespace `Cookies`, the `noConflict` method will allow you to define a new namespace and preserve the original one. This is especially useful when running the script on third party sites e.g. as part of a widget or SDK.
```javascript
// Assign the js-cookie api to a different variable and restore the original "window.Cookies"
var Cookies2 = Cookies.noConflict()
Cookies2.set('name', 'value')
```
_Note: The `.noConflict` method is not necessary when using AMD or CommonJS, thus it is not exposed in those environments._
## Encoding
This project is [RFC 6265](http://tools.ietf.org/html/rfc6265#section-4.1.1) compliant. All special characters that are not allowed in the cookie-name or cookie-value are encoded with each one's UTF-8 Hex equivalent using [percent-encoding](http://en.wikipedia.org/wiki/Percent-encoding).
The only character in cookie-name or cookie-value that is allowed and still encoded is the percent `%` character, it is escaped in order to interpret percent input as literal.
Please note that the default encoding/decoding strategy is meant to be interoperable [only between cookies that are read/written by js-cookie](https://github.com/js-cookie/js-cookie/pull/200#discussion_r63270778). To override the default encoding/decoding strategy you need to use a [converter](#converters).
_Note: According to [RFC 6265](https://tools.ietf.org/html/rfc6265#section-6.1), your cookies may get deleted if they are too big or there are too many cookies in the same domain, [more details here](https://github.com/js-cookie/js-cookie/wiki/Frequently-Asked-Questions#why-are-my-cookies-being-deleted)._
## Cookie Attributes
Cookie attribute defaults can be set globally by creating an instance of the api via `withAttributes()`, or individually for each call to `Cookies.set(...)` by passing a plain object as the last argument. Per-call attributes override the default attributes.
### expires
Define when the cookie will be removed. Value must be a [`Number`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Number) which will be interpreted as days from time of creation or a [`Date`](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Date) instance. If omitted, the cookie becomes a session cookie.
To create a cookie that expires in less than a day, you can check the [FAQ on the Wiki](https://gi
没有合适的资源?快使用搜索试试~ 我知道了~
一个基于Springboot+Vue的前后端分离记账系统Count.zip
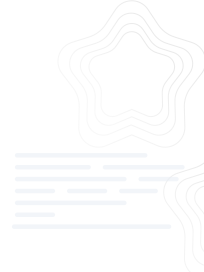
共176个文件
js:24个
java:21个
vue:19个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 87 浏览量
2024-05-19
11:47:47
上传
评论
收藏 62.83MB ZIP 举报
温馨提示
该项目利用了基于springboot + vue + mysql的开发模式框架实现的课设系统,包括了项目的源码资源、sql文件、相关指引文档等等。 【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。 【技术】 Java、Python、Node.js、Spring Boot、Django、Express、MySQL、PostgreSQL、MongoDB、React、Angular、Vue、Bootstrap、Material-UI、Redis、Docker、Kubernetes
资源推荐
资源详情
资源评论
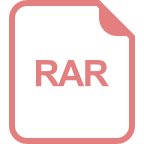
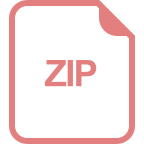
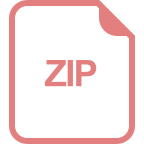
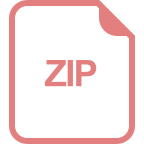
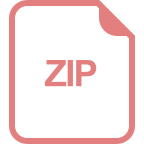
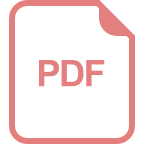
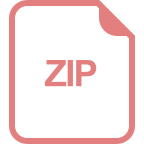
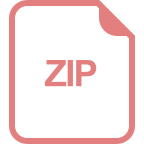
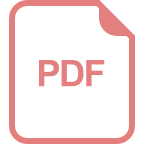
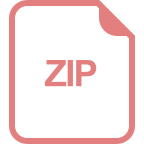
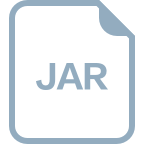
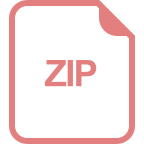
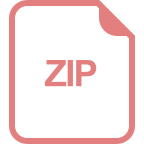
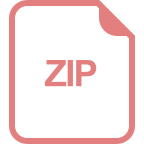
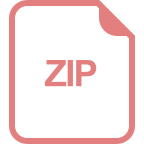
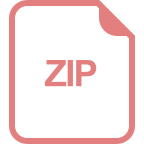
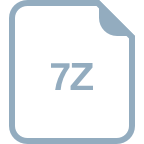
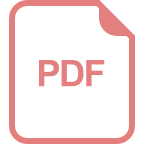
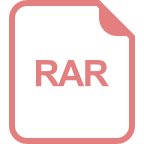
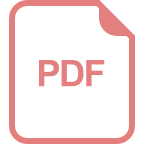
收起资源包目录

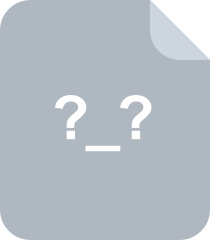
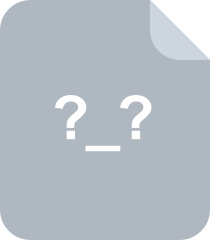
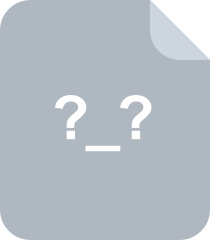
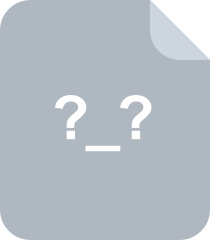
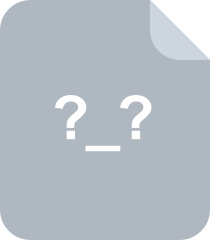
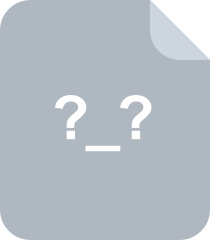
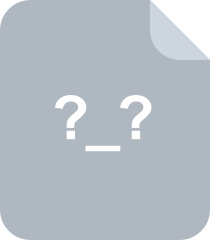
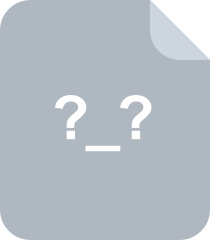
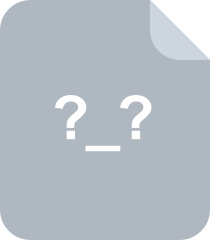
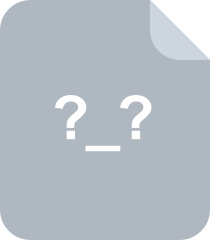
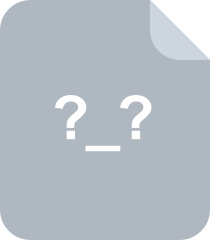
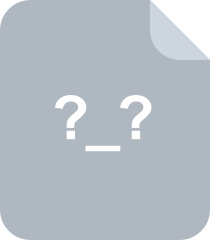
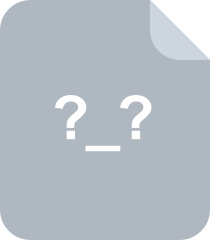
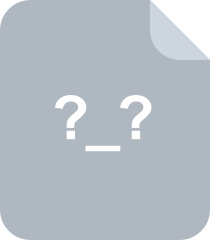
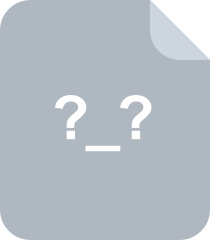
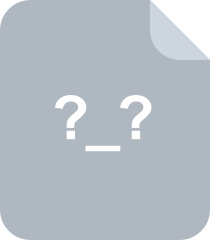
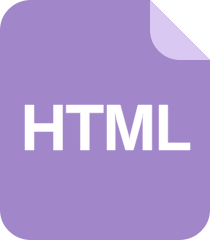
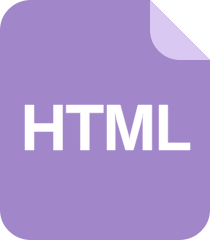
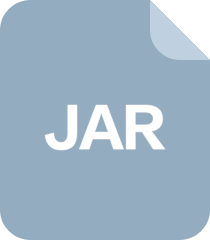
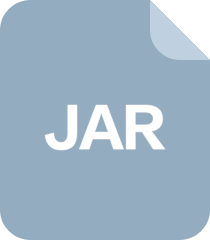
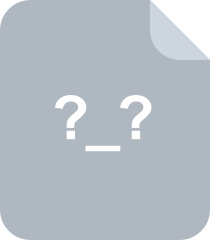
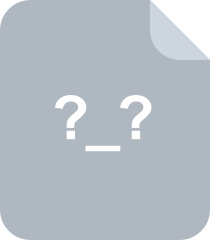
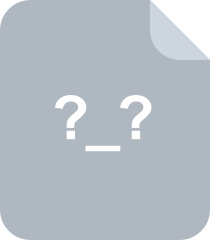
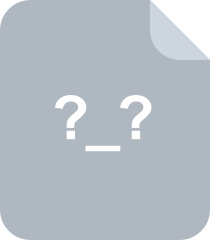
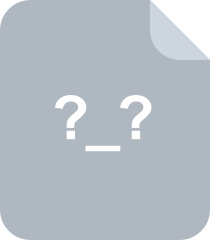
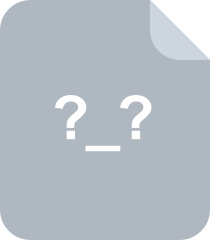
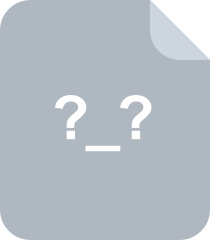
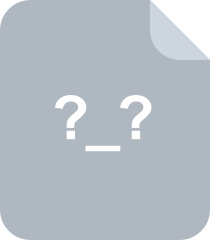
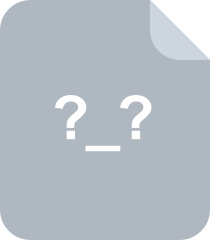
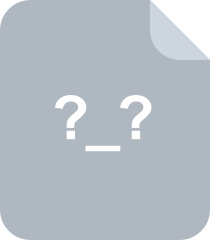
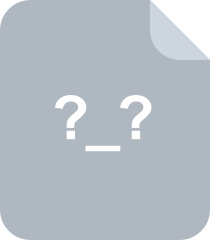
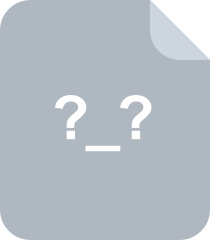
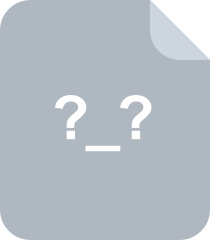
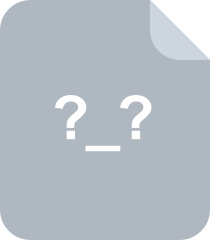
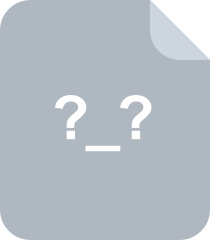
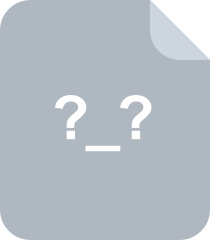
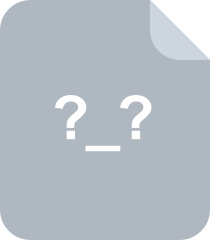
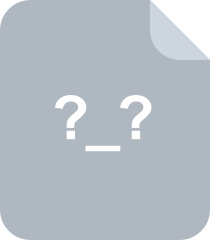
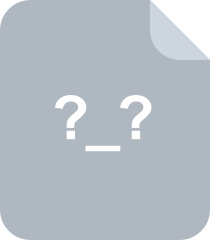
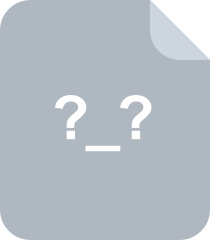
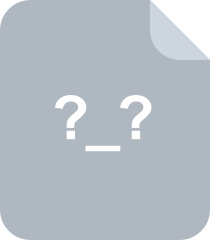
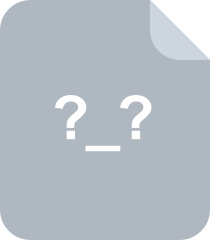
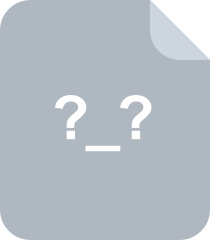
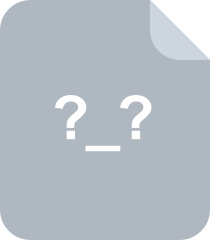
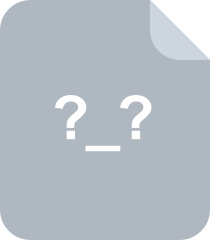
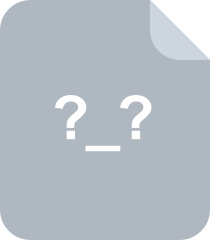
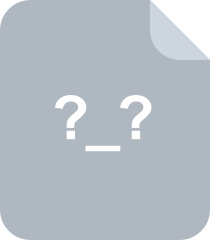
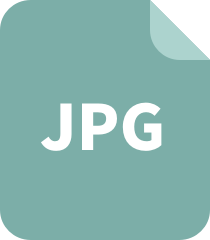
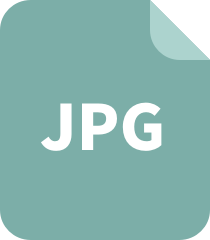
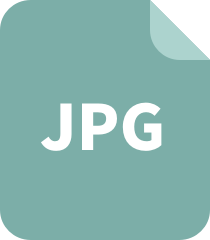
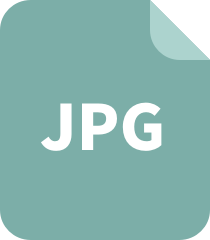
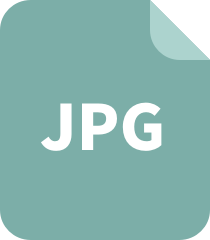
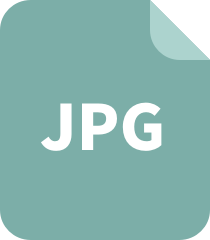
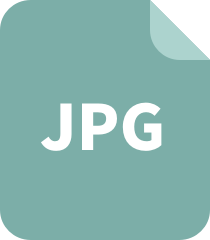
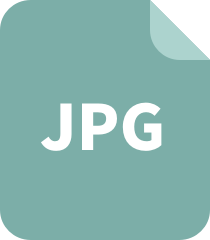
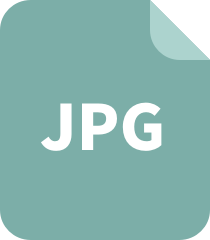
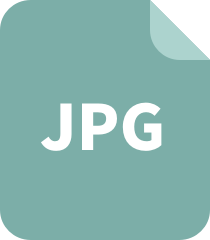
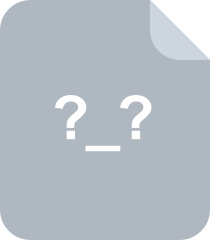
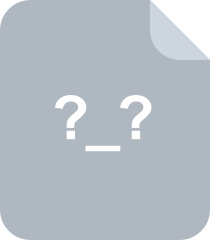
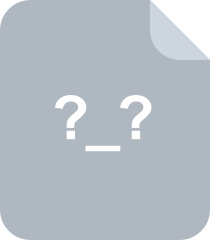
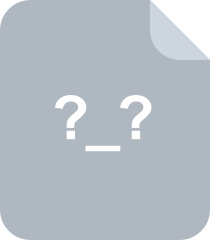
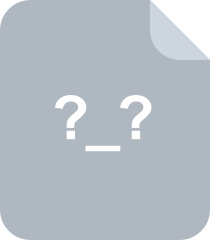
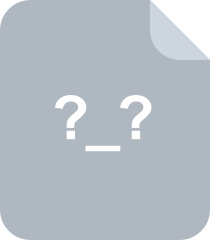
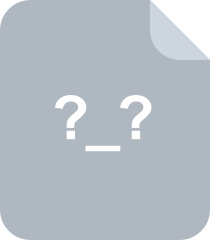
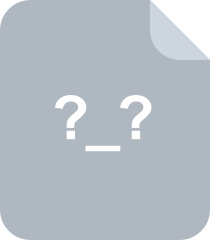
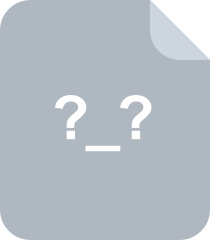
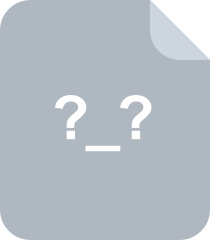
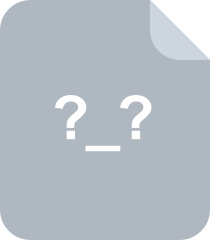
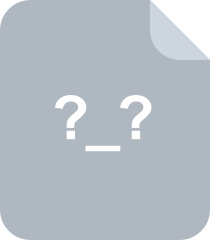
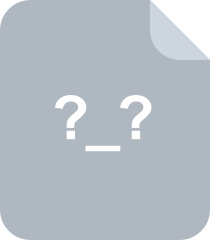
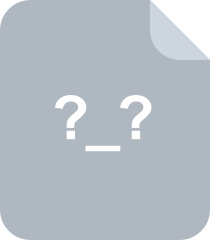
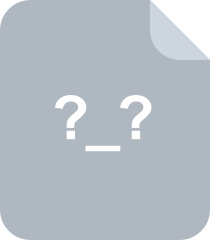
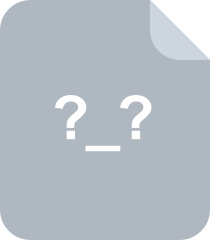
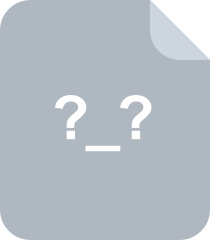
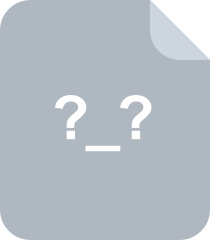
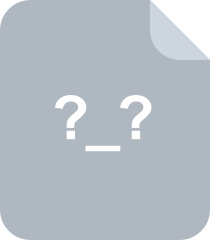
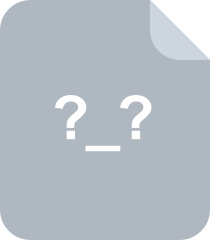
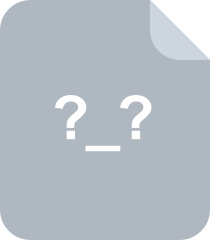
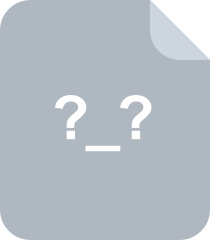
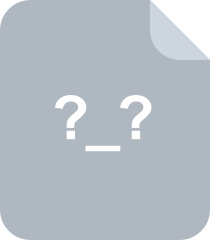
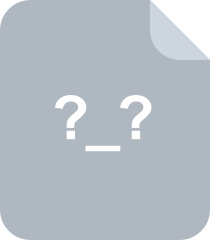
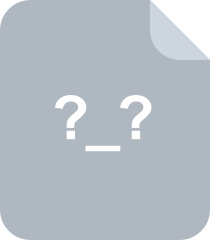
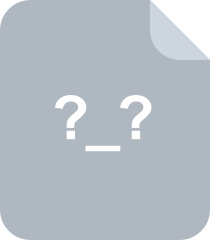
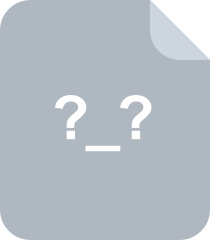
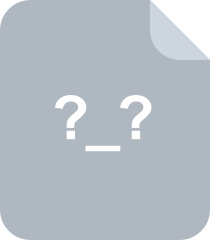
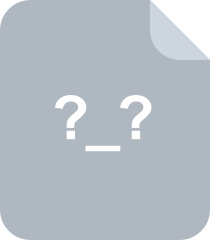
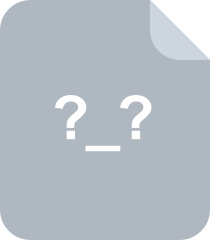
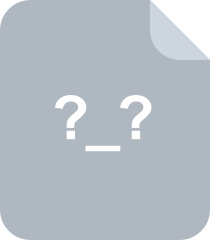
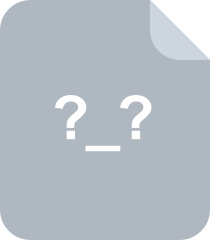
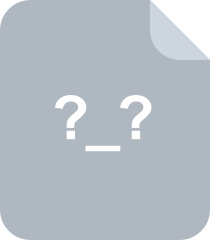
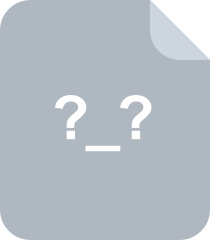
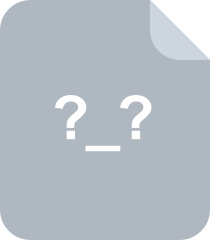
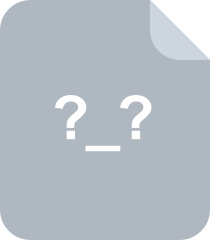
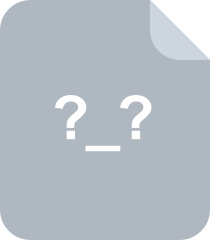
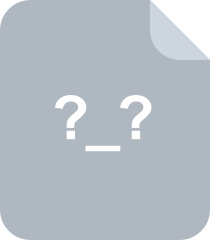
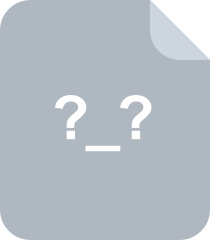
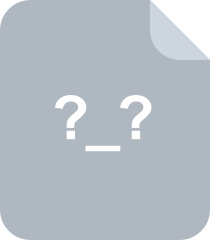
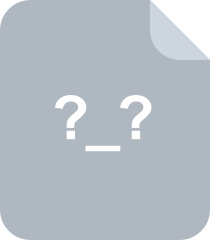
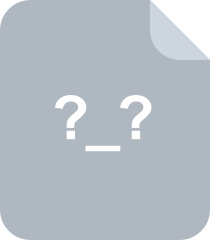
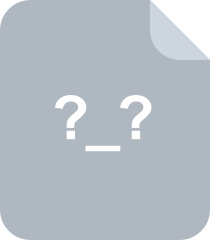
共 176 条
- 1
- 2
资源评论
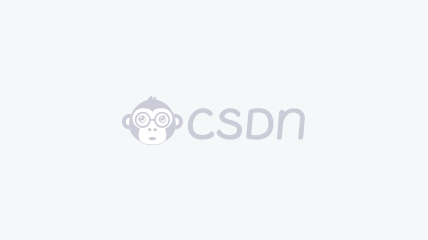

枫蜜柚子茶
- 粉丝: 7540
- 资源: 5155
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

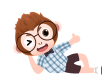
最新资源
- DS18B20温度传感器实战应用与源码解析.zip
- python-leetcode面试题解之第384题打乱数组.zip
- python-leetcode面试题解之第383题赎金信.zip
- python-leetcode面试题解之第380题O1插入删除和获取随机元素.zip
- python-leetcode面试题解之第375题猜数字大小II.zip
- python-leetcode面试题解之第374题猜数字大小.zip
- python-leetcode面试题解之第373题查找和最小的K对数字.zip
- python-leetcode面试题解之第372题超级次方.zip
- python-leetcode面试题解之第371题两整数之和.zip
- python-leetcode面试题解之第370题区间加法.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


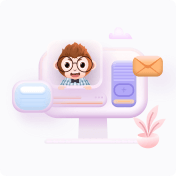
安全验证
文档复制为VIP权益,开通VIP直接复制
