package com.biemo.cloud.bbs.core.upload;
import cn.hutool.core.util.StrUtil;
import com.alibaba.fastjson.JSON;
import com.biemo.cloud.bbs.core.upload.bean.UploadBean;
import com.biemo.cloud.bbs.modular.context.BiemoLoginContext;
import com.biemo.cloud.bbs.modular.domain.BAttachment;
import com.biemo.cloud.bbs.modular.domain.BUser;
import com.biemo.cloud.bbs.modular.service.IBAttachmentService;
import com.biemo.cloud.bbs.utils.ControllerUtil;
import com.biemo.cloud.bbs.utils.PathUtil;
import com.biemo.cloud.bbs.utils.QiniuUtil;
import com.biemo.cloud.core.util.DateUtils;
import com.biemo.cloud.core.util.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import java.io.BufferedOutputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.nio.charset.Charset;
import java.util.Date;
import java.util.UUID;
import static com.sun.org.apache.xerces.internal.impl.dv.util.Base64.decode;
/**
* Description:
*
* @author BIEMO
* @create 2017-07-19
**/
@Component
@Scope("prototype")
public class UploadComponent {
@Value("${virtual.path.enable}")
String enableVirtualPath;
@Value("${virtual.path.windows}")
String windowsUploadPath;
@Value("${virtual.path.linux}")
String linuxUploadPath;
@Value("${qiniu.accessKey}")
private String accessKey;
@Value("${qiniu.secretKey}")
private String secretKey;
@Value("${qiniu.bucketname}")
private String bucketname;
@Value("${qiniu.cdnDomain}")
private String domain;
@Value("${qiniu.upload.enable}")
private String qiniuUpload;
@Value("virtual.path.enableFullPath")
private String enableFullPath = "false";
@Autowired
IBAttachmentService attachmentService;
public UploadBean uploadFile(MultipartFile multipartFile, HttpServletRequest request){
if (!multipartFile.isEmpty()) {
BAttachment attachment = new BAttachment();
/** 获取用户会话 **/
BUser userVo = BiemoLoginContext.me().getCurrentUser();
if(userVo!=null) {
attachment.setUserId(userVo.getId().toString());
attachment.setUserName(userVo.getUsername());
}
attachment.setUploadDate(new Date());
attachment.setUploadIp(ControllerUtil.getRemoteAddress(request));
attachment.setFileSize(Float.valueOf(multipartFile.getSize())/1024);
attachment.setFileExtname(multipartFile.getContentType());
attachment.setFileKey(UUID.randomUUID().toString().replace("-",""));
attachment.setOriginalFileName(multipartFile.getOriginalFilename());
/*创建uploadBean*/
UploadBean result = new UploadBean();
String fileType = this.getFileType(attachment.getOriginalFileName());
String fileName = this.getFileName(fileType) ;
String newName =this.getNewFileName(fileName);
if (!Boolean.parseBoolean(qiniuUpload)) {
File file = new File(this.getUploadPath() + newName);
/*如果不存在就创建*/
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
}
try {
this.writeFile(multipartFile.getBytes(), file);
attachment.setFilePath(newName);
attachment.setFileName(fileName);
if(Boolean.parseBoolean(enableFullPath)) {
result.setUrl(request.getScheme() + "://" + ControllerUtil.getDomain() + "/res/" + attachment.getFileKey() + "." + fileType);
}else {
result.setUrl("/res/" + attachment.getFileKey() + "." + fileType);
}
attachmentService.save(attachment);
} catch (Exception e) {
throw new RuntimeException(e.getMessage());
}
}else {
String qiniuFileResult = QiniuUtil.upload(accessKey, secretKey, bucketname, multipartFile);
if (!StrUtil.isBlank(qiniuFileResult)) {
String fileKey = JSON.parseObject(qiniuFileResult).getString("key");
String fileUrl = domain + "/" + fileKey;
//result.setPreview(fileUrl+"?imageView2/0/w/180/h/180/q/75");
result.setPreview(fileUrl+"?imageMogr2/thumbnail/180x180/format/webp/blur/1x0/quality/75");
if (com.biemo.cloud.core.util.StringUtils.getExtensionName(fileName).equals("jpg") || StringUtils.getExtensionName(fileName).equals("JPG") || StringUtils.getExtensionName(fileName).equals("png") || StringUtils.getExtensionName(fileName).equals("PNG") || StringUtils.getExtensionName(fileName).equals("jpeg") ||StringUtils.getExtensionName(fileName).equals("JPEG")) {
fileUrl += "?imageslim";
}
result.setUrl(fileUrl);
}
}
return result;
}else{
throw new RuntimeException("上传文件不能为空!");
}
}
public String getUploadPath(){
String os = System.getProperty("os.name");
String uploadPath = enableVirtualPath.equals("true")
?(os.toLowerCase().startsWith("win")
?windowsUploadPath
:linuxUploadPath)
: PathUtil.getRootClassPath()+ File.separator + "static";
return uploadPath;
}
public Charset getCharset(){
String os = System.getProperty("os.name");
Charset charset = os.toLowerCase().startsWith("win")
? Charset.forName("gbk")
:Charset.forName("UTF-8");
return charset;
}
public UploadBean uploadBase64File(String data, HttpServletRequest request){
/*创建uploadBean*/
UploadBean result = new UploadBean();
if (!StringUtils.isBlank(data)) {
BAttachment attachment = new BAttachment();
BUser userVo = BiemoLoginContext.me().getCurrentUser();
if(userVo!=null) {
attachment.setUserId(userVo.getId().toString());
attachment.setUserName(userVo.getUsername());
}
attachment.setUploadDate(new Date());
attachment.setUploadIp(ControllerUtil.getRemoteAddress(request));
attachment.setFileSize(this.getBase64FileSize(data).floatValue()/1024);
if(getBase64FileType(data).equals("png")){
attachment.setFileExtname("image/png");
}else if(getBase64FileType(data).equals("gif")){
attachment.setFileExtname("image/gif");
}else if(getBase64FileType(data).equals("jpg")){
attachment.setFileExtname("image/jpeg");
}
attachment.setFileKey(UUID.randomUUID().toString().replace("-",""));
String fileType = this.getBase64FileType(data);
String fileName = this.getFileName(fileType);
String newName = this.getNewFileName(fileName);
attachment.setOriginalFileName(newName);
File file = new File(this.getUploadPath() + newName);
attachment.setFilePath(file.getPath());
attachment.setFileName(fileName);
/*如果不存在就创建*/
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
}
try {
this.writeFile(this.base64StrToByte(data),file);
} catch (Exception e) {
throw new RuntimeException(e.getMessage());
}
if(Boolean.parseBoolean(enableFullPath)) {
没有合适的资源?快使用搜索试试~ 我知道了~
springboot+mybatis-plus+springcloud-HoxtonSR3 开发者论坛社区系统
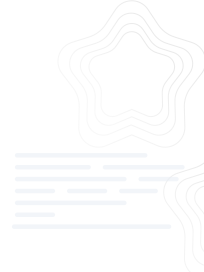
共1636个文件
java:726个
vue:201个
js:170个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 166 浏览量
2024-05-15
22:02:25
上传
评论
收藏 7.53MB ZIP 举报
温馨提示
该项目利用了基于springboot + vue + mysql的开发模式框架实现的课设系统,包括了项目的源码资源、sql文件、相关指引文档等等。 【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。 【技术】 Java、Python、Node.js、Spring Boot、Django、Express、MySQL、PostgreSQL、MongoDB、React、Angular、Vue、Bootstrap、Material-UI、Redis、Docker、Kubernetes
资源推荐
资源详情
资源评论
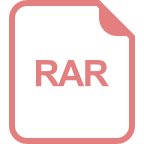
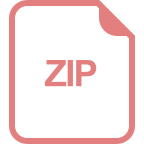
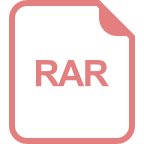
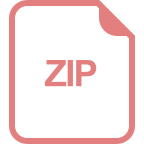
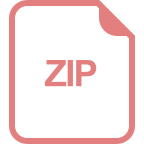
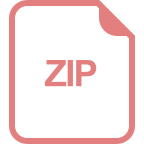
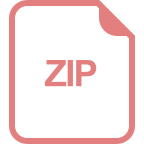
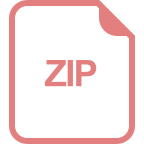
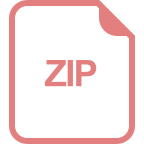
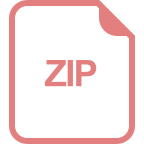
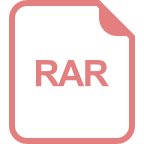
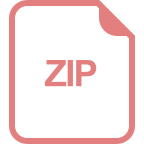
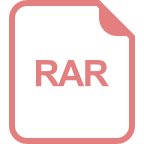
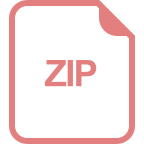
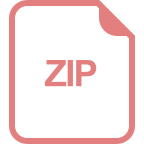
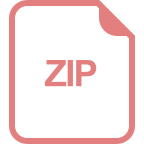
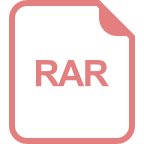
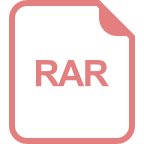
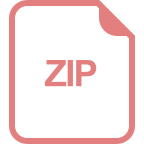
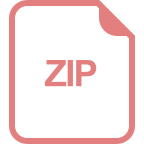
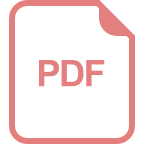
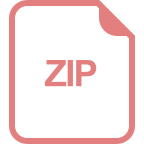
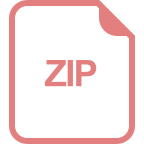
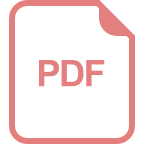
收起资源包目录

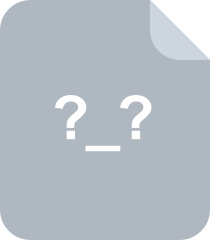
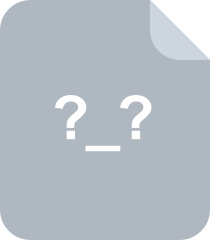
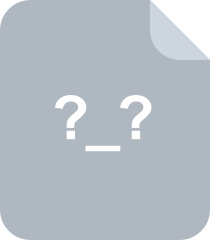
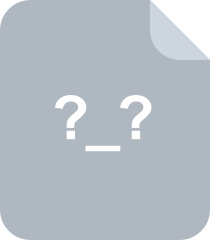
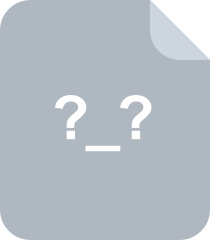
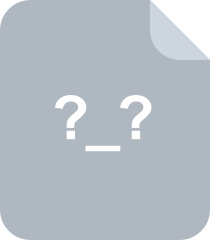
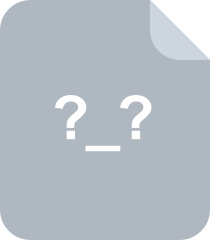
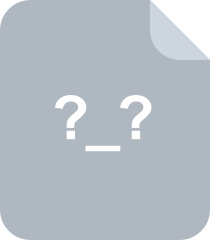
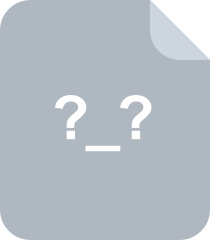
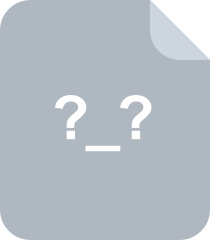
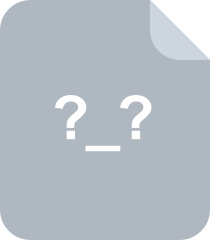
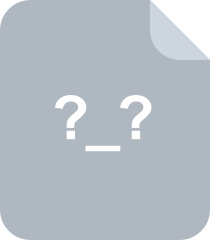
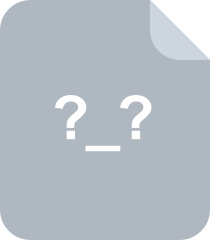
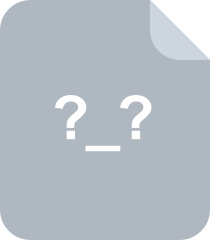
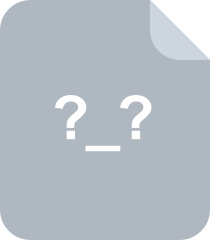
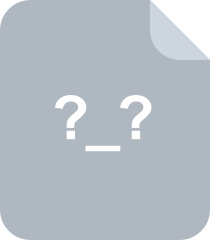
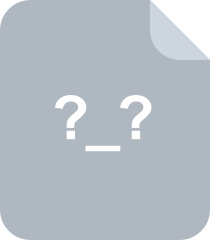
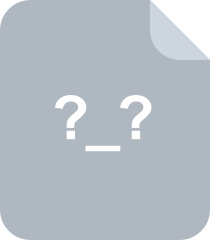
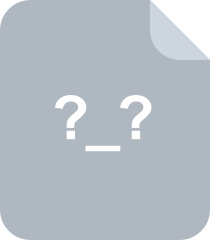
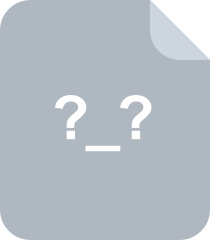
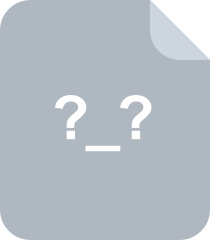
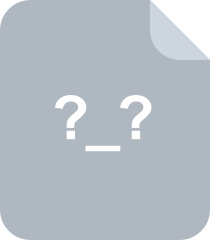
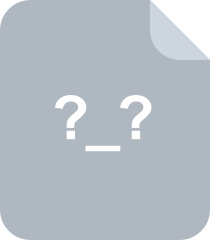
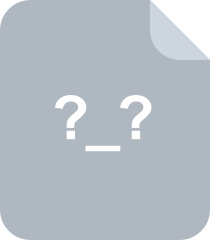
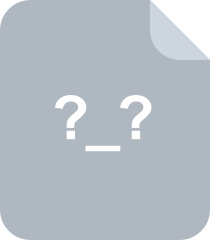
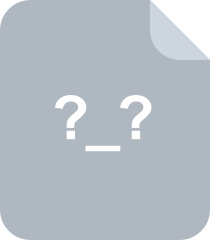
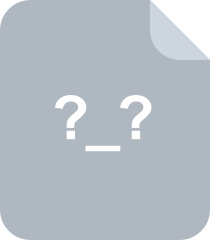
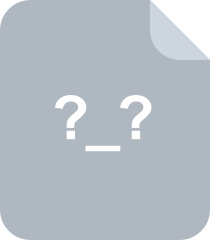
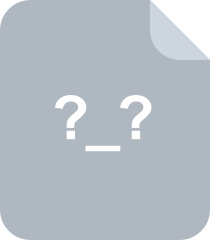
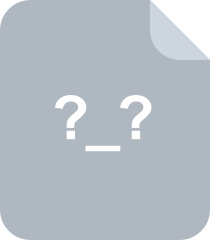
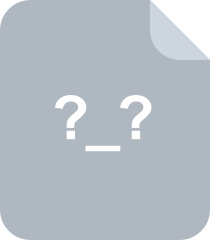
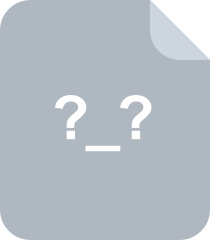
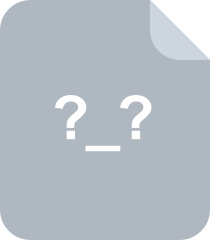
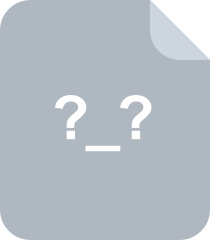
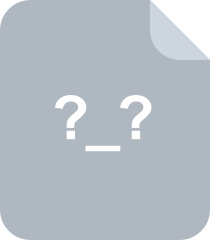
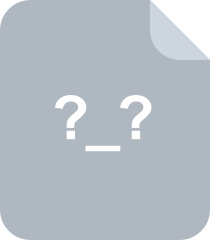
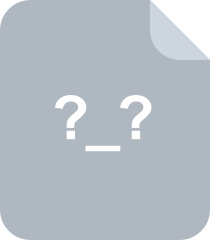
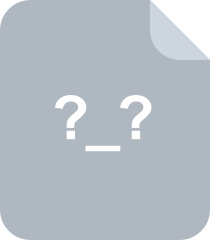
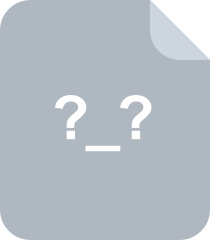
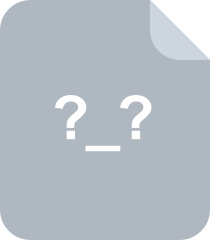
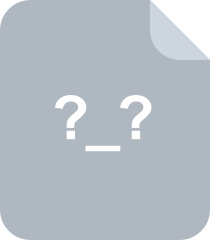
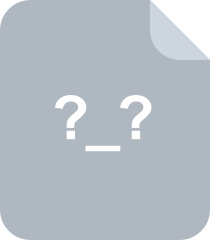
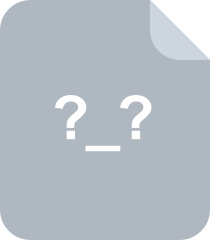
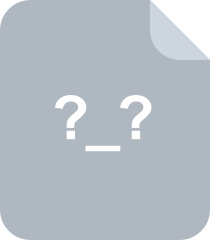
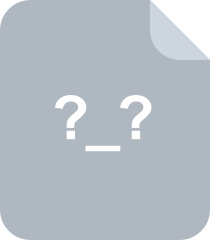
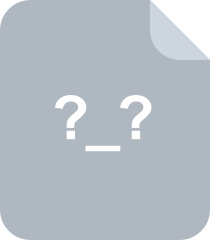
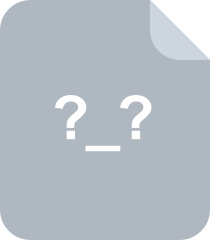
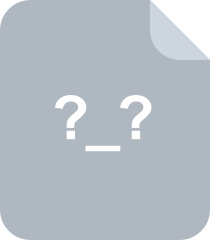
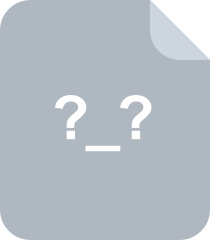
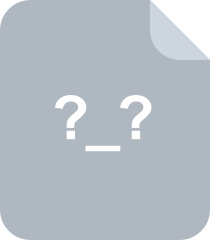
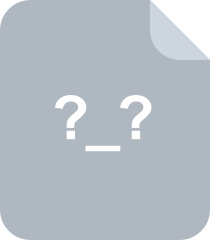
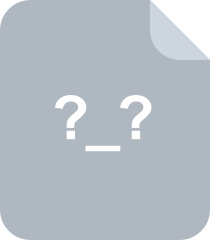
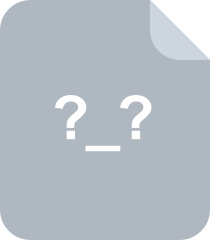
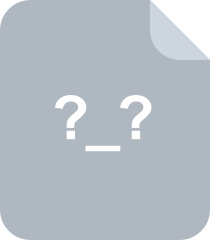
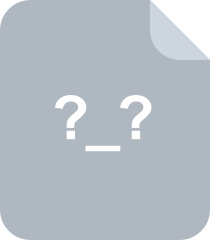
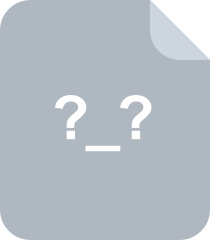
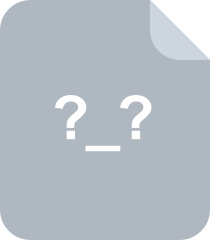
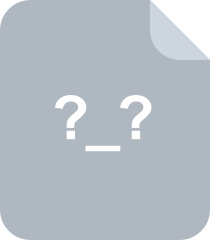
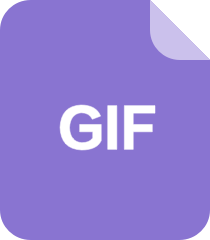
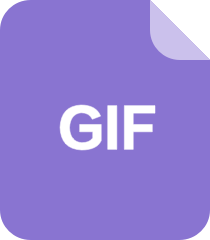
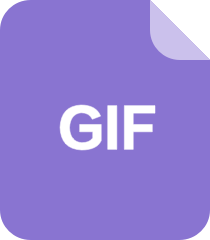
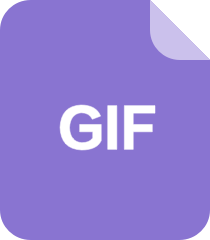
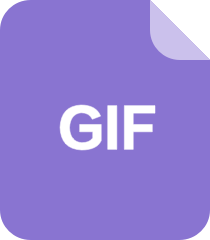
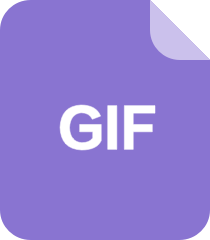
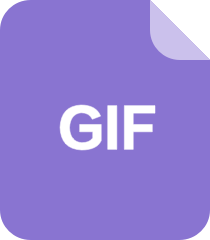
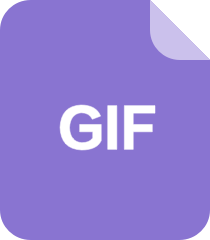
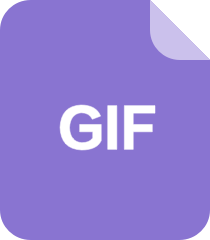
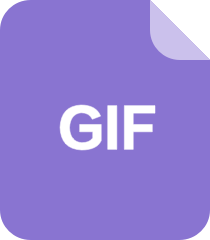
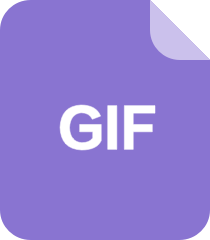
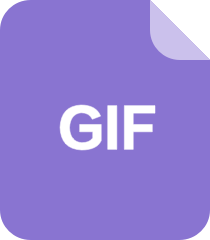
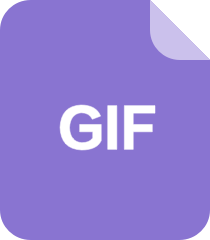
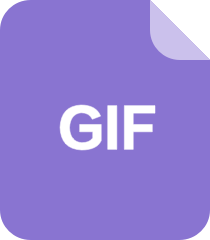
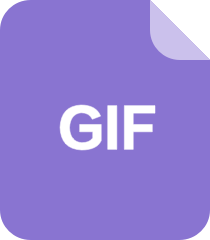
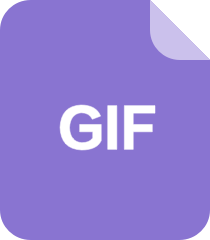
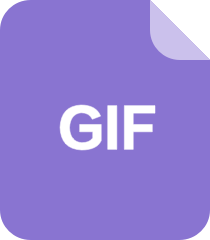
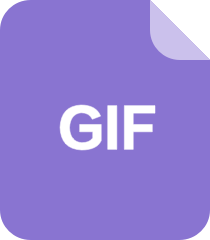
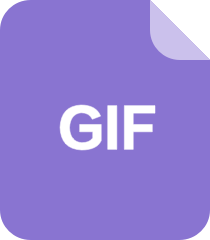
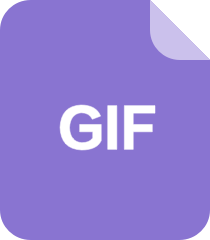
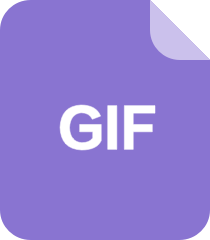
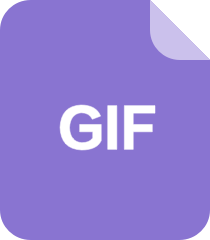
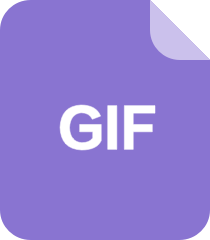
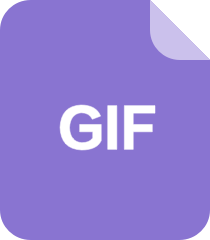
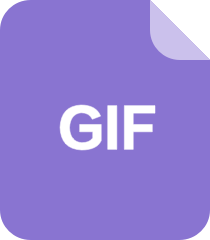
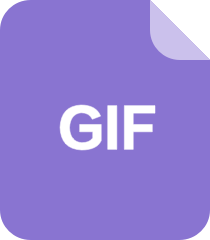
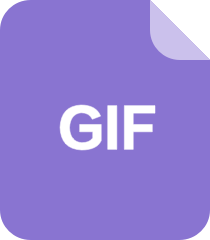
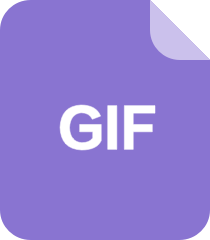
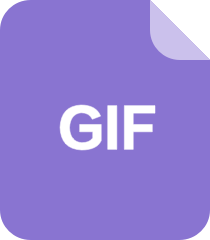
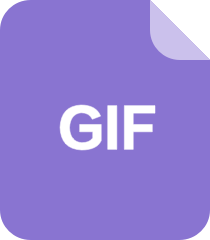
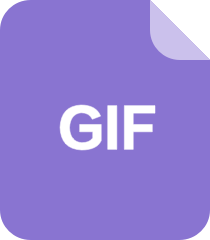
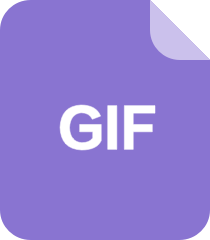
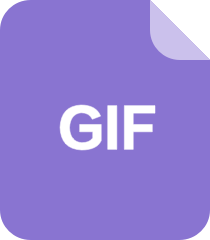
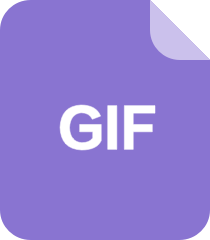
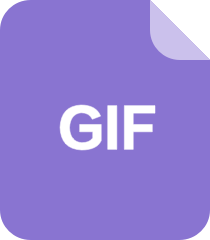
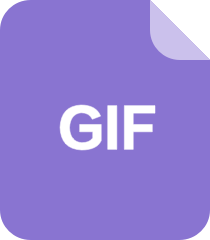
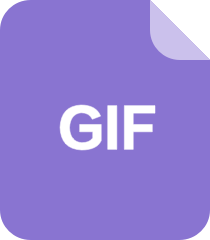
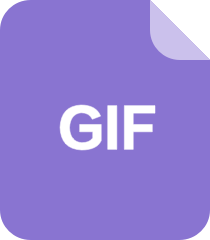
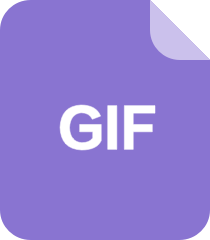
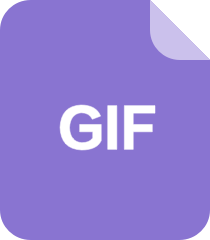
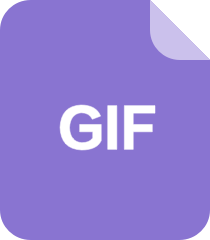
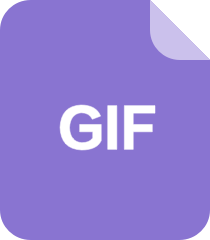
共 1636 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
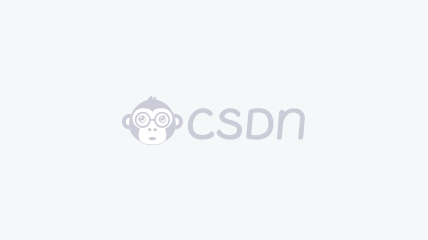

枫蜜柚子茶
- 粉丝: 9051
- 资源: 5352
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

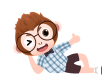
最新资源
- 小程序项目-基于微信小程序的游乐园智慧向导小程序(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的ssm基于微信小程序的付费自习室系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的springboot微信小程序的点餐系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的ssm基于微信小程序的短视频系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的ssm基于微信小程序的跳蚤市场的设计与实现修改(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的智能停车场管理系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的在线办公小程序(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的游泳馆管理系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的ssm基于微信小程序的高校课堂教学管理系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的自助购药小程序(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的自习室预约系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的足浴城消费系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的ssm基于微信小程序的食堂窗口自助点餐系统(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的“健康早知道”(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的“最多跑一次”(包括源码,数据库,教程).zip
- 小程序项目-基于微信小程序的党建工作小秘书(包括源码,数据库,教程).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


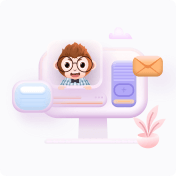
安全验证
文档复制为VIP权益,开通VIP直接复制
