/*
* Copyright (C) 2014 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.xk.trucktrade.ui.custom;
import android.content.Context;
import android.content.res.Resources;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.ColorFilter;
import android.graphics.Paint;
import android.graphics.Path;
import android.graphics.PixelFormat;
import android.graphics.Rect;
import android.graphics.RectF;
import android.graphics.drawable.Animatable;
import android.graphics.drawable.Drawable;
import android.support.annotation.IntDef;
import android.support.annotation.NonNull;
import android.support.v4.view.animation.FastOutSlowInInterpolator;
import android.util.DisplayMetrics;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.Interpolator;
import android.view.animation.LinearInterpolator;
import android.view.animation.Transformation;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.util.ArrayList;
/**
* 从 android.support.v4.widget 包里复制而来, 因为它本身是隐藏的, 不能被外部使用.
* <p/>
* Copy by Lex Luther on 9/30/15.
*/
public class MaterialProgressDrawable extends Drawable implements Animatable {
// Maps to ProgressBar.Large style
public static final int LARGE = 0;
// Maps to ProgressBar default style
public static final int DEFAULT = 1;
/**
* Fancy progress indicator for Material theme.
*/
private static final Interpolator LINEAR_INTERPOLATOR = new LinearInterpolator();
private static final Interpolator MATERIAL_INTERPOLATOR = new FastOutSlowInInterpolator();
private static final float FULL_ROTATION = 1080.0f;
// Maps to ProgressBar default style
private static final int CIRCLE_DIAMETER = 40;
private static final float CENTER_RADIUS = 8.75f; //should add up to 10 when + stroke_width
private static final float STROKE_WIDTH = 2.5f;
// Maps to ProgressBar.Large style
private static final int CIRCLE_DIAMETER_LARGE = 56;
private static final float CENTER_RADIUS_LARGE = 12.5f;
private static final float STROKE_WIDTH_LARGE = 3f;
/**
* The value in the linear interpolator for animating the drawable at which
* the color transition should start
*/
private static final float COLOR_START_DELAY_OFFSET = 0.75f;
private static final float END_TRIM_START_DELAY_OFFSET = 0.5f;
private static final float START_TRIM_DURATION_OFFSET = 0.5f;
/**
* The duration of a single progress spin in milliseconds.
*/
private static final int ANIMATION_DURATION = 1332;
/**
* The number of points in the progress "star".
*/
private static final float NUM_POINTS = 5f;
/**
* Layout info for the arrowhead in dp
*/
private static final int ARROW_WIDTH = 10;
private static final int ARROW_HEIGHT = 5;
private static final float ARROW_OFFSET_ANGLE = 5;
/**
* Layout info for the arrowhead for the large spinner in dp
*/
private static final int ARROW_WIDTH_LARGE = 12;
private static final int ARROW_HEIGHT_LARGE = 6;
private static final float MAX_PROGRESS_ARC = .8f;
private final int[] COLORS = new int[]{
Color.BLACK
};
/**
* The list of animators operating on this drawable.
*/
private final ArrayList<Animation> mAnimators = new ArrayList<Animation>();
/**
* The indicator ring, used to manage animation state.
*/
private final Ring mRing;
private final Callback mCallback = new Callback() {
@Override
public void invalidateDrawable(Drawable d) {
invalidateSelf();
}
@Override
public void scheduleDrawable(Drawable d, Runnable what, long when) {
scheduleSelf(what, when);
}
@Override
public void unscheduleDrawable(Drawable d, Runnable what) {
unscheduleSelf(what);
}
};
boolean mFinishing;
/**
* Canvas rotation in degrees.
*/
private float mRotation;
private Resources mResources;
private View mParent;
private Animation mAnimation;
private float mRotationCount;
private double mWidth;
private double mHeight;
public MaterialProgressDrawable(Context context, View parent) {
mParent = parent;
mResources = context.getResources();
mRing = new Ring(mCallback);
mRing.setColors(COLORS);
updateSizes(DEFAULT);
setupAnimators();
}
private void setSizeParameters(double progressCircleWidth, double progressCircleHeight,
double centerRadius, double strokeWidth, float arrowWidth, float arrowHeight) {
final Ring ring = mRing;
final DisplayMetrics metrics = mResources.getDisplayMetrics();
final float screenDensity = metrics.density;
mWidth = progressCircleWidth * screenDensity;
mHeight = progressCircleHeight * screenDensity;
ring.setStrokeWidth((float) strokeWidth * screenDensity);
ring.setCenterRadius(centerRadius * screenDensity);
ring.setColorIndex(0);
ring.setArrowDimensions(arrowWidth * screenDensity, arrowHeight * screenDensity);
ring.setInsets((int) mWidth, (int) mHeight);
}
/**
* Set the overall size for the progress spinner. This updates the radius
* and stroke width of the ring.
*
* @param size One of {@link MaterialProgressDrawable.LARGE} or
* {@link MaterialProgressDrawable.DEFAULT}
*/
public void updateSizes(@ProgressDrawableSize int size) {
if (size == LARGE) {
setSizeParameters(CIRCLE_DIAMETER_LARGE, CIRCLE_DIAMETER_LARGE, CENTER_RADIUS_LARGE,
STROKE_WIDTH_LARGE, ARROW_WIDTH_LARGE, ARROW_HEIGHT_LARGE);
} else {
setSizeParameters(CIRCLE_DIAMETER, CIRCLE_DIAMETER, CENTER_RADIUS, STROKE_WIDTH,
ARROW_WIDTH, ARROW_HEIGHT);
}
}
/**
* @param show Set to true to display the arrowhead on the progress spinner.
*/
public void showArrow(boolean show) {
mRing.setShowArrow(show);
}
/**
* @param scale Set the scale of the arrowhead for the spinner.
*/
public void setArrowScale(float scale) {
mRing.setArrowScale(scale);
}
/**
* Set the start and end trim for the progress spinner arc.
*
* @param startAngle start angle
* @param endAngle end angle
*/
public void setStartEndTrim(float startAngle, float endAngle) {
mRing.setStartTrim(startAngle);
mRing.setEndTrim(endAngle);
}
/**
* Set the amount of rotation to apply to the progress spinner.
*
* @param rotation Rotation is from [0..1]
*/
public void setProgressRotation(float rotation) {
mRing.setRotation(rotation);
}
/**
* Update the background color of the circle_primary image view.
*/
public void setBackgroundColor(int color) {
mRing.setBackgroundColor(color);
}
/**
* Set the colors used in the progress animation from color resources.
* The first color will also be the color of the bar that grows in response
* to a user swipe gesture.
*
* @param colors colors
*/
public void setColorSchemeColors(int... colors) {
mRing.setColors(colors);
没有合适的资源?快使用搜索试试~ 我知道了~
毕业设计——货车租赁系统。包括服务端和安卓端TruckRent.zip
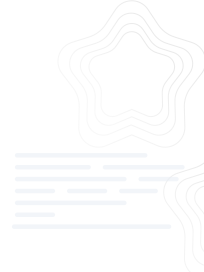
共363个文件
java:102个
xml:92个
png:69个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 51 浏览量
2024-04-28
23:32:27
上传
评论 1
收藏 10.98MB ZIP 举报
温馨提示
【博客个人资源】 包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。 【技术】 Java、Python、Node.js、Spring Boot、Django、Express、MySQL、PostgreSQL、MongoDB、React、Angular、Vue、Bootstrap、Material-UI、Redis、Docker、Kubernetes
资源推荐
资源详情
资源评论
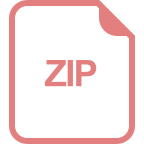
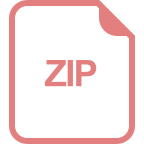
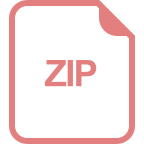
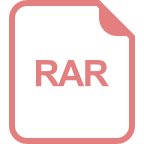
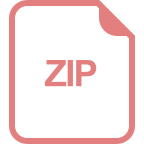
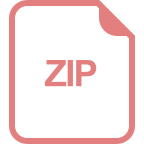
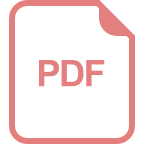
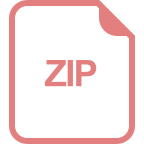
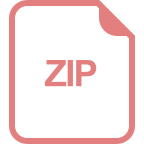
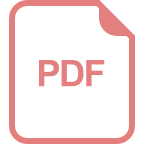
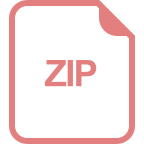
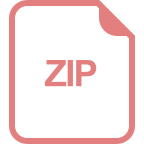
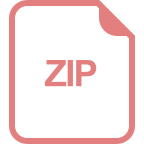
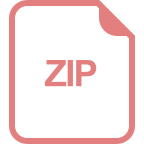
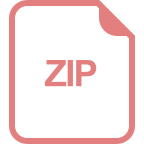
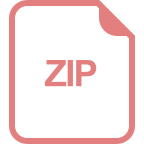
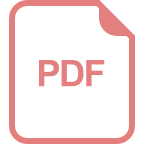
收起资源包目录

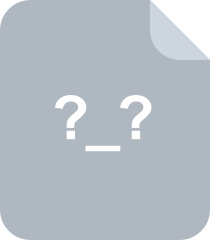
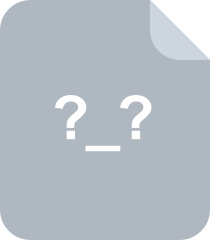
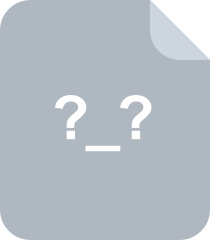
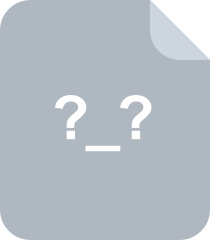
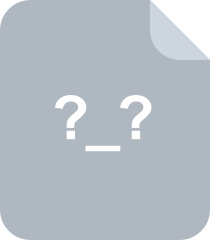
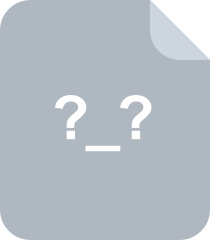
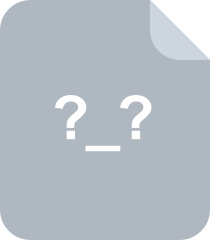
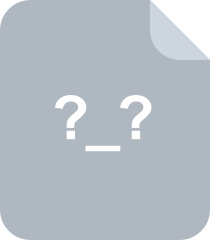
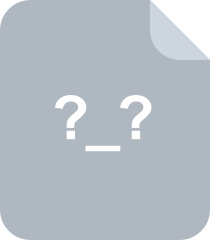
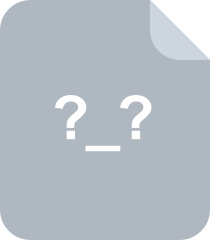
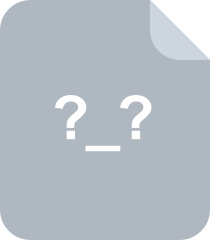
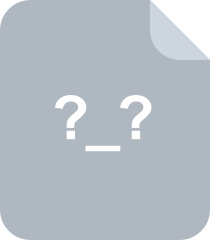
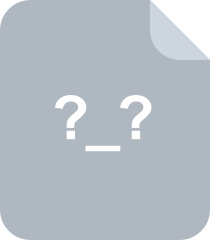
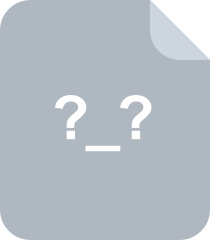
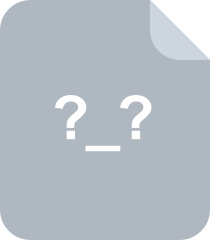
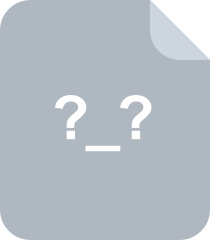
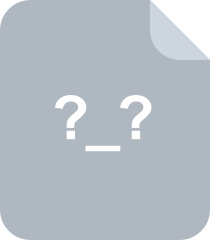
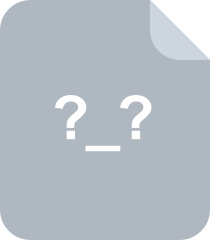
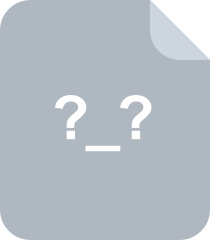
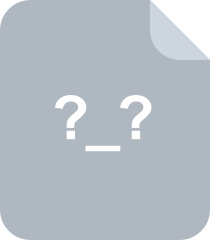
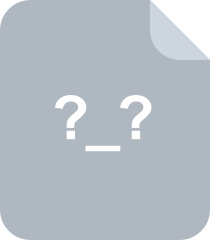
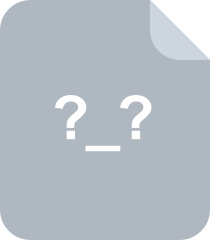
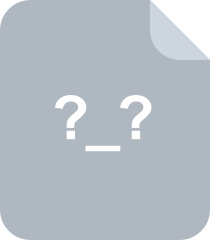
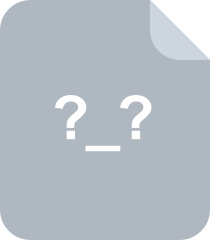
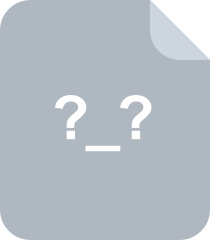
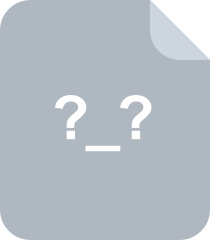
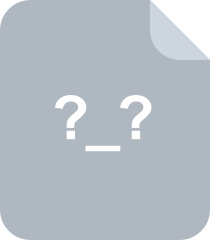
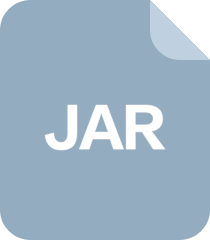
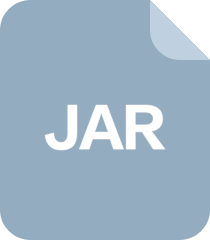
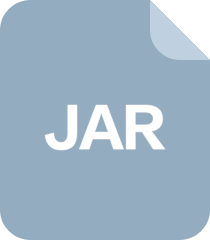
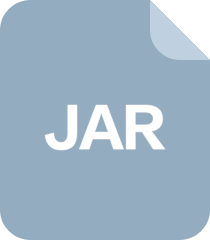
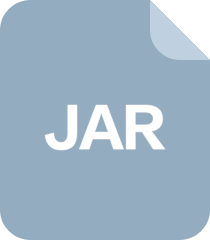
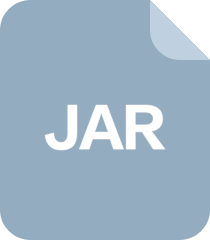
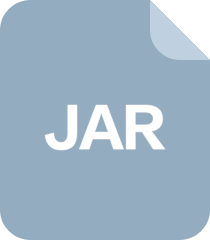
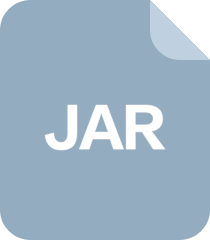
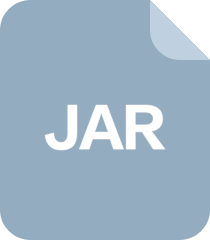
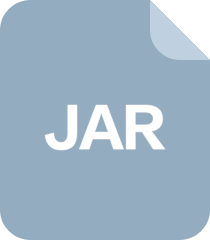
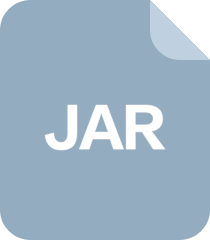
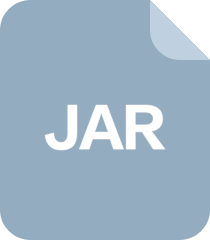
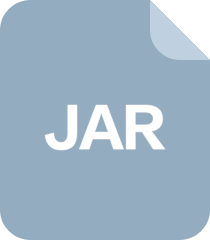
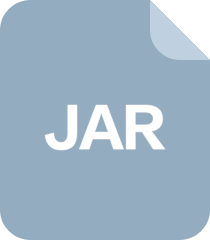
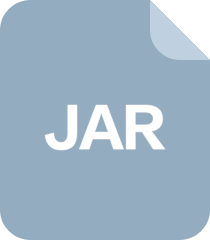
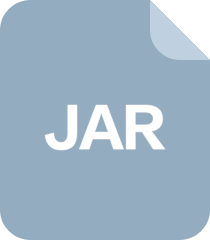
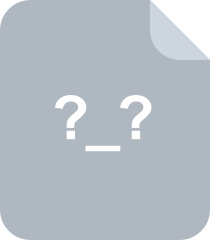
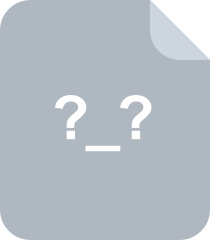
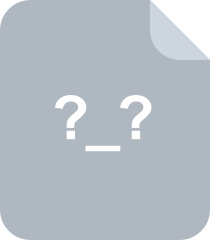
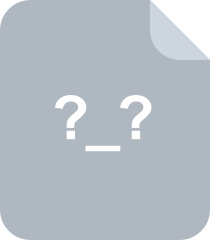
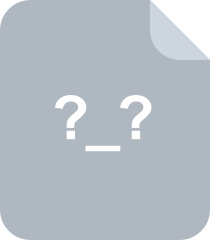
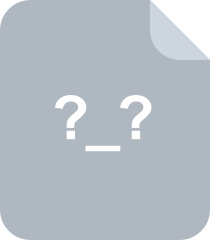
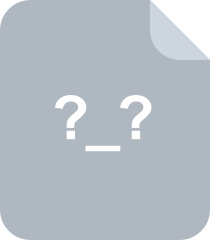
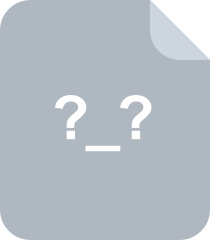
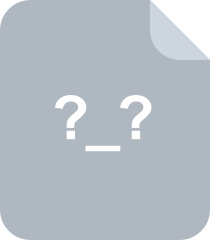
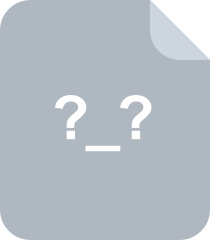
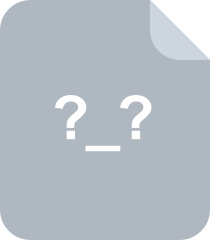
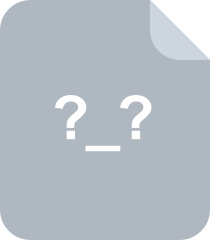
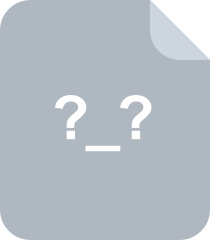
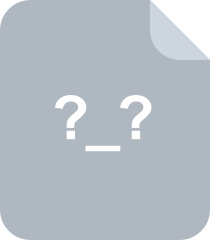
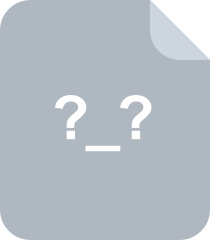
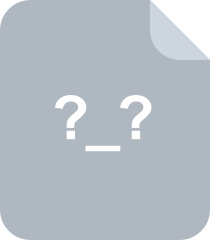
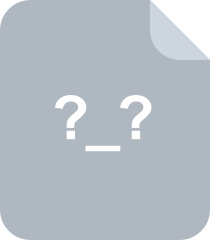
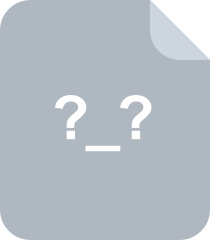
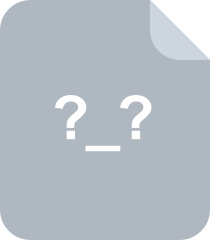
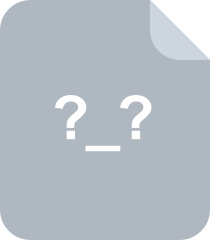
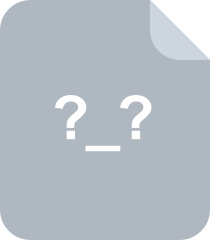
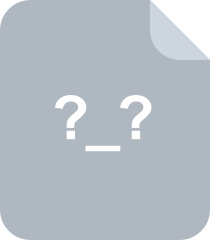
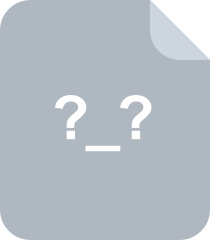
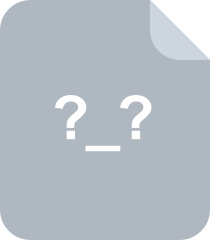
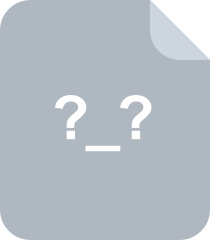
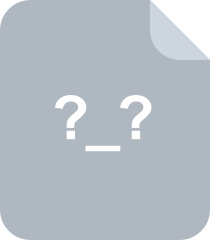
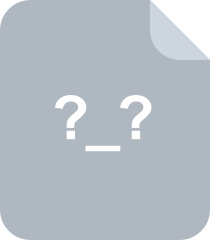
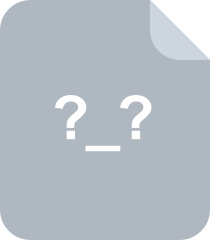
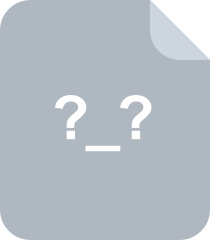
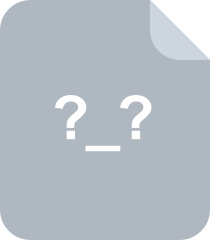
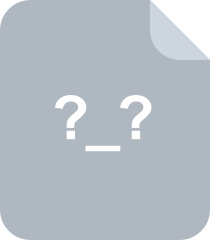
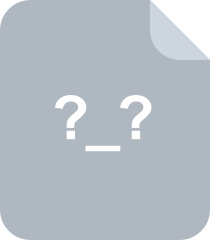
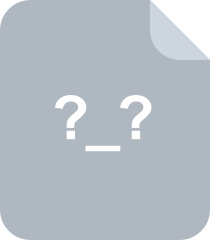
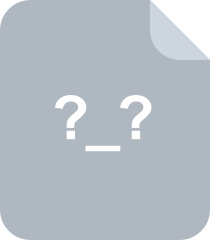
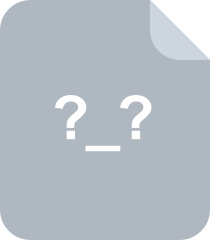
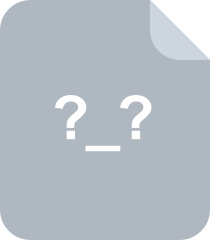
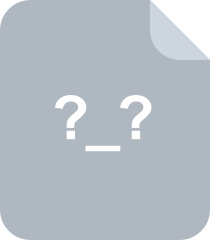
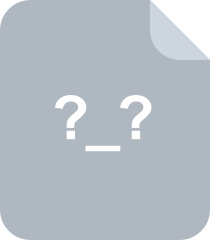
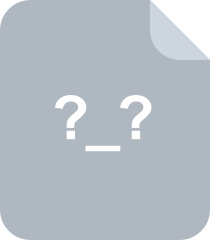
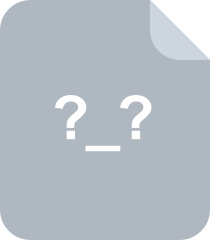
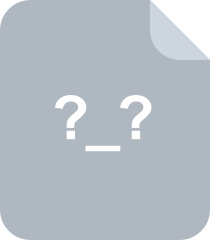
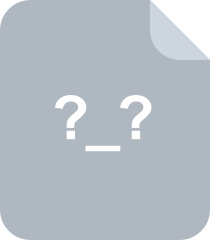
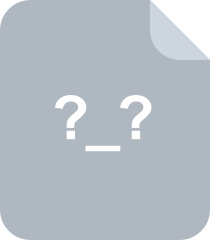
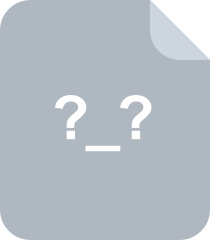
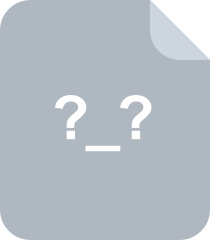
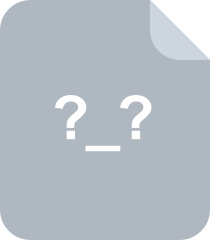
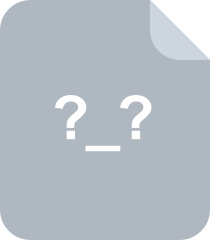
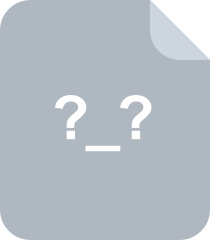
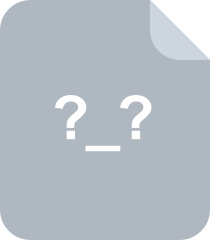
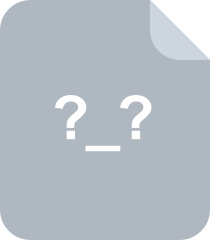
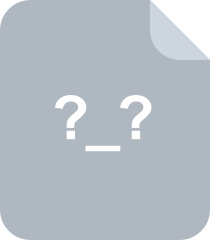
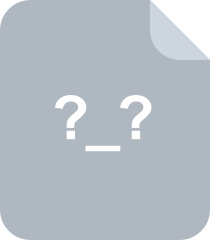
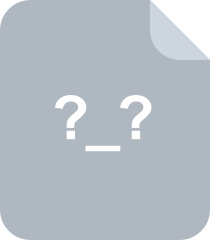
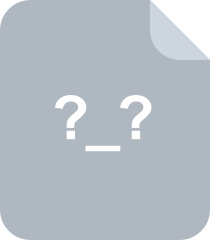
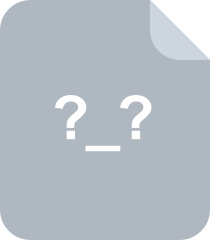
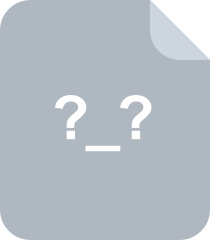
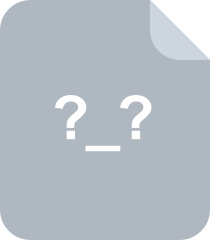
共 363 条
- 1
- 2
- 3
- 4
资源评论
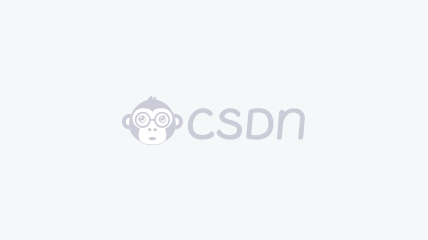

枫蜜柚子茶
- 粉丝: 9019
- 资源: 5350
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

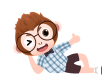
最新资源
- 基于stm32、树莓派,后端使用Java的springboot架构,以微信小程序作为用户控制端的智能家居控制系统详细文档+全部资料+高分项目.zip
- 基于STM32F103的移动底座与ROS通信,包括ROS串口节点、STM32串口收发详细文档+全部资料+高分项目.zip
- 基于STM32+RC522 RFID 驱动详细文档+全部资料+高分项目.zip
- 基于stm32+FreeRTOS+ESP8266的实时天气系统详细文档+全部资料+高分项目.zip
- 基于stm32的12864oled图形库详细文档+全部资料+高分项目.zip
- 数据集-目标检测系列- 蛋糕 检测数据集 cake >> DataBall
- 基于Matlab实现瑞利衰落信道仿真(源码).rar
- 基于STM32的LCD12881显示屏驱动详细文档+全部资料+高分项目.zip
- time-sync.cc
- 基于STM32的PurePursuit算法的实现详细文档+全部资料+高分项目.zip
- 基于STM32的Marlin三轴机械臂控制程序详细文档+全部资料+高分项目.zip
- 基于STM32的车牌识别系统详细文档+全部资料+高分项目.zip
- 基于stm32的宠物RFID阅读器详细文档+全部资料+高分项目.zip
- 基于STM32的SLAM机器人移动底盘详细文档+全部资料+高分项目.zip
- 基于STM32的倒车雷达项目--OLED显示,HC-SR04详细文档+全部资料+高分项目.zip
- 基于STM32的孤立词语音识别详细文档+全部资料+高分项目.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


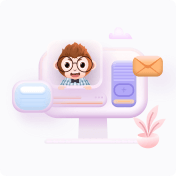
安全验证
文档复制为VIP权益,开通VIP直接复制
