# 更新记录
### 2021-07-23
- 重新使用 Django 3.2.4 构建项目
- 增加 API 文档库 coreapi
## 需要安装的 Python 库
- django
- djangorestframework
- django-cors-headers
- python-jose
- coreapi
- pymysql
- django-filter
## 初始化 Django 工程
```shell
django-admin startproject backend
```
创建好工程后,我们要对目录和配置进行一些调整,首先在根目录下创建两个目录:apps 和 settings,将所有的 app 都存放到 apps 目录里面,把 settings 配置存放在 settings 目录下,这样我们的根目录就更加清晰了

- 调整 settings 配置
首先将 backend 目录下的 settings.py 文件拷贝到 settings 目录下,创建 dev.py 和 pro.py 两个文件,主要用于开发配置和部署配置,将 settings.py 文件中的数据库配置和 DEBUG 移到这两个文件中,内容如下


在 settings.py 文件中把 apps 添加到环境变量中

修改语言和时区

## 增加多数据库配置
- 在 backend 目录下增加 router.py 文件
路由配置文件当中的返回值是我们在 DATABASES 中配置的键,默认是 default,按照一定的条件返回不同的键,每个键内配置不同的数据库连接,就可以实现 Django 项目连接多个数据库
```python
class CustomRouter:
def db_for_read(self, model, **hints):
if model._meta.app_label in settings.DATABASE_APPS_MAPPING:
return settings.DATABASE_APPS_MAPPING[model._meta.app_label]
def db_for_write(self, model, **hints):
if model._meta.app_label in settings.DATABASE_APPS_MAPPING:
return settings.DATABASE_APPS_MAPPING[model._meta.app_label]
def allow_relation(self, obj1, obj2, **hints):
db_obj1 = settings.DATABASE_APPS_MAPPING.get(obj1._meta.app_label)
db_obj2 = settings.DATABASE_APPS_MAPPING.get(obj2._meta.app_label)
if db_obj1 and db_obj2:
if db_obj1 == db_obj2:
return True
else:
return False
def allow_syncdb(self, db, model):
if db in settings.DATABASE_APPS_MAPPING.values():
return settings.DATABASE_APPS_MAPPING.get(model._meta.app_label) == db
elif model._meta.app_label in settings.DATABASE_APPS_MAPPING:
return False
def allow_migrate(self, db, app_label, model=None, **hints):
"""
Make sure the auth app only appears in the 'auth_db'
database.
"""
if db in settings.DATABASE_APPS_MAPPING.values():
return settings.DATABASE_APPS_MAPPING.get(app_label) == db
elif app_label in settings.DATABASE_APPS_MAPPING:
return False
return None
```
- 在 settings.py 文件中增加路由配置
```python
DATABASE_ROUTERS = ['backend.router.CustomRouter']
```
## 设置自定义用户模型
- 在 apps 下增加 users 应用
在 models.py 下增加如下内容
```python
from django.contrib.auth.models import AbstractUser
from django.db import models
from uuid import uuid4
# Create your models here.
class UserInfo(AbstractUser):
uid = models.CharField(max_length=36, default=uuid4, primary_key=True)
nickname = models.CharField(max_length=32, verbose_name='昵称')
telephone = models.CharField(max_length=11, blank=True, null=True, unique=True, verbose_name='手机号码')
create_time = models.DateTimeField(auto_now_add=True, verbose_name='创建时间')
class Meta:
db_table = 'tbl_user'
```
- 在 settings.py 中增加如下内容
```python
INSTALLED_APPS = [
...
'users'
]
AUTH_USER_MODEL = 'users.UserInfo'
```
## 解决跨域问题
为什么会有跨域问题,这里就不做详细解释了,可以看一下两篇文章
- [前后端分离 djangorestframework——解决跨域请求](https://www.cnblogs.com/Eeyhan/p/10440444.html)
- [Django 跨域验证及 OPTIONS 请求](https://w.url.cn/s/AoDZ2R0)
在 settings.py 文件中做如下配置
```python
INSTALLED_APPS = [
...
'corsheaders'
...
]
MIDDLEWARE = [
...
'corsheaders.middleware.CorsMiddleware', # 注意必须放在CommonMiddleware前面
...
]
CORS_ALLOW_CREDENTIALS = True
CORS_ORIGIN_ALLOW_ALL = True
CORS_ALLOW_METHODS = ['*']
CORS_ALLOW_HEADERS = ['*']
```
## jwt 登录认证
我们使用 rest api 接口,一般就很少使用用户名和密码认真,jwt 认证是比较常用的,因此这也是项目初始化必须做的。要注意
- 在根目录下增加 utils 目录,增加两个文件 authentication.py 和 jwt_util.py
- authentication.py 文件
```python
import time
from django.db.models import Q
from rest_framework.authentication import BaseAuthentication
from rest_framework.exceptions import AuthenticationFailed
from users.models import UserInfo
from utils.auth.jwt_util import JwtUtil
from threading import local
_thread_local = local()
class JwtAuthentication(BaseAuthentication):
def authenticate(self, request):
access_token = request.META.get('HTTP_ACCESS_TOKEN', None)
if access_token:
jwt = JwtUtil()
data = jwt.check_jwt_token(access_token)
if data:
username = data.get('username')
telephone = data.get('telephone')
exp = data.get('exp')
if time.time() > exp:
raise AuthenticationFailed('authentication time out')
try:
user = UserInfo.objects.get(Q(username=username) | Q(telephone=telephone))
_thread_local.user = user
except (UserInfo.DoesNotExist, UserInfo.MultipleObjectsReturned) as e:
return (None, None)
else:
return (user, None)
raise AuthenticationFailed('authentication failed')
def get_current_user():
return getattr(_thread_local, 'user', None)
```
- jwt_util.py 文件
```python
import time
from jose import jwt
from django.conf import settings
class JwtUtil:
@staticmethod
def gen_jwt_token(user):
to_encode = {
'username': user.username,
'telephone': user.telephone,
'exp': time.time() + 24 * 3600,
}
encoded_jwt = jwt.encode(to_encode, settings.SECRET_KEY, algorithm=settings.ALGORITHM)
return encoded_jwt
@staticmethod
def check_jwt_token(value):
playload = {}
try:
playload = jwt.decode(value, settings.SECRET_KEY, algorithms=[settings.ALGORITHM]
)
except Exception as e:
logger.exception(e)
return playload
```
- 在 settings.py 中增加跨域认证的字段
```python
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'utils.authentications.JwtAuthentication'
],
'DATETIME_FORMAT': '%Y-%m-%d %H:%M:%S',
'DATETIME_INPUT_FORMATS': '%Y-%m-%d %H:%M:%S'
}
```
## 修改登录认证为 JWT 方式
- 在 utils 目录创建 user_backend.py 文件
```python
from django.contrib.auth import backends
from django.db.models import Q
from users.models import UserInfo
from utils.jwt_util import JwtUtil
class UserBackend(backends.ModelBackend):
def authenticate(self, request, username=None, password=None, **kwargs):
user = UserInfo.objects.get(Q(username=username) | Q(telephone=username))
if u
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
在线考试系统是一种基于互联网的教育技术工具,用于组织、管理和实施在线考试。这种系统通常由软件应用程序支持,为学生和考生提供了在网上参加考试的机会。在线考试系统可以用于各种教育和培训场景,包括学校教育、职业资格认证、招聘考试等。 【主要特点和优势】: 灵活性和便利性:学生和考生可以通过互联网随时随地参加考试,不再受限于特定的地点和时间。 自动化管理:系统能够自动管理考试安排、考生信息、考试成绩等数据。 安全性:在线考试系统通常具有严格的身份验证和防作弊措施。 实时反馈:考试结束后,系统可以立即生成和发布成绩,提供即时反馈。 多样化的题型:系统支持各种题型,包括选择题、填空题、问答题等多种题型。 数据分析:系统可以收集和分析大量的考试数据,帮助教育机构和考试机构更好地了解学生的表现和需求,优化教学和考试内容。 【引流】 Java、Python、Node.js、Spring Boot、Django、Express、MySQL、PostgreSQL、MongoDB、React、Angular、Vue、Bootstrap、Material-UI、Redis、Docker、Kubernetes
资源推荐
资源详情
资源评论
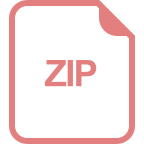
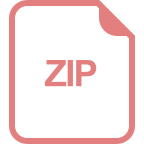
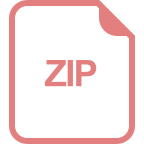
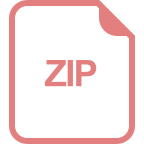
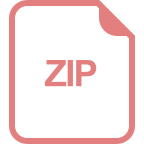
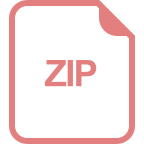
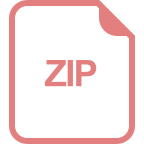
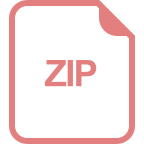
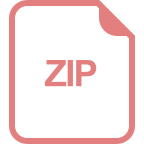
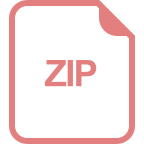
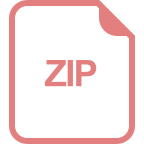
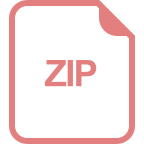
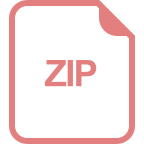
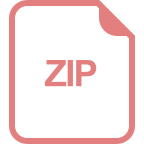
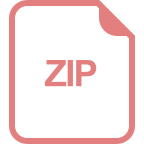
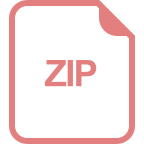
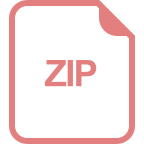
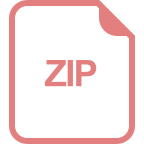
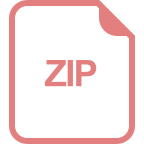
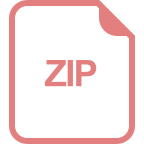
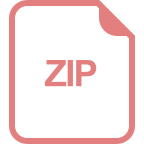
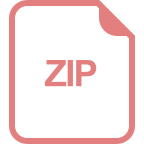
收起资源包目录

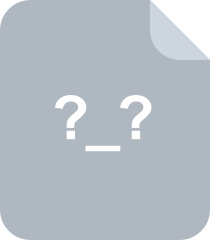
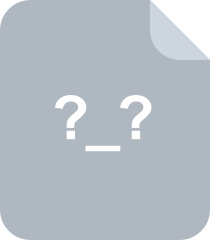
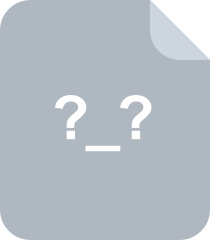
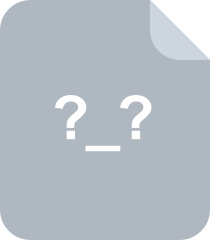
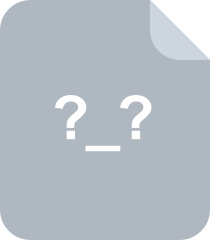
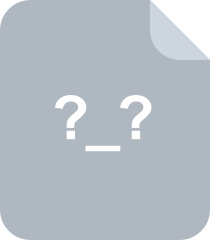
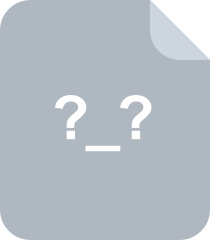
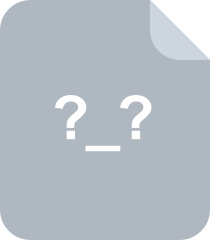
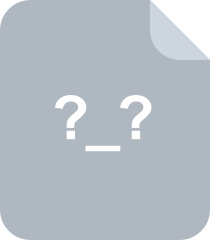
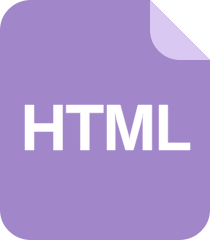
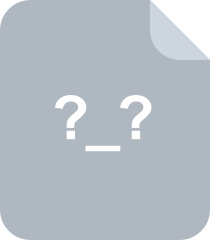
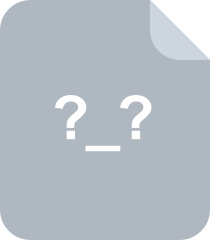
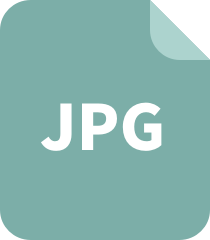
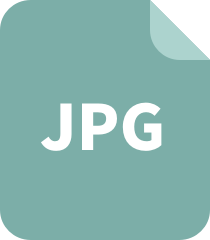
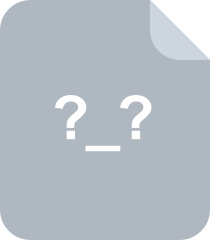
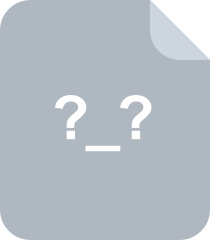
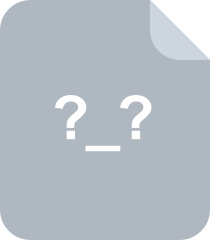
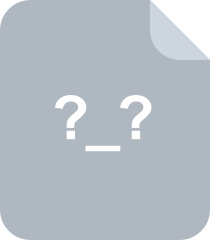
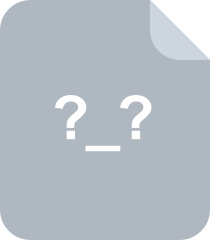
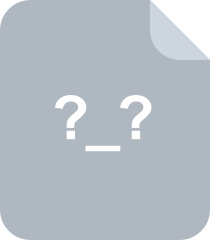
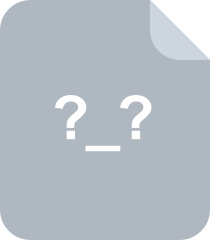
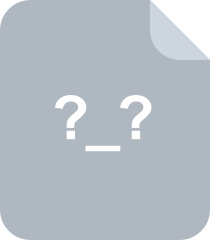
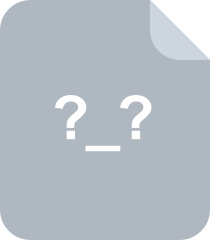
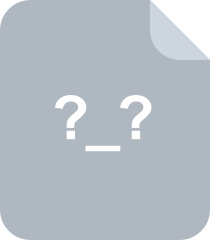
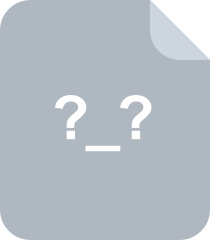
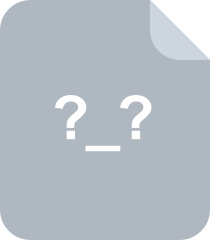
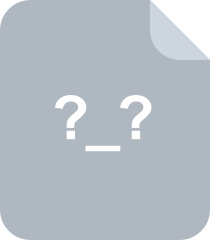
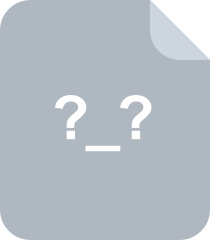
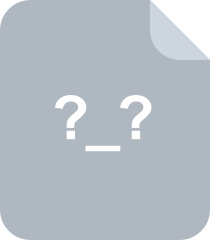
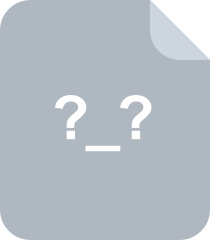
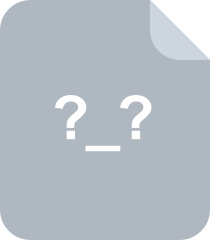
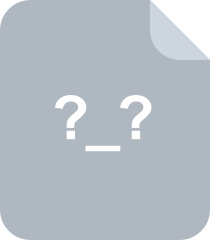
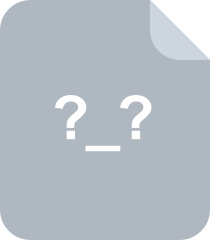
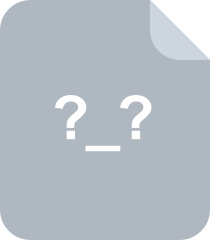
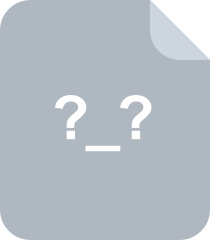
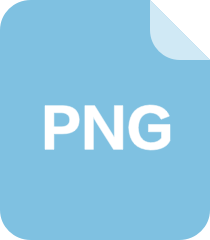
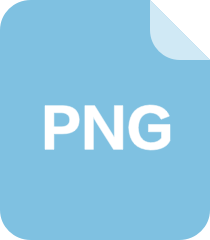
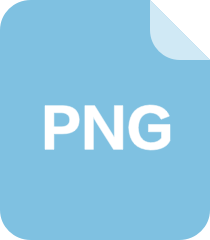
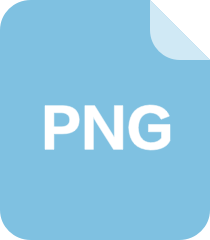
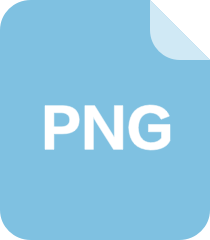
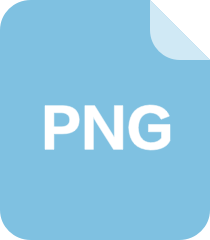
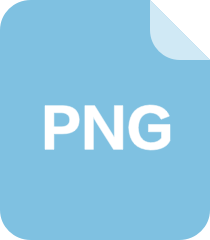
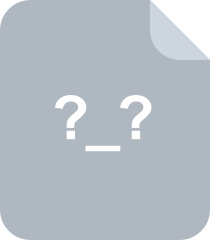
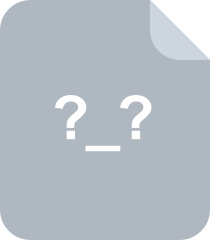
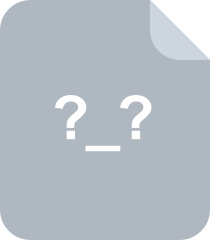
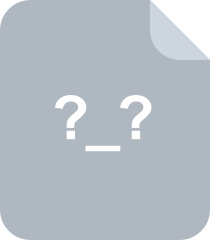
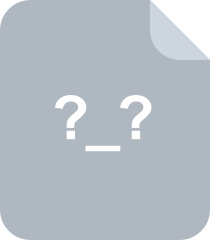
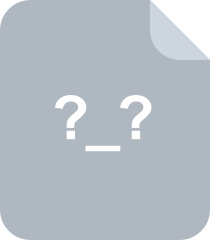
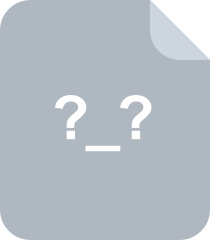
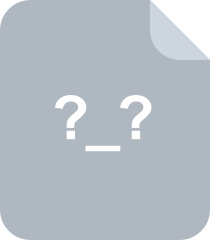
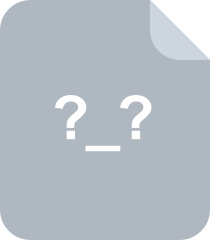
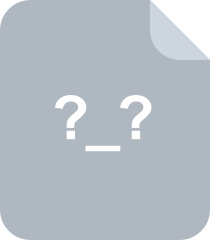
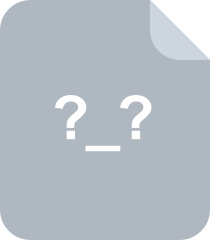
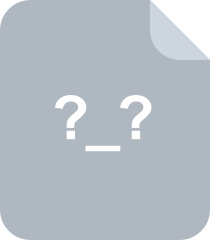
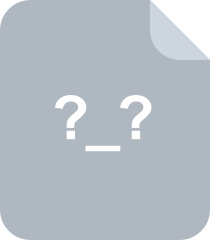
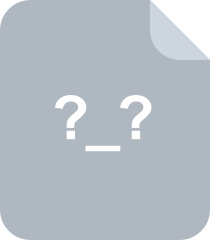
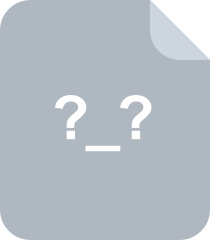
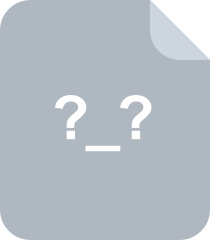
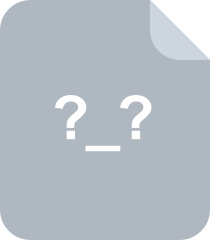
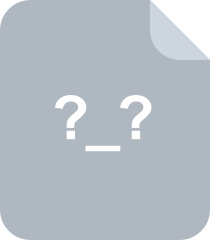
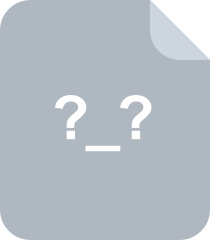
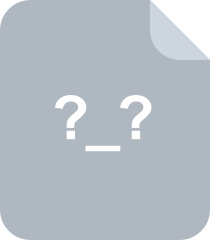
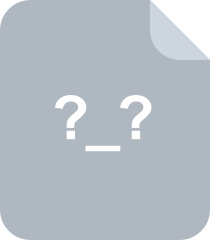
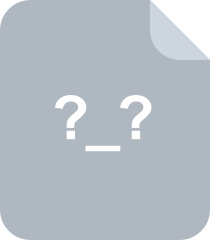
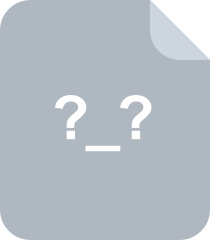
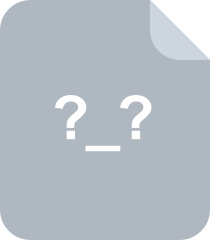
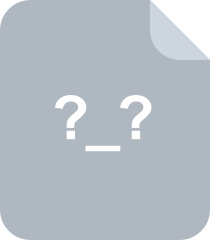
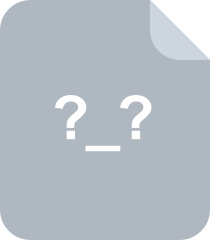
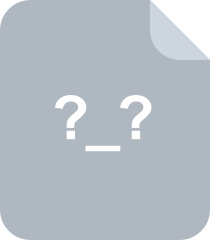
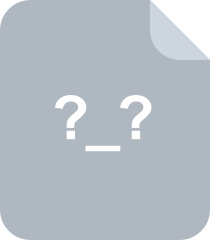
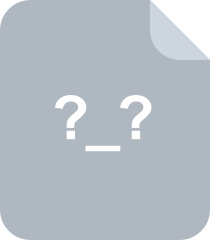
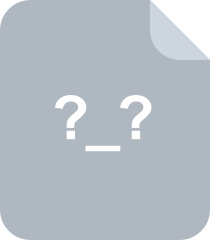
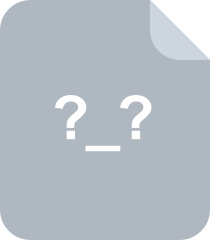
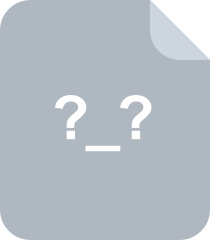
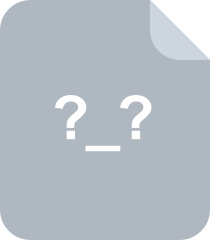
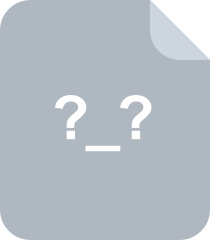
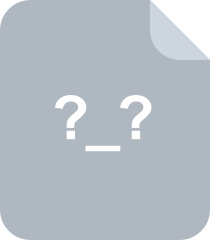
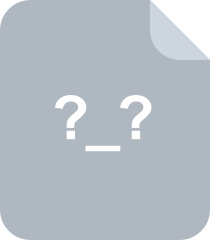
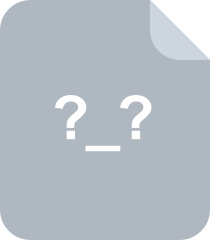
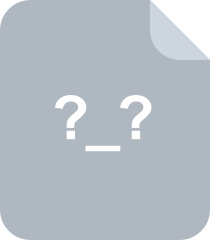
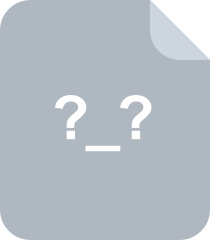
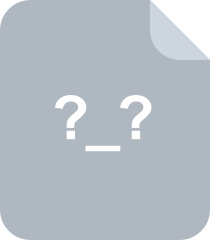
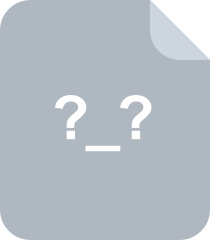
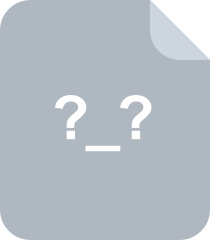
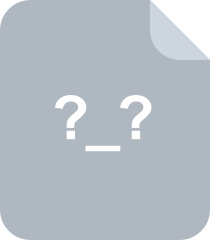
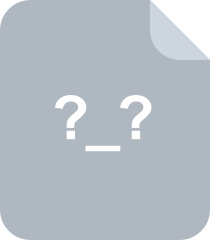
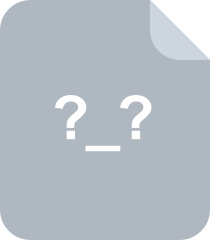
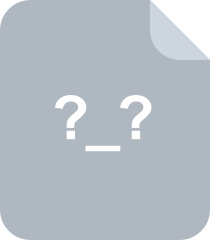
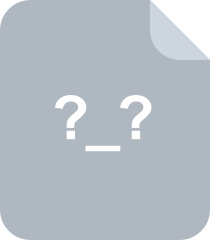
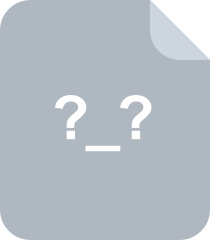
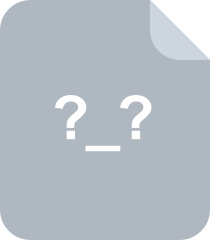
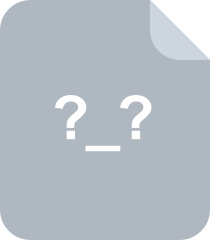
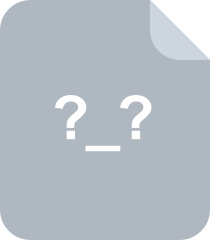
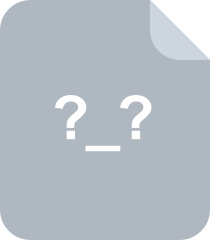
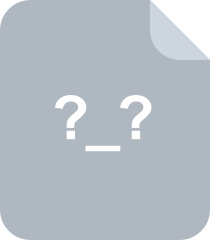
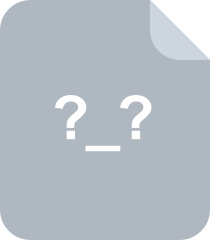
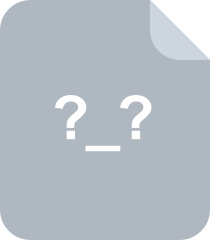
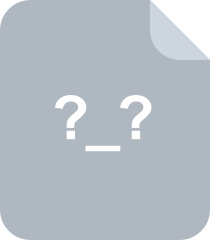
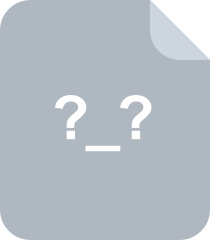
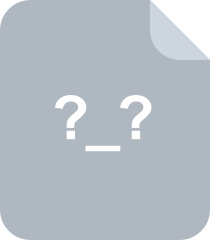
共 190 条
- 1
- 2
资源评论
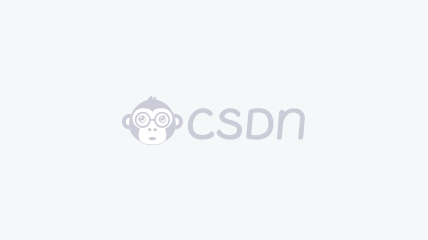

枫蜜柚子茶
- 粉丝: 9019
- 资源: 5351
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

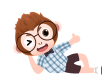
最新资源
- 排序算法中插入排序C++实现及其特性
- 土地出让数据集(2000-2022.12)(104.8W+ 记录,48特征)CSV
- MFC如何修改多文档视图的标签
- 无人机路径规划中基于DDPG算法的MATLAB实现与信噪比优化
- 配电网电压与无功协调优化 以最小化运行成本(包含开关动作成本、功率损耗成本以及设备运行成本)和电压偏差为目标函数,考虑分布式电源的接入,采用线性化和二次松弛方法,将非凸模型转化为二阶锥规划模型,通过优
- MATLAB轴承动力学代码(正常、外圈故障、内圈故障、滚动体故障),根据滚动轴承故障机理建模(含数学方程建立和公式推导)并在MATLAB中采用ODE45进行数值计算 可模拟不同轴承故障类型,输出时域
- comsol模拟冻土水土热力盐四个物理场耦合
- Qt源码~~EQ曲线升级版 代码写的不错,注释也很详细了
- Halcon深度图渲染
- 01前端 / Node.js
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


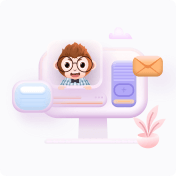
安全验证
文档复制为VIP权益,开通VIP直接复制
