<html>
<head>
<meta charset="utf-8">
<title></title>
<style>
html {
height: 100%;
background-image: -webkit-radial-gradient(ellipse farthest-corner at center center, #1b44e4 0%, #020f3a 100%);
background-image: radial-gradient(ellipse farthest-corner at center center, #1b44e4 0%, #020f3a 100%);
cursor: move;
}
body {
width: 100%;
margin: 0;
overflow: hidden;
}
</style>
</head>
<body>
<canvas id="canv" width="1920" height="572"></canvas>
<script>
var num = 200;
var w = window.innerWidth;
var h = window.innerHeight;
var max = 100;
var _x = 0;
var _y = 0;
var _z = 150;
var dtr = function(d) {
return d * Math.PI / 180;
};
var rnd = function() {
return Math.sin(Math.floor(Math.random() * 360) * Math.PI / 180);
};
var dist = function(p1, p2, p3) {
return Math.sqrt(Math.pow(p2.x - p1.x, 2) + Math.pow(p2.y - p1.y, 2) + Math.pow(p2.z - p1.z, 2));
};
var cam = {
obj: {
x: _x,
y: _y,
z: _z
},
dest: {
x: 0,
y: 0,
z: 1
},
dist: {
x: 0,
y: 0,
z: 200
},
ang: {
cplane: 0,
splane: 0,
ctheta: 0,
stheta: 0
},
zoom: 1,
disp: {
x: w / 2,
y: h / 2,
z: 0
},
upd: function() {
cam.dist.x = cam.dest.x - cam.obj.x;
cam.dist.y = cam.dest.y - cam.obj.y;
cam.dist.z = cam.dest.z - cam.obj.z;
cam.ang.cplane = -cam.dist.z / Math.sqrt(cam.dist.x * cam.dist.x + cam.dist.z * cam.dist.z);
cam.ang.splane = cam.dist.x / Math.sqrt(cam.dist.x * cam.dist.x + cam.dist.z * cam.dist.z);
cam.ang.ctheta = Math.sqrt(cam.dist.x * cam.dist.x + cam.dist.z * cam.dist.z) / Math.sqrt(cam.dist.x * cam.dist.x + cam.dist.y * cam.dist.y + cam.dist.z * cam.dist.z);
cam.ang.stheta = -cam.dist.y / Math.sqrt(cam.dist.x * cam.dist.x + cam.dist.y * cam.dist.y + cam.dist.z * cam.dist.z);
}
};
var trans = {
parts: {
sz: function(p, sz) {
return {
x: p.x * sz.x,
y: p.y * sz.y,
z: p.z * sz.z
};
},
rot: {
x: function(p, rot) {
return {
x: p.x,
y: p.y * Math.cos(dtr(rot.x)) - p.z * Math.sin(dtr(rot.x)),
z: p.y * Math.sin(dtr(rot.x)) + p.z * Math.cos(dtr(rot.x))
};
},
y: function(p, rot) {
return {
x: p.x * Math.cos(dtr(rot.y)) + p.z * Math.sin(dtr(rot.y)),
y: p.y,
z: -p.x * Math.sin(dtr(rot.y)) + p.z * Math.cos(dtr(rot.y))
};
},
z: function(p, rot) {
return {
x: p.x * Math.cos(dtr(rot.z)) - p.y * Math.sin(dtr(rot.z)),
y: p.x * Math.sin(dtr(rot.z)) + p.y * Math.cos(dtr(rot.z)),
z: p.z
};
}
},
pos: function(p, pos) {
return {
x: p.x + pos.x,
y: p.y + pos.y,
z: p.z + pos.z
};
}
},
pov: {
plane: function(p) {
return {
x: p.x * cam.ang.cplane + p.z * cam.ang.splane,
y: p.y,
z: p.x * -cam.ang.splane + p.z * cam.ang.cplane
};
},
theta: function(p) {
return {
x: p.x,
y: p.y * cam.ang.ctheta - p.z * cam.ang.stheta,
z: p.y * cam.ang.stheta + p.z * cam.ang.ctheta
};
},
set: function(p) {
return {
x: p.x - cam.obj.x,
y: p.y - cam.obj.y,
z: p.z - cam.obj.z
};
}
},
persp: function(p) {
return {
x: p.x * cam.dist.z / p.z * cam.zoom,
y: p.y * cam.dist.z / p.z * cam.zoom,
z: p.z * cam.zoom,
p: cam.dist.z / p.z
};
},
disp: function(p, disp) {
return {
x: p.x + disp.x,
y: -p.y + disp.y,
z: p.z + disp.z,
p: p.p
};
},
steps: function(_obj_, sz, rot, pos, disp) {
var _args = trans.parts.sz(_obj_, sz);
_args = trans.parts.rot.x(_args, rot);
_args = trans.parts.rot.y(_args, rot);
_args = trans.parts.rot.z(_args, rot);
_args = trans.parts.pos(_args, pos);
_args = trans.pov.plane(_args);
_args = trans.pov.theta(_args);
_args = trans.pov.set(_args);
_args = trans.persp(_args);
_args = trans.disp(_args, disp);
return _args;
}
};
(function() {
"use strict";
var threeD = function(param) {
this.transIn = {};
this.transOut = {};
this.transIn.vtx = (param.vtx);
this.transIn.sz = (param.sz);
this.transIn.rot = (param.rot);
this.transIn.pos = (param.pos);
};
threeD.prototype.vupd = function() {
this.transOut = trans.steps(
this.transIn.vtx,
this.transIn.sz,
this.transIn.rot,
this.transIn.pos,
cam.disp
);
};
var Build = function() {
this.vel = 0.04;
this.lim = 360;
this.diff = 200;
this.initPos = 100;
this.toX = _x;
this.toY = _y;
this.go();
};
Build.prototype.go = function() {
this.canvas = document.getElementById("canv");
this.canvas.width = window.innerWidth;
this.canvas.height = window.innerHeight;
this.$ = canv.getContext("2d");
this.$.globalCompositeOperation = 'source-over';
this.varr = [];
this.dist = [];
this.calc = [];
for (var i = 0, len = num; i < len; i++) {
this.add();
}
this.rotObj = {
x: 0,
y: 0,
z: 0
};
this.objSz = {
x: w / 5,
y: h / 5,
z: w / 5
};
};
Build.prototype.add = function() {
this.varr.push(new threeD({
vtx: {
x: rnd(),
y: rnd(),
z: rnd()
},
sz: {
x: 0,
y: 0,
z: 0
},
rot: {
x: 20,
y: -20,
z: 0
},
pos: {
x: this.diff * Math.sin(360 * Math.random() * Math.PI / 180),
y: this.diff * Math.sin(360 * Math.random() * Math.PI / 180),
z: this.diff * Math.sin(360 * Math.random() * Math.PI / 180)
}
}));
this.calc.push({
x: 360 * Math.random(),
y: 360 * Math.random(),
z: 360 * Math.random()
});
};
Build.prototype.upd = function() {
cam.obj.x += (this.toX - cam.obj.x) * 0.05;
cam.obj.y += (this.toY - cam.obj.y) * 0.05;
};
Build.prototype.draw = function() {
this.$.clearRect(0, 0, this.canvas.width, this.canvas.height);
cam.upd();
this.rotObj.x += 0.1;
this.rotObj.y += 0.1;
this.rotObj.z += 0.1;
for (var i = 0; i < this.varr.length; i++) {
for (var val in this.calc[i]) {
if (this.calc[i].hasOwnProperty(val)) {
this.calc[i][val] += this.vel;
if (this.calc[i][val] > this.lim) this.calc[i][val] = 0;
}
}
this.varr[i].transIn.pos = {
x: this.diff * Math.cos(this.calc[i].x * Math.PI / 180),
y: this.diff * Math.sin(this.calc[i].y * Math.PI / 180),
z: this.diff * Math.sin(this.calc[i].z * Math.PI / 180)
};
this.varr[i].transIn.rot = this.rotObj;
this.varr[i].transIn.sz = this.objSz;
this.varr[i].vupd();
if (this.varr[i].transOut.p < 0) continue;
var g = this.$.createRadialGradient(this.varr[i].transOut.x, this.varr[i].transOut.y, this.varr[i].transOut.p, this.varr[i].transOut.x, this.varr[i].transOut.y, this.varr[i].transOut.p * 2);
this.$.globalCompositeOperation = 'lighter';
g.addColorStop(0, 'hsla(255, 255%, 255%, 1)');
g.addColorStop(.5, 'hsla(' + (i + 2) + ',85%, 40%,1)');
g.addColorStop(1, 'hsla(' + (i) + ',85%, 40%,.5)');
this.$.fillStyle = g;
this.$.beginPath();
this.$.arc(this.varr[i].transOut.x, this.varr[i].transOut.y, this.varr[i].transOut.p * 2, 0, Math.PI * 2, false);
this.$.fill();
this.$.closePath();
}
};
Build.prototype.anim = function() {
window.requestAnimationFrame = (function() {
return window.requestAnimationFrame ||
function(callback, element) {
window.setTimeout(callback, 1000 / 60);
};
})();
var anim = function() {
this.upd();
this.draw();
window.requestAnimationFrame(anim);
没有合适的资源?快使用搜索试试~ 我知道了~
【前端模板】027 全国消费者情况看板.zip
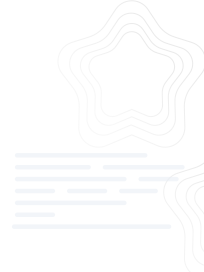
共13个文件
js:5个
png:4个
html:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 149 浏览量
2024-03-19
23:38:24
上传
评论
收藏 1.33MB ZIP 举报
温馨提示
大数据分析页面通常是指用于展示和分析大数据集的界面或页面。这些页面通常包含数据可视化工具、数据过滤器、图表和表格,以便用户能够直观地理解和分析大数据集。大数据分析页面通常用于商业智能、数据科学、市场分析等领域,帮助用户从海量数据中提取有用信息和见解。 大数据分析页面涉及多种技术和工具,用于处理和展示大数据集。以下是一些常用的技术和工具: 大数据处理框架:如Apache Hadoop、Apache Spark等,用于处理大规模数据集的分布式计算框架。 数据存储技术:包括传统的关系型数据库(如MySQL、PostgreSQL)、NoSQL数据库(如MongoDB、Cassandra)以及数据湖(Data Lake)等存储技术。 数据可视化工具:如Tableau、Power BI、D3.js等,用于将数据转换为图表、地图等可视化形式,帮助用户理解数据。 数据分析工具:如Python的pandas、NumPy、SciPy库,R语言等,用于数据处理、统计分析和机器学习。 前端开发技术:如HTML、CSS、JavaScript等,用于构建交互式的数据分析页面。
资源推荐
资源详情
资源评论
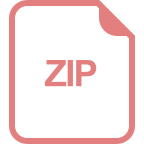
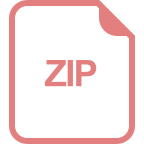
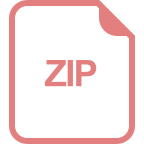
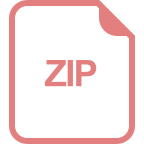
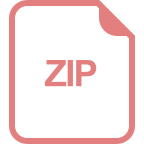
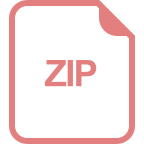
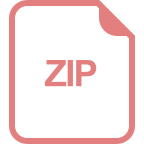
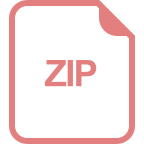
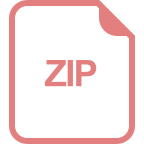
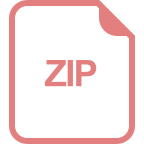
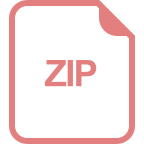
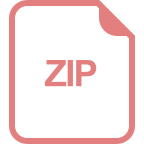
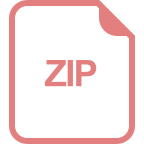
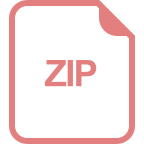
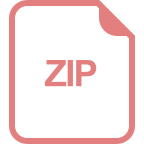
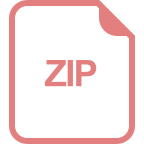
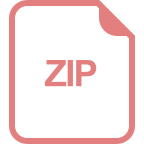
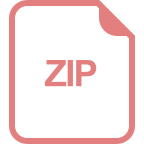
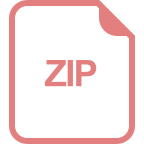
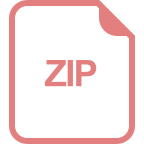
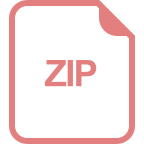
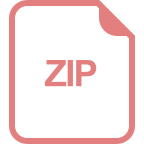
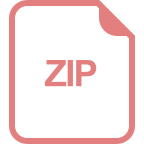
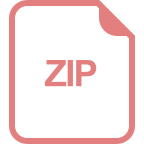
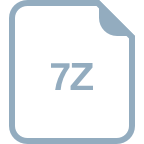
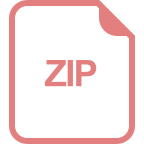
收起资源包目录


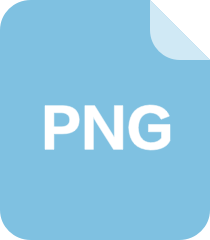

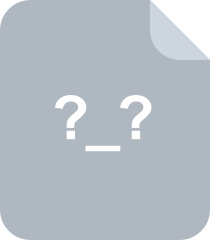
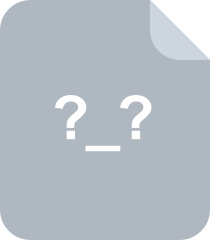
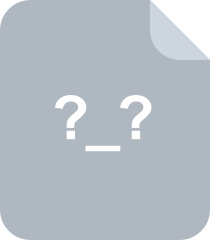
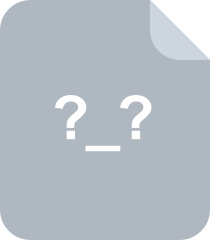
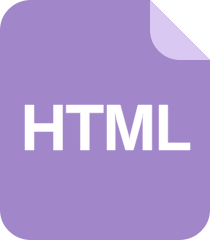
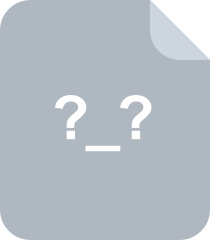

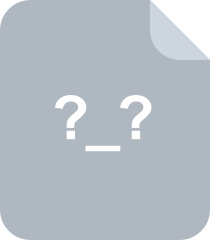
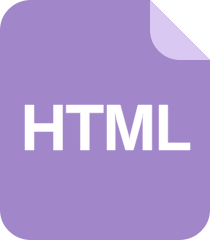

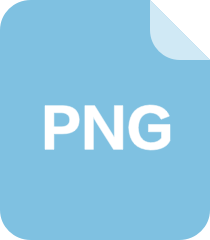
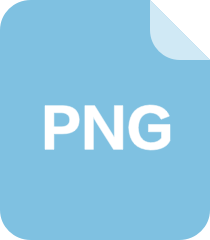
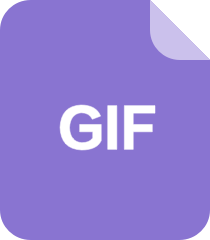
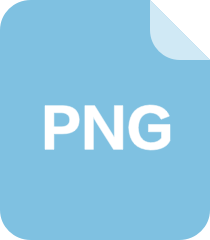
共 13 条
- 1
资源评论
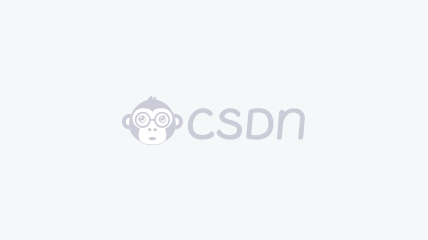

枫蜜柚子茶
- 粉丝: 8971
- 资源: 5351
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

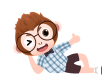
最新资源
- (源码)基于wxWidgets库的QMiniIDE游戏开发环境管理系统.zip
- 通过C++实现原型模式(Prototype Pattern).rar
- 学习记录111111111111111111111111
- 通过java实现原型模式(Prototype Pattern).rar
- 通过python实现原型模式(Prototype Pattern).rar
- xiefrnsdklmkds
- 基于PyQt5+pytorch的在线疲劳检测系统项目源码+文档说明(Python毕业设计)
- Excel表格拆分工具.exe
- Python毕业设计基于PyQt5+pytorch的在线疲劳检测系统项目源码+文档说明
- 基于Unity开发的消消乐小游戏源代码(毕业设计和大作业适用).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


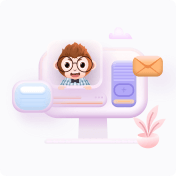
安全验证
文档复制为VIP权益,开通VIP直接复制
