/**
******************************************************************************
* @file stm32f7xx_hal_cryp.c
* @author MCD Application Team
* @brief CRYP HAL module driver.
* This file provides firmware functions to manage the following
* functionalities of the Cryptography (CRYP) peripheral:
* + Initialization, de-initialization, set config and get config functions
* + DES/TDES, AES processing functions
* + DMA callback functions
* + CRYP IRQ handler management
* + Peripheral State functions
*
@verbatim
==============================================================================
##### How to use this driver #####
==============================================================================
[..]
The CRYP HAL driver can be used in CRYP or TinyAES IP as follows:
(#)Initialize the CRYP low level resources by implementing the HAL_CRYP_MspInit():
(##) Enable the CRYP interface clock using __HAL_RCC_CRYP_CLK_ENABLE()or __HAL_RCC_AES_CLK_ENABLE for TinyAES IP
(##) In case of using interrupts (e.g. HAL_CRYP_Encrypt_IT())
(+++) Configure the CRYP interrupt priority using HAL_NVIC_SetPriority()
(+++) Enable the CRYP IRQ handler using HAL_NVIC_EnableIRQ()
(+++) In CRYP IRQ handler, call HAL_CRYP_IRQHandler()
(##) In case of using DMA to control data transfer (e.g. HAL_CRYP_Encrypt_DMA())
(+++) Enable the DMAx interface clock using __RCC_DMAx_CLK_ENABLE()
(+++) Configure and enable two DMA streams one for managing data transfer from
memory to peripheral (input stream) and another stream for managing data
transfer from peripheral to memory (output stream)
(+++) Associate the initialized DMA handle to the CRYP DMA handle
using __HAL_LINKDMA()
(+++) Configure the priority and enable the NVIC for the transfer complete
interrupt on the two DMA Streams. The output stream should have higher
priority than the input stream HAL_NVIC_SetPriority() and HAL_NVIC_EnableIRQ()
(#)Initialize the CRYP according to the specified parameters :
(##) The data type: 1-bit, 8-bit, 16-bit or 32-bit.
(##) The key size: 128, 192 or 256.
(##) The AlgoMode DES/ TDES Algorithm ECB/CBC or AES Algorithm ECB/CBC/CTR/GCM or CCM.
(##) The initialization vector (counter). It is not used in ECB mode.
(##) The key buffer used for encryption/decryption.
(##) The Header used only in AES GCM and CCM Algorithm for authentication.
(##) The HeaderSize The size of header buffer in word.
(##) The B0 block is the first authentication block used only in AES CCM mode.
(#)Three processing (encryption/decryption) functions are available:
(##) Polling mode: encryption and decryption APIs are blocking functions
i.e. they process the data and wait till the processing is finished,
e.g. HAL_CRYP_Encrypt & HAL_CRYP_Decrypt
(##) Interrupt mode: encryption and decryption APIs are not blocking functions
i.e. they process the data under interrupt,
e.g. HAL_CRYP_Encrypt_IT & HAL_CRYP_Decrypt_IT
(##) DMA mode: encryption and decryption APIs are not blocking functions
i.e. the data transfer is ensured by DMA,
e.g. HAL_CRYP_Encrypt_DMA & HAL_CRYP_Decrypt_DMA
(#)When the processing function is called at first time after HAL_CRYP_Init()
the CRYP peripheral is configured and processes the buffer in input.
At second call, no need to Initialize the CRYP, user have to get current configuration via
HAL_CRYP_GetConfig() API, then only HAL_CRYP_SetConfig() is requested to set
new parametres, finally user can start encryption/decryption.
(#)Call HAL_CRYP_DeInit() to deinitialize the CRYP peripheral.
(#)To process a single message with consecutive calls to HAL_CRYP_Encrypt() or HAL_CRYP_Decrypt()
without having to configure again the Key or the Initialization Vector between each API call,
the field KeyIVConfigSkip of the initialization structure must be set to CRYP_KEYIVCONFIG_ONCE.
Same is true for consecutive calls of HAL_CRYP_Encrypt_IT(), HAL_CRYP_Decrypt_IT(), HAL_CRYP_Encrypt_DMA()
or HAL_CRYP_Decrypt_DMA().
[..]
The cryptographic processor supports following standards:
(#) The data encryption standard (DES) and Triple-DES (TDES) supported only by CRYP1 IP:
(##)64-bit data block processing
(##) chaining modes supported :
(+++) Electronic Code Book(ECB)
(+++) Cipher Block Chaining (CBC)
(##) keys length supported :64-bit, 128-bit and 192-bit.
(#) The advanced encryption standard (AES) supported by CRYP1 & TinyAES IP:
(##)128-bit data block processing
(##) chaining modes supported :
(+++) Electronic Code Book(ECB)
(+++) Cipher Block Chaining (CBC)
(+++) Counter mode (CTR)
(+++) Galois/counter mode (GCM/GMAC)
(+++) Counter with Cipher Block Chaining-Message(CCM)
(##) keys length Supported :
(+++) for CRYP1 IP: 128-bit, 192-bit and 256-bit.
(+++) for TinyAES IP: 128-bit and 256-bit
[..] This section describes the AES Galois/counter mode (GCM) supported by both CRYP1 IP:
(#) Algorithm supported :
(##) Galois/counter mode (GCM)
(##) Galois message authentication code (GMAC) :is exactly the same as
GCM algorithm composed only by an header.
(#) Four phases are performed in GCM :
(##) Init phase: IP prepares the GCM hash subkey (H) and do the IV processing
(##) Header phase: IP processes the Additional Authenticated Data (AAD), with hash
computation only.
(##) Payload phase: IP processes the plaintext (P) with hash computation + keystream
encryption + data XORing. It works in a similar way for ciphertext (C).
(##) Final phase: IP generates the authenticated tag (T) using the last block of data.
(#) structure of message construction in GCM is defined as below :
(##) 16 bytes Initial Counter Block (ICB)composed of IV and counter
(##) The authenticated header A (also knows as Additional Authentication Data AAD)
this part of the message is only authenticated, not encrypted.
(##) The plaintext message P is both authenticated and encrypted as ciphertext.
GCM standard specifies that ciphertext has same bit length as the plaintext.
(##) The last block is composed of the length of A (on 64 bits) and the length of ciphertext
(on 64 bits)
[..] This section describe The AES Counter with Cipher Block Chaining-Message
Authentication Code (CCM) supported by both CRYP1 IP:
(#) Specific parameters for CCM :
(##) B0 block : According to NIST Special Publication 800-38C,
The first block B0 is formatted as follows, where l(m) is encoded in
most-significant-byte first order(see below table 3)
(+++) Q: a bit string representation of the octet length of P (plaintext)
(+++) q The octet length of the binary representation of the octet length of the payload
(+++) A nonce (N), n The octet length of the where n+q=15.
(+++) Flags: most significant octet containing four flags for control information,
(+++) t The octet length of the MAC.
(##) B1 block (header) : associated data length(a)
没有合适的资源?快使用搜索试试~ 我知道了~
STM32F767驱动GM196模组,实现UDP网络通信【支持STM32F7系列单片机】.zip
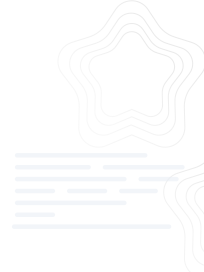
共234个文件
h:123个
c:107个
uvoptx:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 96 浏览量
2023-06-02
18:48:14
上传
评论
收藏 1.65MB ZIP 举报
温馨提示
STM32驱动GM196模组,实现UDP网络通信。 项目代码可直接编译运行~
资源推荐
资源详情
资源评论
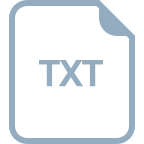
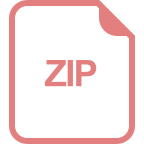
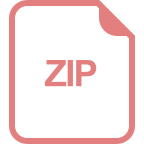
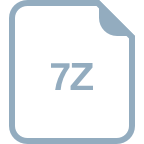
收起资源包目录

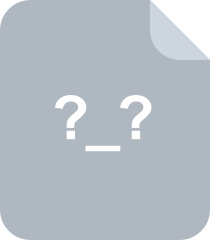
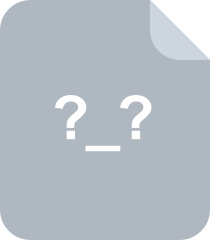
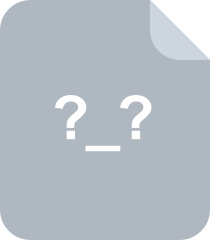
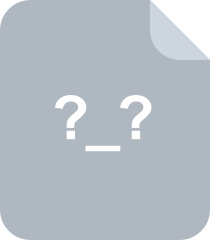
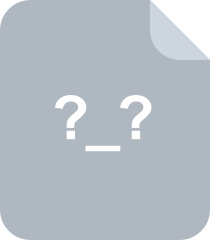
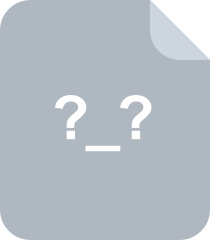
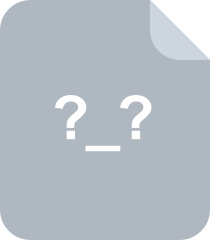
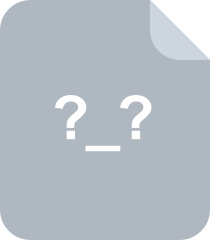
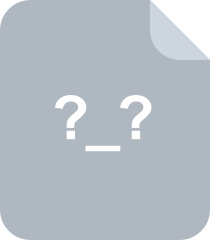
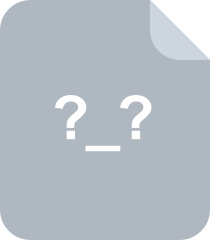
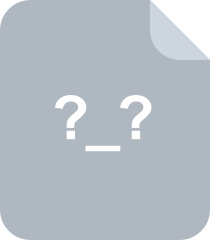
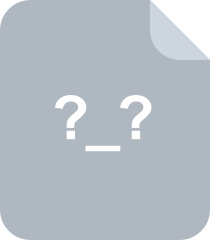
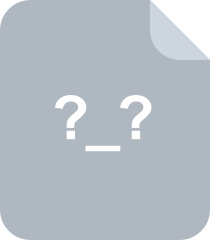
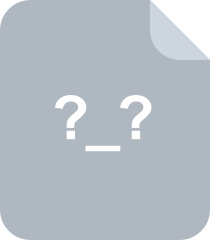
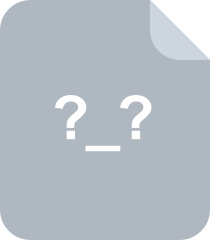
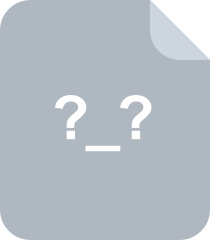
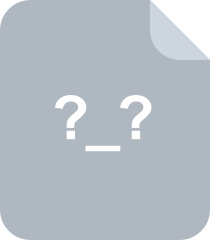
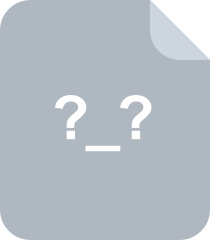
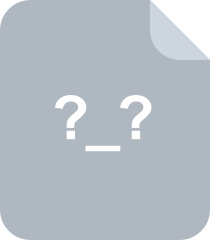
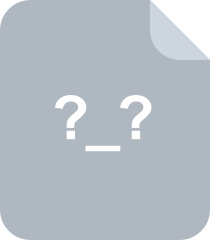
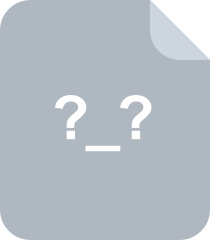
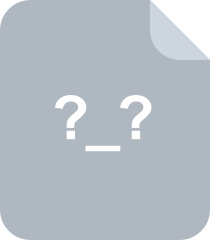
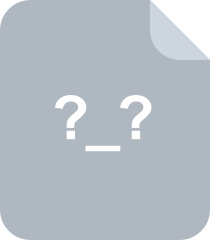
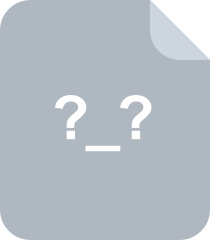
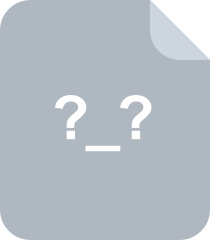
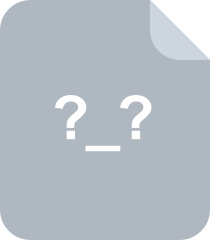
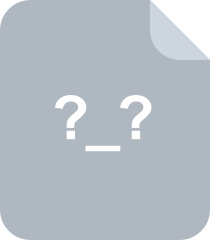
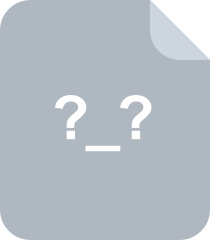
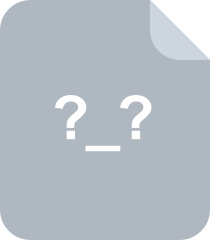
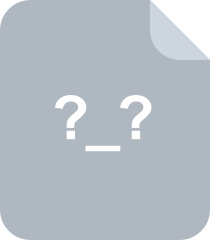
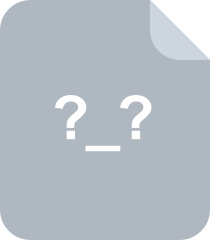
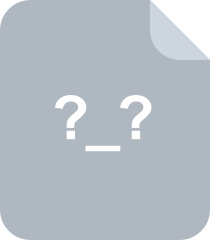
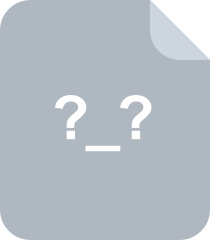
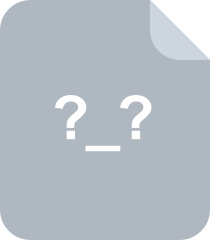
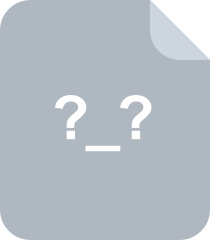
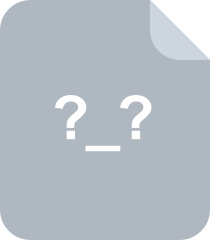
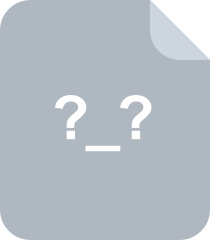
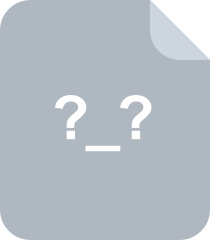
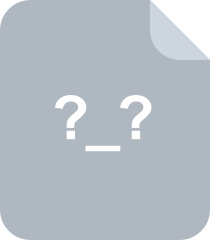
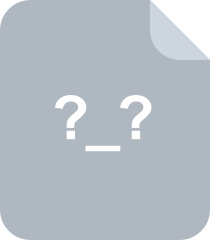
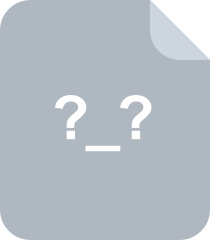
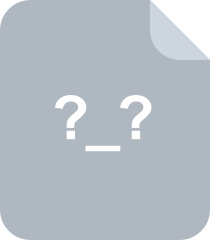
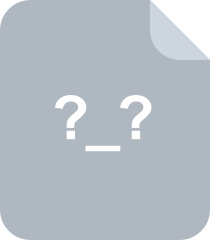
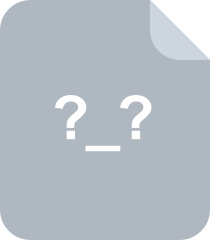
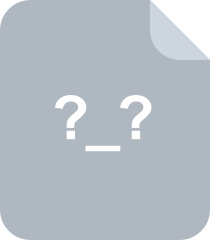
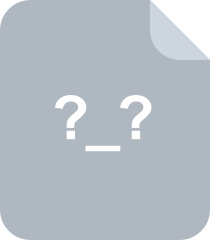
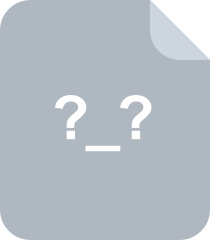
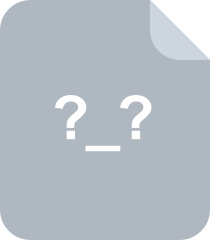
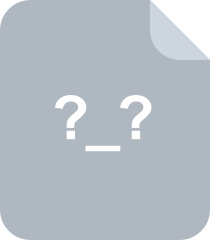
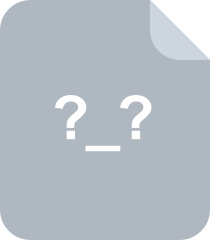
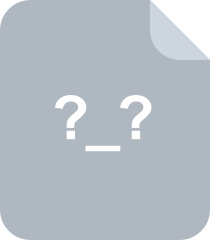
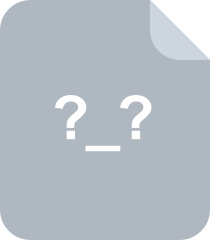
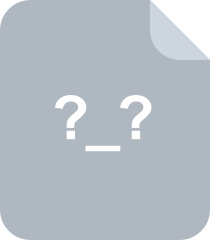
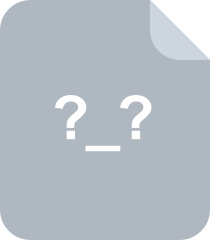
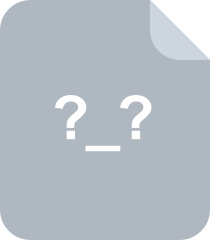
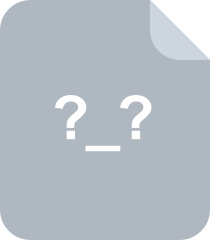
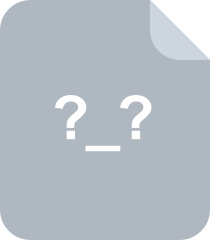
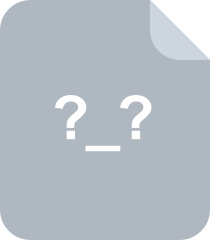
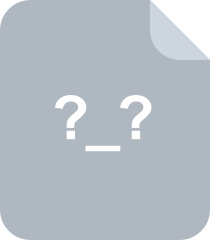
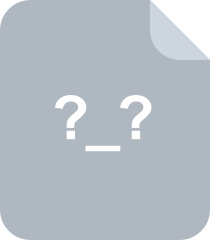
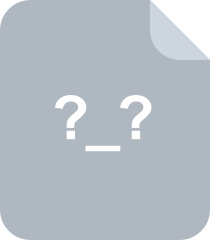
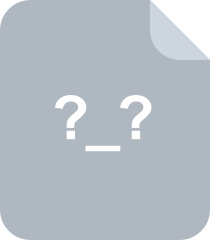
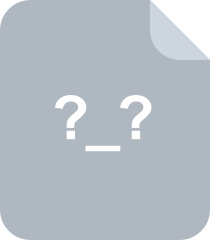
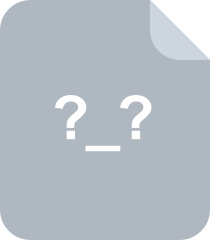
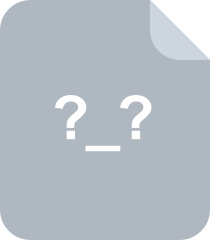
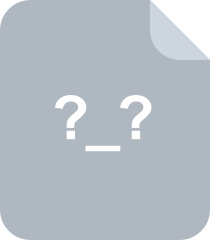
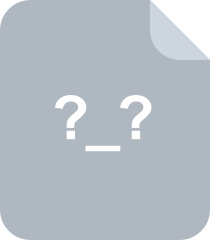
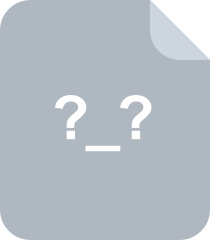
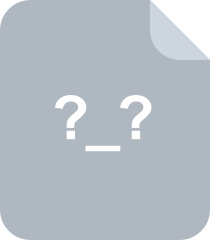
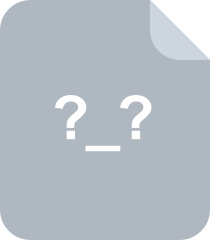
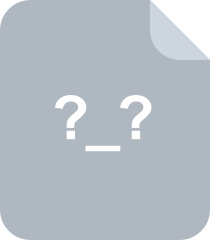
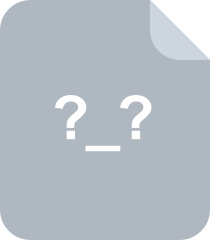
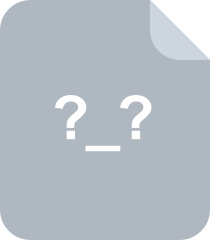
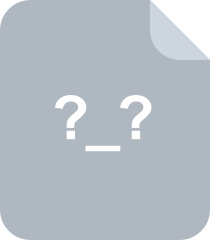
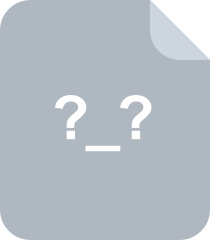
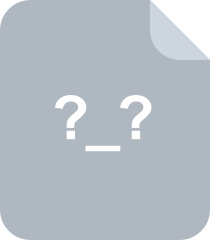
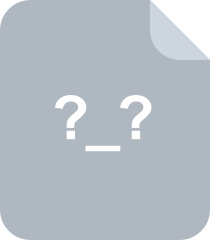
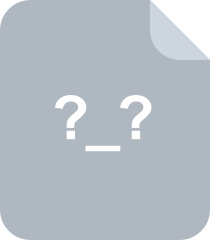
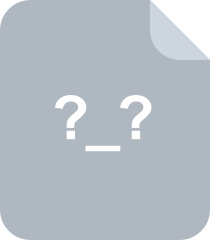
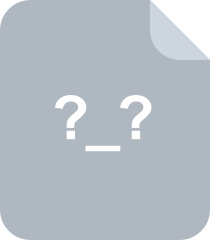
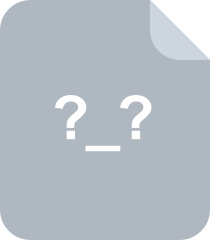
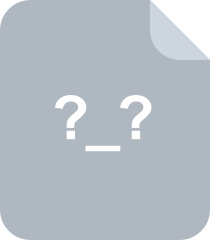
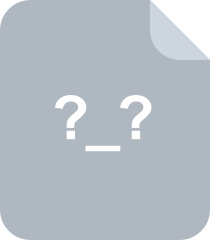
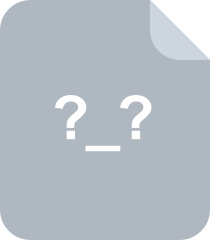
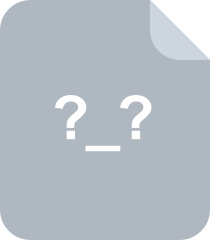
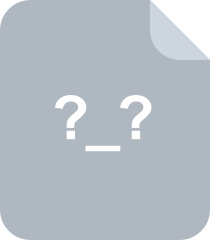
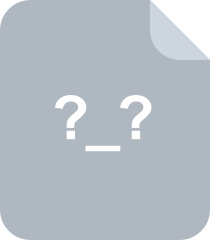
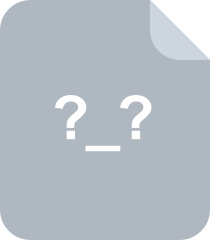
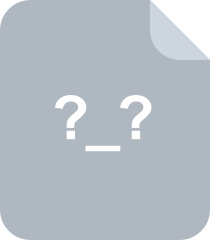
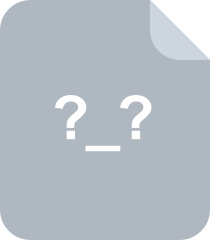
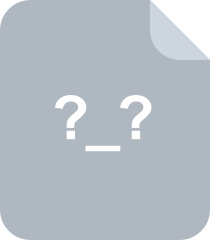
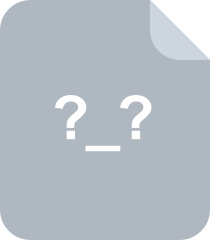
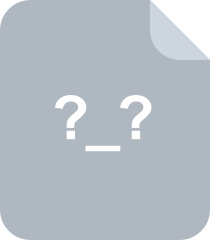
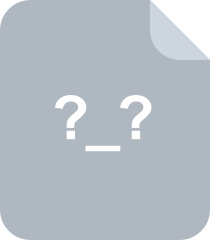
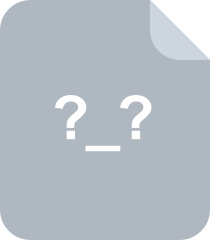
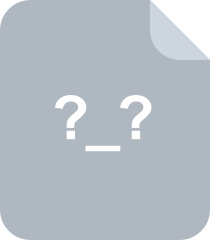
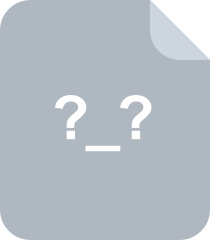
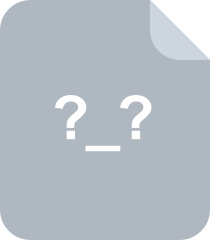
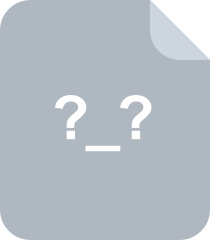
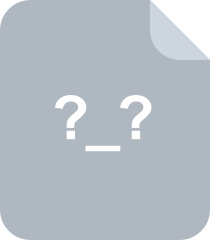
共 234 条
- 1
- 2
- 3
资源评论
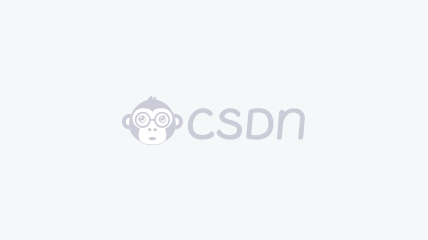
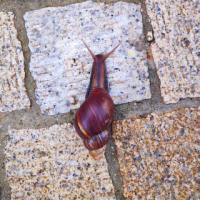

不脱发的程序猿
- 粉丝: 26w+
- 资源: 5888
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

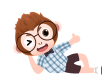
最新资源
- 学习java的多态之接口代码.zip
- 用python编写的一个敏感信息扫描工具
- C++实现的一个管理系统源码.zip
- 机械设计弹簧圈自动分选装盒设备creo5.0非常好的设计图纸100%好用.zip
- c#管理系统小实例源码+数据库库文件(SqlServer)
- 机械设计大产能易撕贴胶纸机sw18非常好的设计图纸100%好用.zip
- 机械设计氮气弹簧装配线sw18可编辑非常好的设计图纸100%好用.zip
- Nacos架构和原理介绍,出自阿里团队
- 机械设计电芯厚度测试仪sw18非常好的设计图纸100%好用.zip
- Spring Boot综合项目瑞吉外卖
- 视频目标检测zzzzzzz
- 基于java+ssm+mysql+vue的公交车信息管理系统开题报告.docx
- 基于java+ssm+mysql的OA办公系统开题报告.docx
- 文字生成图片-可灵1.6
- 基于java+ssm+mysql的毕业设计选题系统任务书.docx
- 机械设计斗山220LM数控车床sw16可编辑非常好的设计图纸100%好用.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


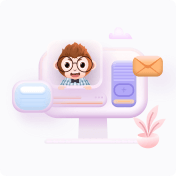
安全验证
文档复制为VIP权益,开通VIP直接复制
