/**
**********************************************************************************************************************
* @file stm32h7rsxx_hal_i3c.c
* @author MCD Application Team
* @brief I3C HAL module driver.
* This file provides firmware functions to manage the following
* functionalities of the Improvement Inter Integrated Circuit (I3C) peripheral:
* + Initialization and de-initialization functions
* + IO operation functions
* + Peripheral State and Errors functions
*
**********************************************************************************************************************
* @attention
*
* Copyright (c) 2022 STMicroelectronics.
* All rights reserved.
*
* This software is licensed under terms that can be found in the LICENSE file
* in the root directory of this software component.
* If no LICENSE file comes with this software, it is provided AS-IS.
*
**********************************************************************************************************************
@verbatim
======================================================================================================================
##### How to use this driver #####
======================================================================================================================
[..]
The I3C HAL driver can be used as follows:
(#) Declare a I3C_HandleTypeDef handle structure, for example:
I3C_HandleTypeDef hi3c;
(#) Declare a I3C_XferTypeDef transfer descriptor structure, for example:
I3C_XferTypeDef ContextBuffers;
(#)Initialize the I3C low level resources by implementing the HAL_I3C_MspInit() API:
(##) Enable the I3Cx interface clock
(##) I3C pins configuration
(+++) Enable the clock for the I3C GPIOs
(+++) Configure I3C pins as alternate function push-pull with no-pull
(##) NVIC configuration if you need to use interrupt process
(+++) Configure the I3Cx interrupt priority
(+++) Enable the NVIC I3C IRQ Channel
(##) DMA Configuration if you need to use DMA process
(+++) Declare a DMA_HandleTypeDef handle structure for
the Command Common Code (CCC) management channel
(+++) Declare a DMA_HandleTypeDef handle structure for
the transmit channel
(+++) Declare a DMA_HandleTypeDef handle structure for
the receive channel
(+++) Declare a DMA_HandleTypeDef handle structure for
the status channel
(+++) Enable the DMAx interface clock
(+++) Configure the DMA handle parameters
(+++) Configure the DMA Command Common Code (CCC) channel
(+++) Configure the DMA Tx channel
(+++) Configure the DMA Rx channel
(+++) Configure the DMA Status channel
(+++) Associate the initialized DMA handle to the hi3c DMA CCC, Tx, Rx or Status handle as necessary
(+++) Configure the priority and enable the NVIC for the transfer complete interrupt on
the DMA CCC, Tx, Rx or Status instance
(#) Configure the HAL I3C Communication Mode as Controller or Target in the hi3c Init structure.
(#) Configure the Controller Communication Bus characterics for Controller mode.
This mean, configure the parameters SDAHoldTime, WaitTime, SCLPPLowDuration,
SCLI3CHighDuration, SCLODLowDuration, SCLI2CHighDuration, BusFreeDuration,
BusIdleDuration in the LL_I3C_CtrlBusConfTypeDef structure through h3c Init structure.
(#) Configure the Target Communication Bus characterics for Target mode.
This mean, configure the parameter BusAvailableDuration in the LL_I3C_TgtBusConfTypeDef structure
through h3c Init structure.
All these parameters for Controller or Target can be configured directly in user code or
by using CubeMx generation.
To help the computation of the different parameters, the recommendation is to use CubeMx.
Those parameters can be modified after the hi3c initialization by using
HAL_I3C_Ctrl_BusCharacteristicConfig() for controller and
HAL_I3C_Tgt_BusCharacteristicConfig() for target.
(#) Initialize the I3C registers by calling the HAL_I3C_Init(), configures also the low level Hardware
(GPIO, CLOCK, NVIC...etc) by calling the customized HAL_I3C_MspInit(&hi3c) API.
(#) Configure the different FIFO parameters in I3C_FifoConfTypeDef structure as RxFifoThreshold, TxFifoThreshold
for Controller or Target mode.
And enable/disable the Control or Status FIFO only for Controller Mode.
Use HAL_I3C_SetConfigFifo() function to finalize the configuration, and HAL_I3C_GetConfigFifo() to retrieve
FIFO configuration.
Possibility to clear the FIFO configuration by using HAL_I3C_ClearConfigFifo() which reset the configuration
FIFO to their default hardware value
(#) Configure the different additional Controller configuration in I3C_CtrlConfTypeDef structure as DynamicAddr,
StallTime, HotJoinAllowed, ACKStallState, CCCStallState, TxStallState, RxStallState, HighKeeperSDA.
Use HAL_I3C_Ctrl_Config() function to finalize the Controller configuration.
(#) Configure the different additional Target configuration in I3C_TgtConfTypeDef structure as Identifier,
MIPIIdentifier, CtrlRoleRequest, HotJoinRequest, IBIRequest, IBIPayload, IBIPayloadSize, MaxReadDataSize,
MaxWriteDataSize, CtrlCapability, GroupAddrCapability, DataTurnAroundDuration, MaxReadTurnAround,
MaxDataSpeed, MaxSpeedLimitation, HandOffActivityState, HandOffDelay, PendingReadMDB.
Use HAL_I3C_Tgt_Config() function to finalize the Target configuration.
(#) Before initiate any IO operation, the application must launch an assignment of the different
Target dynamic address by using HAL_I3C_Ctrl_DynAddrAssign() in polling mode or
HAL_I3C_Ctrl_DynAddrAssign_IT() in interrupt mode.
This procedure is named Enter Dynamic Address Assignment (ENTDAA CCC command).
For the initiation of ENTDAA procedure from the controller, each target connected and powered on the I3C bus
must repond to this particular Command Common Code by sending its proper Payload (a amount of 48bits which
contain the target characteristics)
Each time a target responds to ENTDAA sequence, the application is informed through
HAL_I3C_TgtReqDynamicAddrCallback() of the reception of the target paylaod.
And then application must send a associated dynamic address through HAL_I3C_Ctrl_SetDynAddr().
This procedure in loop automatically in hardware side until a target respond to repeated ENTDAA sequence.
The application is informed of the end of the procedure at reception of HAL_I3C_CtrlDAACpltCallback().
Then application can easily retrieve ENTDAA payload information through HAL_I3C_Get_ENTDAA_Payload_Info()
function.
At the end of procedure, the function HAL_I3C_Ctrl_ConfigBusDevices() must be called to store in hardware
register part the target capabilities as Dynamic address, IBI support with or without additional data byte,
Controller role request support, Controller stop transfer after IBI through I3C_DeviceConfTypeDef structure.
(#) Other action to be done, before initiate any IO operation, the application must prepare the different frame
descriptor with its associated buffer allocation in their side.
Configure the different information related to CCC transfer through I3C_CCCTypeDef structure
Configure the different informati
没有合适的资源?快使用搜索试试~ 我知道了~
STM32H7R实现制作USB读卡器(Slave)【支持STM32H7R系列】
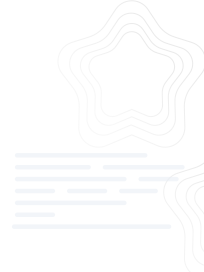
共363个文件
h:187个
c:169个
uvoptx:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
STM32H7R系列驱动程序,代码可直接编译运行~
资源推荐
资源详情
资源评论
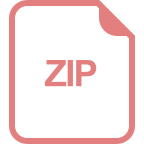
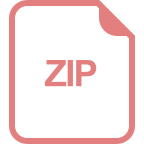
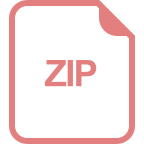
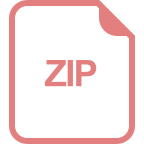
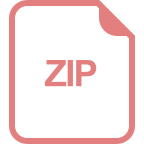
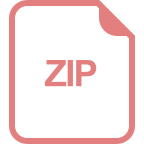
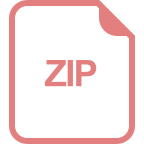
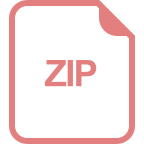
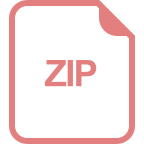
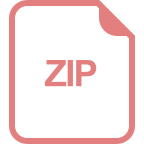
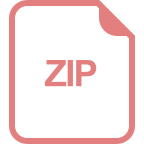
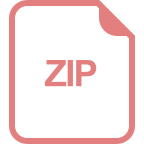
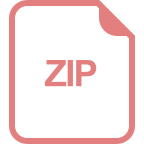
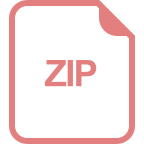
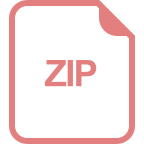
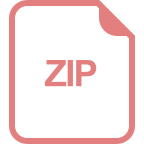
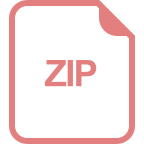
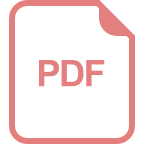
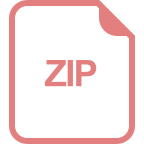
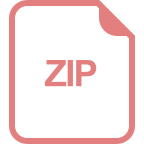
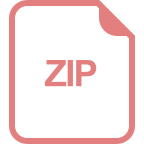
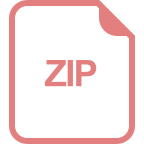
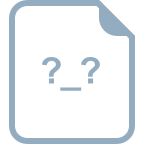
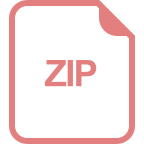
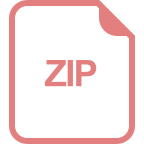
收起资源包目录

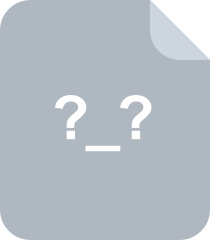
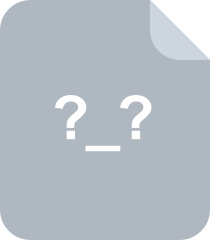
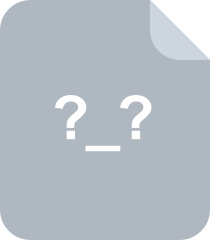
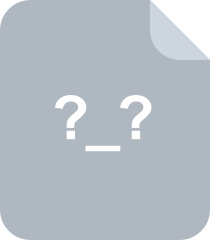
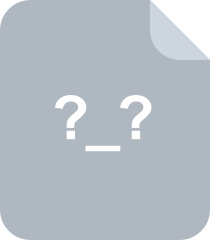
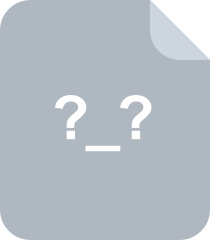
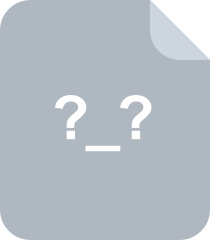
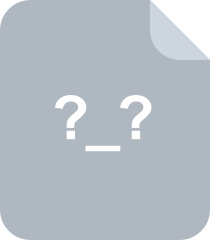
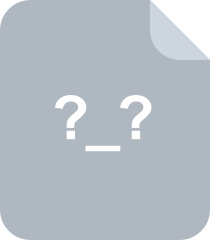
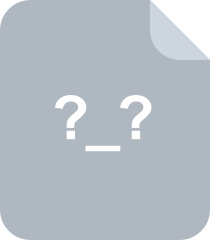
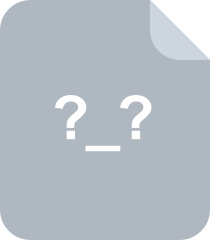
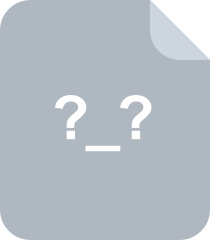
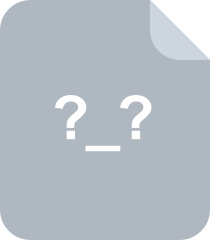
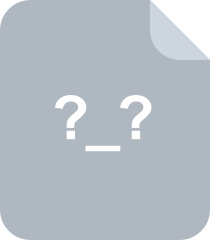
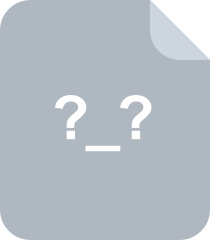
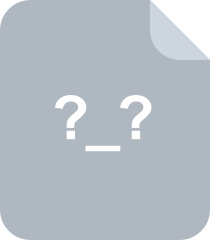
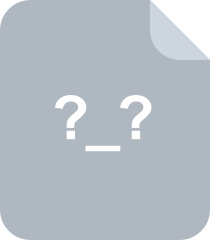
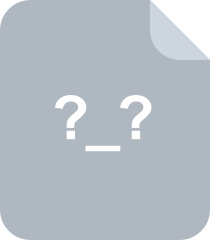
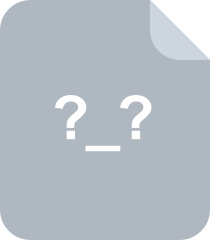
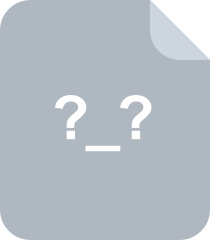
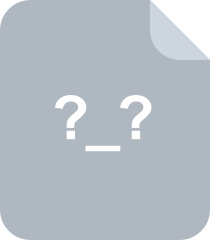
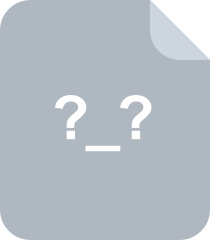
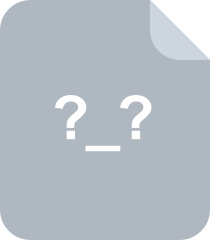
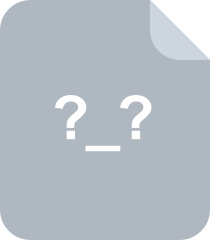
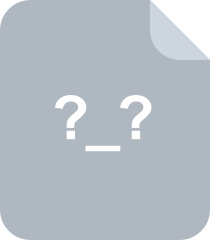
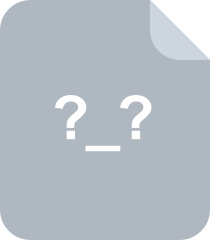
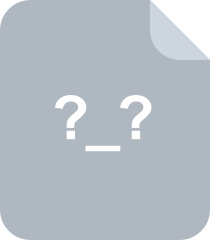
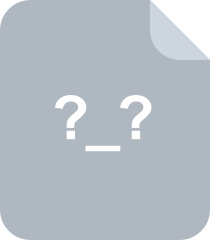
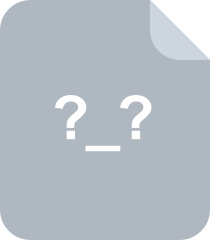
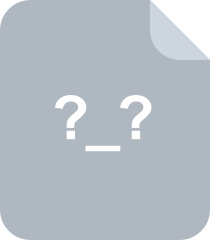
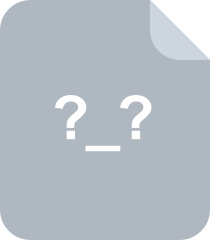
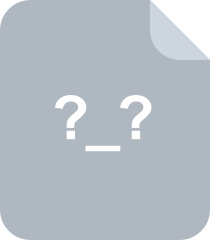
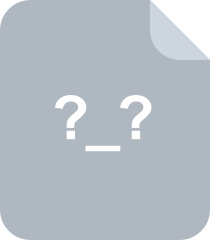
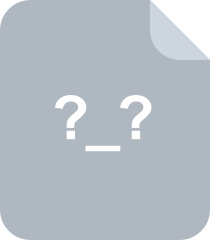
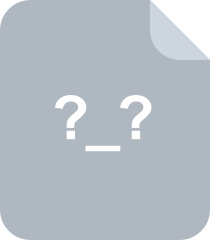
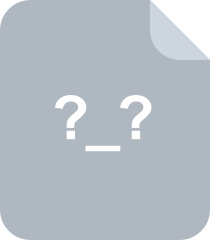
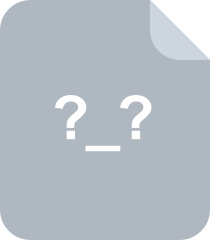
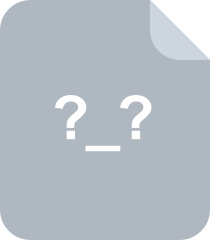
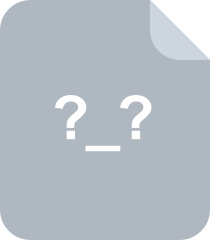
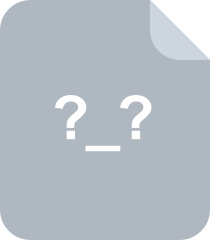
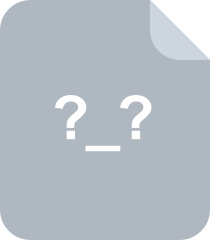
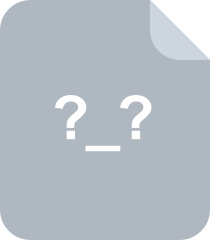
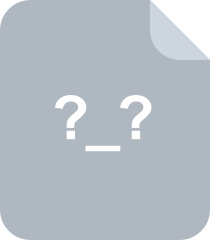
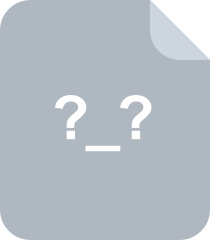
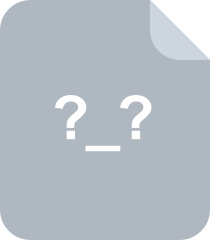
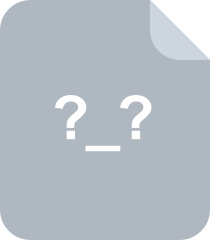
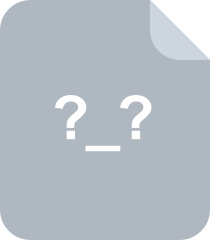
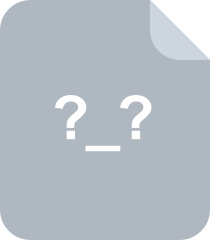
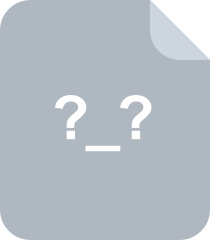
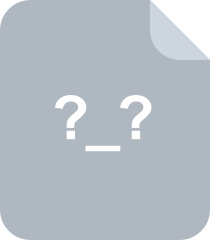
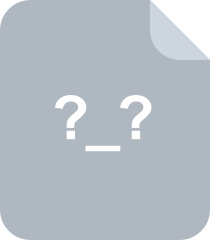
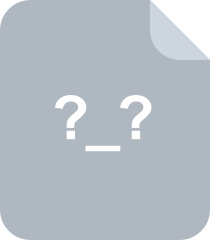
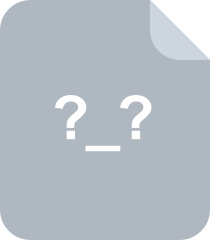
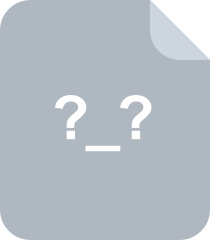
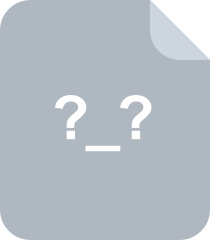
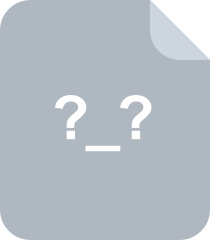
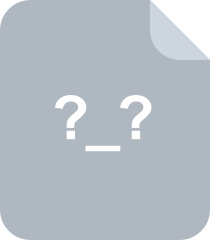
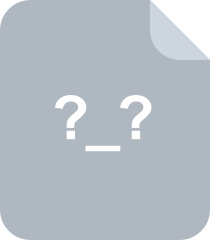
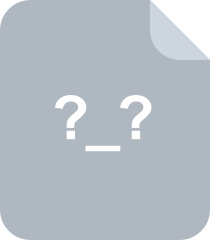
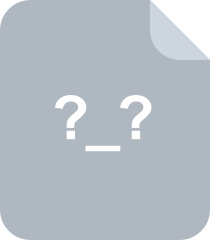
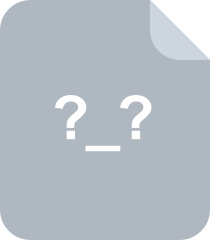
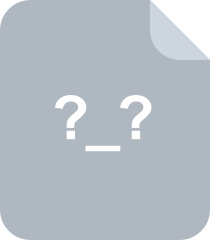
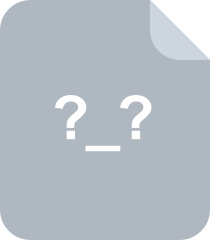
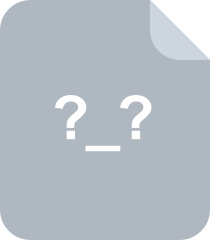
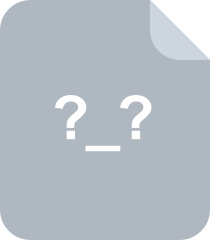
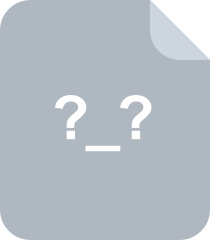
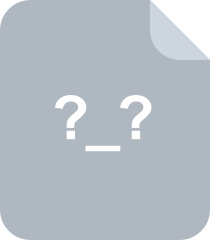
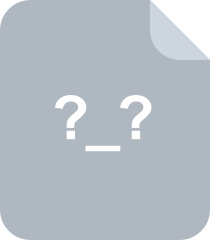
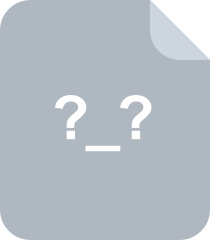
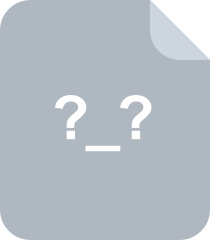
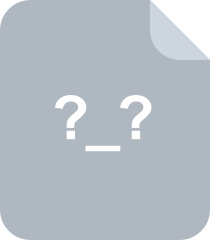
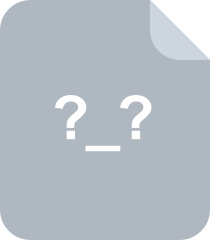
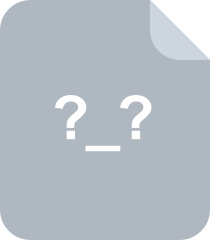
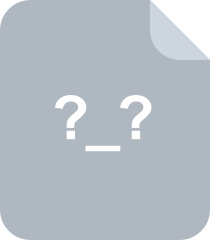
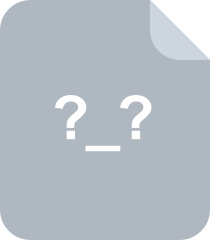
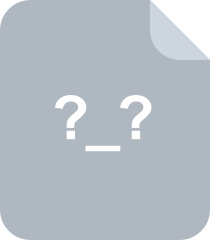
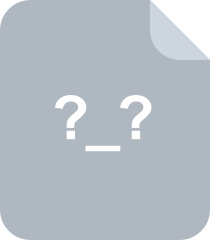
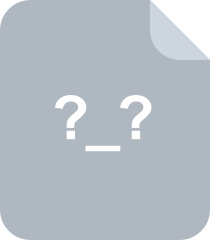
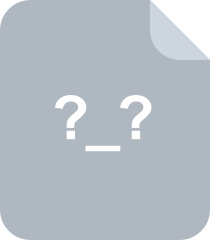
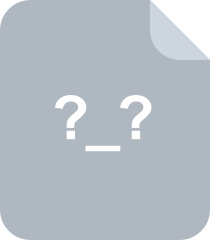
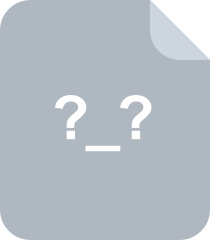
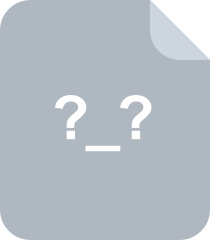
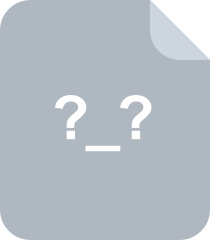
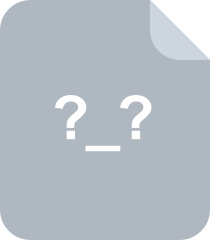
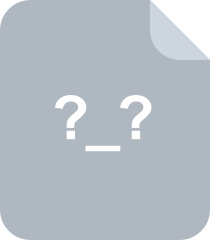
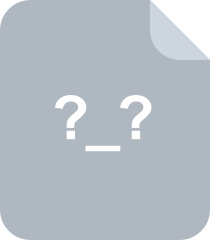
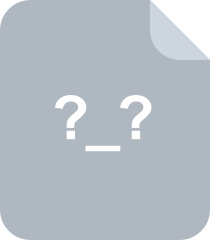
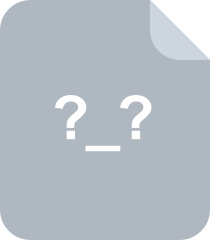
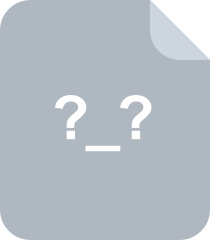
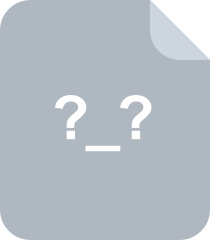
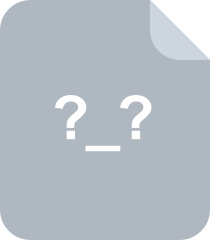
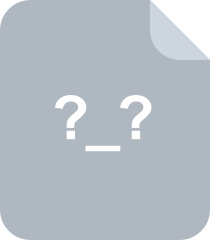
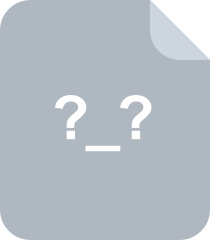
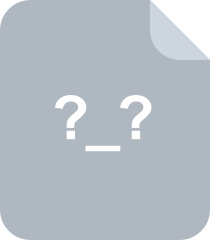
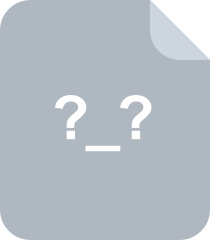
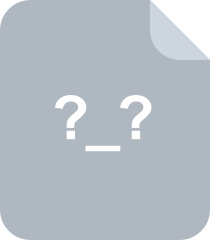
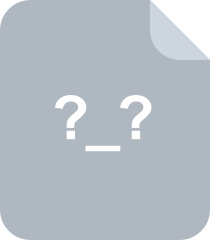
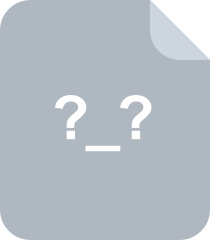
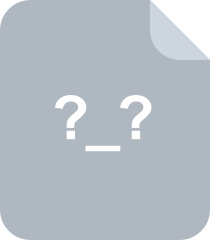
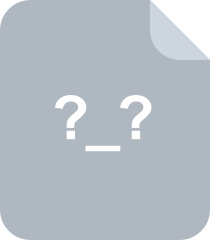
共 363 条
- 1
- 2
- 3
- 4
资源评论
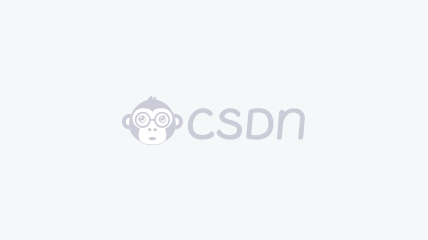
- 2301_823029932024-06-20资源内容详细,总结地很全面,与描述的内容一致,对我启发很大,学习了。
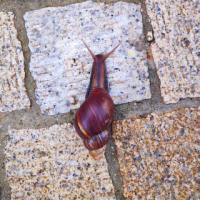

不脱发的程序猿
- 粉丝: 26w+
- 资源: 5817
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

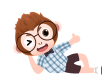
最新资源
- 海外AI应用落地进展梳理:AIGC商业化浪潮将至-多模态能力推动产业变革
- 40ab75cab55a4d9999c4cbd04a426894.mp4
- AIGC应用持续升级,国内大模型布局游戏教育等多元领域
- 基于Servlet+JSP实现毕业生招聘信息的发布与管理系统(论文+源代码+外文翻译)
- Linux独立开发项目-安防监控(基于正点原子I.MX6ULL-ALPHA开发板 )+C语言项目源码+文档说明
- JAVA 根据Word模板生成数据和Word转PDF相关的jar、还有相关的文件
- Helsinki-NLP/opus-100(en-zh)
- OrgsCertMaintenance V001 2024.11.8.rar
- 大模型算法迭代与AIGC产业发展前景探讨
- 2023年OpenAI多模态升级推动AIGC产业发展报告
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


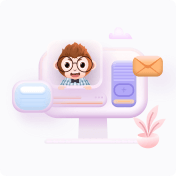
安全验证
文档复制为VIP权益,开通VIP直接复制
