package com.action;
import java.util.ArrayList;
import java.util.List;
import javax.annotation.Resource;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import com.entity.Article;
import com.entity.Cart;
import com.entity.Cate;
import com.entity.City;
import com.entity.Details;
import com.entity.Film;
import com.entity.Orders;
import com.entity.Topic;
import com.entity.Users;
import com.service.ArticleService;
import com.service.CartService;
import com.service.CateService;
import com.service.CinemaService;
import com.service.CityService;
import com.service.DetailsService;
import com.service.FilmService;
import com.service.OrdersService;
import com.service.TopicService;
import com.service.UsersService;
import com.util.VeDate;
//定义为控制器
@Controller
// 设置路径
@RequestMapping("/index")
public class IndexAction extends BaseAction {
@Autowired
@Resource
private UsersService usersService;
@Autowired
@Resource
private ArticleService articleService;
@Autowired
@Resource
private CateService cateService;
@Autowired
@Resource
private CityService cityService;
@Autowired
@Resource
private CinemaService cinemaService;
@Autowired
@Resource
private FilmService filmService;
@Autowired
@Resource
private CartService cartService;
@Autowired
@Resource
private OrdersService ordersService;
@Autowired
@Resource
private DetailsService detailsService;
@Autowired
@Resource
private TopicService topicService;
// 公共方法 提供公共查询数据
private void front() {
this.getRequest().setAttribute("title", "在线电影订票系统");
List<Cate> cateList = this.cateService.getAllCate();
this.getRequest().setAttribute("cateList", cateList);
List<Film> hotList = this.filmService.getFilmByHot();
this.getRequest().setAttribute("hotList", hotList);
}
// 首页显示
@RequestMapping("index.action")
public String index() {
this.front();
List<Cate> cateList = this.cateService.getCateFront();
List<Cate> frontList = new ArrayList<Cate>();
for (Cate cate : cateList) {
List<Film> flimList = this.filmService.getFilmByCate(cate.getCateid());
cate.setFlimList(flimList);
frontList.add(cate);
}
this.getRequest().setAttribute("frontList", frontList);
return "users/index";
}
// 公告
@RequestMapping("article.action")
public String article(String number) {
this.front();
List<Article> articleList = new ArrayList<Article>();
List<Article> tempList = this.articleService.getAllArticle();
int pageNumber = tempList.size();
int maxPage = pageNumber;
if (maxPage % 12 == 0) {
maxPage = maxPage / 12;
} else {
maxPage = maxPage / 12 + 1;
}
if (number == null) {
number = "0";
}
int start = Integer.parseInt(number) * 12;
int over = (Integer.parseInt(number) + 1) * 12;
int count = pageNumber - over;
if (count <= 0) {
over = pageNumber;
}
for (int i = start; i < over; i++) {
Article x = tempList.get(i);
articleList.add(x);
}
String html = "";
StringBuffer buffer = new StringBuffer();
buffer.append(" 共为");
buffer.append(maxPage);
buffer.append("页 共有");
buffer.append(pageNumber);
buffer.append("条 当前为第");
buffer.append((Integer.parseInt(number) + 1));
buffer.append("页 ");
if ((Integer.parseInt(number) + 1) == 1) {
buffer.append("首页");
} else {
buffer.append("<a href=\"index/article.action?number=0\">首页</a>");
}
buffer.append(" ");
if ((Integer.parseInt(number) + 1) == 1) {
buffer.append("上一页");
} else {
buffer.append("<a href=\"index/article.action?number=" + (Integer.parseInt(number) - 1) + "\">上一页</a>");
}
buffer.append(" ");
if (maxPage <= (Integer.parseInt(number) + 1)) {
buffer.append("下一页");
} else {
buffer.append("<a href=\"index/article.action?number=" + (Integer.parseInt(number) + 1) + "\">下一页</a>");
}
buffer.append(" ");
if (maxPage <= (Integer.parseInt(number) + 1)) {
buffer.append("尾页");
} else {
buffer.append("<a href=\"index/article.action?number=" + (maxPage - 1) + "\">尾页</a>");
}
html = buffer.toString();
this.getRequest().setAttribute("html", html);
this.getRequest().setAttribute("articleList", articleList);
return "users/article";
}
// 阅读公告
@RequestMapping("read.action")
public String read(String id) {
this.front();
Article article = this.articleService.getArticleById(id);
article.setHits("" + (Integer.parseInt(article.getHits()) + 1));
this.articleService.updateArticle(article);
this.getRequest().setAttribute("article", article);
return "users/read";
}
// 准备登录
@RequestMapping("preLogin.action")
public String prelogin() {
this.front();
return "users/login";
}
// 用户登录
@RequestMapping("login.action")
public String login() {
this.front();
String username = this.getRequest().getParameter("username");
String password = this.getRequest().getParameter("password");
Users u = new Users();
u.setUsername(username);
List<Users> usersList = this.usersService.getUsersByCond(u);
if (usersList.size() == 0) {
this.getSession().setAttribute("message", "用户名不存在");
return "redirect:/index/preLogin.action";
} else {
Users users = usersList.get(0);
if (password.equals(users.getPassword())) {
this.getSession().setAttribute("userid", users.getUsersid());
this.getSession().setAttribute("username", users.getUsername());
this.getSession().setAttribute("users", users);
return "redirect:/index/index.action";
} else {
this.getSession().setAttribute("message", "密码错误");
return "redirect:/index/preLogin.action";
}
}
}
// 准备注册
@RequestMapping("preReg.action")
public String preReg() {
this.front();
return "users/register";
}
// 用户注册
@RequestMapping("register.action")
public String register(Users users) {
this.front();
Users u = new Users();
u.setUsername(users.getUsername());
List<Users> usersList = this.usersService.getUsersByCond(u);
if (usersList.size() == 0) {
users.setRegdate(VeDate.getStringDateShort());
this.usersService.insertUsers(users);
} else {
this.getSession().setAttribute("message", "用户名已存在");
return "redirect:/index/preReg.action";
}
return "redirect:/index/preLogin.action";
}
// 退出登录
@RequestMapping("exit.action")
public String exit() {
this.front();
this.getSession().removeAttribute("userid");
this.getSession().removeAttribute("username");
this.getSession().removeAttribute("users");
return "index";
}
// 准备修改密码
@RequestMapping("prePwd.action")
public String prePwd() {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
return "users/editpwd";
}
// 修改密码
@RequestMapping("editpwd.action")
public String editpwd() {
this.front();
if (this.getSession().getAttribute("userid") == null) {
return "redirect:/index/preLogin.action";
}
String userid = (String) this.getSession().getAttribute("userid");
String password = this.getRequest().getParameter("password");
String repassword = this.getRequest().getParameter("repassword");
Users users = this.usersService.getUsersById(userid);
if (password.equals(users.getPassword())) {
users.setPassword(repassword);
this.usersService.updateUsers(users);
} else {
this.getSession().setAttribute("message", "旧密码错误");
return "redirect:/index/prePwd.action";
}
return "redirect:/index/preP
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
#### 介绍 本课题要求实现一套在线电影订票系统的开发与实现,主要实现功能包括: 前台:网站公告、推荐电影、全部电影、我的订单、购物车、个人中心, 管理员:管理员信息、网站用户信息、新闻公告信息、电影类型信息、城市信息、影院信息、电影信息、订单信息、电影评价信息等功能。 ## 技术框架 spring springmvc mybatis ## 开发技术 语言:java 服务器:tomcat 数据库:mysql ## 后台账号密码 admin admin ## 下载工具 idea(推荐) 或者 eclipse myeclipse 服务端:mysql,客户端 :navicate,sqlyog tomcat 8 jdk环境,
资源推荐
资源详情
资源评论
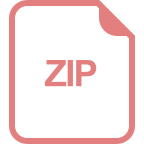
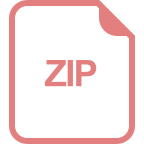
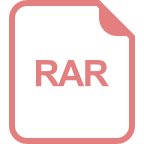
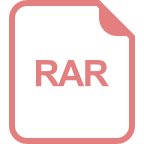
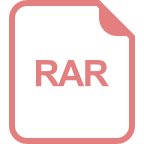
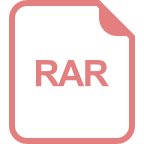
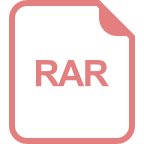
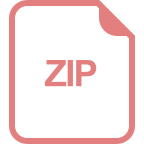
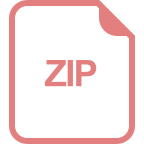
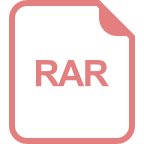
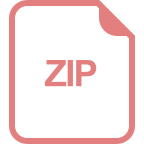
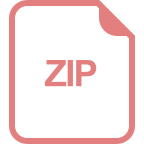
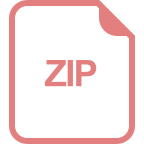
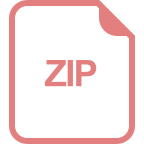
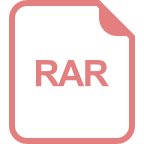
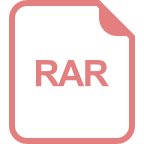
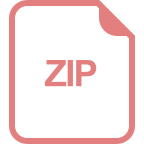
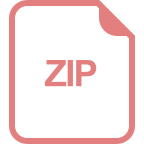
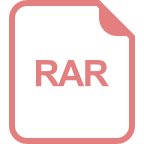
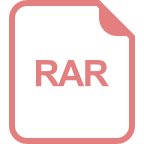
收起资源包目录

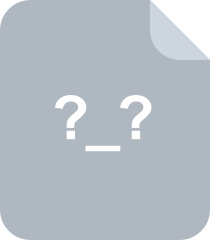
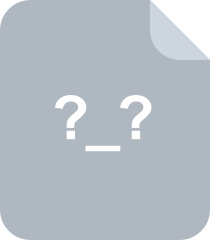
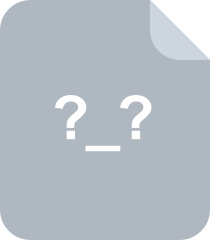
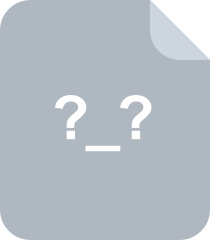
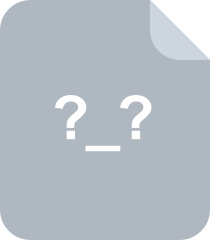
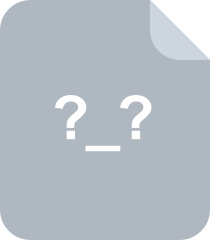
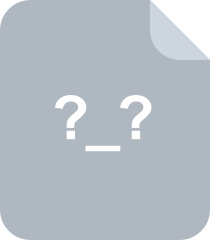
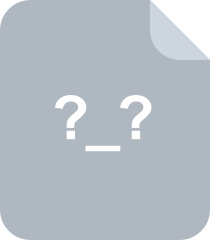
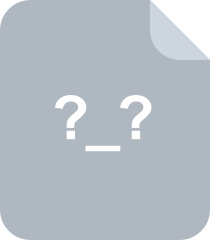
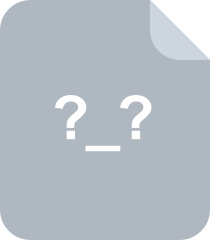
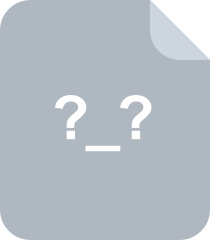
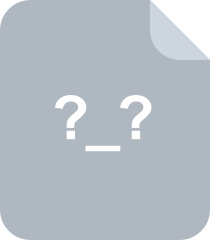
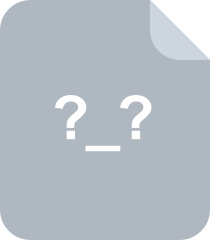
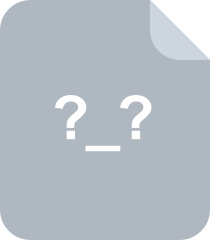
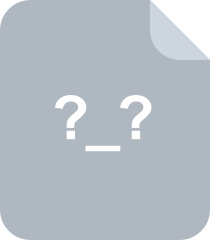
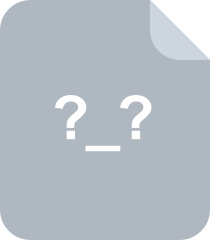
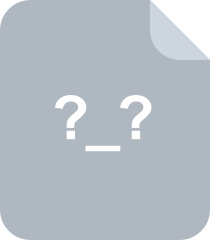
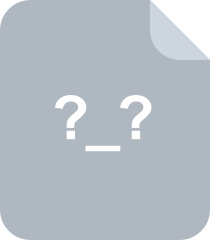
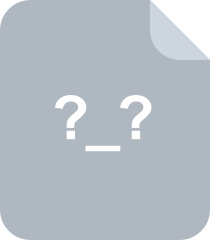
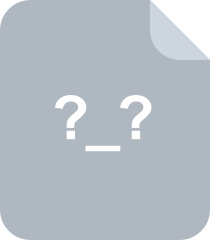
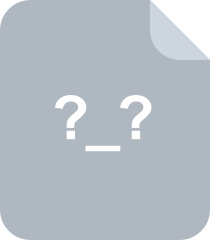
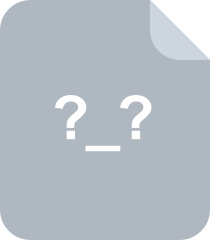
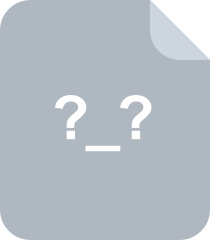
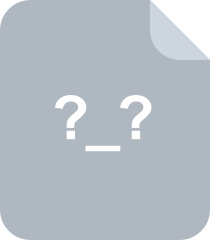
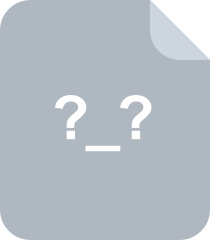
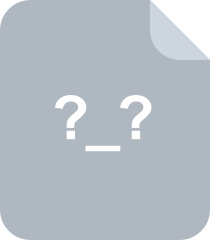
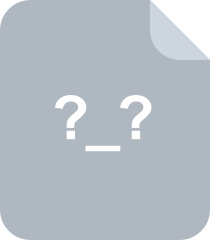
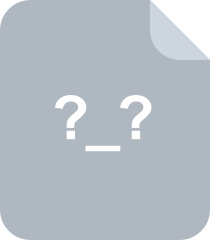
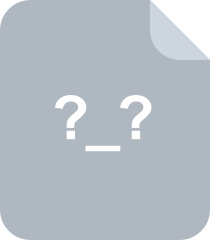
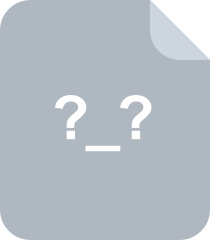
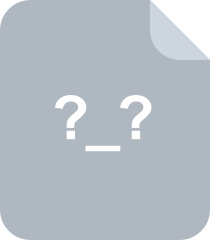
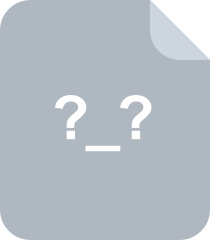
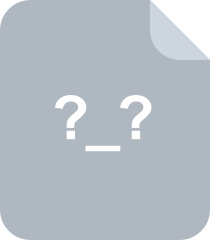
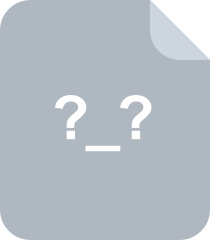
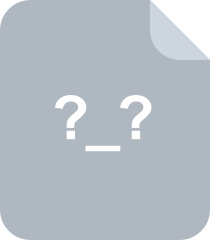
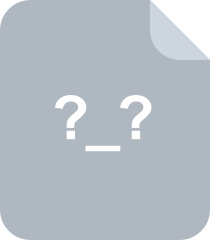
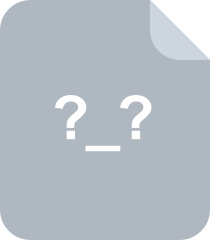
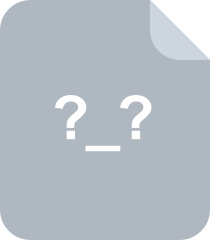
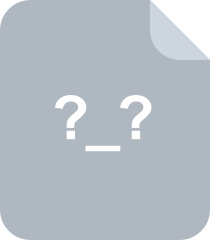
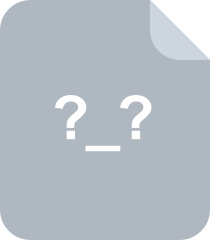
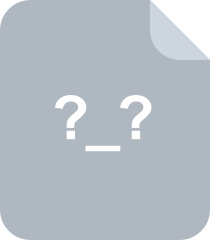
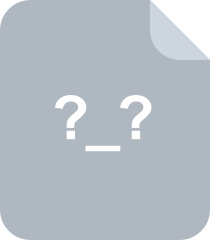
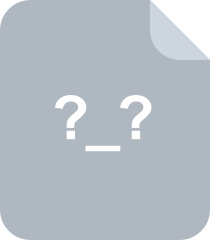
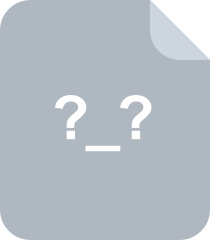
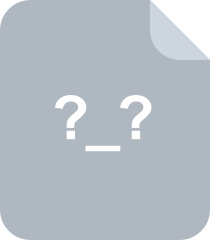
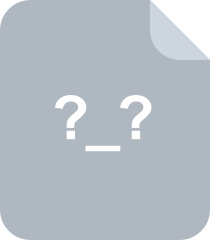
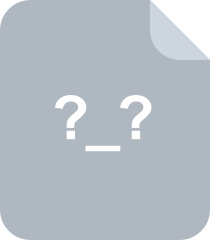
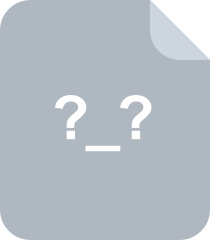
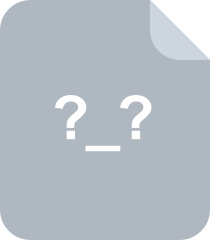
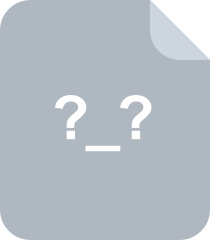
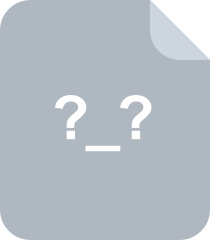
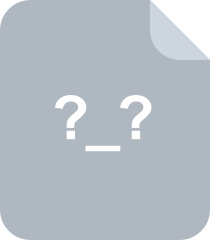
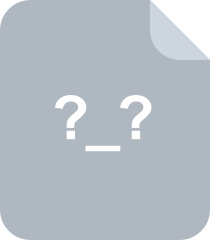
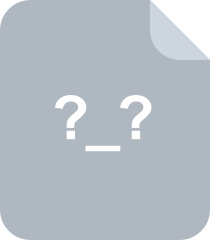
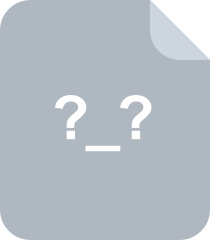
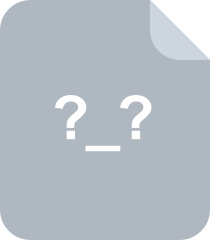
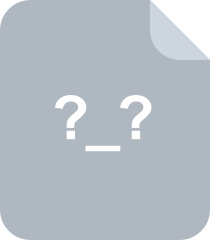
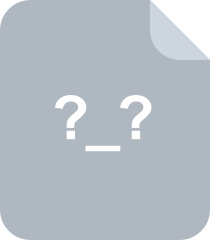
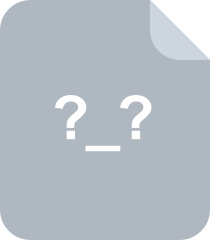
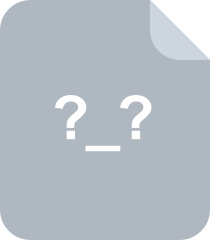
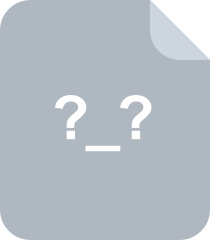
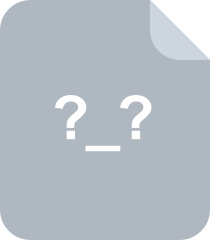
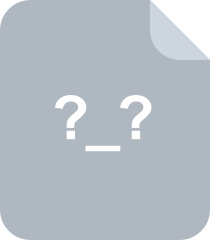
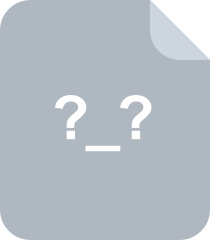
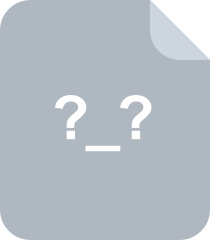
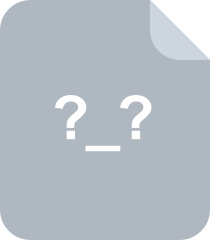
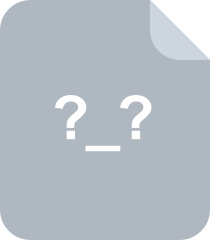
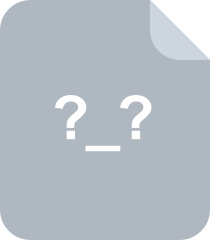
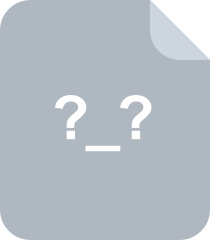
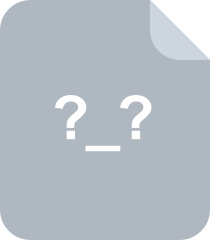
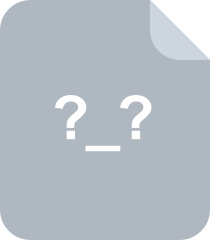
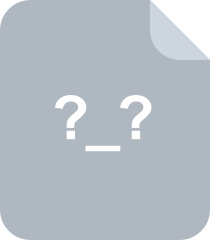
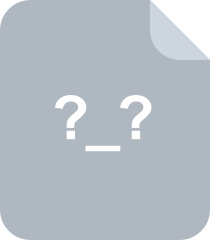
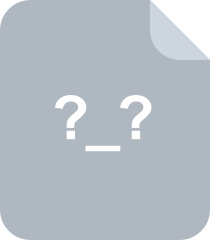
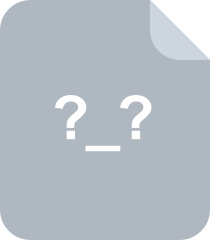
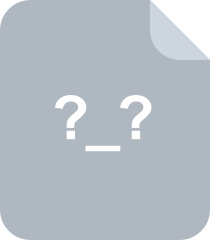
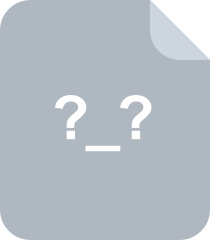
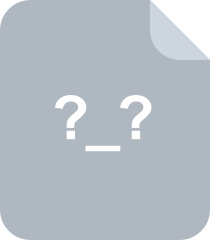
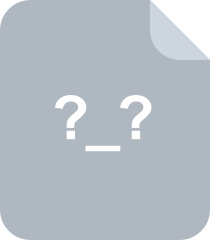
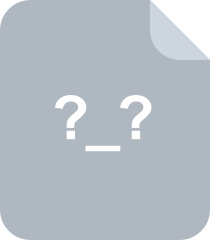
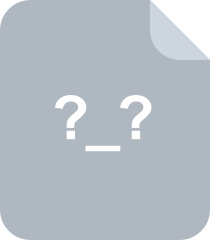
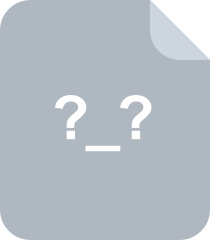
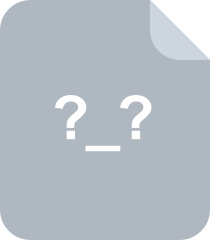
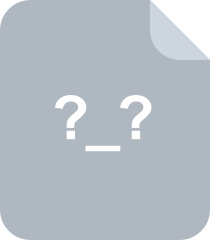
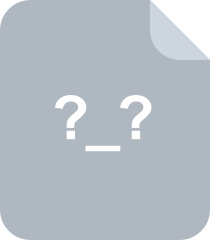
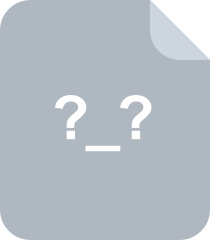
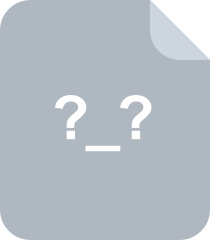
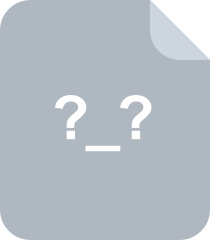
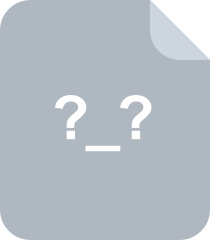
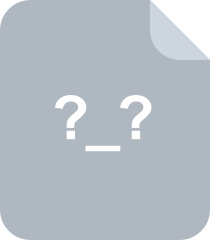
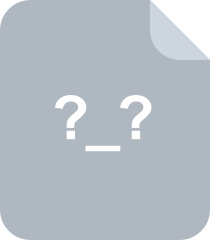
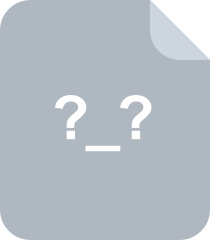
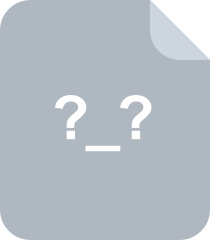
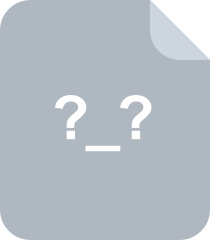
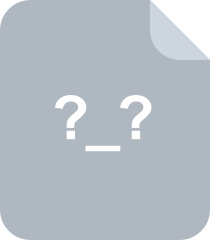
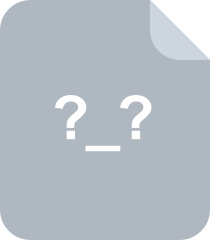
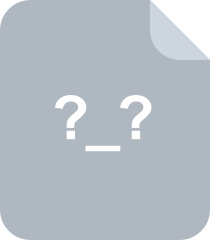
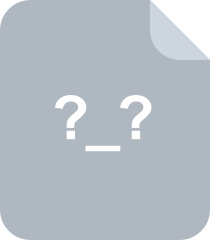
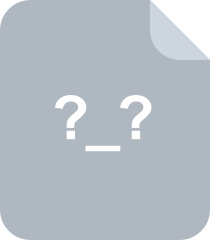
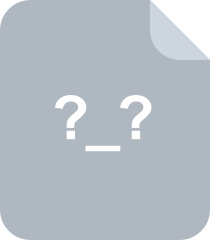
共 6155 条
- 1
- 2
- 3
- 4
- 5
- 6
- 62
资源评论
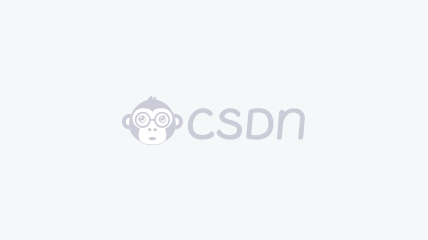

Java旅途
- 粉丝: 1w+
- 资源: 3050
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

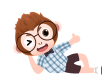
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


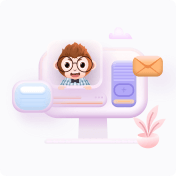
安全验证
文档复制为VIP权益,开通VIP直接复制
