#include<stdio.h>
#include<windows.h>
#include<math.h>
#include<time.h>
#define STB_IMAGE_IMPLEMENTATION
#include "img.h"
typedef struct _PT{
HDC dc1,dc2;
HBITMAP map;
int x,y,w,h,num,size;
DWORD*buf;
}PT;
void init(PT*pt,HANDLE win,int x,int y,int w,int h){
pt->dc1=GetDC(win);
pt->dc2=CreateCompatibleDC(pt->dc1);
pt->map=CreateCompatibleBitmap(pt->dc1,w,h);
pt->w=w;
pt->h=h;
pt->num=w*h;
pt->size=pt->num*4;
pt->buf=(DWORD*)malloc(pt->size);
pt->x=x;
pt->y=y;
SelectObject(pt->dc2,pt->map);
}
void setpixel(PT*pt,int x,int y,DWORD color){
if(x<0||x>=pt->w||y<0||y>=pt->h){
return;
}
int off=y*pt->w+x;
pt->buf[off]=color;
}
float mutD(float x1,float y1,float x2,float y2){
return x1*y2-y1*x2;
}
void ptfan(PT*pt,int x0,int y0,int w,int h,DWORD color){
int x1=x0+w;
int y1=y0+h;
int a0=max(0,x0);
int a1=min(pt->w,x1);
int b0=max(0,y0);
int b1=min(pt->h,y1);
for(int x=a0;x<a1;x++){
for(int y=b0;y<b1;y++){
int off=x+y*pt->w;
pt->buf[off]=color;
}
}
}
void ptimg0(PT*pt,int x0,int y0,DWORD*img,int w,int h,int isgl,DWORD glcolor){
int x1=x0+w;
int y1=y0+h;
int a0=max(0,x0);
int a1=min(pt->w,x1);
int b0=max(0,y0);
int b1=min(pt->h,y1);
for(int x=a0;x<a1;x++){
for(int y=b0;y<b1;y++){
int off=x+y*pt->w;
int off2=(x-a0)+(y-b0)*w;
DWORD color=img[off2];
if(!isgl||glcolor!=(color&0xffffff)){
pt->buf[off]=color;
}
}
}
}
typedef struct _IMG{
int w,h,isgl;
DWORD glcolor;
DWORD*buf;
}IMG;
void ptimg(PT*pt,int x0,int y0,IMG*img){
//printf("buf=%p\n",img->buf);
ptimg0(pt,x0,y0,img->buf,img->w,img->h,img->isgl,img->glcolor);
}
void clear_z(PT*pt){
memset(pt->buf,0,pt->size);
}
void clear(PT*pt,DWORD color){
for(int i=0;i<pt->num;i++){
pt->buf[i]=color;
}
}
void flush(PT*pt){
SetBitmapBits(pt->map,pt->size,pt->buf);
BitBlt(pt->dc1,pt->x,pt->y,pt->w,pt->h,pt->dc2,0,0,SRCCOPY);
}
DWORD*load_img0(char*fname,int*pw,int*ph){
int c;
DWORD*buf=(DWORD*)stbi_load(fname,pw,ph,&c,4);
if(buf){
int size=*pw**ph;
for(int i=0;i<size;i++){
BYTE arr[4];
*(DWORD*)&arr=buf[i];
BYTE t=arr[0];
arr[0]=arr[2];
arr[2]=t;
buf[i]=*(DWORD*)&arr;
}
}
return buf;
}
DWORD*load_img(IMG*img,char*fname,int gl,DWORD glcolor){
int w,h;
DWORD*buf=load_img0(fname,&w,&h);
img->buf=buf;
//printf("load buf=%p\n",img->buf);
img->w=w;
img->h=h;
img->isgl=gl;
img->glcolor=glcolor;
return buf;
}
void*new_img(char*fname,int gl,DWORD color){
IMG*img=(IMG*)malloc(sizeof(IMG));
load_img(img,fname,gl,color);
return img;
}
int main(){
PT pt;
init(&pt,0,0,0,800,700);
IMG fj;
load_img(&fj,"fj.jpg",1,0xffffff);
IMG dj;
load_img(&dj,"dj.jpg",1,0xffffff);
ptimg(&pt,0,0,&fj);
ptimg(&pt,200,0,&dj);
flush(&pt);
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
python 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源! 基于python和C语言开发的飞机大战(源码),开箱即用,非常好的资源!
资源推荐
资源详情
资源评论
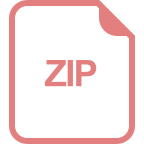
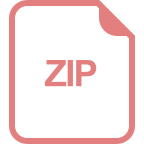
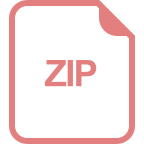
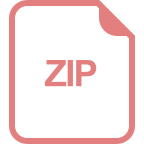
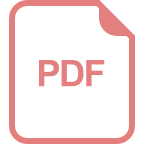
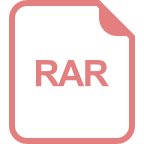
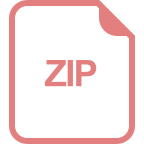
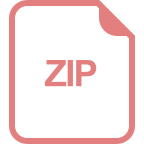
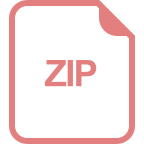
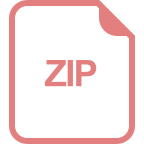
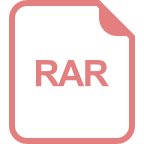
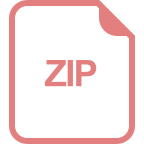
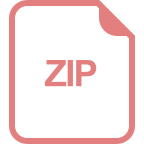
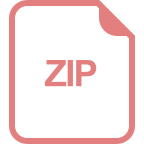
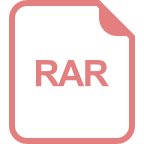
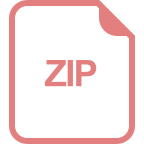
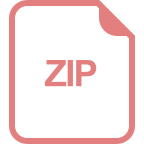
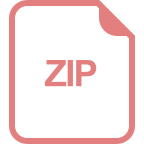
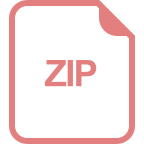
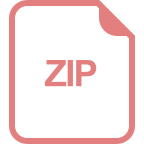
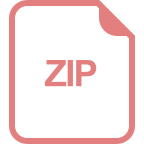
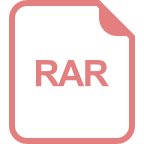
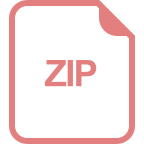
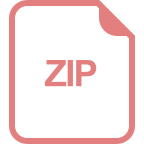
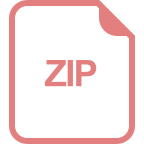
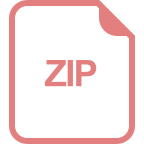
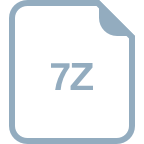
收起资源包目录


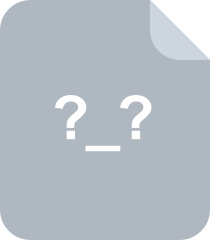
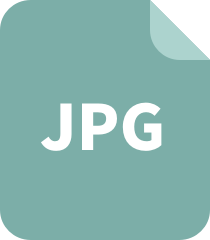
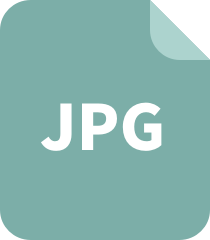
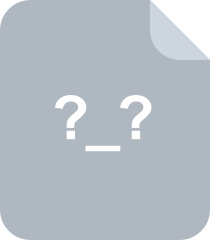
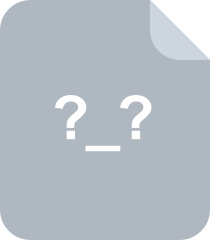
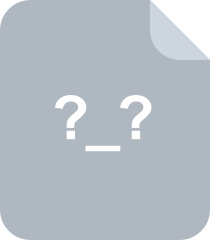
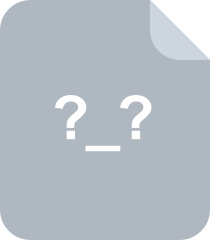
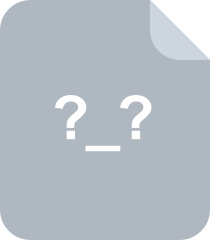
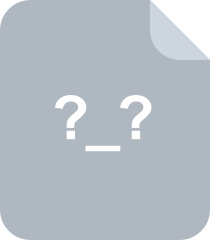
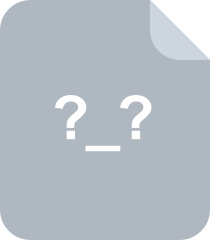
共 10 条
- 1
资源评论
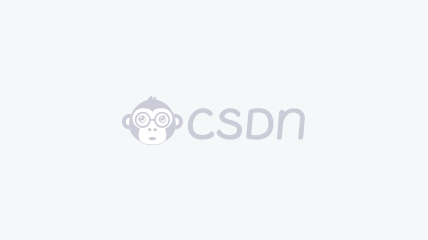

LeonDL168
- 粉丝: 2653
- 资源: 667
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

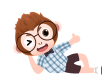
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


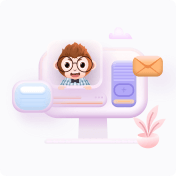
安全验证
文档复制为VIP权益,开通VIP直接复制
