package dao;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.apache.struts2.ServletActionContext;
import tip.Tip;
import user.User;
public class NewsDaoImp implements NewsDao {
String driver="org.hsqldb.jdbc.JDBCDriver";
String uri="jdbc:hsqldb:hsql://localhost:9001/";
String user="SA";
String pass="";
public List<String[]> getAllTips(String boardName) throws Exception {
String sql="select * from tip where boardName='"+boardName+"'";
List<String[]> tips=new ArrayList<>();
Class.forName(driver);
try(Connection con = DriverManager.getConnection(uri, user, pass);
PreparedStatement prstm = con.prepareStatement(sql);
ResultSet rs = prstm.executeQuery();){
while(rs.next()){
String[] r=new String[5];
r[0]=rs.getString("tipId");
r[1]=rs.getString("title");
r[2]=rs.getString("publishTime");
r[3]=rs.getString("userName");
r[4]=rs.getString("boardName");
tips.add(r);
}
}
return tips;
}
@Override
public ResultSet select(int id) {
String condition="SELECT * From board where boardId="+ id;
Statement st = null;
ResultSet rs = null;
try {
Class.forName(driver);
} catch (Exception e) {
e.printStackTrace();
}
try {
rs = st.executeQuery(condition);
} catch (SQLException e) {
e.printStackTrace();
}
return rs;
}
@Override
public boolean add(String title, String cont, String type) throws Exception {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd");
String date=df.format(new Date());
HttpServletRequest request=ServletActionContext.getRequest();
HttpSession session=request.getSession();
String userName=(String) session.getAttribute("name");
String condition ="INSERT INTO tip VALUES(?,?,?,?,?)";
int row=0;
Tip tip=null;
Class.forName(driver);
try (Connection con = DriverManager.getConnection(uri, user, pass);
PreparedStatement prstm = con.prepareStatement(condition);) {
tip=new Tip();
prstm.setString(1,title);
prstm.setString(2,cont);
prstm.setString(3,date);
prstm.setString(4,userName);
prstm.setString(5,type);
tip.setMessage("发布成功");
row=prstm.executeUpdate();
}catch(SQLException e){}
return row > 0 ? true : false;
}
@Override
public Tip show(int tipId) throws Exception {
String sql="select * from tip where tipId='"+tipId+"'";
Tip tips=null;
Class.forName(driver);
try (Connection con = DriverManager.getConnection(uri, user, pass);
PreparedStatement pstmt = con.prepareStatement(sql);
ResultSet rs = pstmt.executeQuery();) {
if(rs.next()){
tips=new Tip();
tips.setTipId(rs.getInt(1));
tips.setCont(rs.getString(3));
}
return tips;
}
}
@Override
public List<String[]> getTips(String key,int type) throws Exception {
HttpServletRequest request=ServletActionContext.getRequest();
HttpSession session=request.getSession();
String userName=(String) session.getAttribute("name");
List<String[]> tips=new ArrayList<>();
String condition=null;
if(type==1){
condition="select * from tip where title='"+key+"' and userName='"+userName+"'";
}
else if(type==2){
condition="select * from tip where userName='"+userName+"'";
}
Class.forName(driver);
try(Connection con = DriverManager.getConnection(uri, user, pass);
PreparedStatement prstm = con.prepareStatement(condition);
ResultSet rs = prstm.executeQuery();){
while(rs.next()){
String[] row=new String[5];
row[0]=rs.getString("tipId");
row[1]=rs.getString("title");
row[2]=rs.getString("publishTime");
row[3]=rs.getString("userName");
row[4]=rs.getString("boardName");
tips.add(row);
}
}
return tips;
}
@Override
public boolean update(Tip tip) throws Exception{
Class.forName(driver);
int id=tip.getTipId();
String condition="update tip set detail=? where tipId=?";
int row=0;
Tip tips=new Tip();
try (Connection con = DriverManager.getConnection(uri, user, pass);
PreparedStatement prstm= con.prepareStatement(condition);
) {
prstm.setString(1,tip.getCont());
prstm.setInt(2,id);
tip.setMessage("修改成功!");
row=prstm.executeUpdate();
return row > 0 ? true : false; }
}
@Override
public Tip query(int tipId) throws Exception {
Tip tip=null;
String condition="select * from tip where tipId='"+tipId+"'";
Class.forName(driver);
try(Connection con=DriverManager.getConnection(uri, user, pass);
Statement st = con.createStatement(ResultSet.TYPE_SCROLL_SENSITIVE,
ResultSet.CONCUR_READ_ONLY);){
ResultSet rs=st.executeQuery(condition);
if(rs.next()){
tip=new Tip();
tip.setTipId(tipId);
tip.setCont(rs.getString(3));
}
}
return tip;
}
@Override
public boolean delById(int tipId) throws Exception {
String sql = "delete from tip where tipId=?";
int row = 0;
Tip tip=new Tip();
Class.forName(driver);
try (Connection con = DriverManager.getConnection(uri, user, pass);
PreparedStatement pstmt = con.prepareStatement(sql);) {
pstmt.setInt(1,tipId);
row = pstmt.executeUpdate();
tip.setMessage("删除成功!");
}
return row > 0 ? true : false;
}
}

xili80
- 粉丝: 9
- 资源: 7
最新资源
- MATLAB 实现基于mRMR( 最大相关最小冗余)进行时间序列预测模型的项目详细实例(含完整的程序,GUI设计和代码详解)
- 球鞋交易平台系统源码+Java-HTML+球鞋交易-商品交易平台+毕设-课设-其他应用
- MATLAB 实现基于GAF(格拉姆角场)进行时间序列预测模型的项目详细实例(含完整的程序,GUI设计和代码详解)
- Matlab基于GWO-RBF灰狼算法优化径向基神经网络的分类预测的详细项目实例(含完整的程序,GUI设计和代码详解)
- MATLAB 实现基于KPCA(核主成分分析)进行时间序列预测模型的项目详细实例(含完整的程序,GUI设计和代码详解)
- Matlab实现VMD-CNN-GRU变分模态分解结合卷积神经网络门控循环单元多变量时间序列预测的详细项目实例(含完整的程序,GUI设计和代码详解)
- 使用Python Scrapy爬取淘宝和天猫2023-2024年度户外骑行自行车销售数据
- Matlab实现GCN基于图卷积神经网络的数据多特征分类预测的详细项目实例(含完整的程序,GUI设计和代码详解)
- 双滚筒升降式移栽机sw19全套技术资料100%好用.zip
- comsol计算非厄米系统中的PT BIC 在一维链中引入PT对称,普通的BIC劈裂为PT BIC和激光阈值模 包含能带,本征模式虚部,品质因子,场分布
- 鼠标键盘模拟软件本元填表精灵
- HTML5实现好看的面包烘焙品牌加盟网站源码.zip
- HTML5实现好看的美食自媒体博客主页模板.zip
- HTML5实现好看的米其林西餐厅网站源码.zip
- HTML5实现好看的木材建筑公司网站源码.zip
- HTML5实现好看的摩托车配件销售商城模板.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


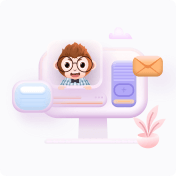