### Java Cook Book(英文原版) - 关键知识点解析 #### 一、书籍概述与适用对象 《Java Cook Book》是一本面向有一定基础的Java开发者的实用指南。它通过一系列问题与解决方案的形式来帮助读者解决实际开发过程中可能遇到的问题。本书不仅涵盖了Java的基础API,还涉及了一些其他书籍较少提及的API领域,如多媒体处理、Servlet等,从而拓宽了读者的技术视野。 #### 二、书籍结构与内容概览 ##### 2.1 编程环境搭建 - **1.2 编译与运行Java代码(JDK)**:介绍了如何使用JDK编译并运行Java程序的基本步骤。 - **1.3 使用带颜色高亮功能的编辑器进行编辑与编译**:推荐了几款常用的带有颜色高亮功能的文本编辑器,并讲解了如何利用这些工具更高效地编写与编译Java代码。 - **1.4 使用集成开发环境(IDE)进行编译、运行与测试**:探讨了使用IDE进行项目管理、代码编译及测试的优势,并列举了几款常用的Java IDE。 - **1.5 如何在自己的项目中使用本书中的类**:指导读者如何将书中的示例代码有效地整合到自己的项目中。 ##### 2.2 开发调试技巧 - **1.6 自动化编译**:介绍了几种实现Java代码自动化编译的方法,包括使用脚本语言以及构建工具如Ant。 - **1.7 运行Applet**:详细解释了如何在Web浏览器中运行Java Applet,并讨论了其在现代Web应用中的地位。 - **1.8 处理废弃警告**:提供了解决Java代码中废弃API使用所带来的警告信息的方法。 - **1.9 条件调试**:介绍了在不使用预处理器指令的情况下实现条件调试的方法。 - **1.10 调试输出**:探讨了如何通过打印语句来进行基本的调试操作。 - **1.11 使用调试器**:讲解了如何利用调试器工具对Java代码进行逐行调试。 - **1.12 单元测试**:强调了单元测试的重要性及其对于减少调试需求的作用。 - **1.13 反编译Java类文件**:讨论了如何反编译Java字节码文件,以及这一过程在逆向工程中的应用。 - **1.14 防止他人反编译Java文件**:提供了几种方法来增强Java代码的安全性,避免被轻易反编译。 - **1.15 获取可读的异常追踪信息**:阐述了如何让Java异常追踪信息更加清晰易懂。 - **1.16 寻找更多的Java源代码资源**:介绍了几个可以获取大量Java源代码的学习平台和网站。 ##### 2.3 与环境交互 - **2.2 获取环境变量**:介绍了如何在Java程序中获取操作系统环境变量的方法。 - **2.3 系统属性**:讨论了如何访问和设置Java虚拟机的系统属性。 - **2.4 编写依赖于JDK版本的代码**:提供了编写兼容不同JDK版本的代码的策略。 - **2.5 编写依赖于操作系统的代码**:探讨了如何根据不同的操作系统环境编写具有针对性的Java代码。 - **2.6 有效利用CLASSPATH**:讲解了如何正确配置CLASSPATH,以确保Java程序能够找到所需的类库文件。 - **2.7 使用扩展机制**:介绍了Java扩展机制的基本原理及其应用场景。 #### 三、结语 《Java Cook Book》为Java开发者提供了一个全面且实用的资源集合。无论是初学者还是经验丰富的程序员,都可以从中找到有价值的代码示例和技术建议。这本书不仅有助于解决具体的技术难题,还能帮助开发者拓展知识面,提高编程技能。《Java Cook Book》是每一位Java开发者不可或缺的参考书籍之一。
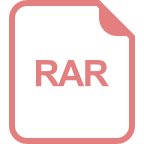
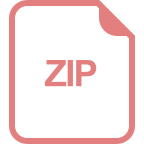
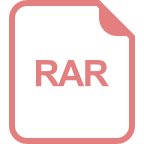
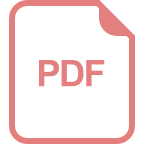
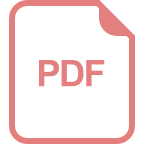
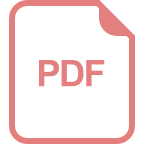
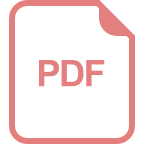
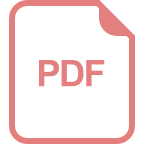
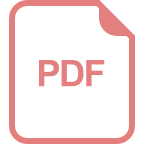
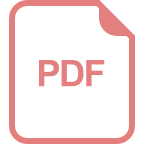
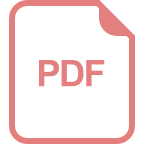
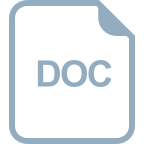
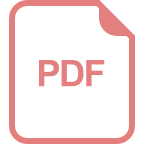
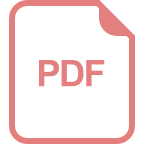
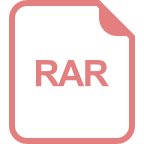
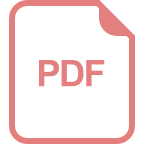
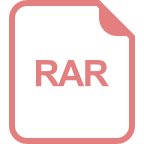
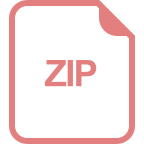
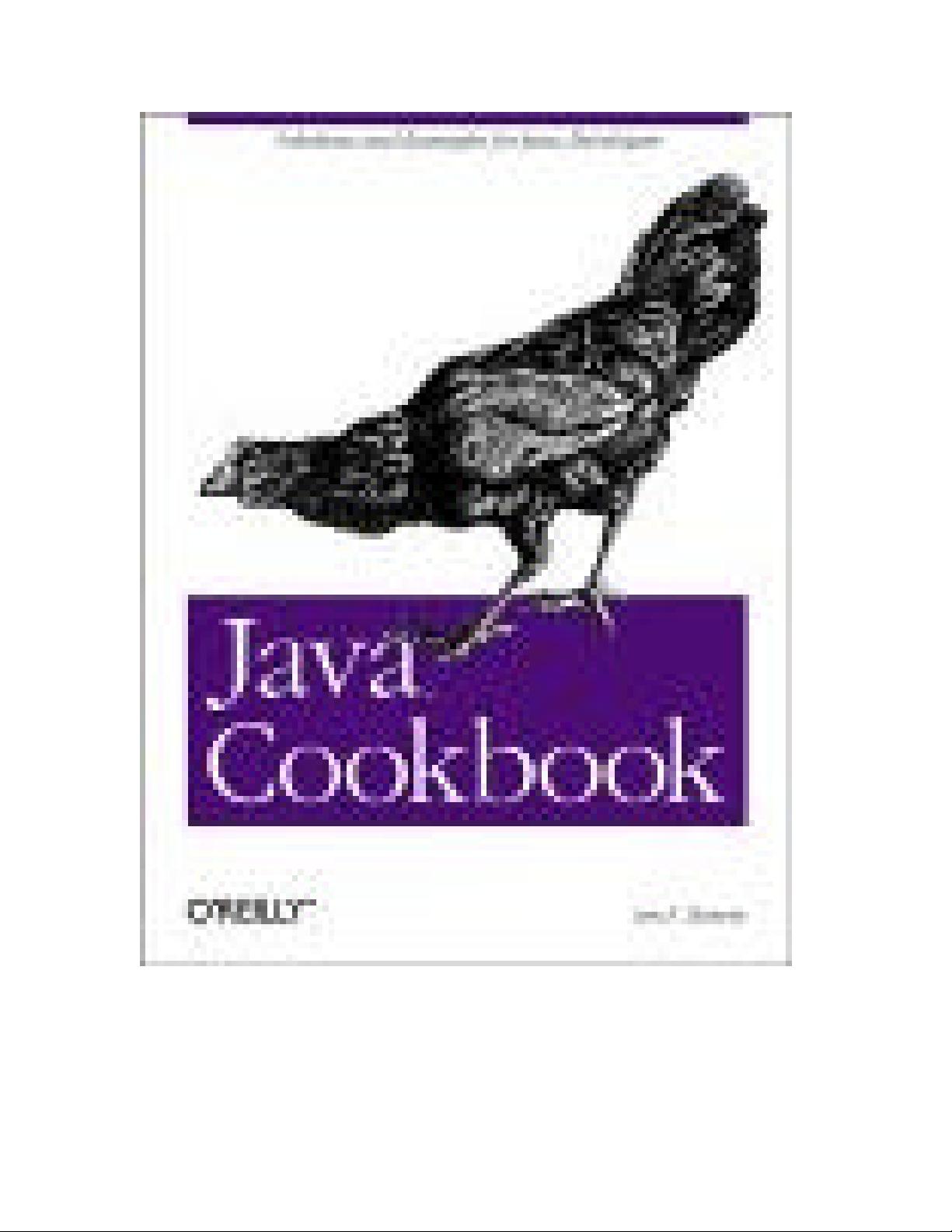
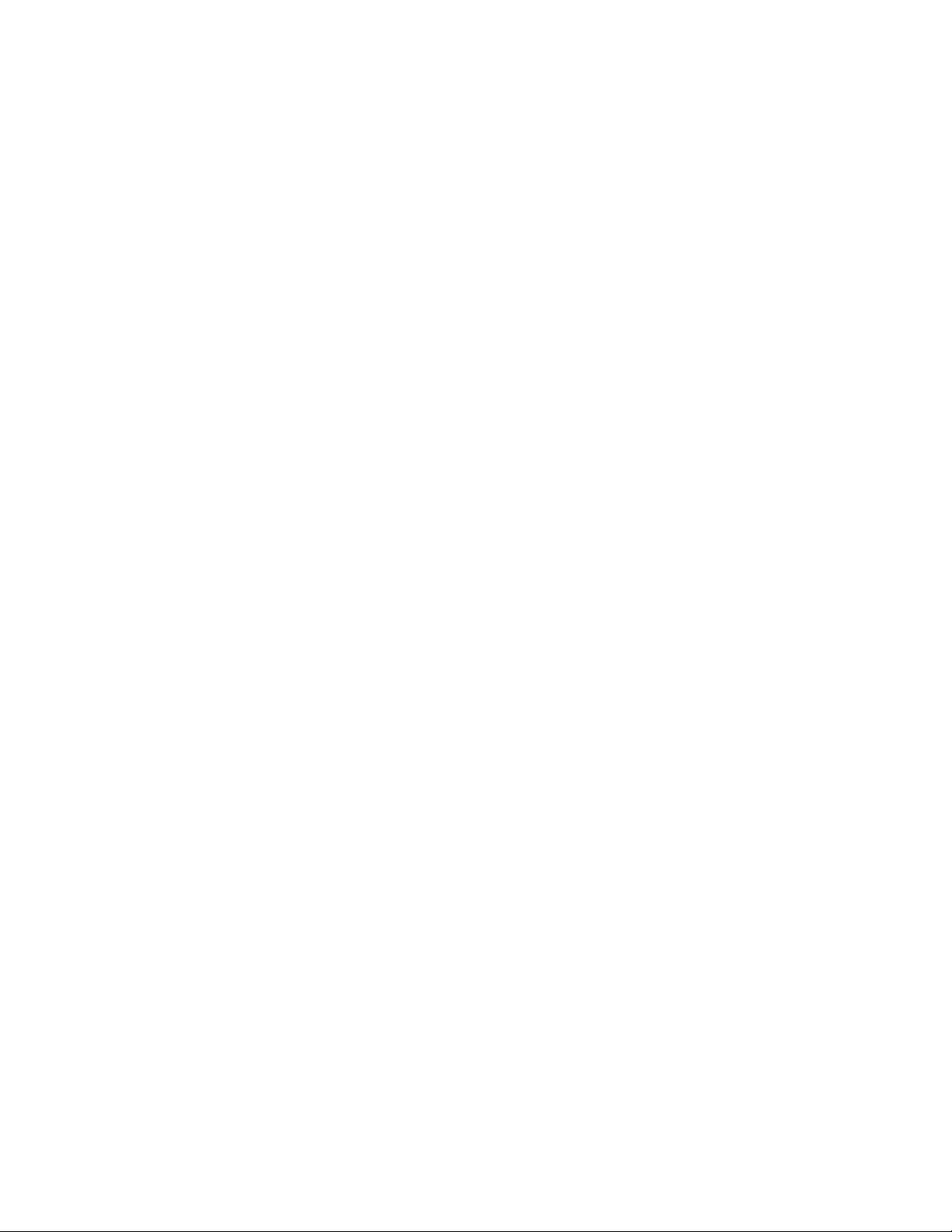
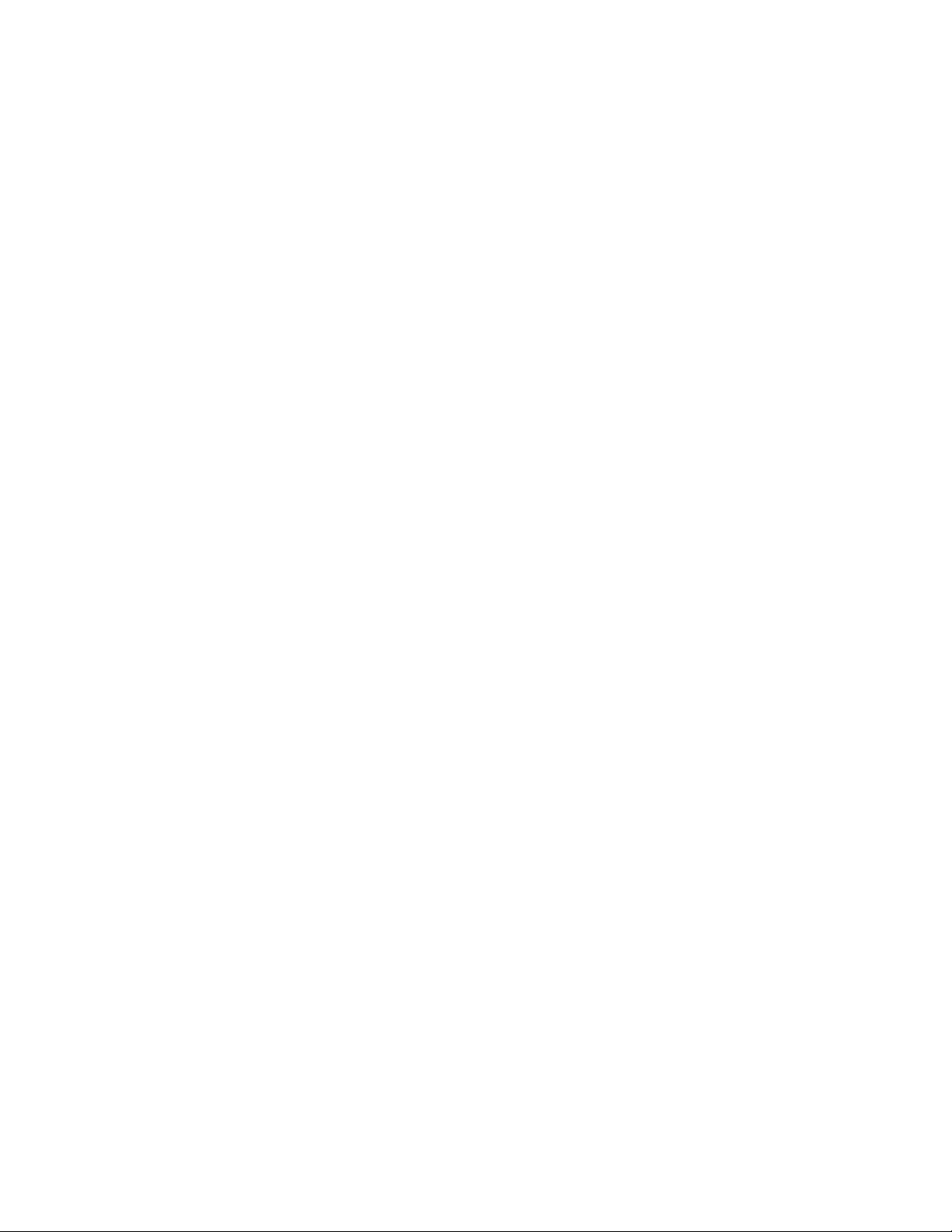
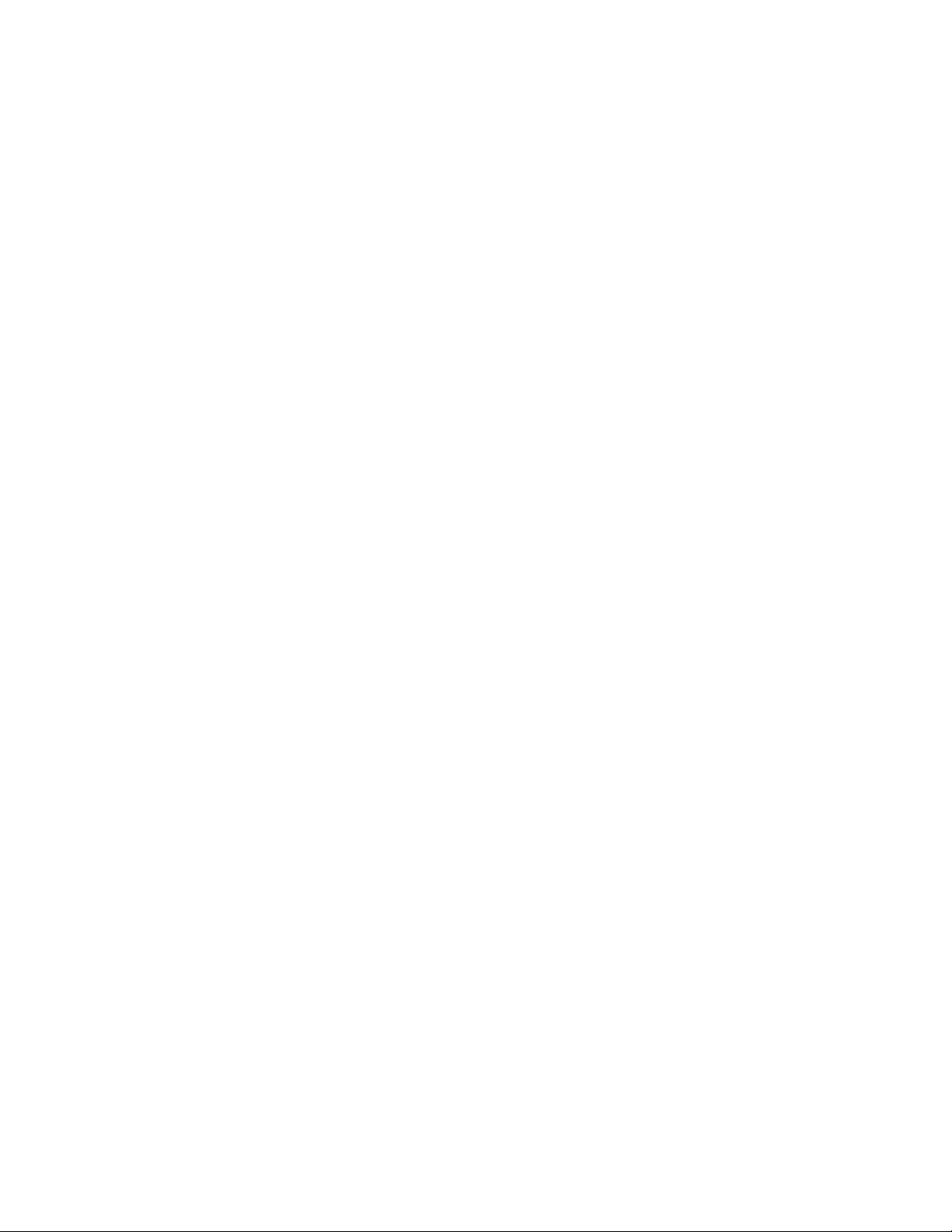
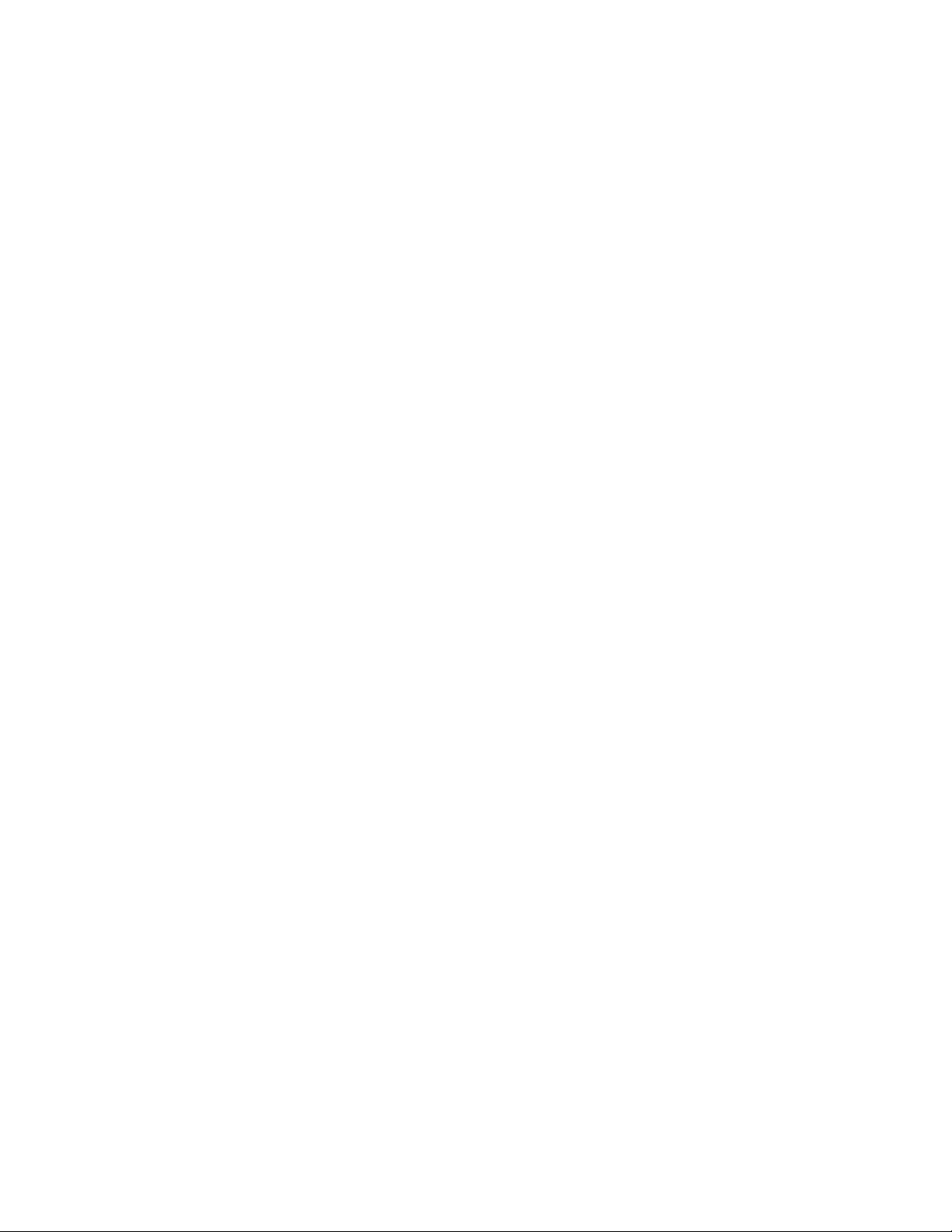
剩余727页未读,继续阅读
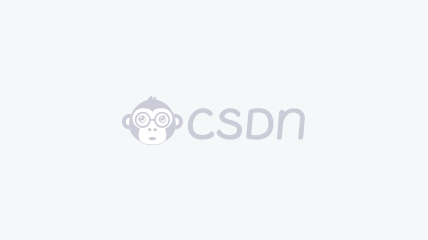

- 粉丝: 8
- 资源: 37
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

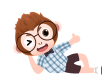
最新资源
- ccceeeeee,ukytkyk/liyihm
- 100kW微型燃气轮机Simulink建模,微燃机包括压缩机模块、容积模块、回热器模块、燃烧室模块、膨胀机模块、转子模块以及控制单元模块 考虑微燃机变工况特性下的流量、压缩绝热效率、膨胀绝热效率、压
- 该模型采用龙贝格观测器进行无传感器控制 其利用 PMSM 数学模型构造观测器模型,根据输出的偏差反馈信号来修正状态变量 当观测的电流实现与实际电流跟随时, 可以从观测的反电势计算得到电机的转子位置信
- 双移线驾驶员模型,多项式双移线模拟 软件使用:Matlab Simulink 适用场景:采用多项式搭建双移线期望路径,基于郭孔辉单点预瞄理论,搭建双移线simulink驾驶员模型 模型包含:双移线
- 0cd39e46e9672ca3fc70d6cb46f099dd_1734832088456_8
- 伺服系统永磁同步电机矢量控制调速系统在线转动惯量辨识Matlab仿真 1.模型简介 模型为永磁同步电机伺服控制仿真,采用Matlab R2018a Simulink搭建 模型内主要包含使
- newEditor.css
- 读QFLASH ID和读4线FLASH数据vitis验证工程
- 欧拉系统(openEuler-22.03-LTS-SP3) suricata rpm安装包
- ADRC自抗扰控制永磁同步电机矢量控制调速系统Matlab仿真模型 1.模型简介 模型为基于自抗扰控制(ADRC)的永磁同步电机矢量控制仿真,采用Matlab R2018a Simulink搭
- ADRC线性自抗扰控制感应电机矢量控制调速Matlab Simulink仿真 1.模型简介 模型为基于线性自抗扰控制(LADRC)的感应(异步)电机矢量控制仿真,采用Matlab R2018a
- 感应电机矢量控制调速仿真PI参数自整定 Matlab Simulink仿真模型 1.模型简介 模型为感应(异步)电机矢量控制调速系统仿真,采用Matlab R2018a Simulink搭建
- CC2530无线zigbee裸机代码实现ADC采集内部温度并串口打印.zip
- CC2530无线zigbee裸机代码实现LED流水灯程序.zip
- CC2530无线zigbee裸机代码实现MQ-2气体传感器数值读取.zip
- CC2530无线zigbee裸机代码实现PWM调光控制.zip

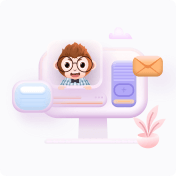
