在本项目中,我们探索的是一个使用Java编程语言编写的简单赛车小游戏,它特别适合Java初学者进行学习和实践。这个游戏的核心目标是提供一个基础的交互式环境,让玩家能够控制赛车在屏幕上移动,体验基本的游戏逻辑。让我们深入研究一下其中涉及到的关键知识点。 Java是这个项目的编程语言,它是一种广泛使用的面向对象的语言,以其“一次编写,到处运行”的特性而闻名。Java在游戏开发中应用广泛,尤其是在小型和中型游戏中,它提供了丰富的库和框架,如JavaFX和libGDX,使得开发更为便捷。 在这个赛车游戏中,开发者选择了Eclipse作为集成开发环境(IDE)。Eclipse是一个强大的开源IDE,支持多种编程语言,尤其是Java。WindowBuilder是Eclipse的一个插件,用于简化图形用户界面(GUI)的设计。通过WindowBuilder,开发者可以直接在GUI界面上拖放控件,而不是手动编写布局代码,这对于创建游戏界面非常有帮助。 游戏的核心机制之一是`Timer`类,这是Java Swing库的一部分。`Timer`类用于实现周期性的任务执行,例如在游戏中的帧率控制。通过设置定时器,开发者可以定期更新游戏状态,比如移动赛车、检查碰撞或渲染新帧。定时器与事件调度紧密相关,是构建实时应用和游戏的关键工具。 源码中可能包含了多个类,每个类代表游戏的不同组件,如赛车类(Car)、游戏面板类(GamePanel)和主窗口类(Main)。赛车类可能包含了赛车的位置、速度等属性,并实现了移动方法。游戏面板类则负责绘制游戏场景,处理用户输入并更新游戏状态。主窗口类则会创建并显示游戏界面,以及启动游戏循环。 在Java中,事件驱动编程是GUI应用程序的基础。赛车游戏可能会使用ActionListener或KeyListener来响应用户的键盘输入,控制赛车的移动。例如,当用户按下箭头键时,赛车类的移动方法会被调用,改变赛车的方向和速度。 为了实现游戏的视觉效果,开发者可能使用了Swing或JavaFX提供的绘图API,如`Graphics2D`,在游戏面板上绘制赛车和其他元素。这包括背景、赛道、赛车本身以及可能的障碍物。 这个Java赛车小游戏为初学者提供了一个很好的学习平台,涵盖了Java基础、GUI设计、事件处理和定时器的使用。通过分析和修改这个游戏的源码,初学者可以深入理解Java编程的许多核心概念,并逐步提升自己的编程技巧。
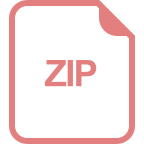
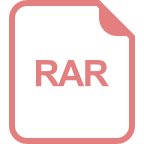
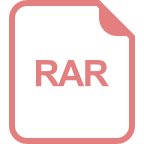
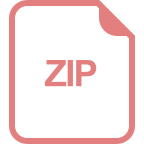
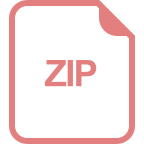
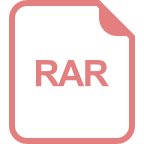
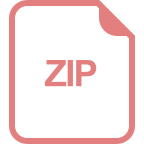
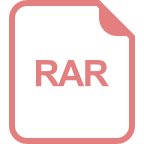
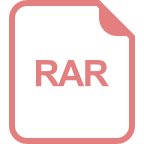
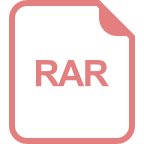
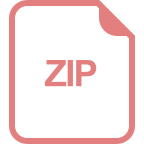
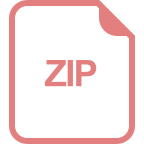
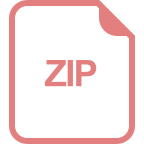


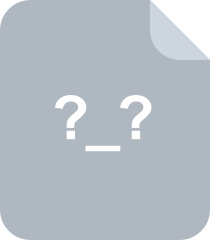


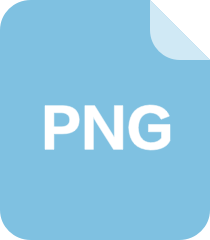
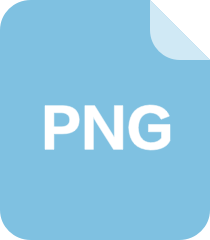
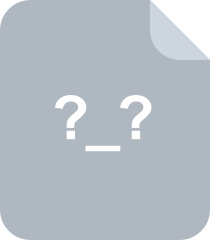
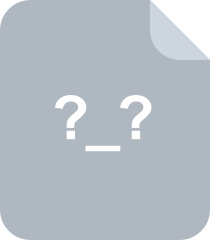
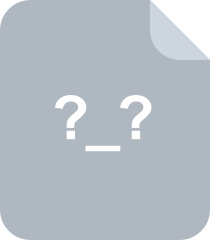

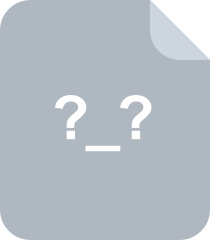
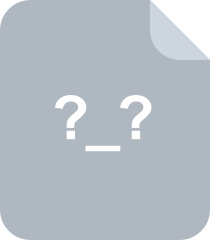

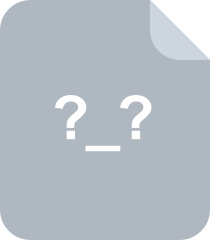
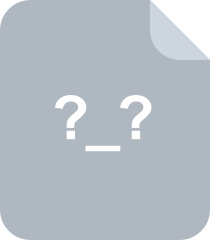

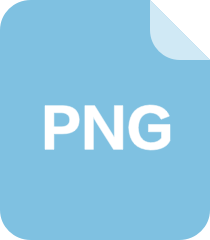
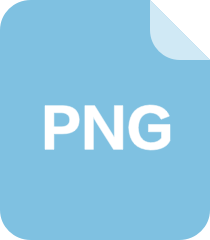
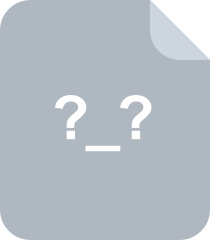
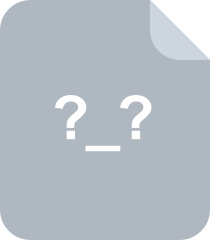
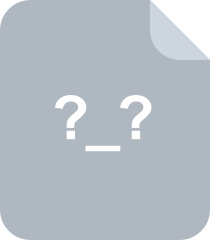
- 1
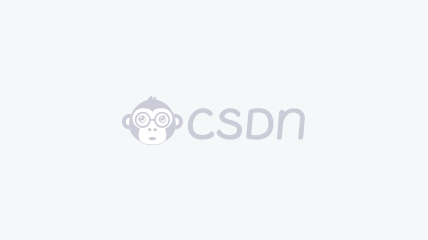
- 大笨熊BigBear2015-05-25感觉一般,可能是自己没有导对包

- 粉丝: 2
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

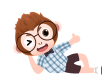
最新资源
- 基于Selenium页面爬取某东商品价格监控:自定义商品价格,降价邮件微信提醒资料齐全+详细文档+源码.zip
- 基于selenium爬取通过搜索关键词采用指定页数的商品信息资料齐全+详细文档+源码.zip
- 基于今日头条自动发文机器人,各大公众平台采集爬虫资料齐全+详细文档+源码.zip
- 基于集众多数据源于一身的爬虫工具箱,旨在安全快捷的帮助用户拿回自己的数据,工具代码开源,流程透明、资料齐全+详细文档+源码.zip
- 基于拼多多爬虫,爬取所有商品、评论等信息资料齐全+详细文档+源码.zip
- 基于爬虫从入门到入狱资料齐全+详细文档+源码.zip
- 基于爬虫学习仓库,适合零基础的人学习,对新手比较友好资料齐全+详细文档+源码.zip
- 基于天眼查爬虫资料齐全+详细文档+源码.zip
- 基于千万级图片爬虫、视频爬虫资料齐全+详细文档+源码.zip
- 基于支付宝账单爬虫资料齐全+详细文档+源码.zip
- 基于SpringBoot+Vue3实现的在线考试系统(三)代码
- 数组-.docx cccccccccccccccccccccc
- Ruby技巧中文最新版本
- Ruby袖珍参考手册pdf英文文字版最新版本
- 融合导航项目全套技术资料100%好用.zip
- 四足机器人技术进展与应用场景

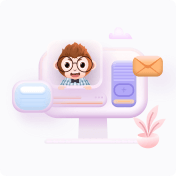
