<?xml version="1.0" encoding="utf-8"?>
<doc>
<assembly>
<name>Devart.Data.Oracle</name>
<version>7.0.27.0</version>
<fullname>Devart.Data.Oracle, Version=7.0.27.0, Culture=neutral, PublicKeyToken=213e03692046d0a5</fullname>
</assembly>
<members>
<member name="N:Devart.Common">
<summary>The <see cref="N:Devart.Common" /> namespace contains classes shared by the Devart data providers. </summary>
</member>
<member name="T:Devart.Common.DbConnectionBase">
<summary>Represents an open connection to a server. </summary>
<remarks>The abstract <see cref="T:Devart.Common.DbConnectionBase" /> class implements some of <see cref="T:Devart.Common.DbConnection" /> functionality that is DBMS-independent. </remarks>
<example>The following sample demonstrates how using base classes helps to create database independent code. <code lang="csharp">public void PrintDept(DbConnectionBase myConnection) {
DbCommandBase myCommand = (DbCommandBase)myConnection.CreateCommand();
myCommand.CommandText = "SELECT * FROM Test.Dept";
myConnection.Open();
DbDataReader myReader = myCommand.ExecuteReader();
try {
while (myReader.Read()) {
Console.WriteLine(myReader.GetInt32(0) + " " +
myReader.GetString(1) + " " + myReader.GetString(2));
}
}
finally {
myReader.Close();
myConnection.Close();
}
} </code><code lang="vb">Public Sub PrintDept(ByVal myConnection As DbConnectionBase)
Dim myCommand As DbCommandBase = myConnection.CreateCommand()
myCommand.CommandText = "SELECT * FROM Test.Dept"
myConnection.Open()
Dim myReader As DbDataReader = myCommand.ExecuteReader()
Try
While myReader.Read()
Console.WriteLine(String.Concat(myReader.GetInt32(0), " ", _
myReader.GetString(1), " ", myReader.GetString(2)))
End While
Finally
myReader.Close()
myConnection.Close()
End Try
End Sub </code></example>
<seealso cref="T:Devart.Common.DbConnection" />
<seealso cref="T:Devart.Data.Oracle.OracleConnection" />
</member>
<member name="M:Devart.Common.DbConnectionBase.BeginOpen(System.AsyncCallback,System.Object)">
<summary>Starts an asynchronous invocation of an <see cref="M:Devart.Common.DbConnectionBase.Open" /> method. </summary>
<remarks>
<para>
<see cref="M:Devart.Common.DbConnectionBase.BeginOpen" /> method enables you to open a connection to server without having current thread blocked. In other words, your program can continue execution while the connection is establishing so you do not have to wait for it.</para>
<para>To start opening a connection, you have to call <see cref="M:Devart.Common.DbConnectionBase.BeginOpen" /> method, which in turn invokes appropriate actions in another thread. Return value of this method must be assigned to <see cref="T:System.IAsyncResult" /> object. After executing this method program flow continues.</para>
<para>When you are ready to use the connection, call <see cref="M:Devart.Common.DbConnectionBase.EndOpen" />. If at the moment you call this method the negotiation process has not yet been finished, application stops and waits till the function returns which means that the connection is open.</para>
<para>Refer to <a href="Asynchronous.html">"Asynchronous Query Execution"</a> article for detailed information.</para> </remarks>
<example>This sample shows how to implement asynchronous opening of a connection. <code lang="csharp">public void AsyncOpen(DbConnectionBase myConnection)
{
IAsyncResult myAsyncResult = myConnection.BeginOpen(null, null);
DbCommandBase myCommand = (DbCommandBase)myConnection.CreateCommand();
myCommand.CommandText = "INSERT INTO Test.Dept(DeptNo, DName) Values('100', 'DEVELOPMENT')";
//some operations here
myConnection.EndOpen(myAsyncResult);
try
{
myCommand.ExecuteNonQuery();
}
finally
{
myConnection.Close();
}
} </code><code lang="vb">Public Sub AsyncOpen(ByVal myConnection As DbConnectionBase)
Dim myAsyncResult As IAsyncResult = myConnection.BeginOpen(Nothing, Nothing)
Dim myCommand As DbCommandBase = myConnection.CreateCommand()
myCommand.CommandText = "INSERT INTO Test.Dept(DeptNo, DName) Values('110', 'QA')"
' some operations here
myConnection.EndOpen(myAsyncResult)
Try
myCommand.ExecuteNonQuery()
Finally
myConnection.Close()
End Try
End Sub </code></example>
<keywords>DbConnectionBase.BeginOpen method </keywords>
<param name="callback">The delegate to call when the asynchronous invoke is complete. If callback is a null reference (Nothing in Visual Basic), the delegate is not called. </param>
<param name="stateObject">State information that is passed on to the delegate. </param>
<returns>An <see cref="T:System.IAsyncResult" /> interface that represents the asynchronous operation started by calling this method. </returns>
</member>
<member name="M:Devart.Common.DbConnectionBase.ChangeDatabase(System.String)">
<summary>Changes the current database associated with an open <see cref="T:Devart.Common.DbConnectionBase" />. </summary>
<remarks>This method is not used in dotConnect for Oracle. </remarks>
<keywords>DbConnectionBase.ChangeDatabase method </keywords>
<param name="value">The database name. </param>
</member>
<member name="M:Devart.Common.DbConnectionBase.Close()">
<summary>Closes the connection to the database. This is the preferred method of closing any open connection. </summary>
<remarks>The <see cref="M:Devart.Common.DbConnectionBase.Close()" /> method rolls back any pending transactions. It then releases the connection to the connection pool, or closes the connection if connection pooling is disabled.
<para>Note that you must manually close all connections opened by the application. If a <see cref="T:Devart.Common.DbConnectionBase" /> goes out of scope, it is not closed automatically till the application shuts down.</para><para>An application can call <see cref="M:Devart.Common.DbConnectionBase.Close()" /> more than one time without generating an exception.</para> </remarks>
<keywords>DbConnectionBase.Close method </keywords>
</member>
<member name="P:Devart.Common.DbConnectionBase.ConnectionString">
<summary>Gets or sets the string used to open a server connection. </summary>
<remarks>
<para>When <see cref="P:Devart.Common.DbConnectionBase.ConnectionString" /> property is assigned a value, connection closes. The connection string is parsed immediately after being set. If errors in syntax are found when parsing, a runtime exception, such as <see cref="T:System.ArgumentException" />, is generated. Other errors can be found only when an attempt is made to <see cref="M:Devart.Common.DbConnectionBase.Open()" /> the connection.</para>
<para>The <see cref="P:Devart.Common.DbConnectionBase.ConnectionString" /> is similar to an OLE DB connection string. Values may be delimited by single or double quotes, (for example, name='value' or name="value"). Either single or double quotes may be used within a connection string by using the other delimiter, for example, name="value's" or name= 'value"s', but not name= 'value's' or name= ""value"". All blank characters, except those placed within a value or within quotes, are ignored. Keyword value pairs must be separated by a semicolon (;). If a semicolon is part of a value, it also must be delimited by quotes. No escape sequences are supported. The value type is irrelevant. Names are not case sensitive.</para>
<para>If a given name occurs more than once in the connection string, the value associated with the last occurrence is used.</para> </remarks>
<keywords>DbConnectionBase.ConnectionString property </keywords>
<value>The connection string that includes the source database name, and other parameters needed to establish
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
我见过最好的Oracle数据库连接组件,性能和效率甚至比官方的高,最爽的是它不需要安装Oracle客户端即可连接数据库。赶快破解了献给大家,希望大家喜欢。使用方法:一、不用安装,直接使用破解文件夹中的组件,使用提供的CHM帮助。二、安装原版,使用VS帮助。第二种方法要做处理,1替换安装文件目录文件。2删除官方安装到GAC中的配件。3注册破解文件夹中的组件到GAC。原因是破解后强名称做了替换,不做处理的话调用的还是原版。
资源推荐
资源详情
资源评论
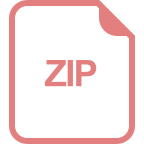
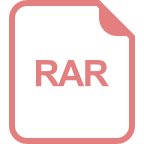
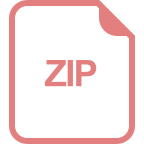
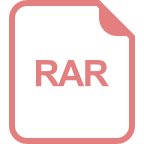
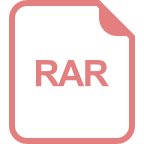
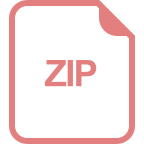
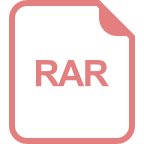
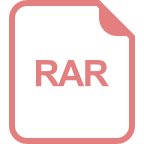
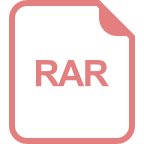
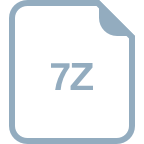
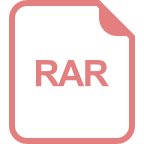
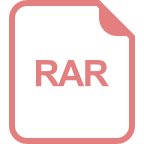
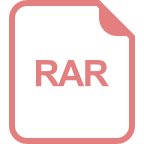
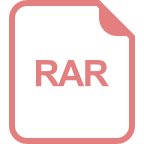
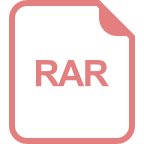
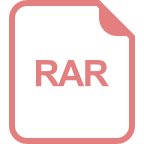
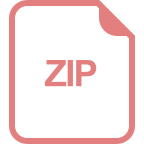
收起资源包目录




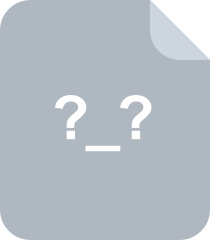

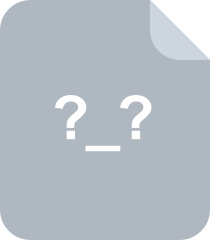
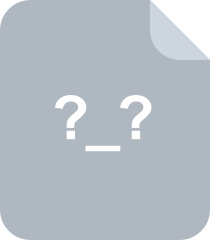
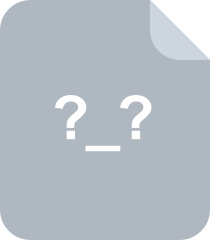
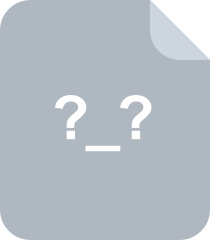

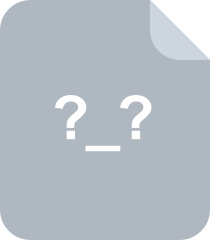
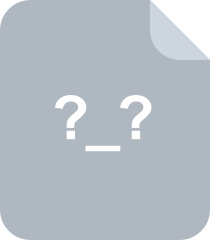
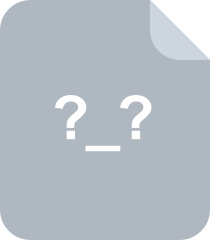
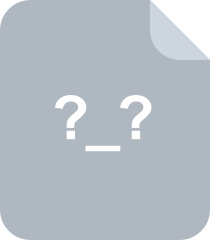
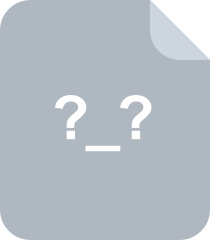
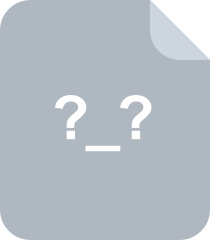

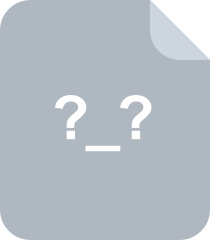
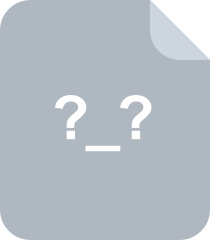
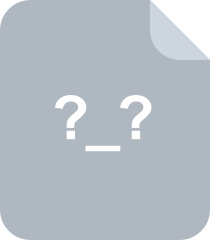
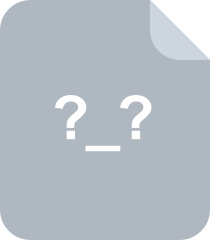
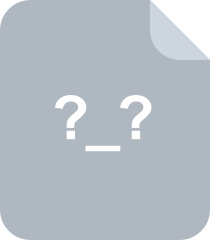
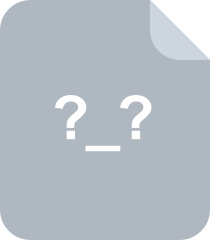
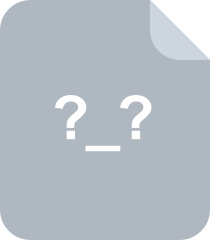
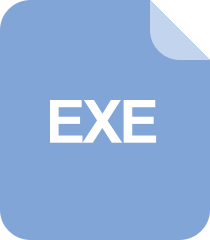
共 19 条
- 1
资源评论
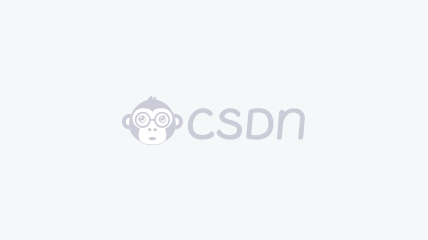
- rico8410092014-05-04可以使用,辛苦了~!
- sxty02202013-01-29谢谢,资料不错,可惜不能使用。
- shuyoufeng2018-08-24可以使用。
- mingd2014-03-13可惜我解压后不太会用
- fj12032016-04-01不太会用,没有什么相关说明

lygisxj
- 粉丝: 26
- 资源: 18
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

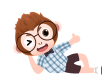
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


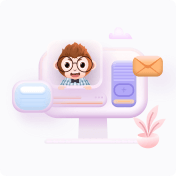
安全验证
文档复制为VIP权益,开通VIP直接复制
