/*******************************************************************************
* *
* Author : Angus Johnson *
* Version : 6.2.1 *
* Date : 31 October 2014 *
* Website : http://www.angusj.com *
* Copyright : Angus Johnson 2010-2014 *
* *
* License: *
* Use, modification & distribution is subject to Boost Software License Ver 1. *
* http://www.boost.org/LICENSE_1_0.txt *
* *
* Attributions: *
* The code in this library is an extension of Bala Vatti's clipping algorithm: *
* "A generic solution to polygon clipping" *
* Communications of the ACM, Vol 35, Issue 7 (July 1992) pp 56-63. *
* http://portal.acm.org/citation.cfm?id=129906 *
* *
* Computer graphics and geometric modeling: implementation and algorithms *
* By Max K. Agoston *
* Springer; 1 edition (January 4, 2005) *
* http://books.google.com/books?q=vatti+clipping+agoston *
* *
* See also: *
* "Polygon Offsetting by Computing Winding Numbers" *
* Paper no. DETC2005-85513 pp. 565-575 *
* ASME 2005 International Design Engineering Technical Conferences *
* and Computers and Information in Engineering Conference (IDETC/CIE2005) *
* September 24-28, 2005 , Long Beach, California, USA *
* http://www.me.berkeley.edu/~mcmains/pubs/DAC05OffsetPolygon.pdf *
* *
*******************************************************************************/
/*******************************************************************************
* *
* This is a translation of the Delphi Clipper library and the naming style *
* used has retained a Delphi flavour. *
* *
*******************************************************************************/
#include "clipper.hpp"
#include <cmath>
#include <vector>
#include <algorithm>
#include <stdexcept>
#include <cstring>
#include <cstdlib>
#include <ostream>
#include <functional>
namespace ClipperLib {
static double const pi = 3.141592653589793238;
static double const two_pi = pi *2;
static double const def_arc_tolerance = 0.25;
enum Direction { dRightToLeft, dLeftToRight };
static int const Unassigned = -1; //edge not currently 'owning' a solution
static int const Skip = -2; //edge that would otherwise close a path
#define HORIZONTAL (-1.0E+40)
#define TOLERANCE (1.0e-20)
#define NEAR_ZERO(val) (((val) > -TOLERANCE) && ((val) < TOLERANCE))
struct TEdge {
IntPoint Bot;
IntPoint Curr;
IntPoint Top;
IntPoint Delta;
double Dx;
PolyType PolyTyp;
EdgeSide Side;
int WindDelta; //1 or -1 depending on winding direction
int WindCnt;
int WindCnt2; //winding count of the opposite polytype
int OutIdx;
TEdge *Next;
TEdge *Prev;
TEdge *NextInLML;
TEdge *NextInAEL;
TEdge *PrevInAEL;
TEdge *NextInSEL;
TEdge *PrevInSEL;
};
struct IntersectNode {
TEdge *Edge1;
TEdge *Edge2;
IntPoint Pt;
};
struct LocalMinimum {
cInt Y;
TEdge *LeftBound;
TEdge *RightBound;
};
struct OutPt;
struct OutRec {
int Idx;
bool IsHole;
bool IsOpen;
OutRec *FirstLeft; //see comments in clipper.pas
PolyNode *PolyNd;
OutPt *Pts;
OutPt *BottomPt;
};
struct OutPt {
int Idx;
IntPoint Pt;
OutPt *Next;
OutPt *Prev;
};
struct Join {
OutPt *OutPt1;
OutPt *OutPt2;
IntPoint OffPt;
};
struct LocMinSorter
{
inline bool operator()(const LocalMinimum& locMin1, const LocalMinimum& locMin2)
{
return locMin2.Y < locMin1.Y;
}
};
//------------------------------------------------------------------------------
//------------------------------------------------------------------------------
inline cInt Round(double val)
{
if ((val < 0)) return static_cast<cInt>(val - 0.5);
else return static_cast<cInt>(val + 0.5);
}
//------------------------------------------------------------------------------
inline cInt Abs(cInt val)
{
return val < 0 ? -val : val;
}
//------------------------------------------------------------------------------
// PolyTree methods ...
//------------------------------------------------------------------------------
void PolyTree::Clear()
{
for (PolyNodes::size_type i = 0; i < AllNodes.size(); ++i)
delete AllNodes[i];
AllNodes.resize(0);
Childs.resize(0);
}
//------------------------------------------------------------------------------
PolyNode* PolyTree::GetFirst() const
{
if (!Childs.empty())
return Childs[0];
else
return 0;
}
//------------------------------------------------------------------------------
int PolyTree::Total() const
{
int result = (int)AllNodes.size();
//with negative offsets, ignore the hidden outer polygon ...
if (result > 0 && Childs[0] != AllNodes[0]) result--;
return result;
}
//------------------------------------------------------------------------------
// PolyNode methods ...
//------------------------------------------------------------------------------
PolyNode::PolyNode(): Childs(), Parent(0), Index(0), m_IsOpen(false)
{
}
//------------------------------------------------------------------------------
int PolyNode::ChildCount() const
{
return (int)Childs.size();
}
//------------------------------------------------------------------------------
void PolyNode::AddChild(PolyNode& child)
{
unsigned cnt = (unsigned)Childs.size();
Childs.push_back(&child);
child.Parent = this;
child.Index = cnt;
}
//------------------------------------------------------------------------------
PolyNode* PolyNode::GetNext() const
{
if (!Childs.empty())
return Childs[0];
else
return GetNextSiblingUp();
}
//------------------------------------------------------------------------------
PolyNode* PolyNode::GetNextSiblingUp() const
{
if (!Parent) //protects against PolyTree.GetNextSiblingUp()
return 0;
else if (Index == Parent->Childs.size() - 1)
return Parent->GetNextSiblingUp();
else
return Parent->Childs[Index + 1];
}
//------------------------------------------------------------------------------
bool PolyNode::IsHole() const
{
bool result = true;
PolyNode* node = Parent;
while (node)
{
result = !result;
node = node->Parent;
}
return result;
}
//------------------------------------------------------------------------------
bool PolyNode::IsOpen() const
{
return m_IsOpen;
}
//------------------------------------------------------------------------------
#ifndef use_int32
//------------------------------------------------------------------------------
// Int128 class (enables safe math on signed 64bit integers)
//
没有合适的资源?快使用搜索试试~ 我知道了~
curaengine-vs2017
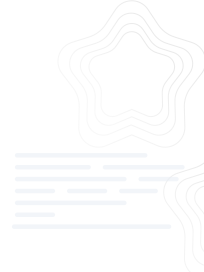
共484个文件
h:364个
cpp:70个
proto:25个


温馨提示
1、右键 curaengine 项目 ---> 属性 ---> VC++目录,修改 “包含目录” 和 “库目录” 里的路径,改为本地的路径。 2、Debug下:“包含目录” 里的三个路径改为本项目下的 “\curaengine”、“\curaengine\src”、“\curaengine\protobuf_d \include”, “库目录”里的路径改为本项目下的 “\curaengine\protobuf_d\lib”。 3、Release下:“包含目录” 里的三个路径改为本项目下的 “\curaengine”、“\curaengine\src”、“\curaengine\protobuf \include”, “库目录”里的路径改为本项目下的 “\curaengine\protobuf\lib”。 4、同样在“属性”里,在 “调试” 里,修改 “命令参数” 里的参数。将 “-j”、“-l”、“-o” 参数后的路径分别改为此仓库中包含 “fdmprinter.def.json” 和 “baymax_print.stl” 两个文件所在路径,以及想要gcode文件生成在的路径。
资源推荐
资源详情
资源评论
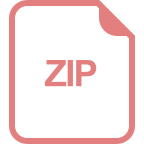
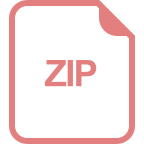
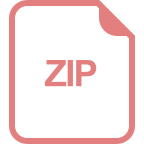
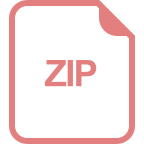
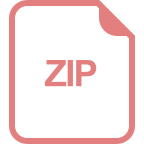
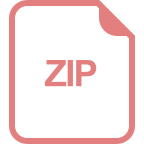
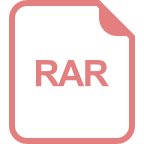
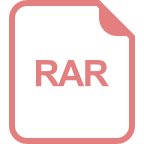
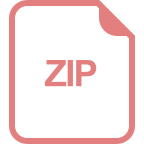
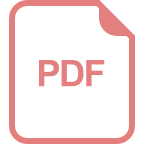
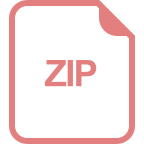
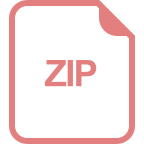
收起资源包目录

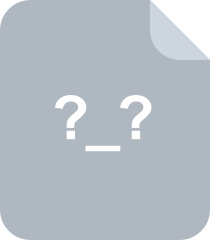
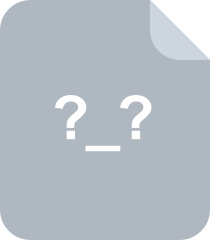
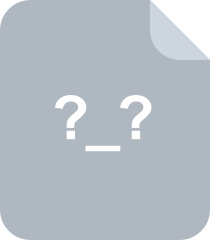
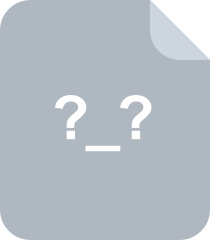
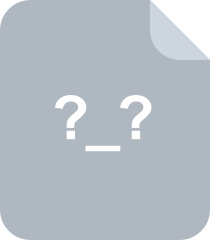
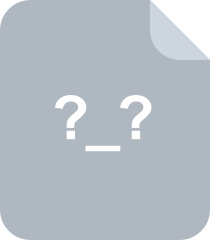
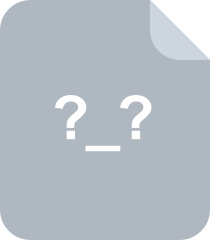
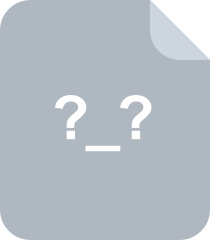
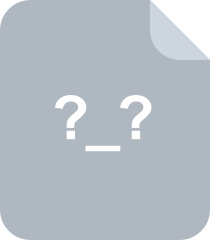
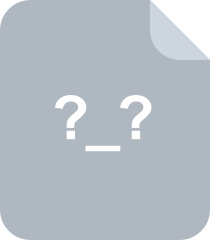
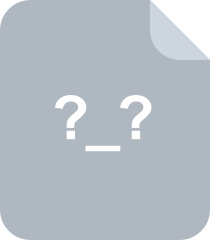
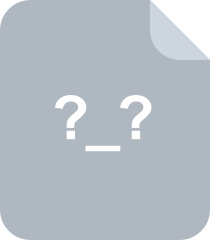
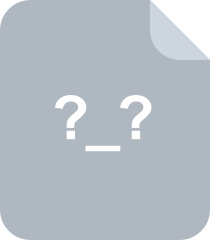
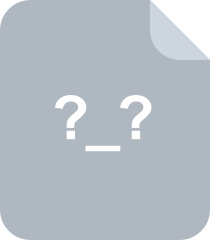
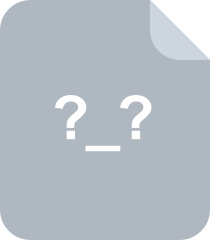
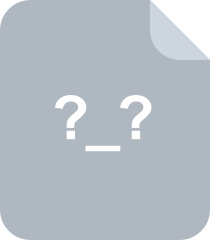
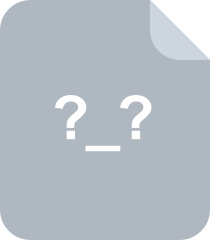
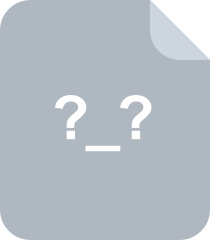
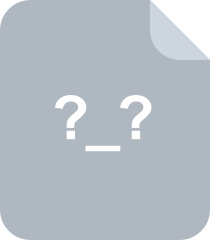
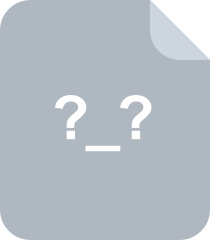
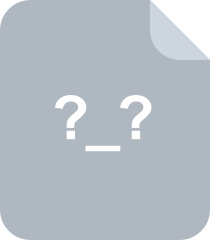
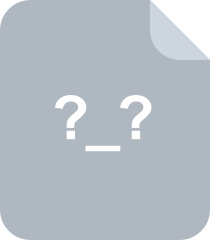
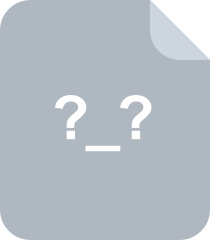
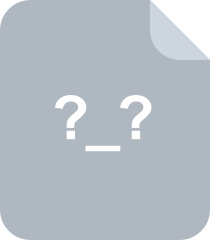
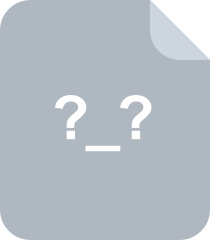
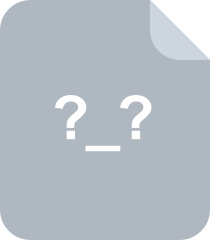
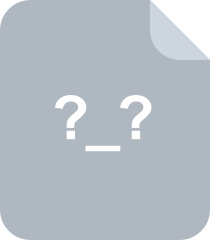
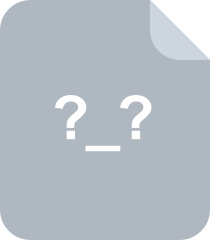
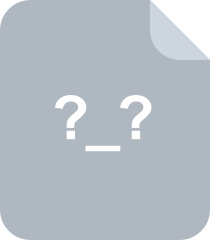
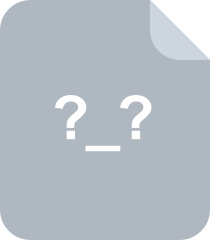
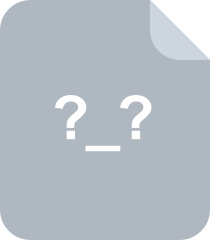
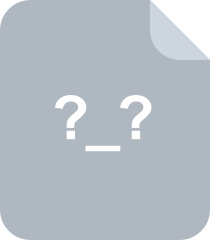
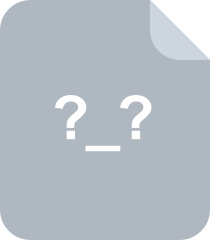
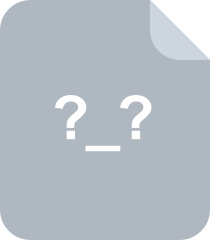
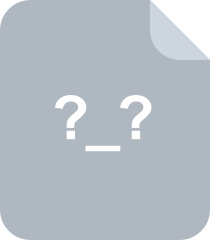
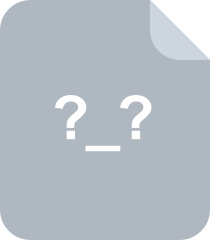
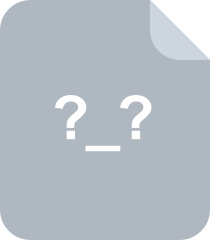
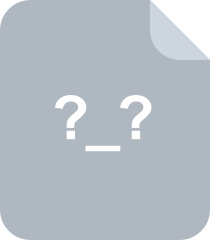
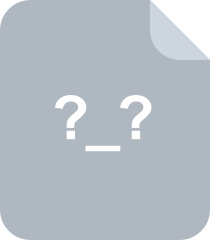
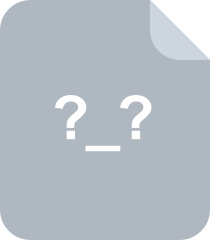
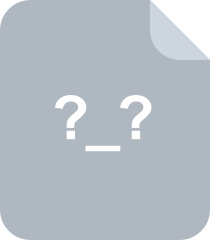
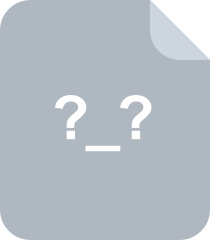
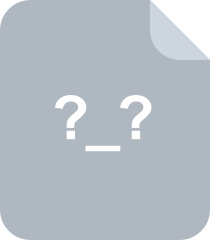
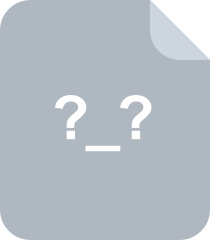
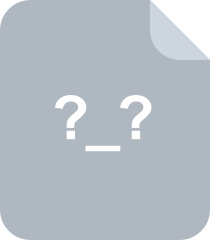
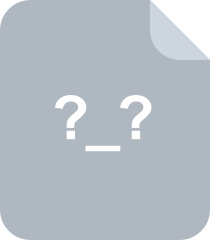
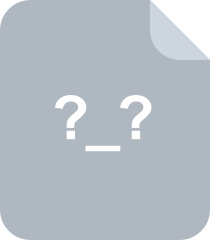
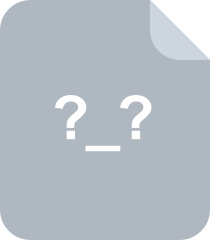
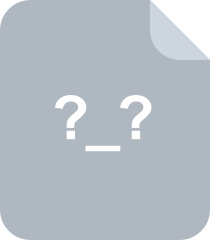
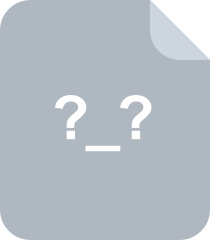
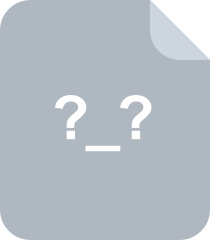
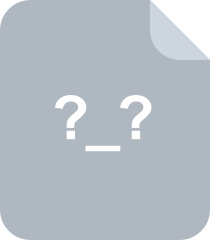
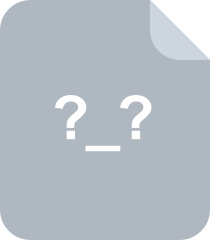
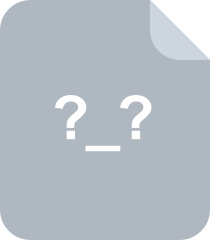
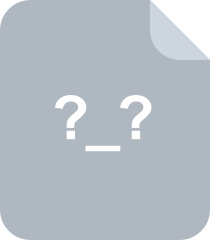
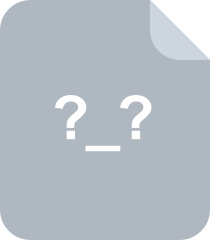
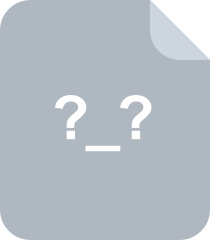
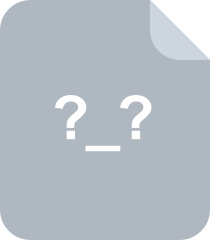
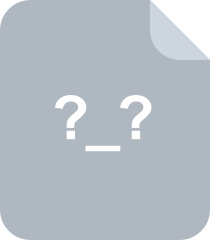
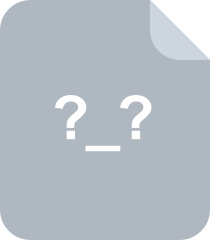
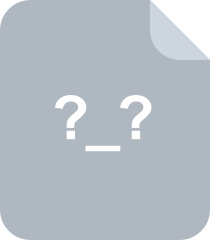
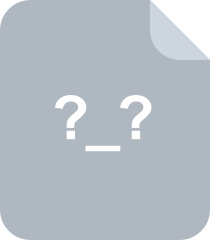
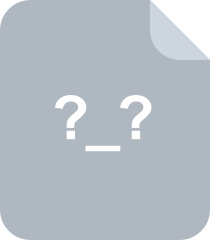
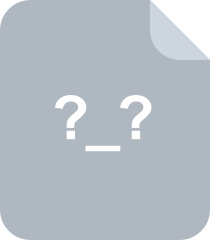
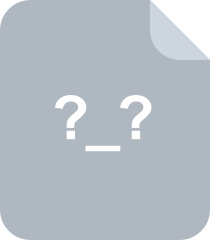
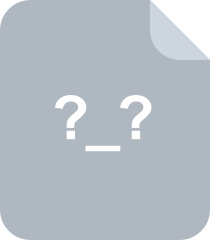
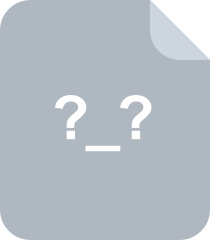
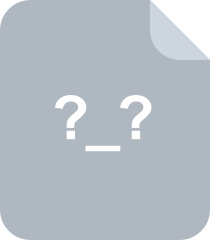
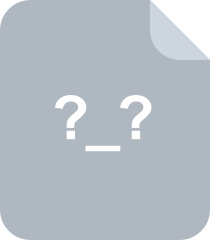
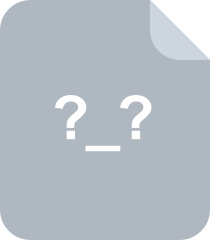
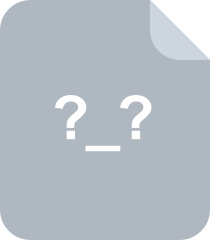
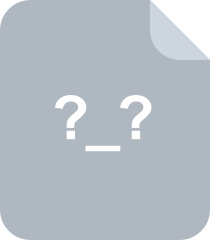
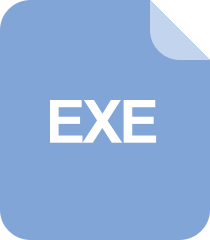
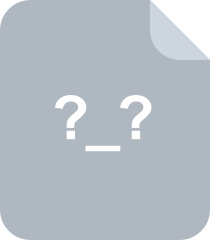
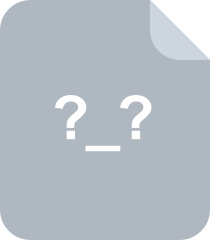
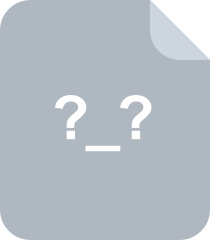
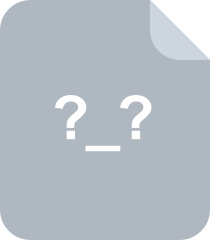
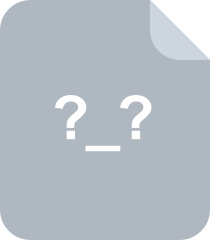
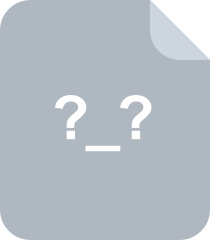
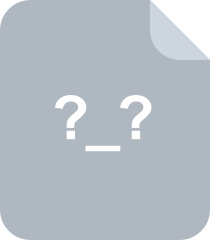
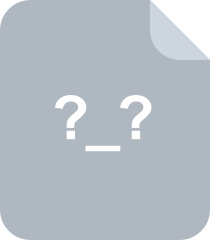
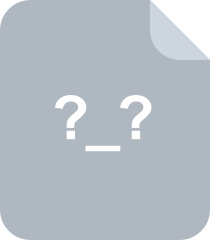
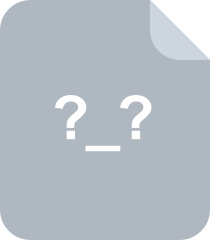
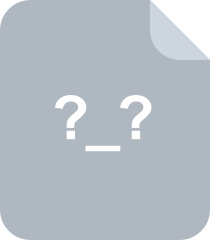
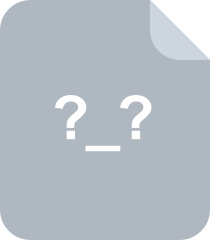
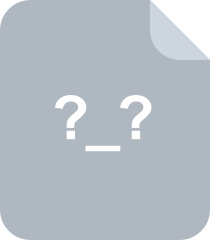
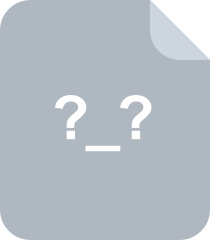
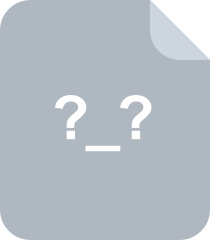
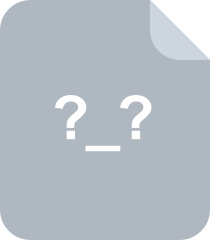
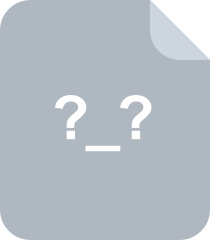
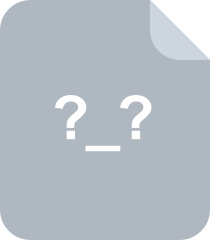
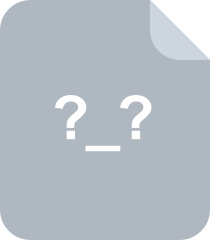
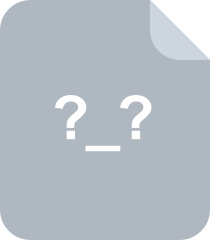
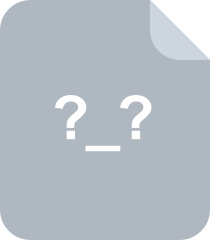
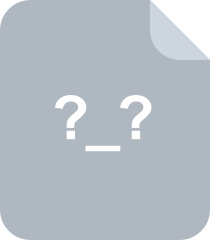
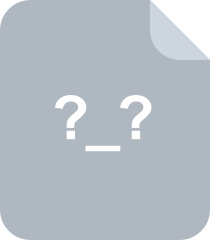
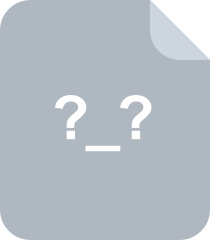
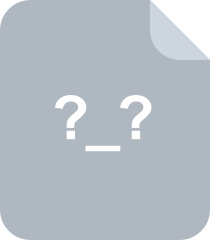
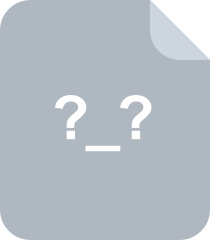
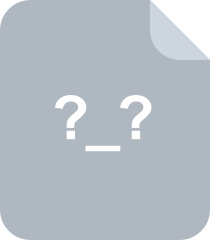
共 484 条
- 1
- 2
- 3
- 4
- 5
资源评论
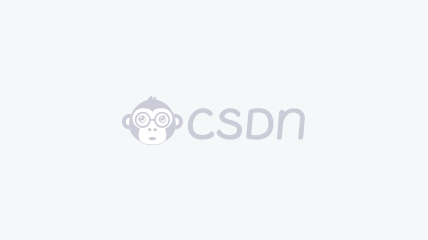
- 郭华112018-04-11编译通不过,与开源版一样多错误,不值这么多积分!!!!

小僵尸蹲丶
- 粉丝: 0
- 资源: 12
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

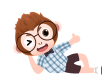
最新资源
- (178047214)基于springboot图书管理系统.zip
- 张郅奇 的Python学习过程
- (23775420)欧姆龙PLC CP1H-E CP1L-E CJ2M CP1E 以太网通讯.zip
- (174590622)计算机课程设计-IP数据包解析
- (175550824)泛海三江全系调试软件PCSet-All2.0.3 1
- (172742832)实验1 - LC并联谐振回路仿真实验报告1
- 网络搭建练习题.pkt
- 搜索引擎soler的相关介绍 从事搜索行业程序研发、人工智能、存储等技术人员和企业
- 搜索引擎lucen的相关介绍 从事搜索行业程序研发、人工智能、存储等技术人员和企业
- 基于opencv-dnn和一些超过330 FPS的npu
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


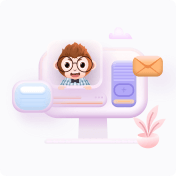
安全验证
文档复制为VIP权益,开通VIP直接复制
