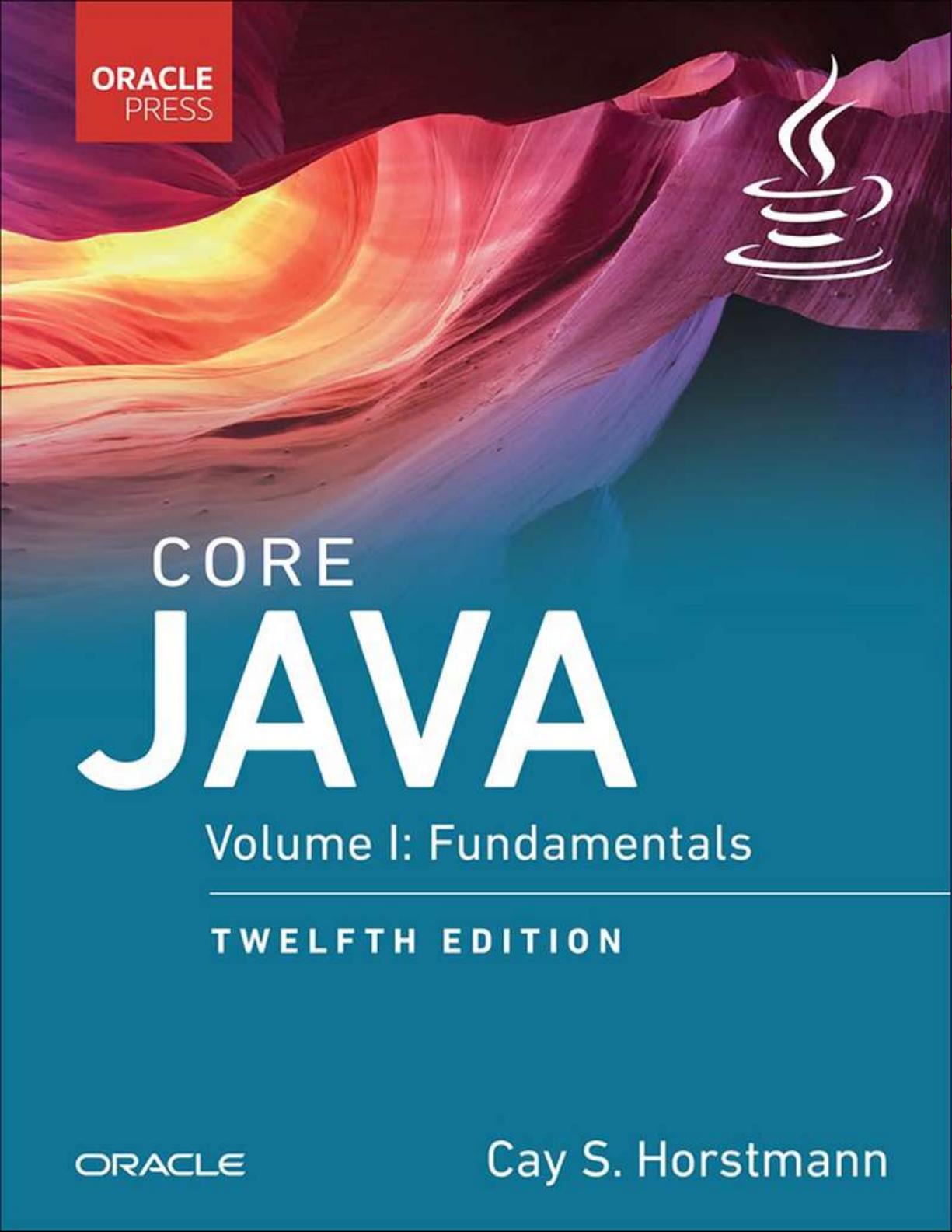
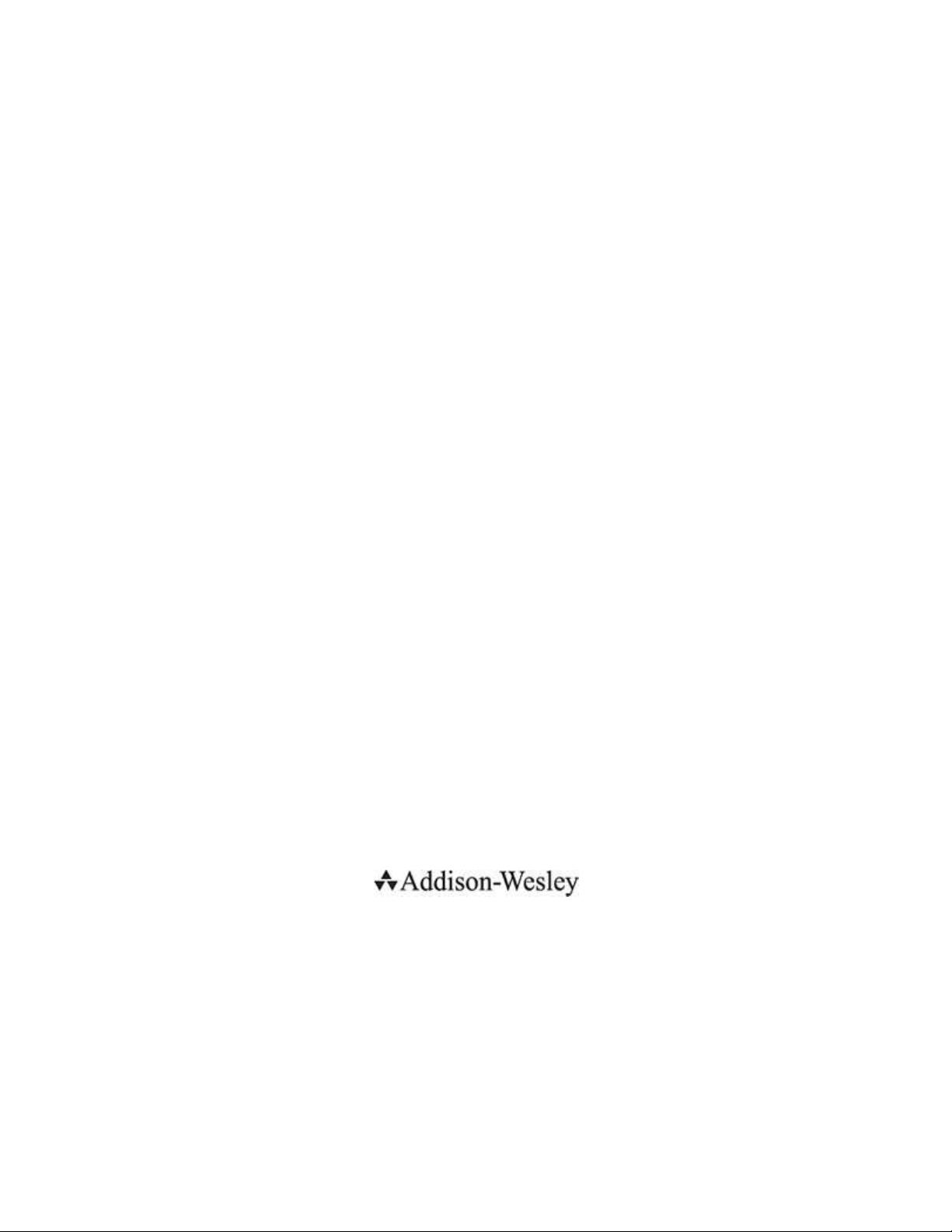
Core Java, Volume I:
Fundamentals
Twelfth Edition
Cay S. Horstmann
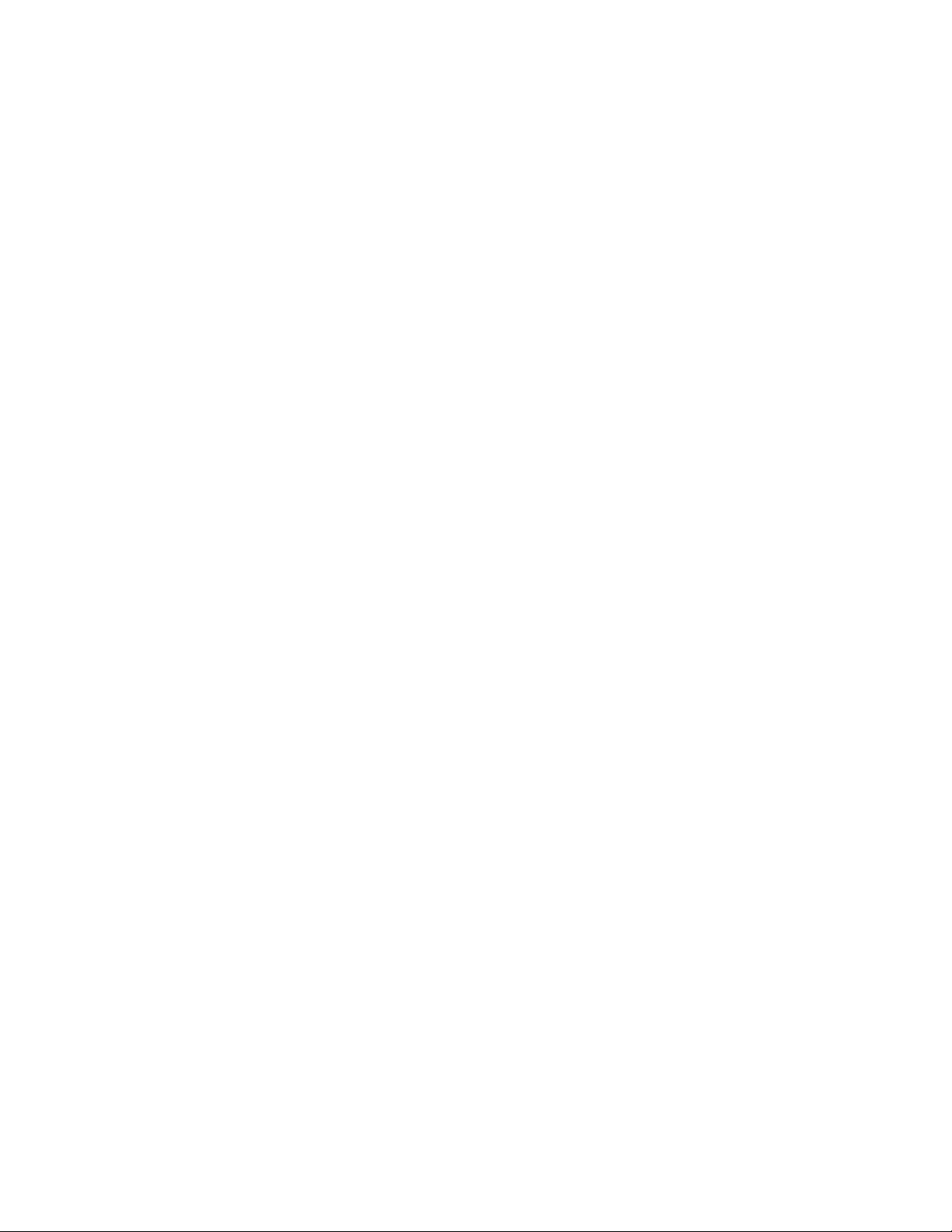
Contents
Preface
Acknowledgments
Chapter 1: An Introduction to Java
Chapter 2: The Java Programming Environment
Chapter 3: Fundamental Programming Structures in Java
Chapter 4: Objects and Classes
Chapter 5: Inheritance
Chapter 7: Exceptions, Assertions, and Logging
Chapter 8: Generic Programming
Chapter 9: Collections
Chapter 10: Graphical User Interface Programming
Chapter 11: User Interface Components with Swing
Chapter 12: Concurrency
Appendix
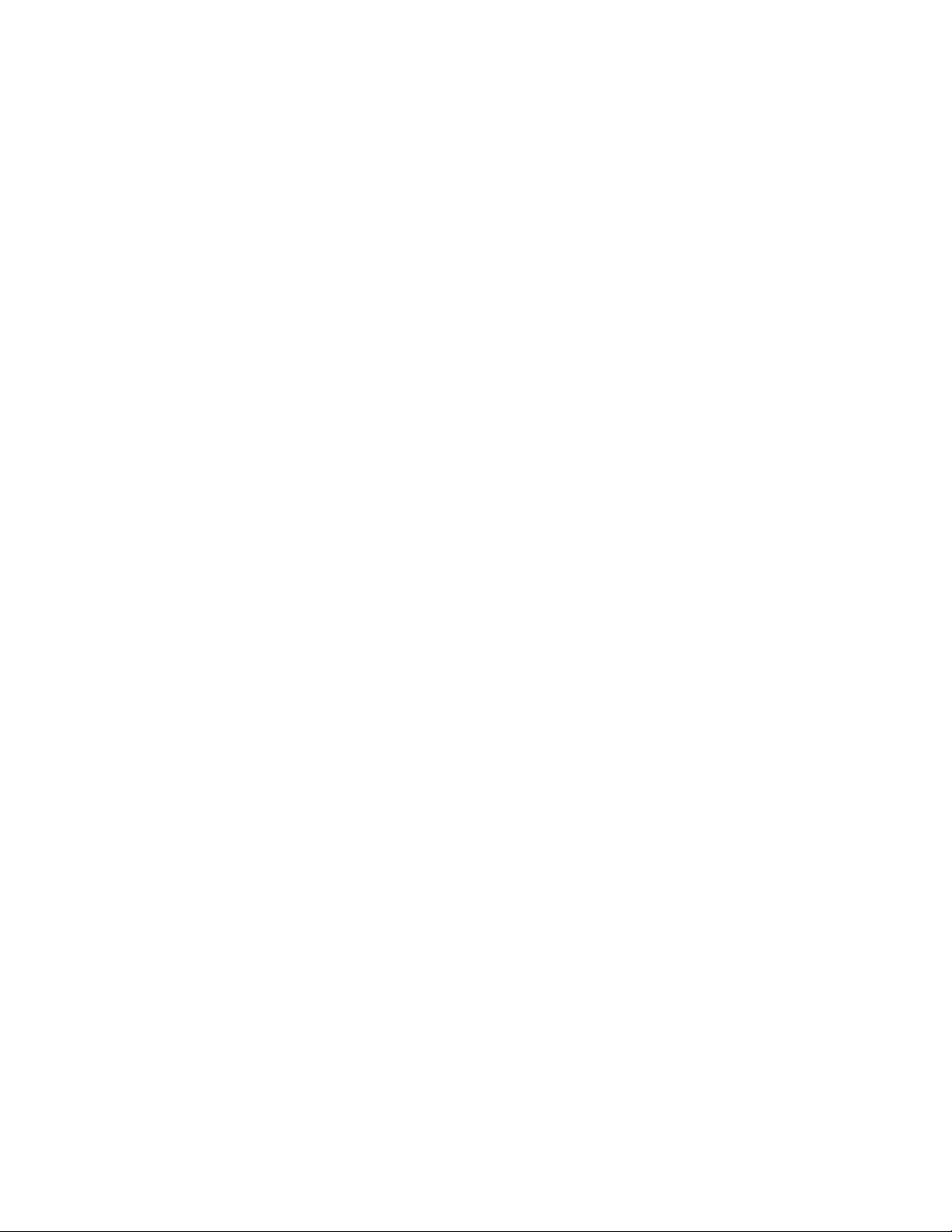
Table of Contents
Preface
Acknowledgments
Chapter 1: An Introduction to Java
1.1 Java as a Programming Platform
1.2 The Java “White Paper” Buzzwords
1.2.1 Simple
1.2.2 Object-Oriented
1.2.3 Distributed
1.2.4 Robust
1.2.5 Secure
1.2.6 Architecture-Neutral
1.2.7 Portable
1.2.8 Interpreted
1.2.9 High-Performance
1.2.10 Multithreaded
1.2.11 Dynamic
1.3 Java Applets and the Internet
1.4 A Short History of Java
1.5 Common Misconceptions about Java
Chapter 2: The Java Programming Environment
2.1 Installing the Java Development Kit
2.1.1 Downloading the JDK
2.1.2 Setting up the JDK
2.1.3 Installing Source Files and Documentation
2.2 Using the Command-Line Tools
2.3 Using an Integrated Development Environment
2.4 JShell
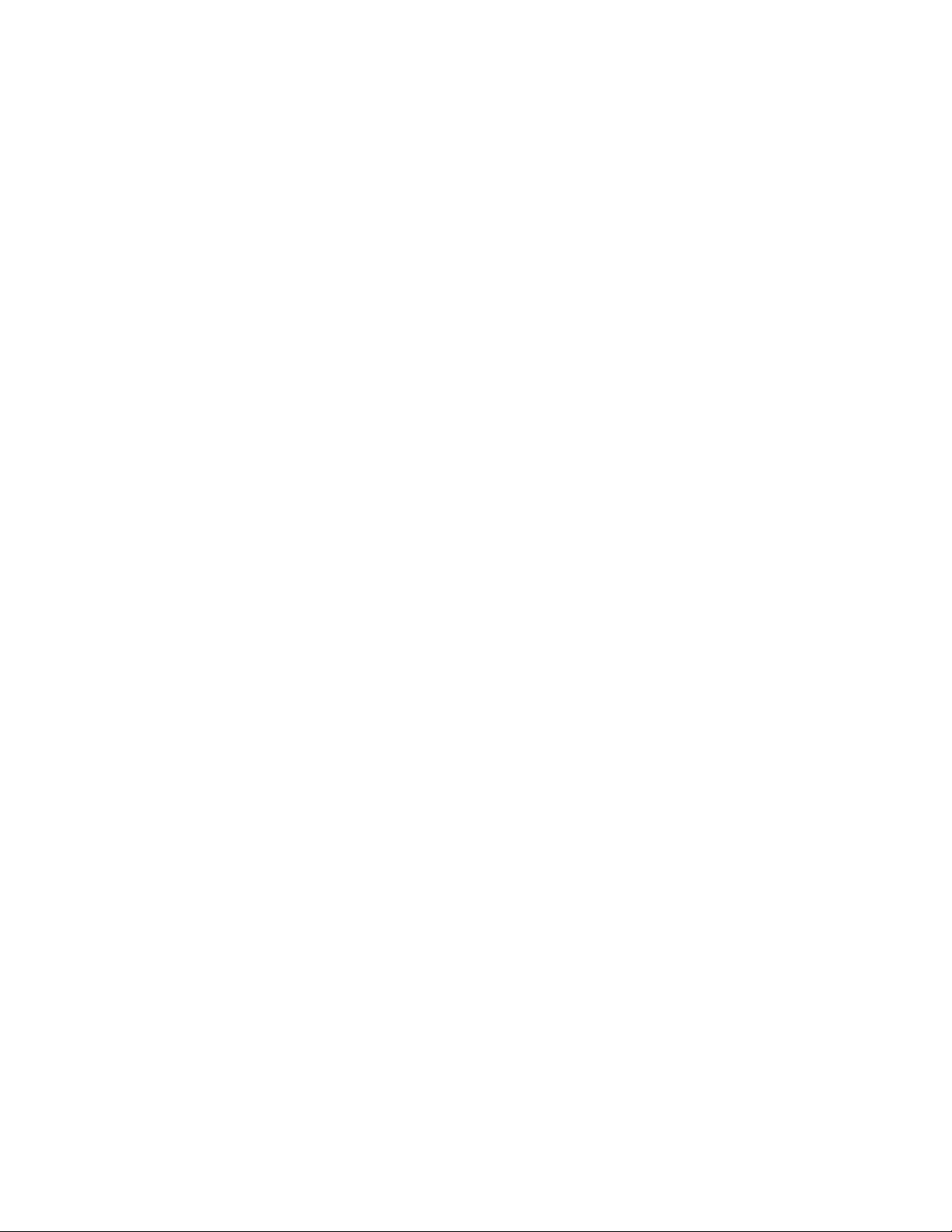
Chapter 3: Fundamental Programming Structures in Java
3.1 A Simple Java Program
3.2 Comments
3.3 Data Types
3.3.1 Integer Types
3.3.2 Floating-Point Types
3.3.3 The char Type
3.3.4 Unicode and the char Type
3.3.5 The boolean Type
3.4 Variables and Constants
3.4.1 Declaring Variables
3.4.2 Initializing Variables
3.4.3 Constants
3.4.4 Enumerated Types
3.5 Operators
3.5.1 Arithmetic Operators
3.5.2 Mathematical Functions and Constants
3.5.3 Conversions between Numeric Types
3.5.4 Casts
3.5.5 Assignment
3.5.6 Increment and Decrement Operators
3.5.7 Relational and boolean Operators
3.5.8 The Conditional Operator
3.5.9 Switch Expressions
3.5.10 Bitwise Operators
3.5.11 Parentheses and Operator Hierarchy
3.6 Strings
3.6.1 Substrings
3.6.2 Concatenation
3.6.3 Strings Are Immutable
3.6.4 Testing Strings for Equality