package org.mypro.entity;
import java.util.Date;
public class User {
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.ID
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private Integer id;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.USERNAME
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String username;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.PASSWORD
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String password;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.SEX
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String sex;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.AGE
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private Integer age;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.EMAIL
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String email;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.ISENABLE
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String isenable;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.TYPE_
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String type;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.CREATETIME
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private Date createtime;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.EXT1
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String ext1;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.EXT2
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String ext2;
/**
*
* This field was generated by MyBatis Generator.
* This field corresponds to the database column user_.EXT3
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
private String ext3;
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column user_.ID
*
* @return the value of user_.ID
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public Integer getId() {
return id;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column user_.ID
*
* @param id the value for user_.ID
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public void setId(Integer id) {
this.id = id;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column user_.USERNAME
*
* @return the value of user_.USERNAME
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public String getUsername() {
return username;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column user_.USERNAME
*
* @param username the value for user_.USERNAME
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public void setUsername(String username) {
this.username = username;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column user_.PASSWORD
*
* @return the value of user_.PASSWORD
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public String getPassword() {
return password;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column user_.PASSWORD
*
* @param password the value for user_.PASSWORD
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public void setPassword(String password) {
this.password = password;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column user_.SEX
*
* @return the value of user_.SEX
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public String getSex() {
return sex;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column user_.SEX
*
* @param sex the value for user_.SEX
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public void setSex(String sex) {
this.sex = sex;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column user_.AGE
*
* @return the value of user_.AGE
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public Integer getAge() {
return age;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column user_.AGE
*
* @param age the value for user_.AGE
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public void setAge(Integer age) {
this.age = age;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column user_.EMAIL
*
* @return the value of user_.EMAIL
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public String getEmail() {
return email;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column user_.EMAIL
*
* @param email the value for user_.EMAIL
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public void setEmail(String email) {
this.email = email;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column user_.ISENABLE
*
* @return the value of user_.ISENABLE
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public String getIsenable() {
return isenable;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column user_.ISENABLE
*
* @param isenable the value for user_.ISENABLE
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public void setIsenable(String isenable) {
this.isenable = isenable;
}
/**
* This method was generated by MyBatis Generator.
* This method returns the value of the database column user_.TYPE_
*
* @return the value of user_.TYPE_
*
* @mbggenerated Sat Feb 18 13:47:27 CST 2017
*/
public String getType() {
return type;
}
/**
* This method was generated by MyBatis Generator.
* This method sets the value of the database column user_.TYPE_
*
* @param
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
主要有以下模块: 管理员用户: 1.实时路况管理:实时路况的信息采用了百度地图进行直观的管理,利用了GIS相关技术进行管理,能够让用户方便的第一时间查看到相应的地图信息,以及实时路况信息。 2.投诉留言管理:实现了对投诉留言信息的查看和回复。 3.系统信息设置:实现了系统的访问数据的统计,以及针对系统的管理员 用户和管理员密码进行管理。 4.用户信息管理:管理了一般用户的基本信息情况,针对用户的资料进行修改管理。 一般用户: 1.用户资料管理:实现了用户个人的资料信息管理。 2.路况信息查看:实现了对路径的实时信息的查看,某个路段在某时间的交通情况的查看,以三种情况代表路况情况(拥挤、缓行和畅通) 3.路况分析:采用了折线图,分析每天或者某个月的路况信息,以折线图形式直观展示。该功能采用jFreeChart库实现。 4.留言发布:针对一些路况信息,进行留言反馈,并能查看管理员反馈信息。
资源推荐
资源详情
资源评论
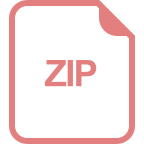
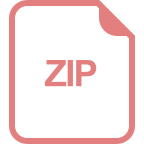
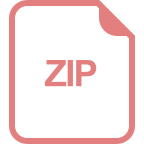
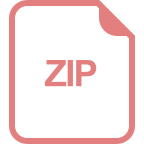
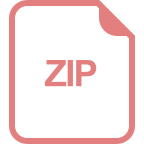
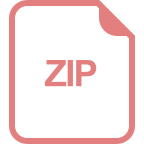
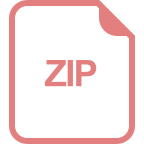
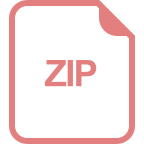
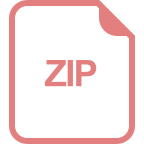
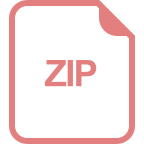
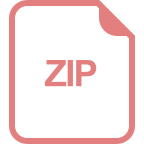
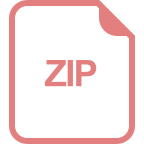
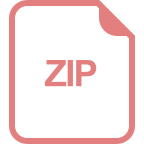
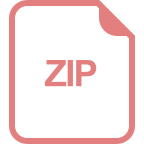
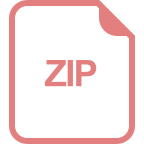
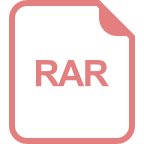
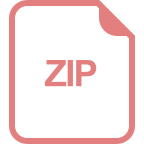
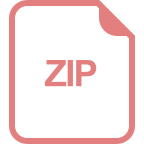
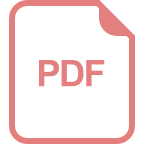
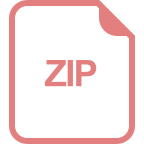
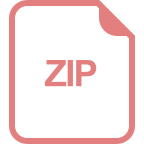
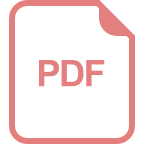
收起资源包目录

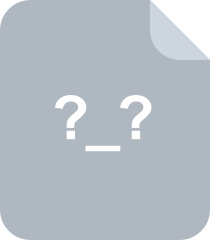
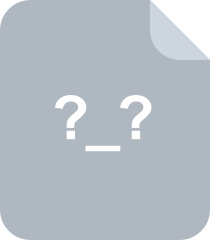
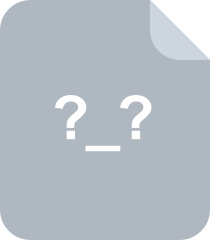
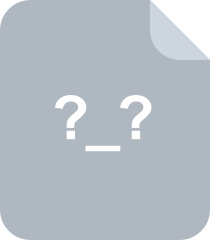
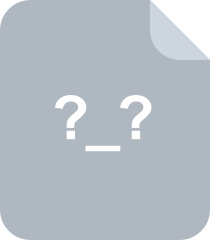
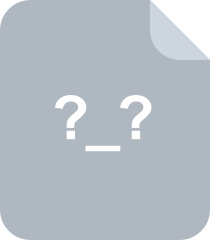
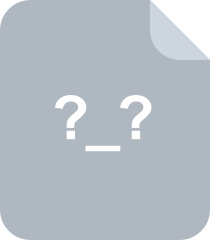
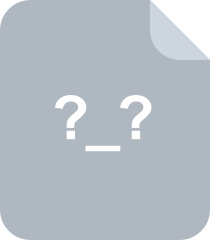
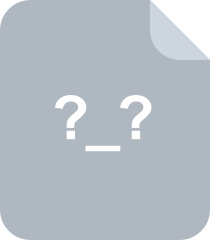
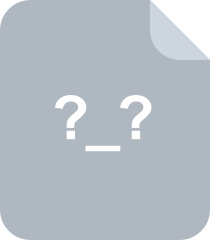
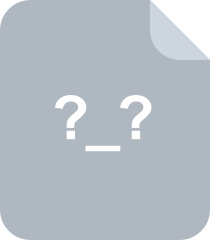
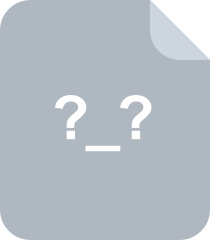
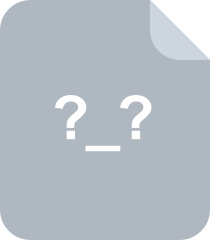
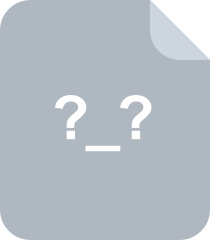
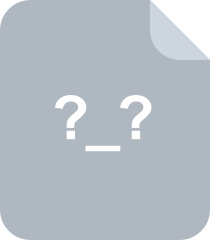
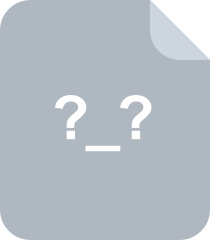
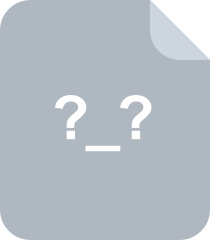
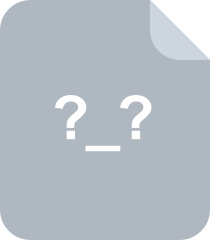
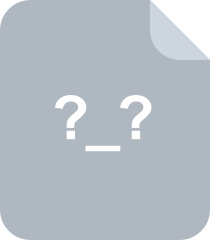
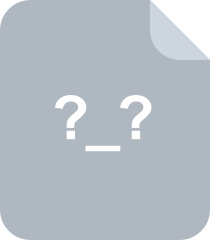
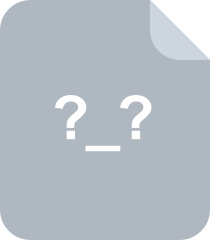
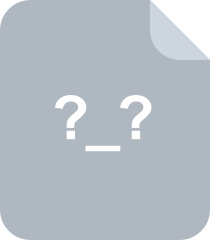
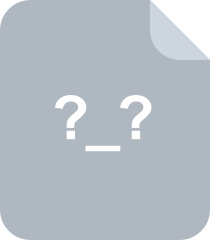
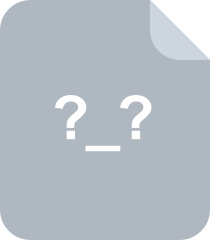
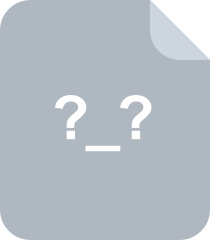
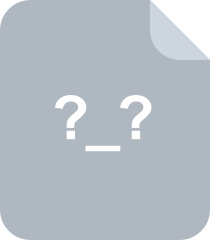
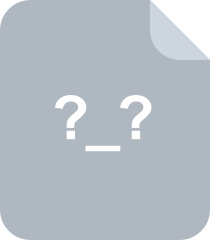
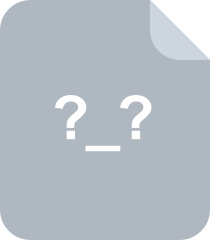
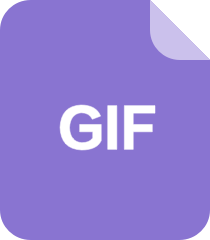
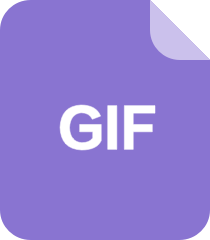
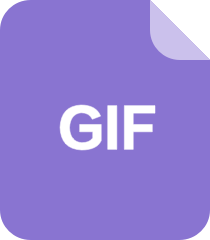
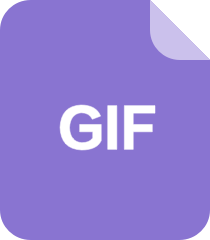
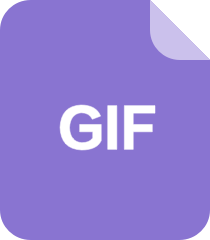
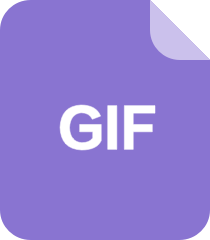
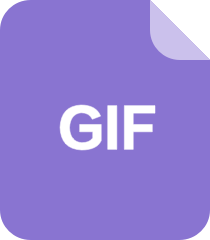
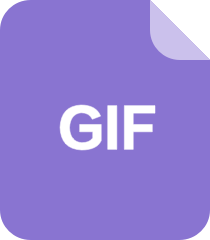
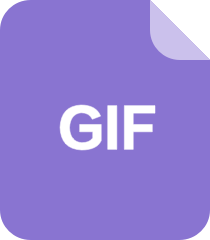
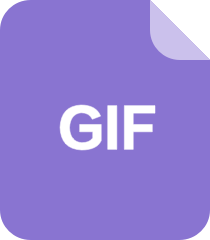
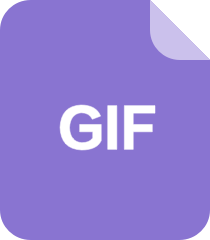
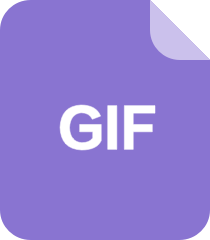
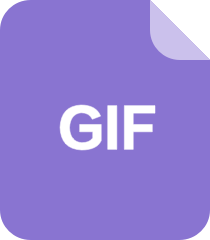
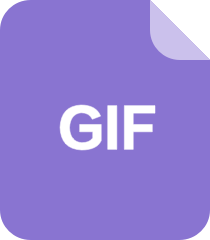
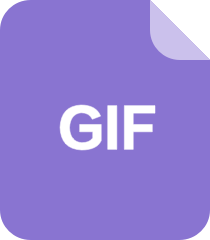
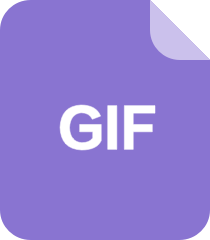
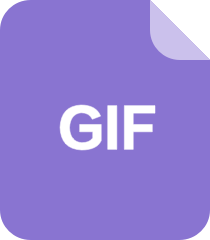
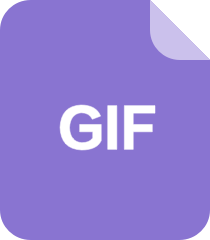
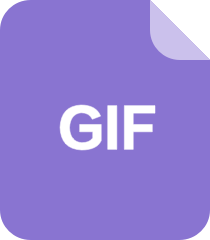
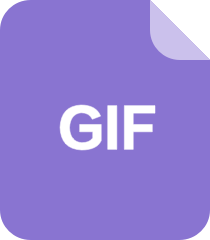
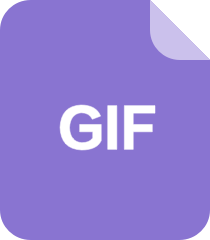
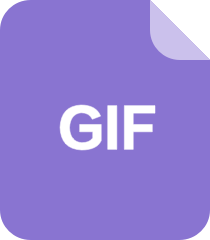
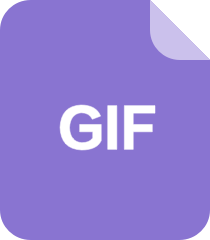
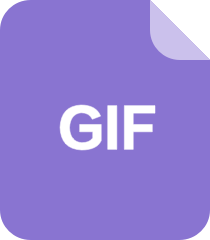
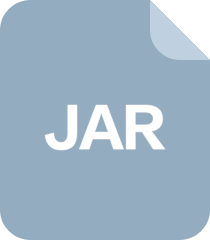
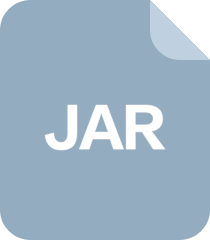
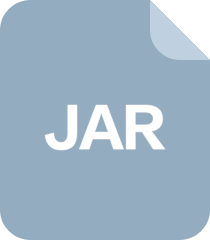
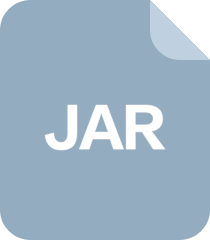
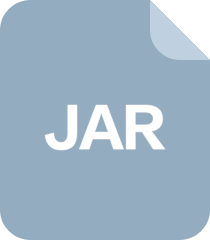
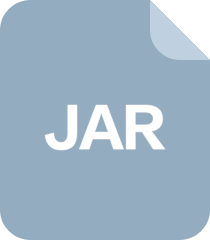
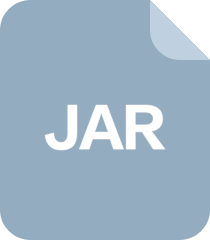
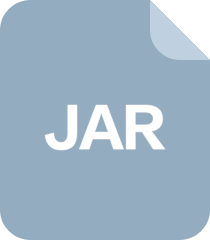
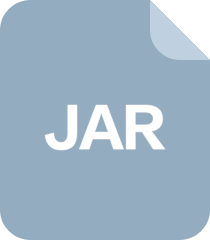
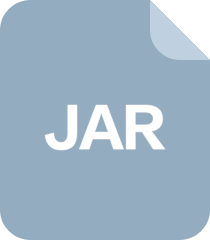
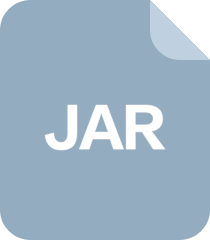
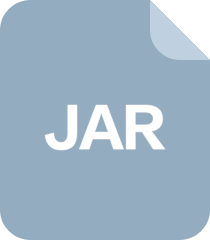
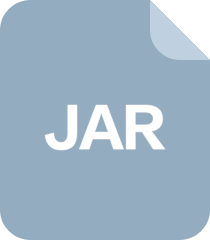
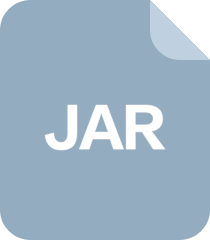
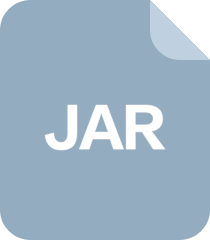
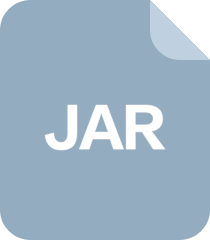
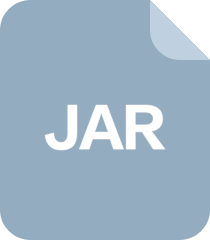
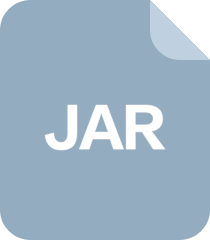
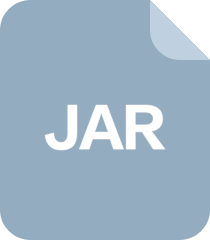
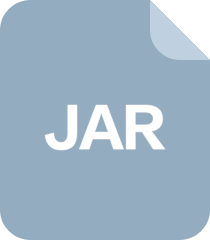
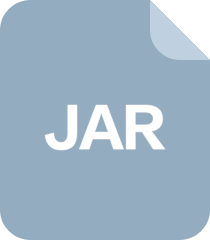
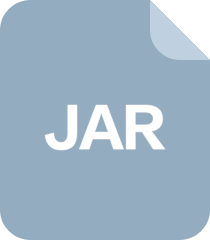
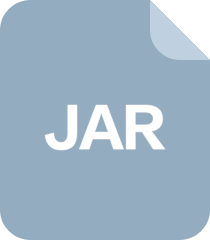
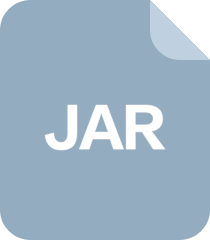
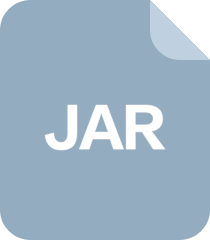
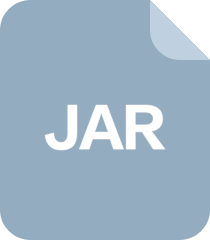
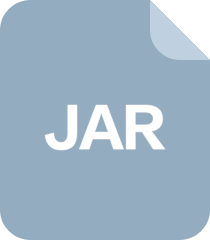
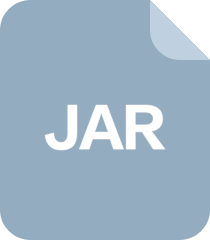
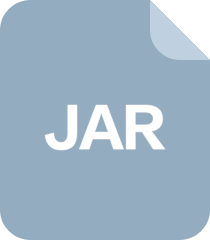
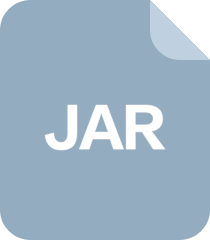
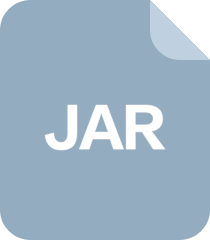
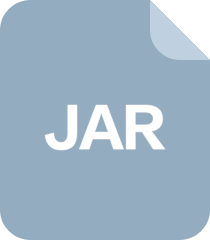
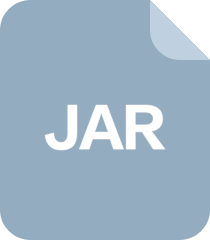
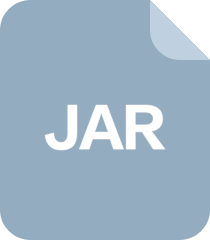
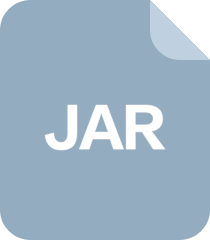
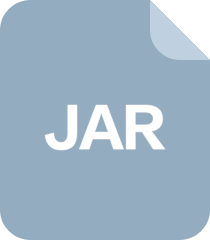
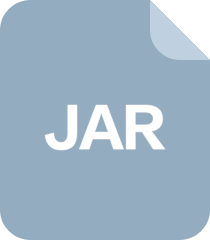
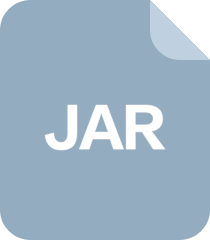
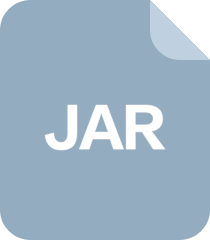
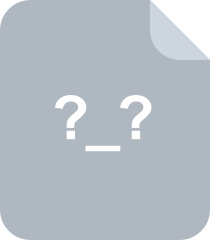
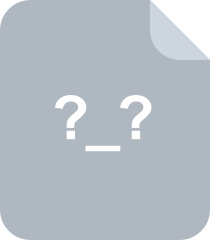
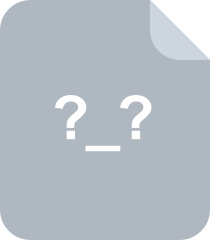
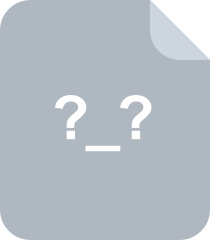
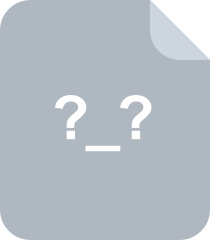
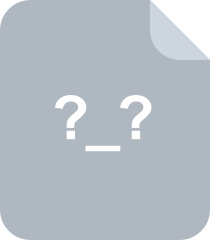
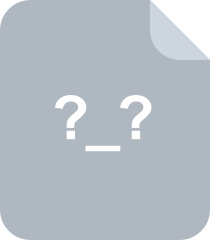
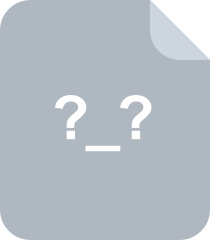
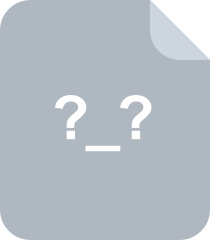
共 194 条
- 1
- 2
资源评论
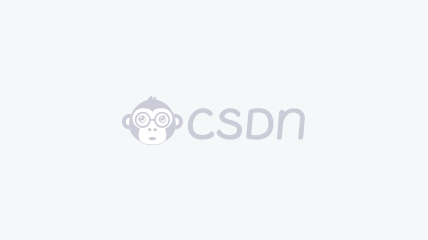

码农落落
- 粉丝: 1001
- 资源: 4364
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

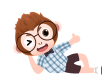
最新资源
- 基于区块链的乳制品溯源系统文档+源码+全部资料+高分项目.zip
- 基于区块链技术之可溯源珠宝电商平台文档+源码+全部资料+高分项目.zip
- 基于区块链的药品溯源系统(学习开发中)文档+源码+全部资料+高分项目.zip
- 基于事件驱动+事件溯源+Saga的微服务示例文档+源码+全部资料+高分项目.zip
- 基于使用Axon框架基于DDD领域驱动设计、CQRS读写分离和事件溯源来实现货物运输系统文档+源码+全部资料+高分项目.zip
- 基于若依后台管理系统的代码溯源系统文档+源码+全部资料+高分项目.zip
- 基于以太坊 Solidity 语言开发秒钛坊区块链智能合约致辞供应链金融信贷周期全流程溯源文档+源码+全部资料+高分项目.zip
- 基于事件溯源基于事件回溯的高性能架构,例如:秒杀、抢红包、12306卖票等,实现cqrs最复杂的模型, 通过事件是追加的特性,然后结合事件批量提交的手段,避免在
- Visual Studio Code中的IntelliSense功能详解.pdf
- 基于溯源图的入侵威胁检测相关论文及阅读笔记文档+源码+全部资料+高分项目.zip
- Keil C51 插件 检测所有if语句
- 基于优雅的Laravel框架开发咖啡壶是一个免费、开源、高效且漂亮的资产管理平台。资产管理、归属使用者追溯、盘点以及可靠的服务器状态管理面板文档+源码+全部资料+高分项目.zip
- 基于云链聚合的隐私保护数据共享与溯源平台文档+源码+全部资料+高分项目.zip
- 各种排序算法java实现的源代码.zip
- java考试题目总132
- 用c语言实现各种排序算法
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


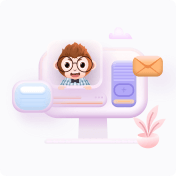
安全验证
文档复制为VIP权益,开通VIP直接复制
