package com.shixin.business.service.impl;
import com.shixin.business.domain.*;
import com.shixin.business.domain.vo.ExportInRegisterEntity;
import com.shixin.business.domain.vo.ExportOutRegisterEntity;
import com.shixin.business.domain.vo.InRegisterExtend;
import com.shixin.business.domain.vo.OutRegisterExtend;
import com.shixin.business.repository.InRegisterRepository;
import com.shixin.business.repository.OutRegisterRepository;
import com.shixin.business.repository.RegisterBatchRepository;
import com.shixin.business.repository.StuRepository;
import com.shixin.business.service.RegisterServiceI;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.PageImpl;
import org.springframework.data.domain.Pageable;
import org.springframework.data.domain.Sort;
import org.springframework.data.jpa.domain.Specification;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import javax.persistence.criteria.Predicate;
import java.lang.reflect.Field;
import java.util.Date;
import java.util.List;
import java.util.Optional;
import java.util.stream.Collectors;
@Service
public class RegisterServiceImpl implements RegisterServiceI {
@Autowired
private InRegisterRepository inRegisterRepository;
@Autowired
private RegisterBatchRepository registerbatchRepository;
@Autowired
private StuRepository stuRepository;
@Autowired
private OutRegisterRepository outRegisterRepository;
@Override
public Boolean isCreateRegister() {
// 查询是否存在未登记完的
List<InRegister> registers = inRegisterRepository.findAllByStatusIn("0", "1");
if (registers != null && registers.size() <= 0) {
return true;
}
return false;
}
@Override
@Transactional
public void createRegister(String name, Staffinfo staffinfo) {
List<Stuinfo> stuinfos = stuRepository.findAllByStatusAndDormidIsLike(0,
staffinfo.getDormname() + staffinfo.getDormno() + "%");
if (stuinfos != null && stuinfos.size() > 0) {
Registerbatch registerbatch = registerbatchRepository.save(new Registerbatch(name, staffinfo.getId(), "in"));
List<InRegister> inRegisters = stuinfos.stream().map(e -> {
InRegister register = new InRegister();
register.setStuid(e.getId());
register.setStaffid(staffinfo.getId());
register.setRebatchid(registerbatch.getId());
register.setStatus("0");
return register;
}).collect(Collectors.toList());
inRegisterRepository.saveAll(inRegisters);
}
}
@Override
public List<ExportInRegisterEntity> findInHistoryList(Staffinfo staffinfo) {
List<InRegister> list = inRegisterRepository.findAll(createExportSpe(staffinfo.getId()), new Sort(Sort.DEFAULT_DIRECTION, "rebatchid"));
return list.stream().map(e -> {
ExportInRegisterEntity entity = new ExportInRegisterEntity();
createEntity(entity, e.getStuid(), e.getRebatchid());
entity.setArrivetime(e.getArrivetime());
return entity;
}).collect(Collectors.toList());
}
private Specification createExportSpe(String staffId) {
return (root, criteriaQuery, criteriaBuilder) -> {
Predicate predicate1 = criteriaBuilder.equal(root.get("status"), "2");
Predicate predicate2 = criteriaBuilder.equal(root.get("staffid"), staffId);
return criteriaBuilder.and(predicate1, predicate2);
};
}
@Override
public List<Registerbatch> getRegisterNames(String staffId, String status) {
String batchId = null;
List<Registerbatch> list = null;
if (StringUtils.equals(status,"in")) {
List<InRegister> registers = inRegisterRepository.findAllByStatusIn("0", "1");
if (registers != null && registers.size() > 0) {
batchId = registers.get(0).getRebatchid();
}
} else {
List<OutRegister> registers = outRegisterRepository.findAllByStatusIn("0", "1");
if (registers != null && registers.size() > 0) {
batchId = registers.get(0).getRebatchid();
}
}
list = registerbatchRepository.findAllByStaffidAndStatus(staffId, status);
if (batchId == null) {
return list;
} else {
if (list != null) {
for (int i = 0; i < list.size(); i++) {
if (list.get(i).getId().equals(batchId)) {
list.remove(i);
break;
}
}
}
}
return list;
}
@Override
public Page<InRegisterExtend> inRegisterList(Pageable pageable, Staffinfo staffinfo) {
Specification<InRegister> registerSpec = (root, criteriaQuery, criteriaBuilder) -> {
Predicate predicate1 = criteriaBuilder.notEqual(root.get("status"), "2");
Predicate predicate2 = criteriaBuilder.equal(root.get("staffid"), staffinfo.getId());
return criteriaBuilder.and(predicate1, predicate2);
};
return createInRegisterExtend(registerSpec, pageable);
}
@Override
public Page<InRegisterExtend> historyList(Pageable pageable, Staffinfo staffinfo, String registerBatchId) {
if (StringUtils.isEmpty(registerBatchId)) {
return null;
}
Specification<InRegister> registerSpec = createSpecification(registerBatchId, staffinfo.getId());
return createInRegisterExtend(registerSpec, pageable);
}
@Override
public Boolean createInHistory(Staffinfo staffinfo) {
try {
inRegisterRepository.updateStatus(staffinfo.getId(), "2");
return true;
} catch (Exception e) {
e.printStackTrace();
}
return false;
}
private Page<InRegisterExtend> createInRegisterExtend(Specification<InRegister> registerSpec, Pageable pageable) {
Page<InRegister> inRegisters = inRegisterRepository.findAll(registerSpec, pageable);
List<InRegisterExtend> extendList = inRegisters.getContent().stream().map(e -> {
InRegisterExtend extend = new InRegisterExtend();
createEntity(extend, e.getStuid(), e.getRebatchid());
extend.setArrivetime(e.getArrivetime());
return extend;
}).collect(Collectors.toList());
return new PageImpl<>(extendList, pageable, inRegisters.getTotalElements());
}
private Specification createSpecification(String registerBatchId, String staffId) {
return (root, criteriaQuery, criteriaBuilder) -> {
Predicate predicate1 = criteriaBuilder.equal(root.get("status"), "2");
Predicate predicate2 = criteriaBuilder.equal(root.get("rebatchid"), registerBatchId);
Predicate predicate3 = criteriaBuilder.equal(root.get("staffid"), staffId);
return criteriaBuilder.and(predicate1, predicate2, predicate3);
};
}
@Override
public InRegister getInRegisterId(String id) {
return inRegisterRepository.findByStuidAndStatus(id, "0");
}
@Override
public void saveStuRegister(Date arrivetime, String inRegisterId) {
Optional<InRegister> register = inRegisterRepository.findById(inRegisterId);
if (register.isPresent()) {
InRegister inRegister = register.get();
inRegister.setArrivetime(arrivetime);
inRegister.setStatus("1");
inRegisterRepository.save(inRegister);
}
}
@Override
public Boolean isStuSaveRegister(String stuId) {
InRegister register = inRegisterRepository.findByStuidAnd
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该项目为基于Java实现的宿舍管理系统,源码包含722个文件,涵盖508个JavaScript文件、63个CSS文件、59个Java源文件、26个JSP文件、12个PNG图片文件、7个HTML文件、6个XML配置文件、6个Map文件、3个Markdown文件、3个Less文件,旨在提供全面的功能和用户界面设计。
资源推荐
资源详情
资源评论
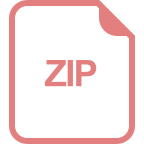
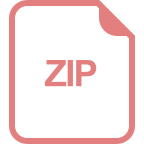
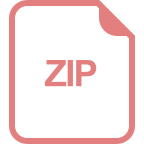
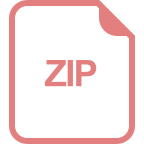
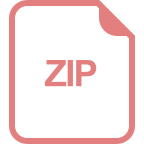
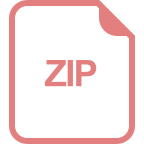
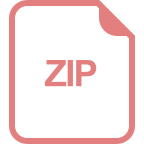
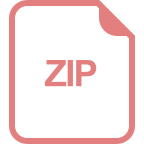
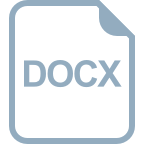
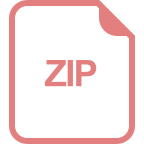
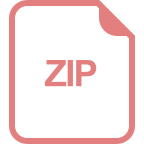
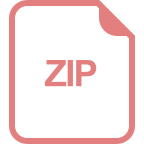
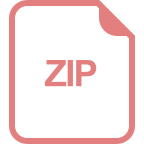
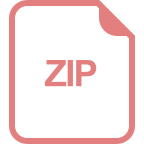
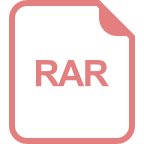
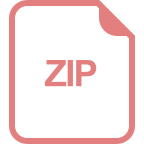
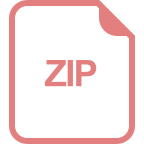
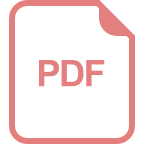
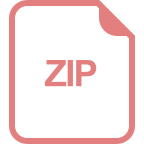
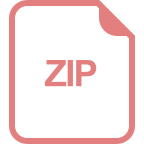
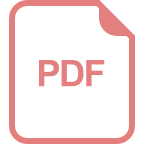
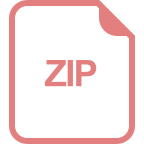
收起资源包目录

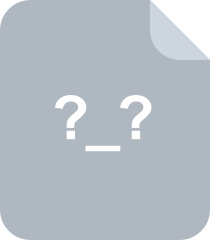
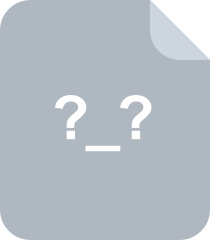
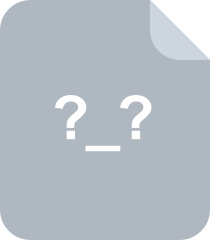
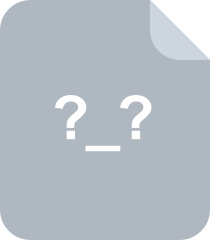
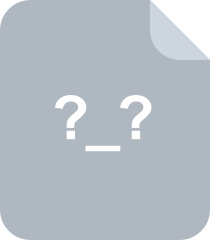
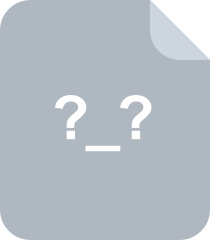
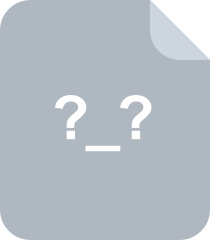
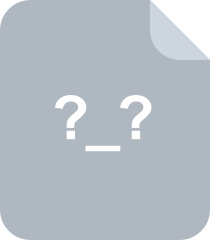
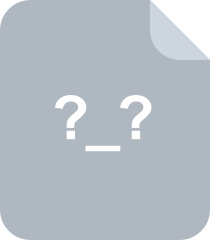
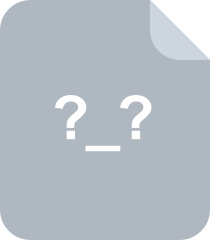
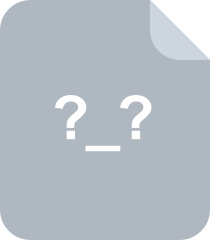
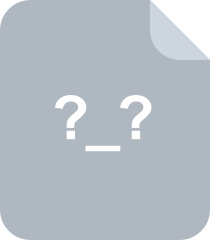
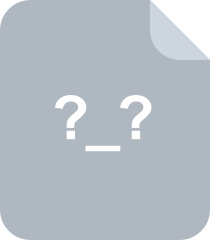
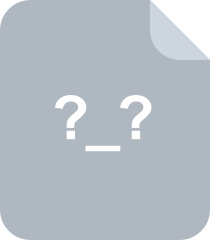
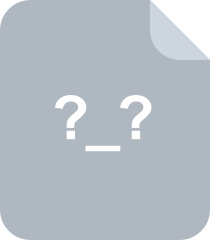
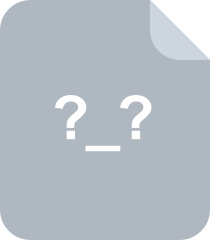
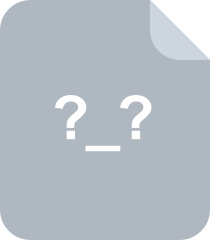
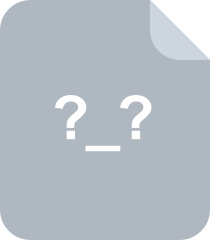
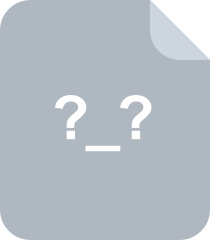
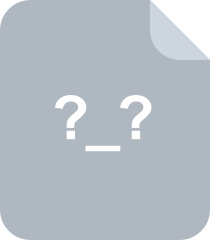
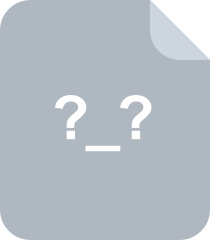
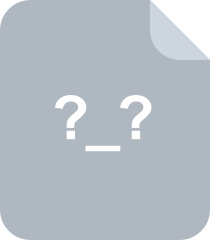
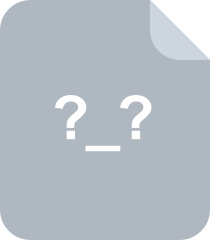
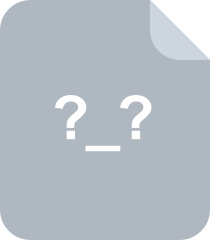
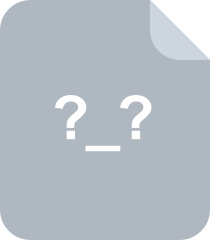
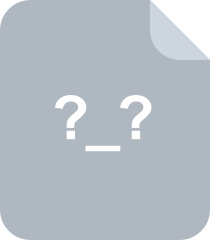
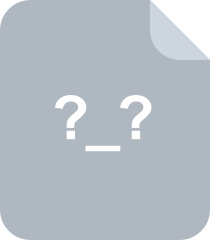
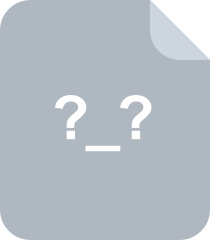
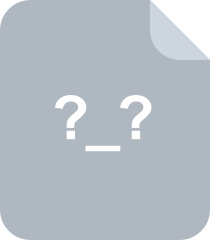
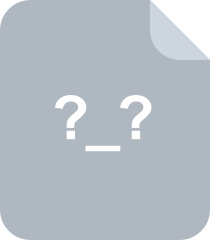
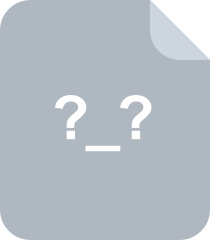
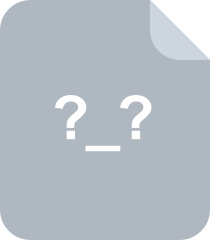
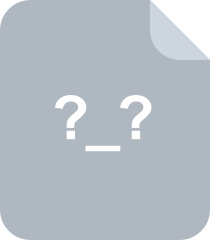
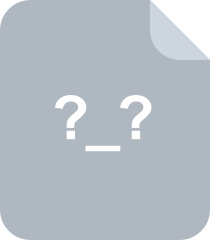
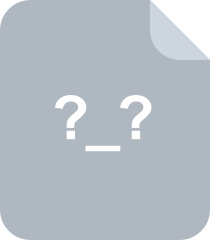
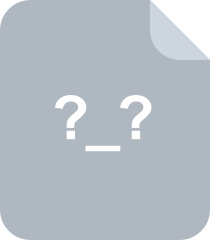
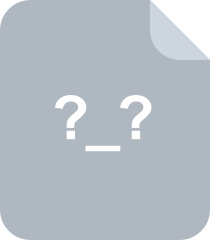
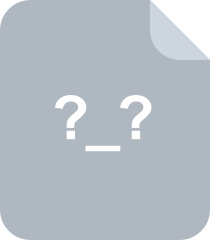
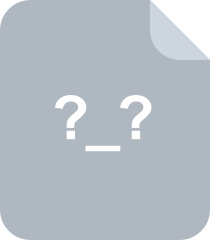
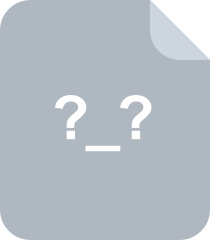
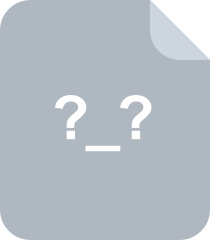
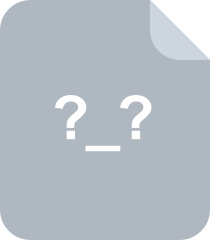
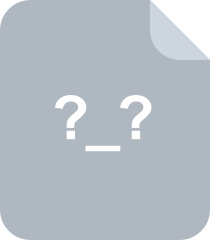
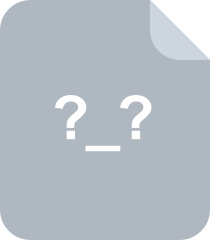
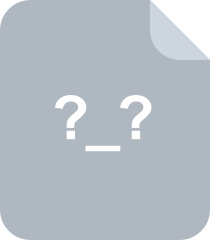
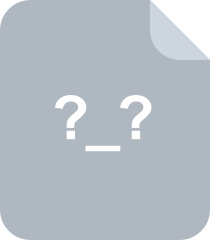
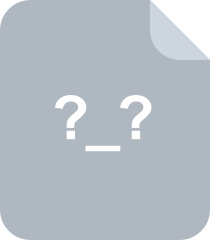
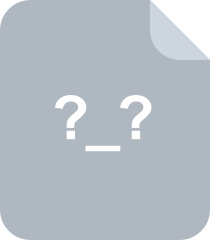
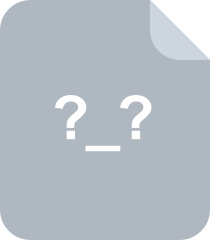
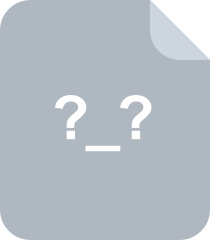
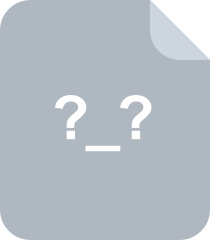
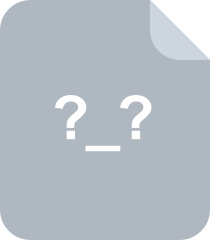
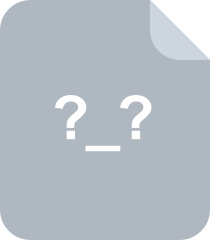
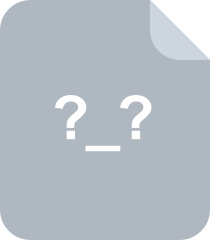
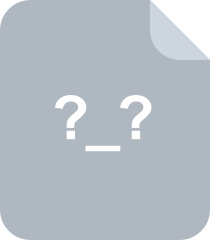
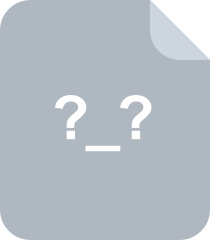
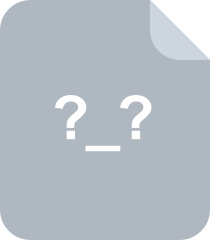
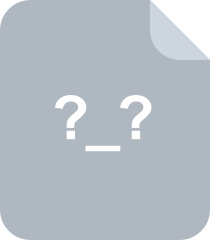
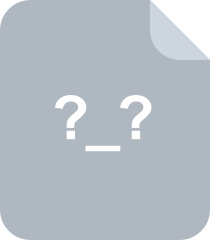
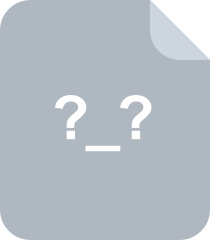
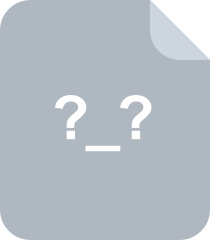
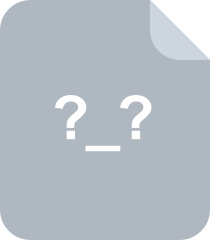
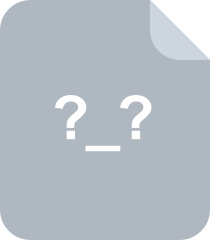
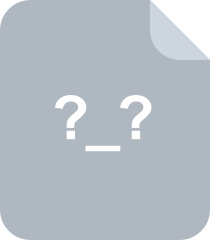
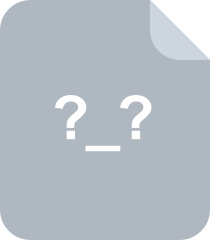
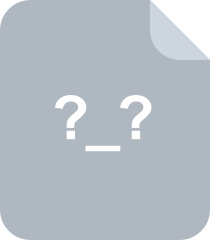
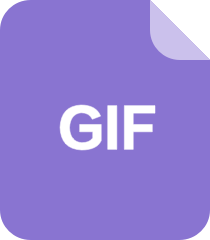
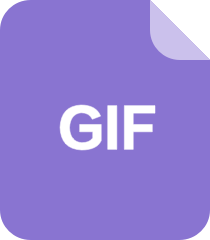
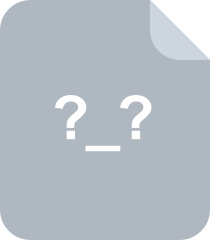
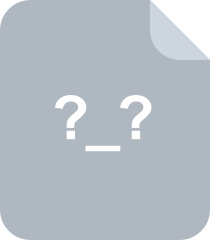
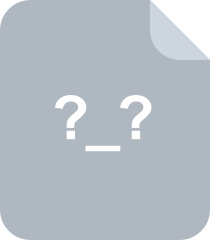
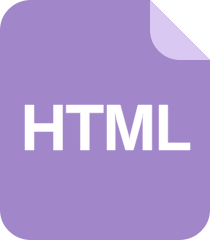
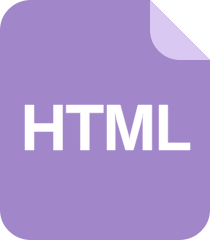
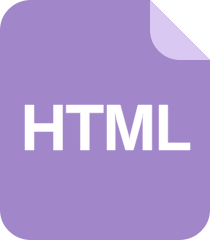
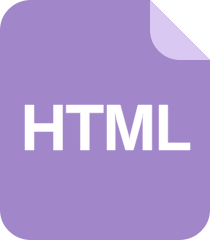
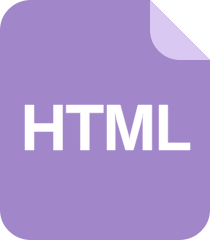
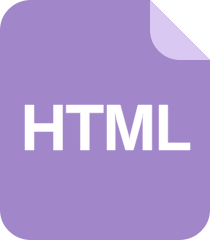
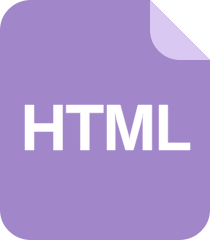
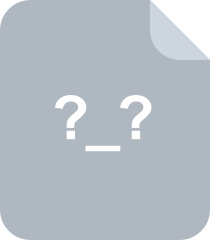
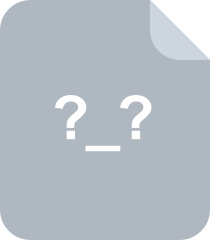
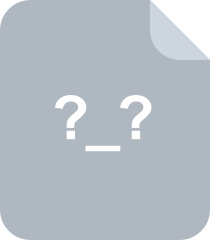
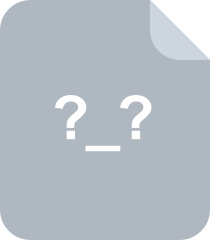
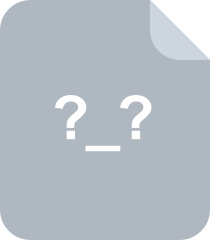
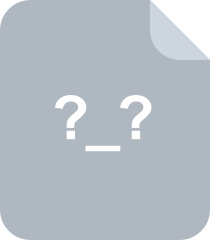
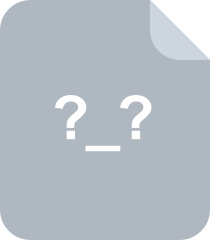
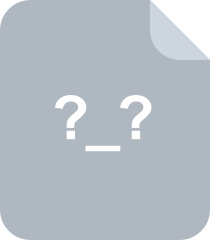
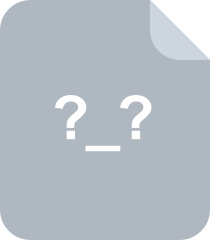
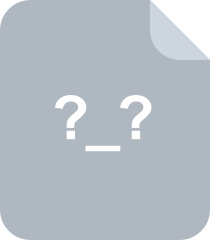
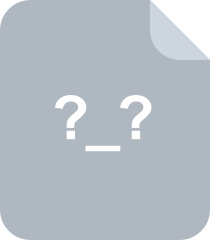
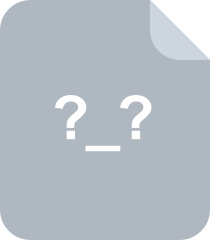
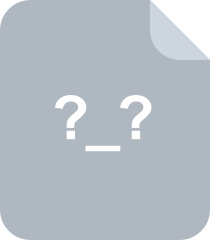
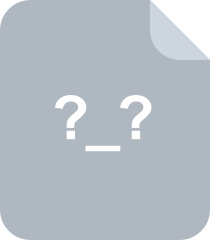
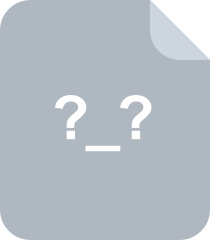
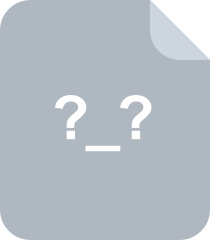
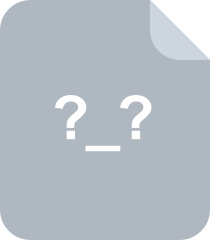
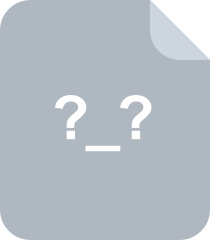
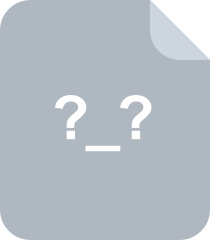
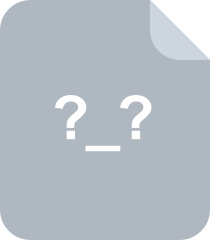
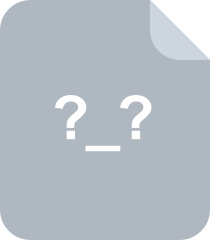
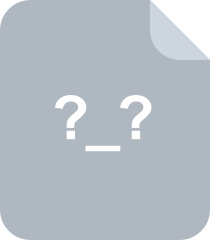
共 720 条
- 1
- 2
- 3
- 4
- 5
- 6
- 8
资源评论
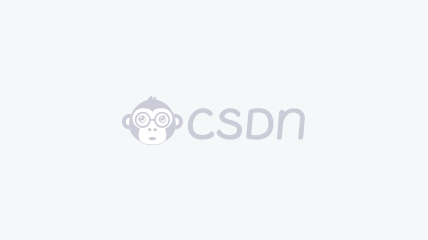

lsx202406
- 粉丝: 2584
- 资源: 5607
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

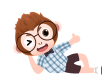
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


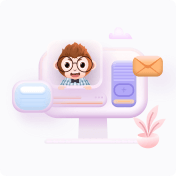
安全验证
文档复制为VIP权益,开通VIP直接复制
