// Copyright 2017 The Chromium Authors. All rights reserved.
// Use of this source code is governed by a BSD-style license that can be
// found in the LICENSE file.
// This file has been automatically generated. Please do not edit it manually.
// To regenerate the file, use:
// dart dev/tools/gen_localizations.dart --overwrite
import 'dart:collection';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:intl/intl.dart' as intl;
import '../material_localizations.dart';
// The classes defined here encode all of the translations found in the
// `flutter_localizations/lib/src/l10n/*.arb` files.
//
// These classes are constructed by the [getTranslation] method at the bottom of
// this file, and used by the [_MaterialLocalizationsDelegate.load] method defined
// in `flutter_localizations/lib/src/material_localizations.dart`.
/// The translations for Arabic (`ar`).
class MaterialLocalizationAr extends GlobalMaterialLocalizations {
/// Create an instance of the translation bundle for Arabic.
///
/// For details on the meaning of the arguments, see [GlobalMaterialLocalizations].
const MaterialLocalizationAr({
String localeName = 'ar',
@required intl.DateFormat fullYearFormat,
@required intl.DateFormat mediumDateFormat,
@required intl.DateFormat longDateFormat,
@required intl.DateFormat yearMonthFormat,
@required intl.NumberFormat decimalFormat,
@required intl.NumberFormat twoDigitZeroPaddedFormat,
}) : super(
localeName: localeName,
fullYearFormat: fullYearFormat,
mediumDateFormat: mediumDateFormat,
longDateFormat: longDateFormat,
yearMonthFormat: yearMonthFormat,
decimalFormat: decimalFormat,
twoDigitZeroPaddedFormat: twoDigitZeroPaddedFormat,
);
@override
String get aboutListTileTitleRaw => r'حول "$applicationName"';
@override
String get alertDialogLabel => r'تنبيه';
@override
String get anteMeridiemAbbreviation => r'ص';
@override
String get backButtonTooltip => r'رجوع';
@override
String get cancelButtonLabel => r'إلغاء';
@override
String get closeButtonLabel => r'إغلاق';
@override
String get closeButtonTooltip => r'إغلاق';
@override
String get collapsedIconTapHint => r'توسيع';
@override
String get continueButtonLabel => r'متابعة';
@override
String get copyButtonLabel => r'نسخ';
@override
String get cutButtonLabel => r'قص';
@override
String get deleteButtonTooltip => r'حذف';
@override
String get dialogLabel => r'مربع حوار';
@override
String get drawerLabel => r'قائمة تنقل';
@override
String get expandedIconTapHint => r'تصغير';
@override
String get hideAccountsLabel => r'إخفاء الحسابات';
@override
String get licensesPageTitle => r'التراخيص';
@override
String get modalBarrierDismissLabel => r'رفض';
@override
String get nextMonthTooltip => r'الشهر التالي';
@override
String get nextPageTooltip => r'الصفحة التالية';
@override
String get okButtonLabel => r'حسنًا';
@override
String get openAppDrawerTooltip => r'فتح قائمة التنقل';
@override
String get pageRowsInfoTitleRaw => r'من $firstRow إلى $lastRow من إجمالي $rowCount';
@override
String get pageRowsInfoTitleApproximateRaw => r'من $firstRow إلى $lastRow من إجمالي $rowCount تقريبًا';
@override
String get pasteButtonLabel => r'لصق';
@override
String get popupMenuLabel => r'قائمة منبثقة';
@override
String get postMeridiemAbbreviation => r'م';
@override
String get previousMonthTooltip => r'الشهر السابق';
@override
String get previousPageTooltip => r'الصفحة السابقة';
@override
String get refreshIndicatorSemanticLabel => r'TBD';
@override
String get remainingTextFieldCharacterCountFew => r'$remainingCount أحرف متبقية';
@override
String get remainingTextFieldCharacterCountMany => r'$remainingCount حرفًا متبقيًا';
@override
String get remainingTextFieldCharacterCountOne => r'حرف واحد متبقٍ';
@override
String get remainingTextFieldCharacterCountOther => r'$remainingCount حرف متبقٍ';
@override
String get remainingTextFieldCharacterCountTwo => r'حرفان ($remainingCount) متبقيان';
@override
String get remainingTextFieldCharacterCountZero => r'لا أحرف متبقية';
@override
String get reorderItemDown => r'نقل لأسفل';
@override
String get reorderItemLeft => r'نقل لليمين';
@override
String get reorderItemRight => r'نقل لليسار';
@override
String get reorderItemToEnd => r'نقل إلى نهاية القائمة';
@override
String get reorderItemToStart => r'نقل إلى بداية القائمة';
@override
String get reorderItemUp => r'نقل لأعلى';
@override
String get rowsPerPageTitle => r'عدد الصفوف في الصفحة:';
@override
ScriptCategory get scriptCategory => ScriptCategory.tall;
@override
String get searchFieldLabel => r'بحث';
@override
String get selectAllButtonLabel => r'اختيار الكل';
@override
String get selectedRowCountTitleFew => r'تم اختيار $selectedRowCount عنصر';
@override
String get selectedRowCountTitleMany => r'تم اختيار $selectedRowCount عنصرًا';
@override
String get selectedRowCountTitleOne => r'تم اختيار عنصر واحد';
@override
String get selectedRowCountTitleOther => r'تم اختيار $selectedRowCount عنصر';
@override
String get selectedRowCountTitleTwo => r'تم اختيار عنصرين ($selectedRowCount)';
@override
String get selectedRowCountTitleZero => r'لم يتم اختيار أي عنصر';
@override
String get showAccountsLabel => r'إظهار الحسابات';
@override
String get showMenuTooltip => r'عرض القائمة';
@override
String get signedInLabel => r'تم تسجيل الدخول';
@override
String get tabLabelRaw => r'علامة التبويب $tabIndex من $tabCount';
@override
TimeOfDayFormat get timeOfDayFormatRaw => TimeOfDayFormat.h_colon_mm_space_a;
@override
String get timePickerHourModeAnnouncement => r'اختيار الساعات';
@override
String get timePickerMinuteModeAnnouncement => r'اختيار الدقائق';
@override
String get viewLicensesButtonLabel => r'الاطّلاع على التراخيص';
}
/// The translations for Bulgarian (`bg`).
class MaterialLocalizationBg extends GlobalMaterialLocalizations {
/// Create an instance of the translation bundle for Bulgarian.
///
/// For details on the meaning of the arguments, see [GlobalMaterialLocalizations].
const MaterialLocalizationBg({
String localeName = 'bg',
@required intl.DateFormat fullYearFormat,
@required intl.DateFormat mediumDateFormat,
@required intl.DateFormat longDateFormat,
@required intl.DateFormat yearMonthFormat,
@required intl.NumberFormat decimalFormat,
@required intl.NumberFormat twoDigitZeroPaddedFormat,
}) : super(
localeName: localeName,
fullYearFormat: fullYearFormat,
mediumDateFormat: mediumDateFormat,
longDateFormat: longDateFormat,
yearMonthFormat: yearMonthFormat,
decimalFormat: decimalFormat,
twoDigitZeroPaddedFormat: twoDigitZeroPaddedFormat,
);
@override
String get aboutListTileTitleRaw => r'Всичко за $applicationName';
@override
String get alertDialogLabel => r'Сигнал';
@override
String get anteMeridiemAbbreviation => r'AM';
@override
String get backButtonTooltip => r'Назад';
@override
String get cancelButtonLabel => r'ОТКАЗ';
@override
String get closeButtonLabel => r'ЗАТВАРЯНЕ';
@override
String get closeButtonTooltip => r'Затваряне';
@override
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目是一款利用Flutter框架构建的移动应用快速开发设计源码,涵盖2906个文件,包括1659个Dart代码文件、272个PNG图片资源、152个模板文件等,支持多种编程语言,如MATLAB、Java、C等。该项目致力于简化移动应用开发过程,提高开发效率。
资源推荐
资源详情
资源评论
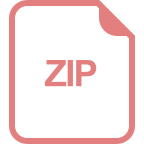
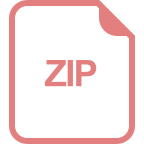
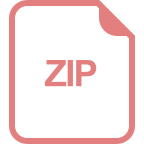
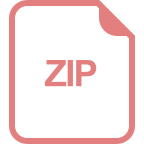
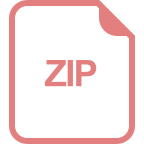
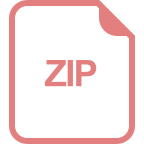
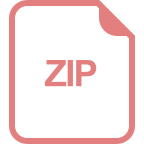
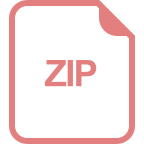
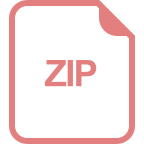
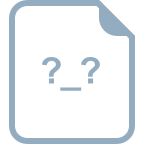
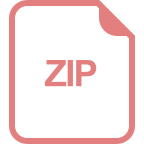
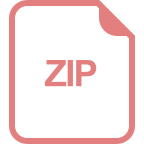
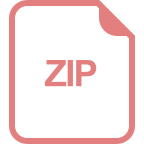
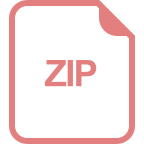
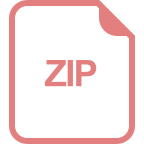
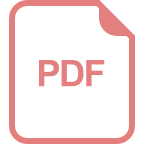
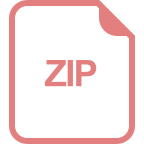
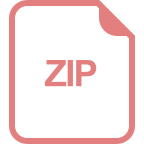
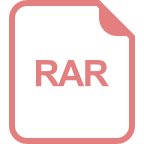
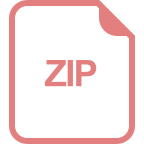
收起资源包目录

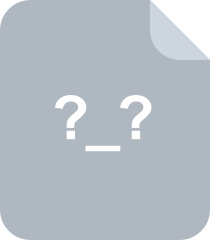
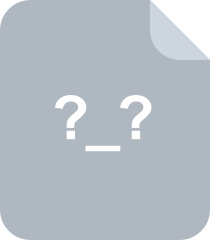
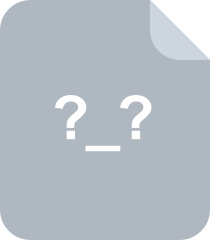
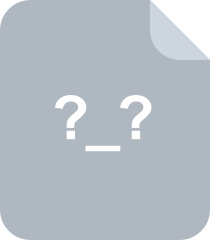
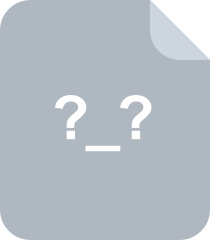
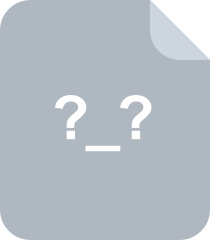
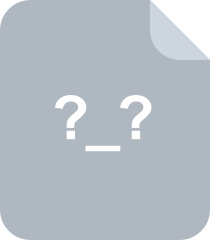
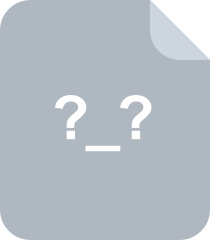
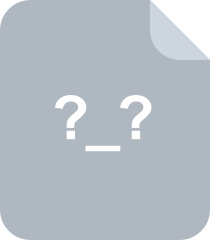
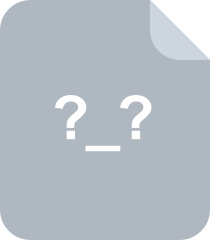
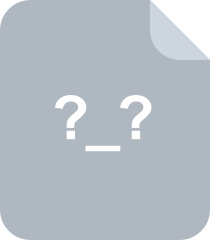
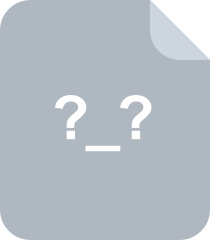
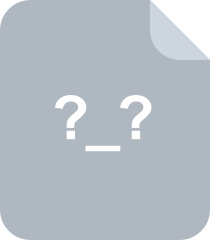
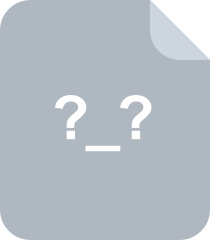
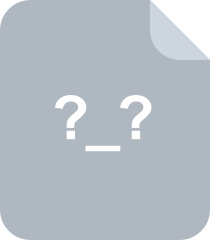
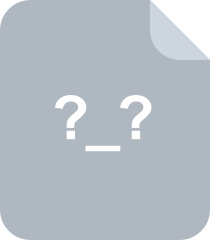
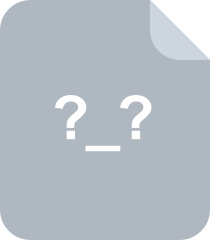
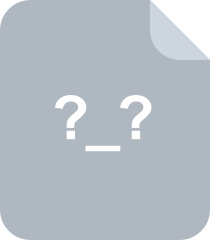
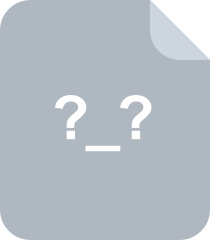
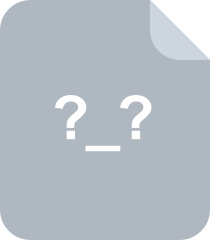
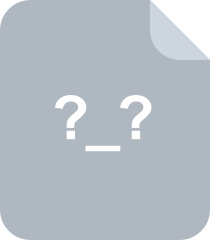
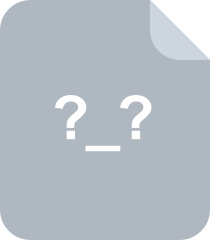
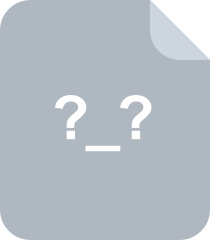
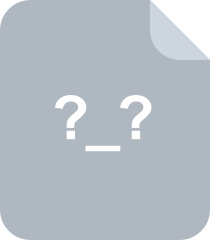
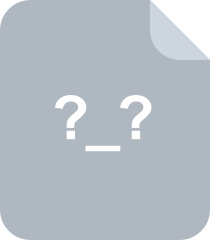
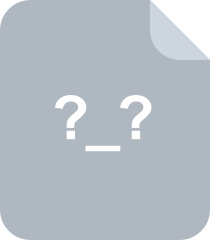
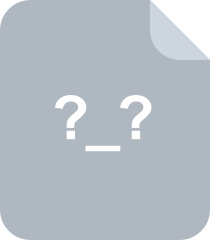
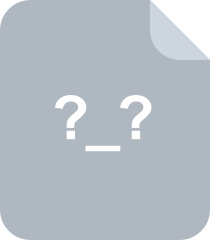
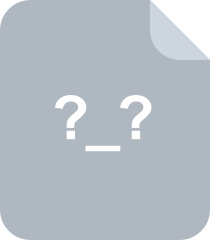
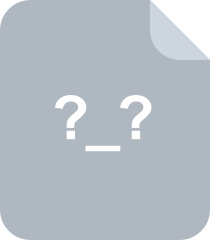
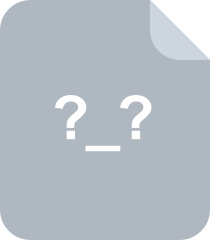
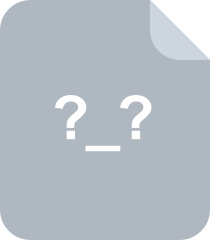
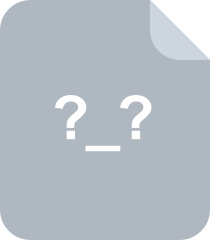
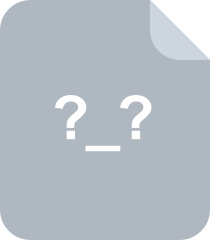
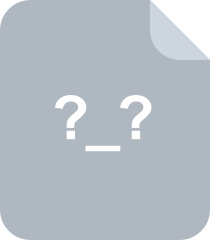
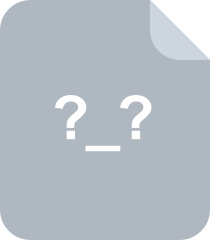
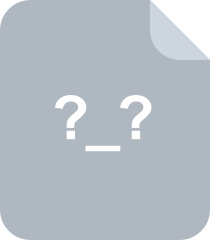
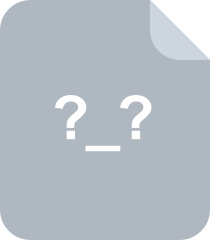
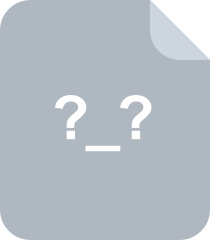
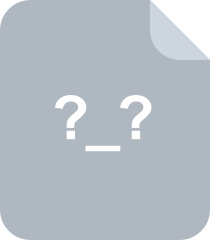
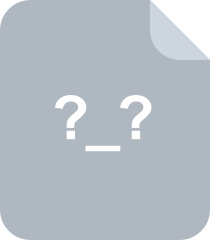
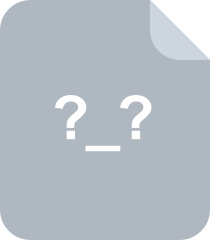
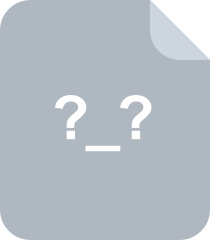
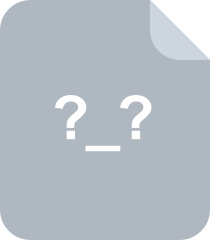
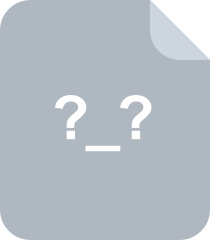
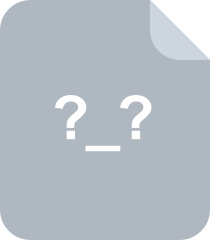
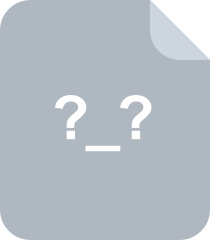
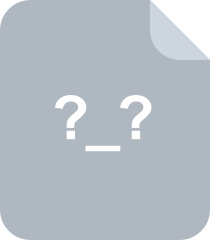
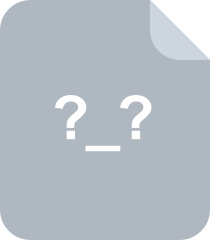
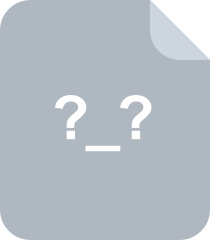
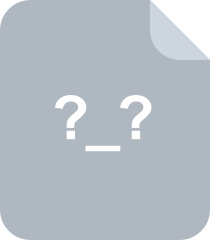
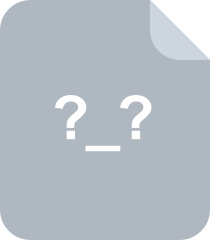
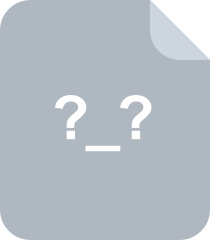
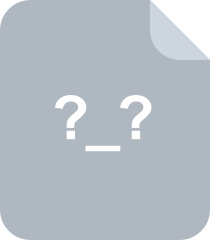
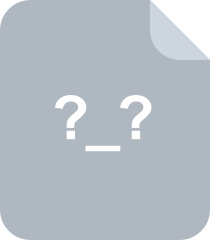
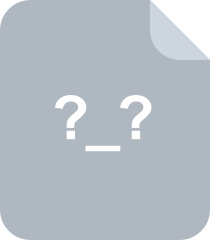
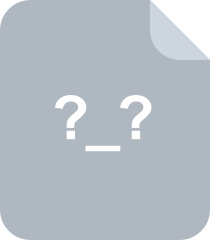
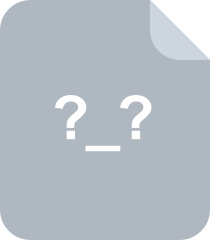
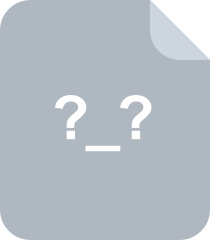
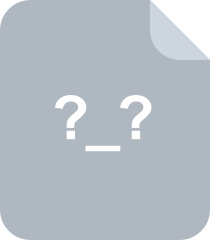
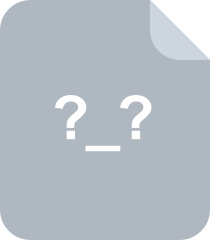
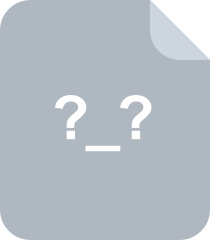
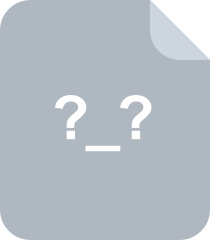
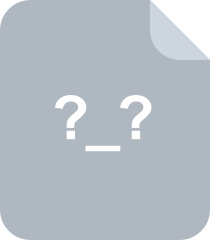
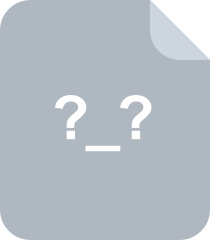
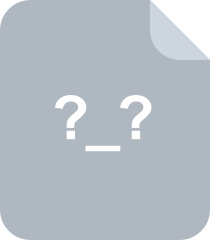
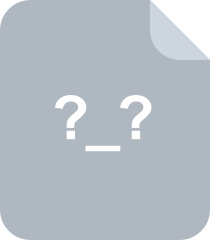
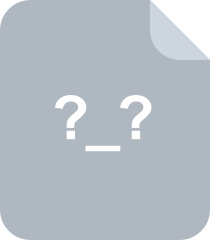
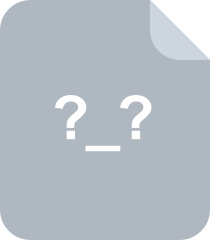
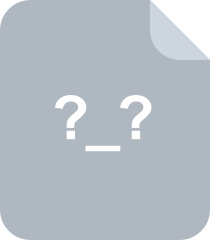
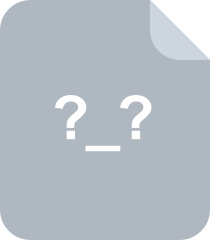
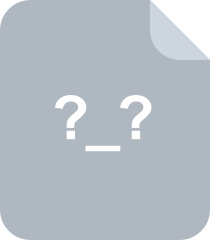
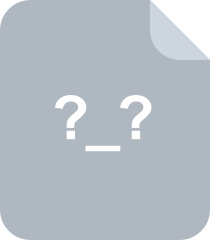
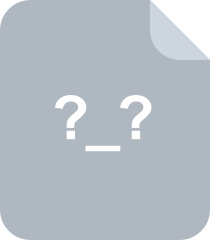
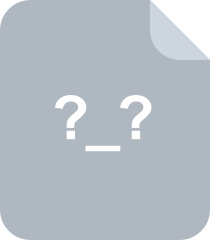
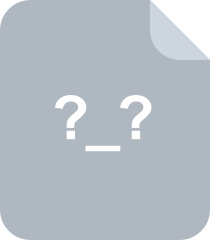
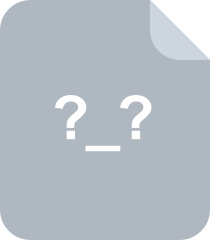
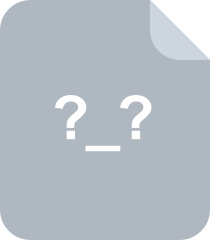
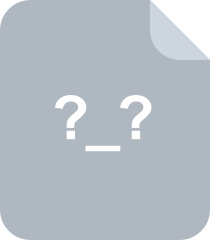
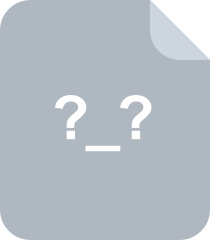
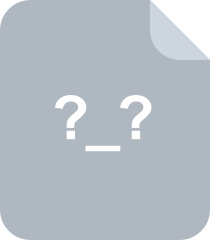
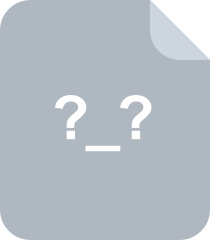
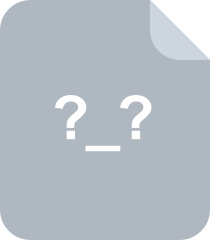
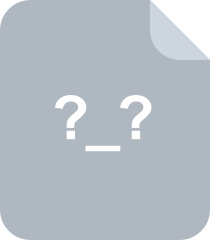
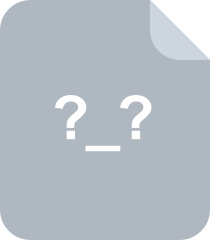
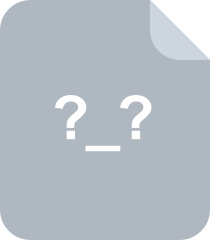
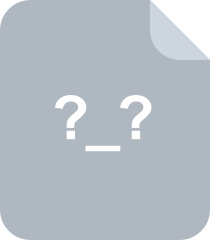
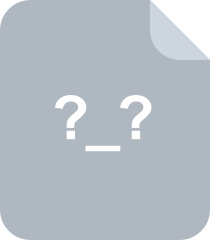
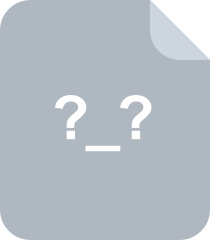
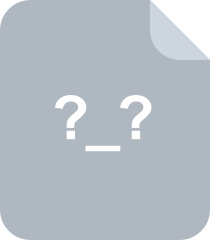
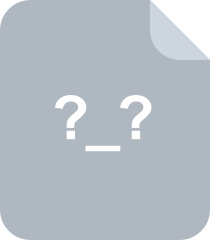
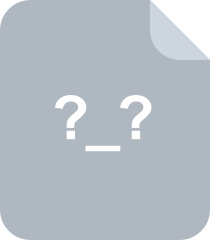
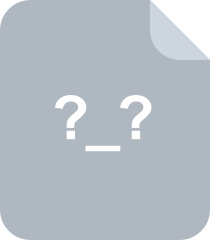
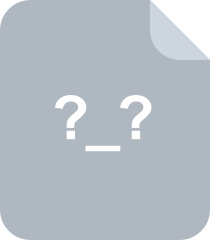
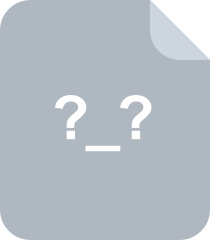
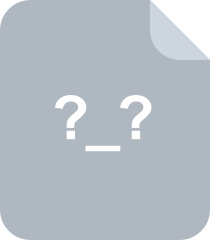
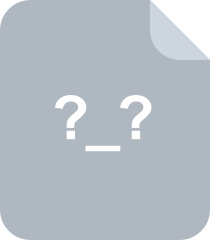
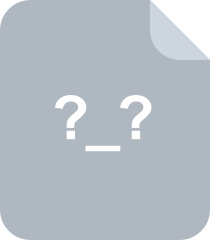
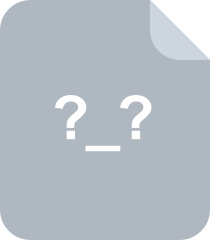
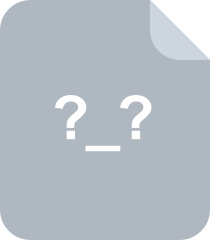
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
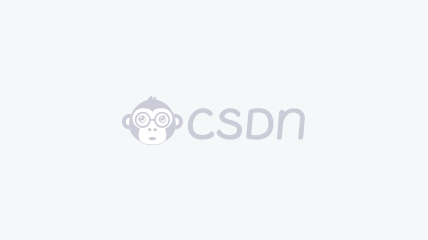

lsx202406
- 粉丝: 2452
- 资源: 5591
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

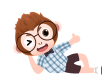
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


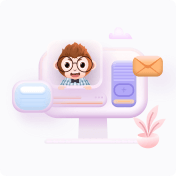
安全验证
文档复制为VIP权益,开通VIP直接复制
