package auto.panel.net.panel;
import androidx.annotation.NonNull;
import com.google.gson.JsonArray;
import com.google.gson.JsonObject;
import java.io.IOException;
import java.util.List;
import auto.panel.bean.panel.PanelAccount;
import auto.panel.bean.panel.PanelDependence;
import auto.panel.bean.panel.PanelEnvironment;
import auto.panel.bean.panel.PanelFile;
import auto.panel.bean.panel.PanelLoginLog;
import auto.panel.bean.panel.PanelSystemConfig;
import auto.panel.bean.panel.PanelSystemInfo;
import auto.panel.bean.panel.PanelTask;
import auto.panel.net.RetrofitFactory;
import auto.panel.net.panel.v15.SystemConfigRes;
import auto.panel.utils.TextUnit;
import okhttp3.MediaType;
import okhttp3.RequestBody;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
/**
* @author wsfsp4
* @version 2023.06.29
*/
public class ApiController {
public static void getSystemInfo(@NonNull String baseUrl, @NonNull SystemInfoCallBack callBack) {
Call<SystemInfoRes> call = RetrofitFactory.build(Api.class, baseUrl).getSystemInfo();
call.enqueue(new Callback<SystemInfoRes>() {
@Override
public void onResponse(@NonNull Call<SystemInfoRes> call, @NonNull Response<SystemInfoRes> response) {
SystemInfoRes res = response.body();
if (NetHandler.handleResponse(response.code(), res, callBack)) {
return;
}
PanelSystemInfo system = new PanelSystemInfo();
system.setInitialized(res.getData().isInitialized());
system.setVersion(res.getData().getVersion());
callBack.onSuccess(system);
}
@Override
public void onFailure(@NonNull Call<SystemInfoRes> call, @NonNull Throwable t) {
NetHandler.handleRequestError(call, t, callBack);
}
});
}
public static void initAccount(@NonNull String baseUrl, @NonNull PanelAccount account, @NonNull BaseCallBack callBack) {
JsonObject jsonObject = new JsonObject();
jsonObject.addProperty("username", account.getUsername());
jsonObject.addProperty("password", account.getPassword());
RequestBody requestBody = RequestBody.create(MediaType.parse("application/json"), jsonObject.toString());
Call<BaseRes> call = RetrofitFactory.build(Api.class, baseUrl).initAccount(requestBody);
call.enqueue(new Callback<BaseRes>() {
@Override
public void onResponse(Call<BaseRes> call, Response<BaseRes> response) {
BaseRes res = response.body();
if (NetHandler.handleResponse(response.code(), res, callBack)) {
return;
}
callBack.onSuccess();
}
@Override
public void onFailure(Call<BaseRes> call, Throwable t) {
NetHandler.handleRequestError(call, t, callBack);
}
});
}
public static void login(@NonNull String baseUrl, @NonNull PanelAccount account, @NonNull LoginCallBack callBack) {
JsonObject jsonObject = new JsonObject();
jsonObject.addProperty("username", account.getUsername());
jsonObject.addProperty("password", account.getPassword());
if (account.getCode() != null) {
jsonObject.addProperty("code", account.getCode());
}
RequestBody requestBody = RequestBody.create(MediaType.parse("application/json"), jsonObject.toString());
Call<LoginRes> call;
if (account.getCode() == null) {
call = RetrofitFactory.build(Api.class, baseUrl).login(requestBody);
} else {
call = RetrofitFactory.build(Api.class, baseUrl).twoFactorLogin(requestBody);
}
call.enqueue(new Callback<LoginRes>() {
@Override
public void onResponse(Call<LoginRes> call, Response<LoginRes> response) {
LoginRes res = response.body();
if (NetHandler.handleResponse(response.code(), res, callBack)) {
return;
}
callBack.onSuccess(res.getData().getToken());
}
@Override
public void onFailure(Call<LoginRes> call, Throwable t) {
NetHandler.handleRequestError(call, t, callBack);
}
});
}
public static void checkAccountToken(@NonNull String baseUrl, @NonNull String token, @NonNull BaseCallBack callBack) {
Call<SystemConfigRes> call = RetrofitFactory.build(Api.class, baseUrl).checkToken(token);
call.enqueue(new Callback<SystemConfigRes>() {
@Override
public void onResponse(Call<SystemConfigRes> call, Response<SystemConfigRes> response) {
SystemConfigRes res = response.body();
if (NetHandler.handleResponse(response.code(), res, callBack)) {
return;
}
callBack.onSuccess();
}
@Override
public void onFailure(Call<SystemConfigRes> call, Throwable t) {
NetHandler.handleRequestError(call, t, callBack);
}
});
}
public static void asyncLogin(@NonNull PanelAccount account, @NonNull AsyncLoginCallback callBack) {
try {
boolean flag = false;
//系统信息
Call<SystemInfoRes> call = RetrofitFactory.build(Api.class, account.getBaseUrl()).getSystemInfo();
Response<SystemInfoRes> r1 = call.execute();
PanelSystemInfo system = new PanelSystemInfo();
system.setInitialized(r1.body().getData().isInitialized());
system.setVersion(r1.body().getData().getVersion());
flag = callBack.onSystemInfo(system);
if (!flag) {
return;
}
//登录
} catch (Exception e) {
e.printStackTrace();
callBack.onFailure(e.toString());
}
}
public static void getTasks(String searchValue, int pageNo, int pageSize, TaskListCallBack callback) {
auto.panel.net.panel.v15.ApiController.getTasks(searchValue, pageNo, pageSize, callback);
}
public static void runTasks(List<Object> keys, BaseCallBack callBack) {
RequestBody body = buildArrayJson(keys);
Call<BaseRes> call = RetrofitFactory.buildWithAuthorization(Api.class).runTasks(body);
call.enqueue(new Callback<BaseRes>() {
@Override
public void onResponse(@NonNull Call<BaseRes> call, @NonNull Response<BaseRes> response) {
BaseRes res = response.body();
if (NetHandler.handleResponse(response.code(), res, callBack)) {
return;
}
callBack.onSuccess();
}
@Override
public void onFailure(@NonNull Call<BaseRes> call, @NonNull Throwable t) {
NetHandler.handleRequestError(call, t, callBack);
}
});
}
public static void stopTasks(List<Object> keys, BaseCallBack callBack) {
RequestBody body = buildArrayJson(keys);
Call<BaseRes> call = RetrofitFactory.buildWithAuthorization(Api.class).stopTasks(body);
call.enqueue(new Callback<BaseRes>() {
@Override
public void onResponse(@NonNull Call<BaseRes> call, @NonNull Response<BaseRes> response) {
BaseRes res = response.body();
if (NetHandler.handleResponse(response.code(), res, callBack)) {
return;
}
callBack.onSuccess();
}
@Override
public void onFailure(@NonNull Call<BaseRes> call, @NonNull Throwable t) {
NetHandler.handleRequestError(call, t, callBack);
}
});
}
public static void enableTasks(List<Object> keys, BaseCallBack callBack)

lsx202406
- 粉丝: 2821
- 资源: 5666
最新资源
- 毕设和企业适用springboot企业数据智能分析平台类及智能农场管理系统源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及智能农业解决方案源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及数字图书馆平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及网络营销平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及个性化广告平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及产品溯源系统源码+论文+视频.zip
- 毕设和企业适用springboot企业数据智能分析平台类及资源调度平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及团队协作平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及无人驾驶系统源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及业务流程自动化平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及销售管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及客户关系管理平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及共享经济平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及客户服务平台源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及跨平台销售系统源码+论文+视频.zip
- 毕设和企业适用springboot企业协作平台类及平台生态系统源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


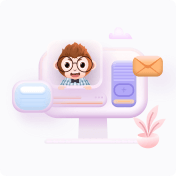