<h2>Full example</h2>
<pre><code>#!/usr/bin/env qore
# database test script
# databases users must be able to create and destroy tables and procedures, etc
# in order to execute all tests
%require-our
%enable-all-warnings
our ($o, $errors, $test_count);
const opts =
( "help" : "h,help",
"host" : "H,host=s",
"pass" : "p,pass=s",
"db" : "d,db=s",
"user" : "u,user=s",
"type" : "t,type=s",
"enc" : "e,encoding=s",
"verbose" : "v,verbose:i+",
"leave" : "l,leave"
);
sub usage()
{
printf("usage: %s [options]
-h,--help this help text
-u,--user=ARG set username
-p,--pass=ARG set password
-d,--db=ARG set database name
-e,--encoding=ARG set database character set encoding (i.e. \"utf8\")
-H,--host=ARG set hostname (for MySQL and PostgreSQL connections)
-t,--type set database driver (default mysql)
-v,--verbose more v's = more information
-l,--leave leave test tables in schema at end\n",
basename($ENV."_"));
exit();
}
const object_map =
( "oracle" :
( "tables" : ora_tables ),
"mysql" :
( "tables" : mysql_tables ),
"pgsql" :
( "tables" : pgsql_tables ),
"sybase" :
( "tables" : syb_tables,
"procs" : sybase_procs ),
"freetds" :
( "tables" : freetds_sybase_tables,
"procs" : sybase_procs ) );
const ora_tables = (
"family" : "create table family (
family_id int not null,
name varchar2(80) not null
)",
"people" : "create table people (
person_id int not null,
family_id int not null,
name varchar2(250) not null,
dob date not null
)",
"attributes" : "create table attributes (
person_id int not null,
attribute varchar2(80) not null,
value varchar2(160) not null
)" );
const mysql_tables = (
"family" : "create table family (
family_id int not null,
name varchar(80) not null
) type = innodb",
"people" : "create table people (
person_id int not null,
family_id int not null,
name varchar(250) not null,
dob date not null
) type = innodb",
"attributes" : "create table attributes (
person_id int not null,
attribute varchar(80) not null,
value varchar(160) not null
) type = innodb" );
const pgsql_tables = (
"family" : "create table family (
family_id int not null,
name varchar(80) not null )",
"people" : "create table people (
person_id int not null,
family_id int not null,
name varchar(250) not null,
dob date not null )",
"attributes" : "create table attributes (
person_id int not null,
attribute varchar(80) not null,
value varchar(160) not null)",
"data_test" : "create table data_test (
int2_f smallint not null,
int4_f integer not null,
int8_f int8 not null,
bool_f boolean not null,
float4_f real not null,
float8_f double precision not null,
number_f numeric(16,3) not null,
money_f money not null,
text_f text not null,
varchar_f varchar(40) not null,
char_f char(40) not null,
name_f name not null,
date_f date not null,
abstime_f abstime not null,
reltime_f reltime not null,
interval_f interval not null,
time_f time not null,
timetz_f time with time zone not null,
timestamp_f timestamp not null,
timestamptz_f timestamp with time zone not null,
tinterval_f tinterval not null,
bytea_f bytea not null
--bit_f bit(11) not null,
--varbit_f bit varying(11) not null
)" );
const syb_tables = (
"family" : "create table family (
family_id int not null,
name varchar(80) not null
)",
"people" : "create table people (
person_id int not null,
family_id int not null,
name varchar(250) not null,
dob date not null
)",
"attributes" : "create table attributes (
person_id int not null,
attribute varchar(80) not null,
value varchar(160) not null
)",
"data_test" : "create table data_test (
null_f char(1) null,
varchar_f varchar(40) not null,
char_f char(40) not null,
unichar_f unichar(40) not null,
univarchar_f univarchar(40) not null,
text_f text not null,
unitext_f unitext not null, -- note that unitext is stored as 'image'
bit_f bit not null,
tinyint_f tinyint not null,
smallint_f smallint not null,
int_f int not null,
int_f2 int not null,
decimal_f decimal(10,4) not null,
float_f float not null, -- 8-bytes
real_f real not null, -- 4-bytes
money_f money not null,
smallmoney_f smallmoney not null,
date_f date not null,
time_f time not null,
datetime_f datetime not null,
smalldatetime_f smalldatetime not null,
binary_f binary(4) not null,
varbinary_f varbinary(4) not null,
image_f image not null
)" );
const sybase_procs = (
"find_family" :
"create procedure find_family @name varchar(80)
as
select * from family where name = @name
commit -- to maintain transaction count
",
"get_values" :
"create procedure get_values @string varchar(80) output, @int int output
as
select @string = 'hello there'
select @int = 150
commit -- to maintain transaction count
",
"get_values_and_select" :
"create procedure get_values_and_select @string varchar(80) output, @int int output
as
select @string = 'hello there'
select @int = 150
select * from family where family_id = 1
commit -- to maintain transaction count
",
"get_values_and_multiple_select" :
"create procedure get_values_and_multiple_select @string varchar(80) output, @int int output
as
select @string = 'hello there'
select @int = 150
select * from family where family_id = 1
select * from people where person_id = 1
commit -- to maintain transaction count
",
"just_select" :
"create procedure just_select
as
select * from family where family_id = 1
commit -- to maintain transaction count
",
"multiple_select" :
"create procedure multiple_select
as
select * from family where family_id = 1
select * from people where person_id = 1
commit -- to maintain transaction count
"
);
const freetds_sybase_tables = (
"family" : "create table family (
family_id int not null,
name varchar(80) not null
)",
"people" : "create table people (
person_id int not null,
family_id int not null,
name varchar(250) not null,
dob date not null
)",
"attributes" : "create table attributes (
person_id int not null,
attribute varchar(80) not null,
value varchar(160) not null
)",
"data_test" : "create table data_test (
null_f char(1) null,
varchar_f varchar(40) not null,
char_f char(40) not null,
text_f text not null,
unitext_f unitext not null, -- note that unitext is stored as 'image'
bit_f bit not null,
tinyint_f tinyint not null,
smallint_f smallint not null,
int_f int not null,
int_f2 int not null,
decimal_f decimal(10,4) not null,
float_f float not null, -- 8-bytes
real_f real not null, -- 4-bytes
money_f money not null,
smallmoney_f smallmoney not null,
date_f date not null,
time_f time not null,
datetime_f datetime not null,
smalldatetime_f smalldatetime not null,
binary_f binary(4) not null,
varbinary_f varbinary(4) not null,
image_f image not null
)" );
const freetds_mssql_tables = (
"family" : "create table family (
family_id int not null,
name varchar(80) not null
)",
"people" : "create table people (
person_id int not null,
family_id int not null,
name varchar(250) not null,
dob datetime not null
)",
"attributes" : "create table attributes (
person_id int not null,
attribute varchar(80) not null,
value varchar(160) not null
)",
"data_test" : "create table data_test (
null_f char(1) null,
varchar_f varchar(40) not null,
char_f char(40) not null,
text_f text not null,
bit_f bit not null,
tinyint_f tinyint not null,
smallint_f smallint not null,
int_f int not null,
int_f2 int not null,
decimal_f decimal(10,4) not null,
float_f float not null, -- 8-bytes
real_f real not null, -- 4-bytes
money_f money not null,
smallmoney_f smallmoney not null,
datetime_f datetime not null,
smalldatetime_f smalldatetime not null,
binary_f binary(4) not null,
varbinary_f varbinary(4) not nul

lsx202406
- 粉丝: 2925
- 资源: 5689
最新资源
- 基于Java的民宿管理系统-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 基于html5的民谣网站的设计与实现-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.zip
- 3b020汽车配件销售管理系统_springboot+vue.zip
- 3b022投票系统_springboot+vue.zip
- 3b021投稿和稿件处理系统_springboot+vue.zip
- 3b024校园运动会管理系统_springboot+vue0.zip
- 基于html5的网上团购系统设计与实现-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- APP论坛社区软件源码网站源码APP封装
- 3b023小区疫苗接种管理系统_springboot+vue.zip
- 基于javaEE的校园二手书交易平台的设计与实现-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 基于BS的老年人体检管理系统-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
- 3b025医院挂号系统_springboot+vue.zip
- 3b027自习室座位预约系统_springboot+vue.zip
- 3b028《升学日》日本大学信息及院校推荐网站_springboot+vue0.zip
- 3b026在线学习网站_springboot+vue.zip
- 基于Java的家政服务平台的设计与实现-springboot毕业项目,适合计算机毕-设、实训项目、大作业学习.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


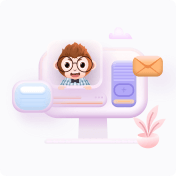