通过MODBUS TCP读写PLC源码

根据提供的文件信息,本文将详细解释如何通过MODBUS TCP协议实现对PLC(可编程逻辑控制器)的读写操作,并具体分析代码中的关键步骤及功能。 ### MODBUS TCP简介 MODBUS TCP是一种广泛应用于工业自动化领域的通信协议,它基于TCP/IP网络协议,允许设备之间进行数据交换。该协议为工业环境下的数据传输提供了高效、稳定的方式,特别是在连接PLC等设备时具有很大的优势。MODBUS TCP简化了传统MODBUS RTU或ASCII版本的报文结构,使得通信过程更为简洁快速。 ### 代码解析 #### 1. 初始化PLC连接 代码中定义了一个`PLCFunction`类,其中包含了一些静态方法用于处理PLC的读写操作。首先来看`init_plc()`方法(虽然在给出的代码片段中未完全展示出来),此方法主要用于初始化与PLC之间的连接。初始化过程可能包括设置TCP/IP参数、创建Socket对象等。 ```csharp public static int init_plc() { // 这里应该是初始化Socket连接的代码 // 创建Socket对象,设置地址和端口等 zdqSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp); zdqSocket.Connect(new IPEndPoint(IPAddress.Parse(plcAddress), port)); // 假设port已经定义 // 返回状态值 return 0; } ``` #### 2. 读取PLC数据 接下来是`ReadWord(int mwAddress)`方法,用于从PLC读取指定内存地址的数据。 - `mwAddress`表示要读取的内存地址。 - 首先调用`init_plc()`确保与PLC的连接已建立。 - 构造发送缓冲区`sendBuf`,并设置命令代码为`0x03`,这对应于MODBUS的功能码“读取保持寄存器”。 - 发送缓冲区到PLC,并等待接收响应。 - 接收响应后,解析返回的缓冲区`recBuf`中的数据,并计算出实际值。 ```csharp public static int ReadWord(int mwAddress) { int rtValue = -99; if (init_plc() == 0) { try { int hi = mwAddress / 256; int low = mwAddress - hi * 256; sendBuf[7] = 3; sendBuf[8] = (byte)hi; sendBuf[9] = (byte)low; sendBuf[10] = 0; sendBuf[11] = 1; // 读取单个寄存器 zdqSocket.Send(sendBuf, 12, 0); Application.DoEvents(); // 更新UI int bytes = zdqSocket.Receive(recBuf, 11, 0); // 接收11字节 if (bytes == 11) { int hi = recBuf[9]; int low = recBuf[10]; rtValue = hi * 256 + low; } trytimes = 0; } catch (Exception te) { if (tiaoshi == "Y") MessageBox.Show(te.ToString()); trytimes++; } } return rtValue; } ``` #### 3. 写入PLC数据 `WriteWord(int mwAddress, int mwValue)`方法用于向PLC写入指定内存地址的数据。 - `mwAddress`表示要写入的内存地址。 - `mwValue`表示要写入的值。 - 类似于读取过程,先调用`init_plc()`确保连接。 - 设置发送缓冲区`sendBuf`,命令代码为`0x06`,对应MODBUS的功能码“写单个寄存器”。 ```csharp public static void WriteWord(int mwAddress, int mwValue) { if (init_plc() == 0) { try { int hi = mwAddress / 256; int low = mwAddress - hi * 256; int hi1 = mwValue / 256; int low1 = mwValue - hi1 * 256; sendBuf[7] = 6; sendBuf[8] = (byte)hi; sendBuf[9] = (byte)low; sendBuf[10] = (byte)hi1; sendBuf[11] = (byte)low1; zdqSocket.Send(sendBuf, 12, 0); Application.DoEvents(); int bytes = zdqSocket.Receive(recBuf, recBuf.Length, 0); trytimes = 0; } catch (Exception te) { if (tiaoshi == "Y") MessageBox.Show(te.ToString()); trytimes++; } } } ``` ### 小结 通过上述分析可以看出,这段代码主要实现了通过MODBUS TCP协议与PLC进行数据交互的功能。它包括了连接初始化、读取PLC寄存器数据以及写入PLC寄存器数据的操作。这些功能在工业自动化控制领域中非常实用,可以方便地实现对PLC设备的远程监控与控制。对于想要深入理解MODBUS TCP协议及其应用的人来说,这段代码提供了一个很好的参考示例。
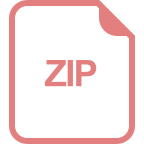
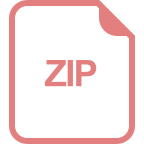
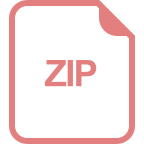
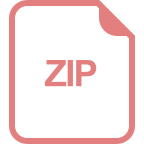
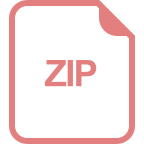
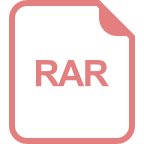
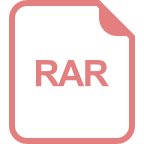
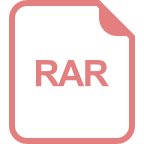
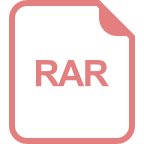
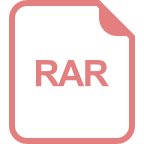
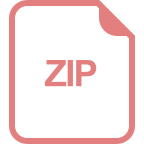
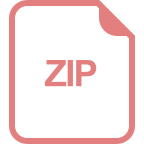
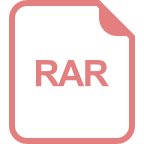
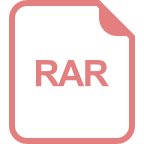
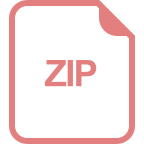
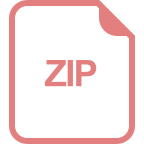
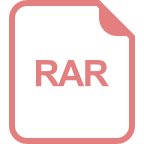
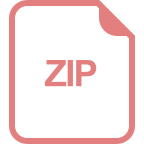
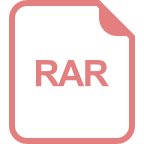
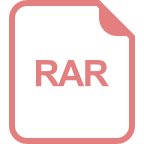
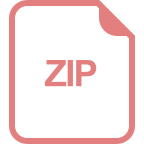
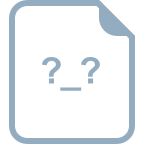
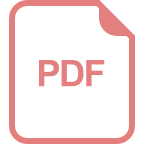
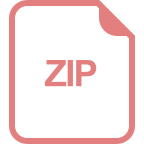
功能模块一:读写PLC主模块
using System;
using System.Net;
using System.Net.Sockets;
using System.Windows.Forms;
/// <summary>
/// 通过modbus TCP读写PLC数据,
///
/// 设计:张东启,2011.4.9
/// 2011.5.13更新,加入Application.DoEvents();以防连续读写时引起前台反应迟顿,同时让PLC有处理等待时间
///
///
///
/// </summary>
public class PLCFunction
{
public static bool Connected = false;
public static int trytimes = 0;
//内部使用变量
private static byte[] sendBuf = { 0, 0, 0, 0, 0, 06, 255, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; //共20个字节
private static byte[] recBuf = { 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0 }; //共20个字节
private static int hi, low, bytes, hi1, low1;
private static Socket zdqSocket;
private static string tiaoshi="N";
private static string plcAddress = "127.0.0.1";
public static int ReadWord(int mwAddress)
{
int rtValue = -99;
if (init_plc() == 0)
{
try
{
hi = mwAddress / 256;
low = mwAddress - hi * 256;
sendBuf[7] = 3;
sendBuf[8] = (byte)hi;
sendBuf[9] = (byte)low;
sendBuf[10] = 0;
sendBuf[11] = 1;
//发送查询
zdqSocket.Send(sendBuf, 12, 0);
Application.DoEvents();
bytes = zdqSocket.Receive(recBuf, 11, 0); //返回11个字节
if (bytes == 11)
{
hi = recBuf[9];
low = recBuf[10];
rtValue = hi * 256 + low;
}
trytimes = 0;
}
剩余7页未读,继续阅读
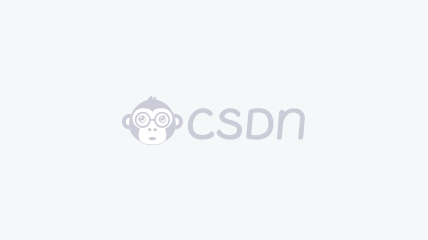
- shlewei2014-07-07程序不完整,不好用。
- LSJ935862014-04-22一般,不完整,不好用。
- delphinewer2014-06-07试了一下,不大好用呀

- 粉丝: 0
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

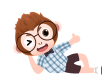
最新资源
- 【岗位说明】行政人事岗位职责.doc
- 【岗位说明】行政人事专员岗位职责.doc
- 【岗位说明】行政人资部部门职责说明书.doc
- 【岗位说明】行政人员岗位职责.doc
- 【岗位说明】行政文员岗位职责.doc
- 【岗位说明】行政文员岗位职责和任职要求.doc
- 【岗位说明】行政助理岗位职责.doc
- 【岗位说明】行政助理.doc
- 【岗位说明】行政中心岗位职责.doc
- 【岗位说明】行政总监岗位职责(全面).doc
- 【岗位说明】后勤组长岗位说明书.doc
- 【岗位说明】行政专员岗位职责说明书.doc
- 【岗位说明】行政总监岗位说明书.doc
- 【岗位说明】绩效考核主管.doc
- 【岗位说明】会计主管岗位说明.doc
- 【岗位说明】化验室主任岗位说明书.doc

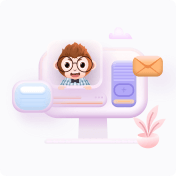
