/* zip.c -- IO on .zip files using zlib
Version 1.1, February 14h, 2010
part of the MiniZip project
Copyright (C) 1998-2010 Gilles Vollant
http://www.winimage.com/zLibDll/minizip.html
Modifications for Zip64 support
Copyright (C) 2009-2010 Mathias Svensson
http://result42.com
Modifications for AES, PKWARE disk spanning
Copyright (C) 2010-2014 Nathan Moinvaziri
This program is distributed under the terms of the same license as zlib.
See the accompanying LICENSE file for the full text of the license.
*/
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include "zlib.h"
#include "zip.h"
#ifdef STDC
# include <stddef.h>
# include <string.h>
# include <stdlib.h>
#endif
#ifdef NO_ERRNO_H
extern int errno;
#else
# include <errno.h>
#endif
#ifdef HAVE_AES
# define AES_METHOD (99)
# define AES_PWVERIFYSIZE (2)
# define AES_AUTHCODESIZE (10)
# define AES_MAXSALTLENGTH (16)
# define AES_VERSION (0x0001)
# define AES_ENCRYPTIONMODE (0x03)
# include "aes.h"
# include "fileenc.h"
# include "prng.h"
# include "entropy.h"
#endif
#ifndef NOCRYPT
# define INCLUDECRYPTINGCODE_IFCRYPTALLOWED
# include "crypt.h"
#endif
#ifndef local
# define local static
#endif
/* compile with -Dlocal if your debugger can't find static symbols */
#define SIZEDATA_INDATABLOCK (4096 - (4 * 4))
#define DISKHEADERMAGIC (0x08074b50)
#define LOCALHEADERMAGIC (0x04034b50)
#define CENTRALHEADERMAGIC (0x02014b50)
#define ENDHEADERMAGIC (0x06054b50)
#define ZIP64ENDHEADERMAGIC (0x06064b50)
#define ZIP64ENDLOCHEADERMAGIC (0x07064b50)
#define FLAG_LOCALHEADER_OFFSET (0x06)
#define CRC_LOCALHEADER_OFFSET (0x0e)
#define SIZECENTRALHEADER (0x2e) /* 46 */
#define SIZECENTRALHEADERLOCATOR (0x14) /* 20 */
#define SIZECENTRALDIRITEM (0x2e)
#define SIZEZIPLOCALHEADER (0x1e)
#ifndef BUFREADCOMMENT
# define BUFREADCOMMENT (0x400)
#endif
#ifndef VERSIONMADEBY
# define VERSIONMADEBY (0x0) /* platform dependent */
#endif
#ifndef Z_BUFSIZE
# define Z_BUFSIZE (64 * 1024)
#endif
#ifndef Z_MAXFILENAMEINZIP
# define Z_MAXFILENAMEINZIP (256)
#endif
#ifndef ALLOC
# define ALLOC(size) (malloc(size))
#endif
#ifndef TRYFREE
# define TRYFREE(p) {if (p) free(p); }
#endif
/* NOT sure that this work on ALL platform */
#define MAKEULONG64(a, b) ((ZPOS64_T)(((unsigned long)(a)) | ((ZPOS64_T)((unsigned long)(b))) << 32))
#ifndef DEF_MEM_LEVEL
# if MAX_MEM_LEVEL >= 8
# define DEF_MEM_LEVEL 8
# else
# define DEF_MEM_LEVEL MAX_MEM_LEVEL
# endif
#endif
const char zip_copyright[] = " zip 1.01 Copyright 1998-2004 Gilles Vollant - http://www.winimage.com/zLibDll";
typedef struct linkedlist_datablock_internal_s {
struct linkedlist_datablock_internal_s *next_datablock;
uLong avail_in_this_block;
uLong filled_in_this_block;
uLong unused; /* for future use and alignment */
unsigned char data[SIZEDATA_INDATABLOCK];
} linkedlist_datablock_internal;
typedef struct linkedlist_data_s {
linkedlist_datablock_internal *first_block;
linkedlist_datablock_internal *last_block;
} linkedlist_data;
typedef struct {
z_stream stream; /* zLib stream structure for inflate */
#ifdef HAVE_BZIP2
bz_stream bstream; /* bzLib stream structure for bziped */
#endif
#ifdef HAVE_AES
fcrypt_ctx aes_ctx;
prng_ctx aes_rng[1];
#endif
int stream_initialised; /* 1 is stream is initialized */
uInt pos_in_buffered_data; /* last written byte in buffered_data */
ZPOS64_T pos_local_header; /* offset of the local header of the file currently writing */
char *central_header; /* central header data for the current file */
uLong size_centralextra;
uLong size_centralheader; /* size of the central header for cur file */
uLong size_centralextrafree; /* Extra bytes allocated to the central header but that are not used */
uLong size_comment;
uLong flag; /* flag of the file currently writing */
int method; /* compression method written to file.*/
int compression_method; /* compression method to use */
int raw; /* 1 for directly writing raw data */
Byte buffered_data[Z_BUFSIZE]; /* buffer contain compressed data to be writ*/
uLong dosDate;
uLong crc32;
int zip64; /* Add ZIP64 extended information in the extra field */
uLong number_disk; /* number of current disk used for spanning ZIP */
ZPOS64_T pos_zip64extrainfo;
ZPOS64_T total_compressed;
ZPOS64_T total_uncompressed;
#ifndef NOCRYPT
unsigned long keys[3]; /* keys defining the pseudo-random sequence */
const unsigned long *pcrc_32_tab;
int crypt_header_size;
#endif
} curfile64_info;
typedef struct {
zlib_filefunc64_32_def z_filefunc;
voidpf filestream; /* io structure of the zipfile */
voidpf filestream_with_CD; /* io structure of the zipfile with the central dir */
linkedlist_data central_dir; /* datablock with central dir in construction*/
int in_opened_file_inzip; /* 1 if a file in the zip is currently writ.*/
int append; /* append mode */
curfile64_info ci; /* info on the file currently writing */
ZPOS64_T begin_pos; /* position of the beginning of the zipfile */
ZPOS64_T add_position_when_writting_offset;
ZPOS64_T number_entry;
ZPOS64_T disk_size; /* size of each disk */
uLong number_disk; /* number of the current disk, used for spanning ZIP */
uLong number_disk_with_CD; /* number the the disk with central dir, used for spanning ZIP */
#ifndef NO_ADDFILEINEXISTINGZIP
char *globalcomment;
#endif
} zip64_internal;
/* Allocate a new data block */
local linkedlist_datablock_internal *allocate_new_datablock OF(());
local linkedlist_datablock_internal *allocate_new_datablock()
{
linkedlist_datablock_internal *ldi;
ldi = (linkedlist_datablock_internal *)ALLOC(sizeof(linkedlist_datablock_internal));
if (ldi != NULL) {
ldi->next_datablock = NULL;
ldi->filled_in_this_block = 0;
ldi->avail_in_this_block = SIZEDATA_INDATABLOCK;
}
return ldi;
}
/* Free data block in linked list */
local void free_datablock OF((linkedlist_datablock_internal * ldi));
local void free_datablock(linkedlist_datablock_internal *ldi)
{
while (ldi != NULL) {
linkedlist_datablock_internal *ldinext = ldi->next_datablock;
TRYFREE(ldi);
ldi = ldinext;
}
}
/* Initialize linked list */
local void init_linkedlist OF((linkedlist_data * ll));
local void init_linkedlist(linkedlist_data *ll)
{
ll->first_block = ll->last_block = NULL;
}
/* Free entire linked list and all data blocks */
local void free_linkedlist OF((linkedlist_data * ll));
local void free_linkedlist(linkedlist_data *ll)
{
free_datablock(ll->first_block);
ll->first_block = ll->last_block = NULL;
}
/* Add data to linked list data block */
local int add_data_in_datablock OF((linkedlist_data * ll, const void *buf, uLong len));
local int add_data_in_datablock(linkedlist_data *ll, const void *buf, uLong len)
{
linkedlist_datablock_internal *ldi;
const unsigned char *from_copy;
if (ll == NULL)
return ZIP_INTERNALERROR;
if (ll->last_block == NULL) {
ll->first_block = ll->last_block = allocate_new_datablock();
if (ll->first_block == NULL)
return ZIP_INTERNALERROR;
}
ldi = ll->last_block;
from_copy = (unsigned char *)buf;
while (len > 0) {
uInt copy_this;
uInt i;
unsigned char *to_copy;
if (ldi->avail_in_this_block == 0) {
ldi->next_datablock = allocate_new_datablock();

love_2016
- 粉丝: 0
- 资源: 3
最新资源
- springboot264基于JAVA的民族婚纱预定系统的设计与实现.zip
- springboot073车辆管理系统设计与实现.zip
- 《基于 Java 的本科生毕业在线考试电子商务web应用程序》(毕业设计,源码,部署教程)在本地部署即可运行。功能完善、界面美观、操作简单,具有很高的实用价值,适合相关专业毕设或课程设计使用。.zip
- 《基于 Java 的本科生毕业设计简单电子商务网站》(毕业设计,源码,部署教程)在本地部署即可运行。功能完善、界面美观、操作简单,具有很高的实用价值,适合相关专业毕设或课程设计使用。.zip
- 《基于 Java 的本科生毕业宠物领养中心开发项目》(毕业设计,源码,部署教程)在本地部署即可运行。功能完善、界面美观、操作简单,具有很高的实用价值,适合相关专业毕设或课程设计使用。.zip
- springboot072基于JavaWeb技术的在线考试系统设计与实现.zip
- 《基于Java 的本科生毕业租赁网站开发项目》(毕业设计,源码,部署教程)在本地部署即可运行。功能完善、界面美观、操作简单,具有很高的实用价值,适合相关专业毕设或课程设计使用。.zip
- springboot264基于JAVA的民族婚纱预定系统的设计与实现_0303174040.zip
- 《基于Java的在线食品订购系统》(毕业设计,源码,部署教程)在本地部署即可运行。功能完善、界面美观、操作简单,具有很高的实用价值,适合相关专业毕设或课程设计使用。.zip
- springboot074智能物流管理系统.zip
- springboot265基于Spring Boot的库存管理系统.zip
- springboot075电影评论网站系统设计与实现.zip
- springboot076基于web的智慧社区设计与实现.zip
- springboot265基于Spring Boot的库存管理系统_0303174040.zip
- springboot266基于Web的农产品直卖平台的设计与实现.zip
- 基于unet的皮肤病分割系统,pytorch开发
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


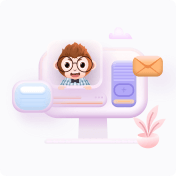