package jicq;
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.border.*;
import jicq.message.*;
import java.lang.NumberFormatException;
public class JUsrRegister
extends JFrame {
private TcpClient tcpClient;
private Thread tcpClientThread;
RegisterMsg rMsg = new RegisterMsg();
long usrID = 0;
int nCardPos = 0;
String[]
sSex = {
"男", "女"}
,
sCountry = {
"中华人民共和国", "韩国", "日本", "美国"}
,
sProvince = {
"江苏", "北京", "上海"}
,
sCFType = {
"身份证", "学生证", "工作证"};
ImageIcon[] iLogos = new ImageIcon[10];
JPanel jPnlMainCard = new JPanel();
JLabel jLabel4 = new JLabel();
JPanel jPanel4 = new JPanel();
JPasswordField jPswCheck = new JPasswordField();
JLabel jLabel1 = new JLabel();
JLabel jLabel3 = new JLabel();
JTextField jTfNickName = new JTextField();
JPanel jPanel3 = new JPanel();
JLabel jLabel2 = new JLabel();
JPanel jPanel5 = new JPanel();
BorderLayout borderLayout1 = new BorderLayout();
JPanel jPnlUsrInfo = new JPanel();
BorderLayout borderLayout2 = new BorderLayout();
JPanel jPnlBasicInfo = new JPanel();
JTextField jTfAge = new JTextField();
JComboBox jCmbSex = new JComboBox(sSex);
JPanel jPnlAreaInfo = new JPanel();
JPasswordField jPswFirst = new JPasswordField();
JPanel jPnlPswInfo = new JPanel();
JLabel jLabel5 = new JLabel();
CardLayout cardLayout = new CardLayout();
BorderLayout borderLayout6 = new BorderLayout();
JPanel jPnlButtons = new JPanel();
JButton jBtnHelp = new JButton();
JButton jBtnCancel = new JButton();
JButton jBtnNext = new JButton();
JButton jBtnPrevious = new JButton();
JPanel jPnlNetSetting = new JPanel();
JPanel jPnlFinalDone = new JPanel();
JLabel jLblFinalInfo = new JLabel();
BorderLayout borderLayout9 = new BorderLayout();
TitledBorder titledBorder1;
Border border1;
TitledBorder titledBorder2;
Border border2;
TitledBorder titledBorder3;
Border border3;
TitledBorder titledBorder4;
Border border4;
TitledBorder titledBorder5;
FlowLayout flowLayout2 = new FlowLayout();
JLabel jLabel8 = new JLabel();
JComboBox jCmbCountry = new JComboBox(sCountry);
JLabel jLabel9 = new JLabel();
JTextField jTfCity = new JTextField();
JComboBox jCmbProvince = new JComboBox(sProvince);
JLabel jLabel10 = new JLabel();
Border border5;
TitledBorder titledBorder6;
JLabel jLabel26 = new JLabel();
JPanel jPanel19 = new JPanel();
JTextField jTextField12 = new JTextField();
GridLayout gridLayout1 = new GridLayout();
JLabel jLabel24 = new JLabel();
JLabel jLabel23 = new JLabel();
JTextField jTextField11 = new JTextField();
JComboBox jComboBox5 = new JComboBox();
JTextField jTextField13 = new JTextField();
JTextField jTextField10 = new JTextField();
JLabel jLabel25 = new JLabel();
JPanel jPanel18 = new JPanel();
FlowLayout flowLayout3 = new FlowLayout();
JLabel jLabel22 = new JLabel();
JCheckBox jCheckBox1 = new JCheckBox();
Border border6;
TitledBorder titledBorder7;
JPanel jPanel1 = new JPanel();
JTextField jTextField9 = new JTextField();
JTextField jTextField8 = new JTextField();
GridLayout gridLayout2 = new GridLayout();
JLabel jLabel19 = new JLabel();
JLabel jLabel18 = new JLabel();
JPanel jPanel17 = new JPanel();
Border border7;
TitledBorder titledBorder8;
FlowLayout flowLayout4 = new FlowLayout();
JLabel jLabel12 = new JLabel();
JLabel jLabel13 = new JLabel();
JTextField jTfQuestion = new JTextField();
JTextField jTfAnswer = new JTextField();
JLabel jLabel14 = new JLabel();
JTextField jTfCFID = new JTextField();
JLabel jLabel6 = new JLabel();
JComboBox jCmbCFType = new JComboBox(sCFType);
JLabel jLabel15 = new JLabel();
JTextField jTfSafeEmail = new JTextField();
FlowLayout flowLayout1 = new FlowLayout();
JPanel jPanel2 = new JPanel();
JComboBox jCmbLogo; // = new JComboBox();
public JUsrRegister() throws HeadlessException {
try {
jbInit();
this.setSize(420, 380);
this.setResizable(false);
this.setLocation(250, 100);
this.setVisible(true);
}
catch (Exception e) {
e.printStackTrace();
}
}
private void jbInit() throws Exception {
for (int i = 1; i <= 10; i++) {
iLogos[i - 1] = new ImageIcon("logos\\" + i + ".gif", String.valueOf(i));
}
jCmbLogo = new JComboBox(iLogos);
titledBorder1 = new TitledBorder("");
border1 = BorderFactory.createEtchedBorder(Color.white,
new Color(148, 145, 140));
titledBorder2 = new TitledBorder(BorderFactory.createEtchedBorder(Color.
white, new Color(148, 145, 140)), "");
border2 = new EtchedBorder(EtchedBorder.RAISED, Color.white,
new Color(148, 145, 140));
titledBorder3 = new TitledBorder(border2, "基本资料");
border3 = new EtchedBorder(EtchedBorder.RAISED, Color.white,
new Color(148, 145, 140));
titledBorder4 = new TitledBorder(border3, "所在地区");
border4 = new EtchedBorder(EtchedBorder.RAISED, Color.white,
new Color(148, 145, 140));
titledBorder5 = new TitledBorder(border4, "密码保护");
border5 = new EtchedBorder(EtchedBorder.RAISED, Color.white,
new Color(148, 145, 140));
titledBorder6 = new TitledBorder(border5, "网络设置");
border6 = new EtchedBorder(EtchedBorder.RAISED, Color.white,
new Color(148, 145, 140));
titledBorder7 = new TitledBorder(border6, "代理服务器设置");
border7 = new EtchedBorder(EtchedBorder.RAISED, Color.white,
new Color(148, 145, 140));
titledBorder8 = new TitledBorder(border7, "网络设置");
this.getContentPane().setLayout(borderLayout6);
jLabel4.setText("确认密码");
jPswCheck.setPreferredSize(new Dimension(98, 22));
jPswCheck.setText("");
jLabel1.setText("性别");
jLabel3.setText("昵称");
jTfNickName.setPreferredSize(new Dimension(95, 22));
jTfNickName.setText("-");
jTfNickName.setInputVerifier(new NickNameVerifier());
jPanel3.setLayout(borderLayout1);
jPanel3.setMinimumSize(new Dimension(149, 64));
jPanel3.setPreferredSize(new Dimension(300, 64));
jLabel2.setText("年龄");
jPnlUsrInfo.setBorder(BorderFactory.createEtchedBorder());
jPnlUsrInfo.setMinimumSize(new Dimension(1160, 600));
jPnlUsrInfo.setPreferredSize(new Dimension(1160, 600));
jPnlBasicInfo.setLayout(borderLayout2);
jPnlBasicInfo.setBorder(titledBorder3);
jPnlBasicInfo.setMinimumSize(new Dimension(380, 85));
jPnlBasicInfo.setPreferredSize(new Dimension(380, 95));
jTfAge.setPreferredSize(new Dimension(45, 22));
jTfAge.setText("0");
jCmbSex.setMinimumSize(new Dimension(40, 25));
jCmbSex.setPreferredSize(new Dimension(45, 25));
jCmbSex.setPopupVisible(false);
jPnlAreaInfo.setLayout(flowLayout2);
jPnlAreaInfo.setBorder(titledBorder4);
jPnlAreaInfo.setMinimumSize(new Dimension(380, 51));
jPnlAreaInfo.setPreferredSize(new Dimension(380, 60));
jPswFirst.setPreferredSize(new Dimension(97, 22));
jPswFirst.setText("");
jPnlPswInfo.setBorder(titledBorder5);
jPnlPswInfo.setMinimumSize(new Dimension(380, 135));
jPnlPswInfo.setPreferredSize(new Dimension(380, 120));
jPnlPswInfo.setToolTipText("注意...");
jPnlPswInfo.setLayout(flowLayout1);
jLabel5.setText("密码");
jPnlMainCard.setLayout(cardLayout);
jBtnHelp.setText("帮助");
jBtnCancel.setText("取消");
jBtnCancel.addActionListener(new JUsrRegister_jBtnCancel_actionAdapter(this));
jBtnNext.setText("下一步");
jBtnNext.addActionListener(new JUsrRegister
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
这是一个用Java实现的QQ聊天程序 import java.net.*; import jicq.message.*; import java.sql.*; import java.util.*; import jicq.friend.*; import jicq.session.*; import oracle.jdbc.driver.*;
资源推荐
资源详情
资源评论
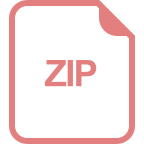
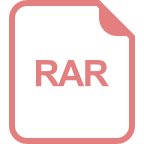
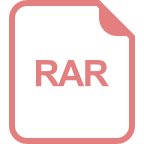
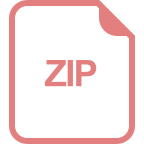
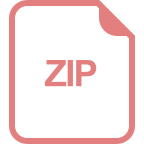
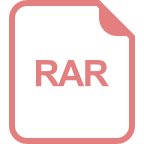
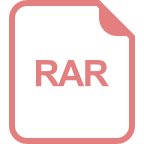
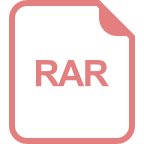
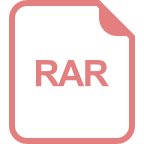
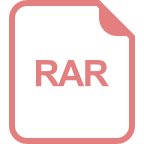
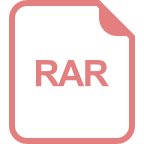
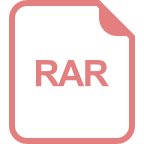
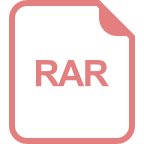
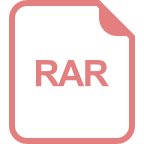
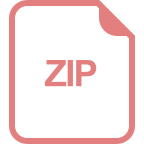
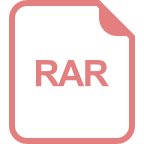
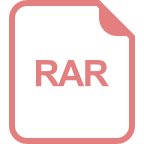
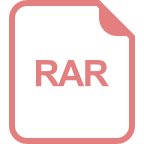
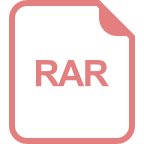
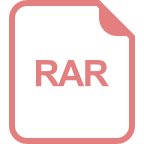
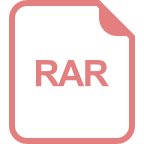
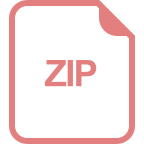
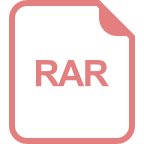
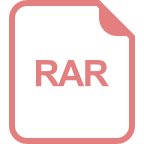
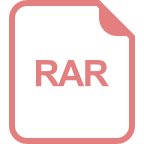
收起资源包目录

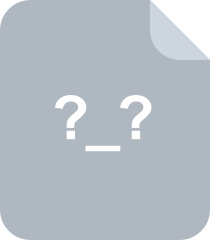
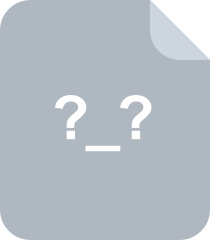
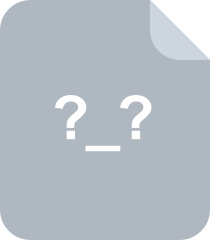
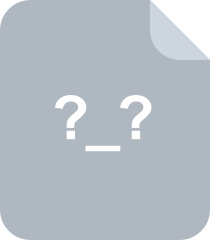
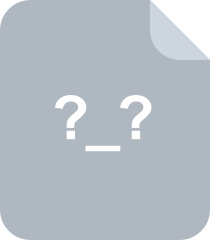
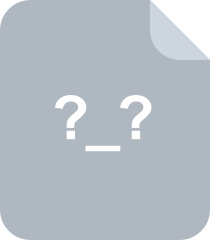
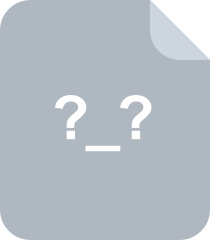
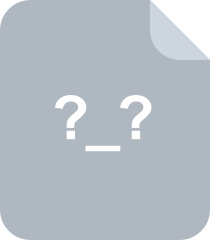
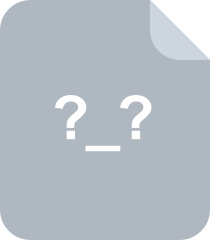
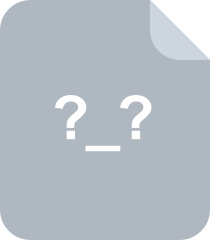
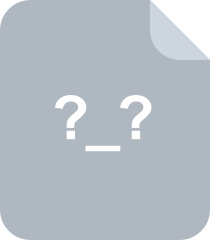
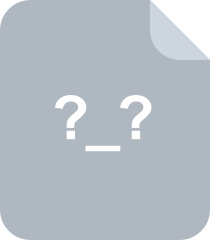
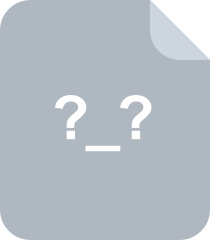
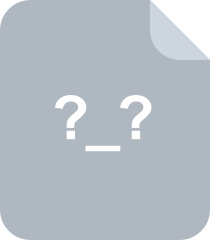
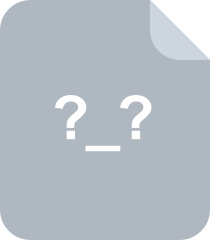
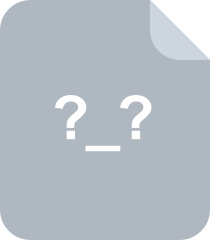
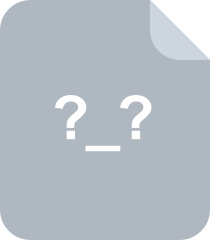
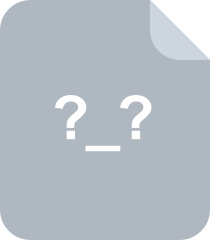
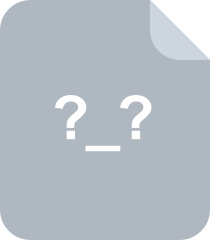
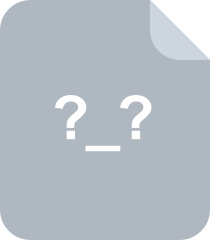
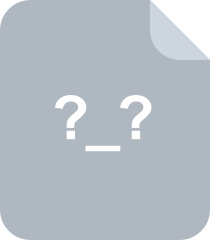
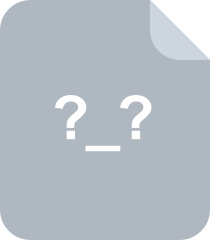
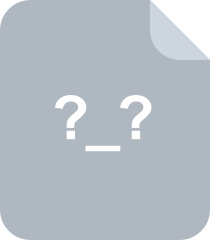
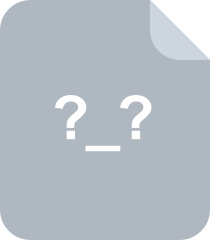
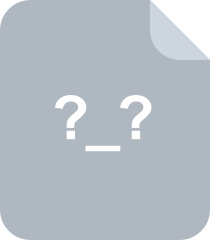
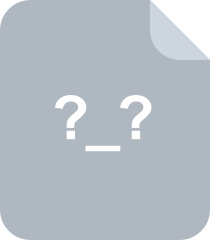
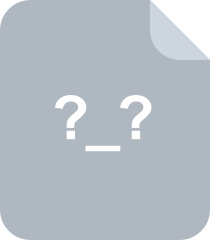
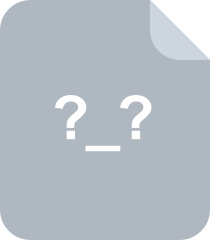
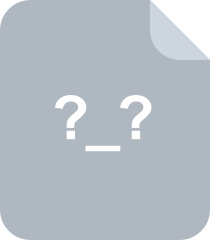
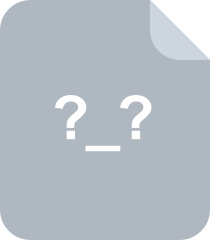
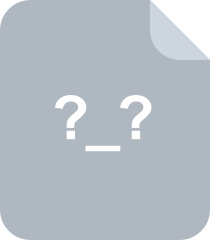
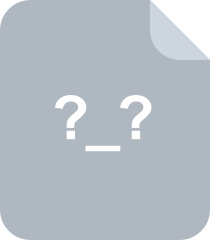
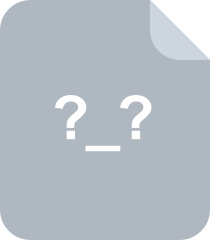
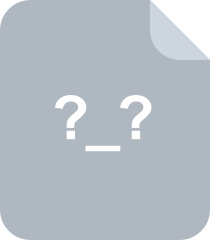
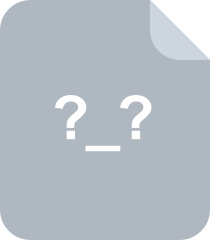
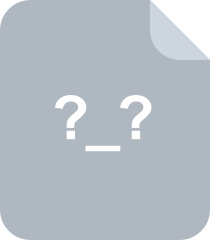
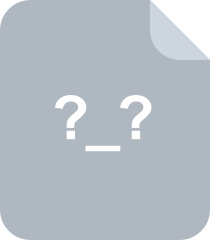
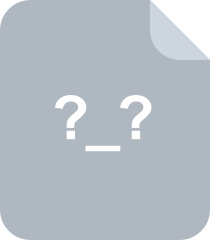
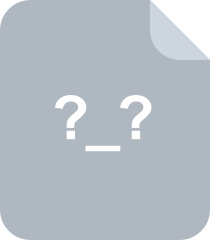
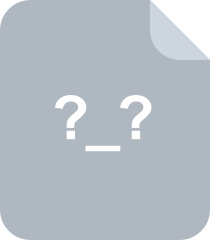
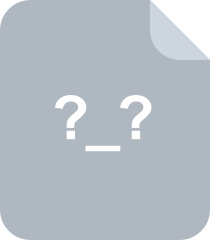
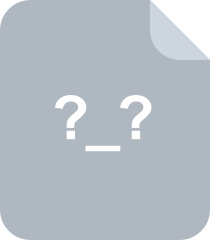
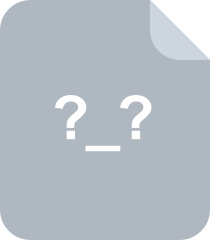
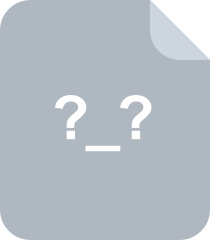
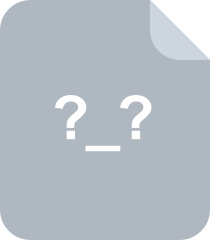
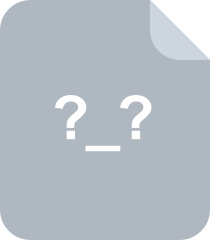
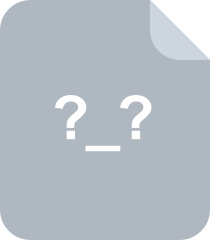
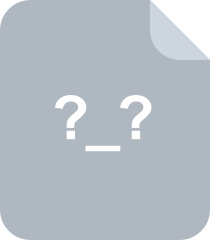
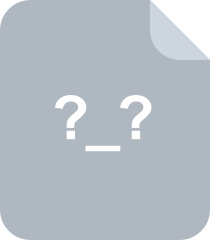
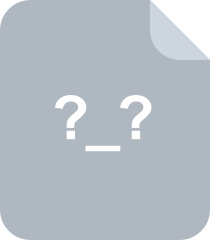
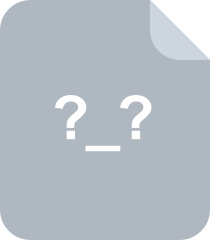
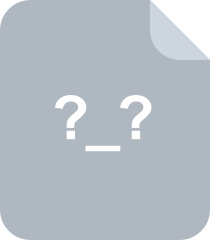
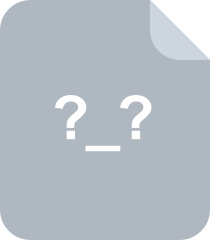
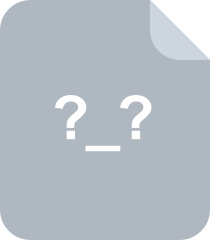
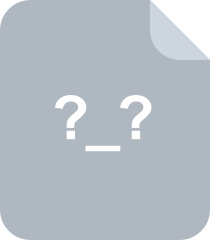
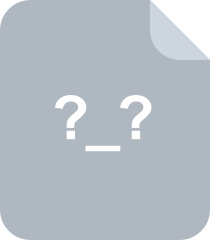
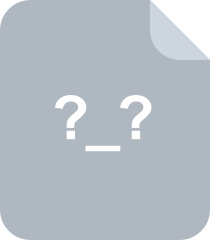
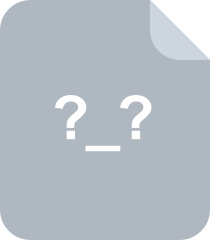
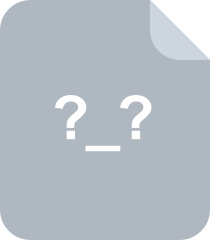
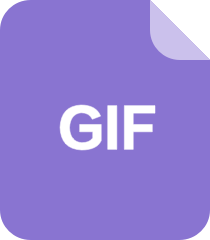
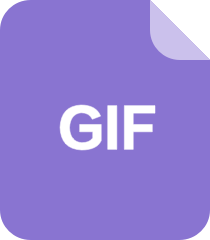
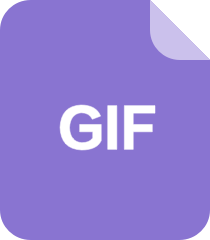
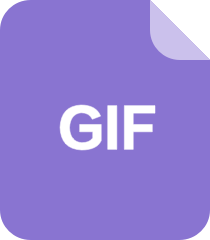
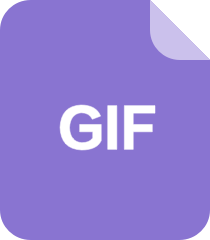
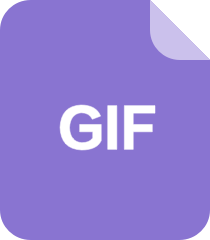
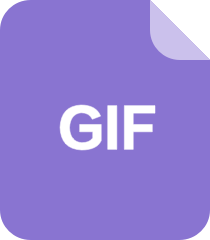
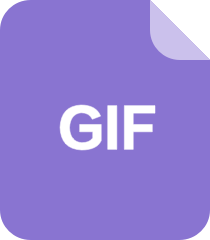
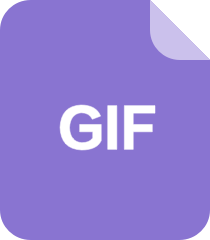
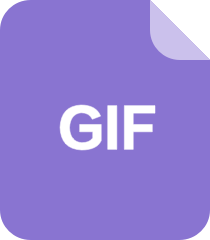
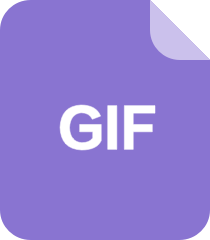
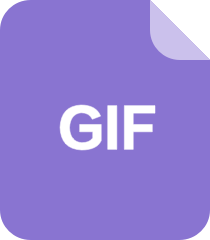
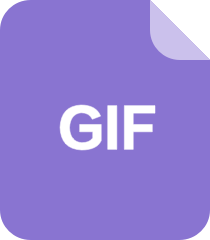
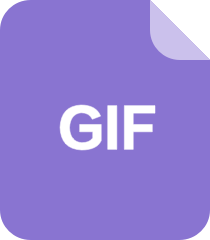
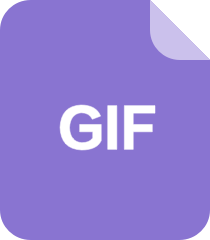
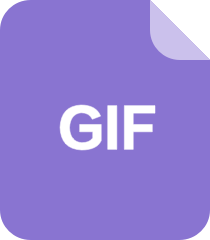
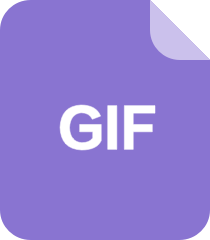
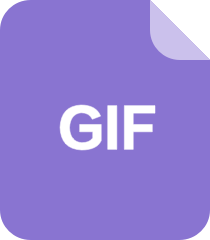
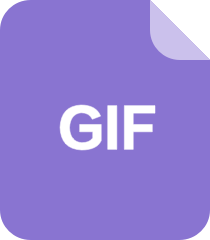
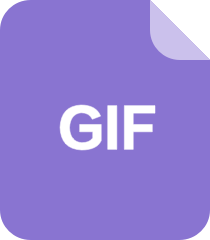
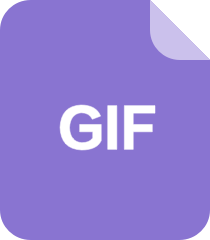
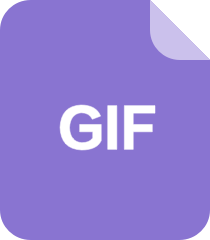
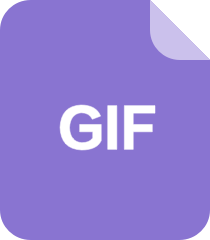
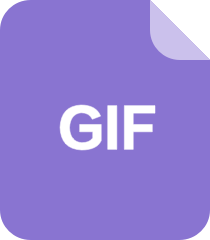
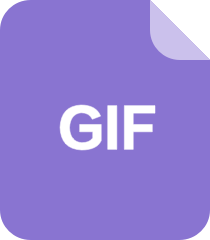
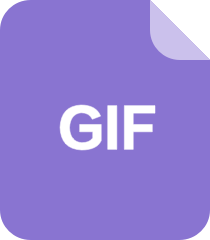
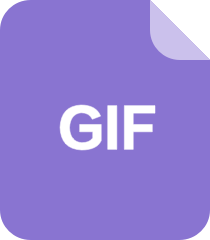
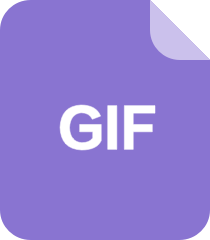
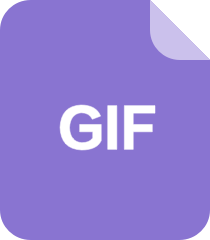
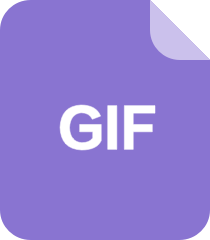
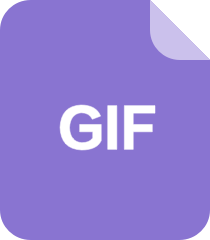
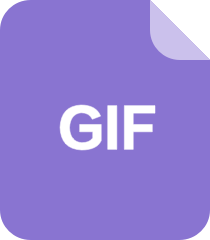
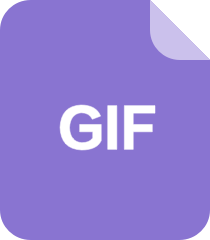
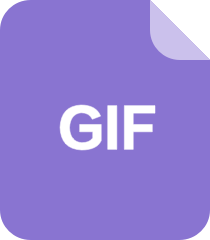
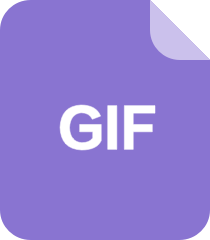
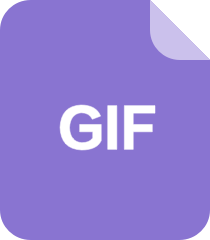
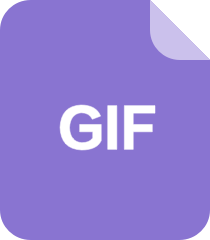
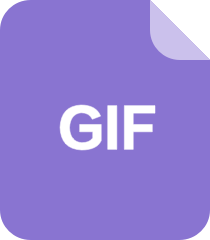
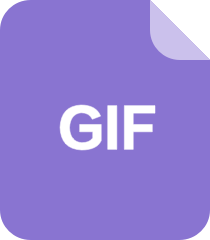
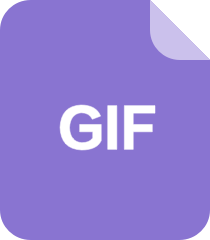
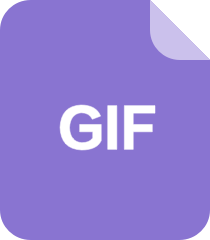
共 385 条
- 1
- 2
- 3
- 4
资源评论
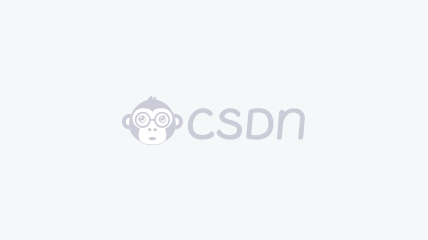

lostinsunday
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

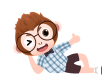
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


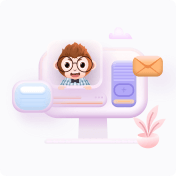
安全验证
文档复制为VIP权益,开通VIP直接复制
