.net 上传图片-缩略图,文字水印,图片水印
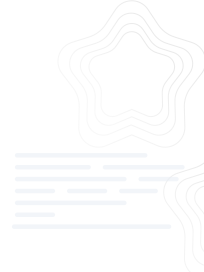

在.NET框架中,处理图像操作是一项常见的任务,包括图片上传、生成缩略图、添加文字水印和图片水印等。这些功能广泛应用于网站开发、社交媒体应用、图像处理软件等场景。下面将详细介绍如何在.NET中实现这些功能。 图片上传是基本操作,通常涉及用户通过网页或其他界面选择本地图片并将其发送到服务器。在ASP.NET中,我们可以使用`HttpPostedFileBase`类来接收上传的文件。以下是一个简单的示例: ```csharp [HttpPost] public ActionResult Upload(HttpPostedFileBase file) { if (file != null && file.ContentLength > 0) { string fileName = Path.GetFileName(file.FileName); string savePath = Path.Combine(Server.MapPath("~/Images/"), fileName); file.SaveAs(savePath); } return RedirectToAction("Index"); } ``` 接下来,生成缩略图是图像处理中的常见需求。我们可以使用.NET Framework自带的`System.Drawing`命名空间中的`Image`类来实现。下面的代码演示了如何创建一个原始图片的缩略版本: ```csharp using System.Drawing; using System.Drawing.Drawing2D; public Image GenerateThumbnail(string originalImagePath, int maxWidth, int maxHeight) { Image originalImage = Image.FromFile(originalImagePath); int width, height; if (originalImage.Width > originalImage.Height) { width = maxWidth; height = (maxHeight * originalImage.Height) / originalImage.Width; } else { height = maxHeight; width = (maxWidth * originalImage.Width) / originalImage.Height; } Bitmap thumbnail = new Bitmap(width, height); using (Graphics graphics = Graphics.FromImage(thumbnail)) { graphics.CompositingQuality = CompositingQuality.HighQuality; graphics.InterpolationMode = InterpolationMode.HighQualityBicubic; graphics.DrawImage(originalImage, 0, 0, width, height); } return thumbnail; } ``` 然后,我们来讨论如何在图片上添加文字水印。使用`Graphics.DrawString()`方法可以实现这一功能: ```csharp public void AddTextWatermark(string inputPath, string outputPath, string watermarkText, Color textColor, float angle) { Image originalImage = Image.FromFile(inputPath); Bitmap watermarkImage = new Bitmap(originalImage.Width, originalImage.Height); using (Graphics graphics = Graphics.FromImage(watermarkImage)) { graphics.SmoothingMode = SmoothingMode.AntiAlias; graphics.InterpolationMode = InterpolationMode.HighQualityBicubic; graphics.DrawString(watermarkText, new Font("Arial", 36), new SolidBrush(textColor), new PointF(10, 10), StringFormat.GenericTypographic); graphics.RotateTransform(angle); } watermarkImage.Save(outputPath); } ``` 添加图片水印则需要将源图片与水印图片合并。这里可以使用`Graphics.DrawImage()`方法,同时调整透明度以实现半透明效果: ```csharp public void AddImageWatermark(string inputPath, string watermarkPath, string outputPath, float opacity) { Image originalImage = Image.FromFile(inputPath); Image watermark = Image.FromFile(watermarkPath); Bitmap combinedImage = new Bitmap(originalImage.Width, originalImage.Height); using (Graphics graphics = Graphics.FromImage(combinedImage)) { graphics.CompositingMode = CompositingMode.SourceOver; graphics.CompositingQuality = CompositingQuality.HighQuality; graphics.InterpolationMode = InterpolationMode.HighQualityBicubic; graphics.DrawImage(originalImage, new Point(0, 0)); ColorMatrix colorMatrix = new ColorMatrix(); colorMatrix.Matrix33 = opacity; ImageAttributes attributes = new ImageAttributes(); attributes.SetColorMatrix(colorMatrix); graphics.DrawImage(watermark, new Rectangle(0, 0, watermark.Width, watermark.Height), 0, 0, watermark.Width, watermark.Height, GraphicsUnit.Pixel, attributes); } combinedImage.Save(outputPath); } ``` 在.NET中处理图片上传、生成缩略图、添加文字水印和图片水印的功能,主要依赖于`System.Drawing`命名空间中的类。通过理解并熟练运用这些类的方法,我们可以轻松实现各种图像处理任务。以上代码只是一个基础示例,实际应用中可能需要根据具体需求进行优化,例如错误处理、性能提升、资源管理等。
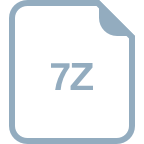
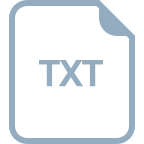
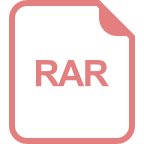
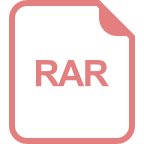
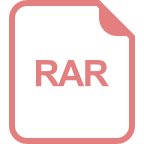
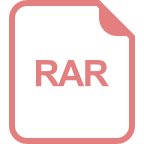
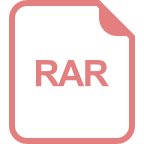
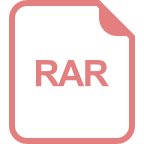
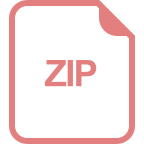
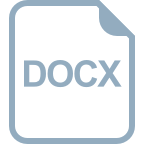
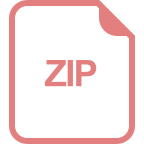
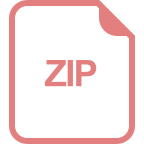
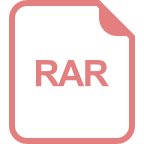
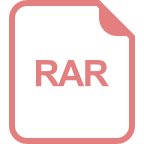
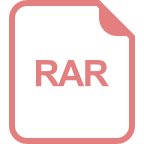
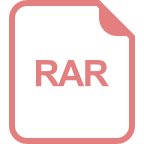
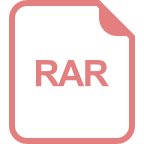



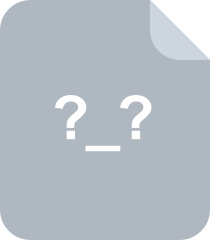
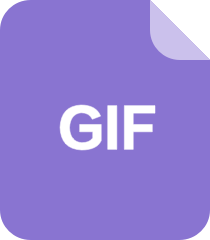
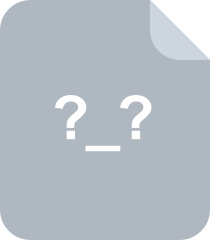

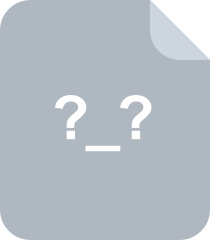
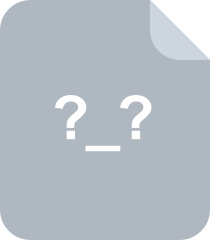

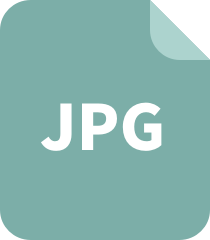
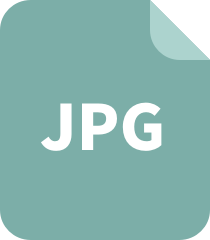
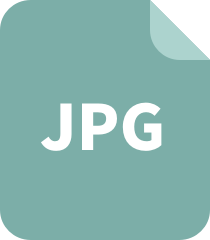
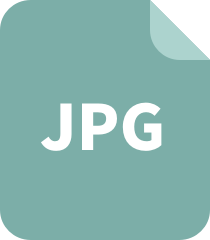
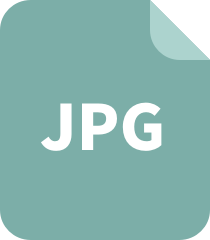
- 1
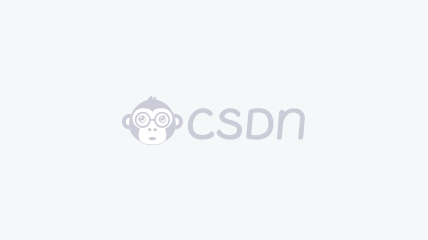

- 粉丝: 0
- 资源: 6
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

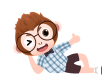
最新资源

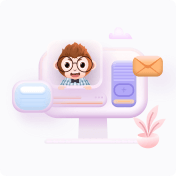
