using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using Microsoft.Kinect;
namespace KinectPowerPointControl
{
public partial class MainWindow : Window
{
KinectSensor kinectSensor;
private byte[] pixelData;
bool isBackGestureActive = false;
bool isForwardGestureActive = false;
public MainWindow()
{
InitializeComponent();
}
private void Window_Loaded(object sender, RoutedEventArgs e)
{
kinectSensor = (from sensor in KinectSensor.KinectSensors
where sensor.Status == KinectStatus.Connected
select sensor).FirstOrDefault();
kinectSensor.ColorStream.Enable(ColorImageFormat.RgbResolution640x480Fps30);
kinectSensor.SkeletonStream.Enable();
kinectSensor.Start();
kinectSensor.ColorFrameReady += kinectSensor_ColorFrameReady;
kinectSensor.SkeletonFrameReady += new EventHandler<SkeletonFrameReadyEventArgs>(kinectSensor_SkeletonFrameReady);
}
private void kinectSensor_ColorFrameReady(object sender, ColorImageFrameReadyEventArgs e)
{
using (ColorImageFrame imageFrame = e.OpenColorImageFrame())
{
if (imageFrame != null)
{
pixelData = new byte[imageFrame.PixelDataLength];
imageFrame.CopyPixelDataTo(this.pixelData);
this.ColorImage.Source = BitmapSource.Create(imageFrame.Width,
imageFrame.Height, 96, 96, PixelFormats.Bgr32, null, pixelData,
imageFrame.Width * imageFrame.BytesPerPixel);
}
}
}
private void kinectSensor_SkeletonFrameReady(object sender, SkeletonFrameReadyEventArgs e)
{
using (SkeletonFrame skeletonFrame = e.OpenSkeletonFrame())
{
if (skeletonFrame != null)
{
Skeleton[] skeletonData = new Skeleton[kinectSensor.SkeletonStream.FrameSkeletonArrayLength];
skeletonFrame.CopySkeletonDataTo(skeletonData);
Skeleton skeleton = (from s in skeletonData where s.TrackingState == SkeletonTrackingState.Tracked select s).FirstOrDefault();
if (skeleton != null)
{
SkeletonCanvas.Visibility = Visibility.Visible;
ProcessGesture(skeleton);
}
}
}
}
private void ProcessGesture(Skeleton s)
{
Joint leftHand = (from j in s.Joints where j.JointType == JointType.HandLeft select j).FirstOrDefault();
Joint rightHand = (from j in s.Joints where j.JointType == JointType.HandRight select j).FirstOrDefault();
Joint head = (from j in s.Joints where j.JointType == JointType.Head select j).FirstOrDefault();
if (rightHand.Position.X > head.Position.X + 0.45)
{
if (!isBackGestureActive && !isForwardGestureActive)
{
isForwardGestureActive = true;
System.Windows.Forms.SendKeys.SendWait("{Right}");
}
}
else
{
isForwardGestureActive = false;
}
if (leftHand.Position.X < head.Position.X - 0.45)
{
if (!isBackGestureActive && !isForwardGestureActive)
{
isBackGestureActive = true;
System.Windows.Forms.SendKeys.SendWait("{Left}");
}
}
else
{
isBackGestureActive = false;
}
SetEllipsePosition(ellipseHead, head, false);
SetEllipsePosition(ellipseLeftHand, leftHand, isBackGestureActive);
SetEllipsePosition(ellipseRightHand, rightHand, isForwardGestureActive);
}
private void SetEllipsePosition(Ellipse ellipse, Joint joint, bool isHighlighted)
{
ColorImagePoint colorImagePoint = kinectSensor.CoordinateMapper.MapSkeletonPointToColorPoint(joint.Position, ColorImageFormat.RgbResolution640x480Fps30);
if (isHighlighted)
{
ellipse.Width = 60;
ellipse.Height = 60;
ellipse.Fill = Brushes.Red;
}
else
{
ellipse.Width = 20;
ellipse.Height = 20;
ellipse.Fill = Brushes.Blue;
}
Canvas.SetLeft(ellipse, colorImagePoint.X - ellipse.ActualWidth / 2);
Canvas.SetTop(ellipse, colorImagePoint.Y - ellipse.ActualHeight / 2);
}
private void Window_Closed(object sender, EventArgs e)
{
kinectSensor.Stop();
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
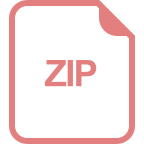
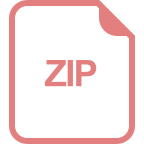
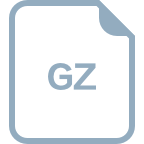
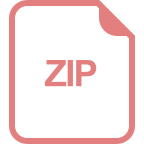
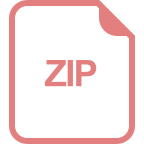
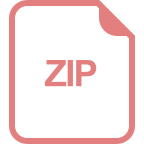
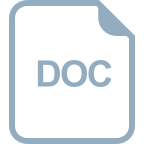
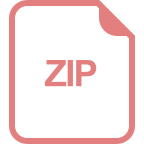
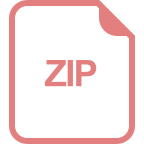
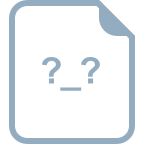
收起资源包目录



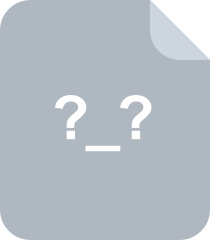
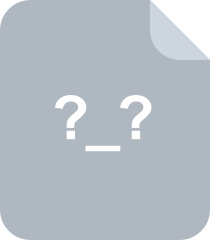
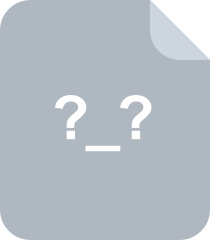
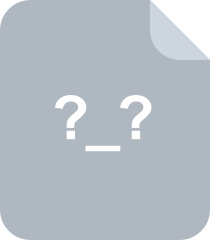
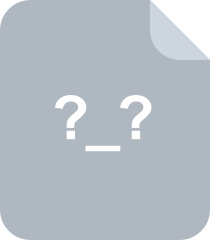

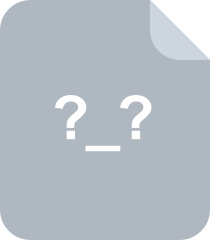
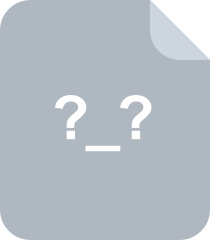
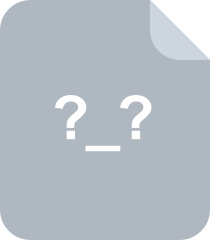
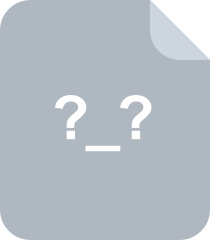
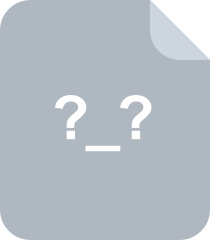
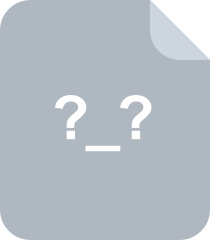



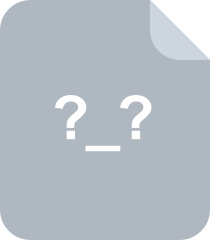
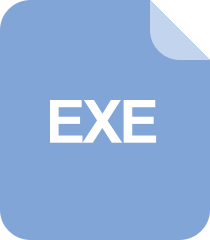
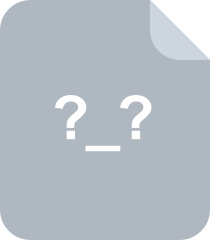

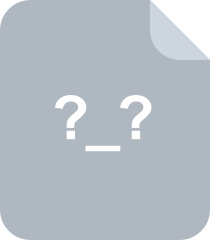
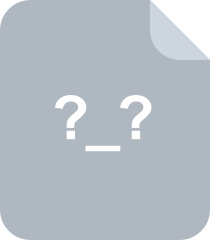
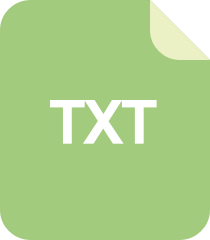
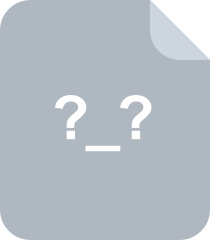
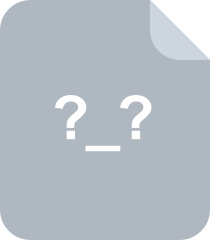
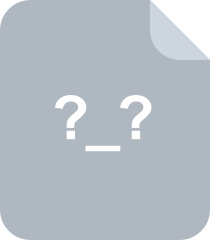
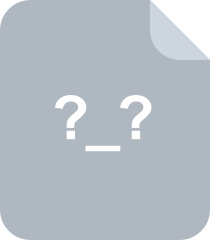
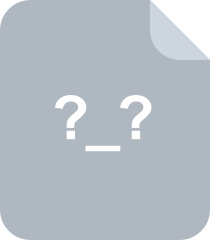
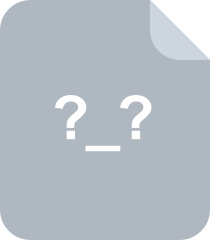
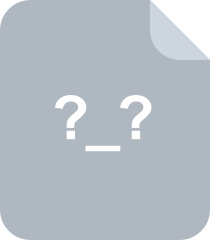
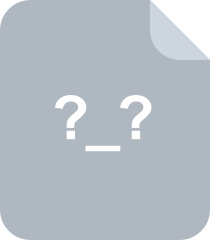
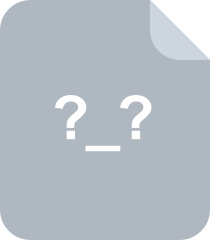
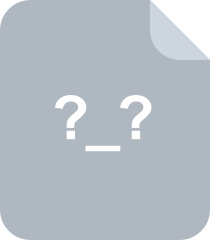
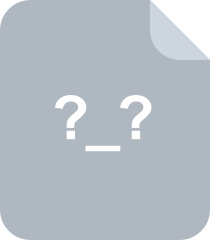
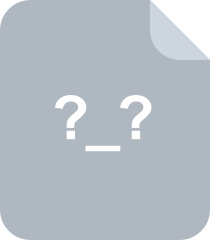


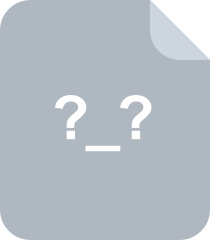
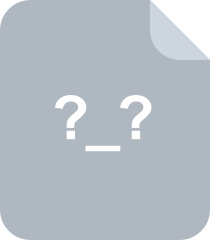
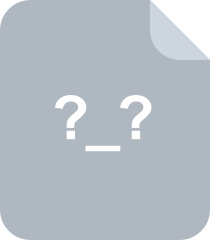
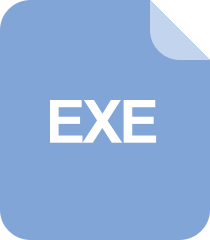
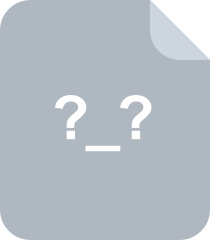
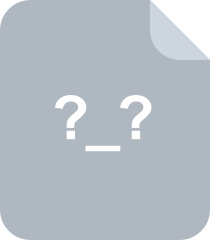
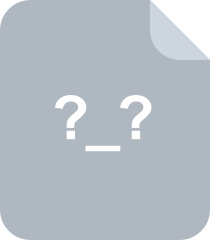
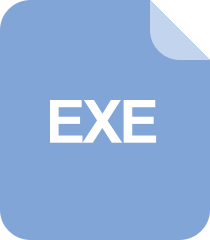
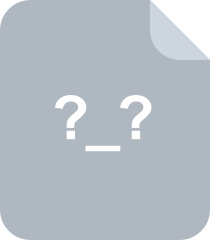
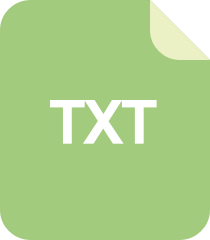
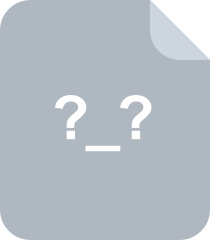
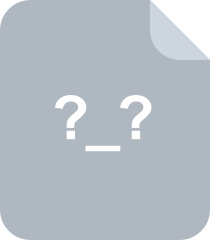
共 41 条
- 1

瀚海城主
- 粉丝: 4
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

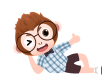
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页