一个Hibernate 的简单教程
【一个Hibernate的简单教程】 在Java开发中,Hibernate是一个非常流行的ORM(对象关系映射)框架,它允许开发者将数据库操作转化为对Java对象的操作,极大地简化了数据持久化的复杂性。本教程旨在提供一个无需Web服务器环境,在Eclipse中直接运行的简单Hibernate应用实例。 你需要对Eclipse有一定的熟悉,它是Java开发中常用的集成开发环境。接着,你需要下载并准备Hibernate所需的JAR文件,这些文件包含了Hibernate的核心库和其他依赖,如JDBC驱动,以便连接到数据库。在这个例子中,我们将使用MySQL数据库,因此还需要安装MySQL并创建对应的数据库和表。 假设我们已经有了一个名为`news`的数据库表,其结构如下: ``` +-----------+--------------+------+-----+---------+----------------+ | Field | Type | Null | Key | Default | Extra | +-----------+--------------+------+-----+---------+----------------+ | id | int(11) | NO | MUL | NULL | auto_increment | | title | varchar(400) | YES | | NULL | | | content | text | YES | | NULL | | | time | datetime | YES | | NULL | | +-----------+--------------+------+-----+---------+----------------+ ``` 该表用于存储新闻的ID、标题、内容和时间。接下来,我们需要在Eclipse中创建一个Java项目,并定义一个与之对应的实体类`News`。以下是一个简单的`News`类实现: ```java package cn.com.nick.hbm; import java.util.Date; public class News { private int id; private String title; private String content; private Date date; // getters and setters public int getId() { return id; } public void setId(int id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getContent() { return content; } public void setContent(String content) { this.content = content; } public Date getDate() { return date; } public void setDate(Date date) { this.date = date; } } ``` 然后,我们需要配置Hibernate的映射文件`News.hbm.xml`,它描述了`News`类与`NEWS`表之间的关系: ```xml <?xml version="1.0"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <!-- 指定News类对应NEWS表 --> <class name="cn.com.nick.hbm.News" table="NEWS"> <!-- 配置主键id --> <id name="id" column="id"> <generator class="native" /> </id> <!-- 配置title属性 --> <property name="title" type="string" column="title"/> <!-- 配置content属性 --> <property name="content" type="text" column="content"/> <!-- 配置time属性 --> <property name="date" type="date" column="time"/> </class> </hibernate-mapping> ``` 配置完成后,你需要创建一个配置文件`hibernate.cfg.xml`,其中包含了数据库连接信息,例如数据库URL、用户名和密码: ```xml <?xml version='1.0' encoding='utf-8'?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property> <property name="hibernate.connection.driver_class">com.mysql.jdbc.Driver</property> <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/news</property> <property name="hibernate.connection.username">your_username</property> <property name="hibernate.connection.password">your_password</property> <property name="hibernate.show_sql">true</property> <mapping resource="cn/com/nick/hbm/News.hbm.xml"/> </session-factory> </hibernate-configuration> ``` 现在,你已经具备了基本的环境和配置,可以开始编写Java代码来实现CRUD(创建、读取、更新、删除)操作了。例如,创建一条新闻记录的代码可能如下: ```java import org.hibernate.HibernateException; import org.hibernate.Session; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; public class HibernateTest { public static void main(String[] args) { Configuration cfg = new Configuration().configure("hibernate.cfg.xml"); Session session = cfg.buildSessionFactory().openSession(); Transaction tx = null; try { tx = session.beginTransaction(); News news = new News(); news.setTitle("Example Title"); news.setContent("This is an example content."); news.setDate(new Date()); session.save(news); tx.commit(); } catch (HibernateException e) { if (tx != null) { tx.rollback(); } e.printStackTrace(); } finally { session.close(); } } } ``` 以上代码通过Hibernate API初始化配置,打开数据库会话,开始事务,创建一个`News`对象并设置属性,然后调用`save()`方法将其保存到数据库。如果发生异常,事务会被回滚,确保数据一致性。 这个简单的教程介绍了如何在Eclipse环境中使用Hibernate进行数据库操作,无需额外的Web服务器或ANT脚本。你可以根据需要扩展这个基础示例,学习更多关于查询、更新和删除操作,以及更复杂的对象关系映射技术。通过这种方式,你可以更好地理解Hibernate如何将Java对象和数据库表之间的交互变得简单易行。
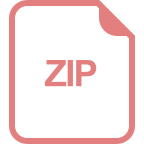
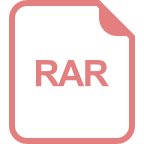
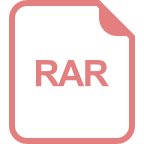
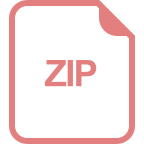
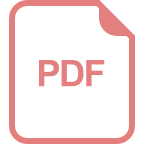
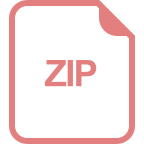
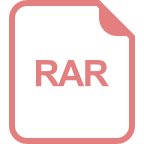
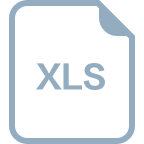
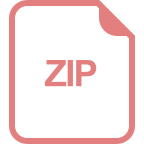
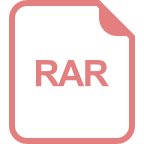
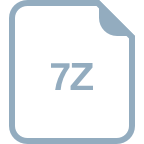
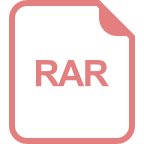
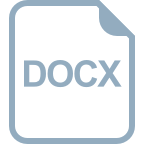
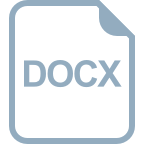
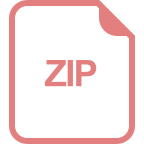
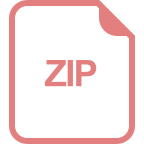
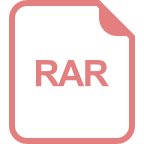
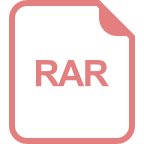
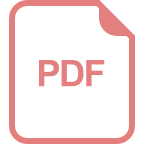
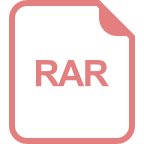
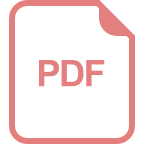
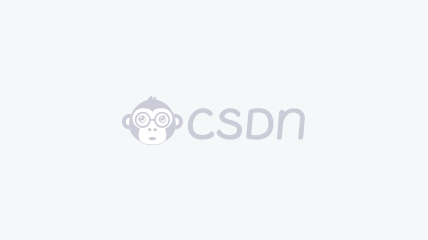

- 粉丝: 15
- 资源: 97
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

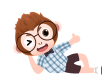
最新资源
- 毕设-java web-ssm-企业门户网站12.zip
- 计算机毕业实习报告+适用于计算机本科毕业
- 毕设-java web-ssm-天下陶网络商城13.zip
- 毕设-java web-ssm-物流配货网17.zip
- 毕设-java web-ssm-网上淘书吧16.zip
- 毕设-java web-ssm-网络购物中心项目源码15.zip
- 毕设-java web-ssm-新奥家电连锁网络系统20.zip
- 毕设-java web-ssm-物资管理系统项目源码18.zip
- 毕设-java web-ssm-校园管理系统源码19.zip
- 毕设-java web-ssm-芝麻开门博客网22.zip
- 美团Mario接口自动化测试框架设计-HTTP/MAPI/Thrift/Pigeon协议的支持与实践
- 毕设-java web-ssm-讯友网络相册21.zip
- 基于PLC的多层升降自动化立体车库设计12000字查重30西门子200,组态王,程序,组态
- ECharts地图-自定义28.zip
- ECharts地图-自定义26.zip
- ECharts地图-自定义29.zip

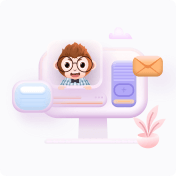
