/**
******************************************************************************
* @file stm32f4xx_tim.c
* @author MCD Application Team
* @version V1.4.0
* @date 04-August-2014
* @brief This file provides firmware functions to manage the following
* functionalities of the TIM peripheral:
* + TimeBase management
* + Output Compare management
* + Input Capture management
* + Advanced-control timers (TIM1 and TIM8) specific features
* + Interrupts, DMA and flags management
* + Clocks management
* + Synchronization management
* + Specific interface management
* + Specific remapping management
*
@verbatim
===============================================================================
##### How to use this driver #####
===============================================================================
[..]
This driver provides functions to configure and program the TIM
of all STM32F4xx devices.
These functions are split in 9 groups:
(#) TIM TimeBase management: this group includes all needed functions
to configure the TM Timebase unit:
(++) Set/Get Prescaler
(++) Set/Get Autoreload
(++) Counter modes configuration
(++) Set Clock division
(++) Select the One Pulse mode
(++) Update Request Configuration
(++) Update Disable Configuration
(++) Auto-Preload Configuration
(++) Enable/Disable the counter
(#) TIM Output Compare management: this group includes all needed
functions to configure the Capture/Compare unit used in Output
compare mode:
(++) Configure each channel, independently, in Output Compare mode
(++) Select the output compare modes
(++) Select the Polarities of each channel
(++) Set/Get the Capture/Compare register values
(++) Select the Output Compare Fast mode
(++) Select the Output Compare Forced mode
(++) Output Compare-Preload Configuration
(++) Clear Output Compare Reference
(++) Select the OCREF Clear signal
(++) Enable/Disable the Capture/Compare Channels
(#) TIM Input Capture management: this group includes all needed
functions to configure the Capture/Compare unit used in
Input Capture mode:
(++) Configure each channel in input capture mode
(++) Configure Channel1/2 in PWM Input mode
(++) Set the Input Capture Prescaler
(++) Get the Capture/Compare values
(#) Advanced-control timers (TIM1 and TIM8) specific features
(++) Configures the Break input, dead time, Lock level, the OSSI,
the OSSR State and the AOE(automatic output enable)
(++) Enable/Disable the TIM peripheral Main Outputs
(++) Select the Commutation event
(++) Set/Reset the Capture Compare Preload Control bit
(#) TIM interrupts, DMA and flags management
(++) Enable/Disable interrupt sources
(++) Get flags status
(++) Clear flags/ Pending bits
(++) Enable/Disable DMA requests
(++) Configure DMA burst mode
(++) Select CaptureCompare DMA request
(#) TIM clocks management: this group includes all needed functions
to configure the clock controller unit:
(++) Select internal/External clock
(++) Select the external clock mode: ETR(Mode1/Mode2), TIx or ITRx
(#) TIM synchronization management: this group includes all needed
functions to configure the Synchronization unit:
(++) Select Input Trigger
(++) Select Output Trigger
(++) Select Master Slave Mode
(++) ETR Configuration when used as external trigger
(#) TIM specific interface management, this group includes all
needed functions to use the specific TIM interface:
(++) Encoder Interface Configuration
(++) Select Hall Sensor
(#) TIM specific remapping management includes the Remapping
configuration of specific timers
@endverbatim
******************************************************************************
* @attention
*
* <h2><center>© COPYRIGHT 2014 STMicroelectronics</center></h2>
*
* Licensed under MCD-ST Liberty SW License Agreement V2, (the "License");
* You may not use this file except in compliance with the License.
* You may obtain a copy of the License at:
*
* http://www.st.com/software_license_agreement_liberty_v2
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f4xx_tim.h"
#include "stm32f4xx_rcc.h"
/** @addtogroup STM32F4xx_StdPeriph_Driver
* @{
*/
/** @defgroup TIM
* @brief TIM driver modules
* @{
*/
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
/* ---------------------- TIM registers bit mask ------------------------ */
#define SMCR_ETR_MASK ((uint16_t)0x00FF)
#define CCMR_OFFSET ((uint16_t)0x0018)
#define CCER_CCE_SET ((uint16_t)0x0001)
#define CCER_CCNE_SET ((uint16_t)0x0004)
#define CCMR_OC13M_MASK ((uint16_t)0xFF8F)
#define CCMR_OC24M_MASK ((uint16_t)0x8FFF)
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
/* Private function prototypes -----------------------------------------------*/
static void TI1_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection,
uint16_t TIM_ICFilter);
static void TI2_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection,
uint16_t TIM_ICFilter);
static void TI3_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection,
uint16_t TIM_ICFilter);
static void TI4_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection,
uint16_t TIM_ICFilter);
/* Private functions ---------------------------------------------------------*/
/** @defgroup TIM_Private_Functions
* @{
*/
/** @defgroup TIM_Group1 TimeBase management functions
* @brief TimeBase management functions
*
@verbatim
===============================================================================
##### TimeBase management functions #####
===============================================================================
##### TIM Driver: how to use it in Timing(Time base) Mode #####
===============================================================================
[..]
To use the Timer in Timing(Time base) mode, the following steps are mandatory:
(#) Enable TIM clock using RCC_APBxPeriphClockCmd(RCC_APBxPeriph_TIMx, ENABLE) function
(#) Fill the TIM_TimeBaseInitStruct with the desired parameters.
(#) Call TIM_TimeBaseInit(TIMx, &TIM_TimeBaseInitStruct) to configure the Time Base unit
with the corresponding configuration
(#) Enable the NVIC if you ne
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
个人花大量时间整理出的真实毕业设计实战成果,内容丰富,文档也很详细。无论做毕业设计还是用于学习技能,或工作中当做参考资料,都能发挥重要作用。 亲们下载我任何一个付费资源后,即可私信联系我免费下载其他相关资源,直到选到您满意的资源为止哦~ 个人花大量时间整理出的真实毕业设计实战成果,内容丰富,文档也很详细。无论做毕业设计还是用于学习技能,或工作中当做参考资料,都能发挥重要作用。 亲们下载我任何一个付费资源后,即可私信联系我免费下载其他相关资源,直到选到您满意的资源为止哦~ 个人花大量时间整理出的真实毕业设计实战成果,内容丰富,文档也很详细。无论做毕业设计还是用于学习技能,或工作中当做参考资料,都能发挥重要作用。 亲们下载我任何一个付费资源后,即可私信联系我免费下载其他相关资源,直到选到您满意的资源为止哦~
资源推荐
资源详情
资源评论
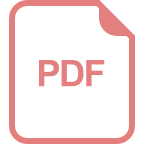
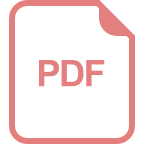
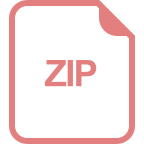
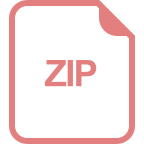
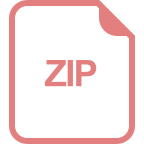
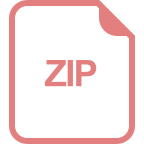
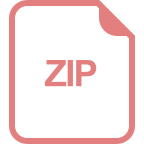
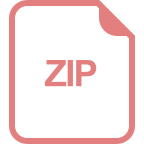
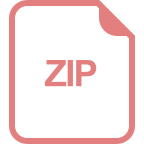
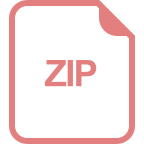
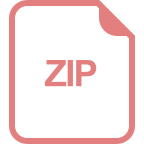
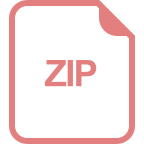
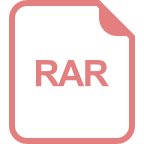
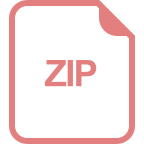
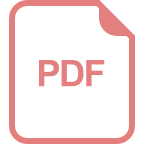
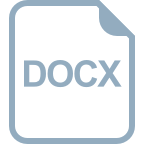
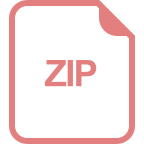
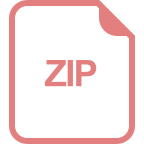
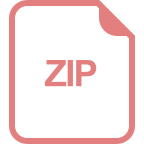
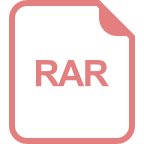
收起资源包目录

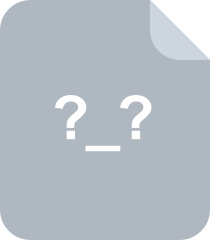
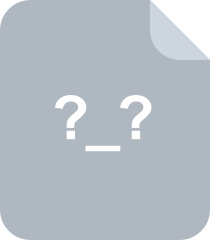
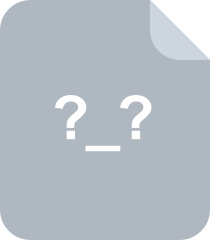
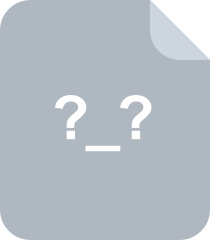
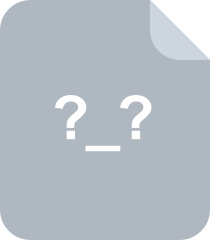
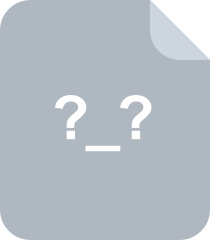
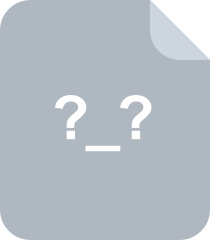
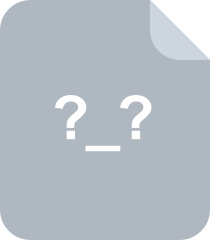
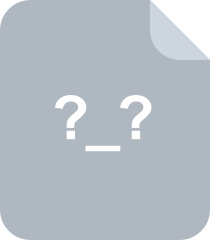
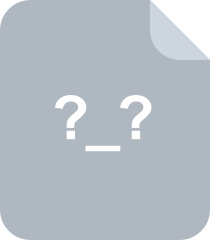
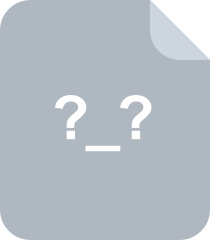
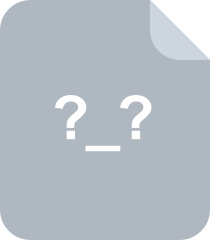
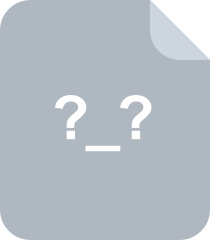
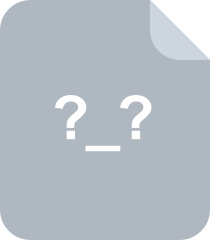
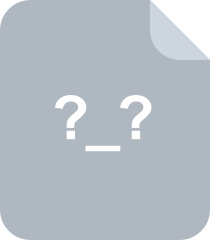
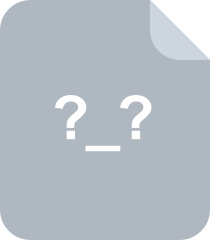
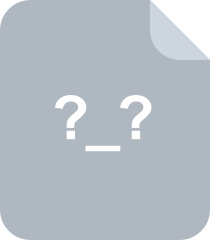
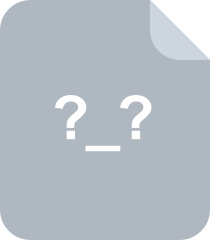
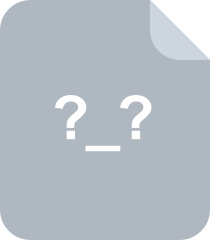
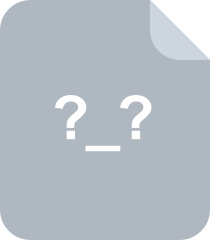
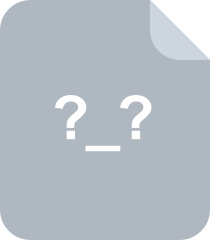
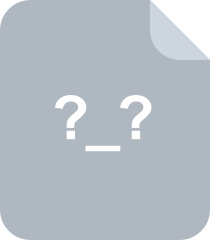
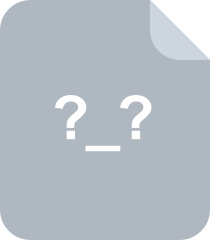
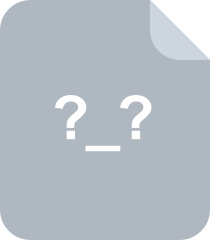
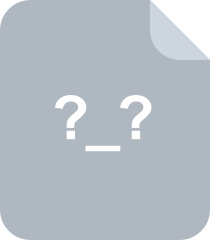
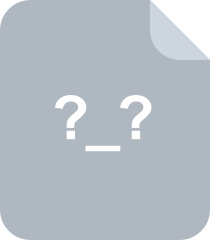
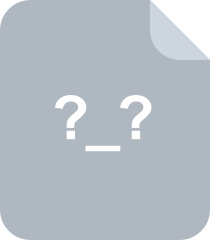
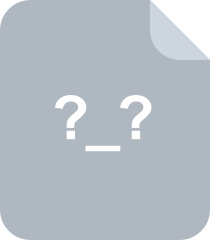
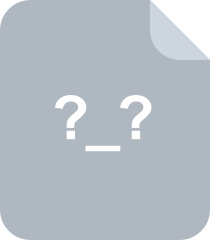
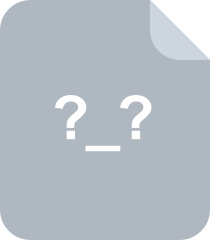
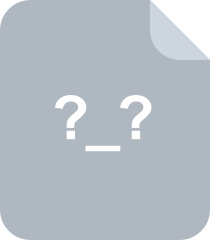
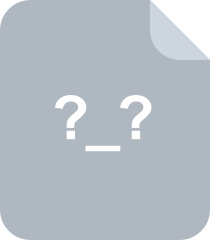
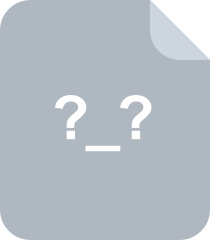
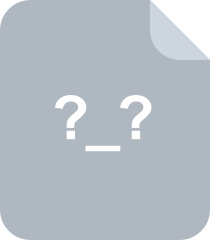
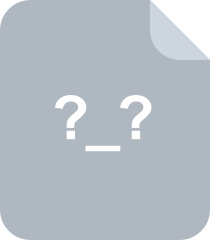
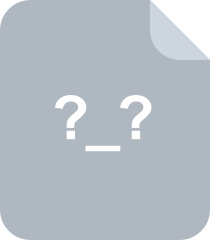
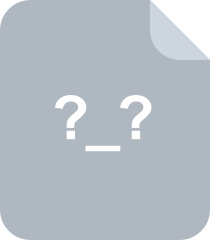
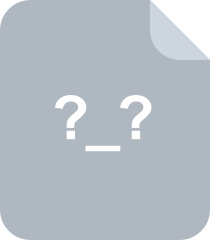
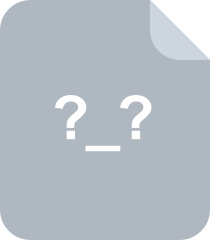
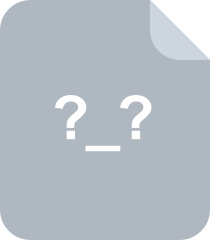
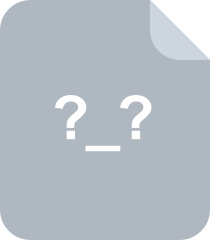
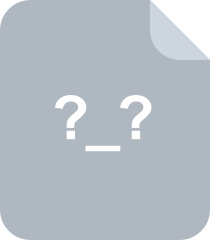
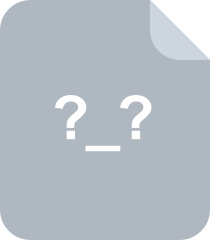
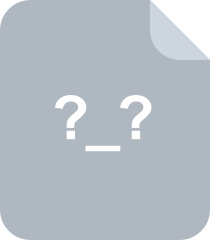
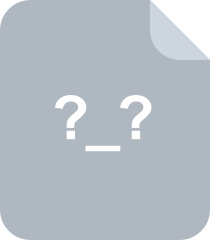
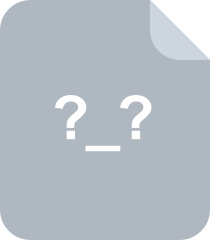
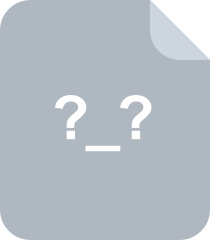
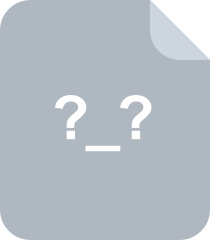
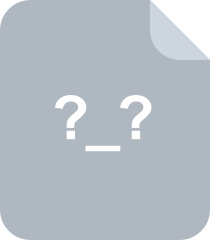
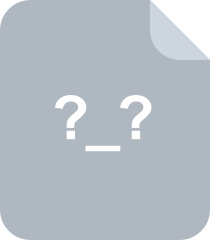
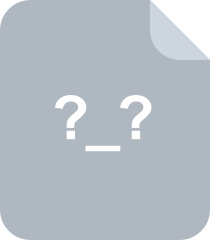
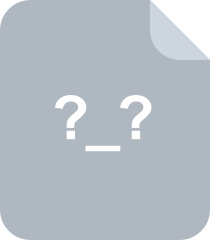
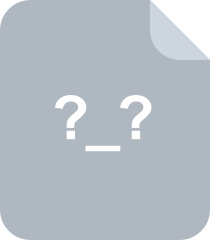
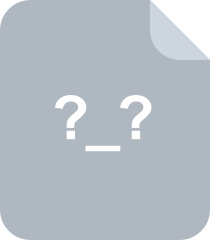
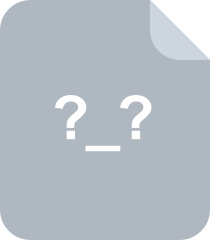
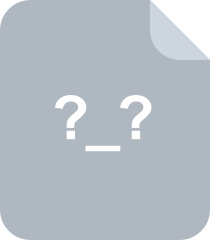
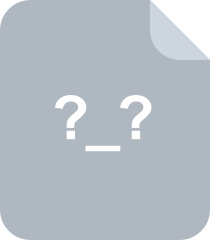
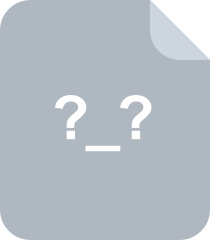
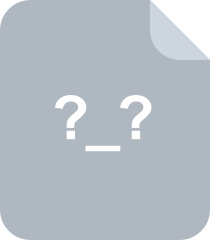
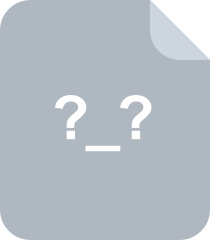
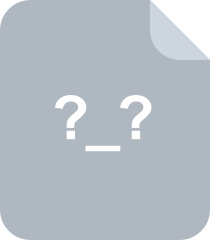
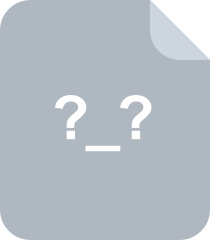
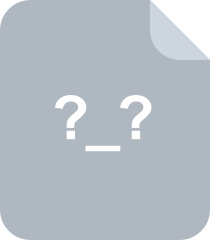
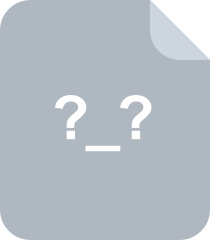
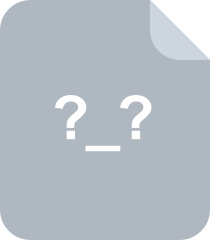
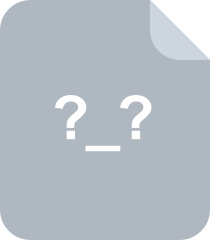
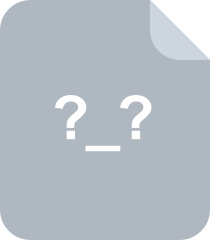
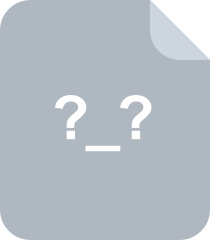
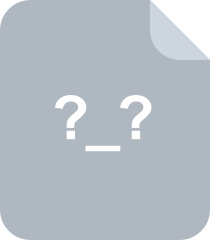
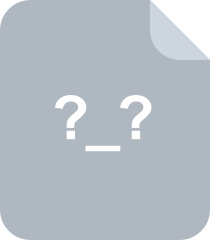
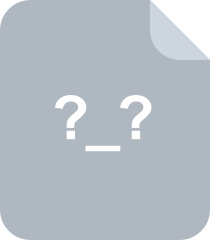
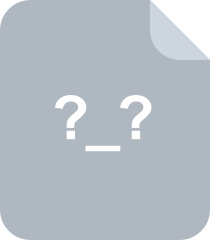
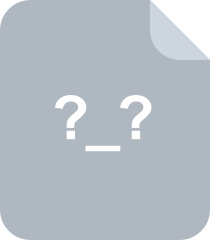
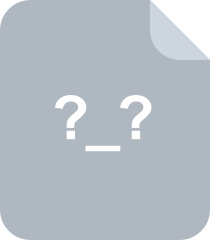
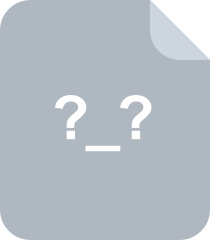
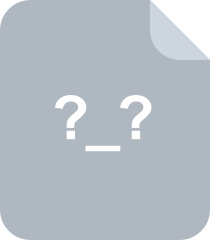
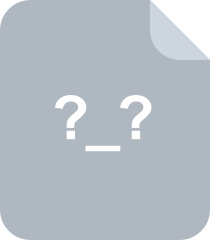
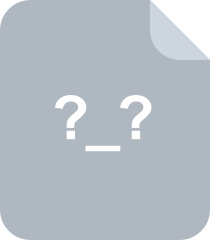
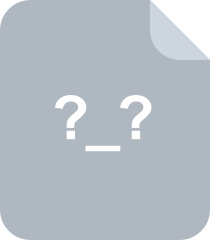
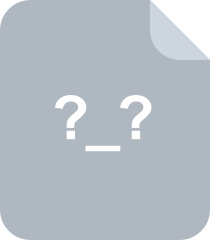
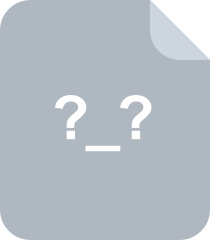
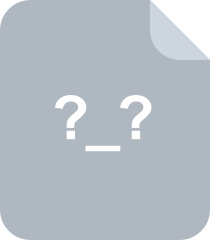
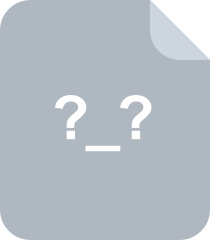
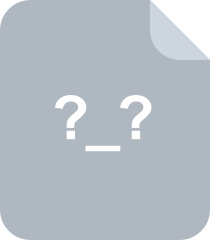
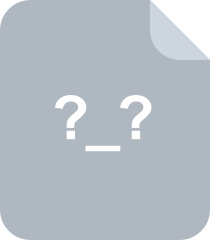
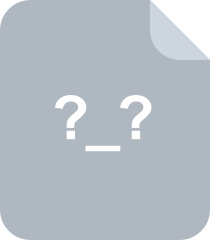
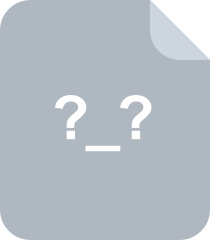
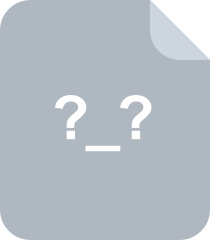
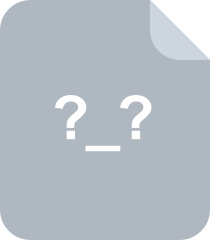
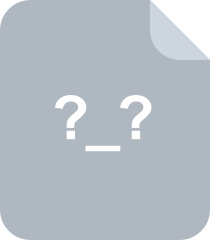
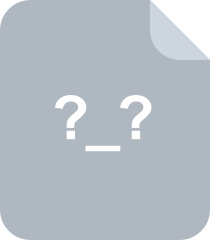
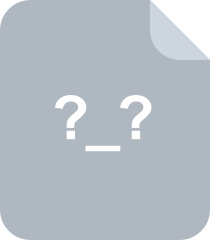
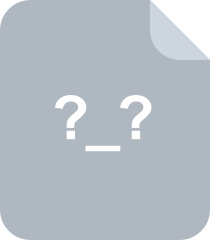
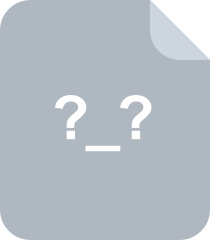
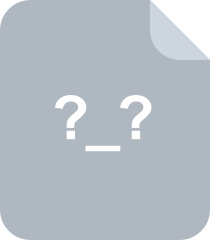
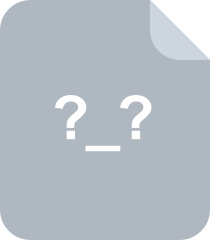
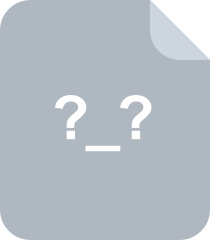
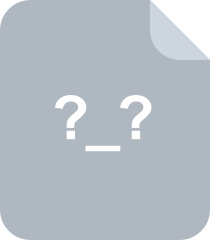
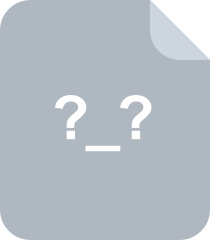
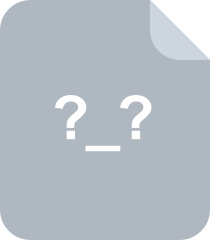
共 1364 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
资源评论
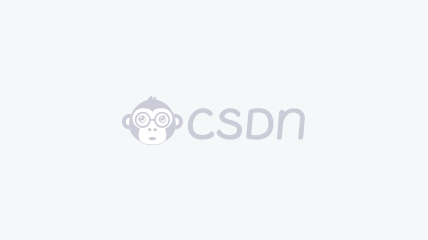

季风泯灭的季节
- 粉丝: 1901
- 资源: 3370
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

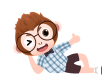
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


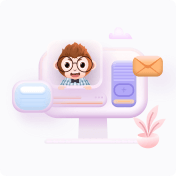
安全验证
文档复制为VIP权益,开通VIP直接复制
