package com.bookstore.controller;
import java.io.IOException;
import java.util.List;
import javax.servlet.http.Cookie;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import net.sf.json.JSONObject;
import org.apache.commons.codec.binary.Base64;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import com.bookstore.domain.CartItem;
import com.bookstore.domain.ShoppingCart;
import com.bookstore.service.ShoppingCartService;
@Controller
public class CartController {
@Autowired
private ShoppingCartService shoppingCartService;
@RequestMapping(value = "addtoCart.action")
public String addCart(String id, HttpServletResponse response,
HttpServletRequest request,HttpSession session) {
String cookievalue = "null";
ShoppingCart sc = new ShoppingCart();
Cookie[] cookies = request.getCookies();
Cookie cookie = shoppingCartService.cookieSearch(cookies);
byte[] by = null;
if (cookie != null) {
cookievalue = Base64.encodeBase64String(shoppingCartService
.addShoppingCart(cookie, id).getBytes());
cookie.setValue(cookievalue);
cookie.setMaxAge(60 * 60 * 24 * 6);// 设置cookie过期时间为6天
response.addCookie(cookie);
by = Base64.decodeBase64(cookie.getValue().getBytes());
} else {
Cookie newcookie = new Cookie("shoppingcart", "initial");
String cookievalue2 = Base64.encodeBase64String(shoppingCartService
.addShoppingCart(newcookie, id).getBytes());
newcookie.setValue(cookievalue2);
newcookie.setMaxAge(60 * 60 * 24 * 6);// 设置cookie过期时间为6天
response.addCookie(newcookie);
by = Base64.decodeBase64(newcookie.getValue().getBytes());
}
// 将cookie中的值转换成对象准备回传。
JSONObject scc = JSONObject.fromObject(new String(by));
ShoppingCart shopcart = (ShoppingCart) JSONObject.toBean(scc,
ShoppingCart.class);
session.setAttribute("shoppingcart2", shopcart);
List<CartItem> cartItem = shoppingCartService.generateCartItem(shopcart);
session.setAttribute("cartItem", cartItem);
return "cart";
}
// 跳转购物车
@RequestMapping(value = "/cart")
public String cart(HttpSession session) {
if(session.getAttribute("shoppingcart2")!=null)
{
ShoppingCart shopcart=(ShoppingCart) session.getAttribute("shoppingcart2");
List<CartItem> cartItem = shoppingCartService.generateCartItem(shopcart);
session.setAttribute("cartItem", cartItem);
}
return "cart";
}
@RequestMapping(value="/addGoods")
public void addGoods(HttpServletRequest request,HttpServletResponse response,HttpSession session,String id) throws IOException
{
Cookie[] cookies = request.getCookies();
Cookie cookie = shoppingCartService.cookieSearch(cookies);
String cookievalue = Base64.encodeBase64String(shoppingCartService
.addShoppingCart(cookie, id).getBytes());
cookie.setValue(cookievalue);
cookie.setMaxAge(60 * 60 * 24 * 6);// 设置cookie过期时间为6天
response.addCookie(cookie);
byte [] by =Base64.decodeBase64(cookie.getValue().getBytes());
JSONObject scc = JSONObject.fromObject(new String(by));
ShoppingCart shopcart = (ShoppingCart) JSONObject.toBean(scc,
ShoppingCart.class);
session.setAttribute("shoppingcart2", shopcart);
List<CartItem> cartItem = shoppingCartService.generateCartItem(shopcart);
session.setAttribute("cartItem", cartItem);
//return null;
}
@RequestMapping(value="/subGoods")
public void subGoods(HttpServletRequest request,HttpServletResponse response,HttpSession session,String id) throws IOException
{
Cookie[] cookies = request.getCookies();
Cookie cookie = shoppingCartService.cookieSearch(cookies);
String cookievalue = Base64.encodeBase64String(shoppingCartService
.subShoppingCart(cookie, id).getBytes());
cookie.setValue(cookievalue);
cookie.setMaxAge(60 * 60 * 24 * 6);// 设置cookie过期时间为6天
response.addCookie(cookie);
byte [] by =Base64.decodeBase64(cookie.getValue().getBytes());
JSONObject scc = JSONObject.fromObject(new String(by));
ShoppingCart shopcart = (ShoppingCart) JSONObject.toBean(scc,
ShoppingCart.class);
session.setAttribute("shoppingcart2", shopcart);
List<CartItem> cartItem = shoppingCartService.generateCartItem(shopcart);
session.setAttribute("cartItem", cartItem);
//return null;
}
@RequestMapping(value="/removeGoods")
public String removeGoods(HttpServletRequest request,HttpServletResponse response,HttpSession session,String id)
{
Cookie[] cookies = request.getCookies();
Cookie cookie = shoppingCartService.cookieSearch(cookies);
String cookievalue = Base64.encodeBase64String(shoppingCartService
.subShoppingCartById(cookie, id).getBytes());
cookie.setValue(cookievalue);
cookie.setMaxAge(60 * 60 * 24 * 6);// 设置cookie过期时间为6天
response.addCookie(cookie);
byte [] by =Base64.decodeBase64(cookie.getValue().getBytes());
JSONObject scc = JSONObject.fromObject(new String(by));
ShoppingCart shopcart = (ShoppingCart) JSONObject.toBean(scc,ShoppingCart.class);
session.setAttribute("shoppingcart2", shopcart);
List<CartItem> cartItem = shoppingCartService.generateCartItem(shopcart);
session.setAttribute("cartItem", cartItem);
return "cart";
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
个人花大量时间整理出的真实毕业设计实战成果,内容丰富,文档也很详细。无论做毕业设计还是用于学习技能,或工作中当做参考资料,都能发挥重要作用 亲们下载我任何一个付费资源后,即可私信联系我免费下载其他相关资源哦~ 个人花大量时间整理出的真实毕业设计实战成果,内容丰富,文档也很详细。无论做毕业设计还是用于学习技能,或工作中当做参考资料,都能发挥重要作用 亲们下载我任何一个付费资源后,即可私信联系我免费下载其他相关资源哦~ 个人花大量时间整理出的真实毕业设计实战成果,内容丰富,文档也很详细。无论做毕业设计还是用于学习技能,或工作中当做参考资料,都能发挥重要作用 亲们下载我任何一个付费资源后,即可私信联系我免费下载其他相关资源哦~
资源推荐
资源详情
资源评论
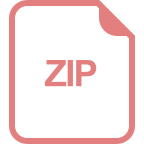
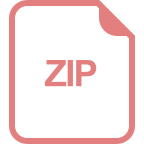
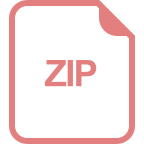
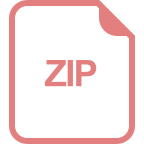
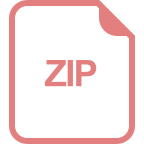
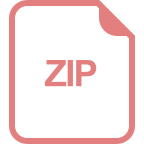
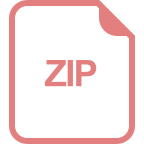
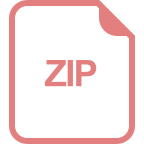
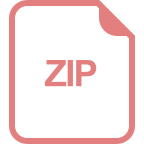
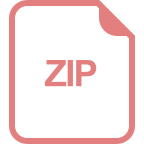
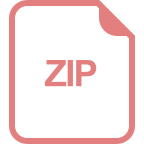
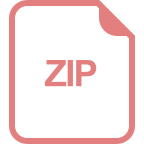
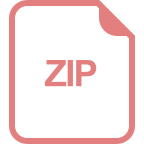
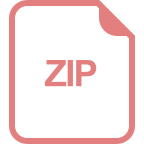
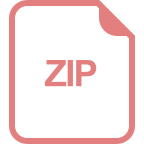
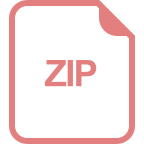
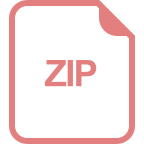
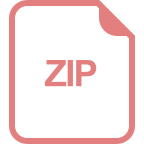
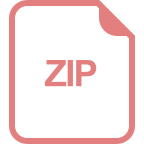
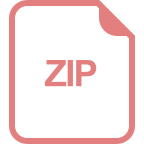
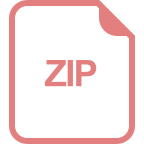
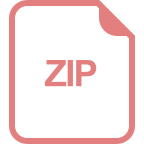
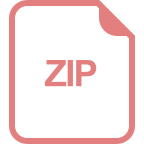
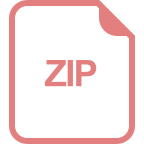
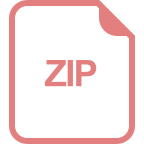
收起资源包目录

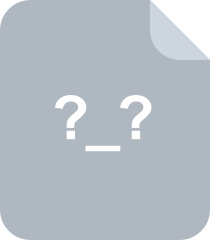
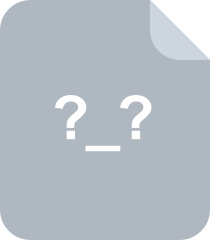
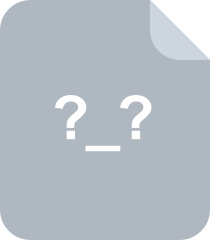
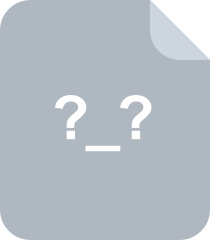
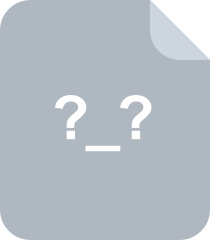
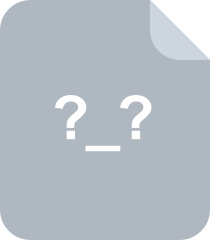
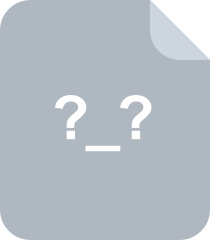
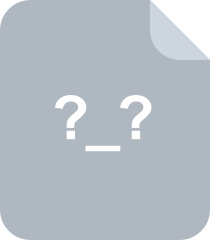
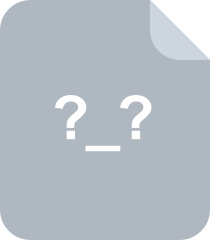
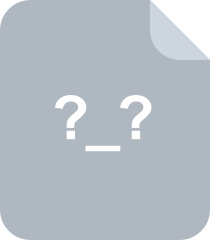
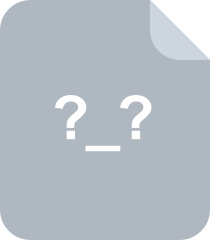
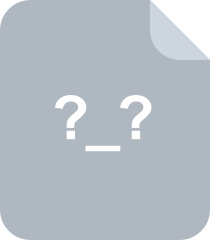
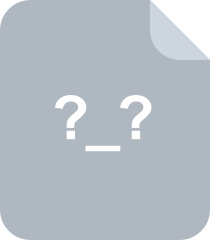
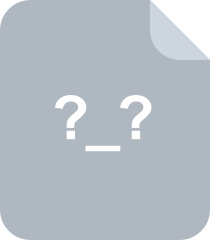
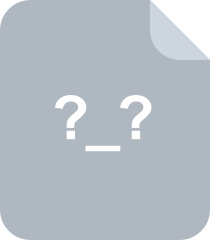
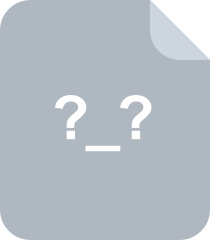
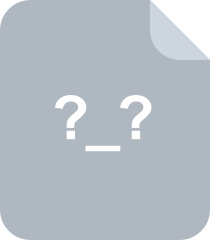
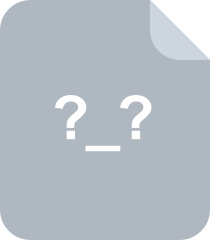
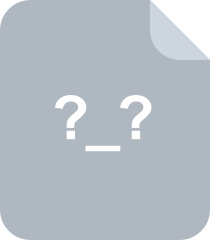
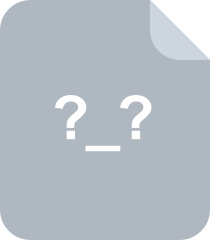
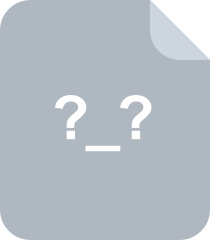
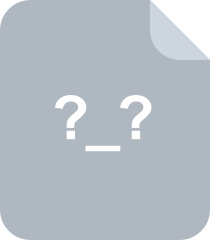
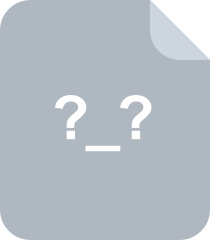
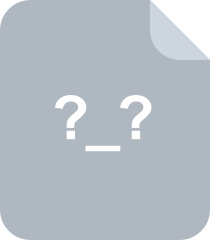
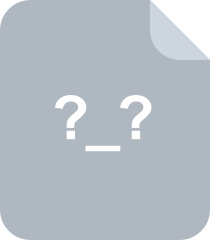
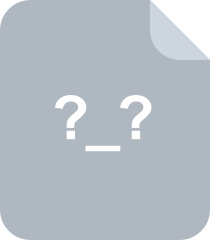
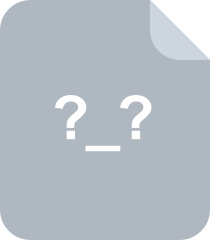
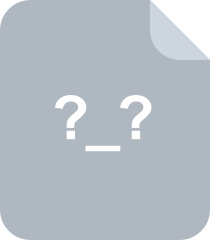
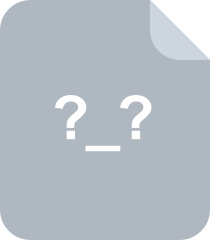
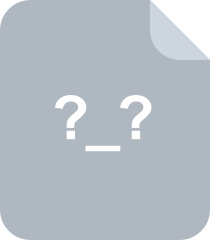
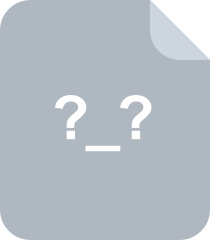
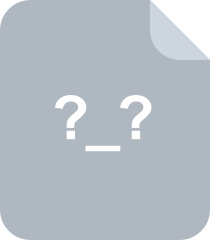
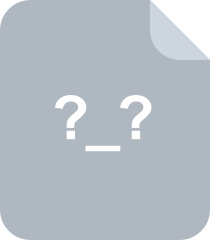
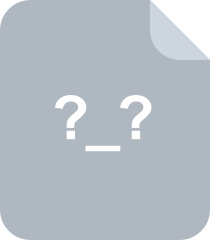
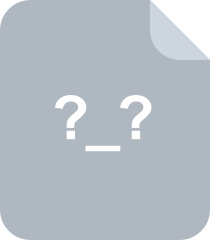
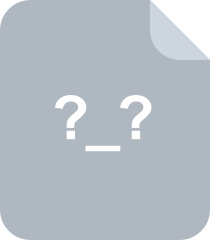
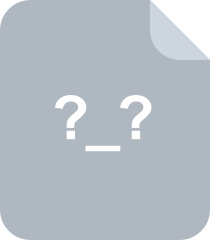
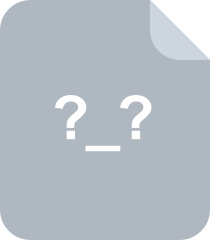
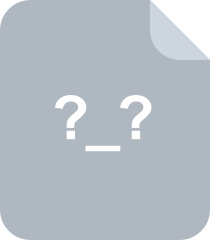
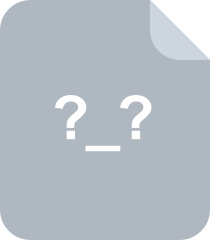
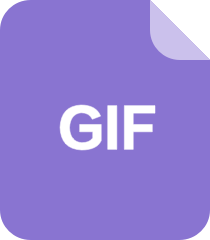
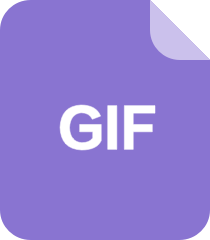
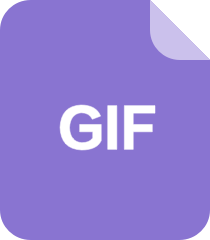
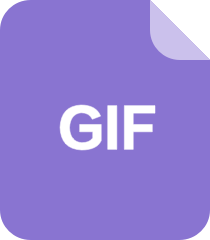
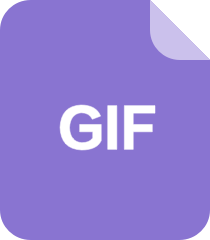
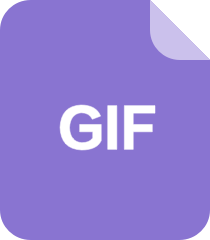
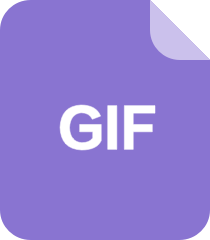
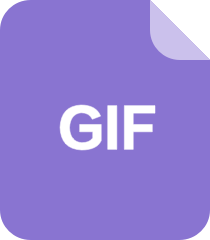
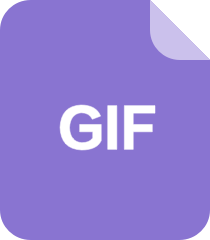
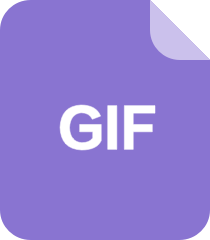
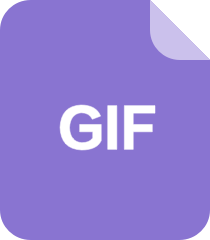
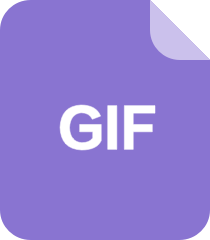
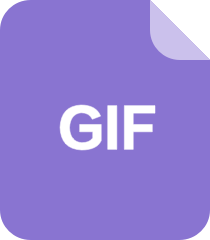
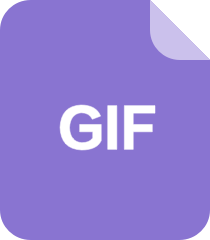
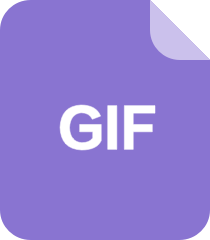
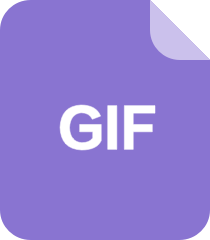
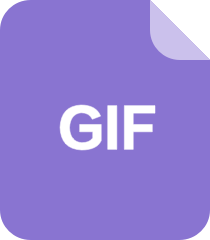
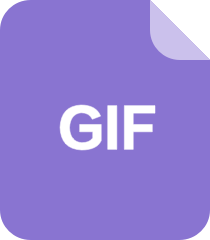
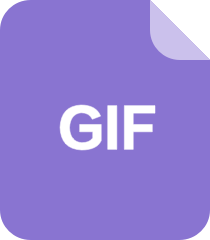
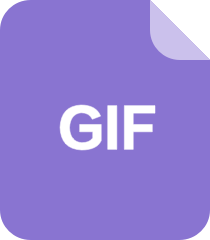
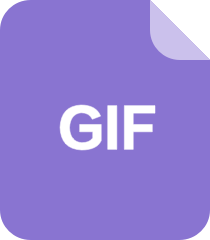
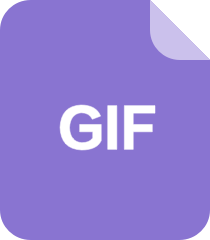
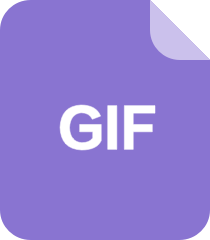
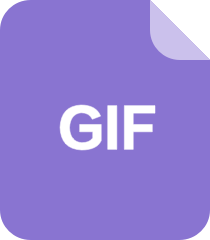
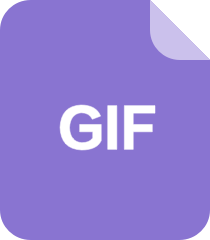
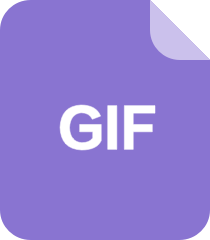
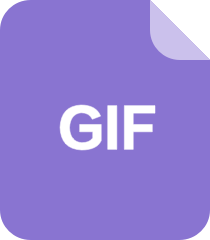
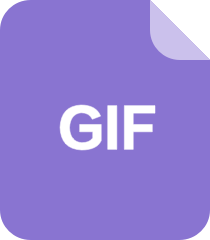
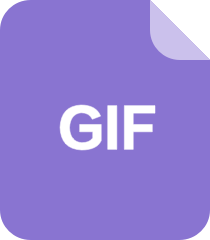
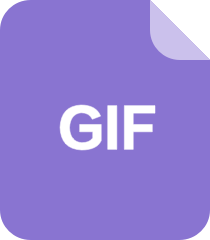
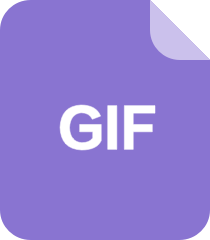
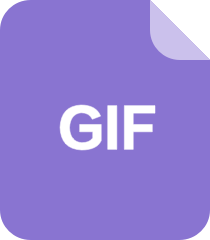
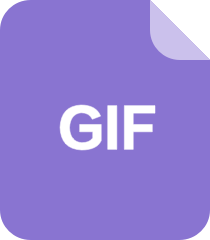
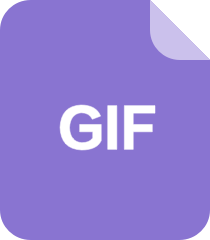
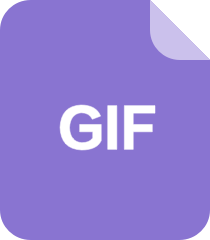
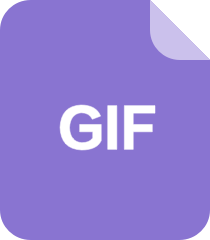
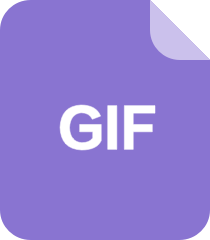
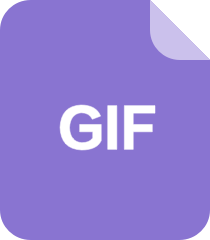
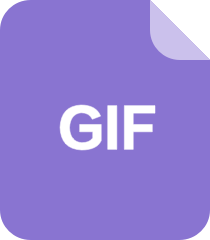
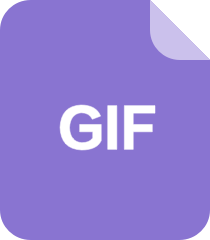
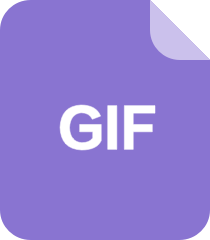
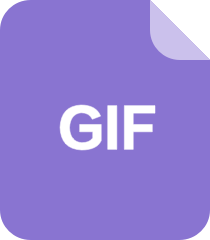
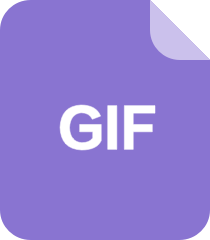
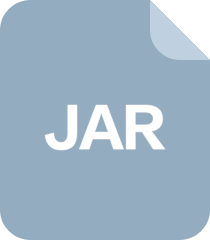
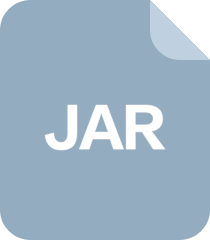
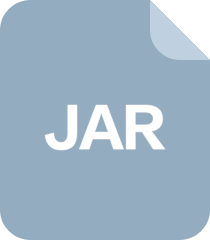
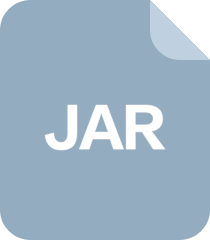
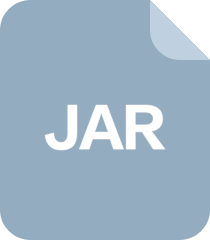
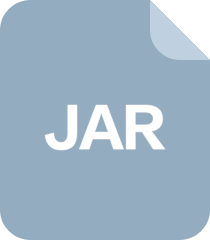
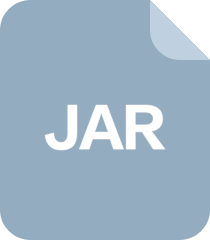
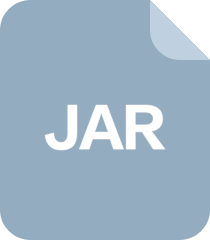
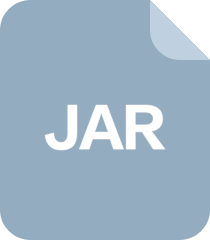
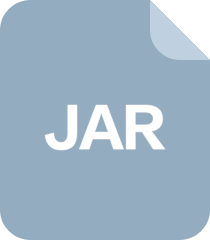
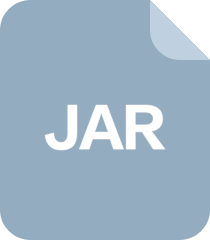
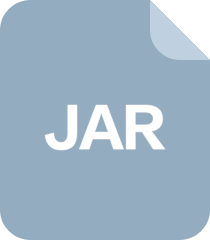
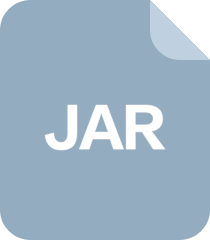
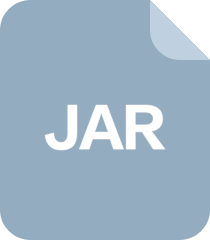
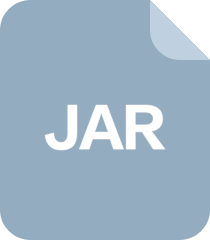
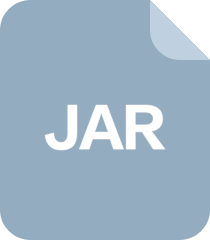
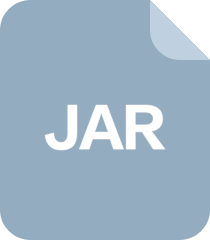
共 218 条
- 1
- 2
- 3
资源评论
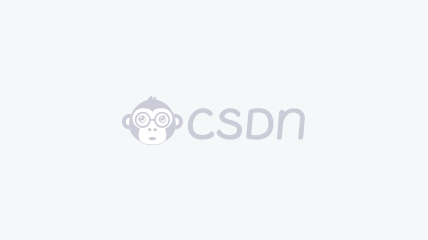

季风泯灭的季节
- 粉丝: 1901
- 资源: 3370

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

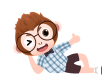
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


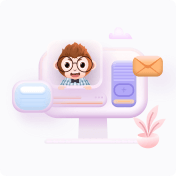
安全验证
文档复制为VIP权益,开通VIP直接复制
