/*
* Copyright (C) 2006 The Android Open Source Project
* Copyright (C) 2013 YIXIA.COM
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.vov.vitamio;
import android.annotation.SuppressLint;
import android.content.ContentResolver;
import android.content.Context;
import android.content.res.AssetFileDescriptor;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.media.AudioFormat;
import android.media.AudioManager;
import android.media.AudioTrack;
import android.net.Uri;
import android.os.Build;
import android.os.Build.VERSION;
import android.os.Bundle;
import android.os.Handler;
import android.os.Looper;
import android.os.Message;
import android.os.PowerManager;
import android.text.TextUtils;
import android.util.SparseArray;
import android.view.Surface;
import android.view.SurfaceHolder;
import io.vov.vitamio.utils.ContextUtils;
import io.vov.vitamio.utils.FileUtils;
import io.vov.vitamio.utils.Log;
import java.io.File;
import java.io.FileDescriptor;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.nio.ByteBuffer;
import java.nio.charset.Charset;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.atomic.AtomicBoolean;
/**
* MediaPlayer class can be used to control playback of audio/video files and
* streams. An example on how to use the methods in this class can be found in
* {@link io.vov.vitamio.widget.VideoView}. This class will function the same as
* android.media.MediaPlayer in most cases. Please see <a
* href="http://developer.android.com/guide/topics/media/index.html">Audio and
* Video</a> for additional help using MediaPlayer.
*/
@SuppressLint("NewApi")
public class MediaPlayer {
public static final int CACHE_TYPE_NOT_AVAILABLE = 1;
public static final int CACHE_TYPE_START = 2;
public static final int CACHE_TYPE_UPDATE = 3;
public static final int CACHE_TYPE_SPEED = 4;
public static final int CACHE_TYPE_COMPLETE = 5;
public static final int CACHE_INFO_NO_SPACE = 1;
public static final int CACHE_INFO_STREAM_NOT_SUPPORT = 2;
public static final int MEDIA_ERROR_UNKNOWN = 1;
public static final int MEDIA_ERROR_NOT_VALID_FOR_PROGRESSIVE_PLAYBACK = 200;
/** File or network related operation errors. */
public static final int MEDIA_ERROR_IO = -5;
/** Bitstream is not conforming to the related coding standard or file spec. */
public static final int MEDIA_ERROR_MALFORMED = -1007;
/** Bitstream is conforming to the related coding standard or file spec, but
* the media framework does not support the feature. */
public static final int MEDIA_ERROR_UNSUPPORTED = -1010;
/** Some operation takes too long to complete, usually more than 3-5 seconds. */
public static final int MEDIA_ERROR_TIMED_OUT = -110;
/**
* The video is too complex for the decoder: it can't decode frames fast
* enough. Possibly only the audio plays fine at this stage.
*
* @see io.vov.vitamio.MediaPlayer.OnInfoListener
*/
public static final int MEDIA_INFO_VIDEO_TRACK_LAGGING = 700;
/**
* MediaPlayer is temporarily pausing playback internally in order to buffer
* more data.
*/
public static final int MEDIA_INFO_BUFFERING_START = 701;
/**
* MediaPlayer is resuming playback after filling buffers.
*/
public static final int MEDIA_INFO_BUFFERING_END = 702;
public static final int MEDIA_INFO_FILE_OPEN_OK = 704;
public static final int MEDIA_INFO_UNKNOW_TYPE = 1001;
public static final int MEDIA_INFO_GET_CODEC_INFO_ERROR = 1002;
/**
* The media cannot be seeked (e.g live stream)
*
* @see io.vov.vitamio.MediaPlayer.OnInfoListener
*/
public static final int MEDIA_INFO_NOT_SEEKABLE = 801;
/**
* The rate in KB/s of av_read_frame()
*
* @see io.vov.vitamio.MediaPlayer.OnInfoListener
*/
public static final int MEDIA_INFO_DOWNLOAD_RATE_CHANGED = 901;
public static final int VIDEOQUALITY_LOW = -16;
public static final int VIDEOQUALITY_MEDIUM = 0;
public static final int VIDEOQUALITY_HIGH = 16;
public static final int VIDEOCHROMA_RGB565 = 0;
public static final int VIDEOCHROMA_RGBA = 1;
/**
* The subtitle displayed is embeded in the movie
*/
public static final int SUBTITLE_INTERNAL = 0;
/**
* The subtitle displayed is an external file
*/
public static final int SUBTITLE_EXTERNAL = 1;
/**
* The external subtitle types which Vitamio supports.
*/
public static final String[] SUB_TYPES = {".srt", ".ssa", ".smi", ".txt", ".sub", ".ass", ".webvtt"};
private static final int MEDIA_NOP = 0;
private static final int MEDIA_PREPARED = 1;
private static final int MEDIA_PLAYBACK_COMPLETE = 2;
private static final int MEDIA_BUFFERING_UPDATE = 3;
private static final int MEDIA_SEEK_COMPLETE = 4;
private static final int MEDIA_SET_VIDEO_SIZE = 5;
private static final int MEDIA_ERROR = 100;
private static final int MEDIA_INFO = 200;
private static final int MEDIA_CACHE = 300;
private static final int MEDIA_HW_ERROR = 400;
private static final int MEDIA_TIMED_TEXT = 1000;
private static final int MEDIA_CACHING_UPDATE = 2000;
private static final String MEDIA_CACHING_SEGMENTS = "caching_segment";
private static final String MEDIA_CACHING_TYPE = "caching_type";
private static final String MEDIA_CACHING_INFO = "caching_info";
private static final String MEDIA_SUBTITLE_STRING = "sub_string";
private static final String MEDIA_SUBTITLE_BYTES = "sub_bytes";
private static final String MEDIA_SUBTITLE_TYPE = "sub_type";
private static final int SUBTITLE_TEXT = 0;
private static final int SUBTITLE_BITMAP = 1;
private static AtomicBoolean NATIVE_OMX_LOADED = new AtomicBoolean(false);
private Context mContext;
private Surface mSurface;
private SurfaceHolder mSurfaceHolder;
private EventHandler mEventHandler;
private PowerManager.WakeLock mWakeLock = null;
private boolean mScreenOnWhilePlaying;
private boolean mStayAwake;
private Metadata mMeta;
private TrackInfo[] mInbandTracks;
private TrackInfo mOutOfBandTracks;
private AssetFileDescriptor mFD = null;
private OnHWRenderFailedListener mOnHWRenderFailedListener;
private OnPreparedListener mOnPreparedListener;
private OnCompletionListener mOnCompletionListener;
private OnBufferingUpdateListener mOnBufferingUpdateListener;
private OnCachingUpdateListener mOnCachingUpdateListener;
private OnSeekCompleteListener mOnSeekCompleteListener;
private OnVideoSizeChangedListener mOnVideoSizeChangedListener;
private OnErrorListener mOnErrorListener;
/**
* Register a callback to be invoked when an info/warning is available.
*
* @param listener
* the callback that will be run
*/
private OnInfoListener mOnInfoListener;
private OnTimedTextListener mOnTimedTextListener;
private AudioTrack mAudioTrack;
private int mAudioTrackBufferSize;
private Surface mLocalSurface;
private Bitmap mBitmap;
private ByteBuffer mByteBuffer;
/**
* Default constructor. The same as Android's MediaPlayer().
* <p>
* When done with the MediaPlayer, you should call {@link #release()}, to free
* the resources. If not released, too many MediaPlayer instances may result
* in an exception.
* </p>
*/
public MediaPlayer(Context ctx) {
this(ctx, false);
}
private static String path;
/**
* Default constructor. The same as Android's MediaPlayer().
* <p>
* When done wit

lly202406
- 粉丝: 3055
- 资源: 5529
最新资源
- 基于.net core的迷你爬虫库高分项目+详细文档+全部资料.zip
- 基于aiohttp、bloomfliter的爬虫框架,爬取所有微博用户高分项目+详细文档+全部资料.zip
- 基于CNN的海贼王人物图像多分类,包含数据集爬虫,数据集处理,模型保存,图表输出,批量测试等,通用模型模板高分项目+详细文档+全部资料.zip
- 基于Beatifulsoup的爬虫,爬取轮船航线高分项目+详细文档+全部资料.zip
- 基于httpclient的清水河畔爬虫高分项目+详细文档+全部资料.zip
- 基于Golang的分布式爬虫管理平台,支持Python、NodeJS、Go、Java、PHP等多种编程语言以及多种爬虫框架。高分项目+详细文档+全部资料.zip
- 基于Node.JS 与puppeteer的纯命令行爬虫软件,以爬取小说网站上的小说资源。高分项目+详细文档+全部资料.zip
- 基于Java爬虫的技术。该案例项目爬取京东的商品展示页面的数据。高分项目+详细文档+全部资料.zip
- 基于java httpparser实现的一个网络爬虫高分项目+详细文档+全部资料.zip
- 基于Python 3的综合性B站(哔哩哔哩弹幕网)数据爬虫。高分项目+详细文档+全部资料.zip
- 基于phpspider的PHP爬虫,爬取一个漫画网站高分项目+详细文档+全部资料.zip
- 基于python3 -先知社区小爬虫,支持关键字搜索和本地图床建立高分项目+详细文档+全部资料.zip
- Lecture 8-August 25.pptx
- 基于Python requests的人人词典数据爬虫 包含:单词、单词词性及翻译、单词发音、单词例句剧照、单词例句及翻译、单词例句发音高分项目+详细文档+全部资料.zip
- 基于Python3的微博爬虫项目,含有按关键字和时间进行微博信息搜索、微博用户资料爬取等功能高分项目+详细文档+全部资料.zip
- 基于Python的scrapy爬虫框架实现爬取招聘网站的信息到数据库高分项目+详细文档+全部资料.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


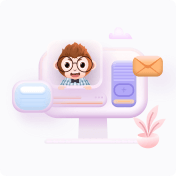