/*
* All content copyright Terracotta, Inc., unless otherwise indicated. All rights reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*
*/
package cn.ponfee.disjob.common.date;
import java.io.Serializable;
import java.text.ParseException;
import java.util.*;
/**
* Provides a parser and evaluator for unix-like cron expressions. Cron
* expressions provide the ability to specify complex time combinations such as
* "At 8:00am every Monday through Friday" or "At 1:30am every
* last Friday of the month".
* <p>
* Cron expressions are comprised of 6 required fields and one optional field
* separated by white space. The fields respectively are described as follows:
* </p>
* <table>
* <caption>Examples of cron expressions and their meanings.</caption>
* <tr>
* <th>Field Name</th>
* <th> </th>
* <th>Allowed Values</th>
* <th> </th>
* <th>Allowed Special Characters</th>
* </tr>
* <tr>
* <td><code>Seconds</code></td>
* <td> </td>
* <td><code>0-59</code></td>
* <td> </td>
* <td><code>, - * /</code></td>
* </tr>
* <tr>
* <td><code>Minutes</code></td>
* <td> </td>
* <td><code>0-59</code></td>
* <td> </td>
* <td><code>, - * /</code></td>
* </tr>
* <tr>
* <td><code>Hours</code></td>
* <td> </td>
* <td><code>0-23</code></td>
* <td> </td>
* <td><code>, - * /</code></td>
* </tr>
* <tr>
* <td><code>Day-of-month</code></td>
* <td> </td>
* <td><code>1-31</code></td>
* <td> </td>
* <td><code>, - * ? / L W</code></td>
* </tr>
* <tr>
* <td><code>Month</code></td>
* <td> </td>
* <td><code>0-11 or JAN-DEC</code></td>
* <td> </td>
* <td><code>, - * /</code></td>
* </tr>
* <tr>
* <td><code>Day-of-Week</code></td>
* <td> </td>
* <td><code>1-7 or SUN-SAT</code></td>
* <td> </td>
* <td><code>, - * ? / L #</code></td>
* </tr>
* <tr>
* <td><code>Year (Optional)</code></td>
* <td> </td>
* <td><code>empty, 1970-2199</code></td>
* <td> </td>
* <td><code>, - * /</code></td>
* </tr>
* </table>
* <p>
* The '*' character is used to specify all values. For example, "*"
* in the minute field means "every minute".
* </p>
* <p>
* The '?' character is allowed for the day-of-month and day-of-week fields. It
* is used to specify 'no specific value'. This is useful when you need to
* specify something in one of the two fields, but not the other.
* <p>
* The '-' character is used to specify ranges For example "10-12" in
* the hour field means "the hours 10, 11 and 12".
* <p>
* The ',' character is used to specify additional values. For example
* "MON,WED,FRI" in the day-of-week field means "the days Monday,
* Wednesday, and Friday".
* </p>
* <p>
* The '/' character is used to specify increments. For example "0/15"
* in the seconds field means "the seconds 0, 15, 30, and 45". And
* "5/15" in the seconds field means "the seconds 5, 20, 35, and
* 50". Specifying '*' before the '/' is equivalent to specifying 0 is
* the value to start with. Essentially, for each field in the expression, there
* is a set of numbers that can be turned on or off. For seconds and minutes,
* the numbers range from 0 to 59. For hours 0 to 23, for days of the month 0 to
* 31, and for months 0 to 11 (JAN to DEC). The "/" character simply helps you turn
* on every "nth" value in the given set. Thus "7/6" in the
* month field only turns on month "7", it does NOT mean every 6th
* month, please note that subtlety.
* </p>
* <p>
* The 'L' character is allowed for the day-of-month and day-of-week fields.
* This character is short-hand for "last", but it has different
* meaning in each of the two fields. For example, the value "L" in
* the day-of-month field means "the last day of the month" - day 31
* for January, day 28 for February on non-leap years. If used in the
* day-of-week field by itself, it simply means "7" or
* "SAT". But if used in the day-of-week field after another value, it
* means "the last xxx day of the month" - for example "6L"
* means "the last friday of the month". You can also specify an offset
* from the last day of the month, such as "L-3" which would mean the third-to-last
* day of the calendar month. <i>When using the 'L' option, it is important not to
* specify lists, or ranges of values, as you'll get confusing/unexpected results.</i>
* </p>
* <p>
* The 'W' character is allowed for the day-of-month field. This character
* is used to specify the weekday (Monday-Friday) nearest the given day. As an
* example, if you were to specify "15W" as the value for the
* day-of-month field, the meaning is: "the nearest weekday to the 15th of
* the month". So if the 15th is a Saturday, the trigger will fire on
* Friday the 14th. If the 15th is a Sunday, the trigger will fire on Monday the
* 16th. If the 15th is a Tuesday, then it will fire on Tuesday the 15th.
* However if you specify "1W" as the value for day-of-month, and the
* 1st is a Saturday, the trigger will fire on Monday the 3rd, as it will not
* 'jump' over the boundary of a month's days. The 'W' character can only be
* specified when the day-of-month is a single day, not a range or list of days.
* </p>
* <p>
* The 'L' and 'W' characters can also be combined for the day-of-month
* expression to yield 'LW', which translates to "last weekday of the
* month".
* </p>
* <p>
* The '#' character is allowed for the day-of-week field. This character is
* used to specify "the nth" XXX day of the month. For example, the
* value of "6#3" in the day-of-week field means the third Friday of
* the month (day 6 = Friday and "#3" = the 3rd one in the month).
* Other examples: "2#1" = the first Monday of the month and
* "4#5" = the fifth Wednesday of the month. Note that if you specify
* "#5" and there is not 5 of the given day-of-week in the month, then
* no firing will occur that month. If the '#' character is used, there can
* only be one expression in the day-of-week field ("3#1,6#3" is
* not valid, since there are two expressions).
* </p>
* <!--The 'C' character is allowed for the day-of-month and day-of-week fields.
* This character is short-hand for "calendar". This means values are
* calculated against the associated calendar, if any. If no calendar is
* associated, then it is equivalent to having an all-inclusive calendar. A
* value of "5C" in the day-of-month field means "the first day included by the
* calendar on or after the 5th". A value of "1C" in the day-of-week field
* means "the first day included by the calendar on or after Sunday".-->
* <p>
* The legal characters and the names of months and days of the week are not
* case sensitive.
*
* <p>
* <b>NOTES:</b>
* </p>
* <ul>
* <li>Support for specifying both a day-of-week and a day-of-month value is
* not complete (you'll need to use the '?' character in one of these fields).
* </li>
* <li>Overflowing ranges is supported - that is, having a larger number on
* the left hand side than the right. You might do 22-2 to catch 10 o'clock
* at night until 2 o'clock in the morning, or you might have NOV-FEB. It is
* very impor

lly202406
- 粉丝: 3036
- 资源: 5531
最新资源
- 快递智能交叉带分拣机3D图纸和工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 毕业设计-基于协议分析的网络取证系统详细文档+全部资料+高分项目.zip
- 毕业设计-基于SPC的产品质量在线分析系统、在线统计过程控制系统,详细文档+全部资料+高分项目.zip
- 基于Android的股票分析系统,包括查询股票信息、模拟炒股、K图分析走势等功能、使用的编程语言是JAVA,编程工具是AndroidStudio3.3、详细文档+全部资料+高分项目.zip
- 基于Android的基金投资分析系统详细文档+全部资料+高分项目.zip
- 基于.NET(C#、VB)仓库管理系统,移动端APP开源项目,支持Android、iOS,SmoWMS仓库管理系统详细文档+全部资料+高分项目.zip
- 基于API HOOK的软件行为分析系统详细文档+全部资料+高分项目.zip
- 基于android系统源代码情景分析,详细文档+全部资料+高分项目.zip
- 基于Django的Web日志分析可视化系统(ECharts+MongoDB)详细文档+全部资料+高分项目.zip
- 基于Django和Vue的学生信息管理及可视化分析系统详细文档+全部资料+高分项目.zip
- 基于Django的的微博转发分析系统详细文档+全部资料+高分项目.zip
- 基于DNSmasq的DNS解析、以及DHCP地址分配系统详细文档+全部资料+高分项目.zip
- 基于Echart+EasyUI+MetroUI+SpringMVC+Hibernate的报表分析系统(连锁酒店)详细文档+全部资料+高分项目.zip
- 基于Flume、Kafka、SparkSql模拟的实时日志分析系统详细文档+全部资料+高分项目.zip
- 基于OCEMOTION的中文微情感分析系统,详细文档+全部资料+高分项目.zip
- 基于LSTM的文本情感分析系统详细文档+全部资料+高分项目.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


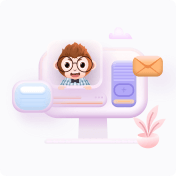