/***
* ASM: a very small and fast Java bytecode manipulation framework
* Copyright (c) 2000-2011 INRIA, France Telecom
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. Neither the name of the copyright holders nor the names of its
* contributors may be used to endorse or promote products derived from
* this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF
* THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.opdar.framework.asm;
/**
* A {@link MethodVisitor} that generates methods in bytecode form. Each visit
* method of this class appends the bytecode corresponding to the visited
* instruction to a byte vector, in the order these methods are called.
*
* @author Eric Bruneton
* @author Eugene Kuleshov
*/
public class MethodWriter extends MethodVisitor {
/**
* Pseudo access flag used to denote constructors.
*/
static final int ACC_CONSTRUCTOR = 0x80000;
/**
* Frame has exactly the same locals as the previous stack map frame and
* number of stack items is zero.
*/
static final int SAME_FRAME = 0; // to 63 (0-3f)
/**
* Frame has exactly the same locals as the previous stack map frame and
* number of stack items is 1
*/
static final int SAME_LOCALS_1_STACK_ITEM_FRAME = 64; // to 127 (40-7f)
/**
* Reserved for future use
*/
static final int RESERVED = 128;
/**
* Frame has exactly the same locals as the previous stack map frame and
* number of stack items is 1. Offset is bigger then 63;
*/
static final int SAME_LOCALS_1_STACK_ITEM_FRAME_EXTENDED = 247; // f7
/**
* Frame where current locals are the same as the locals in the previous
* frame, except that the k last locals are absent. The value of k is given
* by the formula 251-frame_type.
*/
static final int CHOP_FRAME = 248; // to 250 (f8-fA)
/**
* Frame has exactly the same locals as the previous stack map frame and
* number of stack items is zero. Offset is bigger then 63;
*/
static final int SAME_FRAME_EXTENDED = 251; // fb
/**
* Frame where current locals are the same as the locals in the previous
* frame, except that k additional locals are defined. The value of k is
* given by the formula frame_type-251.
*/
static final int APPEND_FRAME = 252; // to 254 // fc-fe
/**
* Full frame
*/
static final int FULL_FRAME = 255; // ff
/**
* Indicates that the stack map frames must be recomputed from scratch. In
* this case the maximum stack size and number of local variables is also
* recomputed from scratch.
*
* @see #compute
*/
private static final int FRAMES = 0;
/**
* Indicates that the maximum stack size and number of local variables must
* be automatically computed.
*
* @see #compute
*/
private static final int MAXS = 1;
/**
* Indicates that nothing must be automatically computed.
*
* @see #compute
*/
private static final int NOTHING = 2;
/**
* The class writer to which this method must be added.
*/
final ClassWriter cw;
/**
* Access flags of this method.
*/
private int access;
/**
* The index of the constant pool item that contains the name of this
* method.
*/
private final int name;
/**
* The index of the constant pool item that contains the descriptor of this
* method.
*/
private final int desc;
/**
* The descriptor of this method.
*/
private final String descriptor;
/**
* The signature of this method.
*/
String signature;
/**
* If not zero, indicates that the code of this method must be copied from
* the ClassReader associated to this writer in <code>cw.cr</code>. More
* precisely, this field gives the index of the first byte to copied from
* <code>cw.cr.b</code>.
*/
int classReaderOffset;
/**
* If not zero, indicates that the code of this method must be copied from
* the ClassReader associated to this writer in <code>cw.cr</code>. More
* precisely, this field gives the number of bytes to copied from
* <code>cw.cr.b</code>.
*/
int classReaderLength;
/**
* Number of exceptions that can be thrown by this method.
*/
int exceptionCount;
/**
* The exceptions that can be thrown by this method. More precisely, this
* array contains the indexes of the constant pool items that contain the
* internal names of these exception classes.
*/
int[] exceptions;
/**
* The annotation default attribute of this method. May be <tt>null</tt>.
*/
private ByteVector annd;
/**
* The runtime visible annotations of this method. May be <tt>null</tt>.
*/
private AnnotationWriter anns;
/**
* The runtime invisible annotations of this method. May be <tt>null</tt>.
*/
private AnnotationWriter ianns;
/**
* The runtime visible type annotations of this method. May be <tt>null</tt>
* .
*/
private AnnotationWriter tanns;
/**
* The runtime invisible type annotations of this method. May be
* <tt>null</tt>.
*/
private AnnotationWriter itanns;
/**
* The runtime visible parameter annotations of this method. May be
* <tt>null</tt>.
*/
private AnnotationWriter[] panns;
/**
* The runtime invisible parameter annotations of this method. May be
* <tt>null</tt>.
*/
private AnnotationWriter[] ipanns;
/**
* The number of synthetic parameters of this method.
*/
private int synthetics;
/**
* The non standard attributes of the method.
*/
private Attribute attrs;
/**
* The bytecode of this method.
*/
private ByteVector code = new ByteVector();
/**
* Maximum stack size of this method.
*/
private int maxStack;
/**
* Maximum number of local variables for this method.
*/
private int maxLocals;
/**
* Number of local variables in the current stack map frame.
*/
private int currentLocals;
/**
* Number of stack map frames in the StackMapTable attribute.
*/
private int frameCount;
/**
* The StackMapTable attribute.
*/
private ByteVector stackMap;
/**
* The offset of the last frame that was written in the StackMapTable
* attribute.
*/
private int previousFrameOffset;
/**
* The last frame that was written in the StackMapTable attribute.
*
* @see #frame
*/
private int[] previousFrame;
/**
* The current stack map fram
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目是一款基于Java和HTML的全栈式Seed快速Web开发框架,源码包含339个文件,其中276个为Java源文件,34个为XML配置文件,9个为IML配置文件,4个为Proto文件,3个为HTML文件,2个为属性文件,1个为Git忽略文件和1个README文件。该框架专注于提升Web开发效率,适用于需要快速构建Web应用的场景。
资源推荐
资源详情
资源评论
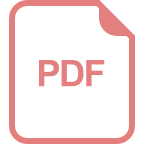
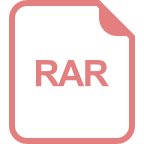
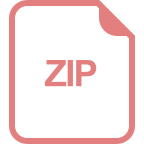
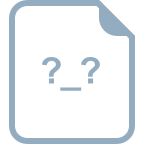
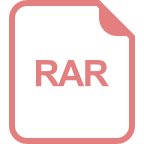
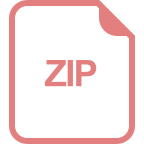
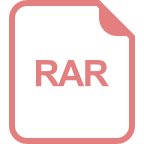
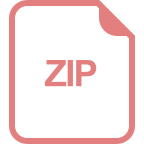
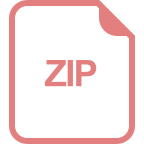
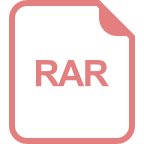
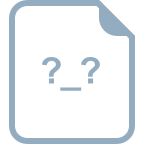
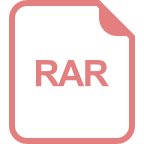
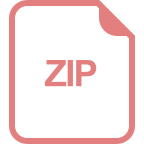
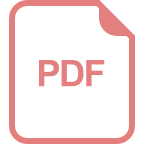
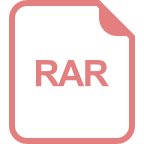
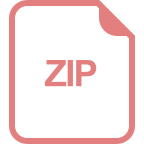
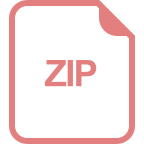
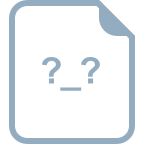
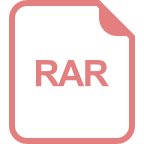
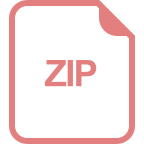
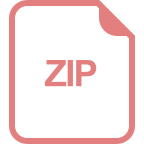
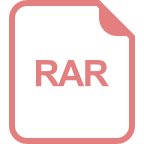
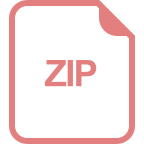
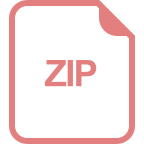
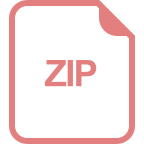
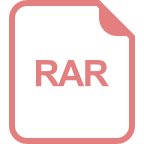
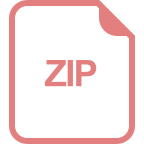
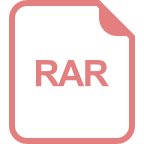
收起资源包目录

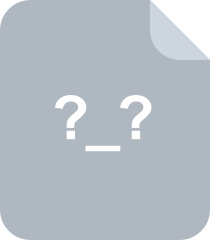
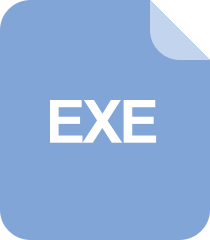
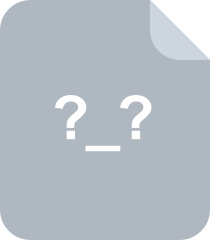
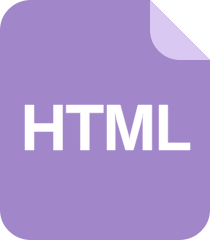
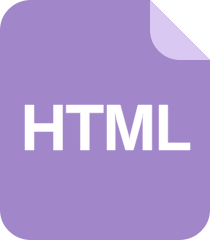
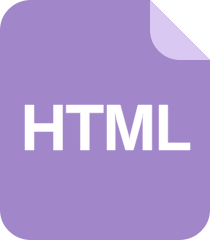
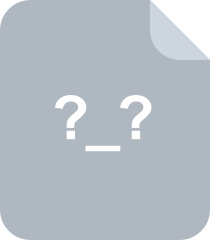
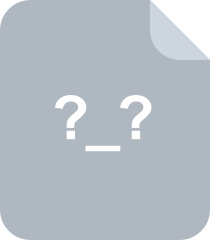
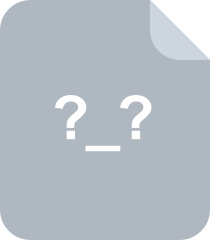
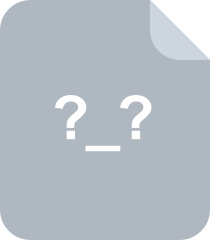
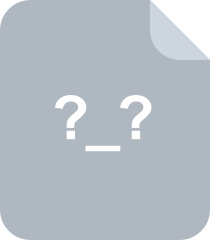
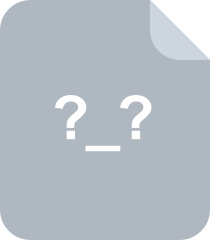
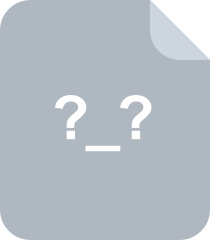
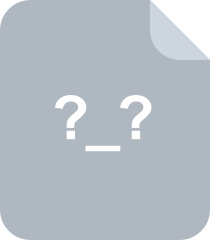
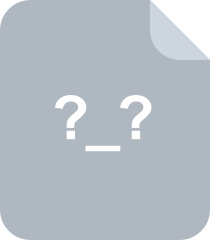
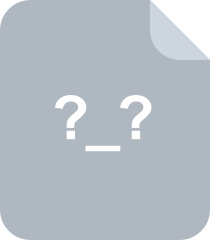
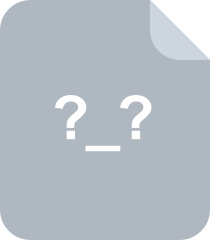
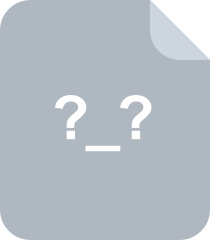
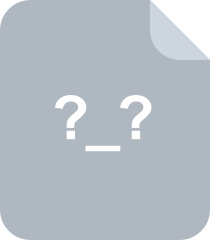
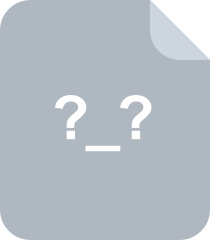
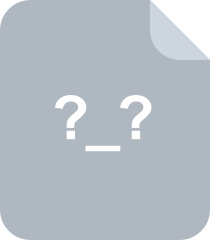
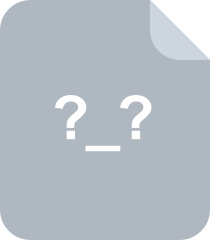
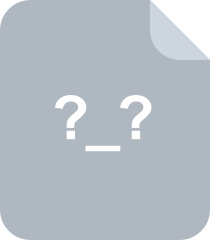
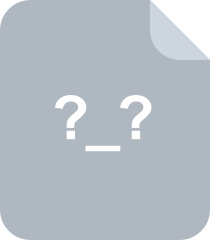
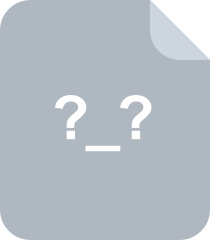
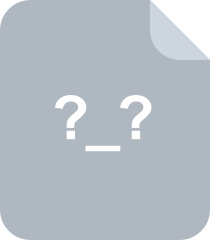
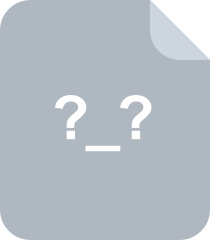
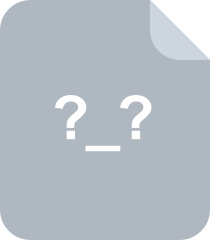
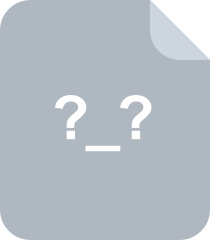
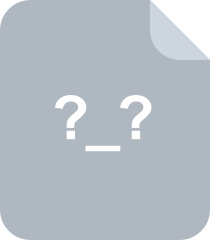
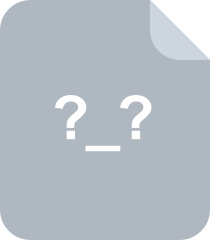
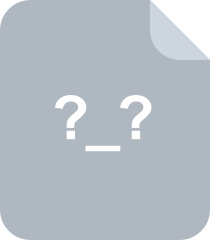
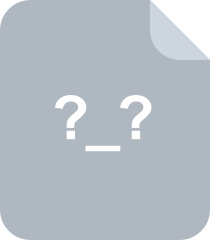
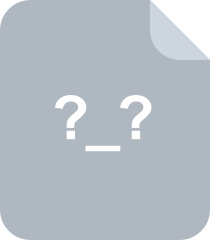
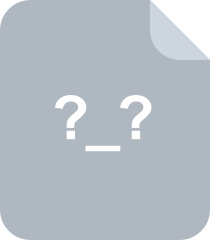
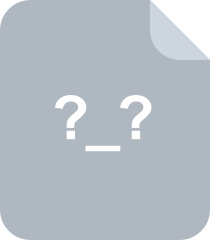
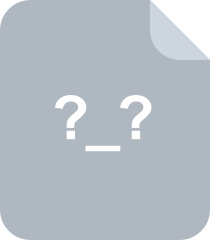
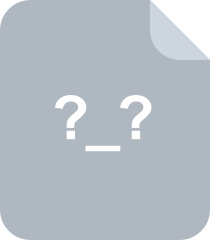
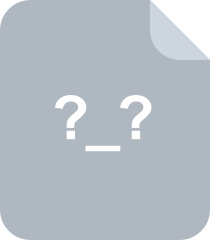
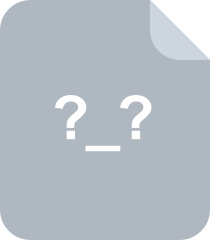
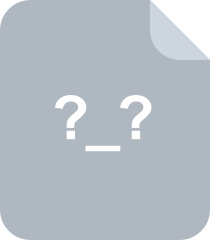
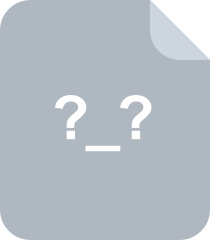
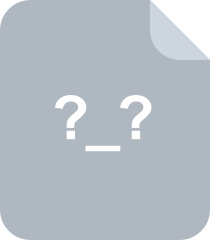
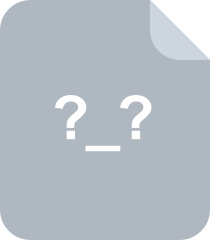
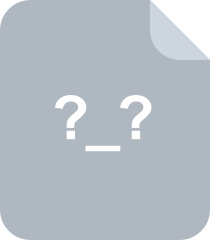
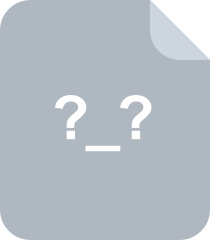
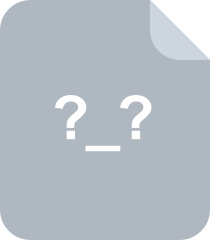
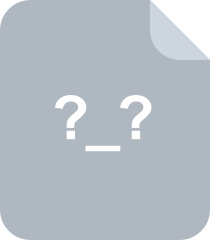
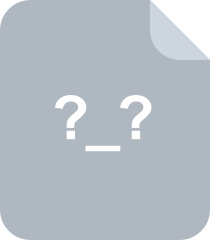
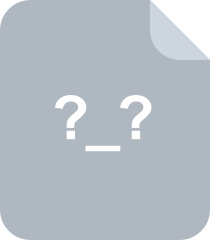
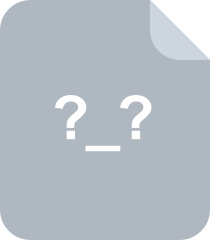
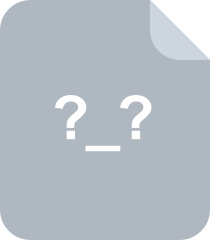
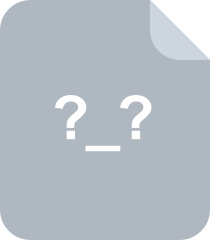
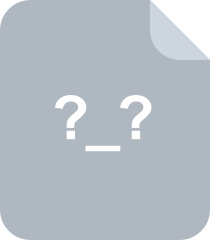
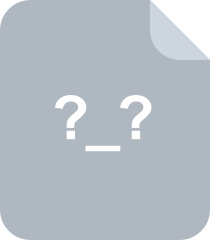
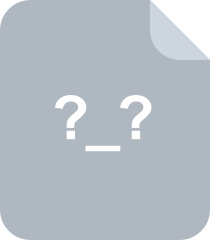
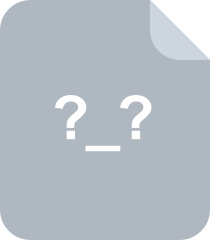
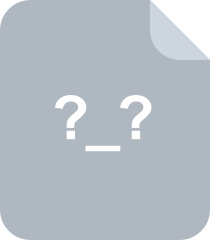
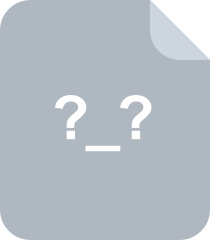
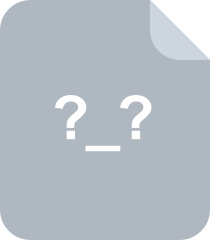
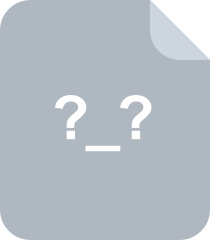
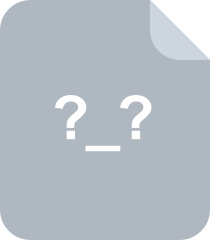
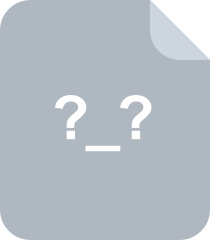
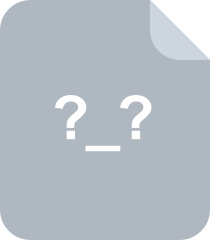
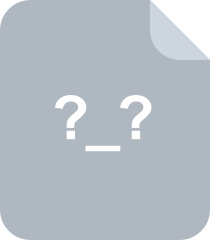
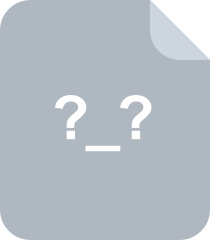
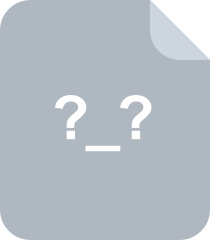
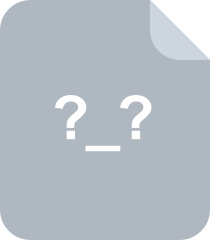
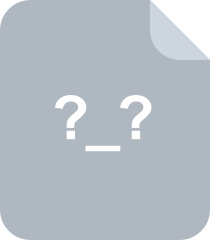
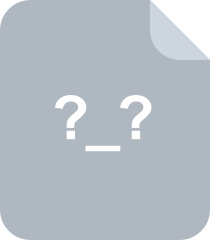
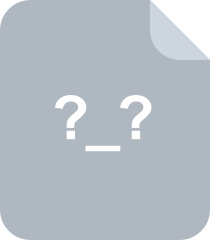
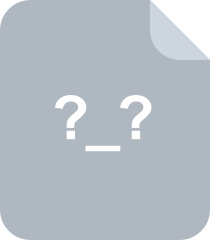
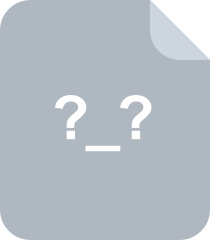
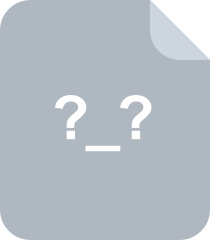
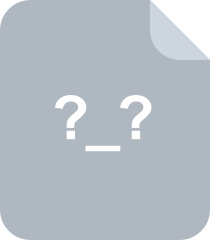
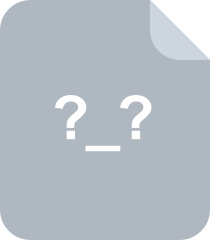
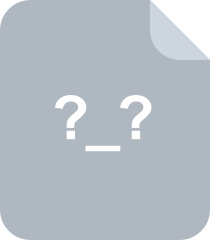
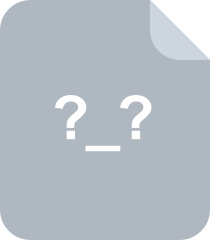
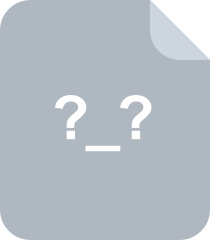
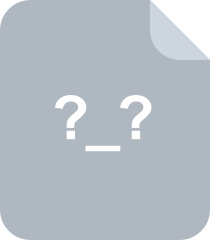
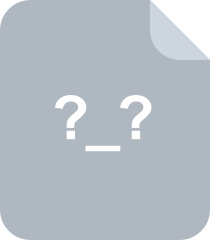
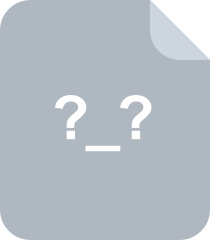
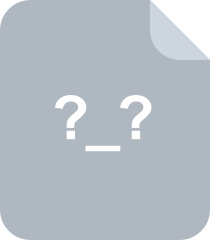
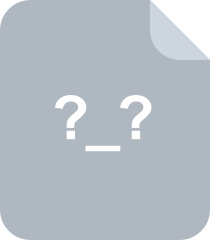
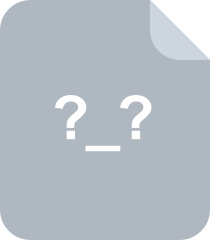
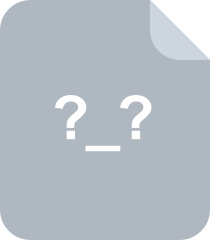
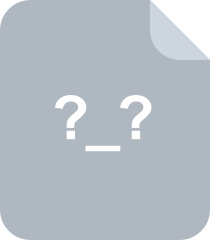
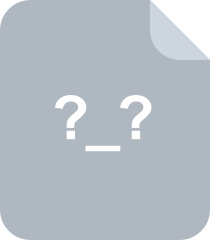
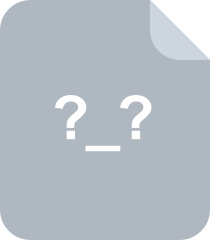
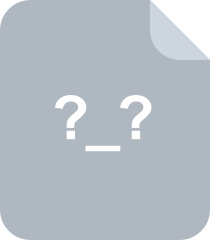
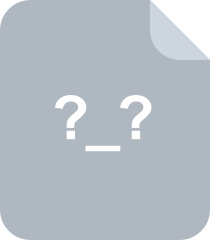
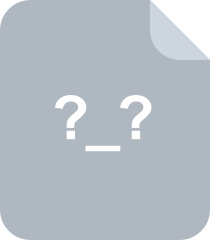
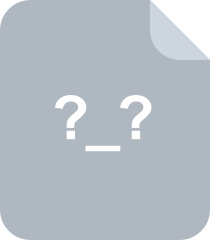
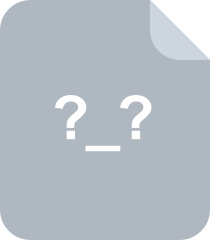
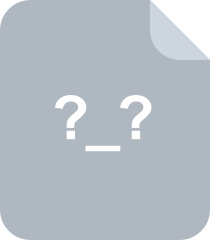
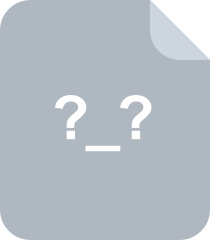
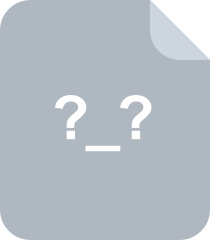
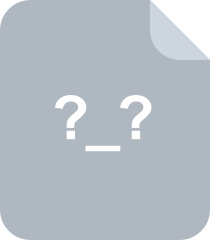
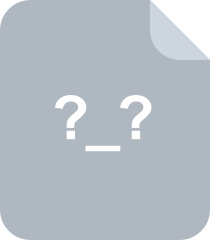
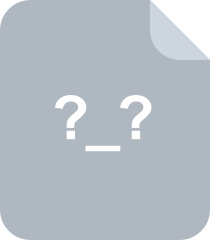
共 339 条
- 1
- 2
- 3
- 4
资源评论
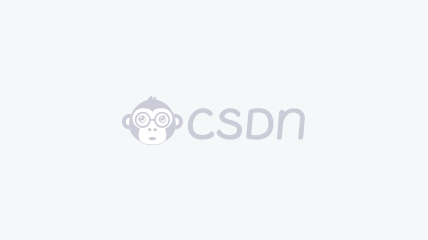

lly202406
- 粉丝: 2613
- 资源: 5446
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

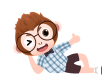
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


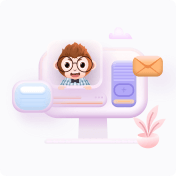
安全验证
文档复制为VIP权益,开通VIP直接复制
