/*
Copyright 2013-2015 David Morrissey
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*/
package com.vondear.rxtools.view.scaleimage;
import android.content.ContentResolver;
import android.content.Context;
import android.content.res.TypedArray;
import android.database.Cursor;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.graphics.Color;
import android.graphics.Matrix;
import android.graphics.Paint;
import android.graphics.Paint.Style;
import android.graphics.Point;
import android.graphics.PointF;
import android.graphics.Rect;
import android.graphics.RectF;
import android.media.ExifInterface;
import android.net.Uri;
import android.os.AsyncTask;
import android.os.Build.VERSION;
import android.os.Handler;
import android.os.Message;
import android.provider.MediaStore;
import android.support.annotation.AnyThread;
import android.support.annotation.NonNull;
import android.util.AttributeSet;
import android.util.DisplayMetrics;
import android.util.Log;
import android.util.TypedValue;
import android.view.GestureDetector;
import android.view.MotionEvent;
import android.view.View;
import android.view.ViewParent;
import com.vondear.rxtools.R;
import com.vondear.rxtools.view.scaleimage.decoder.CompatDecoderFactory;
import com.vondear.rxtools.view.scaleimage.decoder.DecoderFactory;
import com.vondear.rxtools.view.scaleimage.decoder.ImageDecoder;
import com.vondear.rxtools.view.scaleimage.decoder.ImageRegionDecoder;
import com.vondear.rxtools.view.scaleimage.decoder.SkiaImageDecoder;
import com.vondear.rxtools.view.scaleimage.decoder.SkiaImageRegionDecoder;
import java.lang.ref.WeakReference;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.concurrent.Executor;
/**
* Displays an image subsampled as necessary to avoid loading too much image data into memory. After a pinch to zoom in,
* a set of image tiles subsampled at higher resolution are loaded and displayed over the base layer. During pinch and
* zoom, tiles off screen or higher/lower resolution than required are discarded from memory.
* <p>
* Tiles are no larger than the max supported bitmap size, so with large images tiling may be used even when zoomed out.
* <p>
* v prefixes - coordinates, translations and distances measured in screen (view) pixels
* s prefixes - coordinates, translations and distances measured in source image pixels (scaled)
*/
@SuppressWarnings("unused")
public class RxScaleImageView extends View {
/**
* Attempt to use EXIF information on the image to rotate it. Works for external files only.
*/
public static final int ORIENTATION_USE_EXIF = -1;
/**
* Display the image file in its native orientation.
*/
public static final int ORIENTATION_0 = 0;
/**
* Rotate the image 90 degrees clockwise.
*/
public static final int ORIENTATION_90 = 90;
/**
* Rotate the image 180 degrees.
*/
public static final int ORIENTATION_180 = 180;
/**
* Rotate the image 270 degrees clockwise.
*/
public static final int ORIENTATION_270 = 270;
/**
* During zoom animation, keep the point of the image that was tapped in the same place, and scale the image around it.
*/
public static final int ZOOM_FOCUS_FIXED = 1;
/**
* During zoom animation, move the point of the image that was tapped to the center of the screen.
*/
public static final int ZOOM_FOCUS_CENTER = 2;
/**
* Zoom in to and center the tapped point immediately without animating.
*/
public static final int ZOOM_FOCUS_CENTER_IMMEDIATE = 3;
/**
* Quadratic ease out. Not recommended for scale animation, but good for panning.
*/
public static final int EASE_OUT_QUAD = 1;
/**
* Quadratic ease in and out.
*/
public static final int EASE_IN_OUT_QUAD = 2;
/**
* Don't allow the image to be panned off screen. As much of the image as possible is always displayed, centered in the view when it is smaller. This is the best option for galleries.
*/
public static final int PAN_LIMIT_INSIDE = 1;
/**
* Allows the image to be panned until it is just off screen, but no further. The edge of the image will stop when it is flush with the screen edge.
*/
public static final int PAN_LIMIT_OUTSIDE = 2;
/**
* Allows the image to be panned until a corner reaches the center of the screen but no further. Useful when you want to pan any spot on the image to the exact center of the screen.
*/
public static final int PAN_LIMIT_CENTER = 3;
/**
* Scale the image so that both dimensions of the image will be equal to or less than the corresponding dimension of the view. The image is then centered in the view. This is the default behaviour and best for galleries.
*/
public static final int SCALE_TYPE_CENTER_INSIDE = 1;
/**
* Scale the image uniformly so that both dimensions of the image will be equal to or larger than the corresponding dimension of the view. The image is then centered in the view.
*/
public static final int SCALE_TYPE_CENTER_CROP = 2;
/**
* Scale the image so that both dimensions of the image will be equal to or less than the maxScale and equal to or larger than minScale. The image is then centered in the view.
*/
public static final int SCALE_TYPE_CUSTOM = 3;
/**
* State change originated from animation.
*/
public static final int ORIGIN_ANIM = 1;
/**
* State change originated from touch gesture.
*/
public static final int ORIGIN_TOUCH = 2;
/**
* State change originated from a fling momentum anim.
*/
public static final int ORIGIN_FLING = 3;
/**
* State change originated from a double tap zoom anim.
*/
public static final int ORIGIN_DOUBLE_TAP_ZOOM = 4;
private static final String TAG = RxScaleImageView.class.getSimpleName();
private static final List<Integer> VALID_ORIENTATIONS = Arrays.asList(ORIENTATION_0, ORIENTATION_90, ORIENTATION_180, ORIENTATION_270, ORIENTATION_USE_EXIF);
private static final List<Integer> VALID_ZOOM_STYLES = Arrays.asList(ZOOM_FOCUS_FIXED, ZOOM_FOCUS_CENTER, ZOOM_FOCUS_CENTER_IMMEDIATE);
private static final List<Integer> VALID_EASING_STYLES = Arrays.asList(EASE_IN_OUT_QUAD, EASE_OUT_QUAD);
private static final List<Integer> VALID_PAN_LIMITS = Arrays.asList(PAN_LIMIT_INSIDE, PAN_LIMIT_OUTSIDE, PAN_LIMIT_CENTER);
private static final List<Integer> VALID_SCALE_TYPES = Arrays.asList(SCALE_TYPE_CENTER_CROP, SCALE_TYPE_CENTER_INSIDE, SCALE_TYPE_CUSTOM);
private static final int MESSAGE_LONG_CLICK = 1;
// overrides for the dimensions of the generated tiles
public static int TILE_SIZE_AUTO = Integer.MAX_VALUE;
private final Object decoderLock = new Object();
// Current quickscale state
private final float quickScaleThreshold;
// Bitmap (preview or full image)
private Bitmap bitmap;
// Whether the bitmap is a preview image
private boolean bitmapIsPreview;
// Specifies if a cache handler is also referencing the bitmap. Do not recycle if so.
private boolean bitmapIsCached;
// Uri of full size image
private Uri uri;
// Sample size used to display the whole image when fully zoomed out
private int fullImageSampleSize;
// Map of
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目是一个基于Java语言的Android开发工具类集合源码,总计包含665个文件,其中Java源文件323个,XML配置文件152个,PNG图片文件158个,以及其他类型文件如JPG、Gradle、JAR等。集合涵盖了支付宝支付、微信支付、微信分享、UCrop头像选择、二维码条形码生成、常用Dialog、WebView视频播放、滑动验证码、Toast封装、震动、GPS定位、压缩加密、图片缩放、Exif地理位置添加、颜色选择器等功能,旨在为Android开发者提供一站式集成解决方案,编译运行后可发现更多惊喜功能。
资源推荐
资源详情
资源评论
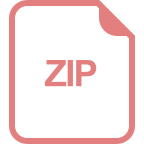
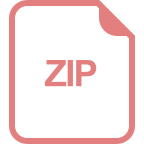
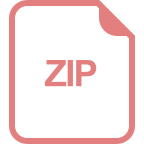
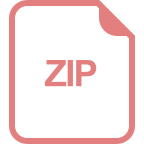
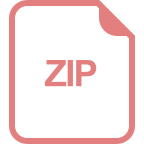
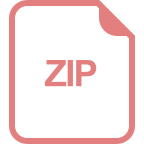
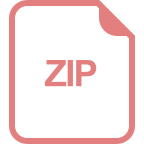
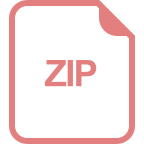
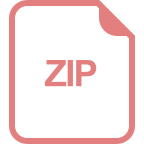
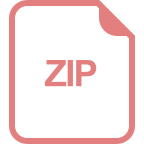
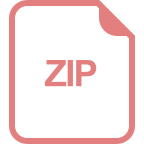
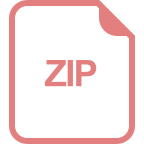
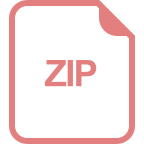
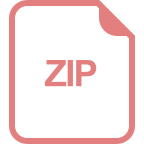
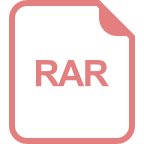
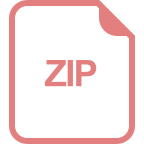
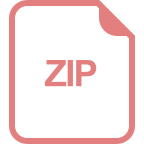
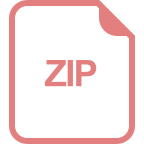
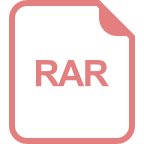
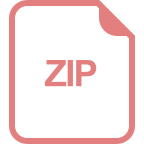
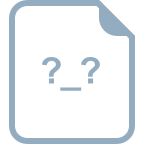
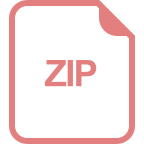
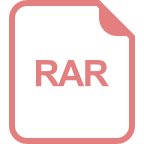
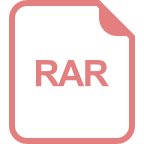
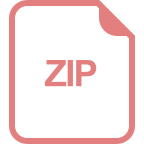
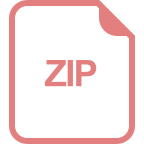
收起资源包目录

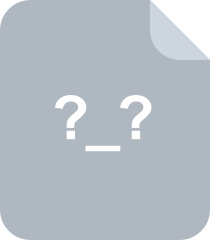
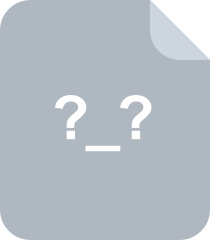
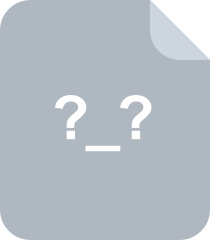
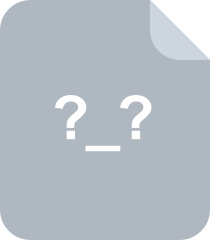
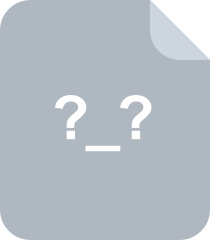
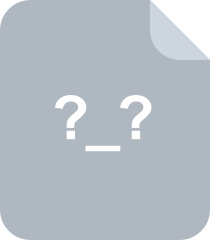
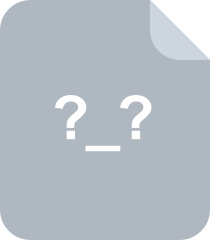
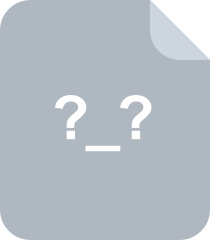
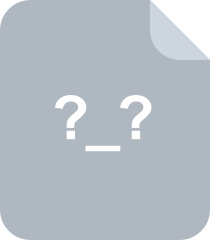
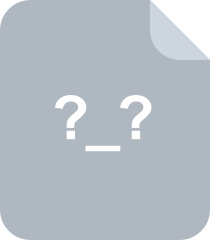
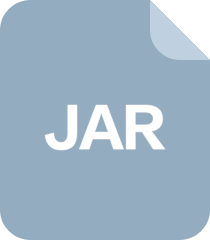
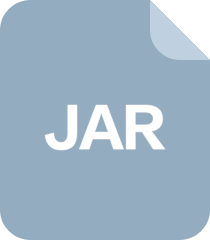
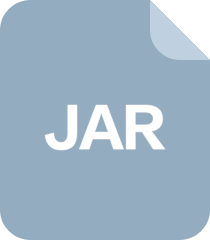
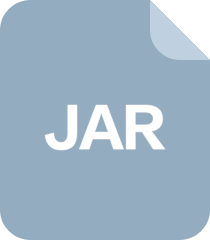
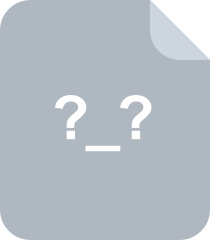
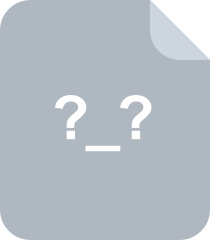
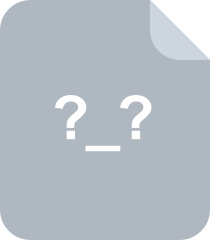
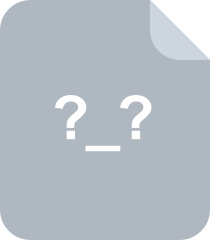
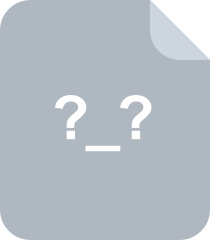
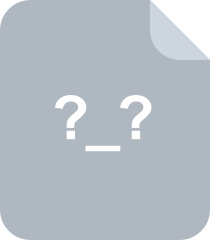
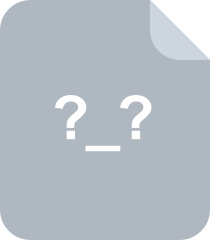
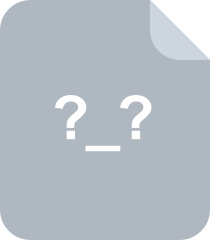
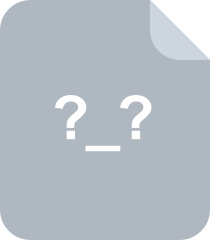
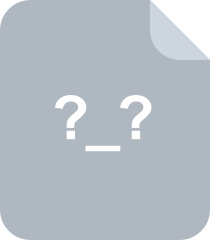
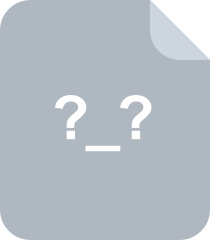
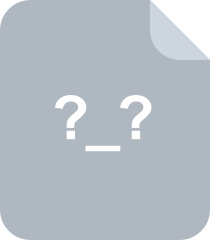
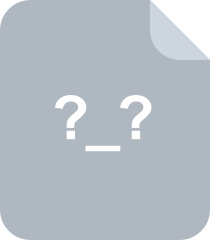
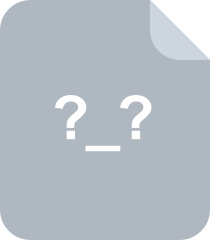
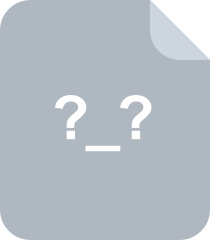
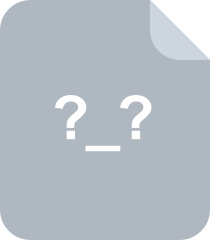
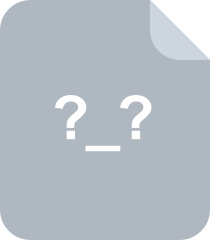
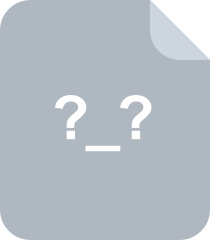
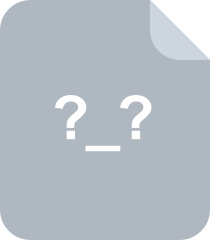
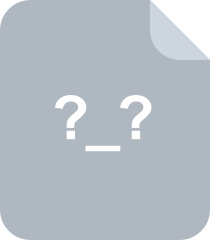
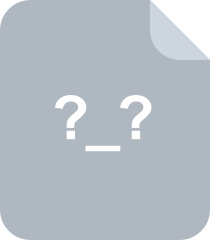
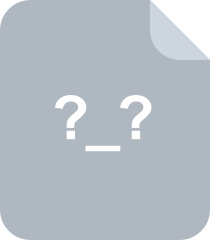
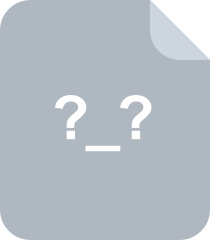
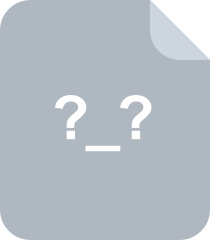
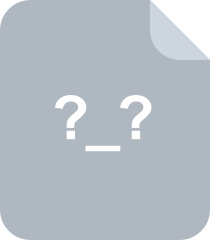
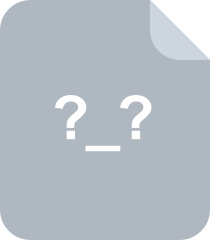
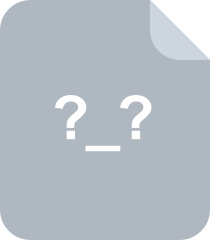
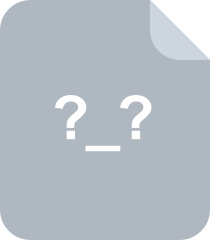
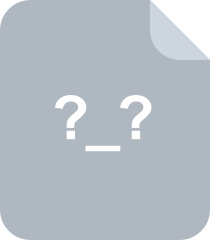
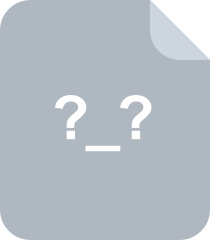
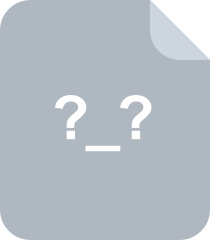
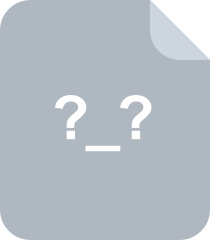
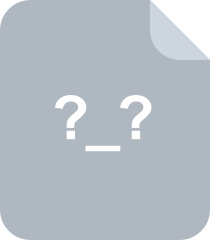
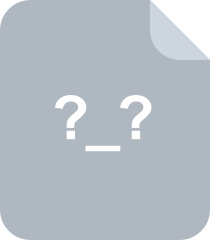
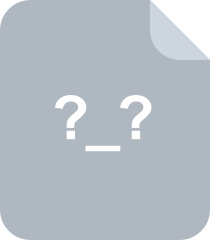
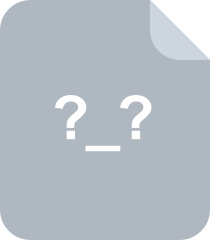
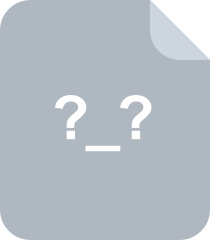
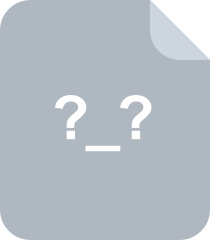
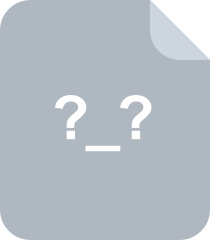
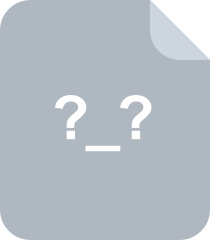
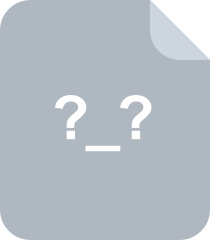
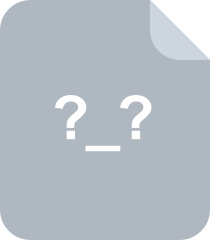
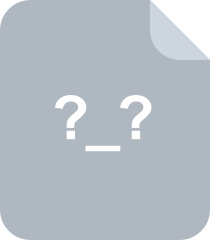
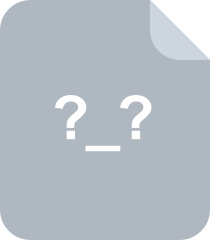
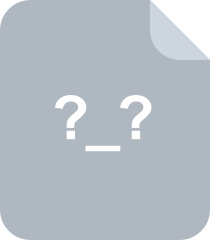
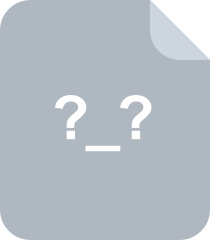
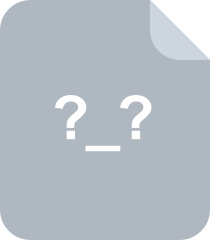
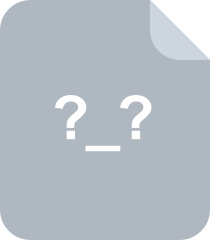
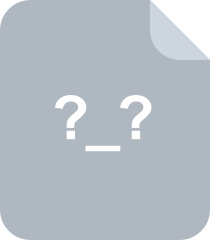
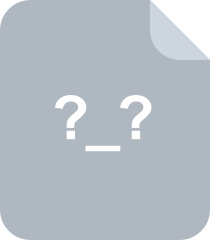
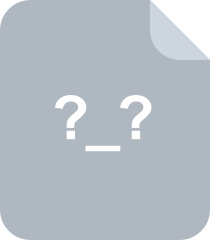
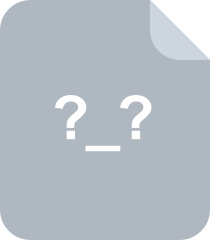
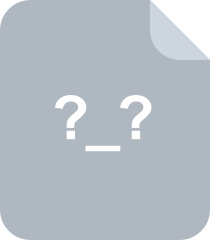
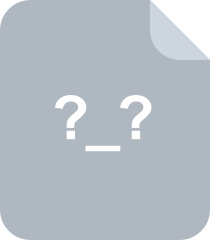
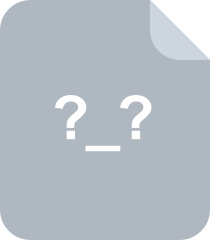
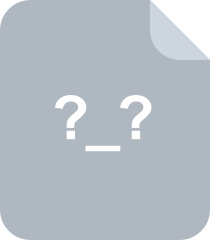
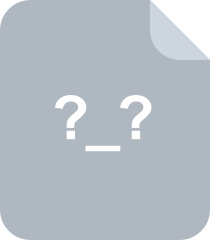
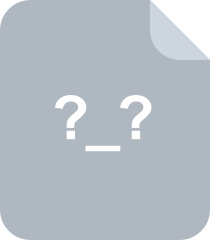
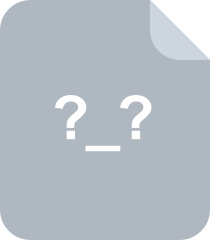
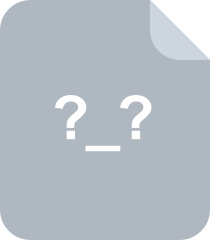
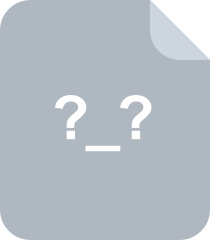
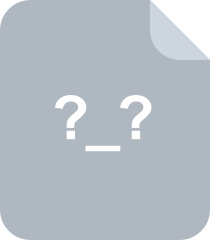
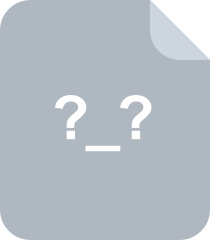
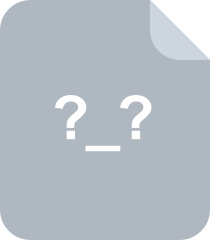
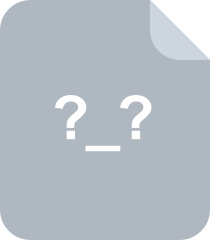
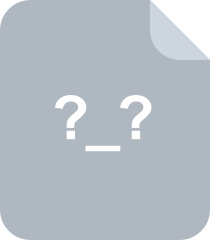
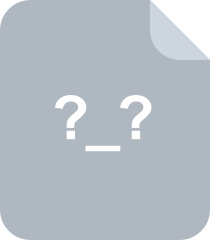
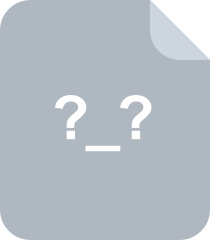
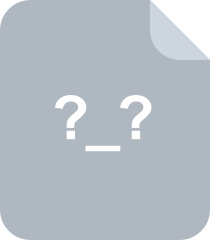
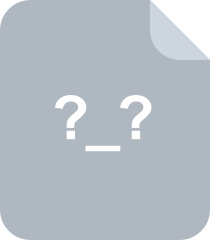
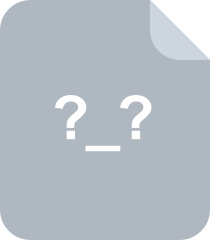
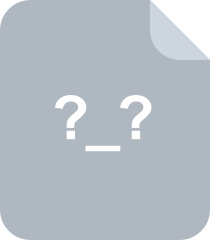
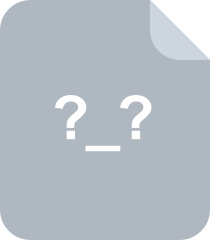
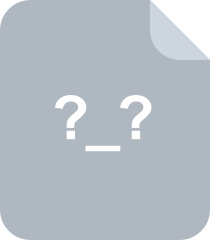
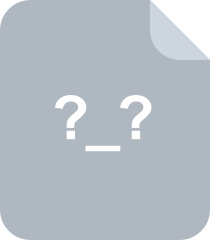
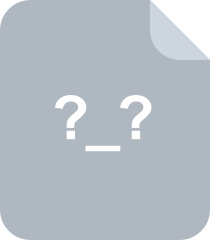
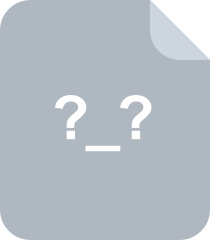
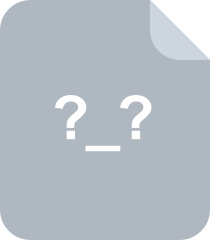
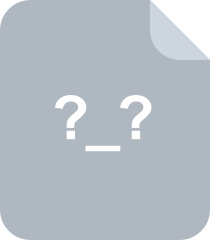
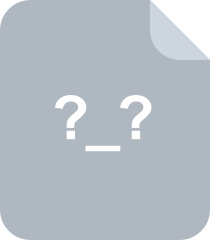
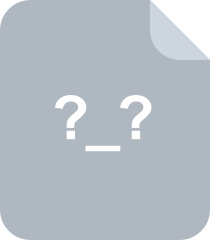
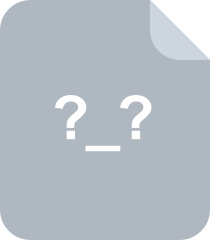
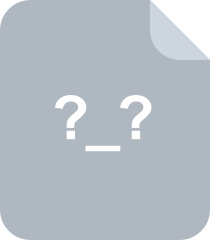
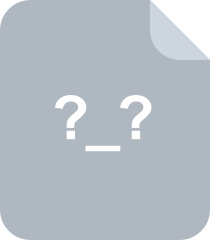
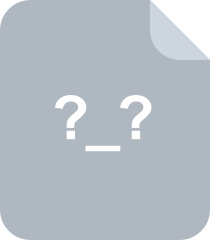
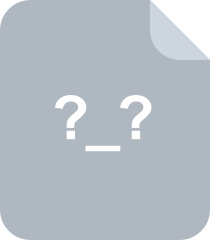
共 665 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
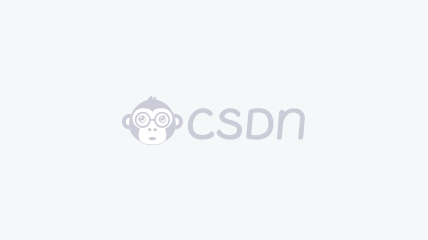

lly202406
- 粉丝: 3004
- 资源: 5525
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

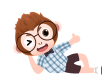
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


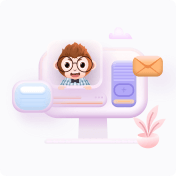
安全验证
文档复制为VIP权益,开通VIP直接复制
