// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: service_entry.proto
package com.alibaba.nacos.istio.model.naming;
/**
* <pre>
* ServiceEntry enables adding additional entries into Istio's internal
* service registry.
* <!-- go code generation tags
* +kubetype-gen
* +kubetype-gen:groupVersion=networking.istio.io/v1alpha3
* +genclient
* +k8s:deepcopy-gen=true
* -->
* </pre>
*
* Protobuf type {@code istio.networking.v1alpha3.ServiceEntry}
*/
public final class ServiceEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:istio.networking.v1alpha3.ServiceEntry)
ServiceEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use ServiceEntry.newBuilder() to construct.
private ServiceEntry(com.google.protobuf.GeneratedMessageV3.Builder<?> builder) {
super(builder);
}
private ServiceEntry() {
hosts_ = com.google.protobuf.LazyStringArrayList.EMPTY;
addresses_ = com.google.protobuf.LazyStringArrayList.EMPTY;
ports_ = java.util.Collections.emptyList();
location_ = 0;
resolution_ = 0;
endpoints_ = java.util.Collections.emptyList();
exportTo_ = com.google.protobuf.LazyStringArrayList.EMPTY;
subjectAltNames_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ServiceEntry();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ServiceEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
hosts_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
hosts_.add(s);
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) != 0)) {
addresses_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
addresses_.add(s);
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) != 0)) {
ports_ = new java.util.ArrayList<com.alibaba.nacos.istio.model.Port>();
mutable_bitField0_ |= 0x00000004;
}
ports_.add(
input.readMessage(com.alibaba.nacos.istio.model.Port.parser(), extensionRegistry));
break;
}
case 32: {
int rawValue = input.readEnum();
location_ = rawValue;
break;
}
case 40: {
int rawValue = input.readEnum();
resolution_ = rawValue;
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000008) != 0)) {
endpoints_ = new java.util.ArrayList<com.alibaba.nacos.istio.model.naming.ServiceEntry.Endpoint>();
mutable_bitField0_ |= 0x00000008;
}
endpoints_.add(
input.readMessage(com.alibaba.nacos.istio.model.naming.ServiceEntry.Endpoint.parser(), extensionRegistry));
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000010) != 0)) {
exportTo_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000010;
}
exportTo_.add(s);
break;
}
case 66: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000020) != 0)) {
subjectAltNames_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000020;
}
subjectAltNames_.add(s);
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
hosts_ = hosts_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000002) != 0)) {
addresses_ = addresses_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000004) != 0)) {
ports_ = java.util.Collections.unmodifiableList(ports_);
}
if (((mutable_bitField0_ & 0x00000008) != 0)) {
endpoints_ = java.util.Collections.unmodifiableList(endpoints_);
}
if (((mutable_bitField0_ & 0x00000010) != 0)) {
exportTo_ = exportTo_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000020) != 0)) {
subjectAltNames_ = subjectAltNames_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.alibaba.nacos.istio.model.naming.ServiceEntryOuterClass.internal_static_istio_networking_v1alpha3_ServiceEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.alibaba.nacos.istio.model.naming.ServiceEntryOuterClass.internal_static_istio_networking_v1alpha3_ServiceEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.alibaba.nacos.istio.model.naming.ServiceEntry.class, com.alibaba.nacos.istio.model.naming.ServiceEntry.Builder.class);
}
/**
* <pre>
* Location specifies whether the service is part of Istio mesh or
* outside the mesh. Location determines the behavior of several
* features, such as service-to-service mTLS authentication, policy
* enforcement, etc. When communicating with services outside the mesh,
* Istio's mTLS authentication is disabled, and policy enforcement is
* performed on the client-side as opposed to server-side.
* </pre>
*
* Protobuf enum {@code istio.networking.v1alpha3.ServiceEntry.Location}
*/
public enum Location
implements com.google.protobuf.ProtocolMessageEnum {
/**
* <pre>
* Signifies that the service is external to the mesh. Typically used
* to indicate external services consumed through APIs.
* </pre>
*
* <code>MESH_EXTERNAL = 0;</code>
*/
MESH_EXTERNAL(0),
/**
* <pre>
* Signifies that the service is part of the mesh. Typically used to
* indicate services added explicitly as part of expanding the service
* mesh to include unmanag
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目是一个集Java、JavaScript、CSS、HTML、Shell、TypeScript多语言于一体的文章收藏与原创设计源码分享平台,包含1023个文件,涵盖457个Java源文件、163个Markdown文档、162个JavaScript文件、多种格式资源文件,如PNG图片、SCSS样式、XML配置等。该平台支持用户收藏和分享文章,既包括原创内容,也涵盖他人佳作,旨在为开发者提供丰富的知识库和资源共享环境。
资源推荐
资源详情
资源评论
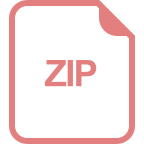
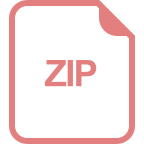
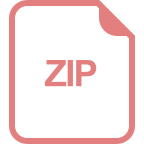
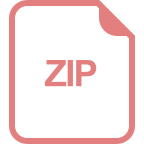
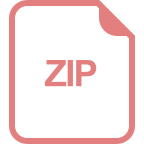
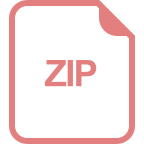
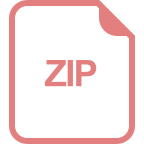
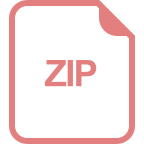
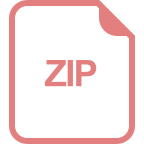
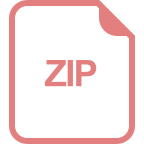
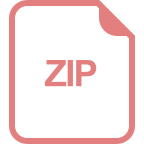
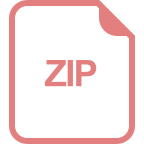
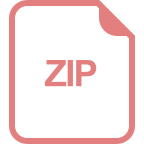
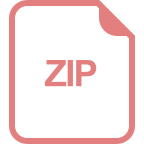
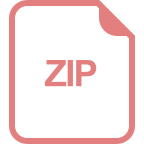
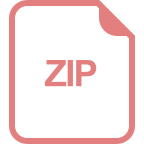
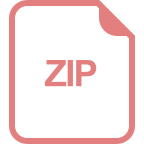
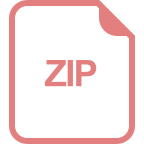
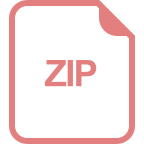
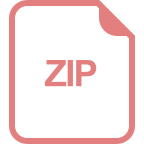
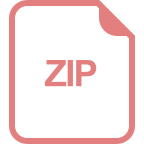
收起资源包目录

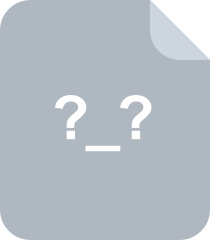
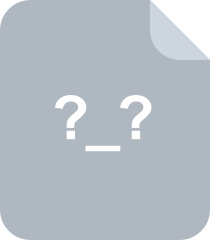
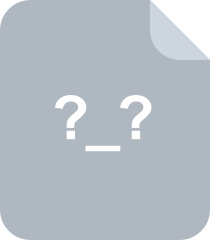
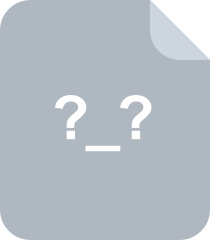
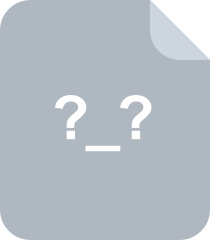
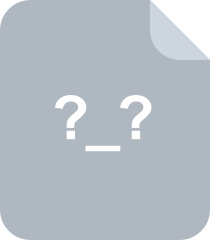
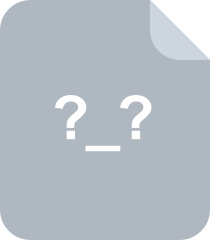
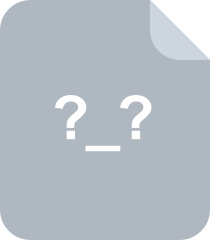
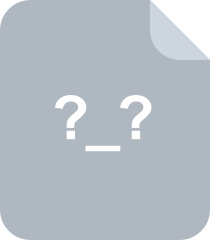
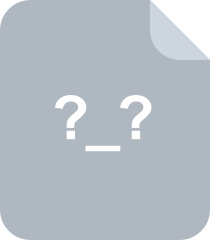
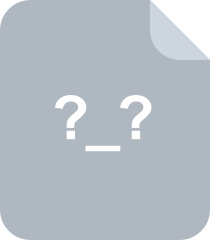
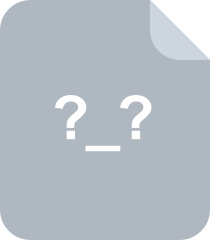
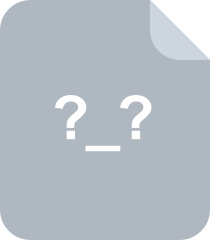
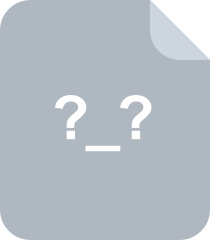
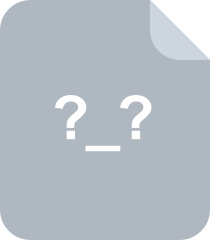
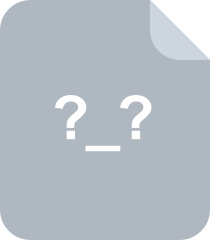
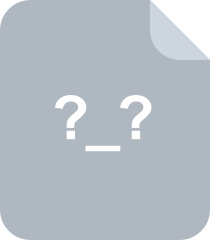
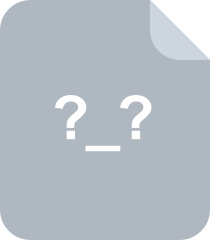
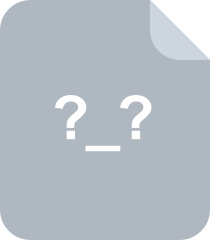
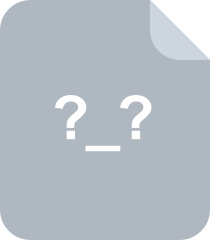
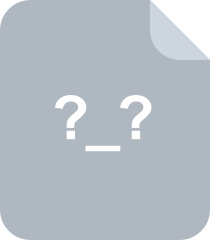
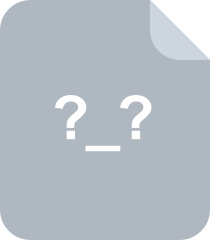
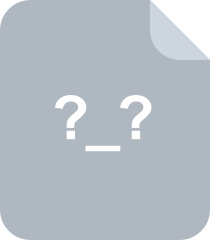
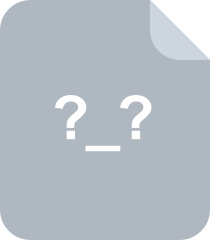
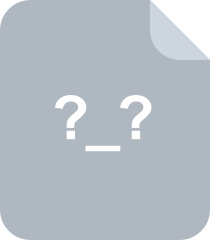
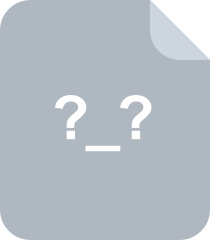
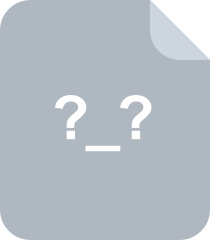
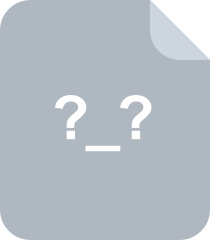
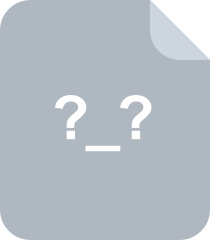
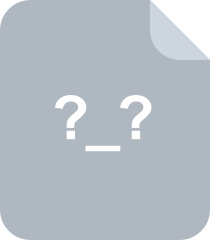
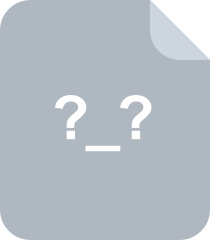
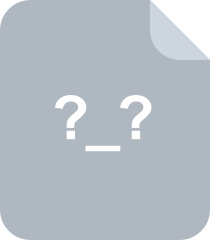
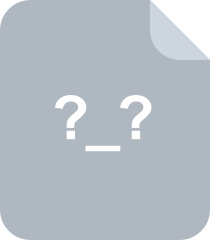
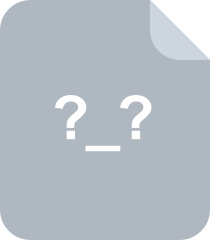
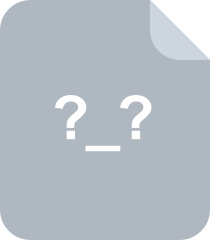
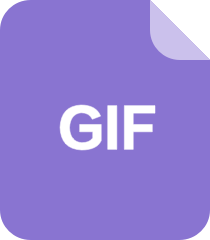
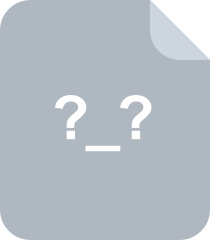
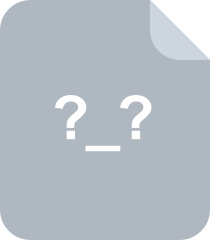
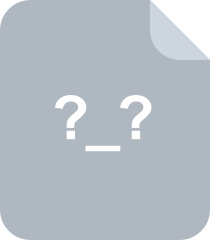
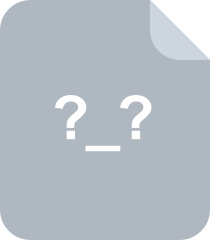
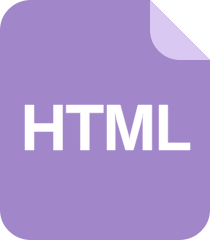
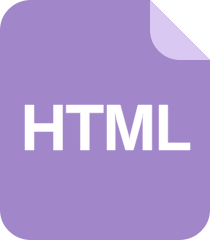
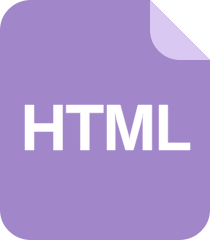
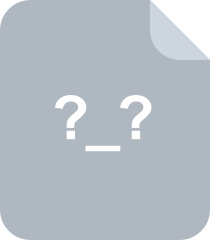
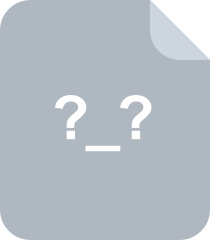
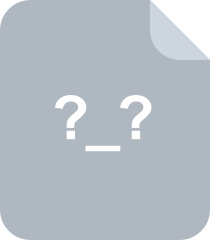
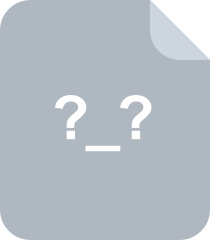
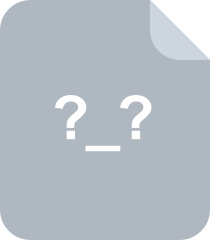
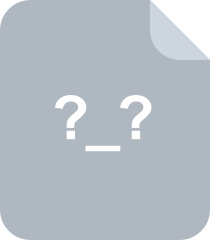
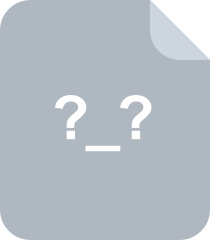
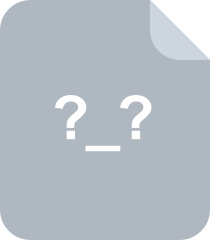
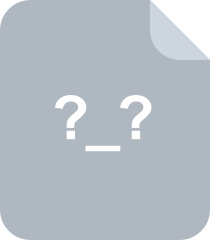
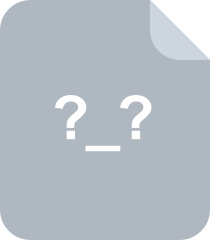
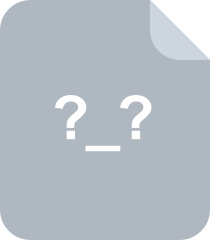
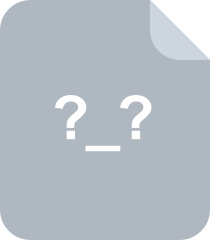
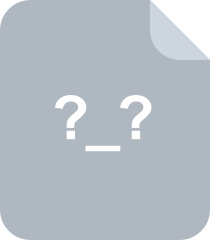
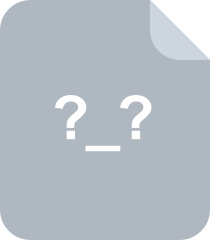
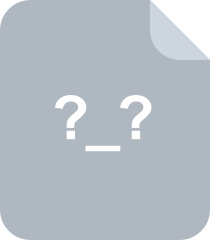
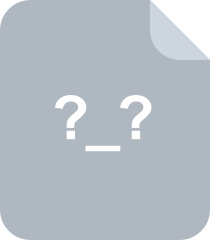
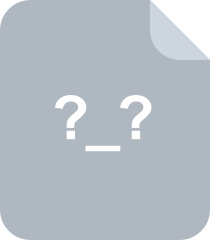
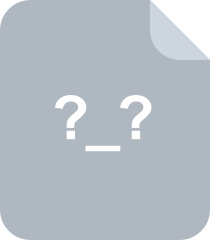
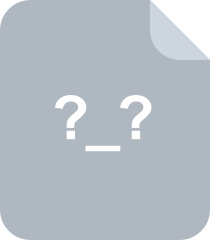
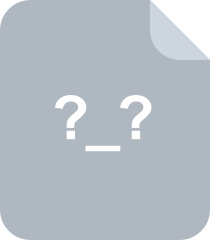
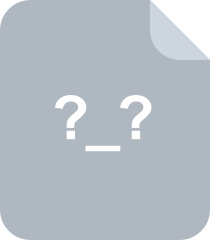
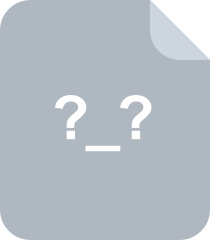
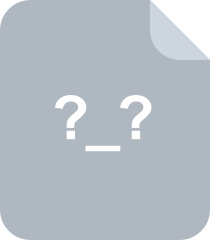
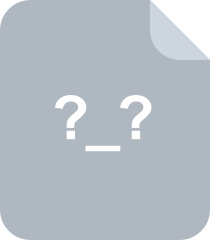
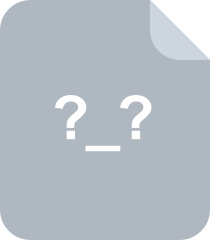
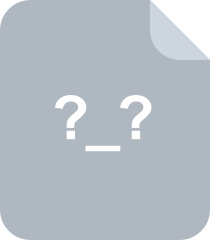
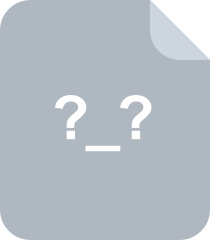
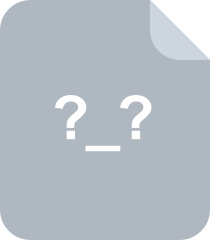
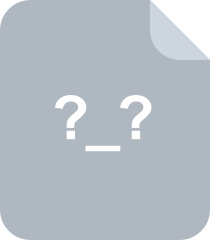
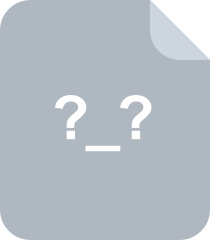
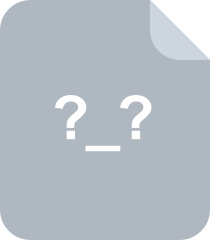
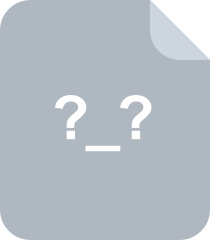
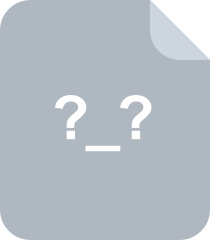
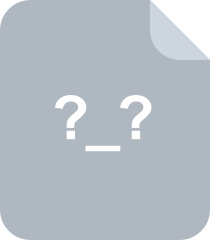
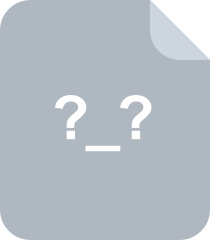
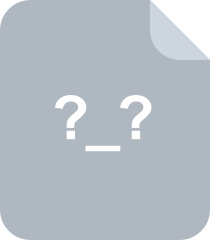
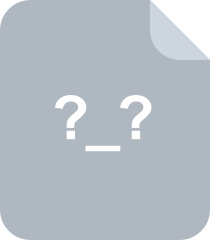
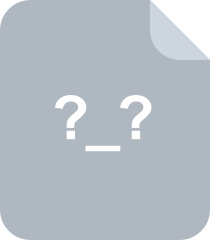
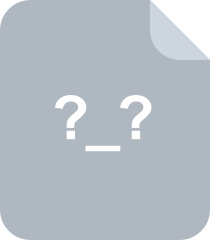
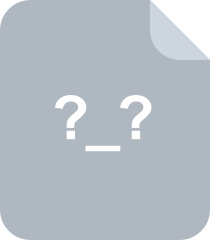
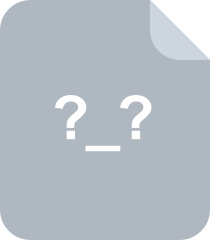
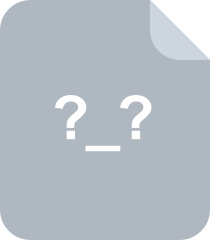
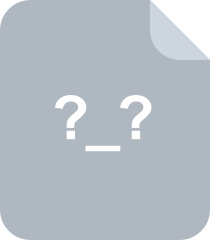
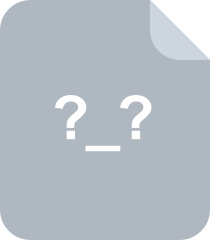
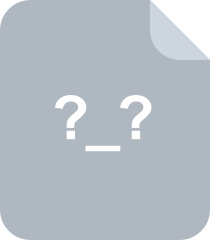
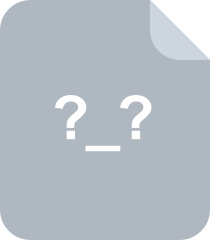
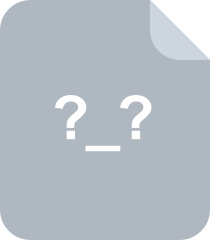
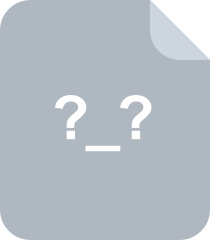
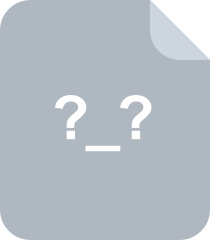
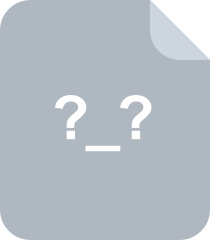
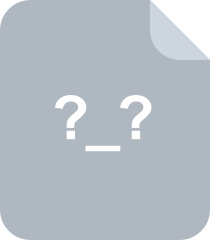
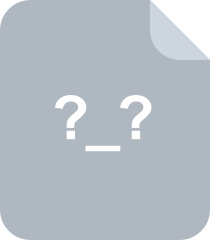
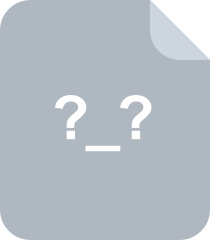
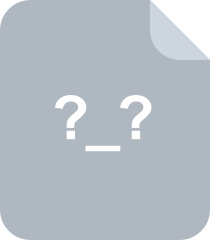
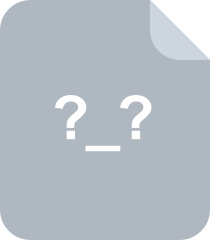
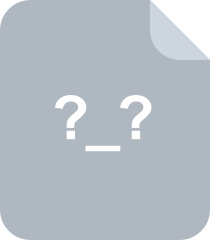
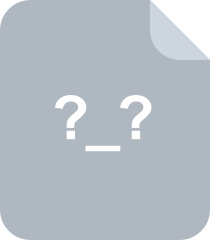
共 1026 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
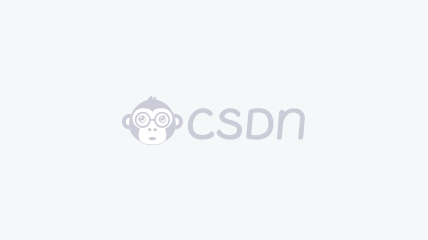

lly202406
- 粉丝: 2096
- 资源: 3854
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

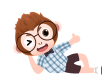
最新资源
- 基于Matlab simulink的异步电机SVPWM变频调速建模仿真
- 基于WebRTC和XMPP的OkEDU-Classroom-Web网页版互动教室设计源码
- 基于人脸识别技术的PyGame PC游戏《NinjaMove》开源设计源码
- 基于Spring MVC+MyBatis的10天快速开发周期CRM系统源码
- 分布式驱动车辆状态估计模型基于Carsim和simulink联合仿真,首先建立分布式驱动车辆轮边电机模型,并使用pid对目标速度
- 元胞自助机CA模拟动态再结晶dDRX过程,输入不同的材料参数,应变 温度 应变率,得到不同的显微组织结果,可以输出再结晶分数,再
- 基于Java开发的二手物流流转平台设计源码
- 基于Vue和Python Flask框架,整合通义千问API的简易版大模型对话界面设计源码
- 基于Spring Boot和Mybatis框架的Java企业级设计源码
- 中压领域三电平VSG并网系统(理论推导) 控制环路:同步发电机控制+电压电流双闭环控制 拓扑:二极管钳位型三电平逆变电路 滤波器
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


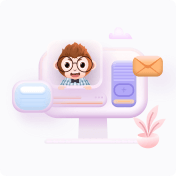
安全验证
文档复制为VIP权益,开通VIP直接复制
