/**
* Swiper 5.4.5
* Most modern mobile touch slider and framework with hardware accelerated transitions
* http://swiperjs.com
*
* Copyright 2014-2020 Vladimir Kharlampidi
*
* Released under the MIT License
*
* Released on: June 16, 2020
*/
(function (global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory() :
typeof define === 'function' && define.amd ? define(factory) :
(global = global || self, global.Swiper = factory());
}(this, (function () { 'use strict';
/**
* SSR Window 2.0.0
* Better handling for window object in SSR environment
* https://github.com/nolimits4web/ssr-window
*
* Copyright 2020, Vladimir Kharlampidi
*
* Licensed under MIT
*
* Released on: May 12, 2020
*/
/* eslint-disable no-param-reassign */
function isObject(obj) {
return (obj !== null &&
typeof obj === 'object' &&
'constructor' in obj &&
obj.constructor === Object);
}
function extend(target, src) {
if (target === void 0) { target = {}; }
if (src === void 0) { src = {}; }
Object.keys(src).forEach(function (key) {
if (typeof target[key] === 'undefined')
{ target[key] = src[key]; }
else if (isObject(src[key]) &&
isObject(target[key]) &&
Object.keys(src[key]).length > 0) {
extend(target[key], src[key]);
}
});
}
var doc = typeof document !== 'undefined' ? document : {};
var ssrDocument = {
body: {},
addEventListener: function () { },
removeEventListener: function () { },
activeElement: {
blur: function () { },
nodeName: '',
},
querySelector: function () {
return null;
},
querySelectorAll: function () {
return [];
},
getElementById: function () {
return null;
},
createEvent: function () {
return {
initEvent: function () { },
};
},
createElement: function () {
return {
children: [],
childNodes: [],
style: {},
setAttribute: function () { },
getElementsByTagName: function () {
return [];
},
};
},
createElementNS: function () {
return {};
},
importNode: function () {
return null;
},
location: {
hash: '',
host: '',
hostname: '',
href: '',
origin: '',
pathname: '',
protocol: '',
search: '',
},
};
extend(doc, ssrDocument);
var win = typeof window !== 'undefined' ? window : {};
var ssrWindow = {
document: ssrDocument,
navigator: {
userAgent: '',
},
location: {
hash: '',
host: '',
hostname: '',
href: '',
origin: '',
pathname: '',
protocol: '',
search: '',
},
history: {
replaceState: function () { },
pushState: function () { },
go: function () { },
back: function () { },
},
CustomEvent: function CustomEvent() {
return this;
},
addEventListener: function () { },
removeEventListener: function () { },
getComputedStyle: function () {
return {
getPropertyValue: function () {
return '';
},
};
},
Image: function () { },
Date: function () { },
screen: {},
setTimeout: function () { },
clearTimeout: function () { },
matchMedia: function () {
return {};
},
};
extend(win, ssrWindow);
/**
* Dom7 2.1.5
* Minimalistic JavaScript library for DOM manipulation, with a jQuery-compatible API
* http://framework7.io/docs/dom.html
*
* Copyright 2020, Vladimir Kharlampidi
* The iDangero.us
* http://www.idangero.us/
*
* Licensed under MIT
*
* Released on: May 15, 2020
*/
var Dom7 = function Dom7(arr) {
var self = this;
// Create array-like object
for (var i = 0; i < arr.length; i += 1) {
self[i] = arr[i];
}
self.length = arr.length;
// Return collection with methods
return this;
};
function $(selector, context) {
var arr = [];
var i = 0;
if (selector && !context) {
if (selector instanceof Dom7) {
return selector;
}
}
if (selector) {
// String
if (typeof selector === 'string') {
var els;
var tempParent;
var html = selector.trim();
if (html.indexOf('<') >= 0 && html.indexOf('>') >= 0) {
var toCreate = 'div';
if (html.indexOf('<li') === 0) { toCreate = 'ul'; }
if (html.indexOf('<tr') === 0) { toCreate = 'tbody'; }
if (html.indexOf('<td') === 0 || html.indexOf('<th') === 0) { toCreate = 'tr'; }
if (html.indexOf('<tbody') === 0) { toCreate = 'table'; }
if (html.indexOf('<option') === 0) { toCreate = 'select'; }
tempParent = doc.createElement(toCreate);
tempParent.innerHTML = html;
for (i = 0; i < tempParent.childNodes.length; i += 1) {
arr.push(tempParent.childNodes[i]);
}
} else {
if (!context && selector[0] === '#' && !selector.match(/[ .<>:~]/)) {
// Pure ID selector
els = [doc.getElementById(selector.trim().split('#')[1])];
} else {
// Other selectors
els = (context || doc).querySelectorAll(selector.trim());
}
for (i = 0; i < els.length; i += 1) {
if (els[i]) { arr.push(els[i]); }
}
}
} else if (selector.nodeType || selector === win || selector === doc) {
// Node/element
arr.push(selector);
} else if (selector.length > 0 && selector[0].nodeType) {
// Array of elements or instance of Dom
for (i = 0; i < selector.length; i += 1) {
arr.push(selector[i]);
}
}
}
return new Dom7(arr);
}
$.fn = Dom7.prototype;
$.Class = Dom7;
$.Dom7 = Dom7;
function unique(arr) {
var uniqueArray = [];
for (var i = 0; i < arr.length; i += 1) {
if (uniqueArray.indexOf(arr[i]) === -1) { uniqueArray.push(arr[i]); }
}
return uniqueArray;
}
// Classes and attributes
function addClass(className) {
if (typeof className === 'undefined') {
return this;
}
var classes = className.split(' ');
for (var i = 0; i < classes.length; i += 1) {
for (var j = 0; j < this.length; j += 1) {
if (typeof this[j] !== 'undefined' && typeof this[j].classList !== 'undefined') { this[j].classList.add(classes[i]); }
}
}
return this;
}
function removeClass(className) {
var classes = className.split(' ');
for (var i = 0; i < classes.length; i += 1) {
for (var j = 0; j < this.length; j += 1) {
if (typeof this[j] !== 'undefined' && typeof this[j].classList !== 'undefined') { this[j].classList.remove(classes[i]); }
}
}
return this;
}
function hasClass(className) {
if (!this[0]) { return false; }
return this[0].classList.contains(className);
}
function toggleClass(className) {
var classes = className.split(' ');
for (var i = 0; i < classes.length; i += 1)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
该项目是一款基于Vue框架构建的动力车间备件管理系统前端设计源码,包含600个文件,涵盖JavaScript, TypeScript, Vue, CSS, HTML等多种编程语言。具体文件分布如下:JavaScript文件286个,TypeScript文件70个,Less文件56个,SCSS文件56个,Markdown文件32个,映射文件23个,JSON文件15个,Vue文件15个,XML文件5个,CSS文件5个。该系统旨在提升动力车间备件管理的效率和便捷性。
资源推荐
资源详情
资源评论
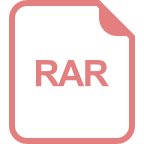
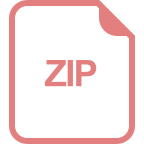
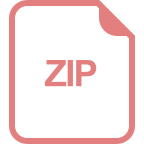
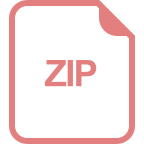
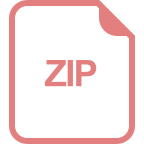
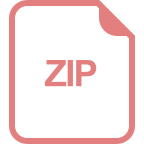
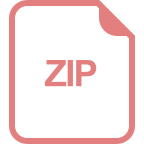
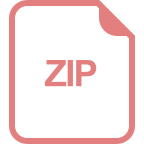
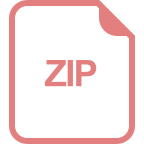
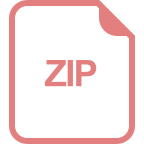
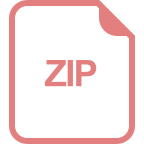
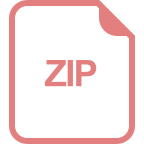
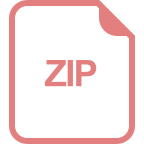
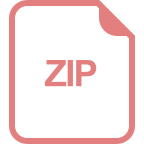
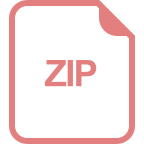
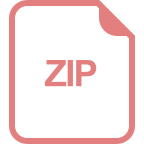
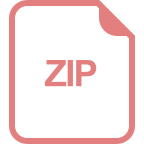
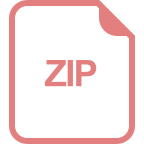
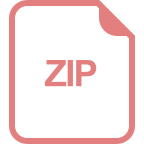
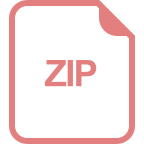
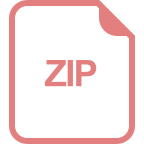
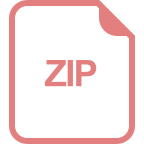
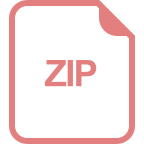
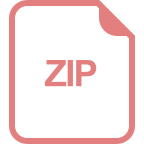
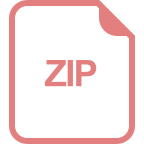
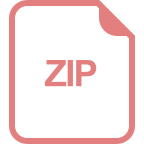
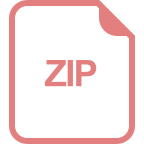
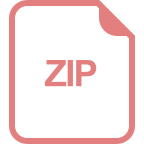
收起资源包目录

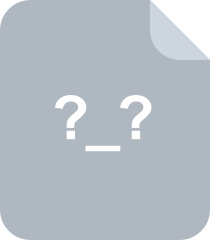
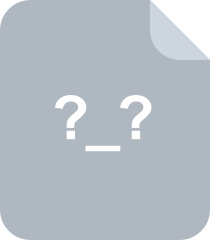
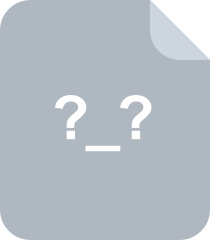
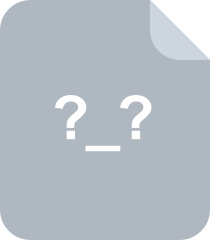
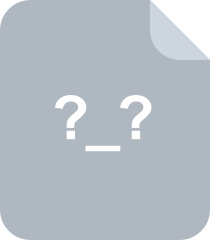
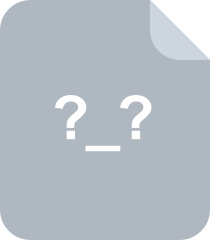
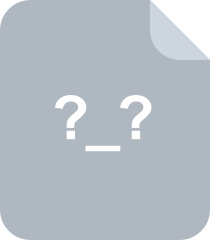
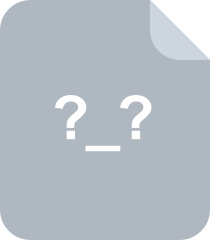
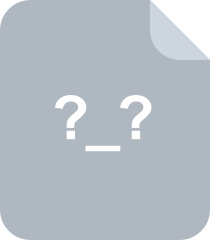
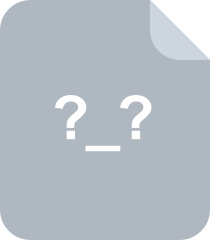
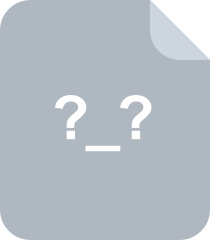
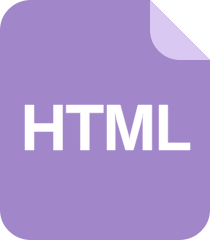
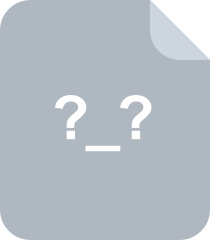
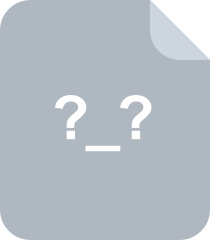
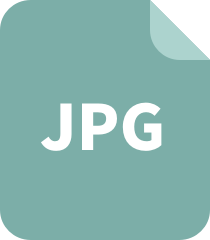
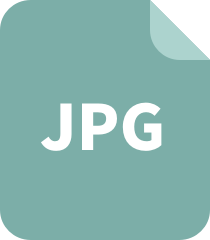
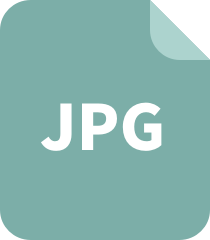
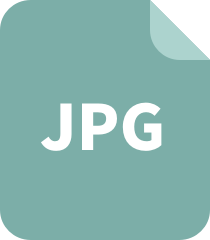
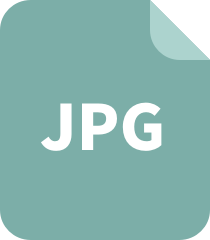
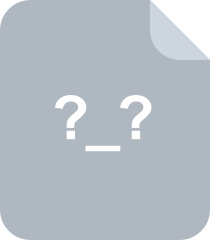
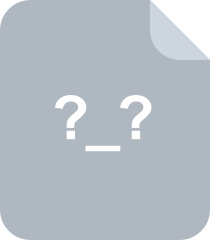
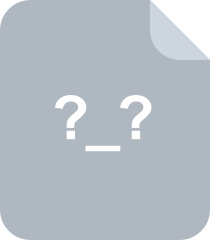
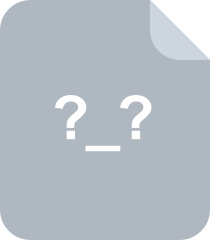
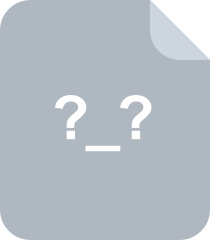
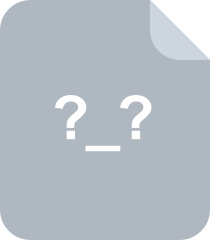
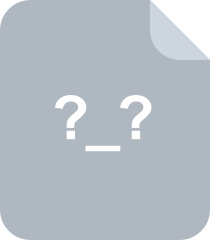
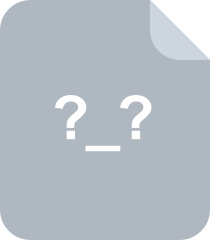
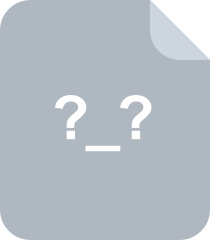
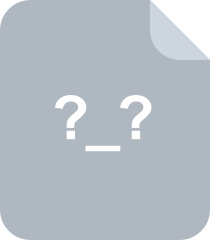
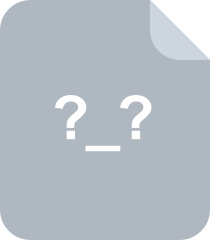
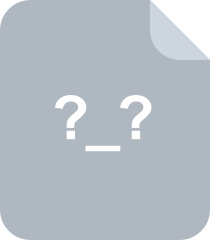
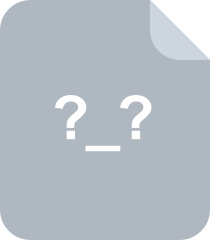
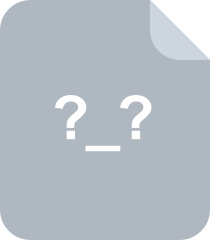
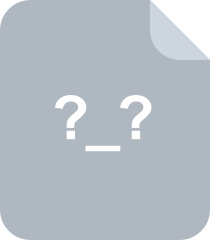
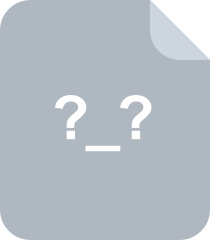
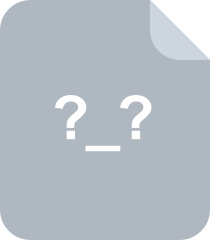
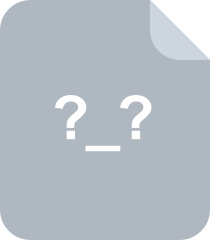
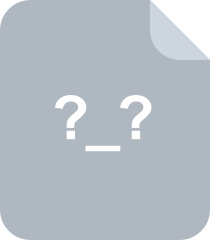
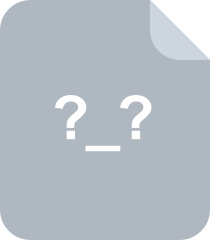
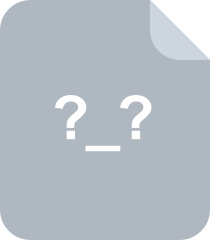
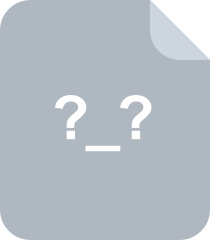
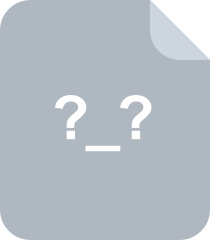
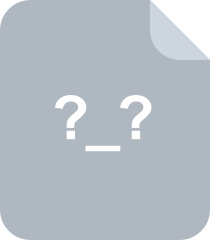
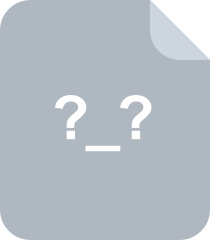
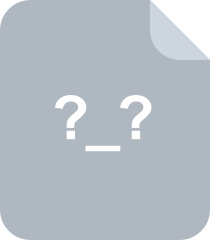
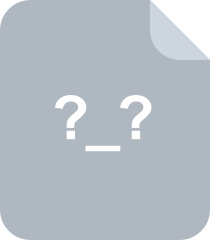
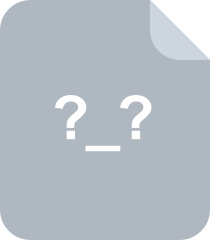
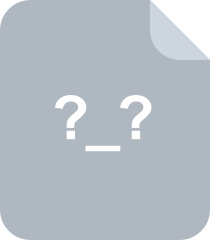
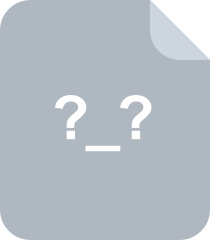
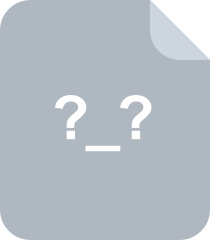
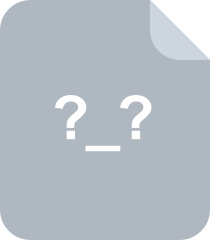
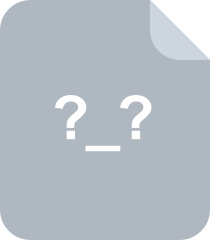
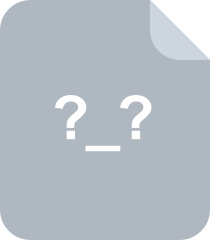
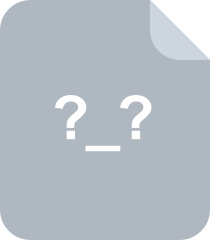
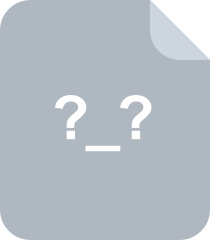
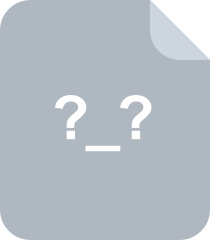
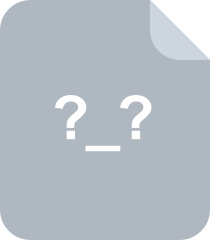
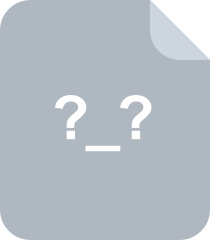
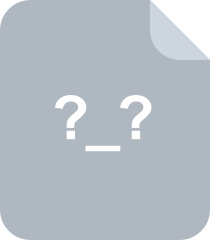
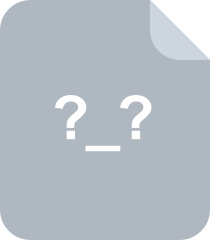
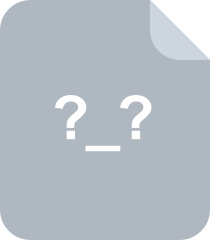
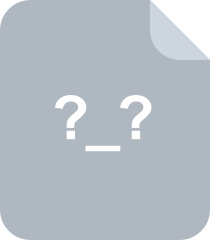
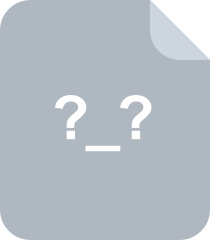
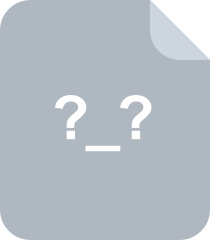
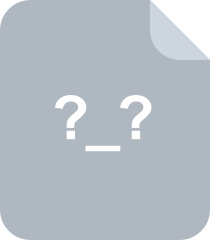
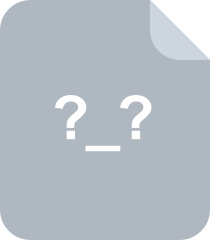
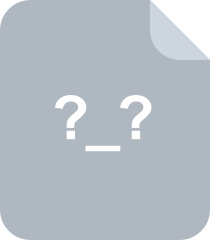
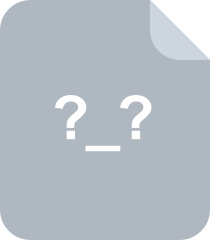
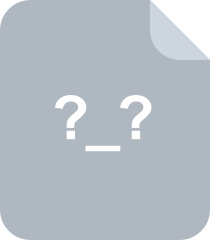
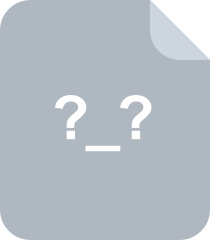
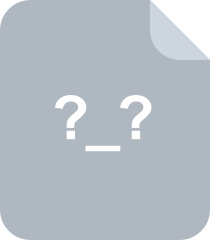
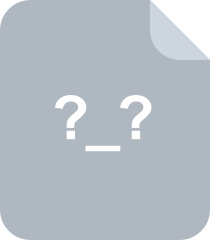
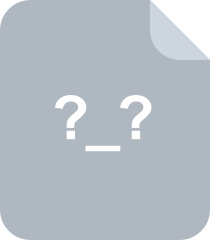
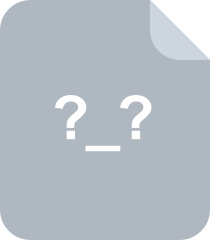
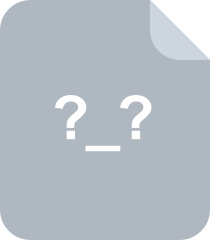
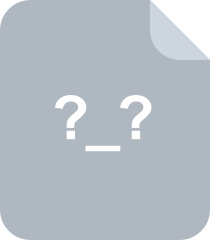
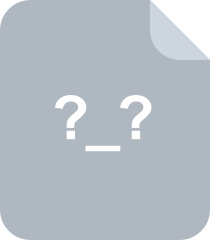
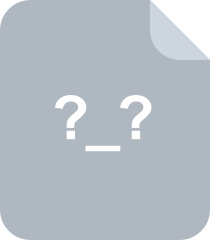
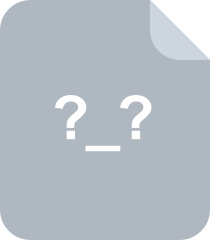
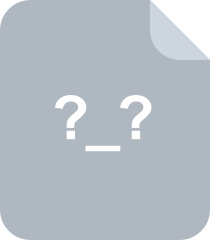
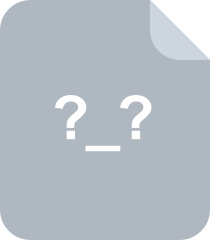
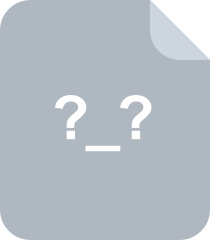
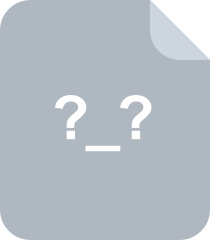
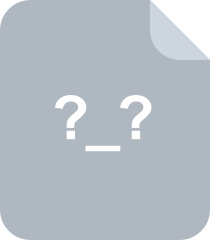
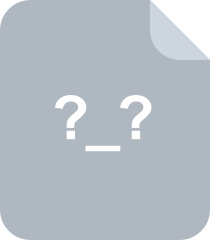
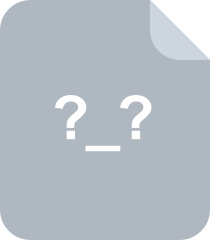
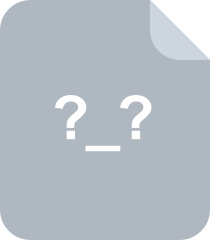
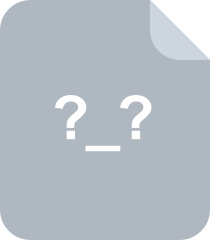
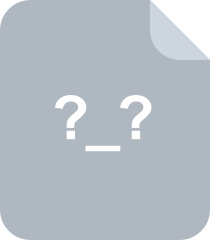
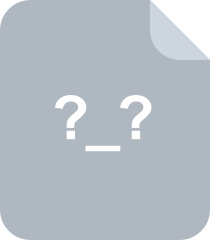
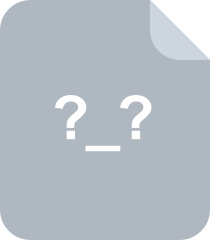
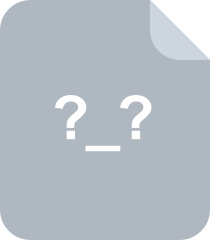
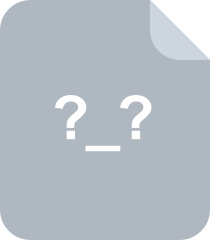
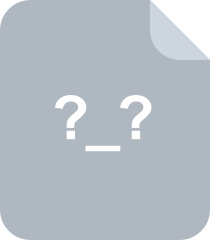
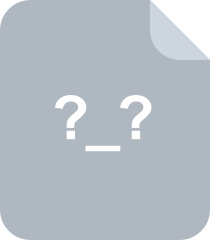
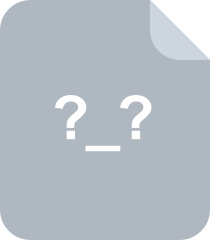
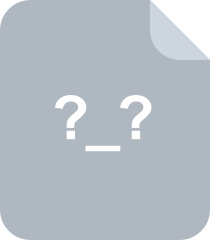
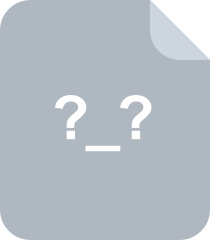
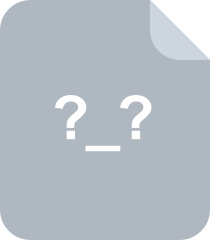
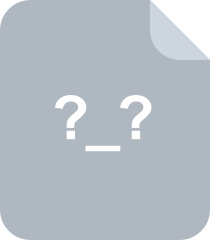
共 598 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
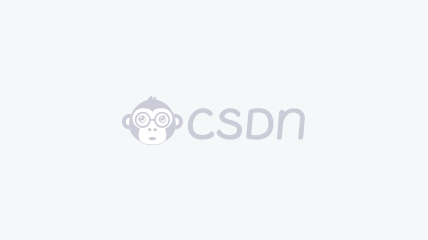

lly202406
- 粉丝: 2613
- 资源: 5446
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

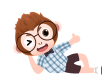
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


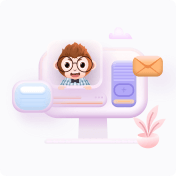
安全验证
文档复制为VIP权益,开通VIP直接复制
