/*!
* Ext JS Library 3.2.0
* Copyright(c) 2006-2010 Ext JS, Inc.
* licensing@extjs.com
* http://www.extjs.com/license
*/
/**
* @class form.date.MyDatePicker
* @extends Ext.Component
* <p>A popup date picker. This class is used by the {@link Ext.form.DateField DateField} class
* to allow browsing and selection of valid dates.</p>
* <p>All the string values documented below may be overridden by including an Ext locale file in
* your page.</p>
* @constructor
* Create a new DatePicker
* @param {Object} config The config object
* @xtype datepicker
*/
Ext.ns("form.date.menu")
form.date.MyDatePicker = Ext.extend(Ext.BoxComponent, {
/**
* @cfg {String} todayText
* The text to display on the button that selects the current date (defaults to <code>'Today'</code>)
*/
todayText : 'Today',
/**
* @cfg {String} okText
* The text to display on the ok button (defaults to <code>' OK '</code> to give the user extra clicking room)
*/
okText : ' OK ',
/**
* @cfg {String} cancelText
* The text to display on the cancel button (defaults to <code>'Cancel'</code>)
*/
cancelText : 'Cancel',
/**
* @cfg {Function} handler
* Optional. A function that will handle the select event of this picker.
* The handler is passed the following parameters:<div class="mdetail-params"><ul>
* <li><code>picker</code> : MyDatePicker<div class="sub-desc">This MyDatePicker.</div></li>
* <li><code>date</code> : Date<div class="sub-desc">The selected date.</div></li>
* </ul></div>
*/
/**
* @cfg {Object} scope
* The scope (<code><b>this</b></code> reference) in which the <code>{@link #handler}</code>
* function will be called. Defaults to this MyDatePicker instance.
*/
/**
* @cfg {String} todayTip
* A string used to format the message for displaying in a tooltip over the button that
* selects the current date. Defaults to <code>'{0} (Spacebar)'</code> where
* the <code>{0}</code> token is replaced by today's date.
*/
todayTip : '{0} (Spacebar)',
/**
* @cfg {String} minText
* The error text to display if the minDate validation fails (defaults to <code>'This date is before the minimum date'</code>)
*/
minText : 'This date is before the minimum date',
/**
* @cfg {String} maxText
* The error text to display if the maxDate validation fails (defaults to <code>'This date is after the maximum date'</code>)
*/
maxText : 'This date is after the maximum date',
/**
* @cfg {String} format
* The default date format string which can be overriden for localization support. The format must be
* valid according to {@link Date#parseDate} (defaults to <code>'m/d/y'</code>).
*/
format : 'm/d/y',
/**
* @cfg {String} disabledDaysText
* The tooltip to display when the date falls on a disabled day (defaults to <code>'Disabled'</code>)
*/
disabledDaysText : 'Disabled',
/**
* @cfg {String} disabledDatesText
* The tooltip text to display when the date falls on a disabled date (defaults to <code>'Disabled'</code>)
*/
disabledDatesText : 'Disabled',
/**
* @cfg {Array} monthNames
* An array of textual month names which can be overriden for localization support (defaults to Date.monthNames)
*/
monthNames : Date.monthNames,
/**
* @cfg {Array} dayNames
* An array of textual day names which can be overriden for localization support (defaults to Date.dayNames)
*/
dayNames : Date.dayNames,
/**
* @cfg {String} nextText
* The next month navigation button tooltip (defaults to <code>'Next Month (Control+Right)'</code>)
*/
nextText : 'Next Month (Control+Right)',
/**
* @cfg {String} prevText
* The previous month navigation button tooltip (defaults to <code>'Previous Month (Control+Left)'</code>)
*/
prevText : 'Previous Month (Control+Left)',
/**
* @cfg {String} monthYearText
* The header month selector tooltip (defaults to <code>'Choose a month (Control+Up/Down to move years)'</code>)
*/
monthYearText : 'MyExt: Choose a month (Control+Up/Down to move years)',
/**
* @cfg {Number} startDay
* Day index at which the week should begin, 0-based (defaults to 0, which is Sunday)
*/
startDay : 0,
/**
* @cfg {Boolean} showToday
* False to hide the footer area containing the Today button and disable the keyboard handler for spacebar
* that selects the current date (defaults to <code>true</code>).
*/
showToday : true,
/**
* @cfg {Date} minDate
* Minimum allowable date (JavaScript date object, defaults to null)
*/
/**
* @cfg {Date} maxDate
* Maximum allowable date (JavaScript date object, defaults to null)
*/
/**
* @cfg {Array} disabledDays
* An array of days to disable, 0-based. For example, [0, 6] disables Sunday and Saturday (defaults to null).
*/
/**
* @cfg {RegExp} disabledDatesRE
* JavaScript regular expression used to disable a pattern of dates (defaults to null). The {@link #disabledDates}
* config will generate this regex internally, but if you specify disabledDatesRE it will take precedence over the
* disabledDates value.
*/
/**
* @cfg {Array} disabledDates
* An array of 'dates' to disable, as strings. These strings will be used to build a dynamic regular
* expression so they are very powerful. Some examples:
* <ul>
* <li>['03/08/2003', '09/16/2003'] would disable those exact dates</li>
* <li>['03/08', '09/16'] would disable those days for every year</li>
* <li>['^03/08'] would only match the beginning (useful if you are using short years)</li>
* <li>['03/../2006'] would disable every day in March 2006</li>
* <li>['^03'] would disable every day in every March</li>
* </ul>
* Note that the format of the dates included in the array should exactly match the {@link #format} config.
* In order to support regular expressions, if you are using a date format that has '.' in it, you will have to
* escape the dot when restricting dates. For example: ['03\\.08\\.03'].
*/
// private
// Set by other components to stop the picker focus being updated when the value changes.
focusOnSelect: true,
// default value used to initialise each date in the MyDatePicker
// (note: 12 noon was chosen because it steers well clear of all DST timezone changes)
initHour: 12, // 24-hour format
// private
initComponent : function(){
form.date.MyDatePicker.superclass.initComponent.call(this);
this.value = this.value ?
this.value.clearTime(true) : new Date().clearTime();
this.addEvents(
/**
* @event select
* Fires when a date is selected
* @param {MyDatePicker} this MyDatePicker
* @param {Date} date The selected date
*/
'select'
);
if(this.handler){
this.on('select', this.handler, this.scope || this);
}
this.initDisabledDays();
},
// private
initDisabledDays : function(){
if(!this.disabledDatesRE && this.disabledDates){
var dd = this.disabledDates,
len = dd.length - 1,
re = '(?:';
Ext.each(dd, function(d, i){
re += Ext.isDate(d) ? '^' + Ext.escapeRe(d.dateFormat(this.format)) + '$' : dd[i];
if(i != len){
re += '|';
}
}, this);
this.disabledDatesRE = new RegExp(re + ')');

LimStore
- 粉丝: 6
- 资源: 18
最新资源
- (源码)基于Django和OpenCV的智能车视频处理系统.zip
- (源码)基于ESP8266的WebDAV服务器与3D打印机管理系统.zip
- (源码)基于Nio实现的Mycat 2.0数据库代理系统.zip
- (源码)基于Java的高校学生就业管理系统.zip
- (源码)基于Spring Boot框架的博客系统.zip
- (源码)基于Spring Boot框架的博客管理系统.zip
- (源码)基于ESP8266和Blynk的IR设备控制系统.zip
- (源码)基于Java和JSP的校园论坛系统.zip
- (源码)基于ROS Kinetic框架的AGV激光雷达导航与SLAM系统.zip
- (源码)基于PythonDjango框架的资产管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


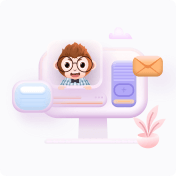
- 1
- 2
- 3
- 4
- 5
- 6
前往页