/*
* ProGuard -- shrinking, optimization, obfuscation, and preverification
* of Java bytecode.
*
* Copyright (c) 2002-2013 Eric Lafortune (eric@graphics.cornell.edu)
*
* This program is free software; you can redistribute it and/or modify it
* under the terms of the GNU General Public License as published by the Free
* Software Foundation; either version 2 of the License, or (at your option)
* any later version.
*
* This program is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for
* more details.
*
* You should have received a copy of the GNU General Public License along
* with this program; if not, write to the Free Software Foundation, Inc.,
* 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package proguard.optimize.peephole;
import proguard.classfile.*;
import proguard.classfile.constant.*;
import proguard.classfile.instruction.*;
import proguard.classfile.visitor.ClassPrinter;
/**
* This class contains a set of instruction sequences and their suggested
* replacements.
*
* @see InstructionSequencesReplacer
* @see InstructionSequenceReplacer
* @author Eric Lafortune
*/
public class InstructionSequenceConstants
{
private static final int X = InstructionSequenceReplacer.X;
private static final int Y = InstructionSequenceReplacer.Y;
private static final int Z = InstructionSequenceReplacer.Z;
private static final int A = InstructionSequenceReplacer.A;
private static final int B = InstructionSequenceReplacer.B;
private static final int C = InstructionSequenceReplacer.C;
private static final int D = InstructionSequenceReplacer.D;
private static final int STRING_A_LENGTH = InstructionSequenceReplacer.STRING_A_LENGTH;
private static final int BOOLEAN_A_STRING = InstructionSequenceReplacer.BOOLEAN_A_STRING;
private static final int CHAR_A_STRING = InstructionSequenceReplacer.CHAR_A_STRING;
private static final int INT_A_STRING = InstructionSequenceReplacer.INT_A_STRING;
private static final int LONG_A_STRING = InstructionSequenceReplacer.LONG_A_STRING;
private static final int FLOAT_A_STRING = InstructionSequenceReplacer.FLOAT_A_STRING;
private static final int DOUBLE_A_STRING = InstructionSequenceReplacer.DOUBLE_A_STRING;
private static final int STRING_A_STRING = InstructionSequenceReplacer.STRING_A_STRING;
private static final int BOOLEAN_B_STRING = InstructionSequenceReplacer.BOOLEAN_B_STRING;
private static final int CHAR_B_STRING = InstructionSequenceReplacer.CHAR_B_STRING;
private static final int INT_B_STRING = InstructionSequenceReplacer.INT_B_STRING;
private static final int LONG_B_STRING = InstructionSequenceReplacer.LONG_B_STRING;
private static final int FLOAT_B_STRING = InstructionSequenceReplacer.FLOAT_B_STRING;
private static final int DOUBLE_B_STRING = InstructionSequenceReplacer.DOUBLE_B_STRING;
private static final int STRING_B_STRING = InstructionSequenceReplacer.STRING_B_STRING;
private static final int I_32768 = 0;
private static final int I_65536 = 1;
private static final int I_16777216 = 2;
// private static final int I_0x000000ff
private static final int I_0x0000ff00 = 3;
private static final int I_0x00ff0000 = 4;
private static final int I_0xff000000 = 5;
private static final int I_0x0000ffff = 6;
private static final int I_0xffff0000 = 7;
private static final int L_M1 = 8;
private static final int L_2 = 9;
private static final int L_4 = 10;
private static final int L_8 = 11;
private static final int L_16 = 12;
private static final int L_32 = 13;
private static final int L_64 = 14;
private static final int L_128 = 15;
private static final int L_256 = 16;
private static final int L_512 = 17;
private static final int L_1024 = 18;
private static final int L_2048 = 19;
private static final int L_4096 = 20;
private static final int L_8192 = 21;
private static final int L_16384 = 22;
private static final int L_32768 = 23;
private static final int L_65536 = 24;
private static final int L_16777216 = 25;
private static final int L_4294967296 = 26;
private static final int L_0x00000000ffffffff = 27;
private static final int L_0xffffffff00000000 = 28;
private static final int F_M1 = 29;
private static final int D_M1 = 30;
private static final int STRING_EMPTY = 31;
private static final int FIELD_I = 32; // Implicitly uses X and Y.
private static final int FIELD_L = 33; // Implicitly uses X and Y.
private static final int FIELD_F = 34; // Implicitly uses X and Y.
private static final int FIELD_D = 35; // Implicitly uses X and Y.
private static final int METHOD_STRING_EQUALS = 36;
private static final int METHOD_STRING_LENGTH = 37;
private static final int METHOD_STRING_VALUEOF_Z = 38;
private static final int METHOD_STRING_VALUEOF_C = 39;
private static final int METHOD_STRING_VALUEOF_I = 40;
private static final int METHOD_STRING_VALUEOF_J = 41;
private static final int METHOD_STRING_VALUEOF_F = 42;
private static final int METHOD_STRING_VALUEOF_D = 43;
private static final int METHOD_STRING_VALUEOF_OBJECT = 44;
private static final int METHOD_STRINGBUFFER_INIT = 45;
private static final int METHOD_STRINGBUFFER_INIT_STRING = 46;
private static final int METHOD_STRINGBUFFER_APPEND_Z = 47;
private static final int METHOD_STRINGBUFFER_APPEND_C = 48;
private static final int METHOD_STRINGBUFFER_APPEND_I = 49;
private static final int METHOD_STRINGBUFFER_APPEND_J = 50;
private static final int METHOD_STRINGBUFFER_APPEND_F = 51;
private static final int METHOD_STRINGBUFFER_APPEND_D = 52;
private static final int METHOD_STRINGBUFFER_APPEND_STRING = 53;
private static final int METHOD_STRINGBUFFER_APPEND_OBJECT = 54;
private static final int METHOD_STRINGBUFFER_LENGTH = 55;
private static final int METHOD_STRINGBUFFER_TOSTRING = 56;
private static final int METHOD_STRINGBUILDER_INIT = 57;
private static final int METHOD_STRINGBUILDER_INIT_STRING = 58;
private static final int METHOD_STRINGBUILDER_APPEND_Z
没有合适的资源?快使用搜索试试~ 我知道了~
android开发无法打包或打包出错
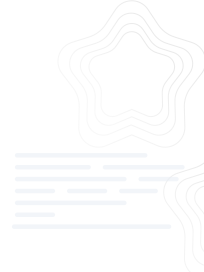
共1446个文件
class:657个
java:623个
html:61个


温馨提示
解决java.io.IOException: Can't read [D:\android_studio\app\libs\libammsdk.jar(;;;;;;!META-INF/MANIFEST.MF)] (Can't process class [com/tencent/wxop/stat/al.class] (Unknown verification type [95] in stack map frame))等无法打包问题, 解压后把android_sdk\tools\下的proguard整个替换。
资源推荐
资源详情
资源评论
















收起资源包目录

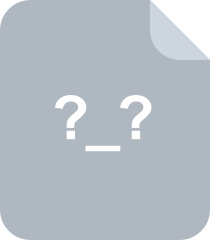
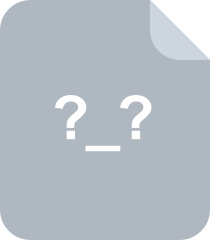
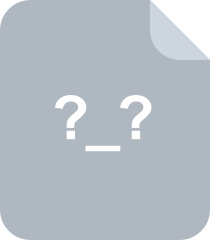
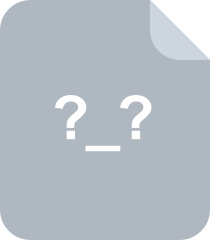
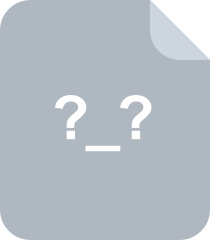
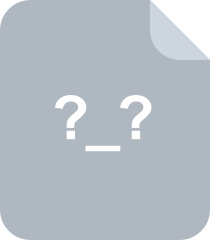
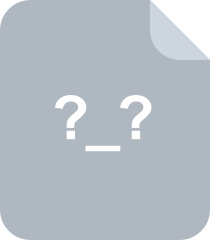
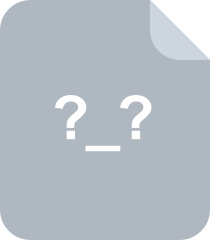
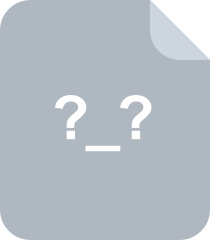
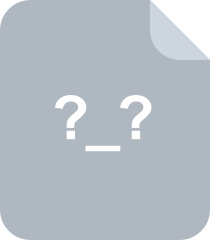
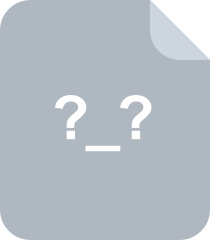
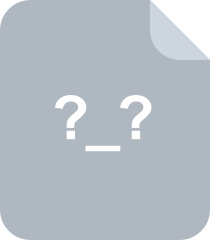
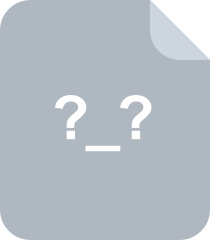
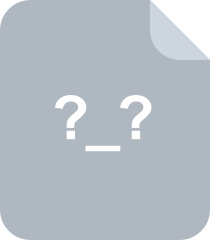
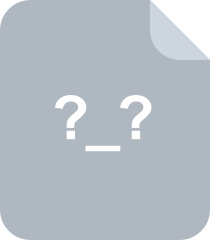
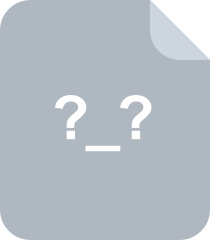
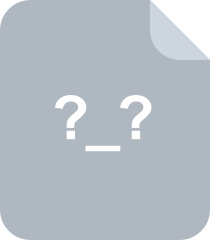
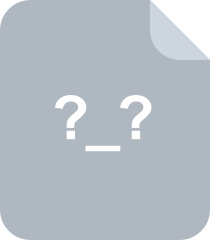
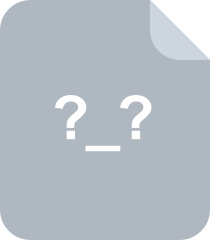
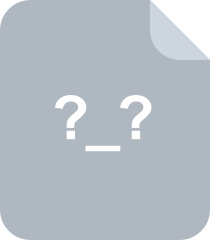
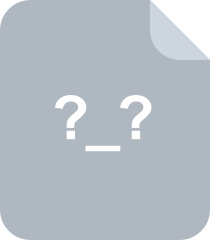
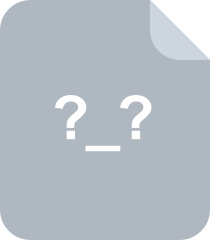
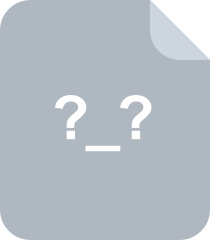
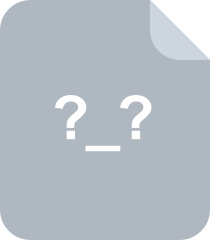
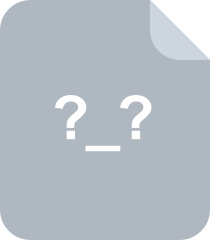
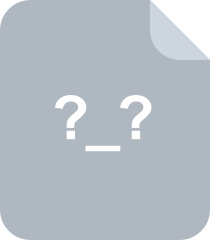
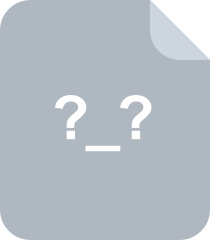
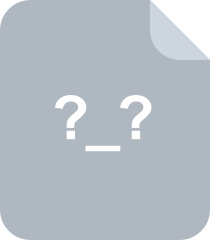
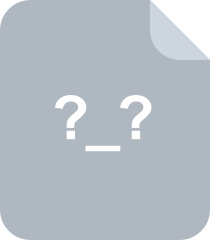
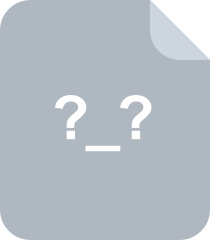
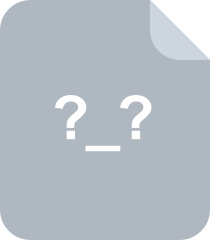
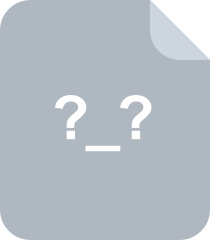
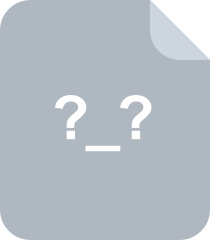
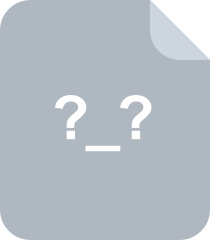
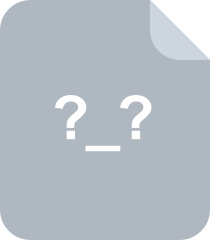
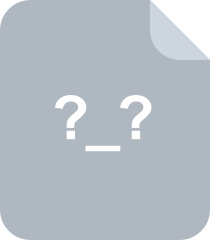
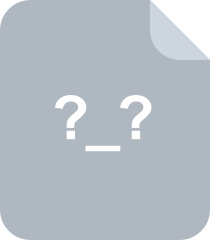
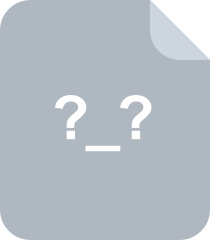
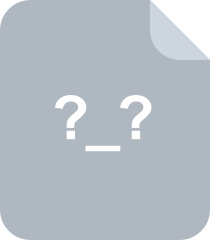
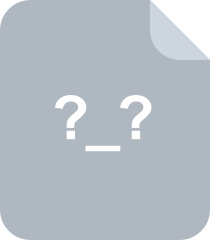
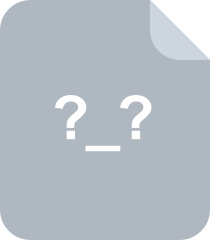
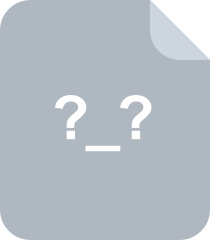
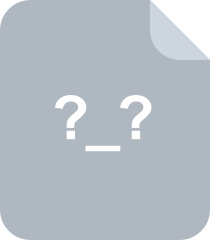
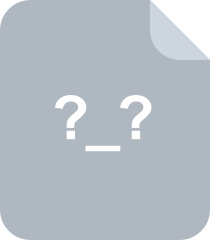
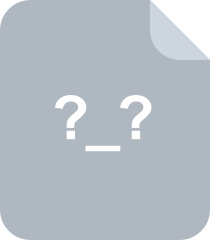
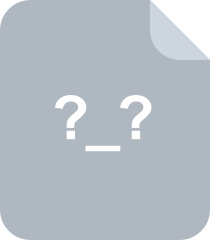
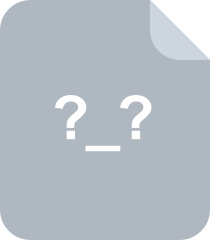
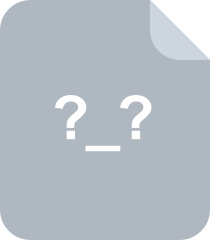
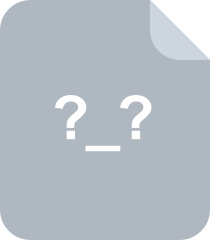
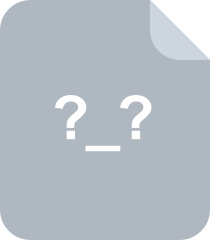
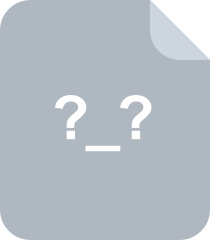
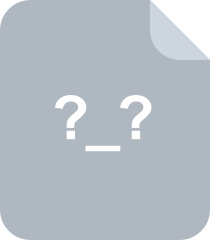
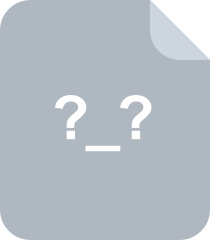
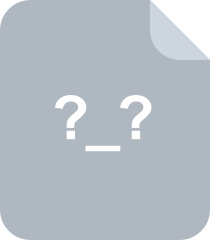
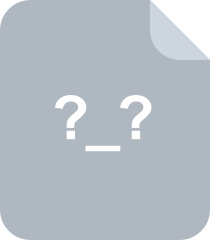
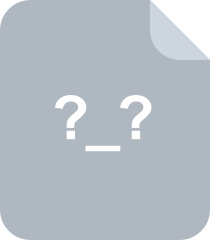
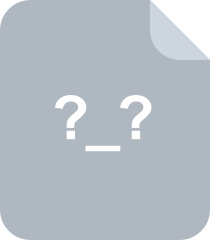
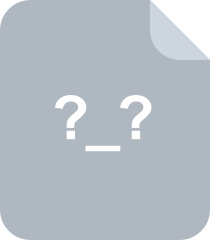
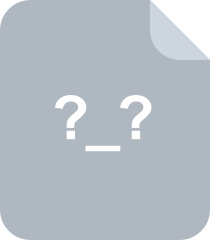
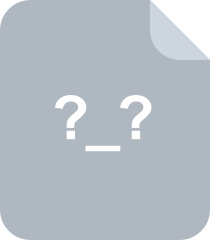
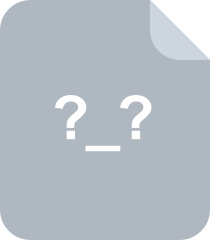
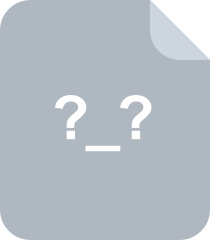
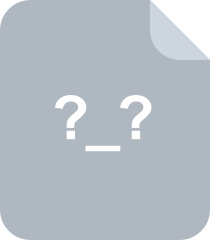
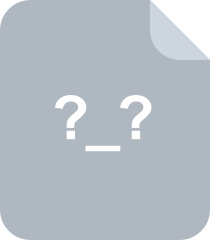
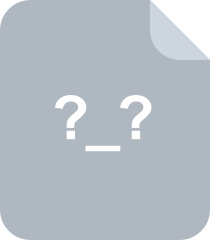
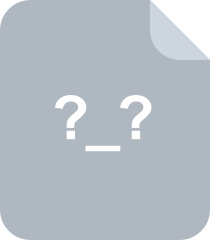
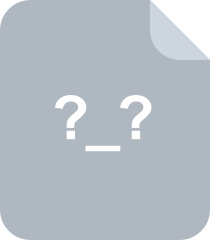
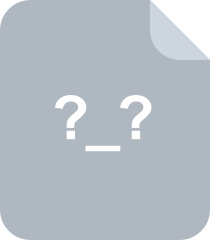
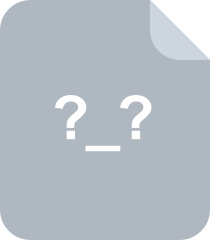
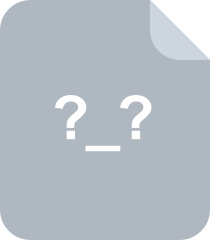
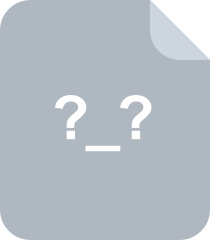
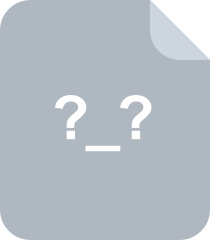
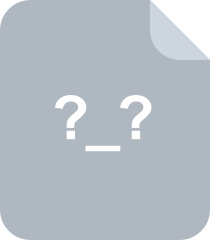
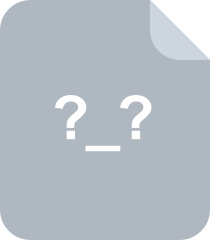
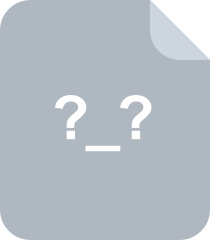
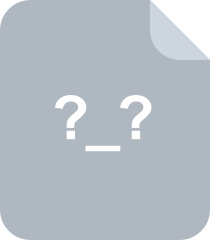
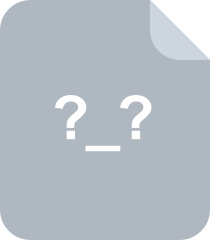
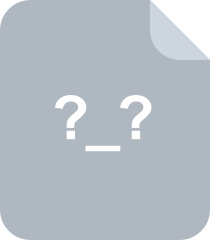
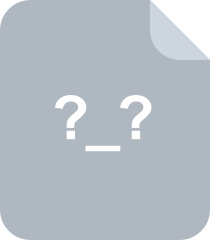
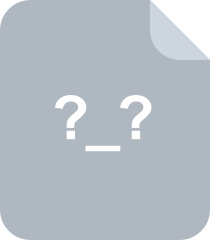
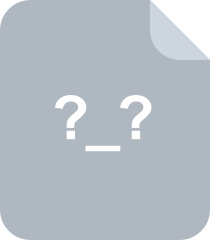
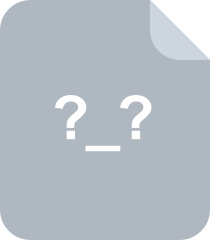
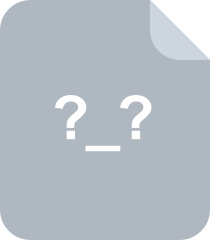
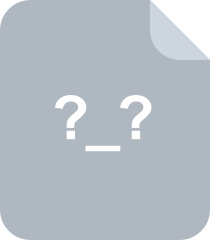
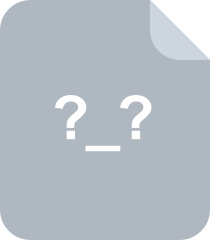
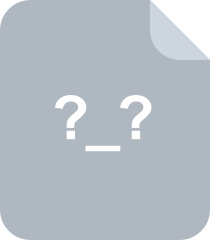
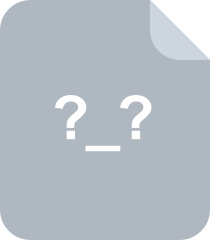
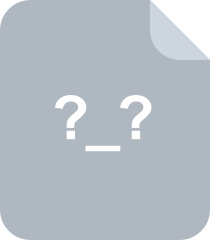
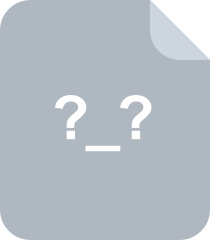
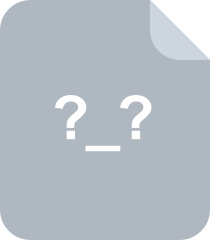
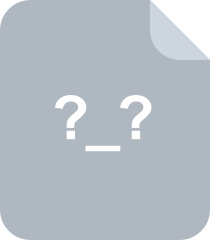
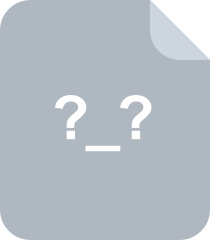
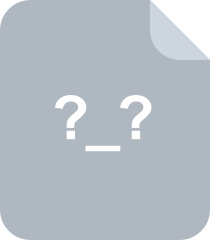
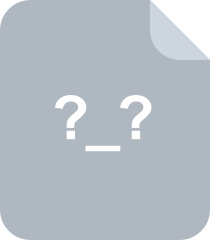
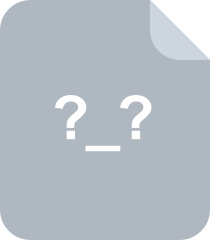
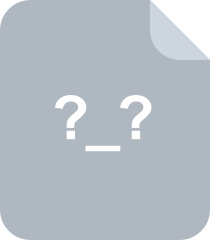
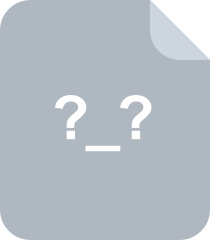
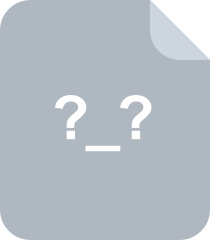
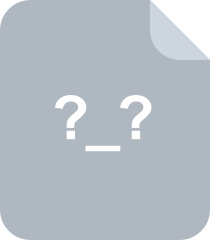
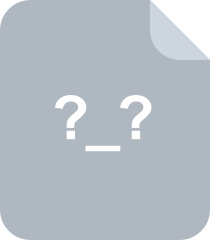
共 1446 条
- 1
- 2
- 3
- 4
- 5
- 6
- 15
资源评论
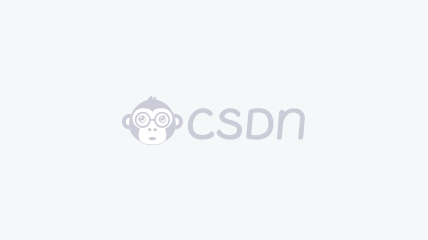
- weixin_547010812022-06-16无法解决问题
- 张振国12018-06-28无法解决问题

ljdmzl
- 粉丝: 4
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

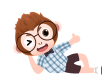
最新资源
- 智慧农业病虫害防治:YOLOv11多光谱叶片病斑检测模型调优.pdf
- 智慧农业实践:YOLOv11叶片病斑分割与生长状态联合分析.pdf
- 智慧农业实践:YOLOv11作物叶片病虫害实时诊断系统开发.pdf
- 智慧农业实战:YOLOv11结合多光谱图像的作物生长状态实时监测系统.pdf
- 智慧物流新方案:YOLOv11包裹分拣机器人视觉系统开发全流程.pdf
- 智慧养殖场应用:YOLOv11牲畜行为识别与健康监测系统搭建.pdf
- 智慧养殖场应用:YOLOv11牲畜行为识别与异常预警系统开发手册.pdf
- 智慧养殖场应用:YOLOv11牲畜行为分析与健康状态监测系统.pdf
- 智能仓储物流优化:YOLOv11动态包裹分拣与体积测量一体化方案.pdf
- 智能零售新基建:YOLOv11+ReID实现客流热力分析及购物行为预测.pdf
- 智慧渔业实践:YOLOv11渔获物种类识别与尺寸测量一体化方案.pdf
- 智慧养殖新突破:YOLOv11实现鱼类行为识别与密度统计.pdf
- 智能安防新范式:YOLOv11夜间低光照环境下的入侵检测算法调优.pdf
- 智能安防新突破——YOLOv11夜间复杂环境行人检测算法优化.pdf
- 自动驾驶感知升级:YOLOv11多目标跟踪与碰撞风险预测系统设计.pdf
- 自动驾驶感知升级:YOLOv11多目标跟踪与距离测量融合方案.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


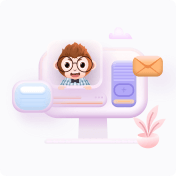
安全验证
文档复制为VIP权益,开通VIP直接复制
