/**
*
* @brief Definition of CAudioPlayerEngine
*
* Copyright (c) EMCC Software Ltd 2003
* @version 1.0
*/
#include "AudioPlayerEngine.h"
#include <eikenv.h>
#include <stringloader.h>
#include <barsread.h>
#include <aknutils.h>
#include "AudioPlayer.rsg"
#include "AudioPlayer.loc"
_LIT(KToPlayFileWav, "\\Ring.wav");
_LIT(KToPlayFileMidi, "\\Fur-Elise.mid");
_LIT(KToPlayFileStream, "\\Stream.pcm");
const TInt KToneFrequency = 3000;
const TInt KToneDuration = 4000000;
const TInt KToneVolumeDenominator = 2;
/**
* C++ constructor
*/
CAudioPlayerEngine::CAudioPlayerEngine(MAudioPlayerEngineObserver& aObserver)
:iObserver(aObserver),
iState(EStopped)
{
}
/**
* Destructor.
*/
CAudioPlayerEngine::~CAudioPlayerEngine()
{
Stop();
}
/**
* Symbian OS 2 phase constructor.
* Constructs the CAudioPlayerEngine popping
* the constructed object from the CleanupStack before returning it.
*
* @param aRect The rectangle for this window
* @return The newly constructed CAudioPlayerAppView
*/
CAudioPlayerEngine* CAudioPlayerEngine::NewL(MAudioPlayerEngineObserver& aObserver)
{
CAudioPlayerEngine* self = new (ELeave) CAudioPlayerEngine(aObserver);
CleanupStack::PushL(self);
self->ConstructL();
CleanupStack::Pop(self);
return self;
}
/**
* Symbian 2nd phase constructor.
* Empty implementation
*/
void CAudioPlayerEngine::ConstructL()
{
}
/**
* MMdaAudioToneObserver derivation
* This has been called as a result of NewL() being called on the tone player
* the tone utility is now ready for playing
*
* @param aError Error code to inform us if preparation has successfully completed
*
*/
void CAudioPlayerEngine::MatoPrepareComplete(TInt aError)
{
if (aError)
{
Stop();
}
else
{
iPlayerTone->SetVolume(iPlayerTone->MaxVolume() / KToneVolumeDenominator);
iPlayerTone->Play();
}
}
/**
* MMdaAudioToneObserver derivation
* This is called when tone playing completes and informs the
* MAudioPlayerEngineObserver playing has finished.
*
* @param aError Error code to inform us if playing has successfully completed
*/
void CAudioPlayerEngine::MatoPlayComplete(TInt /*aError*/)
{
iState = EStopped;
delete iPlayerTone;
iPlayerTone = NULL;
TRAPD(err, iObserver.HandlePlayingStoppedL());
}
/**
* MMdaAudioPlayerCallback derivation
* This has been called as a result of NewFilePlayerL() being called on the file player
* the player utility is now ready for playing
*
* @param aError Status of the audio sample after initialization
* @param aDuration Duration of the audio sample
*/
void CAudioPlayerEngine::MapcInitComplete(TInt aError, const TTimeIntervalMicroSeconds& /*aDuration*/)
{
if (aError == KErrNone)
{
iPlayerFile->SetVolume(iPlayerFile->MaxVolume() / KToneVolumeDenominator);
iPlayerFile->Play();
}
else
{
Stop();
}
}
/**
* MMdaAudioPlayerCallback derivation
* This has been called as a result of playing being completed
*
* @param aError Error code to inform if playing has successfully completed
*/
void CAudioPlayerEngine::MapcPlayComplete(TInt /*aError*/)
{
iState = EStopped;
delete iPlayerFile;
iPlayerFile = NULL;
TRAPD(err, iObserver.HandlePlayingStoppedL());//播放完通知给了它的观察者VIEW
// if (aError == KErrNone)
// {
// iPlayerFile->Close(); /* Closes the current audio clip, so can allow another clip to be opened). */
//
// iPlayerFile->OpenFileL(_L("c:\data\Fur-Elise.mid")); /* 打开另一个音频文件并重复播放流程 */
//
// }
// else
//
// {
// iPlayerFile->Stop();
// }
}
/**
* MMdaAudioOutputStreamCallback derivation
* This has been called as a result of NewL() being called on the stream player
* in order to play the stream, we must 1st write the stream buffer to the client
* once this has been done, the stream will begin playing automatically
*
* @param aError Error code to indicate if open succeeded
*/
void CAudioPlayerEngine::MaoscOpenComplete(TInt aError)
{
if (aError == KErrNone)
{
TRAPD(err, iPlayerStream->WriteL(*iStreamBuffer));
}
else
{
Stop();
}
}
/**
* This has been called in response to WriteL() being called on the stream player
* or in response to Stop() being called on the stream player. in the latter case,
* aError is equal to KErrAbort.
* Otherwise, we add iStreamBuffer to the client queue, so that it will be repeated,
* when the last buffer has finished
*
* @param aError Error code to indicate if copy was successful
* @param aBuffer A reference to the descriptor that has been copied to the server
*/
void CAudioPlayerEngine::MaoscBufferCopied(TInt aError, const TDesC8& /*aBuffer*/)
{//open完调用该回掉,
if (aError == KErrNone)
{
TRAPD(err, iPlayerStream->WriteL(*iStreamBuffer))//完了后指向第一个没有写的数据
iPlayerStream->SetVolume(iPlayerStream->MaxVolume() / KToneVolumeDenominator);
}//所有声音播放完后,MaoscPlayComplete(TInt /*aError*/)被回掉
}
/**
* Callback when playback finishes
*
* @param aError Error code indicates why playback was terminated
*/
void CAudioPlayerEngine::MaoscPlayComplete(TInt /*aError*/)
{
iState = EStopped;
delete iPlayerStream;
iPlayerStream = NULL;
delete iStreamBuffer;
iStreamBuffer = NULL;
delete [] iStreamData;
iStreamData = NULL;
TRAPD(err, iObserver.HandlePlayingStoppedL());
}
//PLAYER FUNCTIONS
/**
* Constructs the tone player;
* This will lead to a call to MapcInitComplete()
*/
void CAudioPlayerEngine::PlayToneL()
{
iState = EPlaying;
iPlayerTone = CMdaAudioToneUtility::NewL(*this);
iPlayerTone->PrepareToPlayTone(KToneFrequency, TTimeIntervalMicroSeconds(KToneDuration));//以一定的频率发出声音
}
/**
* Constructs the file player;
* This will lead to a call to MapcInitComplete()
*/
void CAudioPlayerEngine::PlayWavL()
{
TFileName wavFile(KToPlayFileWav);
User::LeaveIfError(CompleteWithAppPath(wavFile));
iState = EPlaying;
iPlayerFile = CMdaAudioPlayerUtility::NewFilePlayerL(wavFile, *this);//传this是观察者
}
/**
* Constructs the file player;
* This will lead to a call to MapcInitComplete()
* Note: playing midi files can only be supported on device; it is not supported under WINS
*/
void CAudioPlayerEngine::PlayMidiL()
{
#ifndef __WINS__
TFileName midiFile(KToPlayFileMidi);
User::LeaveIfError(CompleteWithAppPath(midiFile));
iState = EPlaying;
iPlayerFile = CMdaAudioPlayerUtility::NewFilePlayerL(midiFile, *this);
#endif
}
/**
* Constructs the stream player
* This will lead to a call to MaoscOpenComplete()
*/
void CAudioPlayerEngine::PlayStreamL()
{
// open the file and load it into the buffers
RFs fs;
CleanupClosePushL(fs); // PUSH
User::LeaveIfError(fs.Connect());
RFile file;
CleanupClosePushL(file); // PUSH
TFileName streamFile(KToPlayFileStream);
User::LeaveIfError(CompleteWithAppPath(streamFile));
User::LeaveIfError(file.Open(fs, streamFile, EFileRead | EFileShareReadersOnly));
TInt fileSize = 0;
file.Size(fileSize);
iStreamData = new (ELeave) TUint8[fileSize];
iStreamBuffer = new (ELeave) TPtr8(iStreamData, fileSize, fileSize);
file.Read(*iStreamBuffer);
CleanupStack::PopAndDestroy(2); // file & fs
iState = EPlaying;
iPlayerStream = CMdaAudioOutputStream::NewL(*this);
iPlayerStream->Open(&iStreamSettings);
}
/**
* Stops whatever is playing and deletes the player
*/
void CAudioPlayerEngine::Stop()
{
if (iPlayerTone)
{
iPlayerTone->CancelPlay();
}
if (iPlayerFile)
{
iPlayerFile->Stop();
}
if (iPlayerStream)
{
iPlayerStream->Stop();
}
delete iPlayerTone;
iPlayerTone = NULL;
delete iPlayerFile;
iPlayerFile = NULL;
delete iPlayerStream;
iPlayerStream = NULL;
delete iStreamBuffer;
iStreamBuffer = NULL;
delete [] iStreamData;
iStreamData = NULL;
iState = EStopped;
}

liyong8267198
- 粉丝: 0
- 资源: 1
最新资源
- IP网络的仿真及实验.doc
- Metropolis-Hastings算法和吉布斯采样(Gibbs sampling)算法Python代码实现
- 高效排序算法:快速排序Java与Python实现详解
- 基于stm32风速风向测量仪V2.0
- 多边形框架物体检测27-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 国产文本编辑器:EverEdit用户手册 1.1.0
- 3.0(1).docx
- 多种土地使用类型图像分类数据集【已标注,约30,000张数据】
- 智慧校园数字孪生,三维可视化
- GigaDevice.GD32F4xx-DFP.2.1.0 器件安装包
- 基于 Spring Cloud 的一个分布式系统套件的整合 具备 JeeSite4 单机版的所有功能,统一身份认证,统一基础数据管理,弱化微服务开发难度
- opcclient源码OPC客户端 DA客户端源码(c#开发) C#开发,源码,可二次开发 本项目为VS2010开发,可转为VS其他版本的编辑器打开项目 已应用到多个行业的几百个应用现场,长时间运
- IMG_4525.jpg
- STM32F427+rtthread下的bootload 网口(webclient)+串口(ymodem)传输,代码无质量,谨慎使用
- FastAdmin后台框架开源且可以免费商用,一键生成CRUD, 一款基于ThinkPHP和Bootstrap的极速后台开发框架,基于Auth验证的权限管理系统,一键生成 CRUD,自动生成控制器等
- GD32F5XX系列的产品数据手册,学习手册,器件安装包
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


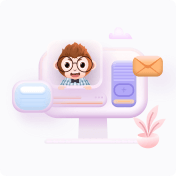